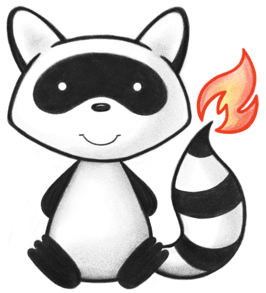
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A sample to be used for analysis. 051 */ 052@ResourceDef(name="Specimen", profile="http://hl7.org/fhir/Profile/Specimen") 053public class Specimen extends DomainResource { 054 055 public enum SpecimenStatus { 056 /** 057 * The physical specimen is present and in good condition. 058 */ 059 AVAILABLE, 060 /** 061 * There is no physical specimen because it is either lost, destroyed or consumed. 062 */ 063 UNAVAILABLE, 064 /** 065 * The specimen cannot be used because of a quality issue such as a broken container, contamination, or too old. 066 */ 067 UNSATISFACTORY, 068 /** 069 * The specimen was entered in error and therefore nullified. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static SpecimenStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("available".equals(codeString)) 080 return AVAILABLE; 081 if ("unavailable".equals(codeString)) 082 return UNAVAILABLE; 083 if ("unsatisfactory".equals(codeString)) 084 return UNSATISFACTORY; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown SpecimenStatus code '"+codeString+"'"); 091 } 092 public String toCode() { 093 switch (this) { 094 case AVAILABLE: return "available"; 095 case UNAVAILABLE: return "unavailable"; 096 case UNSATISFACTORY: return "unsatisfactory"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case AVAILABLE: return "http://hl7.org/fhir/specimen-status"; 105 case UNAVAILABLE: return "http://hl7.org/fhir/specimen-status"; 106 case UNSATISFACTORY: return "http://hl7.org/fhir/specimen-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/specimen-status"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDefinition() { 113 switch (this) { 114 case AVAILABLE: return "The physical specimen is present and in good condition."; 115 case UNAVAILABLE: return "There is no physical specimen because it is either lost, destroyed or consumed."; 116 case UNSATISFACTORY: return "The specimen cannot be used because of a quality issue such as a broken container, contamination, or too old."; 117 case ENTEREDINERROR: return "The specimen was entered in error and therefore nullified."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case AVAILABLE: return "Available"; 125 case UNAVAILABLE: return "Unavailable"; 126 case UNSATISFACTORY: return "Unsatisfactory"; 127 case ENTEREDINERROR: return "Entered-in-error"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 } 133 134 public static class SpecimenStatusEnumFactory implements EnumFactory<SpecimenStatus> { 135 public SpecimenStatus fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("available".equals(codeString)) 140 return SpecimenStatus.AVAILABLE; 141 if ("unavailable".equals(codeString)) 142 return SpecimenStatus.UNAVAILABLE; 143 if ("unsatisfactory".equals(codeString)) 144 return SpecimenStatus.UNSATISFACTORY; 145 if ("entered-in-error".equals(codeString)) 146 return SpecimenStatus.ENTEREDINERROR; 147 throw new IllegalArgumentException("Unknown SpecimenStatus code '"+codeString+"'"); 148 } 149 public Enumeration<SpecimenStatus> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<SpecimenStatus>(this); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("available".equals(codeString)) 158 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.AVAILABLE); 159 if ("unavailable".equals(codeString)) 160 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.UNAVAILABLE); 161 if ("unsatisfactory".equals(codeString)) 162 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.UNSATISFACTORY); 163 if ("entered-in-error".equals(codeString)) 164 return new Enumeration<SpecimenStatus>(this, SpecimenStatus.ENTEREDINERROR); 165 throw new FHIRException("Unknown SpecimenStatus code '"+codeString+"'"); 166 } 167 public String toCode(SpecimenStatus code) { 168 if (code == SpecimenStatus.NULL) 169 return null; 170 if (code == SpecimenStatus.AVAILABLE) 171 return "available"; 172 if (code == SpecimenStatus.UNAVAILABLE) 173 return "unavailable"; 174 if (code == SpecimenStatus.UNSATISFACTORY) 175 return "unsatisfactory"; 176 if (code == SpecimenStatus.ENTEREDINERROR) 177 return "entered-in-error"; 178 return "?"; 179 } 180 public String toSystem(SpecimenStatus code) { 181 return code.getSystem(); 182 } 183 } 184 185 @Block() 186 public static class SpecimenCollectionComponent extends BackboneElement implements IBaseBackboneElement { 187 /** 188 * Person who collected the specimen. 189 */ 190 @Child(name = "collector", type = {Practitioner.class}, order=1, min=0, max=1, modifier=false, summary=true) 191 @Description(shortDefinition="Who collected the specimen", formalDefinition="Person who collected the specimen." ) 192 protected Reference collector; 193 194 /** 195 * The actual object that is the target of the reference (Person who collected the specimen.) 196 */ 197 protected Practitioner collectorTarget; 198 199 /** 200 * Time when specimen was collected from subject - the physiologically relevant time. 201 */ 202 @Child(name = "collected", type = {DateTimeType.class, Period.class}, order=2, min=0, max=1, modifier=false, summary=true) 203 @Description(shortDefinition="Collection time", formalDefinition="Time when specimen was collected from subject - the physiologically relevant time." ) 204 protected Type collected; 205 206 /** 207 * The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample. 208 */ 209 @Child(name = "quantity", type = {SimpleQuantity.class}, order=3, min=0, max=1, modifier=false, summary=false) 210 @Description(shortDefinition="The quantity of specimen collected", formalDefinition="The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample." ) 211 protected SimpleQuantity quantity; 212 213 /** 214 * A coded value specifying the technique that is used to perform the procedure. 215 */ 216 @Child(name = "method", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 217 @Description(shortDefinition="Technique used to perform collection", formalDefinition="A coded value specifying the technique that is used to perform the procedure." ) 218 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-collection-method") 219 protected CodeableConcept method; 220 221 /** 222 * Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens. 223 */ 224 @Child(name = "bodySite", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 225 @Description(shortDefinition="Anatomical collection site", formalDefinition="Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens." ) 226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 227 protected CodeableConcept bodySite; 228 229 private static final long serialVersionUID = -1324142853L; 230 231 /** 232 * Constructor 233 */ 234 public SpecimenCollectionComponent() { 235 super(); 236 } 237 238 /** 239 * @return {@link #collector} (Person who collected the specimen.) 240 */ 241 public Reference getCollector() { 242 if (this.collector == null) 243 if (Configuration.errorOnAutoCreate()) 244 throw new Error("Attempt to auto-create SpecimenCollectionComponent.collector"); 245 else if (Configuration.doAutoCreate()) 246 this.collector = new Reference(); // cc 247 return this.collector; 248 } 249 250 public boolean hasCollector() { 251 return this.collector != null && !this.collector.isEmpty(); 252 } 253 254 /** 255 * @param value {@link #collector} (Person who collected the specimen.) 256 */ 257 public SpecimenCollectionComponent setCollector(Reference value) { 258 this.collector = value; 259 return this; 260 } 261 262 /** 263 * @return {@link #collector} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Person who collected the specimen.) 264 */ 265 public Practitioner getCollectorTarget() { 266 if (this.collectorTarget == null) 267 if (Configuration.errorOnAutoCreate()) 268 throw new Error("Attempt to auto-create SpecimenCollectionComponent.collector"); 269 else if (Configuration.doAutoCreate()) 270 this.collectorTarget = new Practitioner(); // aa 271 return this.collectorTarget; 272 } 273 274 /** 275 * @param value {@link #collector} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Person who collected the specimen.) 276 */ 277 public SpecimenCollectionComponent setCollectorTarget(Practitioner value) { 278 this.collectorTarget = value; 279 return this; 280 } 281 282 /** 283 * @return {@link #collected} (Time when specimen was collected from subject - the physiologically relevant time.) 284 */ 285 public Type getCollected() { 286 return this.collected; 287 } 288 289 /** 290 * @return {@link #collected} (Time when specimen was collected from subject - the physiologically relevant time.) 291 */ 292 public DateTimeType getCollectedDateTimeType() throws FHIRException { 293 if (this.collected == null) 294 return null; 295 if (!(this.collected instanceof DateTimeType)) 296 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.collected.getClass().getName()+" was encountered"); 297 return (DateTimeType) this.collected; 298 } 299 300 public boolean hasCollectedDateTimeType() { 301 return this != null && this.collected instanceof DateTimeType; 302 } 303 304 /** 305 * @return {@link #collected} (Time when specimen was collected from subject - the physiologically relevant time.) 306 */ 307 public Period getCollectedPeriod() throws FHIRException { 308 if (this.collected == null) 309 return null; 310 if (!(this.collected instanceof Period)) 311 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.collected.getClass().getName()+" was encountered"); 312 return (Period) this.collected; 313 } 314 315 public boolean hasCollectedPeriod() { 316 return this != null && this.collected instanceof Period; 317 } 318 319 public boolean hasCollected() { 320 return this.collected != null && !this.collected.isEmpty(); 321 } 322 323 /** 324 * @param value {@link #collected} (Time when specimen was collected from subject - the physiologically relevant time.) 325 */ 326 public SpecimenCollectionComponent setCollected(Type value) throws FHIRFormatError { 327 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 328 throw new FHIRFormatError("Not the right type for Specimen.collection.collected[x]: "+value.fhirType()); 329 this.collected = value; 330 return this; 331 } 332 333 /** 334 * @return {@link #quantity} (The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.) 335 */ 336 public SimpleQuantity getQuantity() { 337 if (this.quantity == null) 338 if (Configuration.errorOnAutoCreate()) 339 throw new Error("Attempt to auto-create SpecimenCollectionComponent.quantity"); 340 else if (Configuration.doAutoCreate()) 341 this.quantity = new SimpleQuantity(); // cc 342 return this.quantity; 343 } 344 345 public boolean hasQuantity() { 346 return this.quantity != null && !this.quantity.isEmpty(); 347 } 348 349 /** 350 * @param value {@link #quantity} (The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.) 351 */ 352 public SpecimenCollectionComponent setQuantity(SimpleQuantity value) { 353 this.quantity = value; 354 return this; 355 } 356 357 /** 358 * @return {@link #method} (A coded value specifying the technique that is used to perform the procedure.) 359 */ 360 public CodeableConcept getMethod() { 361 if (this.method == null) 362 if (Configuration.errorOnAutoCreate()) 363 throw new Error("Attempt to auto-create SpecimenCollectionComponent.method"); 364 else if (Configuration.doAutoCreate()) 365 this.method = new CodeableConcept(); // cc 366 return this.method; 367 } 368 369 public boolean hasMethod() { 370 return this.method != null && !this.method.isEmpty(); 371 } 372 373 /** 374 * @param value {@link #method} (A coded value specifying the technique that is used to perform the procedure.) 375 */ 376 public SpecimenCollectionComponent setMethod(CodeableConcept value) { 377 this.method = value; 378 return this; 379 } 380 381 /** 382 * @return {@link #bodySite} (Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.) 383 */ 384 public CodeableConcept getBodySite() { 385 if (this.bodySite == null) 386 if (Configuration.errorOnAutoCreate()) 387 throw new Error("Attempt to auto-create SpecimenCollectionComponent.bodySite"); 388 else if (Configuration.doAutoCreate()) 389 this.bodySite = new CodeableConcept(); // cc 390 return this.bodySite; 391 } 392 393 public boolean hasBodySite() { 394 return this.bodySite != null && !this.bodySite.isEmpty(); 395 } 396 397 /** 398 * @param value {@link #bodySite} (Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.) 