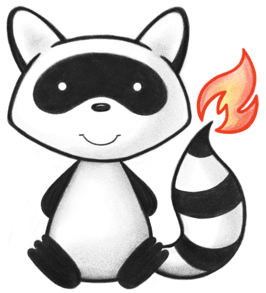
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 041import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.ChildOrder; 049import ca.uhn.fhir.model.api.annotation.Description; 050import ca.uhn.fhir.model.api.annotation.ResourceDef; 051import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 052/** 053 * A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types. 054 */ 055@ResourceDef(name="StructureDefinition", profile="http://hl7.org/fhir/Profile/StructureDefinition") 056@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "keyword", "fhirVersion", "mapping", "kind", "abstract", "contextType", "context", "contextInvariant", "type", "baseDefinition", "derivation", "snapshot", "differential"}) 057public class StructureDefinition extends MetadataResource { 058 059 public enum StructureDefinitionKind { 060 /** 061 * A primitive type that has a value and an extension. These can be used throughout Resource and extension definitions. Only the base specification can define primitive types. 062 */ 063 PRIMITIVETYPE, 064 /** 065 * A complex structure that defines a set of data elements. These can be used throughout Resource and extension definitions, and in logical models. 066 */ 067 COMPLEXTYPE, 068 /** 069 * A resource defined by the FHIR specification. 070 */ 071 RESOURCE, 072 /** 073 * A conceptual package of data that will be mapped to resources for implementation. 074 */ 075 LOGICAL, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 public static StructureDefinitionKind fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("primitive-type".equals(codeString)) 084 return PRIMITIVETYPE; 085 if ("complex-type".equals(codeString)) 086 return COMPLEXTYPE; 087 if ("resource".equals(codeString)) 088 return RESOURCE; 089 if ("logical".equals(codeString)) 090 return LOGICAL; 091 if (Configuration.isAcceptInvalidEnums()) 092 return null; 093 else 094 throw new FHIRException("Unknown StructureDefinitionKind code '"+codeString+"'"); 095 } 096 public String toCode() { 097 switch (this) { 098 case PRIMITIVETYPE: return "primitive-type"; 099 case COMPLEXTYPE: return "complex-type"; 100 case RESOURCE: return "resource"; 101 case LOGICAL: return "logical"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 switch (this) { 108 case PRIMITIVETYPE: return "http://hl7.org/fhir/structure-definition-kind"; 109 case COMPLEXTYPE: return "http://hl7.org/fhir/structure-definition-kind"; 110 case RESOURCE: return "http://hl7.org/fhir/structure-definition-kind"; 111 case LOGICAL: return "http://hl7.org/fhir/structure-definition-kind"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getDefinition() { 117 switch (this) { 118 case PRIMITIVETYPE: return "A primitive type that has a value and an extension. These can be used throughout Resource and extension definitions. Only the base specification can define primitive types."; 119 case COMPLEXTYPE: return "A complex structure that defines a set of data elements. These can be used throughout Resource and extension definitions, and in logical models."; 120 case RESOURCE: return "A resource defined by the FHIR specification."; 121 case LOGICAL: return "A conceptual package of data that will be mapped to resources for implementation."; 122 case NULL: return null; 123 default: return "?"; 124 } 125 } 126 public String getDisplay() { 127 switch (this) { 128 case PRIMITIVETYPE: return "Primitive Data Type"; 129 case COMPLEXTYPE: return "Complex Data Type"; 130 case RESOURCE: return "Resource"; 131 case LOGICAL: return "Logical Model"; 132 case NULL: return null; 133 default: return "?"; 134 } 135 } 136 } 137 138 public static class StructureDefinitionKindEnumFactory implements EnumFactory<StructureDefinitionKind> { 139 public StructureDefinitionKind fromCode(String codeString) throws IllegalArgumentException { 140 if (codeString == null || "".equals(codeString)) 141 if (codeString == null || "".equals(codeString)) 142 return null; 143 if ("primitive-type".equals(codeString)) 144 return StructureDefinitionKind.PRIMITIVETYPE; 145 if ("complex-type".equals(codeString)) 146 return StructureDefinitionKind.COMPLEXTYPE; 147 if ("resource".equals(codeString)) 148 return StructureDefinitionKind.RESOURCE; 149 if ("logical".equals(codeString)) 150 return StructureDefinitionKind.LOGICAL; 151 throw new IllegalArgumentException("Unknown StructureDefinitionKind code '"+codeString+"'"); 152 } 153 public Enumeration<StructureDefinitionKind> fromType(PrimitiveType<?> code) throws FHIRException { 154 if (code == null) 155 return null; 156 if (code.isEmpty()) 157 return new Enumeration<StructureDefinitionKind>(this); 158 String codeString = code.asStringValue(); 159 if (codeString == null || "".equals(codeString)) 160 return null; 161 if ("primitive-type".equals(codeString)) 162 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.PRIMITIVETYPE); 163 if ("complex-type".equals(codeString)) 164 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.COMPLEXTYPE); 165 if ("resource".equals(codeString)) 166 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.RESOURCE); 167 if ("logical".equals(codeString)) 168 return new Enumeration<StructureDefinitionKind>(this, StructureDefinitionKind.LOGICAL); 169 throw new FHIRException("Unknown StructureDefinitionKind code '"+codeString+"'"); 170 } 171 public String toCode(StructureDefinitionKind code) { 172 if (code == StructureDefinitionKind.NULL) 173 return null; 174 if (code == StructureDefinitionKind.PRIMITIVETYPE) 175 return "primitive-type"; 176 if (code == StructureDefinitionKind.COMPLEXTYPE) 177 return "complex-type"; 178 if (code == StructureDefinitionKind.RESOURCE) 179 return "resource"; 180 if (code == StructureDefinitionKind.LOGICAL) 181 return "logical"; 182 return "?"; 183 } 184 public String toSystem(StructureDefinitionKind code) { 185 return code.getSystem(); 186 } 187 } 188 189 public enum ExtensionContext { 190 /** 191 * The context is all elements matching a particular resource element path. 192 */ 193 RESOURCE, 194 /** 195 * The context is all nodes matching a particular data type element path (root or repeating element) or all elements referencing a particular primitive data type (expressed as the datatype name). 196 */ 197 DATATYPE, 198 /** 199 * The context is a particular extension from a particular profile, a uri that identifies the extension definition. 200 */ 201 EXTENSION, 202 /** 203 * added to help the parsers with the generic types 204 */ 205 NULL; 206 public static ExtensionContext fromCode(String codeString) throws FHIRException { 207 if (codeString == null || "".equals(codeString)) 208 return null; 209 if ("resource".equals(codeString)) 210 return RESOURCE; 211 if ("datatype".equals(codeString)) 212 return DATATYPE; 213 if ("extension".equals(codeString)) 214 return EXTENSION; 215 if (Configuration.isAcceptInvalidEnums()) 216 return null; 217 else 218 throw new FHIRException("Unknown ExtensionContext code '"+codeString+"'"); 219 } 220 public String toCode() { 221 switch (this) { 222 case RESOURCE: return "resource"; 223 case DATATYPE: return "datatype"; 224 case EXTENSION: return "extension"; 225 case NULL: return null; 226 default: return "?"; 227 } 228 } 229 public String getSystem() { 230 switch (this) { 231 case RESOURCE: return "http://hl7.org/fhir/extension-context"; 232 case DATATYPE: return "http://hl7.org/fhir/extension-context"; 233 case EXTENSION: return "http://hl7.org/fhir/extension-context"; 234 case NULL: return null; 235 default: return "?"; 236 } 237 } 238 public String getDefinition() { 239 switch (this) { 240 case RESOURCE: return "The context is all elements matching a particular resource element path."; 241 case DATATYPE: return "The context is all nodes matching a particular data type element path (root or repeating element) or all elements referencing a particular primitive data type (expressed as the datatype name)."; 242 case EXTENSION: return "The context is a particular extension from a particular profile, a uri that identifies the extension definition."; 243 case NULL: return null; 244 default: return "?"; 245 } 246 } 247 public String getDisplay() { 248 switch (this) { 249 case RESOURCE: return "Resource"; 250 case DATATYPE: return "Datatype"; 251 case EXTENSION: return "Extension"; 252 case NULL: return null; 253 default: return "?"; 254 } 255 } 256 } 257 258 public static class ExtensionContextEnumFactory implements EnumFactory<ExtensionContext> { 259 public ExtensionContext fromCode(String codeString) throws IllegalArgumentException { 260 if (codeString == null || "".equals(codeString)) 261 if (codeString == null || "".equals(codeString)) 262 return null; 263 if ("resource".equals(codeString)) 264 return ExtensionContext.RESOURCE; 265 if ("datatype".equals(codeString)) 266 return ExtensionContext.DATATYPE; 267 if ("extension".equals(codeString)) 268 return ExtensionContext.EXTENSION; 269 throw new IllegalArgumentException("Unknown ExtensionContext code '"+codeString+"'"); 270 } 271 public Enumeration<ExtensionContext> fromType(PrimitiveType<?> code) throws FHIRException { 272 if (code == null) 273 return null; 274 if (code.isEmpty()) 275 return new Enumeration<ExtensionContext>(this); 276 String codeString = code.asStringValue(); 277 if (codeString == null || "".equals(codeString)) 278 return null; 279 if ("resource".equals(codeString)) 280 return new Enumeration<ExtensionContext>(this, ExtensionContext.RESOURCE); 281 if ("datatype".equals(codeString)) 282 return new Enumeration<ExtensionContext>(this, ExtensionContext.DATATYPE); 283 if ("extension".equals(codeString)) 284 return new Enumeration<ExtensionContext>(this, ExtensionContext.EXTENSION); 285 throw new FHIRException("Unknown ExtensionContext code '"+codeString+"'"); 286 } 287 public String toCode(ExtensionContext code) { 288 if (code == ExtensionContext.NULL) 289 return null; 290 if (code == ExtensionContext.RESOURCE) 291 return "resource"; 292 if (code == ExtensionContext.DATATYPE) 293 return "datatype"; 294 if (code == ExtensionContext.EXTENSION) 295 return "extension"; 296 return "?"; 297 } 298 public String toSystem(ExtensionContext code) { 299 return code.getSystem(); 300 } 301 } 302 303 public enum TypeDerivationRule { 304 /** 305 * This definition defines a new type that adds additional elements to the base type 306 */ 307 SPECIALIZATION, 308 /** 309 * This definition adds additional rules to an existing concrete type 310 */ 311 CONSTRAINT, 312 /** 313 * added to help the parsers with the generic types 314 */ 315 NULL; 316 public static TypeDerivationRule fromCode(String codeString) throws FHIRException { 317 if (codeString == null || "".equals(codeString)) 318 return null; 319 if ("specialization".equals(codeString)) 320 return SPECIALIZATION; 321 if ("constraint".equals(codeString)) 322 return CONSTRAINT; 323 if (Configuration.isAcceptInvalidEnums()) 324 return null; 325 else 326 throw new FHIRException("Unknown TypeDerivationRule code '"+codeString+"'"); 327 } 328 public String toCode() { 329 switch (this) { 330 case SPECIALIZATION: return "specialization"; 331 case CONSTRAINT: return "constraint"; 332 case NULL: return null; 333 default: return "?"; 334 } 335 } 336 public String getSystem() { 337 switch (this) { 338 case SPECIALIZATION: return "http://hl7.org/fhir/type-derivation-rule"; 339 case CONSTRAINT: return "http://hl7.org/fhir/type-derivation-rule"; 340 case NULL: return null; 341 default: return "?"; 342 } 343 } 344 public String getDefinition() { 345 switch (this) { 346 case SPECIALIZATION: return "This definition defines a new type that adds additional elements to the base type"; 347 case CONSTRAINT: return "This definition adds additional rules to an existing concrete type"; 348 case NULL: return null; 349 default: return "?"; 350 } 351 } 352 public String getDisplay() { 353 switch (this) { 354 case SPECIALIZATION: return "Specialization"; 355 case CONSTRAINT: return "Constraint"; 356 case NULL: return null; 357 default: return "?"; 358 } 359 } 360 } 361 362 public static class TypeDerivationRuleEnumFactory implements EnumFactory<TypeDerivationRule> { 363 public TypeDerivationRule fromCode(String codeString) throws IllegalArgumentException { 364 if (codeString == null || "".equals(codeString)) 365 if (codeString == null || "".equals(codeString)) 366 return null; 367 if ("specialization".equals(codeString)) 368 return TypeDerivationRule.SPECIALIZATION; 369 if ("constraint".equals(codeString)) 370 return TypeDerivationRule.CONSTRAINT; 371 throw new IllegalArgumentException("Unknown TypeDerivationRule code '"+codeString+"'"); 372 } 373 public Enumeration<TypeDerivationRule> fromType(PrimitiveType<?> code) throws FHIRException { 374 if (code == null) 375 return null; 376 if (code.isEmpty()) 377 return new Enumeration<TypeDerivationRule>(this); 378 String codeString = code.asStringValue(); 379 if (codeString == null || "".equals(codeString)) 380 return null; 381 if ("specialization".equals(codeString)) 382 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.SPECIALIZATION); 383 if ("constraint".equals(codeString)) 384 return new Enumeration<TypeDerivationRule>(this, TypeDerivationRule.CONSTRAINT); 385 throw new FHIRException("Unknown TypeDerivationRule code '"+codeString+"'"); 386 } 387 public String toCode(TypeDerivationRule code) { 388 if (code == TypeDerivationRule.NULL) 389 return null; 390 if (code == TypeDerivationRule.SPECIALIZATION) 391 return "specialization"; 392 if (code == TypeDerivationRule.CONSTRAINT) 393 return "constraint"; 394 return "?"; 395 } 396 public String toSystem(TypeDerivationRule code) { 397 return code.getSystem(); 398 } 399 } 400 401 @Block() 402 public static class StructureDefinitionMappingComponent extends BackboneElement implements IBaseBackboneElement { 403 /** 404 * An Internal id that is used to identify this mapping set when specific mappings are made. 405 */ 406 @Child(name = "identity", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 407 @Description(shortDefinition="Internal id when this mapping is used", formalDefinition="An Internal id that is used to identify this mapping set when specific mappings are made." ) 408 protected IdType identity; 409 410 /** 411 * An absolute URI that identifies the specification that this mapping is expressed to. 412 */ 413 @Child(name = "uri", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 414 @Description(shortDefinition="Identifies what this mapping refers to", formalDefinition="An absolute URI that identifies the specification that this mapping is expressed to." ) 415 protected UriType uri; 416 417 /** 418 * A name for the specification that is being mapped to. 419 */ 420 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 421 @Description(shortDefinition="Names what this mapping refers to", formalDefinition="A name for the specification that is being mapped to." ) 422 protected StringType name; 423 424 /** 425 * Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 426 */ 427 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 428 @Description(shortDefinition="Versions, Issues, Scope limitations etc.", formalDefinition="Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage." ) 429 protected StringType comment; 430 431 private static final long serialVersionUID = 9610265L; 432 433 /** 434 * Constructor 435 */ 436 public StructureDefinitionMappingComponent() { 437 super(); 438 } 439 440 /** 441 * Constructor 442 */ 443 public StructureDefinitionMappingComponent(IdType identity) { 444 super(); 445 this.identity = identity; 446 } 447 448 /** 449 * @return {@link #identity} (An Internal id that is used to identify this mapping set when specific mappings are made.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 450 */ 451 public IdType getIdentityElement() { 452 if (this.identity == null) 453 if (Configuration.errorOnAutoCreate()) 454 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.identity"); 455 else if (Configuration.doAutoCreate()) 456 this.identity = new IdType(); // bb 457 return this.identity; 458 } 459 460 public boolean hasIdentityElement() { 461 return this.identity != null && !this.identity.isEmpty(); 462 } 463 464 public boolean hasIdentity() { 465 return this.identity != null && !this.identity.isEmpty(); 466 } 467 468 /** 469 * @param value {@link #identity} (An Internal id that is used to identify this mapping set when specific mappings are made.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 470 */ 471 public StructureDefinitionMappingComponent setIdentityElement(IdType value) { 472 this.identity = value; 473 return this; 474 } 475 476 /** 477 * @return An Internal id that is used to identify this mapping set when specific mappings are made. 478 */ 479 public String getIdentity() { 480 return this.identity == null ? null : this.identity.getValue(); 481 } 482 483 /** 484 * @param value An Internal id that is used to identify this mapping set when specific mappings are made. 485 */ 486 public StructureDefinitionMappingComponent setIdentity(String value) { 487 if (this.identity == null) 488 this.identity = new IdType(); 489 this.identity.setValue(value); 490 return this; 491 } 492 493 /** 494 * @return {@link #uri} (An absolute URI that identifies the specification that this mapping is expressed to.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 495 */ 496 public UriType getUriElement() { 497 if (this.uri == null) 498 if (Configuration.errorOnAutoCreate()) 499 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.uri"); 500 else if (Configuration.doAutoCreate()) 501 this.uri = new UriType(); // bb 502 return this.uri; 503 } 504 505 public boolean hasUriElement() { 506 return this.uri != null && !this.uri.isEmpty(); 507 } 508 509 public boolean hasUri() { 510 return this.uri != null && !this.uri.isEmpty(); 511 } 512 513 /** 514 * @param value {@link #uri} (An absolute URI that identifies the specification that this mapping is expressed to.