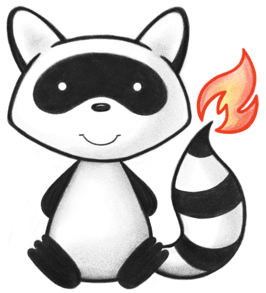
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046/** 047 * A slot of time on a schedule that may be available for booking appointments. 048 */ 049@ResourceDef(name="Slot", profile="http://hl7.org/fhir/Profile/Slot") 050public class Slot extends DomainResource { 051 052 public enum SlotStatus { 053 /** 054 * Indicates that the time interval is busy because one or more events have been scheduled for that interval. 055 */ 056 BUSY, 057 /** 058 * Indicates that the time interval is free for scheduling. 059 */ 060 FREE, 061 /** 062 * Indicates that the time interval is busy and that the interval can not be scheduled. 063 */ 064 BUSYUNAVAILABLE, 065 /** 066 * Indicates that the time interval is busy because one or more events have been tentatively scheduled for that interval. 067 */ 068 BUSYTENTATIVE, 069 /** 070 * This instance should not have been part of this patient's medical record. 071 */ 072 ENTEREDINERROR, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static SlotStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("busy".equals(codeString)) 081 return BUSY; 082 if ("free".equals(codeString)) 083 return FREE; 084 if ("busy-unavailable".equals(codeString)) 085 return BUSYUNAVAILABLE; 086 if ("busy-tentative".equals(codeString)) 087 return BUSYTENTATIVE; 088 if ("entered-in-error".equals(codeString)) 089 return ENTEREDINERROR; 090 if (Configuration.isAcceptInvalidEnums()) 091 return null; 092 else 093 throw new FHIRException("Unknown SlotStatus code '"+codeString+"'"); 094 } 095 public String toCode() { 096 switch (this) { 097 case BUSY: return "busy"; 098 case FREE: return "free"; 099 case BUSYUNAVAILABLE: return "busy-unavailable"; 100 case BUSYTENTATIVE: return "busy-tentative"; 101 case ENTEREDINERROR: return "entered-in-error"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 switch (this) { 108 case BUSY: return "http://hl7.org/fhir/slotstatus"; 109 case FREE: return "http://hl7.org/fhir/slotstatus"; 110 case BUSYUNAVAILABLE: return "http://hl7.org/fhir/slotstatus"; 111 case BUSYTENTATIVE: return "http://hl7.org/fhir/slotstatus"; 112 case ENTEREDINERROR: return "http://hl7.org/fhir/slotstatus"; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 public String getDefinition() { 118 switch (this) { 119 case BUSY: return "Indicates that the time interval is busy because one or more events have been scheduled for that interval."; 120 case FREE: return "Indicates that the time interval is free for scheduling."; 121 case BUSYUNAVAILABLE: return "Indicates that the time interval is busy and that the interval can not be scheduled."; 122 case BUSYTENTATIVE: return "Indicates that the time interval is busy because one or more events have been tentatively scheduled for that interval."; 123 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 public String getDisplay() { 129 switch (this) { 130 case BUSY: return "Busy"; 131 case FREE: return "Free"; 132 case BUSYUNAVAILABLE: return "Busy (Unavailable)"; 133 case BUSYTENTATIVE: return "Busy (Tentative)"; 134 case ENTEREDINERROR: return "Entered in error"; 135 case NULL: return null; 136 default: return "?"; 137 } 138 } 139 } 140 141 public static class SlotStatusEnumFactory implements EnumFactory<SlotStatus> { 142 public SlotStatus fromCode(String codeString) throws IllegalArgumentException { 143 if (codeString == null || "".equals(codeString)) 144 if (codeString == null || "".equals(codeString)) 145 return null; 146 if ("busy".equals(codeString)) 147 return SlotStatus.BUSY; 148 if ("free".equals(codeString)) 149 return SlotStatus.FREE; 150 if ("busy-unavailable".equals(codeString)) 151 return SlotStatus.BUSYUNAVAILABLE; 152 if ("busy-tentative".equals(codeString)) 153 return SlotStatus.BUSYTENTATIVE; 154 if ("entered-in-error".equals(codeString)) 155 return SlotStatus.ENTEREDINERROR; 156 throw new IllegalArgumentException("Unknown SlotStatus code '"+codeString+"'"); 157 } 158 public Enumeration<SlotStatus> fromType(PrimitiveType<?> code) throws FHIRException { 159 if (code == null) 160 return null; 161 if (code.isEmpty()) 162 return new Enumeration<SlotStatus>(this); 163 String codeString = code.asStringValue(); 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("busy".equals(codeString)) 167 return new Enumeration<SlotStatus>(this, SlotStatus.BUSY); 168 if ("free".equals(codeString)) 169 return new Enumeration<SlotStatus>(this, SlotStatus.FREE); 170 if ("busy-unavailable".equals(codeString)) 171 return new Enumeration<SlotStatus>(this, SlotStatus.BUSYUNAVAILABLE); 172 if ("busy-tentative".equals(codeString)) 173 return new Enumeration<SlotStatus>(this, SlotStatus.BUSYTENTATIVE); 174 if ("entered-in-error".equals(codeString)) 175 return new Enumeration<SlotStatus>(this, SlotStatus.ENTEREDINERROR); 176 throw new FHIRException("Unknown SlotStatus code '"+codeString+"'"); 177 } 178 public String toCode(SlotStatus code) { 179 if (code == SlotStatus.NULL) 180 return null; 181 if (code == SlotStatus.BUSY) 182 return "busy"; 183 if (code == SlotStatus.FREE) 184 return "free"; 185 if (code == SlotStatus.BUSYUNAVAILABLE) 186 return "busy-unavailable"; 187 if (code == SlotStatus.BUSYTENTATIVE) 188 return "busy-tentative"; 189 if (code == SlotStatus.ENTEREDINERROR) 190 return "entered-in-error"; 191 return "?"; 192 } 193 public String toSystem(SlotStatus code) { 194 return code.getSystem(); 195 } 196 } 197 198 /** 199 * External Ids for this item. 200 */ 201 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 202 @Description(shortDefinition="External Ids for this item", formalDefinition="External Ids for this item." ) 203 protected List<Identifier> identifier; 204 205 /** 206 * A broad categorisation of the service that is to be performed during this appointment. 