399 */ 400 public SpecimenCollectionComponent setBodySite(CodeableConcept value) { 401 this.bodySite = value; 402 return this; 403 } 404 405 protected void listChildren(List<Property> children) { 406 super.listChildren(children); 407 children.add(new Property("collector", "Reference(Practitioner)", "Person who collected the specimen.", 0, 1, collector)); 408 children.add(new Property("collected[x]", "dateTime|Period", "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected)); 409 children.add(new Property("quantity", "SimpleQuantity", "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.", 0, 1, quantity)); 410 children.add(new Property("method", "CodeableConcept", "A coded value specifying the technique that is used to perform the procedure.", 0, 1, method)); 411 children.add(new Property("bodySite", "CodeableConcept", "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.", 0, 1, bodySite)); 412 } 413 414 @Override 415 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 416 switch (_hash) { 417 case 1883491469: /*collector*/ return new Property("collector", "Reference(Practitioner)", "Person who collected the specimen.", 0, 1, collector); 418 case 1632037015: /*collected[x]*/ return new Property("collected[x]", "dateTime|Period", "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 419 case 1883491145: /*collected*/ return new Property("collected[x]", "dateTime|Period", "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 420 case 2005009924: /*collectedDateTime*/ return new Property("collected[x]", "dateTime|Period", "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 421 case 653185642: /*collectedPeriod*/ return new Property("collected[x]", "dateTime|Period", "Time when specimen was collected from subject - the physiologically relevant time.", 0, 1, collected); 422 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The quantity of specimen collected; for instance the volume of a blood sample, or the physical measurement of an anatomic pathology sample.", 0, 1, quantity); 423 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "A coded value specifying the technique that is used to perform the procedure.", 0, 1, method); 424 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Anatomical location from which the specimen was collected (if subject is a patient). This is the target site. This element is not used for environmental specimens.", 0, 1, bodySite); 425 default: return super.getNamedProperty(_hash, _name, _checkValid); 426 } 427 428 } 429 430 @Override 431 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 432 switch (hash) { 433 case 1883491469: /*collector*/ return this.collector == null ? new Base[0] : new Base[] {this.collector}; // Reference 434 case 1883491145: /*collected*/ return this.collected == null ? new Base[0] : new Base[] {this.collected}; // Type 435 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 436 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 437 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 438 default: return super.getProperty(hash, name, checkValid); 439 } 440 441 } 442 443 @Override 444 public Base setProperty(int hash, String name, Base value) throws FHIRException { 445 switch (hash) { 446 case 1883491469: // collector 447 this.collector = castToReference(value); // Reference 448 return value; 449 case 1883491145: // collected 450 this.collected = castToType(value); // Type 451 return value; 452 case -1285004149: // quantity 453 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 454 return value; 455 case -1077554975: // method 456 this.method = castToCodeableConcept(value); // CodeableConcept 457 return value; 458 case 1702620169: // bodySite 459 this.bodySite = castToCodeableConcept(value); // CodeableConcept 460 return value; 461 default: return super.setProperty(hash, name, value); 462 } 463 464 } 465 466 @Override 467 public Base setProperty(String name, Base value) throws FHIRException { 468 if (name.equals("collector")) { 469 this.collector = castToReference(value); // Reference 470 } else if (name.equals("collected[x]")) { 471 this.collected = castToType(value); // Type 472 } else if (name.equals("quantity")) { 473 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 474 } else if (name.equals("method")) { 475 this.method = castToCodeableConcept(value); // CodeableConcept 476 } else if (name.equals("bodySite")) { 477 this.bodySite = castToCodeableConcept(value); // CodeableConcept 478 } else 479 return super.setProperty(name, value); 480 return value; 481 } 482 483 @Override 484 public Base makeProperty(int hash, String name) throws FHIRException { 485 switch (hash) { 486 case 1883491469: return getCollector(); 487 case 1632037015: return getCollected(); 488 case 1883491145: return getCollected(); 489 case -1285004149: return getQuantity(); 490 case -1077554975: return getMethod(); 491 case 1702620169: return getBodySite(); 492 default: return super.makeProperty(hash, name); 493 } 494 495 } 496 497 @Override 498 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 499 switch (hash) { 500 case 1883491469: /*collector*/ return new String[] {"Reference"}; 501 case 1883491145: /*collected*/ return new String[] {"dateTime", "Period"}; 502 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 503 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 504 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 505 default: return super.getTypesForProperty(hash, name); 506 } 507 508 } 509 510 @Override 511 public Base addChild(String name) throws FHIRException { 512 if (name.equals("collector")) { 513 this.collector = new Reference(); 514 return this.collector; 515 } 516 else if (name.equals("collectedDateTime")) { 517 this.collected = new DateTimeType(); 518 return this.collected; 519 } 520 else if (name.equals("collectedPeriod")) { 521 this.collected = new Period(); 522 return this.collected; 523 } 524 else if (name.equals("quantity")) { 525 this.quantity = new SimpleQuantity(); 526 return this.quantity; 527 } 528 else if (name.equals("method")) { 529 this.method = new CodeableConcept(); 530 return this.method; 531 } 532 else if (name.equals("bodySite")) { 533 this.bodySite = new CodeableConcept(); 534 return this.bodySite; 535 } 536 else 537 return super.addChild(name); 538 } 539 540 public SpecimenCollectionComponent copy() { 541 SpecimenCollectionComponent dst = new SpecimenCollectionComponent(); 542 copyValues(dst); 543 dst.collector = collector == null ? null : collector.copy(); 544 dst.collected = collected == null ? null : collected.copy(); 545 dst.quantity = quantity == null ? null : quantity.copy(); 546 dst.method = method == null ? null : method.copy(); 547 dst.bodySite = bodySite == null ? null : bodySite.copy(); 548 return dst; 549 } 550 551 @Override 552 public boolean equalsDeep(Base other_) { 553 if (!super.equalsDeep(other_)) 554 return false; 555 if (!(other_ instanceof SpecimenCollectionComponent)) 556 return false; 557 SpecimenCollectionComponent o = (SpecimenCollectionComponent) other_; 558 return compareDeep(collector, o.collector, true) && compareDeep(collected, o.collected, true) && compareDeep(quantity, o.quantity, true) 559 && compareDeep(method, o.method, true) && compareDeep(bodySite, o.bodySite, true); 560 } 561 562 @Override 563 public boolean equalsShallow(Base other_) { 564 if (!super.equalsShallow(other_)) 565 return false; 566 if (!(other_ instanceof SpecimenCollectionComponent)) 567 return false; 568 SpecimenCollectionComponent o = (SpecimenCollectionComponent) other_; 569 return true; 570 } 571 572 public boolean isEmpty() { 573 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(collector, collected, quantity 574 , method, bodySite); 575 } 576 577 public String fhirType() { 578 return "Specimen.collection"; 579 580 } 581 582 } 583 584 @Block() 585 public static class SpecimenProcessingComponent extends BackboneElement implements IBaseBackboneElement { 586 /** 587 * Textual description of procedure. 588 */ 589 @Child(name = "description", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 590 @Description(shortDefinition="Textual description of procedure", formalDefinition="Textual description of procedure." ) 591 protected StringType description; 592 593 /** 594 * A coded value specifying the procedure used to process the specimen. 595 */ 596 @Child(name = "procedure", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 597 @Description(shortDefinition="Indicates the treatment step applied to the specimen", formalDefinition="A coded value specifying the procedure used to process the specimen." ) 598 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-processing-procedure") 599 protected CodeableConcept procedure; 600 601 /** 602 * Material used in the processing step. 603 */ 604 @Child(name = "additive", type = {Substance.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 605 @Description(shortDefinition="Material used in the processing step", formalDefinition="Material used in the processing step." ) 606 protected List<Reference> additive; 607 /** 608 * The actual objects that are the target of the reference (Material used in the processing step.) 609 */ 610 protected List<Substance> additiveTarget; 611 612 613 /** 614 * A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin. 615 */ 616 @Child(name = "time", type = {DateTimeType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 617 @Description(shortDefinition="Date and time of specimen processing", formalDefinition="A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin." ) 618 protected Type time; 619 620 private static final long serialVersionUID = 1467214742L; 621 622 /** 623 * Constructor 624 */ 625 public SpecimenProcessingComponent() { 626 super(); 627 } 628 629 /** 630 * @return {@link #description} (Textual description of procedure.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 631 */ 632 public StringType getDescriptionElement() { 633 if (this.description == null) 634 if (Configuration.errorOnAutoCreate()) 635 throw new Error("Attempt to auto-create SpecimenProcessingComponent.description"); 636 else if (Configuration.doAutoCreate()) 637 this.description = new StringType(); // bb 638 return this.description; 639 } 640 641 public boolean hasDescriptionElement() { 642 return this.description != null && !this.description.isEmpty(); 643 } 644 645 public boolean hasDescription() { 646 return this.description != null && !this.description.isEmpty(); 647 } 648 649 /** 650 * @param value {@link #description} (Textual description of procedure.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 651 */ 652 public SpecimenProcessingComponent setDescriptionElement(StringType value) { 653 this.description = value; 654 return this; 655 } 656 657 /** 658 * @return Textual description of procedure. 659 */ 660 public String getDescription() { 661 return this.description == null ? null : this.description.getValue(); 662 } 663 664 /** 665 * @param value Textual description of procedure. 666 */ 667 public SpecimenProcessingComponent setDescription(String value) { 668 if (Utilities.noString(value)) 669 this.description = null; 670 else { 671 if (this.description == null) 672 this.description = new StringType(); 673 this.description.setValue(value); 674 } 675 return this; 676 } 677 678 /** 679 * @return {@link #procedure} (A coded value specifying the procedure used to process the specimen.) 680 */ 681 public CodeableConcept getProcedure() { 682 if (this.procedure == null) 683 if (Configuration.errorOnAutoCreate()) 684 throw new Error("Attempt to auto-create SpecimenProcessingComponent.procedure"); 685 else if (Configuration.doAutoCreate()) 686 this.procedure = new CodeableConcept(); // cc 687 return this.procedure; 688 } 689 690 public boolean hasProcedure() { 691 return this.procedure != null && !this.procedure.isEmpty(); 692 } 693 694 /** 695 * @param value {@link #procedure} (A coded value specifying the procedure used to process the specimen.) 696 */ 697 public SpecimenProcessingComponent setProcedure(CodeableConcept value) { 698 this.procedure = value; 699 return this; 700 } 701 702 /** 703 * @return {@link #additive} (Material used in the processing step.) 704 */ 705 public List<Reference> getAdditive() { 706 if (this.additive == null) 707 this.additive = new ArrayList<Reference>(); 708 return this.additive; 709 } 710 711 /** 712 * @return Returns a reference to <code>this</code> for easy method chaining 713 */ 714 public SpecimenProcessingComponent setAdditive(List<Reference> theAdditive) { 715 this.additive = theAdditive; 716 return this; 717 } 718 719 public boolean hasAdditive() { 720 if (this.additive == null) 721 return false; 722 for (Reference item : this.additive) 723 if (!item.isEmpty()) 724 return true; 725 return false; 726 } 727 728 public Reference addAdditive() { //3 729 Reference t = new Reference(); 730 if (this.additive == null) 731 this.additive = new ArrayList<Reference>(); 732 this.additive.add(t); 733 return t; 734 } 735 736 public SpecimenProcessingComponent addAdditive(Reference t) { //3 737 if (t == null) 738 return this; 739 if (this.additive == null) 740 this.additive = new ArrayList<Reference>(); 741 this.additive.add(t); 742 return this; 743 } 744 745 /** 746 * @return The first repetition of repeating field {@link #additive}, creating it if it does not already exist 747 */ 748 public Reference getAdditiveFirstRep() { 749 if (getAdditive().isEmpty()) { 750 addAdditive(); 751 } 752 return getAdditive().get(0); 753 } 754 755 /** 756 * @deprecated Use Reference#setResource(IBaseResource) instead 757 */ 758 @Deprecated 759 public List<Substance> getAdditiveTarget() { 760 if (this.additiveTarget == null) 761 this.additiveTarget = new ArrayList<Substance>(); 762 return this.additiveTarget; 763 } 764 765 /** 766 * @deprecated Use Reference#setResource(IBaseResource) instead 767 */ 768 @Deprecated 769 public Substance addAdditiveTarget() { 770 Substance r = new Substance(); 771 if (this.additiveTarget == null) 772 this.additiveTarget = new ArrayList<Substance>(); 773 this.additiveTarget.add(r); 774 return r; 775 } 776 777 /** 778 * @return {@link #time} (A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.) 