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 515 */ 516 public StructureDefinitionMappingComponent setUriElement(UriType value) { 517 this.uri = value; 518 return this; 519 } 520 521 /** 522 * @return An absolute URI that identifies the specification that this mapping is expressed to. 523 */ 524 public String getUri() { 525 return this.uri == null ? null : this.uri.getValue(); 526 } 527 528 /** 529 * @param value An absolute URI that identifies the specification that this mapping is expressed to. 530 */ 531 public StructureDefinitionMappingComponent setUri(String value) { 532 if (Utilities.noString(value)) 533 this.uri = null; 534 else { 535 if (this.uri == null) 536 this.uri = new UriType(); 537 this.uri.setValue(value); 538 } 539 return this; 540 } 541 542 /** 543 * @return {@link #name} (A name for the specification that is being mapped to.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 544 */ 545 public StringType getNameElement() { 546 if (this.name == null) 547 if (Configuration.errorOnAutoCreate()) 548 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.name"); 549 else if (Configuration.doAutoCreate()) 550 this.name = new StringType(); // bb 551 return this.name; 552 } 553 554 public boolean hasNameElement() { 555 return this.name != null && !this.name.isEmpty(); 556 } 557 558 public boolean hasName() { 559 return this.name != null && !this.name.isEmpty(); 560 } 561 562 /** 563 * @param value {@link #name} (A name for the specification that is being mapped to.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 564 */ 565 public StructureDefinitionMappingComponent setNameElement(StringType value) { 566 this.name = value; 567 return this; 568 } 569 570 /** 571 * @return A name for the specification that is being mapped to. 572 */ 573 public String getName() { 574 return this.name == null ? null : this.name.getValue(); 575 } 576 577 /** 578 * @param value A name for the specification that is being mapped to. 579 */ 580 public StructureDefinitionMappingComponent setName(String value) { 581 if (Utilities.noString(value)) 582 this.name = null; 583 else { 584 if (this.name == null) 585 this.name = new StringType(); 586 this.name.setValue(value); 587 } 588 return this; 589 } 590 591 /** 592 * @return {@link #comment} (Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 593 */ 594 public StringType getCommentElement() { 595 if (this.comment == null) 596 if (Configuration.errorOnAutoCreate()) 597 throw new Error("Attempt to auto-create StructureDefinitionMappingComponent.comment"); 598 else if (Configuration.doAutoCreate()) 599 this.comment = new StringType(); // bb 600 return this.comment; 601 } 602 603 public boolean hasCommentElement() { 604 return this.comment != null && !this.comment.isEmpty(); 605 } 606 607 public boolean hasComment() { 608 return this.comment != null && !this.comment.isEmpty(); 609 } 610 611 /** 612 * @param value {@link #comment} (Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 613 */ 614 public StructureDefinitionMappingComponent setCommentElement(StringType value) { 615 this.comment = value; 616 return this; 617 } 618 619 /** 620 * @return Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 621 */ 622 public String getComment() { 623 return this.comment == null ? null : this.comment.getValue(); 624 } 625 626 /** 627 * @param value Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage. 628 */ 629 public StructureDefinitionMappingComponent setComment(String value) { 630 if (Utilities.noString(value)) 631 this.comment = null; 632 else { 633 if (this.comment == null) 634 this.comment = new StringType(); 635 this.comment.setValue(value); 636 } 637 return this; 638 } 639 640 protected void listChildren(List<Property> children) { 641 super.listChildren(children); 642 children.add(new Property("identity", "id", "An Internal id that is used to identify this mapping set when specific mappings are made.", 0, 1, identity)); 643 children.add(new Property("uri", "uri", "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 1, uri)); 644 children.add(new Property("name", "string", "A name for the specification that is being mapped to.", 0, 1, name)); 645 children.add(new Property("comment", "string", "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 0, 1, comment)); 646 } 647 648 @Override 649 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 650 switch (_hash) { 651 case -135761730: /*identity*/ return new Property("identity", "id", "An Internal id that is used to identify this mapping set when specific mappings are made.", 0, 1, identity); 652 case 116076: /*uri*/ return new Property("uri", "uri", "An absolute URI that identifies the specification that this mapping is expressed to.", 0, 1, uri); 653 case 3373707: /*name*/ return new Property("name", "string", "A name for the specification that is being mapped to.", 0, 1, name); 654 case 950398559: /*comment*/ return new Property("comment", "string", "Comments about this mapping, including version notes, issues, scope limitations, and other important notes for usage.", 0, 1, comment); 655 default: return super.getNamedProperty(_hash, _name, _checkValid); 656 } 657 658 } 659 660 @Override 661 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 662 switch (hash) { 663 case -135761730: /*identity*/ return this.identity == null ? new Base[0] : new Base[] {this.identity}; // IdType 664 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 665 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 666 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 667 default: return super.getProperty(hash, name, checkValid); 668 } 669 670 } 671 672 @Override 673 public Base setProperty(int hash, String name, Base value) throws FHIRException { 674 switch (hash) { 675 case -135761730: // identity 676 this.identity = castToId(value); // IdType 677 return value; 678 case 116076: // uri 679 this.uri = castToUri(value); // UriType 680 return value; 681 case 3373707: // name 682 this.name = castToString(value); // StringType 683 return value; 684 case 950398559: // comment 685 this.comment = castToString(value); // StringType 686 return value; 687 default: return super.setProperty(hash, name, value); 688 } 689 690 } 691 692 @Override 693 public Base setProperty(String name, Base value) throws FHIRException { 694 if (name.equals("identity")) { 695 this.identity = castToId(value); // IdType 696 } else if (name.equals("uri")) { 697 this.uri = castToUri(value); // UriType 698 } else if (name.equals("name")) { 699 this.name = castToString(value); // StringType 700 } else if (name.equals("comment")) { 701 this.comment = castToString(value); // StringType 702 } else 703 return super.setProperty(name, value); 704 return value; 705 } 706 707 @Override 708 public Base makeProperty(int hash, String name) throws FHIRException { 709 switch (hash) { 710 case -135761730: return getIdentityElement(); 711 case 116076: return getUriElement(); 712 case 3373707: return getNameElement(); 713 case 950398559: return getCommentElement(); 714 default: return super.makeProperty(hash, name); 715 } 716 717 } 718 719 @Override 720 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 721 switch (hash) { 722 case -135761730: /*identity*/ return new String[] {"id"}; 723 case 116076: /*uri*/ return new String[] {"uri"}; 724 case 3373707: /*name*/ return new String[] {"string"}; 725 case 950398559: /*comment*/ return new String[] {"string"}; 726 default: return super.getTypesForProperty(hash, name); 727 } 728 729 } 730 731 @Override 732 public Base addChild(String name) throws FHIRException { 733 if (name.equals("identity")) { 734 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.identity"); 735 } 736 else if (name.equals("uri")) { 737 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.uri"); 738 } 739 else if (name.equals("name")) { 740 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.name"); 741 } 742 else if (name.equals("comment")) { 743 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.comment"); 744 } 745 else 746 return super.addChild(name); 747 } 748 749 public StructureDefinitionMappingComponent copy() { 750 StructureDefinitionMappingComponent dst = new StructureDefinitionMappingComponent(); 751 copyValues(dst); 752 dst.identity = identity == null ? null : identity.copy(); 753 dst.uri = uri == null ? null : uri.copy(); 754 dst.name = name == null ? null : name.copy(); 755 dst.comment = comment == null ? null : comment.copy(); 756 return dst; 757 } 758 759 @Override 760 public boolean equalsDeep(Base other_) { 761 if (!super.equalsDeep(other_)) 762 return false; 763 if (!(other_ instanceof StructureDefinitionMappingComponent)) 764 return false; 765 StructureDefinitionMappingComponent o = (StructureDefinitionMappingComponent) other_; 766 return compareDeep(identity, o.identity, true) && compareDeep(uri, o.uri, true) && compareDeep(name, o.name, true) 767 && compareDeep(comment, o.comment, true); 768 } 769 770 @Override 771 public boolean equalsShallow(Base other_) { 772 if (!super.equalsShallow(other_)) 773 return false; 774 if (!(other_ instanceof StructureDefinitionMappingComponent)) 775 return false; 776 StructureDefinitionMappingComponent o = (StructureDefinitionMappingComponent) other_; 777 return compareValues(identity, o.identity, true) && compareValues(uri, o.uri, true) && compareValues(name, o.name, true) 778 && compareValues(comment, o.comment, true); 779 } 780 781 public boolean isEmpty() { 782 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identity, uri, name, comment 783 ); 784 } 785 786 public String fhirType() { 787 return "StructureDefinition.mapping"; 788 789 } 790 791 } 792 793 @Block() 794 public static class StructureDefinitionSnapshotComponent extends BackboneElement implements IBaseBackboneElement { 795 /** 796 * Captures constraints on each element within the resource. 797 */ 798 @Child(name = "element", type = {ElementDefinition.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 799 @Description(shortDefinition="Definition of elements in the resource (if no StructureDefinition)", formalDefinition="Captures constraints on each element within the resource." ) 800 protected List<ElementDefinition> element; 801 802 private static final long serialVersionUID = 53896641L; 803 804 /** 805 * Constructor 806 */ 807 public StructureDefinitionSnapshotComponent() { 808 super(); 809 } 810 811 /** 812 * @return {@link #element} (Captures constraints on each element within the resource.) 813 */ 814 public List<ElementDefinition> getElement() { 815 if (this.element == null) 816 this.element = new ArrayList<ElementDefinition>(); 817 return this.element; 818 } 819 820 /** 821 * @return Returns a reference to <code>this</code> for easy method chaining 822 */ 823 public StructureDefinitionSnapshotComponent setElement(List<ElementDefinition> theElement) { 824 this.element = theElement; 825 return this; 826 } 827 828 public boolean hasElement() { 829 if (this.element == null) 830 return false; 831 for (ElementDefinition item : this.element) 832 if (!item.isEmpty()) 833 return true; 834 return false; 835 } 836 837 public ElementDefinition addElement() { //3 838 ElementDefinition t = new ElementDefinition(); 839 if (this.element == null) 840 this.element = new ArrayList<ElementDefinition>(); 841 this.element.add(t); 842 return t; 843 } 844 845 public StructureDefinitionSnapshotComponent addElement(ElementDefinition t) { //3 846 if (t == null) 847 return this; 848 if (this.element == null) 849 this.element = new ArrayList<ElementDefinition>(); 850 this.element.add(t); 851 return this; 852 } 853 854 /** 855 * @return The first repetition of repeating field {@link #element}, creating it if it does not already exist 856 */ 857 public ElementDefinition getElementFirstRep() { 858 if (getElement().isEmpty()) { 859 addElement(); 860 } 861 return getElement().get(0); 862 } 863 864 protected void listChildren(List<Property> children) { 865 super.listChildren(children); 866 children.add(new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element)); 867 } 868 869 @Override 870 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 871 switch (_hash) { 872 case -1662836996: /*element*/ return new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element); 873 default: return super.getNamedProperty(_hash, _name, _checkValid); 874 } 875 876 } 877 878 @Override 879 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 880 switch (hash) { 881 case -1662836996: /*element*/ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // ElementDefinition 882 default: return super.getProperty(hash, name, checkValid); 883 } 884 885 } 886 887 @Override 888 public Base setProperty(int hash, String name, Base value) throws FHIRException { 889 switch (hash) { 890 case -1662836996: // element 891 this.getElement().add(castToElementDefinition(value)); // ElementDefinition 892 return value; 893 default: return super.setProperty(hash, name, value); 894 } 895 896 } 897 898 @Override 899 public Base setProperty(String name, Base value) throws FHIRException { 900 if (name.equals("element")) { 901 this.getElement().add(castToElementDefinition(value)); 902 } else 903 return super.setProperty(name, value); 904 return value; 905 } 906 907 @Override 908 public Base makeProperty(int hash, String name) throws FHIRException { 909 switch (hash) { 910 case -1662836996: return addElement(); 911 default: return super.makeProperty(hash, name); 912 } 913 914 } 915 916 @Override 917 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 918 switch (hash) { 919 case -1662836996: /*element*/ return new String[] {"ElementDefinition"}; 920 default: return super.getTypesForProperty(hash, name); 921 } 922 923 } 924 925 @Override 926 public Base addChild(String name) throws FHIRException { 927 if (name.equals("element")) { 928 return addElement(); 929 } 930 else 931 return super.addChild(name); 932 } 933 934 public StructureDefinitionSnapshotComponent copy() { 935 StructureDefinitionSnapshotComponent dst = new StructureDefinitionSnapshotComponent(); 936 copyValues(dst); 937 if (element != null) { 938 dst.element = new ArrayList<ElementDefinition>(); 939 for (ElementDefinition i : element) 940 dst.element.add(i.copy()); 941 }; 942 return dst; 943 } 944 945 @Override 946 public boolean equalsDeep(Base other_) { 947 if (!super.equalsDeep(other_)) 948 return false; 949 if (!(other_ instanceof StructureDefinitionSnapshotComponent)) 950 return false; 951 StructureDefinitionSnapshotComponent o = (StructureDefinitionSnapshotComponent) other_; 952 return compareDeep(element, o.element, true); 953 } 954 955 @Override 956 public boolean equalsShallow(Base other_) { 957 if (!super.equalsShallow(other_)) 958 return false; 959 if (!(other_ instanceof StructureDefinitionSnapshotComponent)) 960 return false; 961 StructureDefinitionSnapshotComponent o = (StructureDefinitionSnapshotComponent) other_; 962 return true; 963 } 964 965 public boolean isEmpty() { 966 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(element); 967 } 968 969 public String fhirType() { 970 return "StructureDefinition.snapshot"; 971 972 } 973 974 } 975 976 @Block() 977 public static class StructureDefinitionDifferentialComponent extends BackboneElement implements IBaseBackboneElement { 978 /** 979 * Captures constraints on each element within the resource. 980 */ 981 @Child(name = "element", type = {ElementDefinition.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 982 @Description(shortDefinition="Definition of elements in the resource (if no StructureDefinition)", formalDefinition="Captures constraints on each element within the resource." ) 983 protected List<ElementDefinition> element; 984 985 private static final long serialVersionUID = 53896641L; 986 987 /** 988 * Constructor 989 */ 990 public StructureDefinitionDifferentialComponent() { 991 super(); 992 } 993 994 /** 995 * @return {@link #element} (Captures constraints on each element within the resource.) 