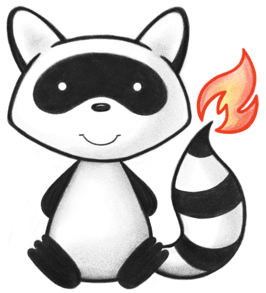
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041// added from java-adornments.txt: 042import org.hl7.fhir.dstu3.utils.StructureMapUtilities; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.exceptions.FHIRFormatError; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import org.hl7.fhir.utilities.Utilities; 047 048import ca.uhn.fhir.model.api.annotation.Block; 049import ca.uhn.fhir.model.api.annotation.Child; 050import ca.uhn.fhir.model.api.annotation.ChildOrder; 051import ca.uhn.fhir.model.api.annotation.Description; 052import ca.uhn.fhir.model.api.annotation.ResourceDef; 053import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 054 055// end addition 056/** 057 * A Map of relationships between 2 structures that can be used to transform data. 058 */ 059@ResourceDef(name="StructureMap", profile="http://hl7.org/fhir/Profile/StructureMap") 060@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "structure", "import", "group"}) 061public class StructureMap extends MetadataResource { 062 063 public enum StructureMapModelMode { 064 /** 065 * This structure describes an instance passed to the mapping engine that is used a source of data 066 */ 067 SOURCE, 068 /** 069 * This structure describes an instance that the mapping engine may ask for that is used a source of data 070 */ 071 QUERIED, 072 /** 073 * This structure describes an instance passed to the mapping engine that is used a target of data 074 */ 075 TARGET, 076 /** 077 * This structure describes an instance that the mapping engine may ask to create that is used a target of data 078 */ 079 PRODUCED, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 public static StructureMapModelMode fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("source".equals(codeString)) 088 return SOURCE; 089 if ("queried".equals(codeString)) 090 return QUERIED; 091 if ("target".equals(codeString)) 092 return TARGET; 093 if ("produced".equals(codeString)) 094 return PRODUCED; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown StructureMapModelMode code '"+codeString+"'"); 099 } 100 public String toCode() { 101 switch (this) { 102 case SOURCE: return "source"; 103 case QUERIED: return "queried"; 104 case TARGET: return "target"; 105 case PRODUCED: return "produced"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case SOURCE: return "http://hl7.org/fhir/map-model-mode"; 113 case QUERIED: return "http://hl7.org/fhir/map-model-mode"; 114 case TARGET: return "http://hl7.org/fhir/map-model-mode"; 115 case PRODUCED: return "http://hl7.org/fhir/map-model-mode"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getDefinition() { 121 switch (this) { 122 case SOURCE: return "This structure describes an instance passed to the mapping engine that is used a source of data"; 123 case QUERIED: return "This structure describes an instance that the mapping engine may ask for that is used a source of data"; 124 case TARGET: return "This structure describes an instance passed to the mapping engine that is used a target of data"; 125 case PRODUCED: return "This structure describes an instance that the mapping engine may ask to create that is used a target of data"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case SOURCE: return "Source Structure Definition"; 133 case QUERIED: return "Queried Structure Definition"; 134 case TARGET: return "Target Structure Definition"; 135 case PRODUCED: return "Produced Structure Definition"; 136 case NULL: return null; 137 default: return "?"; 138 } 139 } 140 } 141 142 public static class StructureMapModelModeEnumFactory implements EnumFactory<StructureMapModelMode> { 143 public StructureMapModelMode fromCode(String codeString) throws IllegalArgumentException { 144 if (codeString == null || "".equals(codeString)) 145 if (codeString == null || "".equals(codeString)) 146 return null; 147 if ("source".equals(codeString)) 148 return StructureMapModelMode.SOURCE; 149 if ("queried".equals(codeString)) 150 return StructureMapModelMode.QUERIED; 151 if ("target".equals(codeString)) 152 return StructureMapModelMode.TARGET; 153 if ("produced".equals(codeString)) 154 return StructureMapModelMode.PRODUCED; 155 throw new IllegalArgumentException("Unknown StructureMapModelMode code '"+codeString+"'"); 156 } 157 public Enumeration<StructureMapModelMode> fromType(PrimitiveType<?> code) throws FHIRException { 158 if (code == null) 159 return null; 160 if (code.isEmpty()) 161 return new Enumeration<StructureMapModelMode>(this); 162 String codeString = code.asStringValue(); 163 if (codeString == null || "".equals(codeString)) 164 return null; 165 if ("source".equals(codeString)) 166 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.SOURCE); 167 if ("queried".equals(codeString)) 168 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.QUERIED); 169 if ("target".equals(codeString)) 170 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.TARGET); 171 if ("produced".equals(codeString)) 172 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.PRODUCED); 173 throw new FHIRException("Unknown StructureMapModelMode code '"+codeString+"'"); 174 } 175 public String toCode(StructureMapModelMode code) { 176 if (code == StructureMapModelMode.NULL) 177 return null; 178 if (code == StructureMapModelMode.SOURCE) 179 return "source"; 180 if (code == StructureMapModelMode.QUERIED) 181 return "queried"; 182 if (code == StructureMapModelMode.TARGET) 183 return "target"; 184 if (code == StructureMapModelMode.PRODUCED) 185 return "produced"; 186 return "?"; 187 } 188 public String toSystem(StructureMapModelMode code) { 189 return code.getSystem(); 190 } 191 } 192 193 public enum StructureMapGroupTypeMode { 194 /** 195 * This group is not a default group for the types 196 */ 197 NONE, 198 /** 199 * This group is a default mapping group for the specified types and for the primary source type 200 */ 201 TYPES, 202 /** 203 * This group is a default mapping group for the specified types 204 */ 205 TYPEANDTYPES, 206 /** 207 * added to help the parsers with the generic types 208 */ 209 NULL; 210 public static StructureMapGroupTypeMode fromCode(String codeString) throws FHIRException { 211 if (codeString == null || "".equals(codeString)) 212 return null; 213 if ("none".equals(codeString)) 214 return NONE; 215 if ("types".equals(codeString)) 216 return TYPES; 217 if ("type-and-types".equals(codeString)) 218 return TYPEANDTYPES; 219 if (Configuration.isAcceptInvalidEnums()) 220 return null; 221 else 222 throw new FHIRException("Unknown StructureMapGroupTypeMode code '"+codeString+"'"); 223 } 224 public String toCode() { 225 switch (this) { 226 case NONE: return "none"; 227 case TYPES: return "types"; 228 case TYPEANDTYPES: return "type-and-types"; 229 case NULL: return null; 230 default: return "?"; 231 } 232 } 233 public String getSystem() { 234 switch (this) { 235 case NONE: return "http://hl7.org/fhir/map-group-type-mode"; 236 case TYPES: return "http://hl7.org/fhir/map-group-type-mode"; 237 case TYPEANDTYPES: return "http://hl7.org/fhir/map-group-type-mode"; 238 case NULL: return null; 239 default: return "?"; 240 } 241 } 242 public String getDefinition() { 243 switch (this) { 244 case NONE: return "This group is not a default group for the types"; 245 case TYPES: return "This group is a default mapping group for the specified types and for the primary source type"; 246 case TYPEANDTYPES: return "This group is a default mapping group for the specified types"; 247 case NULL: return null; 248 default: return "?"; 249 } 250 } 251 public String getDisplay() { 252 switch (this) { 253 case NONE: return "Not a Default"; 254 case TYPES: return "Default for Type Combination"; 255 case TYPEANDTYPES: return "Default for type + combination"; 256 case NULL: return null; 257 default: return "?"; 258 } 259 } 260 } 261 262 public static class StructureMapGroupTypeModeEnumFactory implements EnumFactory<StructureMapGroupTypeMode> { 263 public StructureMapGroupTypeMode fromCode(String codeString) throws IllegalArgumentException { 264 if (codeString == null || "".equals(codeString)) 265 if (codeString == null || "".equals(codeString)) 266 return null; 267 if ("none".equals(codeString)) 268 return StructureMapGroupTypeMode.NONE; 269 if ("types".equals(codeString)) 270 return StructureMapGroupTypeMode.TYPES; 271 if ("type-and-types".equals(codeString)) 272 return StructureMapGroupTypeMode.TYPEANDTYPES; 273 throw new IllegalArgumentException("Unknown StructureMapGroupTypeMode code '"+codeString+"'"); 274 } 275 public Enumeration<StructureMapGroupTypeMode> fromType(PrimitiveType<?> code) throws FHIRException { 276 if (code == null) 277 return null; 278 if (code.isEmpty()) 279 return new Enumeration<StructureMapGroupTypeMode>(this); 280 String codeString = code.asStringValue(); 281 if (codeString == null || "".equals(codeString)) 282 return null; 283 if ("none".equals(codeString)) 284 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.NONE); 285 if ("types".equals(codeString)) 286 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.TYPES); 287 if ("type-and-types".equals(codeString)) 288 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.TYPEANDTYPES); 289 throw new FHIRException("Unknown StructureMapGroupTypeMode code '"+codeString+"'"); 290 } 291 public String toCode(StructureMapGroupTypeMode code) { 292 if (code == StructureMapGroupTypeMode.NULL) 293 return null; 294 if (code == StructureMapGroupTypeMode.NONE) 295 return "none"; 296 if (code == StructureMapGroupTypeMode.TYPES) 297 return "types"; 298 if (code == StructureMapGroupTypeMode.TYPEANDTYPES) 299 return "type-and-types"; 300 return "?"; 301 } 302 public String toSystem(StructureMapGroupTypeMode code) { 303 return code.getSystem(); 304 } 305 } 306 307 public enum StructureMapInputMode { 308 /** 309 * Names an input instance used a source for mapping 310 */ 311 SOURCE, 312 /** 313 * Names an instance that is being populated 314 */ 315 TARGET, 316 /** 317 * added to help the parsers with the generic types 318 */ 319 NULL; 320 public static StructureMapInputMode fromCode(String codeString) throws FHIRException { 321 if (codeString == null || "".equals(codeString)) 322 return null; 323 if ("source".equals(codeString)) 324 return SOURCE; 325 if ("target".equals(codeString)) 326 return TARGET; 327 if (Configuration.isAcceptInvalidEnums()) 328 return null; 329 else 330 throw new FHIRException("Unknown StructureMapInputMode code '"+codeString+"'"); 331 } 332 public String toCode() { 333 switch (this) { 334 case SOURCE: return "source"; 335 case TARGET: return "target"; 336 case NULL: return null; 337 default: return "?"; 338 } 339 } 340 public String getSystem() { 341 switch (this) { 342 case SOURCE: return "http://hl7.org/fhir/map-input-mode"; 343 case TARGET: return "http://hl7.org/fhir/map-input-mode"; 344 case NULL: return null; 345 default: return "?"; 346 } 347 } 348 public String getDefinition() { 349 switch (this) { 350 case SOURCE: return "Names an input instance used a source for mapping"; 351 case TARGET: return "Names an instance that is being populated"; 352 case NULL: return null; 353 default: return "?"; 354 } 355 } 356 public String getDisplay() { 357 switch (this) { 358 case SOURCE: return "Source Instance"; 359 case TARGET: return "Target Instance"; 360 case NULL: return null; 361 default: return "?"; 362 } 363 } 364 } 365 366 public static class StructureMapInputModeEnumFactory implements EnumFactory<StructureMapInputMode> { 367 public StructureMapInputMode fromCode(String codeString) throws IllegalArgumentException { 368 if (codeString == null || "".equals(codeString)) 369 if (codeString == null || "".equals(codeString)) 370 return null; 371 if ("source".equals(codeString)) 372 return StructureMapInputMode.SOURCE; 373 if ("target".equals(codeString)) 374 return StructureMapInputMode.TARGET; 375 throw new IllegalArgumentException("Unknown StructureMapInputMode code '"+codeString+"'"); 376 } 377 public Enumeration<StructureMapInputMode> fromType(PrimitiveType<?