207 */ 208 @Child(name = "serviceCategory", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 209 @Description(shortDefinition="A broad categorisation of the service that is to be performed during this appointment", formalDefinition="A broad categorisation of the service that is to be performed during this appointment." ) 210 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-category") 211 protected CodeableConcept serviceCategory; 212 213 /** 214 * The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource. 215 */ 216 @Child(name = "serviceType", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 217 @Description(shortDefinition="The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource", formalDefinition="The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource." ) 218 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-type") 219 protected List<CodeableConcept> serviceType; 220 221 /** 222 * The specialty of a practitioner that would be required to perform the service requested in this appointment. 223 */ 224 @Child(name = "specialty", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 225 @Description(shortDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment", formalDefinition="The specialty of a practitioner that would be required to perform the service requested in this appointment." ) 226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 227 protected List<CodeableConcept> specialty; 228 229 /** 230 * The style of appointment or patient that may be booked in the slot (not service type). 231 */ 232 @Child(name = "appointmentType", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 233 @Description(shortDefinition="The style of appointment or patient that may be booked in the slot (not service type)", formalDefinition="The style of appointment or patient that may be booked in the slot (not service type)." ) 234 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v2-0276") 235 protected CodeableConcept appointmentType; 236 237 /** 238 * The schedule resource that this slot defines an interval of status information. 239 */ 240 @Child(name = "schedule", type = {Schedule.class}, order=5, min=1, max=1, modifier=false, summary=true) 241 @Description(shortDefinition="The schedule resource that this slot defines an interval of status information", formalDefinition="The schedule resource that this slot defines an interval of status information." ) 242 protected Reference schedule; 243 244 /** 245 * The actual object that is the target of the reference (The schedule resource that this slot defines an interval of status information.) 246 */ 247 protected Schedule scheduleTarget; 248 249 /** 250 * busy | free | busy-unavailable | busy-tentative | entered-in-error. 251 */ 252 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=false, summary=true) 253 @Description(shortDefinition="busy | free | busy-unavailable | busy-tentative | entered-in-error", formalDefinition="busy | free | busy-unavailable | busy-tentative | entered-in-error." ) 254 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/slotstatus") 255 protected Enumeration<SlotStatus> status; 256 257 /** 258 * Date/Time that the slot is to begin. 259 */ 260 @Child(name = "start", type = {InstantType.class}, order=7, min=1, max=1, modifier=false, summary=true) 261 @Description(shortDefinition="Date/Time that the slot is to begin", formalDefinition="Date/Time that the slot is to begin." ) 262 protected InstantType start; 263 264 /** 265 * Date/Time that the slot is to conclude. 266 */ 267 @Child(name = "end", type = {InstantType.class}, order=8, min=1, max=1, modifier=false, summary=true) 268 @Description(shortDefinition="Date/Time that the slot is to conclude", formalDefinition="Date/Time that the slot is to conclude." ) 269 protected InstantType end; 270 271 /** 272 * This slot has already been overbooked, appointments are unlikely to be accepted for this time. 273 */ 274 @Child(name = "overbooked", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=false) 275 @Description(shortDefinition="This slot has already been overbooked, appointments are unlikely to be accepted for this time", formalDefinition="This slot has already been overbooked, appointments are unlikely to be accepted for this time." ) 276 protected BooleanType overbooked; 277 278 /** 279 * Comments on the slot to describe any extended information. Such as custom constraints on the slot. 280 */ 281 @Child(name = "comment", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 282 @Description(shortDefinition="Comments on the slot to describe any extended information. Such as custom constraints on the slot", formalDefinition="Comments on the slot to describe any extended information. Such as custom constraints on the slot." ) 283 protected StringType comment; 284 285 private static final long serialVersionUID = 2085594970L; 286 287 /** 288 * Constructor 289 */ 290 public Slot() { 291 super(); 292 } 293 294 /** 295 * Constructor 296 */ 297 public Slot(Reference schedule, Enumeration<SlotStatus> status, InstantType start, InstantType end) { 298 super(); 299 this.schedule = schedule; 300 this.status = status; 301 this.start = start; 302 this.end = end; 303 } 304 305 /** 306 * @return {@link #identifier} (External Ids for this item.) 307 */ 308 public List<Identifier> getIdentifier() { 309 if (this.identifier == null) 310 this.identifier = new ArrayList<Identifier>(); 311 return this.identifier; 312 } 313 314 /** 315 * @return Returns a reference to <code>this</code> for easy method chaining 316 */ 317 public Slot setIdentifier(List<Identifier> theIdentifier) { 318 this.identifier = theIdentifier; 319 return this; 320 } 321 322 public boolean hasIdentifier() { 323 if (this.identifier == null) 324 return false; 325 for (Identifier item : this.identifier) 326 if (!item.isEmpty()) 327 return true; 328 return false; 329 } 330 331 public Identifier addIdentifier() { //3 332 Identifier t = new Identifier(); 333 if (this.identifier == null) 334 this.identifier = new ArrayList<Identifier>(); 335 this.identifier.add(t); 336 return t; 337 } 338 339 public Slot addIdentifier(Identifier t) { //3 340 if (t == null) 341 return this; 342 if (this.identifier == null) 343 this.identifier = new ArrayList<Identifier>(); 344 this.identifier.add(t); 345 return this; 346 } 347 348 /** 349 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 350 */ 351 public Identifier getIdentifierFirstRep() { 352 if (getIdentifier().isEmpty()) { 353 addIdentifier(); 354 } 355 return getIdentifier().get(0); 356 } 357 358 /** 359 * @return {@link #serviceCategory} (A broad categorisation of the service that is to be performed during this appointment.) 360 */ 361 public CodeableConcept getServiceCategory() { 362 if (this.serviceCategory == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create Slot.serviceCategory"); 365 else if (Configuration.doAutoCreate()) 366 this.serviceCategory = new CodeableConcept(); // cc 367 return this.serviceCategory; 368 } 369 370 public boolean hasServiceCategory() { 371 return this.serviceCategory != null && !this.serviceCategory.isEmpty(); 372 } 373 374 /** 375 * @param value {@link #serviceCategory} (A broad categorisation of the service that is to be performed during this appointment.) 376 */ 377 public Slot setServiceCategory(CodeableConcept value) { 378 this.serviceCategory = value; 379 return this; 380 } 381 382 /** 383 * @return {@link #serviceType} (The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource.) 384 */ 385 public List<CodeableConcept> getServiceType() { 386 if (this.serviceType == null) 387 this.serviceType = new ArrayList<CodeableConcept>(); 388 return this.serviceType; 389 } 390 391 /** 392 * @return Returns a reference to <code>this</code> for easy method chaining 393 */ 394 public Slot setServiceType(List<CodeableConcept> theServiceType) { 395 this.serviceType = theServiceType; 396 return this; 397 } 398 399 public boolean hasServiceType() { 400 if (this.serviceType == null) 401 return false; 402 for (CodeableConcept item : this.serviceType) 403 if (!item.isEmpty()) 404 return true; 405 return false; 406 } 407 408 public CodeableConcept addServiceType() { //3 409 CodeableConcept t = new CodeableConcept(); 410 if (this.serviceType == null) 411 this.serviceType = new ArrayList<CodeableConcept>(); 412 this.serviceType.add(t); 413 return t; 414 } 415 416 public Slot addServiceType(CodeableConcept t) { //3 417 if (t == null) 418 return this; 419 if (this.serviceType == null) 420 this.serviceType = new ArrayList<CodeableConcept>(); 421 this.serviceType.add(t); 422 return this; 423 } 424 425 /** 426 * @return The first repetition of repeating field {@link #serviceType}, creating it if it does not already exist 427 */ 428 public CodeableConcept getServiceTypeFirstRep() { 429 if (getServiceType().isEmpty()) { 430 addServiceType(); 431 } 432 return getServiceType().get(0); 433 } 434 435 /** 436 * @return {@link #specialty} (The specialty of a practitioner that would be required to perform the service requested in this appointment.) 437 */ 438 public List<CodeableConcept> getSpecialty() { 439 if (this.specialty == null) 440 this.specialty = new ArrayList<CodeableConcept>(); 441 return this.specialty; 442 } 443 444 /** 445 * @return Returns a reference to <code>this</code> for easy method chaining 446 */ 447 public Slot setSpecialty(List<CodeableConcept> theSpecialty) { 448 this.specialty = theSpecialty; 449 return this; 450 } 451 452 public boolean hasSpecialty() { 453 if (this.specialty == null) 454 return false; 455 for (CodeableConcept item : this.specialty) 456 if (!item.isEmpty()) 457 return true; 458 return false; 459 } 460 461 public CodeableConcept addSpecialty() { //3 462 CodeableConcept t = new CodeableConcept(); 463 if (this.specialty == null) 464 this.specialty = new ArrayList<CodeableConcept>(); 465 this.specialty.add(t); 466 return t; 467 } 468 469 public Slot addSpecialty(CodeableConcept t) { //3 470 if (t == null) 471 return this; 472 if (this.specialty == null) 473 this.specialty = new ArrayList<CodeableConcept>(); 474 this.specialty.add(t); 475 return this; 476 } 477 478 /** 479 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist 480 */ 481 public CodeableConcept getSpecialtyFirstRep() { 482 if (getSpecialty().isEmpty()) { 483 addSpecialty(); 484 } 485 return getSpecialty().get(0); 486 } 487 488 /** 489 * @return {@link #appointmentType} (The style of appointment or patient that may be booked in the slot (not service type).) 