996 */ 997 public List<ElementDefinition> getElement() { 998 if (this.element == null) 999 this.element = new ArrayList<ElementDefinition>(); 1000 return this.element; 1001 } 1002 1003 /** 1004 * @return Returns a reference to <code>this</code> for easy method chaining 1005 */ 1006 public StructureDefinitionDifferentialComponent setElement(List<ElementDefinition> theElement) { 1007 this.element = theElement; 1008 return this; 1009 } 1010 1011 public boolean hasElement() { 1012 if (this.element == null) 1013 return false; 1014 for (ElementDefinition item : this.element) 1015 if (!item.isEmpty()) 1016 return true; 1017 return false; 1018 } 1019 1020 public ElementDefinition addElement() { //3 1021 ElementDefinition t = new ElementDefinition(); 1022 if (this.element == null) 1023 this.element = new ArrayList<ElementDefinition>(); 1024 this.element.add(t); 1025 return t; 1026 } 1027 1028 public StructureDefinitionDifferentialComponent addElement(ElementDefinition t) { //3 1029 if (t == null) 1030 return this; 1031 if (this.element == null) 1032 this.element = new ArrayList<ElementDefinition>(); 1033 this.element.add(t); 1034 return this; 1035 } 1036 1037 /** 1038 * @return The first repetition of repeating field {@link #element}, creating it if it does not already exist 1039 */ 1040 public ElementDefinition getElementFirstRep() { 1041 if (getElement().isEmpty()) { 1042 addElement(); 1043 } 1044 return getElement().get(0); 1045 } 1046 1047 protected void listChildren(List<Property> children) { 1048 super.listChildren(children); 1049 children.add(new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element)); 1050 } 1051 1052 @Override 1053 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1054 switch (_hash) { 1055 case -1662836996: /*element*/ return new Property("element", "ElementDefinition", "Captures constraints on each element within the resource.", 0, java.lang.Integer.MAX_VALUE, element); 1056 default: return super.getNamedProperty(_hash, _name, _checkValid); 1057 } 1058 1059 } 1060 1061 @Override 1062 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1063 switch (hash) { 1064 case -1662836996: /*element*/ return this.element == null ? new Base[0] : this.element.toArray(new Base[this.element.size()]); // ElementDefinition 1065 default: return super.getProperty(hash, name, checkValid); 1066 } 1067 1068 } 1069 1070 @Override 1071 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1072 switch (hash) { 1073 case -1662836996: // element 1074 this.getElement().add(castToElementDefinition(value)); // ElementDefinition 1075 return value; 1076 default: return super.setProperty(hash, name, value); 1077 } 1078 1079 } 1080 1081 @Override 1082 public Base setProperty(String name, Base value) throws FHIRException { 1083 if (name.equals("element")) { 1084 this.getElement().add(castToElementDefinition(value)); 1085 } else 1086 return super.setProperty(name, value); 1087 return value; 1088 } 1089 1090 @Override 1091 public Base makeProperty(int hash, String name) throws FHIRException { 1092 switch (hash) { 1093 case -1662836996: return addElement(); 1094 default: return super.makeProperty(hash, name); 1095 } 1096 1097 } 1098 1099 @Override 1100 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1101 switch (hash) { 1102 case -1662836996: /*element*/ return new String[] {"ElementDefinition"}; 1103 default: return super.getTypesForProperty(hash, name); 1104 } 1105 1106 } 1107 1108 @Override 1109 public Base addChild(String name) throws FHIRException { 1110 if (name.equals("element")) { 1111 return addElement(); 1112 } 1113 else 1114 return super.addChild(name); 1115 } 1116 1117 public StructureDefinitionDifferentialComponent copy() { 1118 StructureDefinitionDifferentialComponent dst = new StructureDefinitionDifferentialComponent(); 1119 copyValues(dst); 1120 if (element != null) { 1121 dst.element = new ArrayList<ElementDefinition>(); 1122 for (ElementDefinition i : element) 1123 dst.element.add(i.copy()); 1124 }; 1125 return dst; 1126 } 1127 1128 @Override 1129 public boolean equalsDeep(Base other_) { 1130 if (!super.equalsDeep(other_)) 1131 return false; 1132 if (!(other_ instanceof StructureDefinitionDifferentialComponent)) 1133 return false; 1134 StructureDefinitionDifferentialComponent o = (StructureDefinitionDifferentialComponent) other_; 1135 return compareDeep(element, o.element, true); 1136 } 1137 1138 @Override 1139 public boolean equalsShallow(Base other_) { 1140 if (!super.equalsShallow(other_)) 1141 return false; 1142 if (!(other_ instanceof StructureDefinitionDifferentialComponent)) 1143 return false; 1144 StructureDefinitionDifferentialComponent o = (StructureDefinitionDifferentialComponent) other_; 1145 return true; 1146 } 1147 1148 public boolean isEmpty() { 1149 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(element); 1150 } 1151 1152 public String fhirType() { 1153 return "StructureDefinition.differential"; 1154 1155 } 1156 1157 } 1158 1159 /** 1160 * A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 1161 */ 1162 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1163 @Description(shortDefinition="Additional identifier for the structure definition", formalDefinition="A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1164 protected List<Identifier> identifier; 1165 1166 /** 1167 * Explaination of why this structure definition is needed and why it has been designed as it has. 1168 */ 1169 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1170 @Description(shortDefinition="Why this structure definition is defined", formalDefinition="Explaination of why this structure definition is needed and why it has been designed as it has." ) 1171 protected MarkdownType purpose; 1172 1173 /** 1174 * A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. 1175 */ 1176 @Child(name = "copyright", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1177 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition." ) 1178 protected MarkdownType copyright; 1179 1180 /** 1181 * A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates. 1182 */ 1183 @Child(name = "keyword", type = {Coding.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1184 @Description(shortDefinition="Assist with indexing and finding", formalDefinition="A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates." ) 1185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/profile-code") 1186 protected List<Coding> keyword; 1187 1188 /** 1189 * The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version. 1190 */ 1191 @Child(name = "fhirVersion", type = {IdType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1192 @Description(shortDefinition="FHIR Version this StructureDefinition targets", formalDefinition="The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version." ) 1193 protected IdType fhirVersion; 1194 1195 /** 1196 * An external specification that the content is mapped to. 1197 */ 1198 @Child(name = "mapping", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1199 @Description(shortDefinition="External specification that the content is mapped to", formalDefinition="An external specification that the content is mapped to." ) 1200 protected List<StructureDefinitionMappingComponent> mapping; 1201 1202 /** 1203 * Defines the kind of structure that this definition is describing. 1204 */ 1205 @Child(name = "kind", type = {CodeType.class}, order=6, min=1, max=1, modifier=false, summary=true) 1206 @Description(shortDefinition="primitive-type | complex-type | resource | logical", formalDefinition="Defines the kind of structure that this definition is describing." ) 1207 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/structure-definition-kind") 1208 protected Enumeration<StructureDefinitionKind> kind; 1209 1210 /** 1211 * Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems. 1212 */ 1213 @Child(name = "abstract", type = {BooleanType.class}, order=7, min=1, max=1, modifier=false, summary=true) 1214 @Description(shortDefinition="Whether the structure is abstract", formalDefinition="Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems." ) 1215 protected BooleanType abstract_; 1216 1217 /** 1218 * If this is an extension, Identifies the context within FHIR resources where the extension can be used. 1219 */ 1220 @Child(name = "contextType", type = {CodeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1221 @Description(shortDefinition="resource | datatype | extension", formalDefinition="If this is an extension, Identifies the context within FHIR resources where the extension can be used." ) 1222 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/extension-context") 1223 protected Enumeration<ExtensionContext> contextType; 1224 1225 /** 1226 * Identifies the types of resource or data type elements to which the extension can be applied. 1227 */ 1228 @Child(name = "context", type = {StringType.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1229 @Description(shortDefinition="Where the extension can be used in instances", formalDefinition="Identifies the types of resource or data type elements to which the extension can be applied." ) 1230 protected List<StringType> context; 1231 1232 /** 1233 * A set of rules as Fluent Invariants about when the extension can be used (e.g. co-occurrence variants for the extension). 1234 */ 1235 @Child(name = "contextInvariant", type = {StringType.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1236 @Description(shortDefinition="FHIRPath invariants - when the extension can be used", formalDefinition="A set of rules as Fluent Invariants about when the extension can be used (e.g. co-occurrence variants for the extension)." ) 1237 protected List<StringType> contextInvariant; 1238 1239 /** 1240 * The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). 1241 */ 1242 @Child(name = "type", type = {CodeType.class}, order=11, min=1, max=1, modifier=false, summary=true) 1243 @Description(shortDefinition="Type defined or constrained by this structure", formalDefinition="The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type)." ) 1244 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/defined-types") 1245 protected CodeType type; 1246 1247 /** 1248 * An absolute URI that is the base structure from which this type is derived, either by specialization or constraint. 1249 */ 1250 @Child(name = "baseDefinition", type = {UriType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1251 @Description(shortDefinition="Definition that this type is constrained/specialized from", formalDefinition="An absolute URI that is the base structure from which this type is derived, either by specialization or constraint." ) 1252 protected UriType baseDefinition; 1253 1254 /** 1255 * How the type relates to the baseDefinition. 1256 */ 1257 @Child(name = "derivation", type = {CodeType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1258 @Description(shortDefinition="specialization | constraint - How relates to base definition", formalDefinition="How the type relates to the baseDefinition." ) 1259 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/type-derivation-rule") 1260 protected Enumeration<TypeDerivationRule> derivation; 1261 1262 /** 1263 * A snapshot view is expressed in a stand alone form that can be used and interpreted without considering the base StructureDefinition. 1264 */ 1265 @Child(name = "snapshot", type = {}, order=14, min=0, max=1, modifier=false, summary=false) 1266 @Description(shortDefinition="Snapshot view of the structure", formalDefinition="A snapshot view is expressed in a stand alone form that can be used and interpreted without considering the base StructureDefinition." ) 1267 protected StructureDefinitionSnapshotComponent snapshot; 1268 1269 /** 1270 * A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies. 1271 */ 1272 @Child(name = "differential", type = {}, order=15, min=0, max=1, modifier=false, summary=false) 1273 @Description(shortDefinition="Differential view of the structure", formalDefinition="A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies." ) 1274 protected StructureDefinitionDifferentialComponent differential; 1275 1276 private static final long serialVersionUID = -1511739381L; 1277 1278 /** 1279 * Constructor 1280 */ 1281 public StructureDefinition() { 1282 super(); 1283 } 1284 1285 /** 1286 * Constructor 1287 */ 1288 public StructureDefinition(UriType url, StringType name, Enumeration<PublicationStatus> status, Enumeration<StructureDefinitionKind> kind, BooleanType abstract_, CodeType type) { 1289 super(); 1290 this.url = url; 1291 this.name = name; 1292 this.status = status; 1293 this.kind = kind; 1294 this.abstract_ = abstract_; 1295 this.type = type; 1296 } 1297 1298 /** 1299 * @return {@link #url} (An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure definition is (or will be) published. The URL SHOULD include the major version of the structure definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1300 */ 1301 public UriType getUrlElement() { 1302 if (this.url == null) 1303 if (Configuration.errorOnAutoCreate()) 1304 throw new Error("Attempt to auto-create StructureDefinition.url"); 1305 else if (Configuration.doAutoCreate()) 1306 this.url = new UriType(); // bb 1307 return this.url; 1308 } 1309 1310 public boolean hasUrlElement() { 1311 return this.url != null && !this.url.isEmpty(); 1312 } 1313 1314 public boolean hasUrl() { 1315 return this.url != null && !this.url.isEmpty(); 1316 } 1317 1318 /** 1319 * @param value {@link #url} (An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure definition is (or will be) published. The URL SHOULD include the major version of the structure definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1320 */ 1321 public StructureDefinition setUrlElement(UriType value) { 1322 this.url = value; 1323 return this; 1324 } 1325 1326 /** 1327 * @return An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure definition is (or will be) published. The URL SHOULD include the major version of the structure definition. For more information see [Technical and Business Versions](resource.html#versions). 1328 */ 1329 public String getUrl() { 1330 return this.url == null ? null : this.url.getValue(); 1331 } 1332 1333 /** 1334 * @param value An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure definition is (or will be) published. The URL SHOULD include the major version of the structure definition. For more information see [Technical and Business Versions](resource.html#versions). 1335 */ 1336 public StructureDefinition setUrl(String value) { 1337 if (this.url == null) 1338 this.url = new UriType(); 1339 this.url.setValue(value); 1340 return this; 1341 } 1342 1343 /** 1344 * @return {@link #identifier} (A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1345 */ 1346 public List<Identifier> getIdentifier() { 1347 if (this.identifier == null) 1348 this.identifier = new ArrayList<Identifier>(); 1349 return this.identifier; 1350 } 1351 1352 /** 1353 * @return Returns a reference to <code>this</code> for easy method chaining 1354 */ 1355 public StructureDefinition setIdentifier(List<Identifier> theIdentifier) { 1356 this.identifier = theIdentifier; 1357 return this; 1358 } 1359 1360 public boolean hasIdentifier() { 1361 if (this.identifier == null) 1362 return false; 1363 for (Identifier item : this.identifier) 1364 if (!item.isEmpty()) 1365 return true; 1366 return false; 1367 } 1368 1369 public Identifier addIdentifier() { //3 1370 Identifier t = new Identifier(); 1371 if (this.identifier == null) 1372 this.identifier = new ArrayList<Identifier>(); 1373 this.identifier.add(t); 1374 return t; 1375 } 1376 1377 public StructureDefinition addIdentifier(Identifier t) { //3 1378 if (t == null) 1379 return this; 1380 if (this.identifier == null) 1381 this.identifier = new ArrayList<Identifier>(); 1382 this.identifier.add(t); 1383 return this; 1384 } 1385 1386 /** 1387 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1388 */ 1389 public Identifier getIdentifierFirstRep() { 1390 if (getIdentifier().isEmpty()) { 1391 addIdentifier(); 1392 } 1393 return getIdentifier().get(0); 1394 } 1395 1396 /** 1397 * @return {@link #version} (The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1398 */ 1399 public StringType getVersionElement() { 1400 if (this.version == null) 1401 if (Configuration.errorOnAutoCreate()) 1402 throw new Error("Attempt to auto-create StructureDefinition.version"); 1403 else if (Configuration.doAutoCreate()) 1404 this.version = new StringType(); // bb 1405 return this.version; 1406 } 1407 1408 public boolean hasVersionElement() { 1409 return this.version != null && !this.version.isEmpty(); 1410 } 1411 1412 public boolean hasVersion() { 1413 return this.version != null && !this.version.isEmpty(); 1414 } 1415 1416 /** 1417 * @param value {@link #version} (The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1418 */ 1419 public StructureDefinition setVersionElement(StringType value) { 1420 this.version = value; 1421 return this; 1422 } 1423 1424 /** 1425 * @return The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1426 */ 1427 public String getVersion() { 1428 return this.version == null ? null : this.version.getValue(); 1429 } 1430 1431 /** 1432 * @param value The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1433 */ 1434 public StructureDefinition setVersion(String value) { 1435 if (Utilities.noString(value)) 1436 this.version = null; 1437 else { 1438 if (this.version == null) 1439 this.version = new StringType(); 1440 this.version.setValue(value); 1441 } 1442 return this; 1443 } 1444 1445 /** 1446 * @return {@link #name} (A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1447 */ 1448 public StringType getNameElement() { 1449 if (this.name == null) 1450 if (Configuration.errorOnAutoCreate()) 1451 throw new Error("Attempt to auto-create StructureDefinition.name"); 1452 else if (Configuration.doAutoCreate()) 1453 this.name = new StringType(); // bb 1454 return this.name; 1455 } 1456 1457 public boolean hasNameElement() { 1458 return this.name != null && !this.name.isEmpty(); 1459 } 1460 1461 public boolean hasName() { 1462 return this.name != null && !this.name.isEmpty(); 1463 } 1464 1465 /** 1466 * @param value {@link #name} (A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1467 */ 1468 public StructureDefinition setNameElement(StringType value) { 1469 this.name = value; 1470 return this; 1471 } 1472 1473 /** 1474 * @return A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1475 */ 1476 public String getName() { 1477 return this.name == null ? null : this.name.getValue(); 1478 } 1479 1480 /** 1481 * @param value A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1482 */ 1483 public StructureDefinition setName(String value) { 1484 if (this.name == null) 1485 this.name = new StringType(); 1486 this.name.setValue(value); 1487 return this; 1488 } 1489 1490 /** 1491 * @return {@link #title} (A short, descriptive, user-friendly title for the structure definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1492 */ 1493 public StringType getTitleElement() { 1494 if (this.title == null) 1495 if (Configuration.errorOnAutoCreate()) 1496 throw new Error("Attempt to auto-create StructureDefinition.title"); 1497 else if (Configuration.doAutoCreate()) 1498 this.title = new StringType(); // bb 1499 return this.title; 1500 } 1501 1502 public boolean hasTitleElement() { 1503 return this.title != null && !this.title.isEmpty(); 1504 } 1505 1506 public boolean hasTitle() { 1507 return this.title != null && !this.title.isEmpty(); 1508 } 1509 1510 /** 1511 * @param value {@link #title} (A short, descriptive, user-friendly title for the structure definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1512 */ 1513 public StructureDefinition setTitleElement(StringType value) { 1514 this.title = value; 1515 return this; 1516 } 1517 1518 /** 1519 * @return A short, descriptive, user-friendly title for the structure definition. 