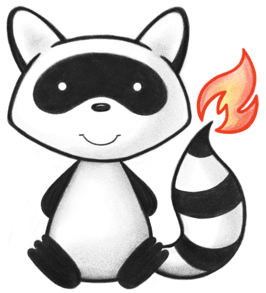
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047/** 048 * A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities. 049 */ 050@DatatypeDef(name="Signature") 051public class Signature extends Type implements ICompositeType { 052 053 /** 054 * An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document. 055 */ 056 @Child(name = "type", type = {Coding.class}, order=0, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 057 @Description(shortDefinition="Indication of the reason the entity signed the object(s)", formalDefinition="An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document." ) 058 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/signature-type") 059 protected List<Coding> type; 060 061 /** 062 * When the digital signature was signed. 063 */ 064 @Child(name = "when", type = {InstantType.class}, order=1, min=1, max=1, modifier=false, summary=true) 065 @Description(shortDefinition="When the signature was created", formalDefinition="When the digital signature was signed." ) 066 protected InstantType when; 067 068 /** 069 * A reference to an application-usable description of the identity that signed (e.g. the signature used their private key). 070 */ 071 @Child(name = "who", type = {UriType.class, Practitioner.class, RelatedPerson.class, Patient.class, Device.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=true) 072 @Description(shortDefinition="Who signed", formalDefinition="A reference to an application-usable description of the identity that signed (e.g. the signature used their private key)." ) 073 protected Type who; 074 075 /** 076 * A reference to an application-usable description of the identity that is represented by the signature. 077 */ 078 @Child(name = "onBehalfOf", type = {UriType.class, Practitioner.class, RelatedPerson.class, Patient.class, Device.class, Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 079 @Description(shortDefinition="The party represented", formalDefinition="A reference to an application-usable description of the identity that is represented by the signature." ) 080 protected Type onBehalfOf; 081 082 /** 083 * A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature, etc. 084 */ 085 @Child(name = "contentType", type = {CodeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 086 @Description(shortDefinition="The technical format of the signature", formalDefinition="A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature, etc." ) 087 protected CodeType contentType; 088 089 /** 090 * The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty. 091 */ 092 @Child(name = "blob", type = {Base64BinaryType.class}, order=5, min=0, max=1, modifier=false, summary=false) 093 @Description(shortDefinition="The actual signature content (XML DigSig. JWT, picture, etc.)", formalDefinition="The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty." ) 094 protected Base64BinaryType blob; 095 096 private static final long serialVersionUID = 1133697310L; 097 098 /** 099 * Constructor 100 */ 101 public Signature() { 102 super(); 103 } 104 105 /** 106 * Constructor 107 */ 108 public Signature(InstantType when, Type who) { 109 super(); 110 this.when = when; 111 this.who = who; 112 } 113 114 /** 115 * @return {@link #type} (An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.) 116 */ 117 public List<Coding> getType() { 118 if (this.type == null) 119 this.type = new ArrayList<Coding>(); 120 return this.type; 121 } 122 123 /** 124 * @return Returns a reference to <code>this</code> for easy method chaining 125 */ 126 public Signature setType(List<Coding> theType) { 127 this.type = theType; 128 return this; 129 } 130 131 public boolean hasType() { 132 if (this.type == null) 133 return false; 134 for (Coding item : this.type) 135 if (!item.isEmpty()) 136 return true; 137 return false; 138 } 139 140 public Coding addType() { //3 141 Coding t = new Coding(); 142 if (this.type == null) 143 this.type = new ArrayList<Coding>(); 144 this.type.add(t); 145 return t; 146 } 147 148 public Signature addType(Coding t) { //3 149 if (t == null) 150 return this; 151 if (this.type == null) 152 this.type = new ArrayList<Coding>(); 153 this.type.add(t); 154 return this; 155 } 156 157 /** 158 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 