490 */ 491 public CodeableConcept getAppointmentType() { 492 if (this.appointmentType == null) 493 if (Configuration.errorOnAutoCreate()) 494 throw new Error("Attempt to auto-create Slot.appointmentType"); 495 else if (Configuration.doAutoCreate()) 496 this.appointmentType = new CodeableConcept(); // cc 497 return this.appointmentType; 498 } 499 500 public boolean hasAppointmentType() { 501 return this.appointmentType != null && !this.appointmentType.isEmpty(); 502 } 503 504 /** 505 * @param value {@link #appointmentType} (The style of appointment or patient that may be booked in the slot (not service type).) 506 */ 507 public Slot setAppointmentType(CodeableConcept value) { 508 this.appointmentType = value; 509 return this; 510 } 511 512 /** 513 * @return {@link #schedule} (The schedule resource that this slot defines an interval of status information.) 514 */ 515 public Reference getSchedule() { 516 if (this.schedule == null) 517 if (Configuration.errorOnAutoCreate()) 518 throw new Error("Attempt to auto-create Slot.schedule"); 519 else if (Configuration.doAutoCreate()) 520 this.schedule = new Reference(); // cc 521 return this.schedule; 522 } 523 524 public boolean hasSchedule() { 525 return this.schedule != null && !this.schedule.isEmpty(); 526 } 527 528 /** 529 * @param value {@link #schedule} (The schedule resource that this slot defines an interval of status information.) 530 */ 531 public Slot setSchedule(Reference value) { 532 this.schedule = value; 533 return this; 534 } 535 536 /** 537 * @return {@link #schedule} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The schedule resource that this slot defines an interval of status information.) 538 */ 539 public Schedule getScheduleTarget() { 540 if (this.scheduleTarget == null) 541 if (Configuration.errorOnAutoCreate()) 542 throw new Error("Attempt to auto-create Slot.schedule"); 543 else if (Configuration.doAutoCreate()) 544 this.scheduleTarget = new Schedule(); // aa 545 return this.scheduleTarget; 546 } 547 548 /** 549 * @param value {@link #schedule} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The schedule resource that this slot defines an interval of status information.) 550 */ 551 public Slot setScheduleTarget(Schedule value) { 552 this.scheduleTarget = value; 553 return this; 554 } 555 556 /** 557 * @return {@link #status} (busy | free | busy-unavailable | busy-tentative | entered-in-error.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 558 */ 559 public Enumeration<SlotStatus> getStatusElement() { 560 if (this.status == null) 561 if (Configuration.errorOnAutoCreate()) 562 throw new Error("Attempt to auto-create Slot.status"); 563 else if (Configuration.doAutoCreate()) 564 this.status = new Enumeration<SlotStatus>(new SlotStatusEnumFactory()); // bb 565 return this.status; 566 } 567 568 public boolean hasStatusElement() { 569 return this.status != null && !this.status.isEmpty(); 570 } 571 572 public boolean hasStatus() { 573 return this.status != null && !this.status.isEmpty(); 574 } 575 576 /** 577 * @param value {@link #status} (busy | free | busy-unavailable | busy-tentative | entered-in-error.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 578 */ 579 public Slot setStatusElement(Enumeration<SlotStatus> value) { 580 this.status = value; 581 return this; 582 } 583 584 /** 585 * @return busy | free | busy-unavailable | busy-tentative | entered-in-error. 586 */ 587 public SlotStatus getStatus() { 588 return this.status == null ? null : this.status.getValue(); 589 } 590 591 /** 592 * @param value busy | free | busy-unavailable | busy-tentative | entered-in-error. 593 */ 594 public Slot setStatus(SlotStatus value) { 595 if (this.status == null) 596 this.status = new Enumeration<SlotStatus>(new SlotStatusEnumFactory()); 597 this.status.setValue(value); 598 return this; 599 } 600 601 /** 602 * @return {@link #start} (Date/Time that the slot is to begin.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 603 */ 604 public InstantType getStartElement() { 605 if (this.start == null) 606 if (Configuration.errorOnAutoCreate()) 607 throw new Error("Attempt to auto-create Slot.start"); 608 else if (Configuration.doAutoCreate()) 609 this.start = new InstantType(); // bb 610 return this.start; 611 } 612 613 public boolean hasStartElement() { 614 return this.start != null && !this.start.isEmpty(); 615 } 616 617 public boolean hasStart() { 618 return this.start != null && !this.start.isEmpty(); 619 } 620 621 /** 622 * @param value {@link #start} (Date/Time that the slot is to begin.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 623 */ 624 public Slot setStartElement(InstantType value) { 625 this.start = value; 626 return this; 627 } 628 629 /** 630 * @return Date/Time that the slot is to begin. 631 */ 632 public Date getStart() { 633 return this.start == null ? null : this.start.getValue(); 634 } 635 636 /** 637 * @param value Date/Time that the slot is to begin. 638 */ 639 public Slot setStart(Date value) { 640 if (this.start == null) 641 this.start = new InstantType(); 642 this.start.setValue(value); 643 return this; 644 } 645 646 /** 647 * @return {@link #end} (Date/Time that the slot is to conclude.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 648 */ 649 public InstantType getEndElement() { 650 if (this.end == null) 651 if (Configuration.errorOnAutoCreate()) 652 throw new Error("Attempt to auto-create Slot.end"); 653 else if (Configuration.doAutoCreate()) 654 this.end = new InstantType(); // bb 655 return this.end; 656 } 657 658 public boolean hasEndElement() { 659 return this.end != null && !this.end.isEmpty(); 660 } 661 662 public boolean hasEnd() { 663 return this.end != null && !this.end.isEmpty(); 664 } 665 666 /** 667 * @param value {@link #end} (Date/Time that the slot is to conclude.