1520 */ 1521 public String getTitle() { 1522 return this.title == null ? null : this.title.getValue(); 1523 } 1524 1525 /** 1526 * @param value A short, descriptive, user-friendly title for the structure definition. 1527 */ 1528 public StructureDefinition setTitle(String value) { 1529 if (Utilities.noString(value)) 1530 this.title = null; 1531 else { 1532 if (this.title == null) 1533 this.title = new StringType(); 1534 this.title.setValue(value); 1535 } 1536 return this; 1537 } 1538 1539 /** 1540 * @return {@link #status} (The status of this structure definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1541 */ 1542 public Enumeration<PublicationStatus> getStatusElement() { 1543 if (this.status == null) 1544 if (Configuration.errorOnAutoCreate()) 1545 throw new Error("Attempt to auto-create StructureDefinition.status"); 1546 else if (Configuration.doAutoCreate()) 1547 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1548 return this.status; 1549 } 1550 1551 public boolean hasStatusElement() { 1552 return this.status != null && !this.status.isEmpty(); 1553 } 1554 1555 public boolean hasStatus() { 1556 return this.status != null && !this.status.isEmpty(); 1557 } 1558 1559 /** 1560 * @param value {@link #status} (The status of this structure definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1561 */ 1562 public StructureDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1563 this.status = value; 1564 return this; 1565 } 1566 1567 /** 1568 * @return The status of this structure definition. Enables tracking the life-cycle of the content. 1569 */ 1570 public PublicationStatus getStatus() { 1571 return this.status == null ? null : this.status.getValue(); 1572 } 1573 1574 /** 1575 * @param value The status of this structure definition. Enables tracking the life-cycle of the content. 1576 */ 1577 public StructureDefinition setStatus(PublicationStatus value) { 1578 if (this.status == null) 1579 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1580 this.status.setValue(value); 1581 return this; 1582 } 1583 1584 /** 1585 * @return {@link #experimental} (A boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1586 */ 1587 public BooleanType getExperimentalElement() { 1588 if (this.experimental == null) 1589 if (Configuration.errorOnAutoCreate()) 1590 throw new Error("Attempt to auto-create StructureDefinition.experimental"); 1591 else if (Configuration.doAutoCreate()) 1592 this.experimental = new BooleanType(); // bb 1593 return this.experimental; 1594 } 1595 1596 public boolean hasExperimentalElement() { 1597 return this.experimental != null && !this.experimental.isEmpty(); 1598 } 1599 1600 public boolean hasExperimental() { 1601 return this.experimental != null && !this.experimental.isEmpty(); 1602 } 1603 1604 /** 1605 * @param value {@link #experimental} (A boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1606 */ 1607 public StructureDefinition setExperimentalElement(BooleanType value) { 1608 this.experimental = value; 1609 return this; 1610 } 1611 1612 /** 1613 * @return A boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1614 */ 1615 public boolean getExperimental() { 1616 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1617 } 1618 1619 /** 1620 * @param value A boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1621 */ 1622 public StructureDefinition setExperimental(boolean value) { 1623 if (this.experimental == null) 1624 this.experimental = new BooleanType(); 1625 this.experimental.setValue(value); 1626 return this; 1627 } 1628 1629 /** 1630 * @return {@link #date} (The date (and optionally time) when the structure definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1631 */ 1632 public DateTimeType getDateElement() { 1633 if (this.date == null) 1634 if (Configuration.errorOnAutoCreate()) 1635 throw new Error("Attempt to auto-create StructureDefinition.date"); 1636 else if (Configuration.doAutoCreate()) 1637 this.date = new DateTimeType(); // bb 1638 return this.date; 1639 } 1640 1641 public boolean hasDateElement() { 1642 return this.date != null && !this.date.isEmpty(); 1643 } 1644 1645 public boolean hasDate() { 1646 return this.date != null && !this.date.isEmpty(); 1647 } 1648 1649 /** 1650 * @param value {@link #date} (The date (and optionally time) when the structure definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1651 */ 1652 public StructureDefinition setDateElement(DateTimeType value) { 1653 this.date = value; 1654 return this; 1655 } 1656 1657 /** 1658 * @return The date (and optionally time) when the structure definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes. 1659 */ 1660 public Date getDate() { 1661 return this.date == null ? null : this.date.getValue(); 1662 } 1663 1664 /** 1665 * @param value The date (and optionally time) when the structure definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes. 1666 */ 1667 public StructureDefinition setDate(Date value) { 1668 if (value == null) 1669 this.date = null; 1670 else { 1671 if (this.date == null) 1672 this.date = new DateTimeType(); 1673 this.date.setValue(value); 1674 } 1675 return this; 1676 } 1677 1678 /** 1679 * @return {@link #publisher} (The name of the individual or organization that published the structure definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1680 */ 1681 public StringType getPublisherElement() { 1682 if (this.publisher == null) 1683 if (Configuration.errorOnAutoCreate()) 1684 throw new Error("Attempt to auto-create StructureDefinition.publisher"); 1685 else if (Configuration.doAutoCreate()) 1686 this.publisher = new StringType(); // bb 1687 return this.publisher; 1688 } 1689 1690 public boolean hasPublisherElement() { 1691 return this.publisher != null && !this.publisher.isEmpty(); 1692 } 1693 1694 public boolean hasPublisher() { 1695 return this.publisher != null && !this.publisher.isEmpty(); 1696 } 1697 1698 /** 1699 * @param value {@link #publisher} (The name of the individual or organization that published the structure definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1700 */ 1701 public StructureDefinition setPublisherElement(StringType value) { 1702 this.publisher = value; 1703 return this; 1704 } 1705 1706 /** 1707 * @return The name of the individual or organization that published the structure definition. 1708 */ 1709 public String getPublisher() { 1710 return this.publisher == null ? null : this.publisher.getValue(); 1711 } 1712 1713 /** 1714 * @param value The name of the individual or organization that published the structure definition. 1715 */ 1716 public StructureDefinition setPublisher(String value) { 1717 if (Utilities.noString(value)) 1718 this.publisher = null; 1719 else { 1720 if (this.publisher == null) 1721 this.publisher = new StringType(); 1722 this.publisher.setValue(value); 1723 } 1724 return this; 1725 } 1726 1727 /** 1728 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1729 */ 1730 public List<ContactDetail> getContact() { 1731 if (this.contact == null) 1732 this.contact = new ArrayList<ContactDetail>(); 1733 return this.contact; 1734 } 1735 1736 /** 1737 * @return Returns a reference to <code>this</code> for easy method chaining 1738 */ 1739 public StructureDefinition setContact(List<ContactDetail> theContact) { 1740 this.contact = theContact; 1741 return this; 1742 } 1743 1744 public boolean hasContact() { 1745 if (this.contact == null) 1746 return false; 1747 for (ContactDetail item : this.contact) 1748 if (!item.isEmpty()) 1749 return true; 1750 return false; 1751 } 1752 1753 public ContactDetail addContact() { //3 1754 ContactDetail t = new ContactDetail(); 1755 if (this.contact == null) 1756 this.contact = new ArrayList<ContactDetail>(); 1757 this.contact.add(t); 1758 return t; 1759 } 1760 1761 public StructureDefinition addContact(ContactDetail t) { //3 1762 if (t == null) 1763 return this; 1764 if (this.contact == null) 1765 this.contact = new ArrayList<ContactDetail>(); 1766 this.contact.add(t); 1767 return this; 1768 } 1769 1770 /** 1771 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 1772 */ 1773 public ContactDetail getContactFirstRep() { 1774 if (getContact().isEmpty()) { 1775 addContact(); 1776 } 1777 return getContact().get(0); 1778 } 1779 1780 /** 1781 * @return {@link #description} (A free text natural language description of the structure definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1782 */ 1783 public MarkdownType getDescriptionElement() { 1784 if (this.description == null) 1785 if (Configuration.errorOnAutoCreate()) 1786 throw new Error("Attempt to auto-create StructureDefinition.description"); 1787 else if (Configuration.doAutoCreate()) 1788 this.description = new MarkdownType(); // bb 1789 return this.description; 1790 } 1791 1792 public boolean hasDescriptionElement() { 1793 return this.description != null && !this.description.isEmpty(); 1794 } 1795 1796 public boolean hasDescription() { 1797 return this.description != null && !this.description.isEmpty(); 1798 } 1799 1800 /** 1801 * @param value {@link #description} (A free text natural language description of the structure definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1802 */ 1803 public StructureDefinition setDescriptionElement(MarkdownType value) { 1804 this.description = value; 1805 return this; 1806 } 1807 1808 /** 1809 * @return A free text natural language description of the structure definition from a consumer's perspective. 1810 */ 1811 public String getDescription() { 1812 return this.description == null ? null : this.description.getValue(); 1813 } 1814 1815 /** 1816 * @param value A free text natural language description of the structure definition from a consumer's perspective. 1817 */ 1818 public StructureDefinition setDescription(String value) { 1819 if (value == null) 1820 this.description = null; 1821 else { 1822 if (this.description == null) 1823 this.description = new MarkdownType(); 1824 this.description.setValue(value); 1825 } 1826 return this; 1827 } 1828 1829 /** 1830 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate structure definition instances.) 1831 */ 1832 public List<UsageContext> getUseContext() { 1833 if (this.useContext == null) 1834 this.useContext = new ArrayList<UsageContext>(); 1835 return this.useContext; 1836 } 1837 1838 /** 1839 * @return Returns a reference to <code>this</code> for easy method chaining 1840 */ 1841 public StructureDefinition setUseContext(List<UsageContext> theUseContext) { 1842 this.useContext = theUseContext; 1843 return this; 1844 } 1845 1846 public boolean hasUseContext() { 1847 if (this.useContext == null) 1848 return false; 1849 for (UsageContext item : this.useContext) 1850 if (!item.isEmpty()) 1851 return true; 1852 return false; 1853 } 1854 1855 public UsageContext addUseContext() { //3 1856 UsageContext t = new UsageContext(); 1857 if (this.useContext == null) 1858 this.useContext = new ArrayList<UsageContext>(); 1859 this.useContext.add(t); 1860 return t; 1861 } 1862 1863 public StructureDefinition addUseContext(UsageContext t) { //3 1864 if (t == null) 1865 return this; 1866 if (this.useContext == null) 1867 this.useContext = new ArrayList<UsageContext>(); 1868 this.useContext.add(t); 1869 return this; 1870 } 1871 1872 /** 1873 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 1874 */ 1875 public UsageContext getUseContextFirstRep() { 1876 if (getUseContext().isEmpty()) { 1877 addUseContext(); 1878 } 1879 return getUseContext().get(0); 1880 } 1881 1882 /** 1883 * @return {@link #jurisdiction} (A legal or geographic region in which the structure definition is intended to be used.) 1884 */ 1885 public List<CodeableConcept> getJurisdiction() { 1886 if (this.jurisdiction == null) 1887 this.jurisdiction = new ArrayList<CodeableConcept>(); 1888 return this.jurisdiction; 1889 } 1890 1891 /** 1892 * @return Returns a reference to <code>this</code> for easy method chaining 1893 */ 1894 public StructureDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1895 this.jurisdiction = theJurisdiction; 1896 return this; 1897 } 1898 1899 public boolean hasJurisdiction() { 1900 if (this.jurisdiction == null) 1901 return false; 1902 for (CodeableConcept item : this.jurisdiction) 1903 if (!item.isEmpty()) 1904 return true; 1905 return false; 1906 } 1907 1908 public CodeableConcept addJurisdiction() { //3 1909 CodeableConcept t = new CodeableConcept(); 1910 if (this.jurisdiction == null) 1911 this.jurisdiction = new ArrayList<CodeableConcept>(); 1912 this.jurisdiction.add(t); 1913 return t; 1914 } 1915 1916 public StructureDefinition addJurisdiction(CodeableConcept t) { //3 1917 if (t == null) 1918 return this; 1919 if (this.jurisdiction == null) 1920 this.jurisdiction = new ArrayList<CodeableConcept>(); 1921 this.jurisdiction.add(t); 1922 return this; 1923 } 1924 1925 /** 1926 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 1927 */ 1928 public CodeableConcept getJurisdictionFirstRep() { 1929 if (getJurisdiction().isEmpty()) { 1930 addJurisdiction(); 1931 } 1932 return getJurisdiction().get(0); 1933 } 1934 1935 /** 1936 * @return {@link #purpose} (Explaination of why this structure definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1937 */ 1938 public MarkdownType getPurposeElement() { 1939 if (this.purpose == null) 1940 if (Configuration.errorOnAutoCreate()) 1941 throw new Error("Attempt to auto-create StructureDefinition.purpose"); 1942 else if (Configuration.doAutoCreate()) 1943 this.purpose = new MarkdownType(); // bb 1944 return this.purpose; 1945 } 1946 1947 public boolean hasPurposeElement() { 1948 return this.purpose != null && !this.purpose.isEmpty(); 1949 } 1950 1951 public boolean hasPurpose() { 1952 return this.purpose != null && !this.purpose.isEmpty(); 1953 } 1954 1955 /** 1956 * @param value {@link #purpose} (Explaination of why this structure definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1957 */ 1958 public StructureDefinition setPurposeElement(MarkdownType value) { 1959 this.purpose = value; 1960 return this; 1961 } 1962 1963 /** 1964 * @return Explaination of why this structure definition is needed and why it has been designed as it has. 1965 */ 1966 public String getPurpose() { 1967 return this.purpose == null ? null : this.purpose.getValue(); 1968 } 1969 1970 /** 1971 * @param value Explaination of why this structure definition is needed and why it has been designed as it has. 1972 */ 1973 public StructureDefinition setPurpose(String value) { 1974 if (value == null) 1975 this.purpose = null; 1976 else { 1977 if (this.purpose == null) 1978 this.purpose = new MarkdownType(); 1979 this.purpose.setValue(value); 1980 } 1981 return this; 1982 } 1983 1984 /** 1985 * @return {@link #copyright} (A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1986 */ 1987 public MarkdownType getCopyrightElement() { 1988 if (this.copyright == null) 1989 if (Configuration.errorOnAutoCreate()) 1990 throw new Error("Attempt to auto-create StructureDefinition.copyright"); 1991 else if (Configuration.doAutoCreate()) 1992 this.copyright = new MarkdownType(); // bb 1993 return this.copyright; 1994 } 1995 1996 public boolean hasCopyrightElement() { 1997 return this.copyright != null && !this.copyright.isEmpty(); 1998 } 1999 2000 public boolean hasCopyright() { 2001 return this.copyright != null && !this.copyright.isEmpty(); 2002 } 2003 2004 /** 2005 * @param value {@link #copyright} (A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2006 */ 2007 public StructureDefinition setCopyrightElement(MarkdownType value) { 2008 this.copyright = value; 2009 return this; 2010 } 2011 2012 /** 2013 * @return A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. 2014 */ 2015 public String getCopyright() { 2016 return this.copyright == null ? null : this.copyright.getValue(); 2017 } 2018 2019 /** 2020 * @param value A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition. 