159 */ 160 public Coding getTypeFirstRep() { 161 if (getType().isEmpty()) { 162 addType(); 163 } 164 return getType().get(0); 165 } 166 167 /** 168 * @return {@link #when} (When the digital signature was signed.). This is the underlying object with id, value and extensions. The accessor "getWhen" gives direct access to the value 169 */ 170 public InstantType getWhenElement() { 171 if (this.when == null) 172 if (Configuration.errorOnAutoCreate()) 173 throw new Error("Attempt to auto-create Signature.when"); 174 else if (Configuration.doAutoCreate()) 175 this.when = new InstantType(); // bb 176 return this.when; 177 } 178 179 public boolean hasWhenElement() { 180 return this.when != null && !this.when.isEmpty(); 181 } 182 183 public boolean hasWhen() { 184 return this.when != null && !this.when.isEmpty(); 185 } 186 187 /** 188 * @param value {@link #when} (When the digital signature was signed.). This is the underlying object with id, value and extensions. The accessor "getWhen" gives direct access to the value 189 */ 190 public Signature setWhenElement(InstantType value) { 191 this.when = value; 192 return this; 193 } 194 195 /** 196 * @return When the digital signature was signed. 197 */ 198 public Date getWhen() { 199 return this.when == null ? null : this.when.getValue(); 200 } 201 202 /** 203 * @param value When the digital signature was signed. 204 */ 205 public Signature setWhen(Date value) { 206 if (this.when == null) 207 this.when = new InstantType(); 208 this.when.setValue(value); 209 return this; 210 } 211 212 /** 213 * @return {@link #who} (A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).) 214 */ 215 public Type getWho() { 216 return this.who; 217 } 218 219 /** 220 * @return {@link #who} (A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).) 221 */ 222 public UriType getWhoUriType() throws FHIRException { 223 if (this.who == null) 224 return null; 225 if (!(this.who instanceof UriType)) 226 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.who.getClass().getName()+" was encountered"); 227 return (UriType) this.who; 228 } 229 230 public boolean hasWhoUriType() { 231 return this != null && this.who instanceof UriType; 232 } 233 234 /** 235 * @return {@link #who} (A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).) 236 */ 237 public Reference getWhoReference() throws FHIRException { 238 if (this.who == null) 239 return null; 240 if (!(this.who instanceof Reference)) 241 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.who.getClass().getName()+" was encountered"); 242 return (Reference) this.who; 243 } 244 245 public boolean hasWhoReference() { 246 return this != null && this.who instanceof Reference; 247 } 248 249 public boolean hasWho() { 250 return this.who != null && !this.who.isEmpty(); 251 } 252 253 /** 254 * @param value {@link #who} (A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).) 255 */ 256 public Signature setWho(Type value) throws FHIRFormatError { 257 if (value != null && !(value instanceof UriType || value instanceof Reference)) 258 throw new FHIRFormatError("Not the right type for Signature.who[x]: "+value.fhirType()); 259 this.who = value; 260 return this; 261 } 262 263 /** 264 * @return {@link #onBehalfOf} (A reference to an application-usable description of the identity that is represented by the signature.) 265 */ 266 public Type getOnBehalfOf() { 267 return this.onBehalfOf; 268 } 269 270 /** 271 * @return {@link #onBehalfOf} (A reference to an application-usable description of the identity that is represented by the signature.) 272 */ 273 public UriType getOnBehalfOfUriType() throws FHIRException { 274 if (this.onBehalfOf == null) 275 return null; 276 if (!(this.onBehalfOf instanceof UriType)) 277 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.onBehalfOf.getClass().getName()+" was encountered"); 278 return (UriType) this.onBehalfOf; 279 } 280 281 public boolean hasOnBehalfOfUriType() { 282 return this != null && this.onBehalfOf instanceof UriType; 283 } 284 285 /** 286 * @return {@link #onBehalfOf} (A reference to an application-usable description of the identity that is represented by the signature.) 287 */ 288 public Reference getOnBehalfOfReference() throws FHIRException { 289 if (this.onBehalfOf == null) 290 return null; 291 if (!