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 668 */ 669 public Slot setEndElement(InstantType value) { 670 this.end = value; 671 return this; 672 } 673 674 /** 675 * @return Date/Time that the slot is to conclude. 676 */ 677 public Date getEnd() { 678 return this.end == null ? null : this.end.getValue(); 679 } 680 681 /** 682 * @param value Date/Time that the slot is to conclude. 683 */ 684 public Slot setEnd(Date value) { 685 if (this.end == null) 686 this.end = new InstantType(); 687 this.end.setValue(value); 688 return this; 689 } 690 691 /** 692 * @return {@link #overbooked} (This slot has already been overbooked, appointments are unlikely to be accepted for this time.). This is the underlying object with id, value and extensions. The accessor "getOverbooked" gives direct access to the value 693 */ 694 public BooleanType getOverbookedElement() { 695 if (this.overbooked == null) 696 if (Configuration.errorOnAutoCreate()) 697 throw new Error("Attempt to auto-create Slot.overbooked"); 698 else if (Configuration.doAutoCreate()) 699 this.overbooked = new BooleanType(); // bb 700 return this.overbooked; 701 } 702 703 public boolean hasOverbookedElement() { 704 return this.overbooked != null && !this.overbooked.isEmpty(); 705 } 706 707 public boolean hasOverbooked() { 708 return this.overbooked != null && !this.overbooked.isEmpty(); 709 } 710 711 /** 712 * @param value {@link #overbooked} (This slot has already been overbooked, appointments are unlikely to be accepted for this time.). This is the underlying object with id, value and extensions. The accessor "getOverbooked" gives direct access to the value 713 */ 714 public Slot setOverbookedElement(BooleanType value) { 715 this.overbooked = value; 716 return this; 717 } 718 719 /** 720 * @return This slot has already been overbooked, appointments are unlikely to be accepted for this time. 721 */ 722 public boolean getOverbooked() { 723 return this.overbooked == null || this.overbooked.isEmpty() ? false : this.overbooked.getValue(); 724 } 725 726 /** 727 * @param value This slot has already been overbooked, appointments are unlikely to be accepted for this time. 728 */ 729 public Slot setOverbooked(boolean value) { 730 if (this.overbooked == null) 731 this.overbooked = new BooleanType(); 732 this.overbooked.setValue(value); 733 return this; 734 } 735 736 /** 737 * @return {@link #comment} (Comments on the slot to describe any extended information. Such as custom constraints on the slot.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 738 */ 739 public StringType getCommentElement() { 740 if (this.comment == null) 741 if (Configuration.errorOnAutoCreate()) 742 throw new Error("Attempt to auto-create Slot.comment"); 743 else if (Configuration.doAutoCreate()) 744 this.comment = new StringType(); // bb 745 return this.comment; 746 } 747 748 public boolean hasCommentElement() { 749 return this.comment != null && !this.comment.isEmpty(); 750 } 751 752 public boolean hasComment() { 753 return this.comment != null && !this.comment.isEmpty(); 754 } 755 756 /** 757 * @param value {@link #comment} (Comments on the slot to describe any extended information. Such as custom constraints on the slot.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 758 */ 759 public Slot setCommentElement(StringType value) { 760 this.comment = value; 761 return this; 762 } 763 764 /** 765 * @return Comments on the slot to describe any extended information. Such as custom constraints on the slot. 766 */ 767 public String getComment() { 768 return this.comment == null ? null : this.comment.getValue(); 769 } 770 771 /** 772 * @param value Comments on the slot to describe any extended information. Such as custom constraints on the slot. 773 */ 774 public Slot setComment(String value) { 775 if (Utilities.noString(value)) 776 this.comment = null; 777 else { 778 if (this.comment == null) 779 this.comment = new StringType(); 780 this.comment.setValue(value); 781 } 782 return this; 783 } 784 785 protected void listChildren(List<Property> children) { 786 super.listChildren(children); 787 children.add(new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, identifier)); 788 children.add(new Property("serviceCategory", "CodeableConcept", "A broad categorisation of the service that is to be performed during this appointment.", 0, 1, serviceCategory)); 789 children.add(new Property("serviceType", "CodeableConcept", "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource.", 0, java.lang.Integer.MAX_VALUE, serviceType)); 790 children.add(new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty)); 791 children.add(new Property("appointmentType", "CodeableConcept", "The style of appointment or patient that may be booked in the slot (not service type).", 0, 1, appointmentType)); 792 children.add(new Property("schedule", "Reference(Schedule)", "The schedule resource that this slot defines an interval of status information.", 0, 1, schedule)); 793 children.add(new Property("status", "code", "busy | free | busy-unavailable | busy-tentative | entered-in-error.", 0, 1, status)); 794 children.add(new Property("start", "instant", "Date/Time that the slot is to begin.", 0, 1, start)); 795 children.add(new Property("end", "instant", "Date/Time that the slot is to