2021 */ 2022 public StructureDefinition setCopyright(String value) { 2023 if (value == null) 2024 this.copyright = null; 2025 else { 2026 if (this.copyright == null) 2027 this.copyright = new MarkdownType(); 2028 this.copyright.setValue(value); 2029 } 2030 return this; 2031 } 2032 2033 /** 2034 * @return {@link #keyword} (A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates.) 2035 */ 2036 public List<Coding> getKeyword() { 2037 if (this.keyword == null) 2038 this.keyword = new ArrayList<Coding>(); 2039 return this.keyword; 2040 } 2041 2042 /** 2043 * @return Returns a reference to <code>this</code> for easy method chaining 2044 */ 2045 public StructureDefinition setKeyword(List<Coding> theKeyword) { 2046 this.keyword = theKeyword; 2047 return this; 2048 } 2049 2050 public boolean hasKeyword() { 2051 if (this.keyword == null) 2052 return false; 2053 for (Coding item : this.keyword) 2054 if (!item.isEmpty()) 2055 return true; 2056 return false; 2057 } 2058 2059 public Coding addKeyword() { //3 2060 Coding t = new Coding(); 2061 if (this.keyword == null) 2062 this.keyword = new ArrayList<Coding>(); 2063 this.keyword.add(t); 2064 return t; 2065 } 2066 2067 public StructureDefinition addKeyword(Coding t) { //3 2068 if (t == null) 2069 return this; 2070 if (this.keyword == null) 2071 this.keyword = new ArrayList<Coding>(); 2072 this.keyword.add(t); 2073 return this; 2074 } 2075 2076 /** 2077 * @return The first repetition of repeating field {@link #keyword}, creating it if it does not already exist 2078 */ 2079 public Coding getKeywordFirstRep() { 2080 if (getKeyword().isEmpty()) { 2081 addKeyword(); 2082 } 2083 return getKeyword().get(0); 2084 } 2085 2086 /** 2087 * @return {@link #fhirVersion} (The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 2088 */ 2089 public IdType getFhirVersionElement() { 2090 if (this.fhirVersion == null) 2091 if (Configuration.errorOnAutoCreate()) 2092 throw new Error("Attempt to auto-create StructureDefinition.fhirVersion"); 2093 else if (Configuration.doAutoCreate()) 2094 this.fhirVersion = new IdType(); // bb 2095 return this.fhirVersion; 2096 } 2097 2098 public boolean hasFhirVersionElement() { 2099 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 2100 } 2101 2102 public boolean hasFhirVersion() { 2103 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 2104 } 2105 2106 /** 2107 * @param value {@link #fhirVersion} (The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 2108 */ 2109 public StructureDefinition setFhirVersionElement(IdType value) { 2110 this.fhirVersion = value; 2111 return this; 2112 } 2113 2114 /** 2115 * @return The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version. 2116 */ 2117 public String getFhirVersion() { 2118 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 2119 } 2120 2121 /** 2122 * @param value The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version. 2123 */ 2124 public StructureDefinition setFhirVersion(String value) { 2125 if (Utilities.noString(value)) 2126 this.fhirVersion = null; 2127 else { 2128 if (this.fhirVersion == null) 2129 this.fhirVersion = new IdType(); 2130 this.fhirVersion.setValue(value); 2131 } 2132 return this; 2133 } 2134 2135 /** 2136 * @return {@link #mapping} (An external specification that the content is mapped to.) 2137 */ 2138 public List<StructureDefinitionMappingComponent> getMapping() { 2139 if (this.mapping == null) 2140 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2141 return this.mapping; 2142 } 2143 2144 /** 2145 * @return Returns a reference to <code>this</code> for easy method chaining 2146 */ 2147 public StructureDefinition setMapping(List<StructureDefinitionMappingComponent> theMapping) { 2148 this.mapping = theMapping; 2149 return this; 2150 } 2151 2152 public boolean hasMapping() { 2153 if (this.mapping == null) 2154 return false; 2155 for (StructureDefinitionMappingComponent item : this.mapping) 2156 if (!item.isEmpty()) 2157 return true; 2158 return false; 2159 } 2160 2161 public StructureDefinitionMappingComponent addMapping() { //3 2162 StructureDefinitionMappingComponent t = new StructureDefinitionMappingComponent(); 2163 if (this.mapping == null) 2164 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2165 this.mapping.add(t); 2166 return t; 2167 } 2168 2169 public StructureDefinition addMapping(StructureDefinitionMappingComponent t) { //3 2170 if (t == null) 2171 return this; 2172 if (this.mapping == null) 2173 this.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 2174 this.mapping.add(t); 2175 return this; 2176 } 2177 2178 /** 2179 * @return The first repetition of repeating field {@link #mapping}, creating it if it does not already exist 2180 */ 2181 public StructureDefinitionMappingComponent getMappingFirstRep() { 2182 if (getMapping().isEmpty()) { 2183 addMapping(); 2184 } 2185 return getMapping().get(0); 2186 } 2187 2188 /** 2189 * @return {@link #kind} (Defines the kind of structure that this definition is describing.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2190 */ 2191 public Enumeration<StructureDefinitionKind> getKindElement() { 2192 if (this.kind == null) 2193 if (Configuration.errorOnAutoCreate()) 2194 throw new Error("Attempt to auto-create StructureDefinition.kind"); 2195 else if (Configuration.doAutoCreate()) 2196 this.kind = new Enumeration<StructureDefinitionKind>(new StructureDefinitionKindEnumFactory()); // bb 2197 return this.kind; 2198 } 2199 2200 public boolean hasKindElement() { 2201 return this.kind != null && !this.kind.isEmpty(); 2202 } 2203 2204 public boolean hasKind() { 2205 return this.kind != null && !this.kind.isEmpty(); 2206 } 2207 2208 /** 2209 * @param value {@link #kind} (Defines the kind of structure that this definition is describing.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 2210 */ 2211 public StructureDefinition setKindElement(Enumeration<StructureDefinitionKind> value) { 2212 this.kind = value; 2213 return this; 2214 } 2215 2216 /** 2217 * @return Defines the kind of structure that this definition is describing. 2218 */ 2219 public StructureDefinitionKind getKind() { 2220 return this.kind == null ? null : this.kind.getValue(); 2221 } 2222 2223 /** 2224 * @param value Defines the kind of structure that this definition is describing. 2225 */ 2226 public StructureDefinition setKind(StructureDefinitionKind value) { 2227 if (this.kind == null) 2228 this.kind = new Enumeration<StructureDefinitionKind>(new StructureDefinitionKindEnumFactory()); 2229 this.kind.setValue(value); 2230 return this; 2231 } 2232 2233 /** 2234 * @return {@link #abstract_} (Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.). This is the underlying object with id, value and extensions. The accessor "getAbstract" gives direct access to the value 2235 */ 2236 public BooleanType getAbstractElement() { 2237 if (this.abstract_ == null) 2238 if (Configuration.errorOnAutoCreate()) 2239 throw new Error("Attempt to auto-create StructureDefinition.abstract_"); 2240 else if (Configuration.doAutoCreate()) 2241 this.abstract_ = new BooleanType(); // bb 2242 return this.abstract_; 2243 } 2244 2245 public boolean hasAbstractElement() { 2246 return this.abstract_ != null && !this.abstract_.isEmpty(); 2247 } 2248 2249 public boolean hasAbstract() { 2250 return this.abstract_ != null && !this.abstract_.isEmpty(); 2251 } 2252 2253 /** 2254 * @param value {@link #abstract_} (Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.). This is the underlying object with id, value and extensions. The accessor "getAbstract" gives direct access to the value 2255 */ 2256 public StructureDefinition setAbstractElement(BooleanType value) { 2257 this.abstract_ = value; 2258 return this; 2259 } 2260 2261 /** 2262 * @return Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems. 2263 */ 2264 public boolean getAbstract() { 2265 return this.abstract_ == null || this.abstract_.isEmpty() ? false : this.abstract_.getValue(); 2266 } 2267 2268 /** 2269 * @param value Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems. 2270 */ 2271 public StructureDefinition setAbstract(boolean value) { 2272 if (this.abstract_ == null) 2273 this.abstract_ = new BooleanType(); 2274 this.abstract_.setValue(value); 2275 return this; 2276 } 2277 2278 /** 2279 * @return {@link #contextType} (If this is an extension, Identifies the context within FHIR resources where the extension can be used.). This is the underlying object with id, value and extensions. The accessor "getContextType" gives direct access to the value 2280 */ 2281 public Enumeration<ExtensionContext> getContextTypeElement() { 2282 if (this.contextType == null) 2283 if (Configuration.errorOnAutoCreate()) 2284 throw new Error("Attempt to auto-create StructureDefinition.contextType"); 2285 else if (Configuration.doAutoCreate()) 2286 this.contextType = new Enumeration<ExtensionContext>(new ExtensionContextEnumFactory()); // bb 2287 return this.contextType; 2288 } 2289 2290 public boolean hasContextTypeElement() { 2291 return this.contextType != null && !this.contextType.isEmpty(); 2292 } 2293 2294 public boolean hasContextType() { 2295 return this.contextType != null && !this.contextType.isEmpty(); 2296 } 2297 2298 /** 2299 * @param value {@link #contextType} (If this is an extension, Identifies the context within FHIR resources where the extension can be used.). This is the underlying object with id, value and extensions. The accessor "getContextType" gives direct access to the value 2300 */ 2301 public StructureDefinition setContextTypeElement(Enumeration<ExtensionContext> value) { 2302 this.contextType = value; 2303 return this; 2304 } 2305 2306 /** 2307 * @return If this is an extension, Identifies the context within FHIR resources where the extension can be used. 2308 */ 2309 public ExtensionContext getContextType() { 2310 return this.contextType == null ? null : this.contextType.getValue(); 2311 } 2312 2313 /** 2314 * @param value If this is an extension, Identifies the context within FHIR resources where the extension can be used. 2315 */ 2316 public StructureDefinition setContextType(ExtensionContext value) { 2317 if (value == null) 2318 this.contextType = null; 2319 else { 2320 if (this.contextType == null) 2321 this.contextType = new Enumeration<ExtensionContext>(new ExtensionContextEnumFactory()); 2322 this.contextType.setValue(value); 2323 } 2324 return this; 2325 } 2326 2327 /** 2328 * @return {@link #context} (Identifies the types of resource or data type elements to which the extension can be applied.) 2329 */ 2330 public List<StringType> getContext() { 2331 if (this.context == null) 2332 this.context = new ArrayList<StringType>(); 2333 return this.context; 2334 } 2335 2336 /** 2337 * @return Returns a reference to <code>this</code> for easy method chaining 2338 */ 2339 public StructureDefinition setContext(List<StringType> theContext) { 2340 this.context = theContext; 2341 return this; 2342 } 2343 2344 public boolean hasContext() { 2345 if (this.context == null) 2346 return false; 2347 for (StringType item : this.context) 2348 if (!item.isEmpty()) 2349 return true; 2350 return false; 2351 } 2352 2353 /** 2354 * @return {@link #context} (Identifies the types of resource or data type elements to which the extension can be applied.) 2355 */ 2356 public StringType addContextElement() {//2 2357 StringType t = new StringType(); 2358 if (this.context == null) 2359 this.context = new ArrayList<StringType>(); 2360 this.context.add(t); 2361 return t; 2362 } 2363 2364 /** 2365 * @param value {@link #context} (Identifies the types of resource or data type elements to which the extension can be applied.) 2366 */ 2367 public StructureDefinition addContext(String value) { //1 2368 StringType t = new StringType(); 2369 t.setValue(value); 2370 if (this.context == null) 2371 this.context = new ArrayList<StringType>(); 2372 this.context.add(t); 2373 return this; 2374 } 2375 2376 /** 2377 * @param value {@link #context} (Identifies the types of resource or data type elements to which the extension can be applied.) 2378 */ 2379 public boolean hasContext(String value) { 2380 if (this.context == null) 2381 return false; 2382 for (StringType v : this.context) 2383 if (v.getValue().equals(value)) // string 2384 return true; 2385 return false; 2386 } 2387 2388 /** 2389 * @return {@link #contextInvariant} (A set of rules as Fluent Invariants about when the extension can be used (e.g. co-occurrence variants for the extension).) 2390 */ 2391 public List<StringType> getContextInvariant() { 2392 if (this.contextInvariant == null) 2393 this.contextInvariant = new ArrayList<StringType>(); 2394 return this.contextInvariant; 2395 } 2396 2397 /** 2398 * @return Returns a reference to <code>this</code> for easy method chaining 2399 */ 2400 public StructureDefinition setContextInvariant(List<StringType> theContextInvariant) { 2401 this.contextInvariant = theContextInvariant; 2402 return this; 2403 } 2404 2405 public boolean hasContextInvariant() { 2406 if (this.contextInvariant == null) 2407 return false; 2408 for (StringType item : this.contextInvariant) 2409 if (!item.isEmpty()) 2410 return true; 2411 return false; 2412 } 2413 2414 /** 2415 * @return {@link #contextInvariant} (A set of rules as Fluent Invariants about when the extension can be used (e.g. co-occurrence variants for the extension).) 2416 */ 2417 public StringType addContextInvariantElement() {//2 2418 StringType t = new StringType(); 2419 if (this.contextInvariant == null) 2420 this.contextInvariant = new ArrayList<StringType>(); 2421 this.contextInvariant.add(t); 2422 return t; 2423 } 2424 2425 /** 2426 * @param value {@link #contextInvariant} (A set of rules as Fluent Invariants about when the extension can be used (e.g. co-occurrence variants for the extension).) 2427 */ 2428 public StructureDefinition addContextInvariant(String value) { //1 2429 StringType t = new StringType(); 2430 t.setValue(value); 2431 if (this.contextInvariant == null) 2432 this.contextInvariant = new ArrayList<StringType>(); 2433 this.contextInvariant.add(t); 2434 return this; 2435 } 2436 2437 /** 2438 * @param value {@link #contextInvariant} (A set of rules as Fluent Invariants about when the extension can be used (e.g. co-occurrence variants for the extension).) 2439 */ 2440 public boolean hasContextInvariant(String value) { 2441 if (this.contextInvariant == null) 2442 return false; 2443 for (StringType v : this.contextInvariant) 2444 if (v.getValue().equals(value)) // string 2445 return true; 2446 return false; 2447 } 2448 2449 /** 2450 * @return {@link #type} (The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2451 */ 2452 public CodeType getTypeElement() { 2453 if (this.type == null) 2454 if (Configuration.errorOnAutoCreate()) 2455 throw new Error("Attempt to auto-create StructureDefinition.type"); 2456 else if (Configuration.doAutoCreate()) 2457 this.type = new CodeType(); // bb 2458 return this.type; 2459 } 2460 2461 public boolean hasTypeElement() { 2462 return this.type != null && !this.type.isEmpty(); 2463 } 2464 2465 public boolean hasType() { 2466 return this.type != null && !this.type.isEmpty(); 2467 } 2468 2469 /** 2470 * @param value {@link #type} (The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type).). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2471 */ 2472 public StructureDefinition setTypeElement(CodeType value) { 2473 this.type = value; 2474 return this; 2475 } 2476 2477 /** 2478 * @return The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). 2479 */ 2480 public String getType() { 2481 return this.type == null ? null : this.type.getValue(); 2482 } 2483 2484 /** 2485 * @param value The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type). 2486 */ 2487 public StructureDefinition setType(String value) { 2488 if (this.type == null) 2489 this.type = new CodeType(); 2490 this.type.setValue(value); 2491 return this; 2492 } 2493 2494 /** 2495 * @return {@link #baseDefinition} (An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.). This is the underlying object with id, value and extensions. The accessor "getBaseDefinition" gives direct access to the value 2496 */ 2497 public UriType getBaseDefinitionElement() { 2498 if (this.baseDefinition == null) 2499 if (Configuration.errorOnAutoCreate()) 2500 throw new Error("Attempt to auto-create StructureDefinition.baseDefinition"); 2501 else if (Configuration.doAutoCreate()) 2502 this.baseDefinition = new UriType(); // bb 2503 return this.baseDefinition; 2504 } 2505 2506 public boolean hasBaseDefinitionElement() { 2507 return this.baseDefinition != null && !this.baseDefinition.isEmpty(); 2508 } 2509 2510 public boolean hasBaseDefinition() { 2511 return this.baseDefinition != null && !this.baseDefinition.isEmpty(); 2512 } 2513 2514 /** 2515 * @param value {@link #baseDefinition} (An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.). This is the underlying object with id, value and extensions. The accessor "getBaseDefinition" gives direct access to the value 2516 */ 2517 public StructureDefinition setBaseDefinitionElement(UriType value) { 2518 this.baseDefinition = value; 2519 return this; 2520 } 2521 2522 /** 2523 * @return An absolute URI that is the base structure from which this type is derived, either by specialization or constraint. 2524 */ 2525 public String getBaseDefinition() { 2526 return this.baseDefinition == null ? null : this.baseDefinition.getValue(); 2527 } 2528 2529 /** 2530 * @param value An absolute URI that is the base structure from which this type is derived, either by specialization or constraint. 2531 */ 2532 public StructureDefinition setBaseDefinition(String value) { 2533 if (Utilities.noString(value)) 2534 this.baseDefinition = null; 2535 else { 2536 if (this.baseDefinition == null) 2537 this.baseDefinition = new UriType(); 2538 this.baseDefinition.setValue(value); 2539 } 2540 return this; 2541 } 2542 2543 /** 2544 * @return {@link #derivation} (How the type relates to the baseDefinition.). This is the underlying object with id, value and extensions. The accessor "getDerivation" gives direct access to the value 2545 */ 2546 public Enumeration<TypeDerivationRule> getDerivationElement() { 2547 if (this.derivation == null) 2548 if (Configuration.errorOnAutoCreate()) 2549 throw new Error("Attempt to auto-create StructureDefinition.derivation"); 2550 else if (Configuration.doAutoCreate()) 2551 this.derivation = new Enumeration<TypeDerivationRule>(new TypeDerivationRuleEnumFactory()); // bb 2552 return this.derivation; 2553 } 2554 2555 public boolean hasDerivationElement() { 2556 return this.derivation != null && !this.derivation.isEmpty(); 2557 } 2558 2559 public boolean hasDerivation() { 2560 return this.derivation != null && !this.derivation.isEmpty(); 2561 } 2562 2563 /** 2564 * @param value {@link #derivation} (How the type relates to the baseDefinition.). This is the underlying object with id, value and extensions. The accessor "getDerivation" gives direct access to the value 2565 */ 2566 public StructureDefinition setDerivationElement(Enumeration<TypeDerivationRule> value) { 2567 this.derivation = value; 2568 return this; 2569 } 2570 2571 /** 2572 * @return How the type relates to the baseDefinition. 