> code) throws FHIRException { 378 if (code == null) 379 return null; 380 if (code.isEmpty()) 381 return new Enumeration<StructureMapInputMode>(this); 382 String codeString = code.asStringValue(); 383 if (codeString == null || "".equals(codeString)) 384 return null; 385 if ("source".equals(codeString)) 386 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.SOURCE); 387 if ("target".equals(codeString)) 388 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.TARGET); 389 throw new FHIRException("Unknown StructureMapInputMode code '"+codeString+"'"); 390 } 391 public String toCode(StructureMapInputMode code) { 392 if (code == StructureMapInputMode.NULL) 393 return null; 394 if (code == StructureMapInputMode.SOURCE) 395 return "source"; 396 if (code == StructureMapInputMode.TARGET) 397 return "target"; 398 return "?"; 399 } 400 public String toSystem(StructureMapInputMode code) { 401 return code.getSystem(); 402 } 403 } 404 405 public enum StructureMapSourceListMode { 406 /** 407 * Only process this rule for the first in the list 408 */ 409 FIRST, 410 /** 411 * Process this rule for all but the first 412 */ 413 NOTFIRST, 414 /** 415 * Only process this rule for the last in the list 416 */ 417 LAST, 418 /** 419 * Process this rule for all but the last 420 */ 421 NOTLAST, 422 /** 423 * Only process this rule is there is only item 424 */ 425 ONLYONE, 426 /** 427 * added to help the parsers with the generic types 428 */ 429 NULL; 430 public static StructureMapSourceListMode fromCode(String codeString) throws FHIRException { 431 if (codeString == null || "".equals(codeString)) 432 return null; 433 if ("first".equals(codeString)) 434 return FIRST; 435 if ("not_first".equals(codeString)) 436 return NOTFIRST; 437 if ("last".equals(codeString)) 438 return LAST; 439 if ("not_last".equals(codeString)) 440 return NOTLAST; 441 if ("only_one".equals(codeString)) 442 return ONLYONE; 443 if (Configuration.isAcceptInvalidEnums()) 444 return null; 445 else 446 throw new FHIRException("Unknown StructureMapSourceListMode code '"+codeString+"'"); 447 } 448 public String toCode() { 449 switch (this) { 450 case FIRST: return "first"; 451 case NOTFIRST: return "not_first"; 452 case LAST: return "last"; 453 case NOTLAST: return "not_last"; 454 case ONLYONE: return "only_one"; 455 case NULL: return null; 456 default: return "?"; 457 } 458 } 459 public String getSystem() { 460 switch (this) { 461 case FIRST: return "http://hl7.org/fhir/map-source-list-mode"; 462 case NOTFIRST: return "http://hl7.org/fhir/map-source-list-mode"; 463 case LAST: return "http://hl7.org/fhir/map-source-list-mode"; 464 case NOTLAST: return "http://hl7.org/fhir/map-source-list-mode"; 465 case ONLYONE: return "http://hl7.org/fhir/map-source-list-mode"; 466 case NULL: return null; 467 default: return "?"; 468 } 469 } 470 public String getDefinition() { 471 switch (this) { 472 case FIRST: return "Only process this rule for the first in the list"; 473 case NOTFIRST: return "Process this rule for all but the first"; 474 case LAST: return "Only process this rule for the last in the list"; 475 case NOTLAST: return "Process this rule for all but the last"; 476 case ONLYONE: return "Only process this rule is there is only item"; 477 case NULL: return null; 478 default: return "?"; 479 } 480 } 481 public String getDisplay() { 482 switch (this) { 483 case FIRST: return "First"; 484 case NOTFIRST: return "All but the first"; 485 case LAST: return "Last"; 486 case NOTLAST: return "All but the last"; 487 case ONLYONE: return "Enforce only one"; 488 case NULL: return null; 489 default: return "?"; 490 } 491 } 492 } 493 494 public static class StructureMapSourceListModeEnumFactory implements EnumFactory<StructureMapSourceListMode> { 495 public StructureMapSourceListMode fromCode(String codeString) throws IllegalArgumentException { 496 if (codeString == null || "".equals(codeString)) 497 if (codeString == null || "".equals(codeString)) 498 return null; 499 if ("first".equals(codeString)) 500 return StructureMapSourceListMode.FIRST; 501 if ("not_first".equals(codeString)) 502 return StructureMapSourceListMode.NOTFIRST; 503 if ("last".equals(codeString)) 504 return StructureMapSourceListMode.LAST; 505 if ("not_last".equals(codeString)) 506 return StructureMapSourceListMode.NOTLAST; 507 if ("only_one".equals(codeString)) 508 return StructureMapSourceListMode.ONLYONE; 509 throw new IllegalArgumentException("Unknown StructureMapSourceListMode code '"+codeString+"'"); 510 } 511 public Enumeration<StructureMapSourceListMode> fromType(PrimitiveType<?> code) throws FHIRException { 512 if (code == null) 513 return null; 514 if (code.isEmpty()) 515 return new Enumeration<StructureMapSourceListMode>(this); 516 String codeString = code.asStringValue(); 517 if (codeString == null || "".equals(codeString)) 518 return null; 519 if ("first".equals(codeString)) 520 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.FIRST); 521 if ("not_first".equals(codeString)) 522 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NOTFIRST); 523 if ("last".equals(codeString)) 524 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.LAST); 525 if ("not_last".equals(codeString)) 526 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NOTLAST); 527 if ("only_one".equals(codeString)) 528 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.ONLYONE); 529 throw new FHIRException("Unknown StructureMapSourceListMode code '"+codeString+"'"); 530 } 531 public String toCode(StructureMapSourceListMode code) { 532 if (code == StructureMapSourceListMode.NULL) 533 return null; 534 if (code == StructureMapSourceListMode.FIRST) 535 return "first"; 536 if (code == StructureMapSourceListMode.NOTFIRST) 537 return "not_first"; 538 if (code == StructureMapSourceListMode.LAST) 539 return "last"; 540 if (code == StructureMapSourceListMode.NOTLAST) 541 return "not_last"; 542 if (code == StructureMapSourceListMode.ONLYONE) 543 return "only_one"; 544 return "?"; 545 } 546 public String toSystem(StructureMapSourceListMode code) { 547 return code.getSystem(); 548 } 549 } 550 551 public enum StructureMapContextType { 552 /** 553 * The context specifies a type 554 */ 555 TYPE, 556 /** 557 * The context specifies a variable 558 */ 559 VARIABLE, 560 /** 561 * added to help the parsers with the generic types 562 */ 563 NULL; 564 public static StructureMapContextType fromCode(String codeString) throws FHIRException { 565 if (codeString == null || "".equals(codeString)) 566 return null; 567 if ("type".equals(codeString)) 568 return TYPE; 569 if ("variable".equals(codeString)) 570 return VARIABLE; 571 if (Configuration.isAcceptInvalidEnums()) 572 return null; 573 else 574 throw new FHIRException("Unknown StructureMapContextType code '"+codeString+"'"); 575 } 576 public String toCode() { 577 switch (this) { 578 case TYPE: return "type"; 579 case VARIABLE: return "variable"; 580 case NULL: return null; 581 default: return "?"; 582 } 583 } 584 public String getSystem() { 585 switch (this) { 586 case TYPE: return "http://hl7.org/fhir/map-context-type"; 587 case VARIABLE: return "http://hl7.org/fhir/map-context-type"; 588 case NULL: return null; 589 default: return "?"; 590 } 591 } 592 public String getDefinition() { 593 switch (this) { 594 case TYPE: return "The context specifies a type"; 595 case VARIABLE: return "The context specifies a variable"; 596 case NULL: return null; 597 default: return "?"; 598 } 599 } 600 public String getDisplay() { 601 switch (this) { 602 case TYPE: return "Type"; 603 case VARIABLE: return "Variable"; 604 case NULL: return null; 605 default: return "?"; 606 } 607 } 608 } 609 610 public static class StructureMapContextTypeEnumFactory implements EnumFactory<StructureMapContextType> { 611 public StructureMapContextType fromCode(String codeString) throws IllegalArgumentException { 612 if (codeString == null || "".equals(codeString)) 613 if (codeString == null || "".equals(codeString)) 614 return null; 615 if ("type".equals(codeString)) 616 return StructureMapContextType.TYPE; 617 if ("variable".equals(codeString)) 618 return StructureMapContextType.VARIABLE; 619 throw new IllegalArgumentException("Unknown StructureMapContextType code '"+codeString+"'"); 620 } 621 public Enumeration<StructureMapContextType> fromType(PrimitiveType<?> code) throws FHIRException { 622 if (code == null) 623 return null; 624 if (code.isEmpty()) 625 return new Enumeration<StructureMapContextType>(this); 626 String codeString = code.asStringValue(); 627 if (codeString == null || "".equals(codeString)) 628 return null; 629 if ("type".equals(codeString)) 630 return new Enumeration<StructureMapContextType>(this, StructureMapContextType.TYPE); 631 if ("variable".equals(codeString)) 632 return new Enumeration<StructureMapContextType>(this, StructureMapContextType.VARIABLE); 633 throw new FHIRException("Unknown StructureMapContextType code '"+codeString+"'"); 634 } 635 public String toCode(StructureMapContextType code) { 636 if (code == StructureMapContextType.NULL) 637 return null; 638 if (code == StructureMapContextType.TYPE) 639 return "type"; 640 if (code == StructureMapContextType.VARIABLE) 641 return "variable"; 642 return "?"; 643 } 644 public String toSystem(StructureMapContextType code) { 645 return code.getSystem(); 646 } 647 } 648 649 public enum StructureMapTargetListMode { 650 /** 651 * when the target list is being assembled, the items for this rule go first. If more that one rule defines a first item (for a given instance of mapping) then this is an error 652 */ 653 FIRST, 654 /** 655 * the target instance is shared with the target instances generated by another rule (up to the first common n items, then create new ones) 656 */ 657 SHARE, 658 /** 659 * when the target list is being assembled, the items for this rule go last. If more that one rule defines a last item (for a given instance of mapping) then this is an error 660 */ 661 LAST, 662 /** 663 * re-use the first item in the list, and keep adding content to it 664 */ 665 COLLATE, 666 /** 667 * added to help the parsers with the generic types 668 */ 669 NULL; 670 public