779 */ 780 public Type getTime() { 781 return this.time; 782 } 783 784 /** 785 * @return {@link #time} (A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.) 786 */ 787 public DateTimeType getTimeDateTimeType() throws FHIRException { 788 if (this.time == null) 789 return null; 790 if (!(this.time instanceof DateTimeType)) 791 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.time.getClass().getName()+" was encountered"); 792 return (DateTimeType) this.time; 793 } 794 795 public boolean hasTimeDateTimeType() { 796 return this != null && this.time instanceof DateTimeType; 797 } 798 799 /** 800 * @return {@link #time} (A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.) 801 */ 802 public Period getTimePeriod() throws FHIRException { 803 if (this.time == null) 804 return null; 805 if (!(this.time instanceof Period)) 806 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.time.getClass().getName()+" was encountered"); 807 return (Period) this.time; 808 } 809 810 public boolean hasTimePeriod() { 811 return this != null && this.time instanceof Period; 812 } 813 814 public boolean hasTime() { 815 return this.time != null && !this.time.isEmpty(); 816 } 817 818 /** 819 * @param value {@link #time} (A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.) 820 */ 821 public SpecimenProcessingComponent setTime(Type value) throws FHIRFormatError { 822 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 823 throw new FHIRFormatError("Not the right type for Specimen.processing.time[x]: "+value.fhirType()); 824 this.time = value; 825 return this; 826 } 827 828 protected void listChildren(List<Property> children) { 829 super.listChildren(children); 830 children.add(new Property("description", "string", "Textual description of procedure.", 0, 1, description)); 831 children.add(new Property("procedure", "CodeableConcept", "A coded value specifying the procedure used to process the specimen.", 0, 1, procedure)); 832 children.add(new Property("additive", "Reference(Substance)", "Material used in the processing step.", 0, java.lang.Integer.MAX_VALUE, additive)); 833 children.add(new Property("time[x]", "dateTime|Period", "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 0, 1, time)); 834 } 835 836 @Override 837 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 838 switch (_hash) { 839 case -1724546052: /*description*/ return new Property("description", "string", "Textual description of procedure.", 0, 1, description); 840 case -1095204141: /*procedure*/ return new Property("procedure", "CodeableConcept", "A coded value specifying the procedure used to process the specimen.", 0, 1, procedure); 841 case -1226589236: /*additive*/ return new Property("additive", "Reference(Substance)", "Material used in the processing step.", 0, java.lang.Integer.MAX_VALUE, additive); 842 case -1313930605: /*time[x]*/ return new Property("time[x]", "dateTime|Period", "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 0, 1, time); 843 case 3560141: /*time*/ return new Property("time[x]", "dateTime|Period", "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 0, 1, time); 844 case 2135345544: /*timeDateTime*/ return new Property("time[x]", "dateTime|Period", "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 0, 1, time); 845 case 693544686: /*timePeriod*/ return new Property("time[x]", "dateTime|Period", "A record of the time or period when the specimen processing occurred. For example the time of sample fixation or the period of time the sample was in formalin.", 0, 1, time); 846 default: return super.getNamedProperty(_hash, _name, _checkValid); 847 } 848 849 } 850 851 @Override 852 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 853 switch (hash) { 854 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 855 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : new Base[] {this.procedure}; // CodeableConcept 856 case -1226589236: /*additive*/ return this.additive == null ? new Base[0] : this.additive.toArray(new Base[this.additive.size()]); // Reference 857 case 3560141: /*time*/ return this.time == null ? new Base[0] : new Base[] {this.time}; // Type 858 default: return super.getProperty(hash, name, checkValid); 859 } 860 861 } 862 863 @Override 864 public Base setProperty(int hash, String name, Base value) throws FHIRException { 865 switch (hash) { 866 case -1724546052: // description 867 this.description = castToString(value); // StringType 868 return value; 869 case -1095204141: // procedure 870 this.procedure = castToCodeableConcept(value); // CodeableConcept 871 return value; 872 case -1226589236: // additive 873 this.getAdditive().add(castToReference(value)); // Reference 874 return value; 875 case 3560141: // time 876 this.time = castToType(value); // Type 877 return value; 878 default: return super.setProperty(hash, name, value); 879 } 880 881 } 882 883 @Override 884 public Base setProperty(String name, Base value) throws FHIRException { 885 if (name.equals("description")) { 886 this.description = castToString(value); // StringType 887 } else if (name.equals("procedure")) { 888 this.procedure = castToCodeableConcept(value); // CodeableConcept 889 } else if (name.equals("additive")) { 890 this.getAdditive().add(castToReference(value)); 891 } else if (name.equals("time[x]")) { 892 this.time = castToType(value); // Type 893 } else 894 return super.setProperty(name, value); 895 return value; 896 } 897 898 @Override 899 public Base makeProperty(int hash, String name) throws FHIRException { 900 switch (hash) { 901 case -1724546052: return getDescriptionElement(); 902 case -1095204141: return getProcedure(); 903 case -1226589236: return addAdditive(); 904 case -1313930605: return getTime(); 905 case 3560141: return getTime(); 906 default: return super.makeProperty(hash, name); 907 } 908 909 } 910 911 @Override 912 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 913 switch (hash) { 914 case -1724546052: /*description*/ return new String[] {"string"}; 915 case -1095204141: /*procedure*/ return new String[] {"CodeableConcept"}; 916 case -1226589236: /*additive*/ return new String[] {"Reference"}; 917 case 3560141: /*time*/ return new String[] {"dateTime", "Period"}; 918 default: return super.getTypesForProperty(hash, name); 919 } 920 921 } 922 923 @Override 924 public Base addChild(String name) throws FHIRException { 925 if (name.equals("description")) { 926 throw new FHIRException("Cannot call addChild on a singleton property Specimen.description"); 927 } 928 else if (name.equals("procedure")) { 929 this.procedure = new CodeableConcept(); 930 return this.procedure; 931 } 932 else if (name.equals("additive")) { 933 return addAdditive(); 934 } 935 else if (name.equals("timeDateTime")) { 936 this.time = new DateTimeType(); 937 return this.time; 938 } 939 else if (name.equals("timePeriod")) { 940 this.time = new Period(); 941 return this.time; 942 } 943 else 944 return super.addChild(name); 945 } 946 947 public SpecimenProcessingComponent copy() { 948 SpecimenProcessingComponent dst = new SpecimenProcessingComponent(); 949 copyValues(dst); 950 dst.description = description == null ? null : description.copy(); 951 dst.procedure = procedure == null ? null : procedure.copy(); 952 if (additive != null) { 953 dst.additive = new ArrayList<Reference>(); 954 for (Reference i : additive) 955 dst.additive.add(i.copy()); 956 }; 957 dst.time = time == null ? null : time.copy(); 958 return dst; 959 } 960 961 @Override 962 public boolean equalsDeep(Base other_) { 963 if (!super.equalsDeep(other_)) 964 return false; 965 if (!(other_ instanceof SpecimenProcessingComponent)) 966 return false; 967 SpecimenProcessingComponent o = (SpecimenProcessingComponent) other_; 968 return compareDeep(description, o.description, true) && compareDeep(procedure, o.procedure, true) 969 && compareDeep(additive, o.additive, true) && compareDeep(time, o.time, true); 970 } 971 972 @Override 973 public boolean equalsShallow(Base other_) { 974 if (!super.equalsShallow(other_)) 975 return false; 976 if (!(other_ instanceof SpecimenProcessingComponent)) 977 return false; 978 SpecimenProcessingComponent o = (SpecimenProcessingComponent) other_; 979 return compareValues(description, o.description, true); 980 } 981 982 public boolean isEmpty() { 983 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, procedure, additive 984 , time); 985 } 986 987 public String fhirType() { 988 return "Specimen.processing"; 989 990 } 991 992 } 993 994 @Block() 995 public static class SpecimenContainerComponent extends BackboneElement implements IBaseBackboneElement { 996 /** 997 * Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances. 998 */ 999 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1000 @Description(shortDefinition="Id for the container", formalDefinition="Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances." ) 1001 protected List<Identifier> identifier; 1002 1003 /** 1004 * Textual description of the container. 1005 */ 1006 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1007 @Description(shortDefinition="Textual description of the container", formalDefinition="Textual description of the container." ) 1008 protected StringType description; 1009 1010 /** 1011 * The type of container associated with the specimen (e.g. slide, aliquot, etc.). 1012 */ 1013 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1014 @Description(shortDefinition="Kind of container directly associated with specimen", formalDefinition="The type of container associated with the specimen (e.g. slide, aliquot, etc.)." ) 1015 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-container-type") 1016 protected CodeableConcept type; 1017 1018 /** 1019 * The capacity (volume or other measure) the container may contain. 1020 */ 1021 @Child(name = "capacity", type = {SimpleQuantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 1022 @Description(shortDefinition="Container volume or size", formalDefinition="The capacity (volume or other measure) the container may contain." ) 1023 protected SimpleQuantity capacity; 1024 1025 /** 1026 * The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type. 1027 */ 1028 @Child(name = "specimenQuantity", type = {SimpleQuantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 1029 @Description(shortDefinition="Quantity of specimen within container", formalDefinition="The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type." ) 1030 protected SimpleQuantity specimenQuantity; 1031 1032 /** 1033 * Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA. 1034 */ 1035 @Child(name = "additive", type = {CodeableConcept.class, Substance.class}, order=6, min=0, max=1, modifier=false, summary=false) 1036 @Description(shortDefinition="Additive associated with container", formalDefinition="Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA." ) 1037 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v2-0371") 1038 protected Type additive; 1039 1040 private static final long serialVersionUID = 187274879L; 1041 1042 /** 1043 * Constructor 1044 */ 1045 public SpecimenContainerComponent() { 1046 super(); 1047 } 1048 1049 /** 1050 * @return {@link #identifier} (Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.) 1051 */ 1052 public List<Identifier> getIdentifier() { 1053 if (this.identifier == null) 1054 this.identifier = new ArrayList<Identifier>(); 1055 return this.identifier; 1056 } 1057 1058 /** 1059 * @return Returns a reference to <code>this</code> for easy method chaining 1060 */ 1061 public SpecimenContainerComponent setIdentifier(List<Identifier> theIdentifier) { 1062 this.identifier = theIdentifier; 1063 return this; 1064 } 1065 1066 public boolean hasIdentifier() { 1067 if (this.identifier == null) 1068 return false; 1069 for (Identifier item : this.identifier) 1070 if (!item.isEmpty()) 1071 return true; 1072 return false; 1073 } 1074 1075 public Identifier addIdentifier() { //3 1076 Identifier t = new Identifier(); 1077 if (this.identifier == null) 1078 this.identifier = new ArrayList<Identifier>(); 1079 this.identifier.add(t); 1080 return t; 1081 } 1082 1083 public SpecimenContainerComponent addIdentifier(Identifier t) { //3 1084 if (t == null) 1085 return this; 1086 if (this.identifier == null) 1087 this.identifier = new ArrayList<Identifier>(); 1088 this.identifier.add(t); 1089 return this; 1090 } 1091 1092 /** 1093 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1094 */ 1095 public Identifier getIdentifierFirstRep() { 1096 if (getIdentifier().isEmpty()) { 1097 addIdentifier(); 1098 } 1099 return getIdentifier().get(0); 1100 } 1101 1102 /** 1103 * @return {@link #description} (Textual description of the container.