2573 */ 2574 public TypeDerivationRule getDerivation() { 2575 return this.derivation == null ? null : this.derivation.getValue(); 2576 } 2577 2578 /** 2579 * @param value How the type relates to the baseDefinition. 2580 */ 2581 public StructureDefinition setDerivation(TypeDerivationRule value) { 2582 if (value == null) 2583 this.derivation = null; 2584 else { 2585 if (this.derivation == null) 2586 this.derivation = new Enumeration<TypeDerivationRule>(new TypeDerivationRuleEnumFactory()); 2587 this.derivation.setValue(value); 2588 } 2589 return this; 2590 } 2591 2592 /** 2593 * @return {@link #snapshot} (A snapshot view is expressed in a stand alone form that can be used and interpreted without considering the base StructureDefinition.) 2594 */ 2595 public StructureDefinitionSnapshotComponent getSnapshot() { 2596 if (this.snapshot == null) 2597 if (Configuration.errorOnAutoCreate()) 2598 throw new Error("Attempt to auto-create StructureDefinition.snapshot"); 2599 else if (Configuration.doAutoCreate()) 2600 this.snapshot = new StructureDefinitionSnapshotComponent(); // cc 2601 return this.snapshot; 2602 } 2603 2604 public boolean hasSnapshot() { 2605 return this.snapshot != null && !this.snapshot.isEmpty(); 2606 } 2607 2608 /** 2609 * @param value {@link #snapshot} (A snapshot view is expressed in a stand alone form that can be used and interpreted without considering the base StructureDefinition.) 2610 */ 2611 public StructureDefinition setSnapshot(StructureDefinitionSnapshotComponent value) { 2612 this.snapshot = value; 2613 return this; 2614 } 2615 2616 /** 2617 * @return {@link #differential} (A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.) 2618 */ 2619 public StructureDefinitionDifferentialComponent getDifferential() { 2620 if (this.differential == null) 2621 if (Configuration.errorOnAutoCreate()) 2622 throw new Error("Attempt to auto-create StructureDefinition.differential"); 2623 else if (Configuration.doAutoCreate()) 2624 this.differential = new StructureDefinitionDifferentialComponent(); // cc 2625 return this.differential; 2626 } 2627 2628 public boolean hasDifferential() { 2629 return this.differential != null && !this.differential.isEmpty(); 2630 } 2631 2632 /** 2633 * @param value {@link #differential} (A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.) 2634 */ 2635 public StructureDefinition setDifferential(StructureDefinitionDifferentialComponent value) { 2636 this.differential = value; 2637 return this; 2638 } 2639 2640 protected void listChildren(List<Property> children) { 2641 super.listChildren(children); 2642 children.add(new Property("url", "uri", "An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure definition is (or will be) published. The URL SHOULD include the major version of the structure definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 2643 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2644 children.add(new Property("version", "string", "The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2645 children.add(new Property("name", "string", "A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2646 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the structure definition.", 0, 1, title)); 2647 children.add(new Property("status", "code", "The status of this structure definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 2648 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 2649 children.add(new Property("date", "dateTime", "The date (and optionally time) when the structure definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.", 0, 1, date)); 2650 children.add(new Property("publisher", "string", "The name of the individual or organization that published the structure definition.", 0, 1, publisher)); 2651 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2652 children.add(new Property("description", "markdown", "A free text natural language description of the structure definition from a consumer's perspective.", 0, 1, description)); 2653 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate structure definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2654 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2655 children.add(new Property("purpose", "markdown", "Explaination of why this structure definition is needed and why it has been designed as it has.", 0, 1, purpose)); 2656 children.add(new Property("copyright", "markdown", "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition.", 0, 1, copyright)); 2657 children.add(new Property("keyword", "Coding", "A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates.", 0, java.lang.Integer.MAX_VALUE, keyword)); 2658 children.add(new Property("fhirVersion", "id", "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version.", 0, 1, fhirVersion)); 2659 children.add(new Property("mapping", "", "An external specification that the content is mapped to.", 0, java.lang.Integer.MAX_VALUE, mapping)); 2660 children.add(new Property("kind", "code", "Defines the kind of structure that this definition is describing.", 0, 1, kind)); 2661 children.add(new Property("abstract", "boolean", "Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.", 0, 1, abstract_)); 2662 children.add(new Property("contextType", "code", "If this is an extension, Identifies the context within FHIR resources where the extension can be used.", 0, 1, contextType)); 2663 children.add(new Property("context", "string", "Identifies the types of resource or data type elements to which the extension can be applied.", 0, java.lang.Integer.MAX_VALUE, context)); 2664 children.add(new Property("contextInvariant", "string", "A set of rules as Fluent Invariants about when the extension can be used (e.g. co-occurrence variants for the extension).", 0, java.lang.Integer.MAX_VALUE, contextInvariant)); 2665 children.add(new Property("type", "code", "The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type).", 0, 1, type)); 2666 children.add(new Property("baseDefinition", "uri", "An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.", 0, 1, baseDefinition)); 2667 children.add(new Property("derivation", "code", "How the type relates to the baseDefinition.", 0, 1, derivation)); 2668 children.add(new Property("snapshot", "", "A snapshot view is expressed in a stand alone form that can be used and interpreted without considering the base StructureDefinition.", 0, 1, snapshot)); 2669 children.add(new Property("differential", "", "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.", 0, 1, differential)); 2670 } 2671 2672 @Override 2673 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2674 switch (_hash) { 2675 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this structure definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure definition is (or will be) published. The URL SHOULD include the major version of the structure definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 2676 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 2677 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the structure definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2678 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the structure definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2679 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the structure definition.", 0, 1, title); 2680 case -892481550: /*status*/ return new Property("status", "code", "The status of this structure definition. Enables tracking the life-cycle of the content.", 0, 1, status); 2681 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this structure definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 2682 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the structure definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure definition changes.", 0, 1, date); 2683 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the structure definition.", 0, 1, publisher); 2684 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2685 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the structure definition from a consumer's perspective.", 0, 1, description); 2686 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate structure definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2687 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2688 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this structure definition is needed and why it has been designed as it has.", 0, 1, purpose); 2689 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the structure definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure definition.", 0, 1, copyright); 2690 case -814408215: /*keyword*/ return new Property("keyword", "Coding", "A set of key words or terms from external terminologies that may be used to assist with indexing and searching of templates.", 0, java.lang.Integer.MAX_VALUE, keyword); 2691 case 461006061: /*fhirVersion*/ return new Property("fhirVersion", "id", "The version of the FHIR specification on which this StructureDefinition is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version.", 0, 1, fhirVersion); 2692 case 837556430: /*mapping*/ return new Property("mapping", "", "An external specification that the content is mapped to.", 0, java.lang.Integer.MAX_VALUE, mapping); 2693 case 3292052: /*kind*/ return new Property("kind", "code", "Defines the kind of structure that this definition is describing.", 0, 1, kind); 2694 case 1732898850: /*abstract*/ return new Property("abstract", "boolean", "Whether structure this definition describes is abstract or not - that is, whether the structure is not intended to be instantiated. For Resources and Data types, abstract types will never be exchanged between systems.", 0, 1, abstract_); 2695 case -102839927: /*contextType*/ return new Property("contextType", "code", "If this is an extension, Identifies the context within FHIR resources where the extension can be used.", 0, 1, contextType); 2696 case 951530927: /*context*/ return new Property("context", "string", "Identifies the types of resource or data type elements to which the extension can be applied.", 0, java.lang.Integer.MAX_VALUE, context); 2697 case -802505007: /*contextInvariant*/ return new Property("contextInvariant", "string", "A set of rules as Fluent Invariants about when the extension can be used (e.g. co-occurrence variants for the extension).", 0, java.lang.Integer.MAX_VALUE, contextInvariant); 2698 case 3575610: /*type*/ return new Property("type", "code", "The type this structure describes. If the derivation kind is 'specialization' then this is the master definition for a type, and there is always one of these (a data type, an extension, a resource, including abstract ones). Otherwise the structure definition is a constraint on the stated type (and in this case, the type cannot be an abstract type).", 0, 1, type); 2699 case 1139771140: /*baseDefinition*/ return new Property("baseDefinition", "uri", "An absolute URI that is the base structure from which this type is derived, either by specialization or constraint.", 0, 1, baseDefinition); 2700 case -1353885513: /*derivation*/ return new Property("derivation", "code", "How the type relates to the baseDefinition.", 0, 1, derivation); 2701 case 284874180: /*snapshot*/ return new Property("snapshot", "", "A snapshot view is expressed in a stand alone form that can be used and interpreted without considering the base StructureDefinition.", 0, 1, snapshot); 2702 case -1196150917: /*differential*/ return new Property("differential", "", "A differential view is expressed relative to the base StructureDefinition - a statement of differences that it applies.", 0, 1, differential); 2703 default: return super.getNamedProperty(_hash, _name, _checkValid); 2704 } 2705 2706 } 2707 2708 @Override 2709 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2710 switch (hash) { 2711 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2712 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2713 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2714 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2715 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2716 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2717 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2718 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2719 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2720 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2721 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2722 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2723 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2724 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2725 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2726 case -814408215: /*keyword*/ return this.keyword == null ? new Base[0] : this.keyword.toArray(new Base[this.keyword.size()]); // Coding 2727 case 461006061: /*fhirVersion*/ return this.fhirVersion == null ? new Base[0] : new Base[] {this.fhirVersion}; // IdType 2728 case 837556430: /*mapping*/ return this.mapping == null ? new Base[0] : this.mapping.toArray(new Base[this.mapping.size()]); // StructureDefinitionMappingComponent 2729 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<StructureDefinitionKind> 2730 case 1732898850: /*abstract*/ return this.abstract_ == null ? new Base[0] : new Base[] {this.abstract_}; // BooleanType 2731 case -102839927: /*contextType*/ return this.contextType == null ? new Base[0] : new Base[] {this.contextType}; // Enumeration<ExtensionContext> 2732 case 951530927: /*context*/ return this.context == null ? new Base[0] : this.context.toArray(new Base[this.context.size()]); // StringType 2733 case -802505007: /*contextInvariant*/ return this.contextInvariant == null ? new Base[0] : this.contextInvariant.toArray(new Base[this.contextInvariant.size()]); // StringType 2734 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeType 2735 case 1139771140: /*baseDefinition*/ return this.baseDefinition == null ? new Base[0] : new Base[] {this.baseDefinition}; // UriType 2736 case -1353885513: /*derivation*/ return this.derivation == null ? new Base[0] : new Base[] {this.derivation}; // Enumeration<TypeDerivationRule> 2737 case 284874180: /*snapshot*/ return this.snapshot == null ? new Base[0] : new Base[] {this.snapshot}; // StructureDefinitionSnapshotComponent 2738 case -1196150917: /*differential*/ return this.differential == null ? new Base[0] : new Base[] {this.differential}; // StructureDefinitionDifferentialComponent 2739 default: return super.getProperty(hash, name, checkValid); 2740 } 2741 2742 } 2743 2744 @Override 2745 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2746 switch (hash) { 2747 case 116079: // url 2748 this.url = castToUri(value); // UriType 2749 return value; 2750 case -1618432855: // identifier 2751 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2752 return value; 2753 case 351608024: // version 2754 this.version = castToString(value); // StringType 2755 return value; 2756 case 3373707: // name 2757 this.name = castToString(value); // StringType 2758 return value; 2759 case 110371416: // title 2760 this.title = castToString(value); // StringType 2761 return value; 2762 case -892481550: // status 2763 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2764 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2765 return value; 2766 case -404562712: // experimental 2767 this.experimental = castToBoolean(value); // BooleanType 2768 return value; 2769 case 3076014: // date 2770 this.date = castToDateTime(value); // DateTimeType 2771 return value; 2772 case 1447404028: // publisher 2773 this.publisher = castToString(value); // StringType 2774 return value; 2775 case 951526432: // contact 2776 this.getContact().add(castToContactDetail(value)); // ContactDetail 2777 return value; 2778 case -1724546052: // description 2779 this.description = castToMarkdown(value); // MarkdownType 2780 return value; 2781 case -669707736: // useContext 2782 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2783 return value; 2784 case -507075711: // jurisdiction 2785 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2786 return value; 2787 case -220463842: // purpose 2788 this.purpose = castToMarkdown(value); // MarkdownType 2789 return value; 2790 case 1522889671: // copyright 2791 this.copyright = castToMarkdown(value); // MarkdownType 2792 return value; 2793 case -814408215: // keyword 2794 this.getKeyword().add(castToCoding(value)); // Coding 2795 return value; 2796 case 461006061: // fhirVersion 2797 this.fhirVersion = castToId(value); // IdType 2798 return value; 2799 case 837556430: // mapping 2800 this.getMapping().add((StructureDefinitionMappingComponent) value); // StructureDefinitionMappingComponent 2801 return value; 2802 case 3292052: // kind 2803 value = new StructureDefinitionKindEnumFactory().fromType(castToCode(value)); 