static StructureMapTargetListMode fromCode(String codeString) throws FHIRException { 671 if (codeString == null || "".equals(codeString)) 672 return null; 673 if ("first".equals(codeString)) 674 return FIRST; 675 if ("share".equals(codeString)) 676 return SHARE; 677 if ("last".equals(codeString)) 678 return LAST; 679 if ("collate".equals(codeString)) 680 return COLLATE; 681 if (Configuration.isAcceptInvalidEnums()) 682 return null; 683 else 684 throw new FHIRException("Unknown StructureMapTargetListMode code '"+codeString+"'"); 685 } 686 public String toCode() { 687 switch (this) { 688 case FIRST: return "first"; 689 case SHARE: return "share"; 690 case LAST: return "last"; 691 case COLLATE: return "collate"; 692 case NULL: return null; 693 default: return "?"; 694 } 695 } 696 public String getSystem() { 697 switch (this) { 698 case FIRST: return "http://hl7.org/fhir/map-target-list-mode"; 699 case SHARE: return "http://hl7.org/fhir/map-target-list-mode"; 700 case LAST: return "http://hl7.org/fhir/map-target-list-mode"; 701 case COLLATE: return "http://hl7.org/fhir/map-target-list-mode"; 702 case NULL: return null; 703 default: return "?"; 704 } 705 } 706 public String getDefinition() { 707 switch (this) { 708 case FIRST: return "when the target list is being assembled, the items for this rule go first. If more that one rule defines a first item (for a given instance of mapping) then this is an error"; 709 case SHARE: return "the target instance is shared with the target instances generated by another rule (up to the first common n items, then create new ones)"; 710 case LAST: return "when the target list is being assembled, the items for this rule go last. If more that one rule defines a last item (for a given instance of mapping) then this is an error"; 711 case COLLATE: return "re-use the first item in the list, and keep adding content to it"; 712 case NULL: return null; 713 default: return "?"; 714 } 715 } 716 public String getDisplay() { 717 switch (this) { 718 case FIRST: return "First"; 719 case SHARE: return "Share"; 720 case LAST: return "Last"; 721 case COLLATE: return "Collate"; 722 case NULL: return null; 723 default: return "?"; 724 } 725 } 726 } 727 728 public static class StructureMapTargetListModeEnumFactory implements EnumFactory<StructureMapTargetListMode> { 729 public StructureMapTargetListMode fromCode(String codeString) throws IllegalArgumentException { 730 if (codeString == null || "".equals(codeString)) 731 if (codeString == null || "".equals(codeString)) 732 return null; 733 if ("first".equals(codeString)) 734 return StructureMapTargetListMode.FIRST; 735 if ("share".equals(codeString)) 736 return StructureMapTargetListMode.SHARE; 737 if ("last".equals(codeString)) 738 return StructureMapTargetListMode.LAST; 739 if ("collate".equals(codeString)) 740 return StructureMapTargetListMode.COLLATE; 741 throw new IllegalArgumentException("Unknown StructureMapTargetListMode code '"+codeString+"'"); 742 } 743 public Enumeration<StructureMapTargetListMode> fromType(PrimitiveType<?> code) throws FHIRException { 744 if (code == null) 745 return null; 746 if (code.isEmpty()) 747 return new Enumeration<StructureMapTargetListMode>(this); 748 String codeString = code.asStringValue(); 749 if (codeString == null || "".equals(codeString)) 750 return null; 751 if ("first".equals(codeString)) 752 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.FIRST); 753 if ("share".equals(codeString)) 754 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.SHARE); 755 if ("last".equals(codeString)) 756 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.LAST); 757 if ("collate".equals(codeString)) 758 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.COLLATE); 759 throw new FHIRException("Unknown StructureMapTargetListMode code '"+codeString+"'"); 760 } 761 public String toCode(StructureMapTargetListMode code) { 762 if (code == StructureMapTargetListMode.NULL) 763 return null; 764 if (code == StructureMapTargetListMode.FIRST) 765 return "first"; 766 if (code == StructureMapTargetListMode.SHARE) 767 return "share"; 768 if (code == StructureMapTargetListMode.LAST) 769 return "last"; 770 if (code == StructureMapTargetListMode.COLLATE) 771 return "collate"; 772 return "?"; 773 } 774 public String toSystem(StructureMapTargetListMode code) { 775 return code.getSystem(); 776 } 777 } 778 779 public enum StructureMapTransform { 780 /** 781 * create(type : string) - type is passed through to the application on the standard API, and must be known by it 782 */ 783 CREATE, 784 /** 785 * copy(source) 786 */ 787 COPY, 788 /** 789 * truncate(source, length) - source must be stringy type 790 */ 791 TRUNCATE, 792 /** 793 * escape(source, fmt1, fmt2) - change source from one kind of escaping to another (plain, java, xml, json). note that this is for when the string itself is escaped 794 */ 795 ESCAPE, 796 /** 797 * cast(source, type?) - case source from one type to another. target type can be left as implicit if there is one and only one target type known 798 */ 799 CAST, 800 /** 801 * append(source...) - source is element or string 802 */ 803 APPEND, 804 /** 805 * translate(source, uri_of_map) - use the translate operation 806 */ 807 TRANSLATE, 808 /** 809 * reference(source : object) - return a string that references the provided tree properly 810 */ 811 REFERENCE, 812 /** 813 * Perform a date operation. *Parameters to be documented* 814 */ 815 DATEOP, 816 /** 817 * Generate a random UUID (in lowercase). No Parameters 818 */ 819 UUID, 820 /** 821 * Return the appropriate string to put in a reference that refers to the resource provided as a parameter 822 */ 823 POINTER, 824 /** 825 * Execute the supplied fluentpath expression and use the value returned by that 826 */ 827 EVALUATE, 828 /** 829 * Create a CodeableConcept. Parameters = (text) or (system. Code[, display]) 830 */ 831 CC, 832 /** 833 * Create a Coding. Parameters = (system. Code[, display]) 834 */ 835 C, 836 /** 837 * Create a quantity. Parameters = (text) or (value, unit, [system, code]) where text is the natural representation e.g. [comparator]value[space]unit 838 */ 839 QTY, 840 /** 841 * Create an identifier. Parameters = (system, value[, type]) where type is a code from the identifier type value set 842 */ 843 ID, 844 /** 845 * Create a contact details. Parameters = (value) or (system, value). If no system is provided, the system should be inferred from the content of the value 846 */ 847 CP, 848 /** 849 * added to help the parsers with the generic types 850 */ 851 NULL; 852 public static StructureMapTransform fromCode(String codeString) throws FHIRException { 853 if (codeString == null || "".equals(codeString)) 854 return null; 855 if ("create".equals(codeString)) 856 return CREATE; 857 if ("copy".equals(codeString)) 858 return COPY; 859 if ("truncate".equals(codeString)) 860 return TRUNCATE; 861 if ("escape".equals(codeString)) 862 return ESCAPE; 863 if ("cast".equals(codeString)) 864 return CAST; 865 if ("append".equals(codeString)) 866 return APPEND; 867 if ("translate".equals(codeString)) 868 return TRANSLATE; 869 if ("reference".equals(codeString)) 870 return REFERENCE; 871 if ("dateOp".equals(codeString)) 872 return DATEOP; 873 if ("uuid".equals(codeString)) 874 return UUID; 875 if ("pointer".equals(codeString)) 876 return POINTER; 877 if ("evaluate".equals(codeString)) 878 return EVALUATE; 879 if ("cc".equals(codeString)) 880 return CC; 881 if ("c".equals(codeString)) 882 return C; 883 if ("qty".equals(codeString)) 884 return QTY; 885 if ("id".equals(codeString)) 886 return ID; 887 if ("cp".equals(codeString)) 888 return CP; 889 if (Configuration.isAcceptInvalidEnums()) 890 return null; 891 else 892 throw new FHIRException("Unknown StructureMapTransform code '"+codeString+"'"); 893 } 894 public String toCode() { 895 switch (this) { 896 case CREATE: return "create"; 897 case COPY: return "copy"; 898 case TRUNCATE: return "truncate"; 899 case ESCAPE: return "escape"; 900 case CAST: return "cast"; 901 case APPEND: return "append"; 902 case TRANSLATE: return "translate"; 903 case REFERENCE: return "reference"; 904 case DATEOP: return "dateOp"; 905 case UUID: return "uuid"; 906 case POINTER: return "pointer"; 907 case EVALUATE: return "evaluate"; 908 case CC: return "cc"; 909 case C: return "c"; 910 case QTY: return "qty"; 911 case ID: return "id"; 912 case CP: return "cp"; 913 case NULL: return null; 914 default: return "?"; 915 } 916 } 917 public String getSystem() { 918 switch (this) { 919 case CREATE: return "http://hl7.org/fhir/map-transform"; 920 case COPY: return "http://hl7.org/fhir/map-transform"; 921 case TRUNCATE: return "http://hl7.org/fhir/map-transform"; 922 case ESCAPE: return "http://hl7.org/fhir/map-transform"; 923 case CAST: return "http://hl7.org/fhir/map-transform"; 924 case APPEND: return "http://hl7.org/fhir/map-transform"; 925 case TRANSLATE: return "http://hl7.org/fhir/map-transform"; 926 case REFERENCE: return "http://hl7.org/fhir/map-transform"; 927 case DATEOP: return "http://hl7.org/fhir/map-transform"; 928 case UUID: return "http://hl7.org/fhir/map-transform"; 929 case POINTER: return "http://hl7.org/fhir/map-transform"; 930 case EVALUATE: return "http://hl7.org/fhir/map-transform"; 931 case CC: return "http://hl7.org/fhir/map-transform"; 932 case C: return "http://hl7.org/fhir/map-transform"; 933 case QTY: return "http://hl7.org/fhir/map-transform"; 934 case ID: return "http://hl7.org/fhir/map-transform"; 935 case CP: return "http://hl7.org/fhir/map-transform"; 936 case NULL: return null; 937 default: return "?"; 938 } 939 } 940 public String getDefinition() { 941 switch (this) { 942 case CREATE: return "create(type : string) - type is passed through to the application on the standard API, and must be known by it"; 943 case COPY: return "copy(source)"; 944 case TRUNCATE: return "truncate(source, length) - source must be stringy type"; 945 case ESCAPE: return "escape(source, fmt1, fmt2) - change source from one kind of escaping to another (plain, java, xml, json). note that this is for when the string itself is escaped"; 946 case CAST: return "cast(source, type?) - case source from one type to another. target type can be left as implicit if there is one and only one target type known"; 947 case APPEND: return "append(source...) - source is element or string"; 948 case TRANSLATE: return "translate(source, uri_of_map) - use the translate operation"; 949 case REFERENCE: return "reference(source : object) - return a string that references the provided tree properly"; 950 case DATEOP: return "Perform a date operation. *Parameters to be documented*"; 951 case UUID: return "Generate a random UUID (in lowercase). No Parameters"; 952 case POINTER: return "Return the appropriate string to put in a reference that refers to the resource provided as a parameter"; 953 case EVALUATE: return "Execute the supplied fluentpath expression and use the value returned by that"; 954 case CC: return "Create a CodeableConcept. Parameters = (text) or (system. Code[, display])"; 955 case C: return "Create a Coding. Parameters = (system. Code[, display])"; 956 case QTY: return "Create a quantity. Parameters = (text) or (value, unit, [system, code]) where text is the natural representation e.g. [comparator]value[space]unit"; 957 case ID: return "Create an identifier. Parameters = (system, value[, type]) where type is a code from the identifier type value set"; 958 case CP: return "Create a contact details. Parameters = (value) or (system, value). If no system is provided, the system should be inferred from the content of the value"; 959 case NULL: return null; 960 default: return "?"; 961 } 962 } 963 public String getDisplay() { 964 switch (this) { 965 case CREATE: return "create"; 966 case COPY: return "copy"; 967 case TRUNCATE: return "truncate"; 968 case ESCAPE: return "escape"; 969 case CAST: return "cast"; 970 case APPEND: return "append"; 971 case TRANSLATE: return "translate"; 972 case REFERENCE: return "reference"; 973 case DATEOP: return "dateOp"; 974 case UUID: return "uuid"; 975 case POINTER: return "pointer"; 976 case EVALUATE: return "evaluate"; 977 case CC: return "cc"; 978 case C: return "c"; 979 case QTY: return "qty"; 980 case ID: return "id"; 981 case CP: return "cp"; 982 case NULL: return null; 983 default: return "?"; 984 } 985 } 986 } 987 988 public static class StructureMapTransformEnumFactory implements EnumFactory<StructureMapTransform> { 989 public StructureMapTransform fromCode(String codeString) throws IllegalArgumentException { 990 if (codeString == null || "".equals(codeString)) 991 if (codeString == null || "".equals(codeString)) 992 return null; 993 if ("create".equals(codeString)) 994 return StructureMapTransform.CREATE; 995 if ("copy".equals(codeString)) 996 return StructureMapTransform.COPY; 997 if ("truncate".equals(codeString)) 998 return StructureMapTransform.TRUNCATE; 999 if ("escape".equals(codeString)) 1000 return StructureMapTransform.ESCAPE; 1001 if ("cast".equals(codeString)) 1002 return StructureMapTransform.CAST; 1003 if ("append".equals(codeString)) 1004 return StructureMapTransform.APPEND; 1005 if ("translate".equals(codeString)) 1006 return StructureMapTransform.TRANSLATE; 1007 if ("reference".equals(codeString)) 1008 return StructureMapTransform.REFERENCE; 1009 if ("dateOp".equals(codeString)) 1010 return StructureMapTransform.DATEOP; 1011 if ("uuid".equals(codeString)) 1012 return StructureMapTransform.UUID; 1013 if ("pointer".equals(codeString)) 1014 return StructureMapTransform.POINTER; 1015 if ("evaluate".equals(codeString)) 1016 return StructureMapTransform.EVALUATE; 1017 if ("cc".equals(codeString)) 1018 return StructureMapTransform.CC; 1019 if ("c".equals(codeString)) 1020 return StructureMapTransform.C; 1021 if ("qty".equals(codeString)) 1022 return StructureMapTransform.QTY; 1023 if ("id".equals(codeString)) 1024 return StructureMapTransform.ID; 1025 if ("cp".equals(codeString)) 1026 return StructureMapTransform.CP; 1027 throw new IllegalArgumentException("Unknown StructureMapTransform code '"+codeString+"'"); 1028 } 1029 public Enumeration<StructureMapTransform> fromType(PrimitiveType<?> code) throws FHIRException { 1030 if (code == null) 1031 return null; 1032 if (code.isEmpty()) 1033 return new Enumeration<StructureMapTransform>(this); 1034 String codeString = code.asStringValue(); 1035 if (codeString == null || "".equals(codeString)) 1036 return null; 1037 if ("create".equals(codeString)) 1038 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CREATE); 1039 if ("copy".equals(codeString)) 1040 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.COPY); 1041 if ("truncate".equals(codeString)) 1042 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.TRUNCATE); 1043 if ("escape".equals(codeString)) 1044 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.ESCAPE); 1045 if ("cast".equals(codeString)) 1046 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CAST); 1047 if ("append".equals(codeString)) 1048 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.APPEND); 1049 if ("translate".equals(codeString)) 1050 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.TRANSLATE); 1051 if ("reference".equals(codeString)) 1052 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.REFERENCE); 1053 if ("dateOp".equals(codeString)) 1054 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.DATEOP); 1055 if ("uuid".equals(codeString)) 1056 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.UUID); 1057 if ("pointer".equals(codeString)) 1058 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.POINTER); 1059 if ("evaluate".equals(codeString)) 1060 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.EVALUATE); 1061 if ("cc".equals(codeString)) 1062 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CC); 1063 if ("c".equals(codeString)) 1064 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.C); 1065 if ("qty".equals(codeString)) 1066 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.QTY); 1067 if ("id".equals(codeString)) 1068 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.ID); 1069 if ("cp".equals(codeString)) 1070 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CP); 1071 throw new FHIRException("Unknown StructureMapTransform code '"+codeString+"'"); 1072 } 1073 public String toCode(StructureMapTransform code) { 1074 if (code == StructureMapTransform.NULL) 1075 return null; 1076 if (code == StructureMapTransform.CREATE) 1077 return "create"; 1078 if (code == StructureMapTransform.COPY) 1079 return "copy"; 1080 if (code == StructureMapTransform.TRUNCATE) 1081 return "truncate"; 1082 if (code == StructureMapTransform.ESCAPE) 1083 return "escape"; 1084 if (code == StructureMapTransform.CAST) 1085 return "cast"; 1086 if (code == StructureMapTransform.APPEND) 1087 return "append"; 1088 if (code == StructureMapTransform.TRANSLATE) 1089 return "translate"; 1090 if (code == StructureMapTransform.REFERENCE) 1091 return "reference"; 1092 if (code == StructureMapTransform.DATEOP) 1093 return "dateOp"; 1094 if (code == StructureMapTransform.UUID) 1095 return "uuid"; 1096 if (code == StructureMapTransform.POINTER) 1097 return "pointer"; 1098 if (code == StructureMapTransform.EVALUATE) 1099 return "evaluate"; 1100 if (code == StructureMapTransform.CC) 1101 return "cc"; 1102 if (code == StructureMapTransform.C) 1103 return "c"; 1104 if (code == StructureMapTransform.QTY) 1105 return "qty"; 1106 if (code == StructureMapTransform.ID) 1107 return "id"; 1108 if (code == StructureMapTransform.CP) 1109 return "cp"; 1110 return "?"; 1111 } 1112 public String toSystem(StructureMapTransform code) { 1113 return code.getSystem(); 1114 } 1115 } 1116 1117 @Block() 1118 public static class StructureMapStructureComponent extends BackboneElement implements IBaseBackboneElement { 1119 /** 1120 * The canonical URL that identifies the structure. 1121 */ 1122 @Child(name = "url", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1123 @Description(shortDefinition="Canonical URL for structure definition", formalDefinition="The canonical URL that identifies the structure." ) 1124 protected UriType url; 1125 1126 /** 1127 * How the referenced structure is used in this mapping. 1128 */ 1129 @Child(name = "mode", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1130 @Description(shortDefinition="source | queried | target | produced", formalDefinition="How the referenced structure is used in this mapping." ) 1131 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-model-mode") 1132 protected Enumeration<StructureMapModelMode> mode; 1133 1134 /** 1135 * The name used for this type in the map. 1136 */ 1137 @Child(name = "alias", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1138 @Description(shortDefinition="Name for type in this map", formalDefinition="The name used for this type in the map." ) 1139 protected StringType alias; 1140 1141 /** 1142 * Documentation that describes how the structure is used in the mapping. 1143 */ 1144 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1145 @Description(shortDefinition="Documentation on use of structure", formalDefinition="Documentation that describes how the structure is used in the mapping." ) 1146 protected StringType documentation; 1147 1148 private static final long serialVersionUID = -277986558L; 1149 1150 /** 1151 * Constructor 1152 */ 1153 public StructureMapStructureComponent() { 1154 super(); 1155 } 1156 1157 /** 1158 * Constructor 1159 */ 1160 public StructureMapStructureComponent(UriType url, Enumeration<StructureMapModelMode> mode) { 1161 super(); 1162 this.url = url; 1163 this.mode = mode; 1164 } 1165 1166 /** 1167 * @return {@link #url} (The canonical URL that identifies the structure.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1168 */ 1169 public UriType getUrlElement() { 1170 if (this.url == null) 1171 if (Configuration.errorOnAutoCreate()) 1172 throw new Error("Attempt to auto-create StructureMapStructureComponent.url"); 1173 else if (Configuration.doAutoCreate()) 1174 this.url = new UriType(); // bb 1175 return this.url; 1176 } 1177 1178 public boolean hasUrlElement() { 1179 return this.url != null && !this.url.isEmpty(); 1180 } 1181 1182 public boolean hasUrl() { 1183 return this.url != null && !this.url.isEmpty(); 1184 } 1185 1186 /** 1187 * @param value {@link #url} (The canonical URL that identifies the structure.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1188 */ 1189 public StructureMapStructureComponent setUrlElement(UriType value) { 1190 this.url = value; 1191 return this; 1192 } 1193 1194 /** 1195 * @return The canonical URL that identifies the structure. 1196 */ 1197 public String getUrl() { 1198 return this.url == null ? null : this.url.getValue(); 1199 } 1200 1201 /** 1202 * @param value The canonical URL that identifies the structure. 1203 */ 1204 public StructureMapStructureComponent setUrl(String value) { 1205 if (this.url == null) 1206 this.url = new UriType(); 1207 this.url.setValue(value); 1208 return this; 1209 } 1210 1211 /** 1212 * @return {@link #mode} (How the referenced structure is used in this mapping.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1213 */ 1214 public Enumeration<StructureMapModelMode> getModeElement() { 1215 if (this.mode == null) 1216 if (Configuration.errorOnAutoCreate()) 1217 throw new Error("Attempt to auto-create StructureMapStructureComponent.mode"); 1218 else if (Configuration.doAutoCreate()) 1219 this.mode = new Enumeration<StructureMapModelMode>(new StructureMapModelModeEnumFactory()); // bb 1220 return this.mode; 1221 } 1222 1223 public boolean hasModeElement() { 1224 return this.mode != null && !this.mode.isEmpty(); 1225 } 1226 1227 public boolean hasMode() { 1228 return this.mode != null && !this.mode.isEmpty(); 1229 } 1230 1231 /** 1232 * @param value {@link #mode} (How the referenced structure is used in this mapping.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1233 */ 1234 public StructureMapStructureComponent setModeElement(Enumeration<StructureMapModelMode> value) { 1235 this.mode = value; 1236 return this; 1237 } 1238 1239 /** 1240 * @return How the referenced structure is used in this mapping. 