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1104 */ 1105 public StringType getDescriptionElement() { 1106 if (this.description == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create SpecimenContainerComponent.description"); 1109 else if (Configuration.doAutoCreate()) 1110 this.description = new StringType(); // bb 1111 return this.description; 1112 } 1113 1114 public boolean hasDescriptionElement() { 1115 return this.description != null && !this.description.isEmpty(); 1116 } 1117 1118 public boolean hasDescription() { 1119 return this.description != null && !this.description.isEmpty(); 1120 } 1121 1122 /** 1123 * @param value {@link #description} (Textual description of the container.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1124 */ 1125 public SpecimenContainerComponent setDescriptionElement(StringType value) { 1126 this.description = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return Textual description of the container. 1132 */ 1133 public String getDescription() { 1134 return this.description == null ? null : this.description.getValue(); 1135 } 1136 1137 /** 1138 * @param value Textual description of the container. 1139 */ 1140 public SpecimenContainerComponent setDescription(String value) { 1141 if (Utilities.noString(value)) 1142 this.description = null; 1143 else { 1144 if (this.description == null) 1145 this.description = new StringType(); 1146 this.description.setValue(value); 1147 } 1148 return this; 1149 } 1150 1151 /** 1152 * @return {@link #type} (The type of container associated with the specimen (e.g. slide, aliquot, etc.).) 1153 */ 1154 public CodeableConcept getType() { 1155 if (this.type == null) 1156 if (Configuration.errorOnAutoCreate()) 1157 throw new Error("Attempt to auto-create SpecimenContainerComponent.type"); 1158 else if (Configuration.doAutoCreate()) 1159 this.type = new CodeableConcept(); // cc 1160 return this.type; 1161 } 1162 1163 public boolean hasType() { 1164 return this.type != null && !this.type.isEmpty(); 1165 } 1166 1167 /** 1168 * @param value {@link #type} (The type of container associated with the specimen (e.g. slide, aliquot, etc.).) 1169 */ 1170 public SpecimenContainerComponent setType(CodeableConcept value) { 1171 this.type = value; 1172 return this; 1173 } 1174 1175 /** 1176 * @return {@link #capacity} (The capacity (volume or other measure) the container may contain.) 1177 */ 1178 public SimpleQuantity getCapacity() { 1179 if (this.capacity == null) 1180 if (Configuration.errorOnAutoCreate()) 1181 throw new Error("Attempt to auto-create SpecimenContainerComponent.capacity"); 1182 else if (Configuration.doAutoCreate()) 1183 this.capacity = new SimpleQuantity(); // cc 1184 return this.capacity; 1185 } 1186 1187 public boolean hasCapacity() { 1188 return this.capacity != null && !this.capacity.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #capacity} (The capacity (volume or other measure) the container may contain.) 1193 */ 1194 public SpecimenContainerComponent setCapacity(SimpleQuantity value) { 1195 this.capacity = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #specimenQuantity} (The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.) 1201 */ 1202 public SimpleQuantity getSpecimenQuantity() { 1203 if (this.specimenQuantity == null) 1204 if (Configuration.errorOnAutoCreate()) 1205 throw new Error("Attempt to auto-create SpecimenContainerComponent.specimenQuantity"); 1206 else if (Configuration.doAutoCreate()) 1207 this.specimenQuantity = new SimpleQuantity(); // cc 1208 return this.specimenQuantity; 1209 } 1210 1211 public boolean hasSpecimenQuantity() { 1212 return this.specimenQuantity != null && !this.specimenQuantity.isEmpty(); 1213 } 1214 1215 /** 1216 * @param value {@link #specimenQuantity} (The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.) 1217 */ 1218 public SpecimenContainerComponent setSpecimenQuantity(SimpleQuantity value) { 1219 this.specimenQuantity = value; 1220 return this; 1221 } 1222 1223 /** 1224 * @return {@link #additive} (Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1225 */ 1226 public Type getAdditive() { 1227 return this.additive; 1228 } 1229 1230 /** 1231 * @return {@link #additive} (Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1232 */ 1233 public CodeableConcept getAdditiveCodeableConcept() throws FHIRException { 1234 if (this.additive == null) 1235 return null; 1236 if (!(this.additive instanceof CodeableConcept)) 1237 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.additive.getClass().getName()+" was encountered"); 1238 return (CodeableConcept) this.additive; 1239 } 1240 1241 public boolean hasAdditiveCodeableConcept() { 1242 return this != null && this.additive instanceof CodeableConcept; 1243 } 1244 1245 /** 1246 * @return {@link #additive} (Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1247 */ 1248 public Reference getAdditiveReference() throws FHIRException { 1249 if (this.additive == null) 1250 return null; 1251 if (!(this.additive instanceof Reference)) 1252 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.additive.getClass().getName()+" was encountered"); 1253 return (Reference) this.additive; 1254 } 1255 1256 public boolean hasAdditiveReference() { 1257 return this != null && this.additive instanceof Reference; 1258 } 1259 1260 public boolean hasAdditive() { 1261 return this.additive != null && !this.additive.isEmpty(); 1262 } 1263 1264 /** 1265 * @param value {@link #additive} (Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.) 1266 */ 1267 public SpecimenContainerComponent setAdditive(Type value) throws FHIRFormatError { 1268 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1269 throw new FHIRFormatError("Not the right type for Specimen.container.additive[x]: "+value.fhirType()); 1270 this.additive = value; 1271 return this; 1272 } 1273 1274 protected void listChildren(List<Property> children) { 1275 super.listChildren(children); 1276 children.add(new Property("identifier", "Identifier", "Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1277 children.add(new Property("description", "string", "Textual description of the container.", 0, 1, description)); 1278 children.add(new Property("type", "CodeableConcept", "The type of container associated with the specimen (e.g. slide, aliquot, etc.).", 0, 1, type)); 1279 children.add(new Property("capacity", "SimpleQuantity", "The capacity (volume or other measure) the container may contain.", 0, 1, capacity)); 1280 children.add(new Property("specimenQuantity", "SimpleQuantity", "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.", 0, 1, specimenQuantity)); 1281 children.add(new Property("additive[x]", "CodeableConcept|Reference(Substance)", "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1, additive)); 1282 } 1283 1284 @Override 1285 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1286 switch (_hash) { 1287 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Id for container. There may be multiple; a manufacturer's bar code, lab assigned identifier, etc. The container ID may differ from the specimen id in some circumstances.", 0, java.lang.Integer.MAX_VALUE, identifier); 1288 case -1724546052: /*description*/ return new Property("description", "string", "Textual description of the container.", 0, 1, description); 1289 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of container associated with the specimen (e.g. slide, aliquot, etc.).", 0, 1, type); 1290 case -67824454: /*capacity*/ return new Property("capacity", "SimpleQuantity", "The capacity (volume or other measure) the container may contain.", 0, 1, capacity); 1291 case 1485980595: /*specimenQuantity*/ return new Property("specimenQuantity", "SimpleQuantity", "The quantity of specimen in the container; may be volume, dimensions, or other appropriate measurements, depending on the specimen type.", 0, 1, specimenQuantity); 1292 case 261915956: /*additive[x]*/ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1, additive); 1293 case -1226589236: /*additive*/ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1, additive); 1294 case 1330272821: /*additiveCodeableConcept*/ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1, additive); 1295 case -386783009: /*additiveReference*/ return new Property("additive[x]", "CodeableConcept|Reference(Substance)", "Introduced substance to preserve, maintain or enhance the specimen. Examples: Formalin, Citrate, EDTA.", 0, 1, additive); 1296 default: return super.getNamedProperty(_hash, _name, _checkValid); 1297 } 1298 1299 } 1300 1301 @Override 1302 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1303 switch (hash) { 1304 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1305 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1306 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1307 case -67824454: /*capacity*/ return this.capacity == null ? new Base[0] : new Base[] {this.capacity}; // SimpleQuantity 1308 case 1485980595: /*specimenQuantity*/ return this.specimenQuantity == null ? new Base[0] : new Base[] {this.specimenQuantity}; // SimpleQuantity 1309 case -1226589236: /*additive*/ return this.additive == null ? new Base[0] : new Base[] {this.additive}; // Type 1310 default: return super.getProperty(hash, name, checkValid); 1311 } 1312 1313 } 1314 1315 @Override 1316 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1317 switch (hash) { 1318 case -1618432855: // identifier 1319 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1320 return value; 1321 case -1724546052: // description 1322 this.description = castToString(value); // StringType 1323 return value; 1324 case 3575610: // type 1325 this.type = castToCodeableConcept(value); // CodeableConcept 1326 return value; 1327 case -67824454: // capacity 1328 this.capacity = castToSimpleQuantity(value); // SimpleQuantity 1329 return value; 1330 case 1485980595: // specimenQuantity 1331 this.specimenQuantity = castToSimpleQuantity(value); // SimpleQuantity 1332 return value; 1333 case -1226589236: // additive 1334 this.additive = castToType(value); // Type 1335 return value; 1336 default: return super.setProperty(hash, name, value); 1337 } 1338 1339 } 1340 1341 @Override 1342 public Base setProperty(String name, Base value) throws FHIRException { 1343 if (name.equals("identifier")) { 1344 this.getIdentifier().add(castToIdentifier(value)); 1345 } else if (name.equals("description")) { 1346 this.description = castToString(value); // StringType 1347 } else if (name.equals("type")) { 1348 this.type = castToCodeableConcept(value); // CodeableConcept 1349 } else if (name.equals("capacity")) { 1350 this.capacity = castToSimpleQuantity(value); // SimpleQuantity 1351 } else if (name.equals("specimenQuantity")) { 1352 this.specimenQuantity = castToSimpleQuantity(value); // SimpleQuantity 1353 } else if (name.equals("additive[x]")) { 1354 this.additive = castToType(value); // Type 1355 } else 1356 return super.setProperty(name, value); 1357 return value; 1358 } 1359 1360 @Override 1361 public Base makeProperty(int hash, String name) throws FHIRException { 1362 switch (hash) { 1363 case -1618432855: return addIdentifier(); 1364 case -1724546052: return getDescriptionElement(); 1365 case 3575610: return getType(); 1366 case -67824454: return getCapacity(); 1367 case 1485980595: return getSpecimenQuantity(); 1368 case 261915956: return getAdditive(); 1369 case -1226589236: return getAdditive(); 1370 default: return super.makeProperty(hash, name); 1371 } 1372 1373 } 1374 1375 @Override 1376 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1377 switch (hash) { 1378 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1379 case -1724546052: /*description*/ return new String[] {"string"}; 1380 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1381 case -67824454: /*capacity*/ return new String[] {"SimpleQuantity"}; 1382 case 1485980595: /*specimenQuantity*/ return new String[] {"SimpleQuantity"}; 1383 case -1226589236: /*additive*/ return new String[] {"CodeableConcept", "Reference"}; 1384 default: return super.getTypesForProperty(hash, name); 1385 } 1386 1387 } 1388 1389 @Override 1390 public Base addChild(String name) throws FHIRException { 1391 if (name.equals("identifier")) { 1392 return addIdentifier(); 1393 } 1394 else if (name.equals("description")) { 1395 throw new FHIRException("Cannot call addChild on a singleton property Specimen.description"); 1396 } 1397 else if (name.equals("type")) { 1398 this.type = new CodeableConcept(); 1399 return this.type; 1400 } 1401 else if (name.equals("capacity")) { 1402 this.capacity = new SimpleQuantity(); 1403 return this.capacity; 1404 } 1405 else if (name.equals("specimenQuantity")) { 1406 this.specimenQuantity = new SimpleQuantity(); 1407 return this.specimenQuantity; 1408 } 1409 else if (name.equals("additiveCodeableConcept")) { 1410 this.additive = new CodeableConcept(); 1411 return this.additive; 1412 } 1413 else if (name.equals("additiveReference")) { 1414 this.additive = new Reference(); 1415 return this.additive; 1416 } 1417 else 1418 return super.addChild(name); 1419 } 1420 1421 public SpecimenContainerComponent copy() { 1422 SpecimenContainerComponent dst = new SpecimenContainerComponent(); 1423 copyValues(dst); 1424 if (identifier != null) { 1425 dst.identifier = new ArrayList<Identifier>(); 1426 for (Identifier i : identifier) 1427 dst.identifier.add(i.copy()); 1428 }; 1429 dst.description = description == null ? null : description.copy(); 1430 dst.type = type == null ? null : type.copy(); 1431 dst.capacity = capacity == null ? null : capacity.copy(); 1432 dst.specimenQuantity = specimenQuantity == null ? null : specimenQuantity.copy(); 1433 dst.additive = additive == null ? null : additive.copy(); 1434 return dst; 1435 } 1436 1437 @Override 1438 public boolean equalsDeep(Base other_) { 1439 if (!super.equalsDeep(other_)) 1440 return false; 1441 if (!