(this.onBehalfOf instanceof Reference)) 292 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.onBehalfOf.getClass().getName()+" was encountered"); 293 return (Reference) this.onBehalfOf; 294 } 295 296 public boolean hasOnBehalfOfReference() { 297 return this != null && this.onBehalfOf instanceof Reference; 298 } 299 300 public boolean hasOnBehalfOf() { 301 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 302 } 303 304 /** 305 * @param value {@link #onBehalfOf} (A reference to an application-usable description of the identity that is represented by the signature.) 306 */ 307 public Signature setOnBehalfOf(Type value) throws FHIRFormatError { 308 if (value != null && !(value instanceof UriType || value instanceof Reference)) 309 throw new FHIRFormatError("Not the right type for Signature.onBehalfOf[x]: "+value.fhirType()); 310 this.onBehalfOf = value; 311 return this; 312 } 313 314 /** 315 * @return {@link #contentType} (A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature, etc.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 316 */ 317 public CodeType getContentTypeElement() { 318 if (this.contentType == null) 319 if (Configuration.errorOnAutoCreate()) 320 throw new Error("Attempt to auto-create Signature.contentType"); 321 else if (Configuration.doAutoCreate()) 322 this.contentType = new CodeType(); // bb 323 return this.contentType; 324 } 325 326 public boolean hasContentTypeElement() { 327 return this.contentType != null && !this.contentType.isEmpty(); 328 } 329 330 public boolean hasContentType() { 331 return this.contentType != null && !this.contentType.isEmpty(); 332 } 333 334 /** 335 * @param value {@link #contentType} (A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature, etc.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 336 */ 337 public Signature setContentTypeElement(CodeType value) { 338 this.contentType = value; 339 return this; 340 } 341 342 /** 343 * @return A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature, etc. 344 */ 345 public String getContentType() { 346 return this.contentType == null ? null : this.contentType.getValue(); 347 } 348 349 /** 350 * @param value A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature, etc. 351 */ 352 public Signature setContentType(String value) { 353 if (Utilities.noString(value)) 354 this.contentType = null; 355 else { 356 if (this.contentType == null) 357 this.contentType = new CodeType(); 358 this.contentType.setValue(value); 359 } 360 return this; 361 } 362 363 /** 364 * @return {@link #blob} (The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.). This is the underlying object with id, value and extensions. The accessor "getBlob" gives direct access to the value 365 */ 366 public Base64BinaryType getBlobElement() { 367 if (this.blob == null) 368 if (Configuration.errorOnAutoCreate()) 369 throw new Error("Attempt to auto-create Signature.blob"); 370 else if (Configuration.doAutoCreate()) 371 this.blob = new Base64BinaryType(); // bb 372 return this.blob; 373 } 374 375 public boolean hasBlobElement() { 376 return this.blob != null && !this.blob.isEmpty(); 377 } 378 379 public boolean hasBlob() { 380 return this.blob != null && !this.blob.isEmpty(); 381 } 382 383 /** 384 * @param value {@link #blob} (The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.). This is the underlying object with id, value and extensions. The accessor "getBlob" gives direct access to the value 385 */ 386 public Signature setBlobElement(Base64BinaryType value) { 387 this.blob = value; 388 return this; 389 } 390 391 /** 392 * @return The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty. 393 */ 394 public byte[] getBlob() { 395 return this.blob == null ? null : this.blob.getValue(); 396 } 397 398 /** 399 * @param value The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty. 400 */ 401 public Signature setBlob(byte[] value) { 402 if (value == null) 403 this.blob = null; 404 else { 405 if (this.blob == null) 406 this.blob = new Base64BinaryType(); 407 this.blob.setValue(value); 408 } 409 return this; 410 } 411 412 protected void listChildren(List<Property> children) { 413 super.listChildren(children); 414 children.add(new Property("type", "Coding", "An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.", 0, java.lang.Integer.MAX_VALUE, type)); 415 