1241 */ 1242 public StructureMapModelMode getMode() { 1243 return this.mode == null ? null : this.mode.getValue(); 1244 } 1245 1246 /** 1247 * @param value How the referenced structure is used in this mapping. 1248 */ 1249 public StructureMapStructureComponent setMode(StructureMapModelMode value) { 1250 if (this.mode == null) 1251 this.mode = new Enumeration<StructureMapModelMode>(new StructureMapModelModeEnumFactory()); 1252 this.mode.setValue(value); 1253 return this; 1254 } 1255 1256 /** 1257 * @return {@link #alias} (The name used for this type in the map.). This is the underlying object with id, value and extensions. The accessor "getAlias" gives direct access to the value 1258 */ 1259 public StringType getAliasElement() { 1260 if (this.alias == null) 1261 if (Configuration.errorOnAutoCreate()) 1262 throw new Error("Attempt to auto-create StructureMapStructureComponent.alias"); 1263 else if (Configuration.doAutoCreate()) 1264 this.alias = new StringType(); // bb 1265 return this.alias; 1266 } 1267 1268 public boolean hasAliasElement() { 1269 return this.alias != null && !this.alias.isEmpty(); 1270 } 1271 1272 public boolean hasAlias() { 1273 return this.alias != null && !this.alias.isEmpty(); 1274 } 1275 1276 /** 1277 * @param value {@link #alias} (The name used for this type in the map.). This is the underlying object with id, value and extensions. The accessor "getAlias" gives direct access to the value 1278 */ 1279 public StructureMapStructureComponent setAliasElement(StringType value) { 1280 this.alias = value; 1281 return this; 1282 } 1283 1284 /** 1285 * @return The name used for this type in the map. 1286 */ 1287 public String getAlias() { 1288 return this.alias == null ? null : this.alias.getValue(); 1289 } 1290 1291 /** 1292 * @param value The name used for this type in the map. 1293 */ 1294 public StructureMapStructureComponent setAlias(String value) { 1295 if (Utilities.noString(value)) 1296 this.alias = null; 1297 else { 1298 if (this.alias == null) 1299 this.alias = new StringType(); 1300 this.alias.setValue(value); 1301 } 1302 return this; 1303 } 1304 1305 /** 1306 * @return {@link #documentation} (Documentation that describes how the structure is used in the mapping.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1307 */ 1308 public StringType getDocumentationElement() { 1309 if (this.documentation == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create StructureMapStructureComponent.documentation"); 1312 else if (Configuration.doAutoCreate()) 1313 this.documentation = new StringType(); // bb 1314 return this.documentation; 1315 } 1316 1317 public boolean hasDocumentationElement() { 1318 return this.documentation != null && !this.documentation.isEmpty(); 1319 } 1320 1321 public boolean hasDocumentation() { 1322 return this.documentation != null && !this.documentation.isEmpty(); 1323 } 1324 1325 /** 1326 * @param value {@link #documentation} (Documentation that describes how the structure is used in the mapping.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1327 */ 1328 public StructureMapStructureComponent setDocumentationElement(StringType value) { 1329 this.documentation = value; 1330 return this; 1331 } 1332 1333 /** 1334 * @return Documentation that describes how the structure is used in the mapping. 1335 */ 1336 public String getDocumentation() { 1337 return this.documentation == null ? null : this.documentation.getValue(); 1338 } 1339 1340 /** 1341 * @param value Documentation that describes how the structure is used in the mapping. 1342 */ 1343 public StructureMapStructureComponent setDocumentation(String value) { 1344 if (Utilities.noString(value)) 1345 this.documentation = null; 1346 else { 1347 if (this.documentation == null) 1348 this.documentation = new StringType(); 1349 this.documentation.setValue(value); 1350 } 1351 return this; 1352 } 1353 1354 protected void listChildren(List<Property> children) { 1355 super.listChildren(children); 1356 children.add(new Property("url", "uri", "The canonical URL that identifies the structure.", 0, 1, url)); 1357 children.add(new Property("mode", "code", "How the referenced structure is used in this mapping.", 0, 1, mode)); 1358 children.add(new Property("alias", "string", "The name used for this type in the map.", 0, 1, alias)); 1359 children.add(new Property("documentation", "string", "Documentation that describes how the structure is used in the mapping.", 0, 1, documentation)); 1360 } 1361 1362 @Override 1363 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1364 switch (_hash) { 1365 case 116079: /*url*/ return new Property("url", "uri", "The canonical URL that identifies the structure.", 0, 1, url); 1366 case 3357091: /*mode*/ return new Property("mode", "code", "How the referenced structure is used in this mapping.", 0, 1, mode); 1367 case 92902992: /*alias*/ return new Property("alias", "string", "The name used for this type in the map.", 0, 1, alias); 1368 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Documentation that describes how the structure is used in the mapping.", 0, 1, documentation); 1369 default: return super.getNamedProperty(_hash, _name, _checkValid); 1370 } 1371 1372 } 1373 1374 @Override 1375 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1376 switch (hash) { 1377 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1378 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<StructureMapModelMode> 1379 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : new Base[] {this.alias}; // StringType 1380 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 1381 default: return super.getProperty(hash, name, checkValid); 1382 } 1383 1384 } 1385 1386 @Override 1387 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1388 switch (hash) { 1389 case 116079: // url 1390 this.url = castToUri(value); // UriType 1391 return value; 1392 case 3357091: // mode 1393 value = new StructureMapModelModeEnumFactory().fromType(castToCode(value)); 1394 this.mode = (Enumeration) value; // Enumeration<StructureMapModelMode> 1395 return value; 1396 case 92902992: // alias 1397 this.alias = castToString(value); // StringType 1398 return value; 1399 case 1587405498: // documentation 1400 this.documentation = castToString(value); // StringType 1401 return value; 1402 default: return super.setProperty(hash, name, value); 1403 } 1404 1405 } 1406 1407 @Override 1408 public Base setProperty(String name, Base value) throws FHIRException { 1409 if (name.equals("url")) { 1410 this.url = castToUri(value); // UriType 1411 } else if (name.equals("mode")) { 1412 value = new StructureMapModelModeEnumFactory().fromType(castToCode(value)); 1413 this.mode = (Enumeration) value; // Enumeration<StructureMapModelMode> 1414 } else if (name.equals("alias")) { 1415 this.alias = castToString(value); // StringType 1416 } else if (name.equals("documentation")) { 1417 this.documentation = castToString(value); // StringType 1418 } else 1419 return super.setProperty(name, value); 1420 return value; 1421 } 1422 1423 @Override 1424 public Base makeProperty(int hash, String name) throws FHIRException { 1425 switch (hash) { 1426 case 116079: return getUrlElement(); 1427 case 3357091: return getModeElement(); 1428 case 92902992: return getAliasElement(); 1429 case 1587405498: return getDocumentationElement(); 1430 default: return super.makeProperty(hash, name); 1431 } 1432 1433 } 1434 1435 @Override 1436 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1437 switch (hash) { 1438 case 116079: /*url*/ return new String[] {"uri"}; 1439 case 3357091: /*mode*/ return new String[] {"code"}; 1440 case 92902992: /*alias*/ return new String[] {"string"}; 1441 case 1587405498: /*documentation*/ return new String[] {"string"}; 1442 default: return super.getTypesForProperty(hash, name); 1443 } 1444 1445 } 1446 1447 @Override 1448 public Base addChild(String name) throws FHIRException { 1449 if (name.equals("url")) { 1450 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.url"); 1451 } 1452 else if (name.equals("mode")) { 1453 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.mode"); 1454 } 1455 else if (name.equals("alias")) { 1456 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.alias"); 1457 } 1458 else if (name.equals("documentation")) { 1459 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.documentation"); 1460 } 1461 else 1462 return super.addChild(name); 1463 } 1464 1465 public StructureMapStructureComponent copy() { 1466 StructureMapStructureComponent dst = new StructureMapStructureComponent(); 1467 copyValues(dst); 1468 dst.url = url == null ? null : url.copy(); 1469 dst.mode = mode == null ? null : mode.copy(); 1470 dst.alias = alias == null ? null : alias.copy(); 1471 dst.documentation = documentation == null ? null : documentation.copy(); 1472 return dst; 1473 } 1474 1475 @Override 1476 public boolean equalsDeep(Base other_) { 1477 if (!super.equalsDeep(other_)) 1478 return false; 1479 if (!(other_ instanceof StructureMapStructureComponent)) 1480 return false; 1481 StructureMapStructureComponent o = (StructureMapStructureComponent) other_; 1482 return compareDeep(url, o.url, true) && compareDeep(mode, o.mode, true) && compareDeep(alias, o.alias, true) 1483 && compareDeep(documentation, o.documentation, true); 1484 } 1485 1486 @Override 1487 public boolean equalsShallow(Base other_) { 1488 if (!super.equalsShallow(other_)) 1489 return false; 1490 if (!(other_ instanceof StructureMapStructureComponent)) 1491 return false; 1492 StructureMapStructureComponent o = (StructureMapStructureComponent) other_; 1493 return compareValues(url, o.url, true) && compareValues(mode, o.mode, true) && compareValues(alias, o.alias, true) 1494 && compareValues(documentation, o.documentation, true); 1495 } 1496 1497 public boolean isEmpty() { 1498 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, mode, alias, documentation 1499 ); 1500 } 1501 1502 public String fhirType() { 1503 return "StructureMap.structure"; 1504 1505 } 1506 1507 } 1508 1509 @Block() 1510 public static class StructureMapGroupComponent extends BackboneElement implements IBaseBackboneElement { 1511 /** 1512 * A unique name for the group for the convenience of human readers. 1513 */ 1514 @Child(name = "name", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1515 @Description(shortDefinition="Human-readable label", formalDefinition="A unique name for the group for the convenience of human readers." ) 1516 protected IdType name; 1517 1518 /** 1519 * Another group that this group adds rules to. 1520 */ 1521 @Child(name = "extends", type = {IdType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1522 @Description(shortDefinition="Another group that this group adds rules to", formalDefinition="Another group that this group adds rules to." ) 1523 protected IdType extends_; 1524 1525 /** 1526 * If this is the default rule set to apply for thie source type, or this combination of types. 