(other_ instanceof SpecimenContainerComponent)) 1442 return false; 1443 SpecimenContainerComponent o = (SpecimenContainerComponent) other_; 1444 return compareDeep(identifier, o.identifier, true) && compareDeep(description, o.description, true) 1445 && compareDeep(type, o.type, true) && compareDeep(capacity, o.capacity, true) && compareDeep(specimenQuantity, o.specimenQuantity, true) 1446 && compareDeep(additive, o.additive, true); 1447 } 1448 1449 @Override 1450 public boolean equalsShallow(Base other_) { 1451 if (!super.equalsShallow(other_)) 1452 return false; 1453 if (!(other_ instanceof SpecimenContainerComponent)) 1454 return false; 1455 SpecimenContainerComponent o = (SpecimenContainerComponent) other_; 1456 return compareValues(description, o.description, true); 1457 } 1458 1459 public boolean isEmpty() { 1460 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, description, type 1461 , capacity, specimenQuantity, additive); 1462 } 1463 1464 public String fhirType() { 1465 return "Specimen.container"; 1466 1467 } 1468 1469 } 1470 1471 /** 1472 * Id for specimen. 1473 */ 1474 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1475 @Description(shortDefinition="External Identifier", formalDefinition="Id for specimen." ) 1476 protected List<Identifier> identifier; 1477 1478 /** 1479 * The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures. 1480 */ 1481 @Child(name = "accessionIdentifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1482 @Description(shortDefinition="Identifier assigned by the lab", formalDefinition="The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures." ) 1483 protected Identifier accessionIdentifier; 1484 1485 /** 1486 * The availability of the specimen. 1487 */ 1488 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 1489 @Description(shortDefinition="available | unavailable | unsatisfactory | entered-in-error", formalDefinition="The availability of the specimen." ) 1490 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/specimen-status") 1491 protected Enumeration<SpecimenStatus> status; 1492 1493 /** 1494 * The kind of material that forms the specimen. 1495 */ 1496 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 1497 @Description(shortDefinition="Kind of material that forms the specimen", formalDefinition="The kind of material that forms the specimen." ) 1498 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v2-0487") 1499 protected CodeableConcept type; 1500 1501 /** 1502 * Where the specimen came from. This may be from the patient(s) or from the environment or a device. 1503 */ 1504 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Substance.class}, order=4, min=1, max=1, modifier=false, summary=true) 1505 @Description(shortDefinition="Where the specimen came from. This may be from the patient(s) or from the environment or a device", formalDefinition="Where the specimen came from. This may be from the patient(s) or from the environment or a device." ) 1506 protected Reference subject; 1507 1508 /** 1509 * The actual object that is the target of the reference (Where the specimen came from. This may be from the patient(s) or from the environment or a device.) 1510 */ 1511 protected Resource subjectTarget; 1512 1513 /** 1514 * Time when specimen was received for processing or testing. 1515 */ 1516 @Child(name = "receivedTime", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1517 @Description(shortDefinition="The time when specimen was received for processing", formalDefinition="Time when specimen was received for processing or testing." ) 1518 protected DateTimeType receivedTime; 1519 1520 /** 1521 * Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen. 1522 */ 1523 @Child(name = "parent", type = {Specimen.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1524 @Description(shortDefinition="Specimen from which this specimen originated", formalDefinition="Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen." ) 1525 protected List<Reference> parent; 1526 /** 1527 * The actual objects that are the target of the reference (Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.) 1528 */ 1529 protected List<Specimen> parentTarget; 1530 1531 1532 /** 1533 * Details concerning a test or procedure request that required a specimen to be collected. 1534 */ 1535 @Child(name = "request", type = {ProcedureRequest.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1536 @Description(shortDefinition="Why the specimen was collected", formalDefinition="Details concerning a test or procedure request that required a specimen to be collected." ) 1537 protected List<Reference> request; 1538 /** 1539 * The actual objects that are the target of the reference (Details concerning a test or procedure request that required a specimen to be collected.) 1540 */ 1541 protected List<ProcedureRequest> requestTarget; 1542 1543 1544 /** 1545 * Details concerning the specimen collection. 1546 */ 1547 @Child(name = "collection", type = {}, order=8, min=0, max=1, modifier=false, summary=false) 1548 @Description(shortDefinition="Collection details", formalDefinition="Details concerning the specimen collection." ) 1549 protected SpecimenCollectionComponent collection; 1550 1551 /** 1552 * Details concerning processing and processing steps for the specimen. 1553 */ 1554 @Child(name = "processing", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1555 @Description(shortDefinition="Processing and processing step details", formalDefinition="Details concerning processing and processing steps for the specimen." ) 1556 protected List<SpecimenProcessingComponent> processing; 1557 1558 /** 1559 * The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here. 1560 */ 1561 @Child(name = "container", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1562 @Description(shortDefinition="Direct container of specimen (tube/slide, etc.)", formalDefinition="The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here." ) 1563 protected List<SpecimenContainerComponent> container; 1564 1565 /** 1566 * To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen). 1567 */ 1568 @Child(name = "note", type = {Annotation.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1569 @Description(shortDefinition="Comments", formalDefinition="To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen)." ) 1570 protected List<Annotation> note; 1571 1572 private static final long serialVersionUID = -743921079L; 1573 1574 /** 1575 * Constructor 1576 */ 1577 public Specimen() { 1578 super(); 1579 } 1580 1581 /** 1582 * Constructor 1583 */ 1584 public Specimen(Reference subject) { 1585 super(); 1586 this.subject = subject; 1587 } 1588 1589 /** 1590 * @return {@link #identifier} (Id for specimen.) 1591 */ 1592 public List<Identifier> getIdentifier() { 1593 if (this.identifier == null) 1594 this.identifier = new ArrayList<Identifier>(); 1595 return this.identifier; 1596 } 1597 1598 /** 1599 * @return Returns a reference to <code>this</code> for easy method chaining 1600 */ 1601 public Specimen setIdentifier(List<Identifier> theIdentifier) { 1602 this.identifier = theIdentifier; 1603 return this; 1604 } 1605 1606 public boolean hasIdentifier() { 1607 if (this.identifier == null) 1608 return false; 1609 for (Identifier item : this.identifier) 1610 if (!item.isEmpty()) 1611 return true; 1612 return false; 1613 } 1614 1615 public Identifier addIdentifier() { //3 1616 Identifier t = new Identifier(); 1617 if (this.identifier == null) 1618 this.identifier = new ArrayList<Identifier>(); 1619 this.identifier.add(t); 1620 return t; 1621 } 1622 1623 public Specimen addIdentifier(Identifier t) { //3 1624 if (t == null) 1625 return this; 1626 if (this.identifier == null) 1627 this.identifier = new ArrayList<Identifier>(); 1628 this.identifier.add(t); 1629 return this; 1630 } 1631 1632 /** 1633 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1634 */ 1635 public Identifier getIdentifierFirstRep() { 1636 if (getIdentifier().isEmpty()) { 1637 addIdentifier(); 1638 } 1639 return getIdentifier().get(0); 1640 } 1641 1642 /** 1643 * @return {@link #accessionIdentifier} (The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.) 1644 */ 1645 public Identifier getAccessionIdentifier() { 1646 if (this.accessionIdentifier == null) 1647 if (Configuration.errorOnAutoCreate()) 1648 throw new Error("Attempt to auto-create Specimen.accessionIdentifier"); 1649 else if (Configuration.doAutoCreate()) 1650 this.accessionIdentifier = new Identifier(); // cc 1651 return this.accessionIdentifier; 1652 } 1653 1654 public boolean hasAccessionIdentifier() { 1655 return this.accessionIdentifier != null && !this.accessionIdentifier.isEmpty(); 1656 } 1657 1658 /** 1659 * @param value {@link #accessionIdentifier} (The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.) 1660 */ 1661 public Specimen setAccessionIdentifier(Identifier value) { 1662 this.accessionIdentifier = value; 1663 return this; 1664 } 1665 1666 /** 1667 * @return {@link #status} (The availability of the specimen.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1668 */ 1669 public Enumeration<SpecimenStatus> getStatusElement() { 1670 if (this.status == null) 1671 if (Configuration.errorOnAutoCreate()) 1672 throw new Error("Attempt to auto-create Specimen.status"); 1673 else if (Configuration.doAutoCreate()) 1674 this.status = new Enumeration<SpecimenStatus>(new SpecimenStatusEnumFactory()); // bb 1675 return this.status; 1676 } 1677 1678 public boolean hasStatusElement() { 1679 return this.status != null && !this.status.isEmpty(); 1680 } 1681 1682 public boolean hasStatus() { 1683 return this.status != null && !this.status.isEmpty(); 1684 } 1685 1686 /** 1687 * @param value {@link #status} (The availability of the specimen.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1688 */ 1689 public Specimen setStatusElement(Enumeration<SpecimenStatus> value) { 1690 this.status = value; 1691 return this; 1692 } 1693 1694 /** 1695 * @return The availability of the specimen. 1696 */ 1697 public SpecimenStatus getStatus() { 1698 return this.status == null ? null : this.status.getValue(); 1699 } 1700 1701 /** 1702 * @param value The availability of the specimen. 1703 */ 1704 public Specimen setStatus(SpecimenStatus value) { 1705 if (value == null) 1706 this.status = null; 1707 else { 1708 if (this.status == null) 1709 this.status = new Enumeration<SpecimenStatus>(new SpecimenStatusEnumFactory()); 1710 this.status.setValue(value); 1711 } 1712 return this; 1713 } 1714 1715 /** 1716 * @return {@link #type} (The kind of material that forms the specimen.) 1717 */ 1718 public CodeableConcept getType() { 1719 if (this.type == null) 1720 if (Configuration.errorOnAutoCreate()) 1721 throw new Error("Attempt to auto-create Specimen.type"); 1722 else if (Configuration.doAutoCreate()) 1723 this.type = new CodeableConcept(); // cc 1724 return this.type; 1725 } 1726 1727 public boolean hasType() { 1728 return this.type != null && !this.type.isEmpty(); 1729 } 1730 1731 /** 1732 * @param value {@link #type} (The kind of material that forms the specimen.) 