conclude.", 0, 1, end)); 796 children.add(new Property("overbooked", "boolean", "This slot has already been overbooked, appointments are unlikely to be accepted for this time.", 0, 1, overbooked)); 797 children.add(new Property("comment", "string", "Comments on the slot to describe any extended information. Such as custom constraints on the slot.", 0, 1, comment)); 798 } 799 800 @Override 801 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 802 switch (_hash) { 803 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, identifier); 804 case 1281188563: /*serviceCategory*/ return new Property("serviceCategory", "CodeableConcept", "A broad categorisation of the service that is to be performed during this appointment.", 0, 1, serviceCategory); 805 case -1928370289: /*serviceType*/ return new Property("serviceType", "CodeableConcept", "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource.", 0, java.lang.Integer.MAX_VALUE, serviceType); 806 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 0, java.lang.Integer.MAX_VALUE, specialty); 807 case -1596426375: /*appointmentType*/ return new Property("appointmentType", "CodeableConcept", "The style of appointment or patient that may be booked in the slot (not service type).", 0, 1, appointmentType); 808 case -697920873: /*schedule*/ return new Property("schedule", "Reference(Schedule)", "The schedule resource that this slot defines an interval of status information.", 0, 1, schedule); 809 case -892481550: /*status*/ return new Property("status", "code", "busy | free | busy-unavailable | busy-tentative | entered-in-error.", 0, 1, status); 810 case 109757538: /*start*/ return new Property("start", "instant", "Date/Time that the slot is to begin.", 0, 1, start); 811 case 100571: /*end*/ return new Property("end", "instant", "Date/Time that the slot is to conclude.", 0, 1, end); 812 case 2068545308: /*overbooked*/ return new Property("overbooked", "boolean", "This slot has already been overbooked, appointments are unlikely to be accepted for this time.", 0, 1, overbooked); 813 case 950398559: /*comment*/ return new Property("comment", "string", "Comments on the slot to describe any extended information. Such as custom constraints on the slot.", 0, 1, comment); 814 default: return super.getNamedProperty(_hash, _name, _checkValid); 815 } 816 817 } 818 819 @Override 820 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 821 switch (hash) { 822 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 823 case 1281188563: /*serviceCategory*/ return this.serviceCategory == null ? new Base[0] : new Base[] {this.serviceCategory}; // CodeableConcept 824 case -1928370289: /*serviceType*/ return this.serviceType == null ? new Base[0] : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableConcept 825 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 826 case -1596426375: /*appointmentType*/ return this.appointmentType == null ? new Base[0] : new Base[] {this.appointmentType}; // CodeableConcept 827 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : new Base[] {this.schedule}; // Reference 828 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SlotStatus> 829 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // InstantType 830 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // InstantType 831 case 2068545308: /*overbooked*/ return this.overbooked == null ? new Base[0] : new Base[] {this.overbooked}; // BooleanType 832 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 833 default: return super.getProperty(hash, name, checkValid); 834 } 835 836 } 837 838 @Override 839 public Base setProperty(int hash, String name, Base value) throws FHIRException { 840 switch (hash) { 841 case -1618432855: // identifier 842 this.getIdentifier().add(castToIdentifier(value)); // Identifier 843 return value; 844 case 1281188563: // serviceCategory 845 this.serviceCategory = castToCodeableConcept(value); // CodeableConcept 846 return value; 847 case -1928370289: // serviceType 848 this.getServiceType().add(castToCodeableConcept(value)); // CodeableConcept 849 return value; 850 case -1694759682: // specialty 851 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 852 return value; 853 case -1596426375: // appointmentType 854 this.appointmentType = castToCodeableConcept(value); // CodeableConcept 855 return value; 856 case -697920873: // schedule 857 this.schedule = castToReference(value); // Reference 858 return value; 859 case -892481550: // status 860 value = new SlotStatusEnumFactory().fromType(castToCode(value)); 861 this.status = (Enumeration) value; // Enumeration<SlotStatus> 862 return value; 863 case 109757538: // start 864 this.start = castToInstant(value); // InstantType 865 return value; 866 case 100571: // end 867 this.end = castToInstant(value); // InstantType 868 return value; 869 case 2068545308: // overbooked 870 this.overbooked = castToBoolean(value); // BooleanType 871 return value; 872 case 950398559: // comment 873 this.comment = castToString(value); // StringType 874 return value; 875 default: return super.setProperty(hash, name, value); 876 } 877 878 } 879 880 @Override 881 public Base setProperty(String name, Base value) throws FHIRException { 882 if (name.equals("identifier")) { 883 this.getIdentifier().add(castToIdentifier(value)); 884 } else if (name.equals("serviceCategory")) { 885 this.serviceCategory = castToCodeableConcept(value); // CodeableConcept 886 } else if (name.equals("serviceType")) { 887 