1527 */ 1528 @Child(name = "typeMode", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1529 @Description(shortDefinition="none | types | type-and-types", formalDefinition="If this is the default rule set to apply for thie source type, or this combination of types." ) 1530 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-group-type-mode") 1531 protected Enumeration<StructureMapGroupTypeMode> typeMode; 1532 1533 /** 1534 * Additional supporting documentation that explains the purpose of the group and the types of mappings within it. 1535 */ 1536 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1537 @Description(shortDefinition="Additional description/explaination for group", formalDefinition="Additional supporting documentation that explains the purpose of the group and the types of mappings within it." ) 1538 protected StringType documentation; 1539 1540 /** 1541 * A name assigned to an instance of data. The instance must be provided when the mapping is invoked. 1542 */ 1543 @Child(name = "input", type = {}, order=5, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1544 @Description(shortDefinition="Named instance provided when invoking the map", formalDefinition="A name assigned to an instance of data. The instance must be provided when the mapping is invoked." ) 1545 protected List<StructureMapGroupInputComponent> input; 1546 1547 /** 1548 * Transform Rule from source to target. 1549 */ 1550 @Child(name = "rule", type = {}, order=6, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1551 @Description(shortDefinition="Transform Rule from source to target", formalDefinition="Transform Rule from source to target." ) 1552 protected List<StructureMapGroupRuleComponent> rule; 1553 1554 private static final long serialVersionUID = -1474595081L; 1555 1556 /** 1557 * Constructor 1558 */ 1559 public StructureMapGroupComponent() { 1560 super(); 1561 } 1562 1563 /** 1564 * Constructor 1565 */ 1566 public StructureMapGroupComponent(IdType name, Enumeration<StructureMapGroupTypeMode> typeMode) { 1567 super(); 1568 this.name = name; 1569 this.typeMode = typeMode; 1570 } 1571 1572 /** 1573 * @return {@link #name} (A unique name for the group for the convenience of human readers.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1574 */ 1575 public IdType getNameElement() { 1576 if (this.name == null) 1577 if (Configuration.errorOnAutoCreate()) 1578 throw new Error("Attempt to auto-create StructureMapGroupComponent.name"); 1579 else if (Configuration.doAutoCreate()) 1580 this.name = new IdType(); // bb 1581 return this.name; 1582 } 1583 1584 public boolean hasNameElement() { 1585 return this.name != null && !this.name.isEmpty(); 1586 } 1587 1588 public boolean hasName() { 1589 return this.name != null && !this.name.isEmpty(); 1590 } 1591 1592 /** 1593 * @param value {@link #name} (A unique name for the group for the convenience of human readers.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1594 */ 1595 public StructureMapGroupComponent setNameElement(IdType value) { 1596 this.name = value; 1597 return this; 1598 } 1599 1600 /** 1601 * @return A unique name for the group for the convenience of human readers. 1602 */ 1603 public String getName() { 1604 return this.name == null ? null : this.name.getValue(); 1605 } 1606 1607 /** 1608 * @param value A unique name for the group for the convenience of human readers. 1609 */ 1610 public StructureMapGroupComponent setName(String value) { 1611 if (this.name == null) 1612 this.name = new IdType(); 1613 this.name.setValue(value); 1614 return this; 1615 } 1616 1617 /** 1618 * @return {@link #extends_} (Another group that this group adds rules to.). This is the underlying object with id, value and extensions. The accessor "getExtends" gives direct access to the value 1619 */ 1620 public IdType getExtendsElement() { 1621 if (this.extends_ == null) 1622 if (Configuration.errorOnAutoCreate()) 1623 throw new Error("Attempt to auto-create StructureMapGroupComponent.extends_"); 1624 else if (Configuration.doAutoCreate()) 1625 this.extends_ = new IdType(); // bb 1626 return this.extends_; 1627 } 1628 1629 public boolean hasExtendsElement() { 1630 return this.extends_ != null && !this.extends_.isEmpty(); 1631 } 1632 1633 public boolean hasExtends() { 1634 return this.extends_ != null && !this.extends_.isEmpty(); 1635 } 1636 1637 /** 1638 * @param value {@link #extends_} (Another group that this group adds rules to.). This is the underlying object with id, value and extensions. The accessor "getExtends" gives direct access to the value 1639 */ 1640 public StructureMapGroupComponent setExtendsElement(IdType value) { 1641 this.extends_ = value; 1642 return this; 1643 } 1644 1645 /** 1646 * @return Another group that this group adds rules to. 1647 */ 1648 public String getExtends() { 1649 return this.extends_ == null ? null : this.extends_.getValue(); 1650 } 1651 1652 /** 1653 * @param value Another group that this group adds rules to. 1654 */ 1655 public StructureMapGroupComponent setExtends(String value) { 1656 if (Utilities.noString(value)) 1657 this.extends_ = null; 1658 else { 1659 if (this.extends_ == null) 1660 this.extends_ = new IdType(); 1661 this.extends_.setValue(value); 1662 } 1663 return this; 1664 } 1665 1666 /** 1667 * @return {@link #typeMode} (If this is the default rule set to apply for thie source type, or this combination of types.). This is the underlying object with id, value and extensions. The accessor "getTypeMode" gives direct access to the value 1668 */ 1669 public Enumeration<StructureMapGroupTypeMode> getTypeModeElement() { 1670 if (this.typeMode == null) 1671 if (Configuration.errorOnAutoCreate()) 1672 throw new Error("Attempt to auto-create StructureMapGroupComponent.typeMode"); 1673 else if (Configuration.doAutoCreate()) 1674 this.typeMode = new Enumeration<StructureMapGroupTypeMode>(new StructureMapGroupTypeModeEnumFactory()); // bb 1675 return this.typeMode; 1676 } 1677 1678 public boolean hasTypeModeElement() { 1679 return this.typeMode != null && !this.typeMode.isEmpty(); 1680 } 1681 1682 public boolean hasTypeMode() { 1683 return this.typeMode != null && !this.typeMode.isEmpty(); 1684 } 1685 1686 /** 1687 * @param value {@link #typeMode} (If this is the default rule set to apply for thie source type, or this combination of types.). This is the underlying object with id, value and extensions. The accessor "getTypeMode" gives direct access to the value 1688 */ 1689 public StructureMapGroupComponent setTypeModeElement(Enumeration<StructureMapGroupTypeMode> value) { 1690 this.typeMode = value; 1691 return this; 1692 } 1693 1694 /** 1695 * @return If this is the default rule set to apply for thie source type, or this combination of types. 1696 */ 1697 public StructureMapGroupTypeMode getTypeMode() { 1698 return this.typeMode == null ? null : this.typeMode.getValue(); 1699 } 1700 1701 /** 1702 * @param value If this is the default rule set to apply for thie source type, or this combination of types. 1703 */ 1704 public StructureMapGroupComponent setTypeMode(StructureMapGroupTypeMode value) { 1705 if (this.typeMode == null) 1706 this.typeMode = new Enumeration<StructureMapGroupTypeMode>(new StructureMapGroupTypeModeEnumFactory()); 1707 this.typeMode.setValue(value); 1708 return this; 1709 } 1710 1711 /** 1712 * @return {@link #documentation} (Additional supporting documentation that explains the purpose of the group and the types of mappings within it.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1713 */ 1714 public StringType getDocumentationElement() { 1715 if (this.documentation == null) 1716 if (Configuration.errorOnAutoCreate()) 1717 throw new Error("Attempt to auto-create StructureMapGroupComponent.documentation"); 1718 else if (Configuration.doAutoCreate()) 1719 this.documentation = new StringType(); // bb 1720 return this.documentation; 1721 } 1722 1723 public boolean hasDocumentationElement() { 1724 return this.documentation != null && !this.documentation.isEmpty(); 1725 } 1726 1727 public boolean hasDocumentation() { 1728 return this.documentation != null && !this.documentation.isEmpty(); 1729 } 1730 1731 /** 1732 * @param value {@link #documentation} (Additional supporting documentation that explains the purpose of the group and the types of mappings within it.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 1733 */ 1734 public StructureMapGroupComponent setDocumentationElement(StringType value) { 1735 this.documentation = value; 1736 return this; 1737 } 1738 1739 /** 1740 * @return Additional supporting documentation that explains the purpose of the group and the types of mappings within it. 1741 */ 1742 public String getDocumentation() { 1743 return this.documentation == null ? null : this.documentation.getValue(); 1744 } 1745 1746 /** 1747 * @param value Additional supporting documentation that explains the purpose of the group and the types of mappings within it. 1748 */ 1749 public StructureMapGroupComponent setDocumentation(String value) { 1750 if (Utilities.noString(value)) 1751 this.documentation = null; 1752 else { 1753 if (this.documentation == null) 1754 this.documentation = new StringType(); 1755 this.documentation.setValue(value); 1756 } 1757 return this; 1758 } 1759 1760 /** 1761 * @return {@link #input} (A name assigned to an instance of data. The instance must be provided when the mapping is invoked.) 1762 */ 1763 public List<StructureMapGroupInputComponent> getInput() { 1764 if (this.input == null) 1765 this.input = new ArrayList<StructureMapGroupInputComponent>(); 1766 return this.input; 1767 } 1768 1769 /** 1770 * @return Returns a reference to <code>this</code> for easy method chaining 1771 */ 1772 public StructureMapGroupComponent setInput(List<StructureMapGroupInputComponent> theInput) { 1773 this.input = theInput; 1774 return this; 1775 } 1776 1777 public boolean hasInput() { 1778 if (this.input == null) 1779 return false; 1780 for (StructureMapGroupInputComponent item : this.input) 1781 if (!item.isEmpty()) 1782 return true; 1783 return false; 1784 } 1785 1786 public StructureMapGroupInputComponent addInput() { //3 1787 StructureMapGroupInputComponent t = new StructureMapGroupInputComponent(); 1788 if (this.input == null) 1789 this.input = new ArrayList<StructureMapGroupInputComponent>(); 1790 this.input.add(t); 1791 return t; 1792 } 1793 1794 public StructureMapGroupComponent addInput(StructureMapGroupInputComponent t) { //3 1795 if (t == null) 1796 return this; 1797 if (this.input == null) 1798 this.input = new ArrayList<StructureMapGroupInputComponent>(); 1799 this.input.add(t); 1800 return this; 1801 } 1802 1803 /** 1804 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist 1805 */ 1806 public StructureMapGroupInputComponent getInputFirstRep() { 1807 if (getInput().isEmpty()) { 1808 addInput(); 1809 } 1810 return getInput().get(0); 1811 } 1812 1813 /** 1814 * @return {@link #rule} (Transform Rule from source to target.) 