1733 */ 1734 public Specimen setType(CodeableConcept value) { 1735 this.type = value; 1736 return this; 1737 } 1738 1739 /** 1740 * @return {@link #subject} (Where the specimen came from. This may be from the patient(s) or from the environment or a device.) 1741 */ 1742 public Reference getSubject() { 1743 if (this.subject == null) 1744 if (Configuration.errorOnAutoCreate()) 1745 throw new Error("Attempt to auto-create Specimen.subject"); 1746 else if (Configuration.doAutoCreate()) 1747 this.subject = new Reference(); // cc 1748 return this.subject; 1749 } 1750 1751 public boolean hasSubject() { 1752 return this.subject != null && !this.subject.isEmpty(); 1753 } 1754 1755 /** 1756 * @param value {@link #subject} (Where the specimen came from. This may be from the patient(s) or from the environment or a device.) 1757 */ 1758 public Specimen setSubject(Reference value) { 1759 this.subject = value; 1760 return this; 1761 } 1762 1763 /** 1764 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Where the specimen came from. This may be from the patient(s) or from the environment or a device.) 1765 */ 1766 public Resource getSubjectTarget() { 1767 return this.subjectTarget; 1768 } 1769 1770 /** 1771 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Where the specimen came from. This may be from the patient(s) or from the environment or a device.) 1772 */ 1773 public Specimen setSubjectTarget(Resource value) { 1774 this.subjectTarget = value; 1775 return this; 1776 } 1777 1778 /** 1779 * @return {@link #receivedTime} (Time when specimen was received for processing or testing.). This is the underlying object with id, value and extensions. The accessor "getReceivedTime" gives direct access to the value 1780 */ 1781 public DateTimeType getReceivedTimeElement() { 1782 if (this.receivedTime == null) 1783 if (Configuration.errorOnAutoCreate()) 1784 throw new Error("Attempt to auto-create Specimen.receivedTime"); 1785 else if (Configuration.doAutoCreate()) 1786 this.receivedTime = new DateTimeType(); // bb 1787 return this.receivedTime; 1788 } 1789 1790 public boolean hasReceivedTimeElement() { 1791 return this.receivedTime != null && !this.receivedTime.isEmpty(); 1792 } 1793 1794 public boolean hasReceivedTime() { 1795 return this.receivedTime != null && !this.receivedTime.isEmpty(); 1796 } 1797 1798 /** 1799 * @param value {@link #receivedTime} (Time when specimen was received for processing or testing.). This is the underlying object with id, value and extensions. The accessor "getReceivedTime" gives direct access to the value 1800 */ 1801 public Specimen setReceivedTimeElement(DateTimeType value) { 1802 this.receivedTime = value; 1803 return this; 1804 } 1805 1806 /** 1807 * @return Time when specimen was received for processing or testing. 1808 */ 1809 public Date getReceivedTime() { 1810 return this.receivedTime == null ? null : this.receivedTime.getValue(); 1811 } 1812 1813 /** 1814 * @param value Time when specimen was received for processing or testing. 1815 */ 1816 public Specimen setReceivedTime(Date value) { 1817 if (value == null) 1818 this.receivedTime = null; 1819 else { 1820 if (this.receivedTime == null) 1821 this.receivedTime = new DateTimeType(); 1822 this.receivedTime.setValue(value); 1823 } 1824 return this; 1825 } 1826 1827 /** 1828 * @return {@link #parent} (Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.) 1829 */ 1830 public List<Reference> getParent() { 1831 if (this.parent == null) 1832 this.parent = new ArrayList<Reference>(); 1833 return this.parent; 1834 } 1835 1836 /** 1837 * @return Returns a reference to <code>this</code> for easy method chaining 1838 */ 1839 public Specimen setParent(List<Reference> theParent) { 1840 this.parent = theParent; 1841 return this; 1842 } 1843 1844 public boolean hasParent() { 1845 if (this.parent == null) 1846 return false; 1847 for (Reference item : this.parent) 1848 if (!item.isEmpty()) 1849 return true; 1850 return false; 1851 } 1852 1853 public Reference addParent() { //3 1854 Reference t = new Reference(); 1855 if (this.parent == null) 1856 this.parent = new ArrayList<Reference>(); 1857 this.parent.add(t); 1858 return t; 1859 } 1860 1861 public Specimen addParent(Reference t) { //3 1862 if (t == null) 1863 return this; 1864 if (this.parent == null) 1865 this.parent = new ArrayList<Reference>(); 1866 this.parent.add(t); 1867 return this; 1868 } 1869 1870 /** 1871 * @return The first repetition of repeating field {@link #parent}, creating it if it does not already exist 1872 */ 1873 public Reference getParentFirstRep() { 1874 if (getParent().isEmpty()) { 1875 addParent(); 1876 } 1877 return getParent().get(0); 1878 } 1879 1880 /** 1881 * @deprecated Use Reference#setResource(IBaseResource) instead 1882 */ 1883 @Deprecated 1884 public List<Specimen> getParentTarget() { 1885 if (this.parentTarget == null) 1886 this.parentTarget = new ArrayList<Specimen>(); 1887 return this.parentTarget; 1888 } 1889 1890 /** 1891 * @deprecated Use Reference#setResource(IBaseResource) instead 1892 */ 1893 @Deprecated 1894 public Specimen addParentTarget() { 1895 Specimen r = new Specimen(); 1896 if (this.parentTarget == null) 1897 this.parentTarget = new ArrayList<Specimen>(); 1898 this.parentTarget.add(r); 1899 return r; 1900 } 1901 1902 /** 1903 * @return {@link #request} (Details concerning a test or procedure request that required a specimen to be collected.) 1904 */ 1905 public List<Reference> getRequest() { 1906 if (this.request == null) 1907 this.request = new ArrayList<Reference>(); 1908 return this.request; 1909 } 1910 1911 /** 1912 * @return Returns a reference to <code>this</code> for easy method chaining 1913 */ 1914 public Specimen setRequest(List<Reference> theRequest) { 1915 this.request = theRequest; 1916 return this; 1917 } 1918 1919 public boolean hasRequest() { 1920 if (this.request == null) 1921 return false; 1922 for (Reference item : this.request) 1923 if (!item.isEmpty()) 1924 return true; 1925 return false; 1926 } 1927 1928 public Reference addRequest() { //3 1929 Reference t = new Reference(); 1930 if (this.request == null) 1931 this.request = new ArrayList<Reference>(); 1932 this.request.add(t); 1933 return t; 1934 } 1935 1936 public Specimen addRequest(Reference t) { //3 1937 if (t == null) 1938 return this; 1939 if (this.request == null) 1940 this.request = new ArrayList<Reference>(); 1941 this.request.add(t); 1942 return this; 1943 } 1944 1945 /** 1946 * @return The first repetition of repeating field {@link #request}, creating it if it does not already exist 1947 */ 1948 public Reference getRequestFirstRep() { 1949 if (getRequest().isEmpty()) { 1950 addRequest(); 1951 } 1952 return getRequest().get(0); 1953 } 1954 1955 /** 1956 * @deprecated Use Reference#setResource(IBaseResource) instead 1957 */ 1958 @Deprecated 1959 public List<ProcedureRequest> getRequestTarget() { 1960 if (this.requestTarget == null) 1961 this.requestTarget = new ArrayList<ProcedureRequest>(); 1962 return this.requestTarget; 1963 } 1964 1965 /** 1966 * @deprecated Use Reference#setResource(IBaseResource) instead 1967 */ 1968 @Deprecated 1969 public ProcedureRequest addRequestTarget() { 1970 ProcedureRequest r = new ProcedureRequest(); 1971 if (this.requestTarget == null) 1972 this.requestTarget = new ArrayList<ProcedureRequest>(); 1973 this.requestTarget.add(r); 1974 return r; 1975 } 1976 1977 /** 1978 * @return {@link #collection} (Details concerning the specimen collection.) 1979 */ 1980 public SpecimenCollectionComponent getCollection() { 1981 if (this.collection == null) 1982 if (Configuration.errorOnAutoCreate()) 1983 throw new Error("Attempt to auto-create Specimen.collection"); 1984 else if (Configuration.doAutoCreate()) 1985 this.collection = new SpecimenCollectionComponent(); // cc 1986 return this.collection; 1987 } 1988 1989 public boolean hasCollection() { 1990 return this.collection != null && !this.collection.isEmpty(); 1991 } 1992 1993 /** 1994 * @param value {@link #collection} (Details concerning the specimen collection.) 1995 */ 1996 public Specimen setCollection(SpecimenCollectionComponent value) { 1997 this.collection = value; 1998 return this; 1999 } 2000 2001 /** 2002 * @return {@link #processing} (Details concerning processing and processing steps for the specimen.) 2003 */ 2004 public List<SpecimenProcessingComponent> getProcessing() { 2005 if (this.processing == null) 2006 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2007 return this.processing; 2008 } 2009 2010 /** 2011 * @return Returns a reference to <code>this</code> for easy method chaining 2012 */ 2013 public Specimen setProcessing(List<SpecimenProcessingComponent> theProcessing) { 2014 this.processing = theProcessing; 2015 return this; 2016 } 2017 2018 public boolean hasProcessing() { 2019 if (this.processing == null) 2020 return false; 2021 for (SpecimenProcessingComponent item : this.processing) 2022 if (!item.isEmpty()) 2023 return true; 2024 return false; 2025 } 2026 2027 public SpecimenProcessingComponent addProcessing() { //3 2028 SpecimenProcessingComponent t = new SpecimenProcessingComponent(); 2029 if (this.processing == null) 2030 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2031 this.processing.add(t); 2032 return t; 2033 } 2034 2035 public Specimen addProcessing(SpecimenProcessingComponent t) { //3 2036 if (t == null) 2037 return this; 2038 if (this.processing == null) 2039 this.processing = new ArrayList<SpecimenProcessingComponent>(); 2040 this.processing.add(t); 2041 return this; 2042 } 2043 2044 /** 2045 * @return The first repetition of repeating field {@link #processing}, creating it if it does not already exist 2046 */ 2047 public SpecimenProcessingComponent getProcessingFirstRep() { 2048 if (getProcessing().isEmpty()) { 2049 addProcessing(); 2050 } 2051 return getProcessing().get(0); 2052 } 2053 2054 /** 2055 * @return {@link #container} (The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.) 2056 */ 2057 public List<SpecimenContainerComponent> getContainer() { 2058 if (this.container == null) 2059 this.container = new ArrayList<SpecimenContainerComponent>(); 2060 return this.container; 2061 } 2062 2063 /** 2064 * @return Returns a reference to <code>this</code> for easy method chaining 2065 */ 2066 public Specimen setContainer(List<SpecimenContainerComponent> theContainer) { 2067 this.container = theContainer; 2068 return this; 2069 } 2070 2071 public boolean hasContainer() { 2072 if (this.container == null) 2073 return false; 2074 for (SpecimenContainerComponent item : this.container) 2075 if (!item.isEmpty()) 2076 return true; 2077 return false; 2078 } 2079 2080 public SpecimenContainerComponent addContainer() { //3 2081 SpecimenContainerComponent t = new SpecimenContainerComponent(); 2082 if (this.container == null) 2083 this.container = new ArrayList<SpecimenContainerComponent>(); 2084 this.container.add(t); 2085 return t; 2086 } 2087 2088 public Specimen addContainer(SpecimenContainerComponent t) { //3 2089 if (t == null) 2090 return this; 2091 if (this.container == null) 2092 this.container = new ArrayList<SpecimenContainerComponent>(); 2093 this.container.add(t); 2094 return this; 2095 } 2096 2097 /** 2098 * @return The first repetition of repeating field {@link #container}, creating it if it does not already exist 2099 */ 2100 public SpecimenContainerComponent getContainerFirstRep() { 2101 if (getContainer().isEmpty()) { 2102 addContainer(); 2103 } 2104 return getContainer().get(0); 2105 } 2106 2107 /** 2108 * @return {@link #note} (To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).) 2109 */ 2110 public List<Annotation> getNote() { 2111 if (this.note == null) 2112 this.note = new ArrayList<Annotation>(); 2113 return this.note; 2114 } 2115 2116 /** 2117 * @return Returns a reference to <code>this</code> for easy method chaining 2118 */ 2119 public Specimen setNote(List<Annotation> theNote) { 2120 this.note = theNote; 2121 return this; 2122 } 2123 2124 public boolean hasNote() { 2125 if (this.note == null) 2126 return false; 2127 for (Annotation item : this.note) 2128 if (!item.isEmpty()) 2129 return true; 2130 return false; 2131 } 2132 2133 public Annotation addNote() { //3 2134 Annotation t = new Annotation(); 2135 if (this.note == null) 2136 this.note = new ArrayList<Annotation>(); 2137 this.note.add(t); 2138 return t; 2139 } 2140 2141 public Specimen addNote(Annotation t) { //3 2142 if (t == null) 2143 return this; 2144 if (this.note == null) 2145 this.note = new ArrayList<Annotation>(); 2146 this.note.add(t); 2147 return this; 2148 } 2149 2150 /** 2151 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2152 */ 2153 public Annotation getNoteFirstRep() { 2154 if (getNote().isEmpty()) { 2155 addNote(); 2156 } 2157 return getNote().get(0); 2158 } 2159 2160 protected void listChildren(List<Property> children) { 2161 super.listChildren(children); 2162 children.add(new Property("identifier", "Identifier", "Id for specimen.