this.getServiceType().add(castToCodeableConcept(value)); 888 } else if (name.equals("specialty")) { 889 this.getSpecialty().add(castToCodeableConcept(value)); 890 } else if (name.equals("appointmentType")) { 891 this.appointmentType = castToCodeableConcept(value); // CodeableConcept 892 } else if (name.equals("schedule")) { 893 this.schedule = castToReference(value); // Reference 894 } else if (name.equals("status")) { 895 value = new SlotStatusEnumFactory().fromType(castToCode(value)); 896 this.status = (Enumeration) value; // Enumeration<SlotStatus> 897 } else if (name.equals("start")) { 898 this.start = castToInstant(value); // InstantType 899 } else if (name.equals("end")) { 900 this.end = castToInstant(value); // InstantType 901 } else if (name.equals("overbooked")) { 902 this.overbooked = castToBoolean(value); // BooleanType 903 } else if (name.equals("comment")) { 904 this.comment = castToString(value); // StringType 905 } else 906 return super.setProperty(name, value); 907 return value; 908 } 909 910 @Override 911 public Base makeProperty(int hash, String name) throws FHIRException { 912 switch (hash) { 913 case -1618432855: return addIdentifier(); 914 case 1281188563: return getServiceCategory(); 915 case -1928370289: return addServiceType(); 916 case -1694759682: return addSpecialty(); 917 case -1596426375: return getAppointmentType(); 918 case -697920873: return getSchedule(); 919 case -892481550: return getStatusElement(); 920 case 109757538: return getStartElement(); 921 case 100571: return getEndElement(); 922 case 2068545308: return getOverbookedElement(); 923 case 950398559: return getCommentElement(); 924 default: return super.makeProperty(hash, name); 925 } 926 927 } 928 929 @Override 930 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 931 switch (hash) { 932 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 933 case 1281188563: /*serviceCategory*/ return new String[] {"CodeableConcept"}; 934 case -1928370289: /*serviceType*/ return new String[] {"CodeableConcept"}; 935 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 936 case -1596426375: /*appointmentType*/ return new String[] {"CodeableConcept"}; 937 case -697920873: /*schedule*/ return new String[] {"Reference"}; 938 case -892481550: /*status*/ return new String[] {"code"}; 939 case 109757538: /*start*/ return new String[] {"instant"}; 940 case 100571: /*end*/ return new String[] {"instant"}; 941 case 2068545308: /*overbooked*/ return new String[] {"boolean"}; 942 case 950398559: /*comment*/ return new String[] {"string"}; 943 default: return super.getTypesForProperty(hash, name); 944 } 945 946 } 947 948 @Override 949 public Base addChild(String name) throws FHIRException { 950 if (name.equals("identifier")) { 951 return addIdentifier(); 952 } 953 else if (name.equals("serviceCategory")) { 954 this.serviceCategory = new CodeableConcept(); 955 return this.serviceCategory; 956 } 957 else if (name.equals("serviceType")) { 958 return addServiceType(); 959 } 960 else if (name.equals("specialty")) { 961 return addSpecialty(); 962 } 963 else if (name.equals("appointmentType")) { 964 this.appointmentType = new CodeableConcept(); 965 return this.appointmentType; 966 } 967 else if (name.equals("schedule")) { 968 this.schedule = new Reference(); 969 return this.schedule; 970 } 971 else if (name.equals("status")) { 972 throw new FHIRException("Cannot call addChild on a singleton property Slot.status"); 973 } 974 else if (name.equals("start")) { 975 throw new FHIRException("Cannot call addChild on a singleton property Slot.start"); 976 } 977 else if (name.equals("end")) { 978 throw new FHIRException("Cannot call addChild on a singleton property Slot.end"); 979 } 980 else if (name.equals("overbooked")) { 981 throw new FHIRException("Cannot call addChild on a singleton property Slot.overbooked"); 982 } 983 else if (name.equals("comment")) { 984 throw new FHIRException("Cannot call addChild on a singleton property Slot.comment"); 985 } 986 else 987 return super.addChild(name); 988 } 989 990 public String fhirType() { 991 return "Slot"; 992 993 } 994 995 public Slot copy() { 996 Slot dst = new Slot(); 997 copyValues(dst); 998 if (identifier != null) { 999 dst.identifier = new ArrayList<Identifier>(); 1000 for (Identifier i : identifier) 1001 dst.identifier.add(i.copy()); 1002 }; 1003 dst.serviceCategory = serviceCategory == null ? null : serviceCategory.copy(); 1004 if (serviceType != null) { 1005 dst.serviceType = new ArrayList<CodeableConcept>(); 1006 for (CodeableConcept i : serviceType) 1007 dst.serviceType.add(i.copy()); 1008 }; 1009 if (specialty != null) { 1010 dst.specialty = new ArrayList<CodeableConcept>(); 1011 for (CodeableConcept i : specialty) 1012 dst.specialty.add(i.copy()); 1013 }; 1014 dst.appointmentType = appointmentType == null ? null : appointmentType.copy(); 1015 dst.schedule = schedule == null ? null : schedule.copy(); 1016 dst.status = status == null ? null : status.copy(); 1017 dst.start = start == null ? null : start.copy(); 1018 dst.end = end == null ? null : end.copy(); 1019 dst.overbooked = overbooked == null ? null : overbooked.copy(); 1020 dst.comment = comment == null ? null : comment.copy(); 1021 return dst; 1022 } 1023 1024 protected Slot typedCopy() { 1025 return copy(); 1026 } 1027 1028 @Override 1029 public boolean equalsDeep(Base other_) { 1030 if (!super.equalsDeep(other_)) 1031 return false; 1032 if (!