1815 */ 1816 public List<StructureMapGroupRuleComponent> getRule() { 1817 if (this.rule == null) 1818 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 1819 return this.rule; 1820 } 1821 1822 /** 1823 * @return Returns a reference to <code>this</code> for easy method chaining 1824 */ 1825 public StructureMapGroupComponent setRule(List<StructureMapGroupRuleComponent> theRule) { 1826 this.rule = theRule; 1827 return this; 1828 } 1829 1830 public boolean hasRule() { 1831 if (this.rule == null) 1832 return false; 1833 for (StructureMapGroupRuleComponent item : this.rule) 1834 if (!item.isEmpty()) 1835 return true; 1836 return false; 1837 } 1838 1839 public StructureMapGroupRuleComponent addRule() { //3 1840 StructureMapGroupRuleComponent t = new StructureMapGroupRuleComponent(); 1841 if (this.rule == null) 1842 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 1843 this.rule.add(t); 1844 return t; 1845 } 1846 1847 public StructureMapGroupComponent addRule(StructureMapGroupRuleComponent t) { //3 1848 if (t == null) 1849 return this; 1850 if (this.rule == null) 1851 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 1852 this.rule.add(t); 1853 return this; 1854 } 1855 1856 /** 1857 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist 1858 */ 1859 public StructureMapGroupRuleComponent getRuleFirstRep() { 1860 if (getRule().isEmpty()) { 1861 addRule(); 1862 } 1863 return getRule().get(0); 1864 } 1865 1866 protected void listChildren(List<Property> children) { 1867 super.listChildren(children); 1868 children.add(new Property("name", "id", "A unique name for the group for the convenience of human readers.", 0, 1, name)); 1869 children.add(new Property("extends", "id", "Another group that this group adds rules to.", 0, 1, extends_)); 1870 children.add(new Property("typeMode", "code", "If this is the default rule set to apply for thie source type, or this combination of types.", 0, 1, typeMode)); 1871 children.add(new Property("documentation", "string", "Additional supporting documentation that explains the purpose of the group and the types of mappings within it.", 0, 1, documentation)); 1872 children.add(new Property("input", "", "A name assigned to an instance of data. The instance must be provided when the mapping is invoked.", 0, java.lang.Integer.MAX_VALUE, input)); 1873 children.add(new Property("rule", "", "Transform Rule from source to target.", 0, java.lang.Integer.MAX_VALUE, rule)); 1874 } 1875 1876 @Override 1877 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1878 switch (_hash) { 1879 case 3373707: /*name*/ return new Property("name", "id", "A unique name for the group for the convenience of human readers.", 0, 1, name); 1880 case -1305664359: /*extends*/ return new Property("extends", "id", "Another group that this group adds rules to.", 0, 1, extends_); 1881 case -676524035: /*typeMode*/ return new Property("typeMode", "code", "If this is the default rule set to apply for thie source type, or this combination of types.", 0, 1, typeMode); 1882 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Additional supporting documentation that explains the purpose of the group and the types of mappings within it.", 0, 1, documentation); 1883 case 100358090: /*input*/ return new Property("input", "", "A name assigned to an instance of data. The instance must be provided when the mapping is invoked.", 0, java.lang.Integer.MAX_VALUE, input); 1884 case 3512060: /*rule*/ return new Property("rule", "", "Transform Rule from source to target.", 0, java.lang.Integer.MAX_VALUE, rule); 1885 default: return super.getNamedProperty(_hash, _name, _checkValid); 1886 } 1887 1888 } 1889 1890 @Override 1891 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1892 switch (hash) { 1893 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 1894 case -1305664359: /*extends*/ return this.extends_ == null ? new Base[0] : new Base[] {this.extends_}; // IdType 1895 case -676524035: /*typeMode*/ return this.typeMode == null ? new Base[0] : new Base[] {this.typeMode}; // Enumeration<StructureMapGroupTypeMode> 1896 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 1897 case 100358090: /*input*/ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // StructureMapGroupInputComponent 1898 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // StructureMapGroupRuleComponent 1899 default: return super.getProperty(hash, name, checkValid); 1900 } 1901 1902 } 1903 1904 @Override 1905 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1906 switch (hash) { 1907 case 3373707: // name 1908 this.name = castToId(value); // IdType 1909 return value; 1910 case -1305664359: // extends 1911 this.extends_ = castToId(value); // IdType 1912 return value; 1913 case -676524035: // typeMode 1914 value = new StructureMapGroupTypeModeEnumFactory().fromType(castToCode(value)); 1915 this.typeMode = (Enumeration) value; // Enumeration<StructureMapGroupTypeMode> 1916 return value; 1917 case 1587405498: // documentation 1918 this.documentation = castToString(value); // StringType 1919 return value; 1920 case 100358090: // input 1921 this.getInput().add((StructureMapGroupInputComponent) value); // StructureMapGroupInputComponent 1922 return value; 1923 case 3512060: // rule 1924 this.getRule().add((StructureMapGroupRuleComponent) value); // StructureMapGroupRuleComponent 1925 return value; 1926 default: return super.setProperty(hash, name, value); 1927 } 1928 1929 } 1930 1931 @Override 1932 public Base setProperty(String name, Base value) throws FHIRException { 1933 if (name.equals("name")) { 1934 this.name = castToId(value); // IdType 1935 } else if (name.equals("extends")) { 1936 this.extends_ = castToId(value); // IdType 1937 } else if (name.equals("typeMode")) { 1938 value = new StructureMapGroupTypeModeEnumFactory().fromType(castToCode(value)); 1939 this.typeMode = (Enumeration) value; // Enumeration<StructureMapGroupTypeMode> 1940 } else if (name.equals("documentation")) { 1941 this.documentation = castToString(value); // StringType 1942 } else if (name.equals("input")) { 1943 this.getInput().add((StructureMapGroupInputComponent) value); 1944 } else if (name.equals("rule")) { 1945 this.getRule().add((StructureMapGroupRuleComponent) value); 1946 } else 1947 return super.setProperty(name, value); 1948 return value; 1949 } 1950 1951 @Override 1952 public Base makeProperty(int hash, String name) throws FHIRException { 1953 switch (hash) { 1954 case 3373707: return getNameElement(); 1955 case -1305664359: return getExtendsElement(); 1956 case -676524035: return getTypeModeElement(); 1957 case 1587405498: return getDocumentationElement(); 1958 case 100358090: return addInput(); 1959 case 3512060: return addRule(); 1960 default: return super.makeProperty(hash, name); 1961 } 1962 1963 } 1964 1965 @Override 1966 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1967 switch (hash) { 1968 case 3373707: /*name*/ return new String[] {"id"}; 1969 case -1305664359: /*extends*/ return new String[] {"id"}; 1970 case -676524035: /*typeMode*/ return new String[] {"code"}; 1971 case 1587405498: /*documentation*/ return new String[] {"string"}; 1972 case 100358090: /*input*/ return new String[] {}; 1973 case 3512060: /*rule*/ return new String[] {}; 1974 default: return super.getTypesForProperty(hash, name); 1975 } 1976 1977 } 1978 1979 @Override 1980 public Base addChild(String name) throws FHIRException { 1981 if (name.equals("name")) { 1982 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 1983 } 1984 else if (name.equals("extends")) { 1985 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.extends"); 1986 } 1987 else if (name.equals("typeMode")) { 1988 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.typeMode"); 1989 } 1990 else if (name.equals("documentation")) { 1991 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.documentation"); 1992 } 1993 else if (name.equals("input")) { 1994 return addInput(); 1995 } 1996 else if (name.equals("rule")) { 1997 return addRule(); 1998 } 1999 else 2000 return super.addChild(name); 2001 } 2002 2003 public StructureMapGroupComponent copy() { 2004 StructureMapGroupComponent dst = new StructureMapGroupComponent(); 2005 copyValues(dst); 2006 dst.name = name == null ? null : name.copy(); 2007 dst.extends_ = extends_ == null ? null : extends_.copy(); 2008 dst.typeMode = typeMode == null ? null : typeMode.copy(); 2009 dst.documentation = documentation == null ? null : documentation.copy(); 2010 if (input != null) { 2011 dst.input = new ArrayList<StructureMapGroupInputComponent>(); 2012 for (StructureMapGroupInputComponent i : input) 2013 dst.input.add(i.copy()); 2014 }; 2015 if (rule != null) { 2016 dst.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2017 for (StructureMapGroupRuleComponent i : rule) 2018 dst.rule.add(i.copy()); 2019 }; 2020 return dst; 2021 } 2022 2023 @Override 2024 public boolean equalsDeep(Base other_) { 2025 if (!super.equalsDeep(other_)) 2026 return false; 2027 if (!(other_ instanceof StructureMapGroupComponent)) 2028 return false; 2029 StructureMapGroupComponent o = (StructureMapGroupComponent) other_; 2030 return compareDeep(name, o.name, true) && compareDeep(extends_, o.extends_, true) && compareDeep(typeMode, o.typeMode, true) 2031 && compareDeep(documentation, o.documentation, true) && compareDeep(input, o.input, true) && compareDeep(rule, o.rule, true) 2032 ; 2033 } 2034 2035 @Override 2036 public boolean equalsShallow(Base other_) { 2037 if (!super.equalsShallow(other_)) 2038 return false; 2039 if (!(other_ instanceof StructureMapGroupComponent)) 2040 return false; 2041 StructureMapGroupComponent o = (StructureMapGroupComponent) other_; 2042 return compareValues(name, o.name, true) && compareValues(extends_, o.extends_, true) && compareValues(typeMode, o.typeMode, true) 2043 && compareValues(documentation, o.documentation, true); 2044 } 2045 2046 public boolean isEmpty() { 2047 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, extends_, typeMode 2048 , documentation, input, rule); 2049 } 2050 2051 public String fhirType() { 2052 return "StructureMap.group"; 2053 2054 } 2055 2056// added from java-adornments.txt: 2057 2058 public String toString() { 2059 return StructureMapUtilities.groupToString(this); 2060 } 2061 2062 2063// end addition 2064 } 2065 2066 @Block() 2067 public static class StructureMapGroupInputComponent extends BackboneElement implements IBaseBackboneElement { 2068 /** 2069 * Name for this instance of data. 2070 */ 2071 @Child(name = "name", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2072 @Description(shortDefinition="Name for this instance of data", formalDefinition="Name for this instance of data." ) 2073 protected IdType name; 2074 2075 /** 2076 * Type for this instance of data. 2077 */ 2078 @Child(name = "type", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2079 @Description(shortDefinition="Type for this instance of data", formalDefinition="Type for this instance of data." ) 2080 protected StringType type; 2081 2082 /** 2083 * Mode for this instance of data. 2084 */ 2085 @Child(name = "mode", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 2086 @Description(shortDefinition="source | target", formalDefinition="Mode for this instance of data." ) 2087 