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2163 children.add(new Property("accessionIdentifier", "Identifier", "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.", 0, 1, accessionIdentifier)); 2164 children.add(new Property("status", "code", "The availability of the specimen.", 0, 1, status)); 2165 children.add(new Property("type", "CodeableConcept", "The kind of material that forms the specimen.", 0, 1, type)); 2166 children.add(new Property("subject", "Reference(Patient|Group|Device|Substance)", "Where the specimen came from. This may be from the patient(s) or from the environment or a device.", 0, 1, subject)); 2167 children.add(new Property("receivedTime", "dateTime", "Time when specimen was received for processing or testing.", 0, 1, receivedTime)); 2168 children.add(new Property("parent", "Reference(Specimen)", "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.", 0, java.lang.Integer.MAX_VALUE, parent)); 2169 children.add(new Property("request", "Reference(ProcedureRequest)", "Details concerning a test or procedure request that required a specimen to be collected.", 0, java.lang.Integer.MAX_VALUE, request)); 2170 children.add(new Property("collection", "", "Details concerning the specimen collection.", 0, 1, collection)); 2171 children.add(new Property("processing", "", "Details concerning processing and processing steps for the specimen.", 0, java.lang.Integer.MAX_VALUE, processing)); 2172 children.add(new Property("container", "", "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.", 0, java.lang.Integer.MAX_VALUE, container)); 2173 children.add(new Property("note", "Annotation", "To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).", 0, java.lang.Integer.MAX_VALUE, note)); 2174 } 2175 2176 @Override 2177 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2178 switch (_hash) { 2179 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Id for specimen.", 0, java.lang.Integer.MAX_VALUE, identifier); 2180 case 818734061: /*accessionIdentifier*/ return new Property("accessionIdentifier", "Identifier", "The identifier assigned by the lab when accessioning specimen(s). This is not necessarily the same as the specimen identifier, depending on local lab procedures.", 0, 1, accessionIdentifier); 2181 case -892481550: /*status*/ return new Property("status", "code", "The availability of the specimen.", 0, 1, status); 2182 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of material that forms the specimen.", 0, 1, type); 2183 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Substance)", "Where the specimen came from. This may be from the patient(s) or from the environment or a device.", 0, 1, subject); 2184 case -767961010: /*receivedTime*/ return new Property("receivedTime", "dateTime", "Time when specimen was received for processing or testing.", 0, 1, receivedTime); 2185 case -995424086: /*parent*/ return new Property("parent", "Reference(Specimen)", "Reference to the parent (source) specimen which is used when the specimen was either derived from or a component of another specimen.", 0, java.lang.Integer.MAX_VALUE, parent); 2186 case 1095692943: /*request*/ return new Property("request", "Reference(ProcedureRequest)", "Details concerning a test or procedure request that required a specimen to be collected.", 0, java.lang.Integer.MAX_VALUE, request); 2187 case -1741312354: /*collection*/ return new Property("collection", "", "Details concerning the specimen collection.", 0, 1, collection); 2188 case 422194963: /*processing*/ return new Property("processing", "", "Details concerning processing and processing steps for the specimen.", 0, java.lang.Integer.MAX_VALUE, processing); 2189 case -410956671: /*container*/ return new Property("container", "", "The container holding the specimen. The recursive nature of containers; i.e. blood in tube in tray in rack is not addressed here.", 0, java.lang.Integer.MAX_VALUE, container); 2190 case 3387378: /*note*/ return new Property("note", "Annotation", "To communicate any details or issues about the specimen or during the specimen collection. (for example: broken vial, sent with patient, frozen).", 0, java.lang.Integer.MAX_VALUE, note); 2191 default: return super.getNamedProperty(_hash, _name, _checkValid); 2192 } 2193 2194 } 2195 2196 @Override 2197 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2198 switch (hash) { 2199 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2200 case 818734061: /*accessionIdentifier*/ return this.accessionIdentifier == null ? new Base[0] : new Base[] {this.accessionIdentifier}; // Identifier 2201 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SpecimenStatus> 2202 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2203 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2204 case -767961010: /*receivedTime*/ return this.receivedTime == null ? new Base[0] : new Base[] {this.receivedTime}; // DateTimeType 2205 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // Reference 2206 case 1095692943: /*request*/ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 2207 case -1741312354: /*collection*/ return this.collection == null ? new Base[0] : new Base[] {this.collection}; // SpecimenCollectionComponent 2208 case 422194963: /*processing*/ return this.processing == null ? new Base[0] : this.processing.toArray(new Base[this.processing.size()]); // SpecimenProcessingComponent 2209 case -410956671: /*container*/ return this.container == null ? new Base[0] : this.container.toArray(new Base[this.container.size()]); // SpecimenContainerComponent 2210 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2211 default: return super.getProperty(hash, name, checkValid); 2212 } 2213 2214 } 2215 2216 @Override 2217 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2218 switch (hash) { 2219 case -1618432855: // identifier 2220 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2221 return value; 2222 case 818734061: // accessionIdentifier 2223 this.accessionIdentifier = castToIdentifier(value); // Identifier 2224 return value; 2225 case -892481550: // status 2226 value = new SpecimenStatusEnumFactory().fromType(castToCode(value)); 2227 this.status = (Enumeration) value; // Enumeration<SpecimenStatus> 2228 return value; 2229 case 3575610: // type 2230 this.type = castToCodeableConcept(value); // CodeableConcept 2231 return value; 2232 case -1867885268: // subject 2233 this.subject = castToReference(value); // Reference 2234 return value; 2235 case -767961010: // receivedTime 2236 this.receivedTime = castToDateTime(value); // DateTimeType 2237 return value; 2238 case -995424086: // parent 2239 this.getParent().add(castToReference(value)); // Reference 2240 return value; 2241 case 1095692943: // request 2242 this.getRequest().add(castToReference(value)); // Reference 2243 return value; 2244 case -1741312354: // collection 2245 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 2246 return value; 2247 case 422194963: // processing 2248 this.getProcessing().add((SpecimenProcessingComponent) value); // SpecimenProcessingComponent 2249 return value; 2250 case -410956671: // container 2251 this.getContainer().add((SpecimenContainerComponent) value); // SpecimenContainerComponent 2252 return value; 2253 case 3387378: // note 2254 this.getNote().add(castToAnnotation(value)); // Annotation 2255 return value; 2256 default: return super.setProperty(hash, name, value); 2257 } 2258 2259 } 2260 2261 @Override 2262 public Base setProperty(String name, Base value) throws FHIRException { 2263 if (name.equals("identifier")) { 2264 this.getIdentifier().add(castToIdentifier(value)); 2265 } else if (name.equals("accessionIdentifier")) { 2266 this.accessionIdentifier = castToIdentifier(value); // Identifier 2267 } else if (name.equals("status")) { 2268 value = new SpecimenStatusEnumFactory().fromType(castToCode(value)); 2269 this.status = (Enumeration) value; // Enumeration<SpecimenStatus> 2270 } else if (name.equals("type")) { 2271 this.type = castToCodeableConcept(value); // CodeableConcept 2272 } else if (name.equals("subject")) { 2273 this.subject = castToReference(value); // Reference 2274 } else if (name.equals("receivedTime")) { 2275 this.receivedTime = castToDateTime(value); // DateTimeType 2276 } else if (name.equals("parent")) { 2277 this.getParent().add(castToReference(value)); 2278 } else if (name.equals("request")) { 2279 this.getRequest().add(castToReference(value)); 2280 } else if (name.equals("collection")) { 2281 this.collection = (SpecimenCollectionComponent) value; // SpecimenCollectionComponent 2282 } else if (name.equals("processing")) { 2283 this.getProcessing().add((SpecimenProcessingComponent) value); 2284 } else if (name.equals("container")) { 2285 this.getContainer().add((SpecimenContainerComponent) value); 2286 } else if (name.equals("note")) { 2287 this.getNote().add(castToAnnotation(value)); 2288 } else 2289 return super.setProperty(name, value); 2290 return value; 2291 } 2292 2293 @Override 2294 public Base makeProperty(int hash, String name) throws FHIRException { 2295 switch (hash) { 2296 case -1618432855: return addIdentifier(); 2297 case 818734061: return getAccessionIdentifier(); 2298 case -892481550: return getStatusElement(); 2299 case 3575610: return getType(); 2300 case -1867885268: return getSubject(); 2301 case -767961010: return getReceivedTimeElement(); 2302 case -995424086: return addParent(); 2303 case 1095692943: return addRequest(); 2304 case -1741312354: return getCollection(); 2305 case 422194963: return addProcessing(); 2306 case -410956671: return addContainer(); 2307 case 3387378: return addNote(); 2308 default: return super.makeProperty(hash, name); 2309 } 2310 2311 } 2312 2313 @Override 2314 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2315 switch (hash) { 2316 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2317 case 818734061: /*accessionIdentifier*/ return new String[] {"Identifier"}; 2318 case -892481550: /*status*/ return new String[] {"code"}; 2319 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2320 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2321 case -767961010: /*receivedTime*/ return new String[] {"dateTime"}; 2322 case -995424086: /*parent*/ return new String[] {"Reference"}; 2323 case 1095692943: /*request*/ return new String[] {"Reference"}; 2324 case -1741312354: /*collection*/ return new String[] {}; 2325 case 422194963: /*processing*/ return new String[] {}; 2326 case -410956671: /*container*/ return new String[] {}; 2327 case 3387378: /*note*/ return new String[] {"Annotation"}; 2328 default: return super.getTypesForProperty(hash, name); 2329 } 2330 2331 } 2332 2333 @Override 2334 public Base addChild(String name) throws FHIRException { 2335 if (name.equals("identifier")) { 2336 return addIdentifier(); 2337 } 2338 else if (name.equals("accessionIdentifier")) { 2339 this.accessionIdentifier = new Identifier(); 2340 return this.accessionIdentifier; 2341 } 2342 else if (name.equals("status")) { 2343 throw new FHIRException("Cannot call addChild on a singleton property Specimen.status"); 2344 } 2345 else if (name.equals("type")) { 2346 this.type = new CodeableConcept(); 2347 return this.type; 2348 } 2349 else if (name.equals("subject")) { 2350 this.subject = new Reference(); 2351 return this.subject; 2352 } 2353 else if (name.equals("receivedTime")) { 2354 throw new FHIRException("Cannot call addChild on a singleton property Specimen.receivedTime"); 2355 } 2356 else if (name.equals("parent")) { 2357 return addParent(); 2358 } 2359 else if (name.equals("request")) { 2360 return addRequest(); 2361 } 2362 else if (name.equals("collection")) { 2363 this.collection = new SpecimenCollectionComponent(); 2364 return this.collection; 2365 } 2366 else if (name.equals("processing")) { 2367 return addProcessing(); 2368 } 2369 else if (name.equals("container")) { 2370 return addContainer(); 2371 } 2372 else if (name.equals("note")) { 2373 return addNote(); 2374 } 2375 else 2376 return super.addChild(name); 2377 } 2378 2379 public String fhirType() { 2380 return "Specimen"; 2381 2382 } 2383 2384 public Specimen copy() { 2385 Specimen dst = new Specimen(); 2386 copyValues(dst); 2387 if (identifier != null) { 2388 dst.identifier = new ArrayList<Identifier>(); 2389 for (Identifier i : identifier) 2390 dst.identifier.add(i.copy()); 2391 }; 2392 dst.accessionIdentifier = accessionIdentifier == null ? null : accessionIdentifier.copy(); 2393 dst.status = status == null ? null : status.copy(); 2394 dst.type = type == null ? null : type.copy(); 2395 dst.subject = subject == null ? null : subject.copy(); 2396 dst.receivedTime = receivedTime == null ? null : receivedTime.copy(); 2397 if (parent != null) { 2398 dst.parent = new ArrayList<Reference>(); 2399 for (Reference i : parent) 2400 dst.parent.add(i.copy()); 2401 }; 2402 if (request != null) { 2403 dst.request = new ArrayList<Reference>(); 2404 for (Reference i : request) 2405 dst.request.add(i.copy()); 2406 }; 2407 dst.collection = collection == null ? null : collection.copy(); 2408 if (processing != null) { 2409 dst.processing = new ArrayList<SpecimenProcessingComponent>(); 2410 for (SpecimenProcessingComponent i : processing) 2411 dst.processing.add(i.copy()); 2412 }; 2413 if (container != null) { 2414 dst.container = new ArrayList<SpecimenContainerComponent>(); 2415 for (SpecimenContainerComponent i : container) 2416 dst.container.add(i.copy()); 2417 }; 2418 if (note != null) { 2419 dst.note = new ArrayList<Annotation>(); 2420 for (Annotation i : note) 2421 dst.note.add(i.copy()); 2422 }; 2423 return dst; 2424 } 2425 2426 protected Specimen typedCopy() { 2427 return copy(); 2428 } 2429 2430 @Override 2431 public boolean equalsDeep(Base other_) { 2432 if (!super.equalsDeep(other_)) 2433 return false; 2434 if (!