children.add(new Property("when", "instant", "When the digital signature was signed.", 0, 1, when)); 416 children.add(new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).", 0, 1, who)); 417 children.add(new Property("onBehalfOf[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that is represented by the signature.", 0, 1, onBehalfOf)); 418 children.add(new Property("contentType", "code", "A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature, etc.", 0, 1, contentType)); 419 children.add(new Property("blob", "base64Binary", "The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.", 0, 1, blob)); 420 } 421 422 @Override 423 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 424 switch (_hash) { 425 case 3575610: /*type*/ return new Property("type", "Coding", "An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.", 0, java.lang.Integer.MAX_VALUE, type); 426 case 3648314: /*when*/ return new Property("when", "instant", "When the digital signature was signed.", 0, 1, when); 427 case -788654078: /*who[x]*/ return new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).", 0, 1, who); 428 case 117694: /*who*/ return new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).", 0, 1, who); 429 case -788660018: /*whoUri*/ return new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).", 0, 1, who); 430 case 1017243949: /*whoReference*/ return new Property("who[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).", 0, 1, who); 431 case 418120340: /*onBehalfOf[x]*/ return new Property("onBehalfOf[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that is represented by the signature.", 0, 1, onBehalfOf); 432 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that is represented by the signature.", 0, 1, onBehalfOf); 433 case 418114400: /*onBehalfOfUri*/ return new Property("onBehalfOf[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that is represented by the signature.", 0, 1, onBehalfOf); 434 case -1136255425: /*onBehalfOfReference*/ return new Property("onBehalfOf[x]", "uri|Reference(Practitioner|RelatedPerson|Patient|Device|Organization)", "A reference to an application-usable description of the identity that is represented by the signature.", 0, 1, onBehalfOf); 435 case -389131437: /*contentType*/ return new Property("contentType", "code", "A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature, etc.", 0, 1, contentType); 436 case 3026845: /*blob*/ return new Property("blob", "base64Binary", "The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.", 0, 1, blob); 437 default: return super.getNamedProperty(_hash, _name, _checkValid); 438 } 439 440 } 441 442 @Override 443 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 444 switch (hash) { 445 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // Coding 446 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // InstantType 447 case 117694: /*who*/ return this.who == null ? new Base[0] : new Base[] {this.who}; // Type 448 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Type 449 case -389131437: /*contentType*/ return this.contentType == null ? new Base[0] : new Base[] {this.contentType}; // CodeType 450 case 3026845: /*blob*/ return this.blob == null ? new Base[0] : new Base[] {this.blob}; // Base64BinaryType 451 default: return super.getProperty(hash, name, checkValid); 452 } 453 454 } 455 456 @Override 457 public Base setProperty(int hash, String name, Base value) throws FHIRException { 458 switch (hash) { 459 case 3575610: // type 460 this.getType().add(castToCoding(value)); // Coding 461 return value; 462 case 3648314: // when 463 this.when = castToInstant(value); // InstantType 464 return value; 465 case 117694: // who 466 this.who = castToType(value); // Type 467 return value; 468 case -14402964: // onBehalfOf 469 this.onBehalfOf = castToType(value); // Type 470 return value; 471 case -389131437: // contentType 472 this.contentType = castToCode(value); // CodeType 473 return value; 474 case 3026845: // blob 475 this.blob = castToBase64Binary(value); // Base64BinaryType 476 return value; 477 default: return super.setProperty(hash, name, value); 478 } 479 480 } 481 482 @Override 483 public Base setProperty(String name, Base value) throws FHIRException { 484 if (name.equals("type")) { 485 this.getType().add(castToCoding(value)); 