2804 this.kind = (Enumeration) value; // Enumeration<StructureDefinitionKind> 2805 return value; 2806 case 1732898850: // abstract 2807 this.abstract_ = castToBoolean(value); // BooleanType 2808 return value; 2809 case -102839927: // contextType 2810 value = new ExtensionContextEnumFactory().fromType(castToCode(value)); 2811 this.contextType = (Enumeration) value; // Enumeration<ExtensionContext> 2812 return value; 2813 case 951530927: // context 2814 this.getContext().add(castToString(value)); // StringType 2815 return value; 2816 case -802505007: // contextInvariant 2817 this.getContextInvariant().add(castToString(value)); // StringType 2818 return value; 2819 case 3575610: // type 2820 this.type = castToCode(value); // CodeType 2821 return value; 2822 case 1139771140: // baseDefinition 2823 this.baseDefinition = castToUri(value); // UriType 2824 return value; 2825 case -1353885513: // derivation 2826 value = new TypeDerivationRuleEnumFactory().fromType(castToCode(value)); 2827 this.derivation = (Enumeration) value; // Enumeration<TypeDerivationRule> 2828 return value; 2829 case 284874180: // snapshot 2830 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 2831 return value; 2832 case -1196150917: // differential 2833 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 2834 return value; 2835 default: return super.setProperty(hash, name, value); 2836 } 2837 2838 } 2839 2840 @Override 2841 public Base setProperty(String name, Base value) throws FHIRException { 2842 if (name.equals("url")) { 2843 this.url = castToUri(value); // UriType 2844 } else if (name.equals("identifier")) { 2845 this.getIdentifier().add(castToIdentifier(value)); 2846 } else if (name.equals("version")) { 2847 this.version = castToString(value); // StringType 2848 } else if (name.equals("name")) { 2849 this.name = castToString(value); // StringType 2850 } else if (name.equals("title")) { 2851 this.title = castToString(value); // StringType 2852 } else if (name.equals("status")) { 2853 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2854 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2855 } else if (name.equals("experimental")) { 2856 this.experimental = castToBoolean(value); // BooleanType 2857 } else if (name.equals("date")) { 2858 this.date = castToDateTime(value); // DateTimeType 2859 } else if (name.equals("publisher")) { 2860 this.publisher = castToString(value); // StringType 2861 } else if (name.equals("contact")) { 2862 this.getContact().add(castToContactDetail(value)); 2863 } else if (name.equals("description")) { 2864 this.description = castToMarkdown(value); // MarkdownType 2865 } else if (name.equals("useContext")) { 2866 this.getUseContext().add(castToUsageContext(value)); 2867 } else if (name.equals("jurisdiction")) { 2868 this.getJurisdiction().add(castToCodeableConcept(value)); 2869 } else if (name.equals("purpose")) { 2870 this.purpose = castToMarkdown(value); // MarkdownType 2871 } else if (name.equals("copyright")) { 2872 this.copyright = castToMarkdown(value); // MarkdownType 2873 } else if (name.equals("keyword")) { 2874 this.getKeyword().add(castToCoding(value)); 2875 } else if (name.equals("fhirVersion")) { 2876 this.fhirVersion = castToId(value); // IdType 2877 } else if (name.equals("mapping")) { 2878 this.getMapping().add((StructureDefinitionMappingComponent) value); 2879 } else if (name.equals("kind")) { 2880 value = new StructureDefinitionKindEnumFactory().fromType(castToCode(value)); 2881 this.kind = (Enumeration) value; // Enumeration<StructureDefinitionKind> 2882 } else if (name.equals("abstract")) { 2883 this.abstract_ = castToBoolean(value); // BooleanType 2884 } else if (name.equals("contextType")) { 2885 value = new ExtensionContextEnumFactory().fromType(castToCode(value)); 2886 this.contextType = (Enumeration) value; // Enumeration<ExtensionContext> 2887 } else if (name.equals("context")) { 2888 this.getContext().add(castToString(value)); 2889 } else if (name.equals("contextInvariant")) { 2890 this.getContextInvariant().add(castToString(value)); 2891 } else if (name.equals("type")) { 2892 this.type = castToCode(value); // CodeType 2893 } else if (name.equals("baseDefinition")) { 2894 this.baseDefinition = castToUri(value); // UriType 2895 } else if (name.equals("derivation")) { 2896 value = new TypeDerivationRuleEnumFactory().fromType(castToCode(value)); 2897 this.derivation = (Enumeration) value; // Enumeration<TypeDerivationRule> 2898 } else if (name.equals("snapshot")) { 2899 this.snapshot = (StructureDefinitionSnapshotComponent) value; // StructureDefinitionSnapshotComponent 2900 } else if (name.equals("differential")) { 2901 this.differential = (StructureDefinitionDifferentialComponent) value; // StructureDefinitionDifferentialComponent 2902 } else 2903 return super.setProperty(name, value); 2904 return value; 2905 } 2906 2907 @Override 2908 public Base makeProperty(int hash, String name) throws FHIRException { 2909 switch (hash) { 2910 case 116079: return getUrlElement(); 2911 case -1618432855: return addIdentifier(); 2912 case 351608024: return getVersionElement(); 2913 case 3373707: return getNameElement(); 2914 case 110371416: return getTitleElement(); 2915 case -892481550: return getStatusElement(); 2916 case -404562712: return getExperimentalElement(); 2917 case 3076014: return getDateElement(); 2918 case 1447404028: return getPublisherElement(); 2919 case 951526432: return addContact(); 2920 case -1724546052: return getDescriptionElement(); 2921 case -669707736: return addUseContext(); 2922 case -507075711: return addJurisdiction(); 2923 case -220463842: return getPurposeElement(); 2924 case 1522889671: return getCopyrightElement(); 2925 case -814408215: return addKeyword(); 2926 case 461006061: return getFhirVersionElement(); 2927 case 837556430: return addMapping(); 2928 case 3292052: return getKindElement(); 2929 case 1732898850: return getAbstractElement(); 2930 case -102839927: return getContextTypeElement(); 2931 case 951530927: return addContextElement(); 2932 case -802505007: return addContextInvariantElement(); 2933 case 3575610: return getTypeElement(); 2934 case 1139771140: return getBaseDefinitionElement(); 2935 case -1353885513: return getDerivationElement(); 2936 case 284874180: return getSnapshot(); 2937 case -1196150917: return getDifferential(); 2938 default: return super.makeProperty(hash, name); 2939 } 2940 2941 } 2942 2943 @Override 2944 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2945 switch (hash) { 2946 case 116079: /*url*/ return new String[] {"uri"}; 2947 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2948 case 351608024: /*version*/ return new String[] {"string"}; 2949 case 3373707: /*name*/ return new String[] {"string"}; 2950 case 110371416: /*title*/ return new String[] {"string"}; 2951 case -892481550: /*status*/ return new String[] {"code"}; 2952 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2953 case 3076014: /*date*/ return new String[] {"dateTime"}; 2954 case 1447404028: /*publisher*/ return new String[] {"string"}; 2955 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2956 case -1724546052: /*description*/ return new String[] {"markdown"}; 2957 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2958 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2959 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2960 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2961 case -814408215: /*keyword*/ return new String[] {"Coding"}; 2962 case 461006061: /*fhirVersion*/ return new String[] {"id"}; 2963 case 837556430: /*mapping*/ return new String[] {}; 2964 case 3292052: /*kind*/ return new String[] {"code"}; 2965 case 1732898850: /*abstract*/ return new String[] {"boolean"}; 2966 case -102839927: /*contextType*/ return new String[] {"code"}; 2967 case 951530927: /*context*/ return new String[] {"string"}; 2968 case -802505007: /*contextInvariant*/ return new String[] {"string"}; 2969 case 3575610: /*type*/ return new String[] {"code"}; 2970 case 1139771140: /*baseDefinition*/ return new String[] {"uri"}; 2971 case -1353885513: /*derivation*/ return new String[] {"code"}; 2972 case 284874180: /*snapshot*/ return new String[] {}; 2973 case -1196150917: /*differential*/ return new String[] {}; 2974 default: return super.getTypesForProperty(hash, name); 2975 } 2976 2977 } 2978 2979 @Override 2980 public Base addChild(String name) throws FHIRException { 2981 if (name.equals("url")) { 2982 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.url"); 2983 } 2984 else if (name.equals("identifier")) { 2985 return addIdentifier(); 2986 } 2987 else if (name.equals("version")) { 2988 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.version"); 2989 } 2990 else if (name.equals("name")) { 2991 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.name"); 2992 } 2993 else if (name.equals("title")) { 2994 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.title"); 2995 } 2996 else if (name.equals("status")) { 2997 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.status"); 2998 } 2999 else if (name.equals("experimental")) { 3000 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.experimental"); 3001 } 3002 else if (name.equals("date")) { 3003 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.date"); 3004 } 3005 else if (name.equals("publisher")) { 3006 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.publisher"); 3007 } 3008 else if (name.equals("contact")) { 3009 return addContact(); 3010 } 3011 else if (name.equals("description")) { 3012 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.description"); 3013 } 3014 else if (name.equals("useContext")) { 3015 return addUseContext(); 3016 } 3017 else if (name.equals("jurisdiction")) { 3018 return addJurisdiction(); 3019 } 3020 else if (name.equals("purpose")) { 3021 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.purpose"); 3022 } 3023 else if (name.equals("copyright")) { 3024 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.copyright"); 3025 } 3026 else if (name.equals("keyword")) { 3027 return addKeyword(); 3028 } 3029 else if (name.equals("fhirVersion")) { 3030 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.fhirVersion"); 3031 } 3032 else if (name.equals("mapping")) { 3033 return addMapping(); 3034 } 3035 else if (name.equals("kind")) { 3036 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.kind"); 3037 } 3038 else if (name.equals("abstract")) { 3039 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.abstract"); 3040 } 3041 else if (name.equals("contextType")) { 3042 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.contextType"); 3043 } 3044 else if (name.equals("context")) { 3045 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.context"); 3046 } 3047 else if (name.equals("contextInvariant")) { 3048 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.contextInvariant"); 3049 } 3050 else if (name.equals("type")) { 3051 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.type"); 3052 } 3053 else if (name.equals("baseDefinition")) { 3054 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.baseDefinition"); 3055 } 3056 else if (name.equals("derivation")) { 3057 throw new FHIRException("Cannot call addChild on a singleton property StructureDefinition.derivation"); 3058 } 3059 else if (name.equals("snapshot")) { 3060 this.snapshot = new StructureDefinitionSnapshotComponent(); 3061 return this.snapshot; 3062 } 3063 else if (name.equals("differential")) { 3064 this.differential = new StructureDefinitionDifferentialComponent(); 3065 return this.differential; 3066 } 3067 else 3068 return super.addChild(name); 3069 } 3070 3071 public String fhirType() { 3072 return "StructureDefinition"; 3073 3074 } 3075 3076 public StructureDefinition copy() { 3077 StructureDefinition dst = new StructureDefinition(); 3078 copyValues(dst); 3079 dst.url = url == null ? null : url.copy(); 3080 if (identifier != null) { 3081 dst.identifier = new ArrayList<Identifier>(); 3082 for (Identifier i : identifier) 3083 dst.identifier.add(i.copy()); 3084 }; 3085 dst.version = version == null ? null : version.copy(); 3086 dst.name = name == null ? null : name.copy(); 3087 dst.title = title == null ? null : title.copy(); 3088 dst.status = status == null ? null : status.copy(); 3089 dst.experimental = experimental == null ? null : experimental.copy(); 3090 dst.date = date == null ? null : date.copy(); 3091 dst.publisher = publisher == null ? null : publisher.copy(); 3092 if (contact != null) { 3093 dst.contact = new ArrayList<ContactDetail>(); 3094 for (ContactDetail i : contact) 3095 dst.contact.add(i.copy()); 3096 }; 3097 dst.description = description == null ? null : description.copy(); 3098 if (useContext != null) { 3099 dst.useContext = new ArrayList<UsageContext>(); 3100 for (UsageContext i : useContext) 3101 dst.useContext.add(i.copy()); 3102 }; 3103 if (jurisdiction != null) { 3104 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3105 for (CodeableConcept i : jurisdiction) 3106 dst.jurisdiction.add(i.copy()); 3107 }; 3108 dst.purpose = purpose == null ? null : purpose.copy(); 3109 dst.copyright = copyright == null ? null : copyright.copy(); 3110 if (keyword != null) { 3111 dst.keyword = new ArrayList<Coding>(); 3112 for (Coding i : keyword) 3113 dst.keyword.add(i.copy()); 3114 }; 3115 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 3116 if (mapping != null) { 3117 dst.mapping = new ArrayList<StructureDefinitionMappingComponent>(); 3118 for (StructureDefinitionMappingComponent i : mapping) 3119 dst.mapping.add(i.copy()); 3120 }; 3121 dst.kind = kind == null ? null : kind.copy(); 3122 dst.abstract_ = abstract_ == null ? null : abstract_.copy(); 3123 dst.contextType = contextType == null ? null : contextType.copy(); 3124 if (context != null) { 3125 dst.context = new ArrayList<StringType>(); 3126 for (StringType i : context) 3127 dst.context.add(i.copy()); 3128 }; 3129 if (contextInvariant != null) { 3130 dst.contextInvariant = new ArrayList<StringType>(); 3131 for (StringType i : contextInvariant) 3132 dst.contextInvariant.add(i.copy()); 3133 }; 3134 dst.type = type == null ? null : type.copy(); 3135 dst.baseDefinition = baseDefinition == null ? null : baseDefinition.copy(); 3136 dst.derivation = derivation == null ? null : derivation.copy(); 3137 dst.snapshot = snapshot == null ? null : snapshot.copy(); 3138 dst.differential = differential == null ? null : differential.copy(); 3139 return dst; 3140 } 3141 3142 protected StructureDefinition typedCopy() { 3143 return copy(); 3144 } 3145 3146 @Override 3147 public boolean equalsDeep(Base other_) { 3148 if (!super.equalsDeep(other_)) 3149 return false; 3150 if (!(other_ instanceof StructureDefinition)) 3151 return false; 3152 StructureDefinition o = (StructureDefinition) other_; 3153 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 3154 && compareDeep(keyword, o.keyword, true) && compareDeep(fhirVersion, o.fhirVersion, true) && compareDeep(mapping, o.mapping, true) 3155 && compareDeep(kind, o.kind, true) && compareDeep(abstract_, o.abstract_, true) && compareDeep(contextType, o.contextType, true) 3156 && compareDeep(context, o.context, true) && compareDeep(contextInvariant, o.contextInvariant, true) 3157 && compareDeep(type, o.type, true) && compareDeep(baseDefinition, o.baseDefinition, true) && compareDeep(derivation, o.derivation, true) 3158 && compareDeep(snapshot, o.snapshot, true) && compareDeep(differential, o.differential, true); 3159 } 3160 3161 @Override 3162 public boolean equalsShallow(Base other_) { 3163 if (!super.equalsShallow(other_)) 3164 return false; 3165 if (!(other_ instanceof StructureDefinition)) 3166 return false; 3167 StructureDefinition o = (StructureDefinition) other_; 3168 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(fhirVersion, o.fhirVersion, true) 3169 && compareValues(kind, o.kind, true) && compareValues(abstract_, o.abstract_, true) && compareValues(contextType, o.contextType, true) 3170 && compareValues(context, o.context, true) && compareValues(contextInvariant, o.contextInvariant, true) 3171 && compareValues(type, o.type, true) && compareValues(baseDefinition, o.baseDefinition, true) && compareValues(derivation, o.derivation, true) 3172 ; 3173 } 3174 3175 public boolean isEmpty() { 3176 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright 3177 , keyword, fhirVersion, mapping, kind, abstract_, contextType, context, contextInvariant 3178 , type, baseDefinition, derivation, snapshot, differential); 3179 } 3180 3181 @Override 3182 public ResourceType getResourceType() { 3183 return ResourceType.StructureDefinition; 3184 } 3185 3186 /** 3187 * Search parameter: <b>date</b> 3188 * <p> 3189 * Description: <b>The structure definition publication date</b><br> 3190 * Type: <b>date</b><br> 3191 * Path: <b>StructureDefinition.date</b><br> 3192 * </p> 3193 */ 3194 @SearchParamDefinition(name="date", path="StructureDefinition.date", description="The structure definition publication date", type="date" ) 3195 public static final String SP_DATE = "date"; 3196 /** 3197 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3198 * <p> 3199 * Description: <b>The structure definition publication date</b><br> 3200 * Type: <b>date</b><br> 3201 * Path: <b>StructureDefinition.date</b><br> 3202 * </p> 3203 */ 3204 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3205 3206 /** 3207 * Search parameter: <b>identifier</b> 3208 * <p> 3209 * Description: <b>External identifier for the structure definition</b><br> 3210 * Type: <b>token</b><br> 3211 * Path: <b>StructureDefinition.identifier</b><br> 3212 * </p> 3213 */ 3214 @SearchParamDefinition(name="identifier", path="StructureDefinition.identifier", description="External identifier for the structure definition", type="token" ) 3215 public static final String SP_IDENTIFIER = "identifier"; 3216 /** 3217 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3218 * <p> 3219 * Description: <b>External identifier for the structure definition</b><br> 3220 * Type: <b>token</b><br> 3221 * Path: <b>StructureDefinition.identifier</b><br> 3222 * </p> 3223 */ 3224 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3225 3226 /** 3227 * Search parameter: <b>valueset</b> 3228 * <p> 3229 * Description: <b>A vocabulary binding reference</b><br> 3230 * Type: <b>reference</b><br> 3231 * Path: <b>StructureDefinition.snapshot.element.binding.valueSet[x]</b><br> 3232 * </p> 3233 */ 3234 @SearchParamDefinition(name="valueset", path="StructureDefinition.snapshot.element.binding.valueSet", description="A vocabulary binding reference", type="reference", target={ValueSet.class } ) 3235 public static final String SP_VALUESET = "valueset"; 3236 /** 3237 * <b>Fluent Client</b> search parameter constant for <b>valueset</b> 3238 * <p> 3239 * Description: <b>A vocabulary binding reference</b><br> 3240 * Type: <b>reference</b><br> 3241 * Path: <b>StructureDefinition.snapshot.element.binding.valueSet[x]</b><br> 3242 * </p> 3243 */ 3244 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam VALUESET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_VALUESET); 3245 3246/** 3247 * Constant for fluent queries to be used to add include statements. Specifies 3248 * the path value of "<b>StructureDefinition:valueset</b>". 