(other_ instanceof Specimen)) 2435 return false; 2436 Specimen o = (Specimen) other_; 2437 return compareDeep(identifier, o.identifier, true) && compareDeep(accessionIdentifier, o.accessionIdentifier, true) 2438 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) 2439 && compareDeep(receivedTime, o.receivedTime, true) && compareDeep(parent, o.parent, true) && compareDeep(request, o.request, true) 2440 && compareDeep(collection, o.collection, true) && compareDeep(processing, o.processing, true) && compareDeep(container, o.container, true) 2441 && compareDeep(note, o.note, true); 2442 } 2443 2444 @Override 2445 public boolean equalsShallow(Base other_) { 2446 if (!super.equalsShallow(other_)) 2447 return false; 2448 if (!(other_ instanceof Specimen)) 2449 return false; 2450 Specimen o = (Specimen) other_; 2451 return compareValues(status, o.status, true) && compareValues(receivedTime, o.receivedTime, true); 2452 } 2453 2454 public boolean isEmpty() { 2455 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, accessionIdentifier 2456 , status, type, subject, receivedTime, parent, request, collection, processing 2457 , container, note); 2458 } 2459 2460 @Override 2461 public ResourceType getResourceType() { 2462 return ResourceType.Specimen; 2463 } 2464 2465 /** 2466 * Search parameter: <b>container</b> 2467 * <p> 2468 * Description: <b>The kind of specimen container</b><br> 2469 * Type: <b>token</b><br> 2470 * Path: <b>Specimen.container.type</b><br> 2471 * </p> 2472 */ 2473 @SearchParamDefinition(name="container", path="Specimen.container.type", description="The kind of specimen container", type="token" ) 2474 public static final String SP_CONTAINER = "container"; 2475 /** 2476 * <b>Fluent Client</b> search parameter constant for <b>container</b> 2477 * <p> 2478 * Description: <b>The kind of specimen container</b><br> 2479 * Type: <b>token</b><br> 2480 * Path: <b>Specimen.container.type</b><br> 2481 * </p> 2482 */ 2483 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTAINER); 2484 2485 /** 2486 * Search parameter: <b>identifier</b> 2487 * <p> 2488 * Description: <b>The unique identifier associated with the specimen</b><br> 2489 * Type: <b>token</b><br> 2490 * Path: <b>Specimen.identifier</b><br> 2491 * </p> 2492 */ 2493 @SearchParamDefinition(name="identifier", path="Specimen.identifier", description="The unique identifier associated with the specimen", type="token" ) 2494 public static final String SP_IDENTIFIER = "identifier"; 2495 /** 2496 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2497 * <p> 2498 * Description: <b>The unique identifier associated with the specimen</b><br> 2499 * Type: <b>token</b><br> 2500 * Path: <b>Specimen.identifier</b><br> 2501 * </p> 2502 */ 2503 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2504 2505 /** 2506 * Search parameter: <b>parent</b> 2507 * <p> 2508 * Description: <b>The parent of the specimen</b><br> 2509 * Type: <b>reference</b><br> 2510 * Path: <b>Specimen.parent</b><br> 2511 * </p> 2512 */ 2513 @SearchParamDefinition(name="parent", path="Specimen.parent", description="The parent of the specimen", type="reference", target={Specimen.class } ) 2514 public static final String SP_PARENT = "parent"; 2515 /** 2516 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 2517 * <p> 2518 * Description: <b>The parent of the specimen</b><br> 2519 * Type: <b>reference</b><br> 2520 * Path: <b>Specimen.parent</b><br> 2521 * </p> 2522 */ 2523 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARENT); 2524 2525/** 2526 * Constant for fluent queries to be used to add include statements. Specifies 2527 * the path value of "<b>Specimen:parent</b>". 2528 */ 2529 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include("Specimen:parent").toLocked(); 2530 2531 /** 2532 * Search parameter: <b>container-id</b> 2533 * <p> 2534 * Description: <b>The unique identifier associated with the specimen container</b><br> 2535 * Type: <b>token</b><br> 2536 * Path: <b>Specimen.container.identifier</b><br> 2537 * </p> 2538 */ 2539 @SearchParamDefinition(name="container-id", path="Specimen.container.identifier", description="The unique identifier associated with the specimen container", type="token" ) 2540 public static final String SP_CONTAINER_ID = "container-id"; 2541 /** 2542 * <b>Fluent Client</b> search parameter constant for <b>container-id</b> 2543 * <p> 2544 * Description: <b>The unique identifier associated with the specimen container</b><br> 2545 * Type: <b>token</b><br> 2546 * Path: <b>Specimen.container.identifier</b><br> 2547 * </p> 2548 */ 2549 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTAINER_ID); 2550 2551 /** 2552 * Search parameter: <b>bodysite</b> 2553 * <p> 2554 * Description: <b>The code for the body site from where the specimen originated</b><br> 2555 * Type: <b>token</b><br> 2556 * Path: <b>Specimen.collection.bodySite</b><br> 2557 * </p> 2558 */ 2559 @SearchParamDefinition(name="bodysite", path="Specimen.collection.bodySite", description="The code for the body site from where the specimen originated", type="token" ) 2560 public static final String SP_BODYSITE = "bodysite"; 2561 /** 2562 * <b>Fluent Client</b> search parameter constant for <b>bodysite</b> 2563 * <p> 2564 * Description: <b>The code for the body site from where the specimen originated</b><br> 2565 * Type: <b>token</b><br> 2566 * Path: <b>Specimen.collection.bodySite</b><br> 2567 * </p> 2568 */ 2569 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODYSITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODYSITE); 2570 2571 /** 2572 * Search parameter: <b>subject</b> 2573 * <p> 2574 * Description: <b>The subject of the specimen</b><br> 2575 * Type: <b>reference</b><br> 2576 * Path: <b>Specimen.subject</b><br> 2577 * </p> 2578 */ 2579 @SearchParamDefinition(name="subject", path="Specimen.subject", description="The subject of the specimen", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Device.class, Group.class, Patient.class, Substance.class } ) 2580 public static final String SP_SUBJECT = "subject"; 2581 /** 2582 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2583 * <p> 2584 * Description: <b>The subject of the specimen</b><br> 2585 * Type: <b>reference</b><br> 2586 * Path: <b>Specimen.subject</b><br> 2587 * </p> 2588 */ 2589 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2590 2591/** 2592 * Constant for fluent queries to be used to add include statements. Specifies 2593 * the path value of "<b>Specimen:subject</b>". 2594 */ 2595 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Specimen:subject").toLocked(); 2596 2597 /** 2598 * Search parameter: <b>patient</b> 2599 * <p> 2600 * Description: <b>The patient the specimen comes from</b><br> 2601 * Type: <b>reference</b><br> 2602 * Path: <b>Specimen.subject</b><br> 2603 * </p> 2604 */ 2605 @SearchParamDefinition(name="patient", path="Specimen.subject", description="The patient the specimen comes from", type="reference", target={Patient.class } ) 2606 public static final String SP_PATIENT = "patient"; 2607 /** 2608 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2609 * <p> 2610 * Description: <b>The patient the specimen comes from</b><br> 2611 * Type: <b>reference</b><br> 2612 * Path: <b>Specimen.subject</b><br> 2613 * </p> 2614 */ 2615 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2616 2617/** 2618 * Constant for fluent queries to be used to add include statements. Specifies 2619 * the path value of "<b>Specimen:patient</b>". 2620 */ 2621 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Specimen:patient").toLocked(); 2622 2623 /** 2624 * Search parameter: <b>collected</b> 2625 * <p> 2626 * Description: <b>The date the specimen was collected</b><br> 2627 * Type: <b>date</b><br> 2628 * Path: <b>Specimen.collection.collected[x]</b><br> 2629 * </p> 2630 */ 2631 @SearchParamDefinition(name="collected", path="Specimen.collection.collected", description="The date the specimen was collected", type="date" ) 2632 public static final String SP_COLLECTED = "collected"; 2633 /** 2634 * <b>Fluent Client</b> search parameter constant for <b>collected</b> 2635 * <p> 2636 * Description: <b>The date the specimen was collected</b><br> 2637 * Type: <b>date</b><br> 2638 * Path: <b>Specimen.collection.collected[x]</b><br> 2639 * </p> 2640 */ 2641 public static final ca.uhn.fhir.rest.gclient.DateClientParam COLLECTED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_COLLECTED); 2642 2643 /** 2644 * Search parameter: <b>accession</b> 2645 * <p> 2646 * Description: <b>The accession number associated with the specimen</b><br> 2647 * Type: <b>token</b><br> 2648 * Path: <b>Specimen.accessionIdentifier</b><br> 2649 * </p> 2650 */ 2651 @SearchParamDefinition(name="accession", path="Specimen.accessionIdentifier", description="The accession number associated with the specimen", type="token" ) 2652 public static final String SP_ACCESSION = "accession"; 2653 /** 2654 * <b>Fluent Client</b> search parameter constant for <b>accession</b> 2655 * <p> 2656 * Description: <b>The accession number associated with the specimen</b><br> 2657 * Type: <b>token</b><br> 2658 * Path: <b>Specimen.accessionIdentifier</b><br> 2659 * </p> 2660 */ 2661 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACCESSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACCESSION); 2662 2663 /** 2664 * Search parameter: <b>type</b> 2665 * <p> 2666 * Description: <b>The specimen type</b><br> 2667 * Type: <b>token</b><br> 2668 * Path: <b>Specimen.type</b><br> 2669 * </p> 2670 */ 2671 @SearchParamDefinition(name="type", path="Specimen.type", description="The specimen type", type="token" ) 2672 public static final String SP_TYPE = "type"; 2673 /** 2674 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2675 * <p> 2676 * Description: <b>The specimen type</b><br> 2677 * Type: <b>token</b><br> 2678 * Path: <b>Specimen.type</b><br> 2679 * </p> 2680 */ 2681 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2682 2683 /** 2684 * Search parameter: <b>collector</b> 2685 * <p> 2686 * Description: <b>Who collected the specimen</b><br> 2687 * Type: <b>reference</b><br> 2688 * Path: <b>Specimen.collection.collector</b><br> 2689 * </p> 2690 */ 2691 @SearchParamDefinition(name="collector", path="Specimen.collection.collector", description="Who collected the specimen", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2692 public static final String SP_COLLECTOR = "collector"; 2693 /** 2694 * <b>Fluent Client</b> search parameter constant for <b>collector</b> 2695 * <p> 2696 * Description: <b>Who collected the specimen</b><br> 2697 * Type: <b>reference</b><br> 2698 * Path: <b>Specimen.collection.collector</b><br> 2699 * </p> 2700 */ 2701 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COLLECTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COLLECTOR); 2702 2703/** 2704 * Constant for fluent queries to be used to add include statements. Specifies 2705 * the path value of "<b>Specimen:collector</b>". 2706 */ 2707 public static final ca.uhn.fhir.model.api.Include INCLUDE_COLLECTOR = new ca.uhn.fhir.model.api.Include("Specimen:collector").toLocked(); 2708 2709 /** 2710 * Search parameter: <b>status</b> 2711 * <p> 2712 * Description: <b>available | unavailable | unsatisfactory | entered-in-error</b><br> 2713 * Type: <b>token</b><br> 2714 * Path: <b>Specimen.status</b><br> 2715 * </p> 2716 */ 2717 @SearchParamDefinition(name="status", path="Specimen.status", description="available | unavailable | unsatisfactory | entered-in-error", type="token" ) 2718 public static final String SP_STATUS = "status"; 2719 /** 2720 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2721 * <p> 2722 * Description: <b>available | unavailable | unsatisfactory | entered-in-error</b><br> 2723 * Type: <b>token</b><br> 2724 * Path: <b>Specimen.status</b><br> 2725 * </p> 2726 */ 2727 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2728 2729 2730}