486 } else if (name.equals("when")) { 487 this.when = castToInstant(value); // InstantType 488 } else if (name.equals("who[x]")) { 489 this.who = castToType(value); // Type 490 } else if (name.equals("onBehalfOf[x]")) { 491 this.onBehalfOf = castToType(value); // Type 492 } else if (name.equals("contentType")) { 493 this.contentType = castToCode(value); // CodeType 494 } else if (name.equals("blob")) { 495 this.blob = castToBase64Binary(value); // Base64BinaryType 496 } else 497 return super.setProperty(name, value); 498 return value; 499 } 500 501 @Override 502 public Base makeProperty(int hash, String name) throws FHIRException { 503 switch (hash) { 504 case 3575610: return addType(); 505 case 3648314: return getWhenElement(); 506 case -788654078: return getWho(); 507 case 117694: return getWho(); 508 case 418120340: return getOnBehalfOf(); 509 case -14402964: return getOnBehalfOf(); 510 case -389131437: return getContentTypeElement(); 511 case 3026845: return getBlobElement(); 512 default: return super.makeProperty(hash, name); 513 } 514 515 } 516 517 @Override 518 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 519 switch (hash) { 520 case 3575610: /*type*/ return new String[] {"Coding"}; 521 case 3648314: /*when*/ return new String[] {"instant"}; 522 case 117694: /*who*/ return new String[] {"uri", "Reference"}; 523 case -14402964: /*onBehalfOf*/ return new String[] {"uri", "Reference"}; 524 case -389131437: /*contentType*/ return new String[] {"code"}; 525 case 3026845: /*blob*/ return new String[] {"base64Binary"}; 526 default: return super.getTypesForProperty(hash, name); 527 } 528 529 } 530 531 @Override 532 public Base addChild(String name) throws FHIRException { 533 if (name.equals("type")) { 534 return addType(); 535 } 536 else if (name.equals("when")) { 537 throw new FHIRException("Cannot call addChild on a singleton property Signature.when"); 538 } 539 else if (name.equals("whoUri")) { 540 this.who = new UriType(); 541 return this.who; 542 } 543 else if (name.equals("whoReference")) { 544 this.who = new Reference(); 545 return this.who; 546 } 547 else if (name.equals("onBehalfOfUri")) { 548 this.onBehalfOf = new UriType(); 549 return this.onBehalfOf; 550 } 551 else if (name.equals("onBehalfOfReference")) { 552 this.onBehalfOf = new Reference(); 553 return this.onBehalfOf; 554 } 555 else if (name.equals("contentType")) { 556 throw new FHIRException("Cannot call addChild on a singleton property Signature.contentType"); 557 } 558 else if (name.equals("blob")) { 559 throw new FHIRException("Cannot call addChild on a singleton property Signature.blob"); 560 } 561 else 562 return super.addChild(name); 563 } 564 565 public String fhirType() { 566 return "Signature"; 567 568 } 569 570 public Signature copy() { 571 Signature dst = new Signature(); 572 copyValues(dst); 573 if (type != null) { 574 dst.type = new ArrayList<Coding>(); 575 for (Coding i : type) 576 dst.type.add(i.copy()); 577 }; 578 dst.when = when == null ? null : when.copy(); 579 dst.who = who == null ? null : who.copy(); 580 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 581 dst.contentType = contentType == null ? null : contentType.copy(); 582 dst.blob = blob == null ? null : blob.copy(); 583 return dst; 584 } 585 586 protected Signature typedCopy() { 587 return copy(); 588 } 589 590 @Override 591 public boolean equalsDeep(Base other_) { 592 if (!super.equalsDeep(other_)) 593 return false; 594 if (!(other_ instanceof Signature)) 595 return false; 596 Signature o = (Signature) other_; 597 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true) && compareDeep(who, o.who, true) 598 && compareDeep(onBehalfOf, o.onBehalfOf, true) && compareDeep(contentType, o.contentType, true) 599 && compareDeep(blob, o.blob, true); 600 } 601 602 @Override 603 public boolean equalsShallow(Base other_) { 604 if (!super.equalsShallow(other_)) 605 return false; 606 if (!(other_ instanceof Signature)) 607 return false; 608 Signature o = (Signature) other_; 609 return compareValues(when, o.when, true) && compareValues(contentType, o.contentType, true) && compareValues(blob, o.blob, true) 610 ; 611 } 612 613 public boolean isEmpty() { 614 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, when, who, onBehalfOf 615 , contentType, blob); 616 } 617 618 619}