3249 */ 3250 public static final ca.uhn.fhir.model.api.Include INCLUDE_VALUESET = new ca.uhn.fhir.model.api.Include("StructureDefinition:valueset").toLocked(); 3251 3252 /** 3253 * Search parameter: <b>kind</b> 3254 * <p> 3255 * Description: <b>primitive-type | complex-type | resource | logical</b><br> 3256 * Type: <b>token</b><br> 3257 * Path: <b>StructureDefinition.kind</b><br> 3258 * </p> 3259 */ 3260 @SearchParamDefinition(name="kind", path="StructureDefinition.kind", description="primitive-type | complex-type | resource | logical", type="token" ) 3261 public static final String SP_KIND = "kind"; 3262 /** 3263 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 3264 * <p> 3265 * Description: <b>primitive-type | complex-type | resource | logical</b><br> 3266 * Type: <b>token</b><br> 3267 * Path: <b>StructureDefinition.kind</b><br> 3268 * </p> 3269 */ 3270 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KIND); 3271 3272 /** 3273 * Search parameter: <b>jurisdiction</b> 3274 * <p> 3275 * Description: <b>Intended jurisdiction for the structure definition</b><br> 3276 * Type: <b>token</b><br> 3277 * Path: <b>StructureDefinition.jurisdiction</b><br> 3278 * </p> 3279 */ 3280 @SearchParamDefinition(name="jurisdiction", path="StructureDefinition.jurisdiction", description="Intended jurisdiction for the structure definition", type="token" ) 3281 public static final String SP_JURISDICTION = "jurisdiction"; 3282 /** 3283 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3284 * <p> 3285 * Description: <b>Intended jurisdiction for the structure definition</b><br> 3286 * Type: <b>token</b><br> 3287 * Path: <b>StructureDefinition.jurisdiction</b><br> 3288 * </p> 3289 */ 3290 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3291 3292 /** 3293 * Search parameter: <b>description</b> 3294 * <p> 3295 * Description: <b>The description of the structure definition</b><br> 3296 * Type: <b>string</b><br> 3297 * Path: <b>StructureDefinition.description</b><br> 3298 * </p> 3299 */ 3300 @SearchParamDefinition(name="description", path="StructureDefinition.description", description="The description of the structure definition", type="string" ) 3301 public static final String SP_DESCRIPTION = "description"; 3302 /** 3303 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3304 * <p> 3305 * Description: <b>The description of the structure definition</b><br> 3306 * Type: <b>string</b><br> 3307 * Path: <b>StructureDefinition.description</b><br> 3308 * </p> 3309 */ 3310 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3311 3312 /** 3313 * Search parameter: <b>experimental</b> 3314 * <p> 3315 * Description: <b>For testing purposes, not real usage</b><br> 3316 * Type: <b>token</b><br> 3317 * Path: <b>StructureDefinition.experimental</b><br> 3318 * </p> 3319 */ 3320 @SearchParamDefinition(name="experimental", path="StructureDefinition.experimental", description="For testing purposes, not real usage", type="token" ) 3321 public static final String SP_EXPERIMENTAL = "experimental"; 3322 /** 3323 * <b>Fluent Client</b> search parameter constant for <b>experimental</b> 3324 * <p> 3325 * Description: <b>For testing purposes, not real usage</b><br> 3326 * Type: <b>token</b><br> 3327 * Path: <b>StructureDefinition.experimental</b><br> 3328 * </p> 3329 */ 3330 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXPERIMENTAL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXPERIMENTAL); 3331 3332 /** 3333 * Search parameter: <b>context-type</b> 3334 * <p> 3335 * Description: <b>resource | datatype | extension</b><br> 3336 * Type: <b>token</b><br> 3337 * Path: <b>StructureDefinition.contextType</b><br> 3338 * </p> 3339 */ 3340 @SearchParamDefinition(name="context-type", path="StructureDefinition.contextType", description="resource | datatype | extension", type="token" ) 3341 public static final String SP_CONTEXT_TYPE = "context-type"; 3342 /** 3343 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3344 * <p> 3345 * Description: <b>resource | datatype | extension</b><br> 3346 * Type: <b>token</b><br> 3347 * Path: <b>StructureDefinition.contextType</b><br> 3348 * </p> 3349 */ 3350 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3351 3352 /** 3353 * Search parameter: <b>abstract</b> 3354 * <p> 3355 * Description: <b>Whether the structure is abstract</b><br> 3356 * Type: <b>token</b><br> 3357 * Path: <b>StructureDefinition.abstract</b><br> 3358 * </p> 3359 */ 3360 @SearchParamDefinition(name="abstract", path="StructureDefinition.abstract", description="Whether the structure is abstract", type="token" ) 3361 public static final String SP_ABSTRACT = "abstract"; 3362 /** 3363 * <b>Fluent Client</b> search parameter constant for <b>abstract</b> 3364 * <p> 3365 * Description: <b>Whether the structure is abstract</b><br> 3366 * Type: <b>token</b><br> 3367 * Path: <b>StructureDefinition.abstract</b><br> 3368 * </p> 3369 */ 3370 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ABSTRACT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ABSTRACT); 3371 3372 /** 3373 * Search parameter: <b>title</b> 3374 * <p> 3375 * Description: <b>The human-friendly name of the structure definition</b><br> 3376 * Type: <b>string</b><br> 3377 * Path: <b>StructureDefinition.title</b><br> 3378 * </p> 3379 */ 3380 @SearchParamDefinition(name="title", path="StructureDefinition.title", description="The human-friendly name of the structure definition", type="string" ) 3381 public static final String SP_TITLE = "title"; 3382 /** 3383 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3384 * <p> 3385 * Description: <b>The human-friendly name of the structure definition</b><br> 3386 * Type: <b>string</b><br> 3387 * Path: <b>StructureDefinition.title</b><br> 3388 * </p> 3389 */ 3390 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 3391 3392 /** 3393 * Search parameter: <b>type</b> 3394 * <p> 3395 * Description: <b>Type defined or constrained by this structure</b><br> 3396 * Type: <b>token</b><br> 3397 * Path: <b>StructureDefinition.type</b><br> 3398 * </p> 3399 */ 3400 @SearchParamDefinition(name="type", path="StructureDefinition.type", description="Type defined or constrained by this structure", type="token" ) 3401 public static final String SP_TYPE = "type"; 3402 /** 3403 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3404 * <p> 3405 * Description: <b>Type defined or constrained by this structure</b><br> 3406 * Type: <b>token</b><br> 3407 * Path: <b>StructureDefinition.type</b><br> 3408 * </p> 3409 */ 3410 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3411 3412 /** 3413 * Search parameter: <b>version</b> 3414 * <p> 3415 * Description: <b>The business version of the structure definition</b><br> 3416 * Type: <b>token</b><br> 3417 * Path: <b>StructureDefinition.version</b><br> 3418 * </p> 3419 */ 3420 @SearchParamDefinition(name="version", path="StructureDefinition.version", description="The business version of the structure definition", type="token" ) 3421 public static final String SP_VERSION = "version"; 3422 /** 3423 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3424 * <p> 3425 * Description: <b>The business version of the structure definition</b><br> 3426 * Type: <b>token</b><br> 3427 * Path: <b>StructureDefinition.version</b><br> 3428 * </p> 3429 */ 3430 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3431 3432 /** 3433 * Search parameter: <b>url</b> 3434 * <p> 3435 * Description: <b>The uri that identifies the structure definition</b><br> 3436 * Type: <b>uri</b><br> 3437 * Path: <b>StructureDefinition.url</b><br> 3438 * </p> 3439 */ 3440 @SearchParamDefinition(name="url", path="StructureDefinition.url", description="The uri that identifies the structure definition", type="uri" ) 3441 public static final String SP_URL = "url"; 3442 /** 3443 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3444 * <p> 3445 * Description: <b>The uri that identifies the structure definition</b><br> 3446 * Type: <b>uri</b><br> 3447 * Path: <b>StructureDefinition.url</b><br> 3448 * </p> 3449 */ 3450 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3451 3452 /** 3453 * Search parameter: <b>path</b> 3454 * <p> 3455 * Description: <b>A path that is constrained in the profile</b><br> 3456 * Type: <b>token</b><br> 3457 * Path: <b>StructureDefinition.snapshot.element.path, StructureDefinition.differential.element.path</b><br> 3458 * </p> 3459 */ 3460 @SearchParamDefinition(name="path", path="StructureDefinition.snapshot.element.path | StructureDefinition.differential.element.path", description="A path that is constrained in the profile", type="token" ) 3461 public static final String SP_PATH = "path"; 3462 /** 3463 * <b>Fluent Client</b> search parameter constant for <b>path</b> 3464 * <p> 3465 * Description: <b>A path that is constrained in the profile</b><br> 3466 * Type: <b>token</b><br> 3467 * Path: <b>StructureDefinition.snapshot.element.path, StructureDefinition.differential.element.path</b><br> 3468 * </p> 3469 */ 3470 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PATH = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PATH); 3471 3472 /** 3473 * Search parameter: <b>ext-context</b> 3474 * <p> 3475 * Description: <b>Where the extension can be used in instances</b><br> 3476 * Type: <b>string</b><br> 3477 * Path: <b>StructureDefinition.context</b><br> 3478 * </p> 3479 */ 3480 @SearchParamDefinition(name="ext-context", path="StructureDefinition.context", description="Where the extension can be used in instances", type="string" ) 3481 public static final String SP_EXT_CONTEXT = "ext-context"; 3482 /** 3483 * <b>Fluent Client</b> search parameter constant for <b>ext-context</b> 3484 * <p> 3485 * Description: <b>Where the extension can be used in instances</b><br> 3486 * Type: <b>string</b><br> 3487 * Path: <b>StructureDefinition.context</b><br> 3488 * </p> 3489 */ 3490 public static final ca.uhn.fhir.rest.gclient.StringClientParam EXT_CONTEXT = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_EXT_CONTEXT); 3491 3492 /** 3493 * Search parameter: <b>name</b> 3494 * <p> 3495 * Description: <b>Computationally friendly name of the structure definition</b><br> 3496 * Type: <b>string</b><br> 3497 * Path: <b>StructureDefinition.name</b><br> 3498 * </p> 3499 */ 3500 @SearchParamDefinition(name="name", path="StructureDefinition.name", description="Computationally friendly name of the structure definition", type="string" ) 3501 public static final String SP_NAME = "name"; 3502 /** 3503 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3504 * <p> 3505 * Description: <b>Computationally friendly name of the structure definition</b><br> 3506 * Type: <b>string</b><br> 3507 * Path: <b>StructureDefinition.name</b><br> 3508 * </p> 3509 */ 3510 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3511 3512 /** 3513 * Search parameter: <b>base-path</b> 3514 * <p> 3515 * Description: <b>Path that identifies the base element</b><br> 3516 * Type: <b>token</b><br> 3517 * Path: <b>StructureDefinition.snapshot.element.base.path, StructureDefinition.differential.element.base.path</b><br> 3518 * </p> 3519 */ 3520 @SearchParamDefinition(name="base-path", path="StructureDefinition.snapshot.element.base.path | StructureDefinition.differential.element.base.path", description="Path that identifies the base element", type="token" ) 3521 public static final String SP_BASE_PATH = "base-path"; 3522 /** 3523 * <b>Fluent Client</b> search parameter constant for <b>base-path</b> 3524 * <p> 3525 * Description: <b>Path that identifies the base element</b><br> 3526 * Type: <b>token</b><br> 3527 * Path: <b>StructureDefinition.snapshot.element.base.path, StructureDefinition.differential.element.base.path</b><br> 3528 * </p> 3529 */ 3530 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BASE_PATH = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BASE_PATH); 3531 3532 /** 3533 * Search parameter: <b>publisher</b> 3534 * <p> 3535 * Description: <b>Name of the publisher of the structure definition</b><br> 3536 * Type: <b>string</b><br> 3537 * Path: <b>StructureDefinition.publisher</b><br> 3538 * </p> 3539 */ 3540 @SearchParamDefinition(name="publisher", path="StructureDefinition.publisher", description="Name of the publisher of the structure definition", type="string" ) 3541 public static final String SP_PUBLISHER = "publisher"; 3542 /** 3543 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3544 * <p> 3545 * Description: <b>Name of the publisher of the structure definition</b><br> 3546 * Type: <b>string</b><br> 3547 * Path: <b>StructureDefinition.publisher</b><br> 3548 * </p> 3549 */ 3550 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3551 3552 /** 3553 * Search parameter: <b>derivation</b> 3554 * <p> 3555 * Description: <b>specialization | constraint - How relates to base definition</b><br> 3556 * Type: <b>token</b><br> 3557 * Path: <b>StructureDefinition.derivation</b><br> 3558 * </p> 3559 */ 3560 @SearchParamDefinition(name="derivation", path="StructureDefinition.derivation", description="specialization | constraint - How relates to base definition", type="token" ) 3561 public static final String SP_DERIVATION = "derivation"; 3562 /** 3563 * <b>Fluent Client</b> search parameter constant for <b>derivation</b> 3564 * <p> 3565 * Description: <b>specialization | constraint - How relates to base definition</b><br> 3566 * Type: <b>token</b><br> 3567 * Path: <b>StructureDefinition.derivation</b><br> 3568 * </p> 3569 */ 3570 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DERIVATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DERIVATION); 3571 3572 /** 3573 * Search parameter: <b>keyword</b> 3574 * <p> 3575 * Description: <b>A code for the profile</b><br> 3576 * Type: <b>token</b><br> 3577 * Path: <b>StructureDefinition.keyword</b><br> 3578 * </p> 3579 */ 3580 @SearchParamDefinition(name="keyword", path="StructureDefinition.keyword", description="A code for the profile", type="token" ) 3581 public static final String SP_KEYWORD = "keyword"; 3582 /** 3583 * <b>Fluent Client</b> search parameter constant for <b>keyword</b> 3584 * <p> 3585 * Description: <b>A code for the profile</b><br> 3586 * Type: <b>token</b><br> 3587 * Path: <b>StructureDefinition.keyword</b><br> 3588 * </p> 3589 */ 3590 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KEYWORD = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_KEYWORD); 3591 3592 /** 3593 * Search parameter: <b>status</b> 3594 * <p> 3595 * Description: <b>The current status of the structure definition</b><br> 3596 * Type: <b>token</b><br> 3597 * Path: <b>StructureDefinition.status</b><br> 3598 * </p> 3599 */ 3600 @SearchParamDefinition(name="status", path="StructureDefinition.status", description="The current status of the structure definition", type="token" ) 3601 public static final String SP_STATUS = "status"; 3602 /** 3603 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3604 * <p> 3605 * Description: <b>The current status of the structure definition</b><br> 3606 * Type: <b>token</b><br> 3607 * Path: <b>StructureDefinition.status</b><br> 3608 * </p> 3609 */ 3610 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3611 3612 /** 3613 * Search parameter: <b>base</b> 3614 * <p> 3615 * Description: <b>Definition that this type is constrained/specialized from</b><br> 3616 * Type: <b>uri</b><br> 3617 * Path: <b>StructureDefinition.baseDefinition</b><br> 3618 * </p> 3619 */ 3620 @SearchParamDefinition(name="base", path="StructureDefinition.baseDefinition", description="Definition that this type is constrained/specialized from", type="uri" ) 3621 public static final String SP_BASE = "base"; 3622 /** 3623 * <b>Fluent Client</b> search parameter constant for <b>base</b> 3624 * <p> 3625 * Description: <b>Definition that this type is constrained/specialized from</b><br> 3626 * Type: <b>uri</b><br> 3627 * Path: <b>StructureDefinition.baseDefinition</b><br> 3628 * </p> 3629 */ 3630 public static final ca.uhn.fhir.rest.gclient.UriClientParam BASE = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_BASE); 3631 3632 3633}