(other_ instanceof Slot)) 1033 return false; 1034 Slot o = (Slot) other_; 1035 return compareDeep(identifier, o.identifier, true) && compareDeep(serviceCategory, o.serviceCategory, true) 1036 && compareDeep(serviceType, o.serviceType, true) && compareDeep(specialty, o.specialty, true) && compareDeep(appointmentType, o.appointmentType, true) 1037 && compareDeep(schedule, o.schedule, true) && compareDeep(status, o.status, true) && compareDeep(start, o.start, true) 1038 && compareDeep(end, o.end, true) && compareDeep(overbooked, o.overbooked, true) && compareDeep(comment, o.comment, true) 1039 ; 1040 } 1041 1042 @Override 1043 public boolean equalsShallow(Base other_) { 1044 if (!super.equalsShallow(other_)) 1045 return false; 1046 if (!(other_ instanceof Slot)) 1047 return false; 1048 Slot o = (Slot) other_; 1049 return compareValues(status, o.status, true) && compareValues(start, o.start, true) && compareValues(end, o.end, true) 1050 && compareValues(overbooked, o.overbooked, true) && compareValues(comment, o.comment, true); 1051 } 1052 1053 public boolean isEmpty() { 1054 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, serviceCategory 1055 , serviceType, specialty, appointmentType, schedule, status, start, end, overbooked 1056 , comment); 1057 } 1058 1059 @Override 1060 public ResourceType getResourceType() { 1061 return ResourceType.Slot; 1062 } 1063 1064 /** 1065 * Search parameter: <b>schedule</b> 1066 * <p> 1067 * Description: <b>The Schedule Resource that we are seeking a slot within</b><br> 1068 * Type: <b>reference</b><br> 1069 * Path: <b>Slot.schedule</b><br> 1070 * </p> 1071 */ 1072 @SearchParamDefinition(name="schedule", path="Slot.schedule", description="The Schedule Resource that we are seeking a slot within", type="reference", target={Schedule.class } ) 1073 public static final String SP_SCHEDULE = "schedule"; 1074 /** 1075 * <b>Fluent Client</b> search parameter constant for <b>schedule</b> 1076 * <p> 1077 * Description: <b>The Schedule Resource that we are seeking a slot within</b><br> 1078 * Type: <b>reference</b><br> 1079 * Path: <b>Slot.schedule</b><br> 1080 * </p> 1081 */ 1082 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SCHEDULE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SCHEDULE); 1083 1084/** 1085 * Constant for fluent queries to be used to add include statements. Specifies 1086 * the path value of "<b>Slot:schedule</b>". 1087 */ 1088 public static final ca.uhn.fhir.model.api.Include INCLUDE_SCHEDULE = new ca.uhn.fhir.model.api.Include("Slot:schedule").toLocked(); 1089 1090 /** 1091 * Search parameter: <b>identifier</b> 1092 * <p> 1093 * Description: <b>A Slot Identifier</b><br> 1094 * Type: <b>token</b><br> 1095 * Path: <b>Slot.identifier</b><br> 1096 * </p> 1097 */ 1098 @SearchParamDefinition(name="identifier", path="Slot.identifier", description="A Slot Identifier", type="token" ) 1099 public static final String SP_IDENTIFIER = "identifier"; 1100 /** 1101 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1102 * <p> 1103 * Description: <b>A Slot Identifier</b><br> 1104 * Type: <b>token</b><br> 1105 * Path: <b>Slot.identifier</b><br> 1106 * </p> 1107 */ 1108 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1109 1110 /** 1111 * Search parameter: <b>start</b> 1112 * <p> 1113 * Description: <b>Appointment date/time.</b><br> 1114 * Type: <b>date</b><br> 1115 * Path: <b>Slot.start</b><br> 1116 * </p> 1117 */ 1118 @SearchParamDefinition(name="start", path="Slot.start", description="Appointment date/time.", type="date" ) 1119 public static final String SP_START = "start"; 1120 /** 1121 * <b>Fluent Client</b> search parameter constant for <b>start</b> 1122 * <p> 1123 * Description: <b>Appointment date/time.</b><br> 1124 * Type: <b>date</b><br> 1125 * Path: <b>Slot.start</b><br> 1126 * </p> 1127 */ 1128 public static final ca.uhn.fhir.rest.gclient.DateClientParam START = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_START); 1129 1130 /** 1131 * Search parameter: <b>slot-type</b> 1132 * <p> 1133 * Description: <b>The type of appointments that can be booked into the slot</b><br> 1134 * Type: <b>token</b><br> 1135 * Path: <b>Slot.serviceType</b><br> 1136 * </p> 1137 */ 1138 @SearchParamDefinition(name="slot-type", path="Slot.serviceType", description="The type of appointments that can be booked into the slot", type="token" ) 1139 public static final String SP_SLOT_TYPE = "slot-type"; 1140 /** 1141 * <b>Fluent Client</b> search parameter constant for <b>slot-type</b> 1142 * <p> 1143 * Description: <b>The type of appointments that can be booked into the slot</b><br> 1144 * Type: <b>token</b><br> 1145 * Path: <b>Slot.serviceType</b><br> 1146 * </p> 1147 */ 1148 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SLOT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SLOT_TYPE); 1149 1150 /** 1151 * Search parameter: <b>status</b> 1152 * <p> 1153 * Description: <b>The free/busy status of the appointment</b><br> 1154 * Type: <b>token</b><br> 1155 * Path: <b>Slot.status</b><br> 1156 * </p> 1157 */ 1158 @SearchParamDefinition(name="status", path="Slot.status", description="The free/busy status of the appointment", type="token" ) 1159 public static final String SP_STATUS = "status"; 1160 /** 1161 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1162 * <p> 1163 * Description: <b>The free/busy status of the appointment</b><br> 1164 * Type: <b>token</b><br> 1165 * Path: <b>Slot.status</b><br> 1166 * </p> 1167 */ 1168 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1169 1170 1171}