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-input-mode") 2088 protected Enumeration<StructureMapInputMode> mode; 2089 2090 /** 2091 * Documentation for this instance of data. 2092 */ 2093 @Child(name = "documentation", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2094 @Description(shortDefinition="Documentation for this instance of data", formalDefinition="Documentation for this instance of data." ) 2095 protected StringType documentation; 2096 2097 private static final long serialVersionUID = -25050724L; 2098 2099 /** 2100 * Constructor 2101 */ 2102 public StructureMapGroupInputComponent() { 2103 super(); 2104 } 2105 2106 /** 2107 * Constructor 2108 */ 2109 public StructureMapGroupInputComponent(IdType name, Enumeration<StructureMapInputMode> mode) { 2110 super(); 2111 this.name = name; 2112 this.mode = mode; 2113 } 2114 2115 /** 2116 * @return {@link #name} (Name for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2117 */ 2118 public IdType getNameElement() { 2119 if (this.name == null) 2120 if (Configuration.errorOnAutoCreate()) 2121 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.name"); 2122 else if (Configuration.doAutoCreate()) 2123 this.name = new IdType(); // bb 2124 return this.name; 2125 } 2126 2127 public boolean hasNameElement() { 2128 return this.name != null && !this.name.isEmpty(); 2129 } 2130 2131 public boolean hasName() { 2132 return this.name != null && !this.name.isEmpty(); 2133 } 2134 2135 /** 2136 * @param value {@link #name} (Name for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2137 */ 2138 public StructureMapGroupInputComponent setNameElement(IdType value) { 2139 this.name = value; 2140 return this; 2141 } 2142 2143 /** 2144 * @return Name for this instance of data. 2145 */ 2146 public String getName() { 2147 return this.name == null ? null : this.name.getValue(); 2148 } 2149 2150 /** 2151 * @param value Name for this instance of data. 2152 */ 2153 public StructureMapGroupInputComponent setName(String value) { 2154 if (this.name == null) 2155 this.name = new IdType(); 2156 this.name.setValue(value); 2157 return this; 2158 } 2159 2160 /** 2161 * @return {@link #type} (Type for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2162 */ 2163 public StringType getTypeElement() { 2164 if (this.type == null) 2165 if (Configuration.errorOnAutoCreate()) 2166 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.type"); 2167 else if (Configuration.doAutoCreate()) 2168 this.type = new StringType(); // bb 2169 return this.type; 2170 } 2171 2172 public boolean hasTypeElement() { 2173 return this.type != null && !this.type.isEmpty(); 2174 } 2175 2176 public boolean hasType() { 2177 return this.type != null && !this.type.isEmpty(); 2178 } 2179 2180 /** 2181 * @param value {@link #type} (Type for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2182 */ 2183 public StructureMapGroupInputComponent setTypeElement(StringType value) { 2184 this.type = value; 2185 return this; 2186 } 2187 2188 /** 2189 * @return Type for this instance of data. 2190 */ 2191 public String getType() { 2192 return this.type == null ? null : this.type.getValue(); 2193 } 2194 2195 /** 2196 * @param value Type for this instance of data. 2197 */ 2198 public StructureMapGroupInputComponent setType(String value) { 2199 if (Utilities.noString(value)) 2200 this.type = null; 2201 else { 2202 if (this.type == null) 2203 this.type = new StringType(); 2204 this.type.setValue(value); 2205 } 2206 return this; 2207 } 2208 2209 /** 2210 * @return {@link #mode} (Mode for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 2211 */ 2212 public Enumeration<StructureMapInputMode> getModeElement() { 2213 if (this.mode == null) 2214 if (Configuration.errorOnAutoCreate()) 2215 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.mode"); 2216 else if (Configuration.doAutoCreate()) 2217 this.mode = new Enumeration<StructureMapInputMode>(new StructureMapInputModeEnumFactory()); // bb 2218 return this.mode; 2219 } 2220 2221 public boolean hasModeElement() { 2222 return this.mode != null && !this.mode.isEmpty(); 2223 } 2224 2225 public boolean hasMode() { 2226 return this.mode != null && !this.mode.isEmpty(); 2227 } 2228 2229 /** 2230 * @param value {@link #mode} (Mode for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 2231 */ 2232 public StructureMapGroupInputComponent setModeElement(Enumeration<StructureMapInputMode> value) { 2233 this.mode = value; 2234 return this; 2235 } 2236 2237 /** 2238 * @return Mode for this instance of data. 2239 */ 2240 public StructureMapInputMode getMode() { 2241 return this.mode == null ? null : this.mode.getValue(); 2242 } 2243 2244 /** 2245 * @param value Mode for this instance of data. 2246 */ 2247 public StructureMapGroupInputComponent setMode(StructureMapInputMode value) { 2248 if (this.mode == null) 2249 this.mode = new Enumeration<StructureMapInputMode>(new StructureMapInputModeEnumFactory()); 2250 this.mode.setValue(value); 2251 return this; 2252 } 2253 2254 /** 2255 * @return {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2256 */ 2257 public StringType getDocumentationElement() { 2258 if (this.documentation == null) 2259 if (Configuration.errorOnAutoCreate()) 2260 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.documentation"); 2261 else if (Configuration.doAutoCreate()) 2262 this.documentation = new StringType(); // bb 2263 return this.documentation; 2264 } 2265 2266 public boolean hasDocumentationElement() { 2267 return this.documentation != null && !this.documentation.isEmpty(); 2268 } 2269 2270 public boolean hasDocumentation() { 2271 return this.documentation != null && !this.documentation.isEmpty(); 2272 } 2273 2274 /** 2275 * @param value {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2276 */ 2277 public StructureMapGroupInputComponent setDocumentationElement(StringType value) { 2278 this.documentation = value; 2279 return this; 2280 } 2281 2282 /** 2283 * @return Documentation for this instance of data. 2284 */ 2285 public String getDocumentation() { 2286 return this.documentation == null ? null : this.documentation.getValue(); 2287 } 2288 2289 /** 2290 * @param value Documentation for this instance of data. 2291 */ 2292 public StructureMapGroupInputComponent setDocumentation(String value) { 2293 if (Utilities.noString(value)) 2294 this.documentation = null; 2295 else { 2296 if (this.documentation == null) 2297 this.documentation = new StringType(); 2298 this.documentation.setValue(value); 2299 } 2300 return this; 2301 } 2302 2303 protected void listChildren(List<Property> children) { 2304 super.listChildren(children); 2305 children.add(new Property("name", "id", "Name for this instance of data.", 0, 1, name)); 2306 children.add(new Property("type", "string", "Type for this instance of data.", 0, 1, type)); 2307 children.add(new Property("mode", "code", "Mode for this instance of data.", 0, 1, mode)); 2308 children.add(new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation)); 2309 } 2310 2311 @Override 2312 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2313 switch (_hash) { 2314 case 3373707: /*name*/ return new Property("name", "id", "Name for this instance of data.", 0, 1, name); 2315 case 3575610: /*type*/ return new Property("type", "string", "Type for this instance of data.", 0, 1, type); 2316 case 3357091: /*mode*/ return new Property("mode", "code", "Mode for this instance of data.", 0, 1, mode); 2317 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation); 2318 default: return super.getNamedProperty(_hash, _name, _checkValid); 2319 } 2320 2321 } 2322 2323 @Override 2324 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2325 switch (hash) { 2326 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 2327 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // StringType 2328 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<StructureMapInputMode> 2329 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 2330 default: return super.getProperty(hash, name, checkValid); 2331 } 2332 2333 } 2334 2335 @Override 2336 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2337 switch (hash) { 2338 case 3373707: // name 2339 this.name = castToId(value); // IdType 2340 return value; 2341 case 3575610: // type 2342 this.type = castToString(value); // StringType 2343 return value; 2344 case 3357091: // mode 2345 value = new StructureMapInputModeEnumFactory().fromType(castToCode(value)); 2346 this.mode = (Enumeration) value; // Enumeration<StructureMapInputMode> 2347 return value; 2348 case 1587405498: // documentation 2349 this.documentation = castToString(value); // StringType 2350 return value; 2351 default: return super.setProperty(hash, name, value); 2352 } 2353 2354 } 2355 2356 @Override 2357 public Base setProperty(String name, Base value) throws FHIRException { 2358 if (name.equals("name")) { 2359 this.name = castToId(value); // IdType 2360 } else if (name.equals("type")) { 2361 this.type = castToString(value); // StringType 2362 } else if (name.equals("mode")) { 2363 value = new StructureMapInputModeEnumFactory().fromType(castToCode(value)); 2364 this.mode = (Enumeration) value; // Enumeration<StructureMapInputMode> 2365 } else if (name.equals("documentation")) { 2366 this.documentation = castToString(value); // StringType 2367 } else 2368 return super.setProperty(name, value); 2369 return value; 2370 } 2371 2372 @Override 2373 public Base makeProperty(int hash, String name) throws FHIRException { 2374 switch (hash) { 2375 case 3373707: return getNameElement(); 2376 case 3575610: return getTypeElement(); 2377 case 3357091: return getModeElement(); 2378 case 1587405498: return getDocumentationElement(); 2379 default: return super.makeProperty(hash, name); 2380 } 2381 2382 } 2383 2384 @Override 2385 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2386 switch (hash) { 2387 case 3373707: /*name*/ return new String[] {"id"}; 2388 case 3575610: /*type*/ return new String[] {"string"}; 2389 case 3357091: /*mode*/ return new String[] {"code"}; 2390 case 1587405498: /*documentation*/ return new String[] {"string"}; 2391 default: return super.getTypesForProperty(hash, name); 2392 } 2393 2394 } 2395 2396 @Override 2397 public Base addChild(String name) throws FHIRException { 2398 if (name.equals("name")) { 2399 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 2400 } 2401 else if (name.equals("type")) { 2402 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.type"); 2403 } 2404 else if (name.equals("mode")) { 2405 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.mode"); 2406 } 2407 else if (name.equals("documentation")) { 2408 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.documentation"); 2409 } 2410 else 2411 return super.addChild(name); 2412 } 2413 2414 public StructureMapGroupInputComponent copy() { 2415 StructureMapGroupInputComponent dst = new StructureMapGroupInputComponent(); 2416 copyValues(dst); 2417 dst.name = name == null ? null : name.copy(); 2418 dst.type = type == null ? null : type.copy(); 2419 dst.mode = mode == null ? null : mode.copy(); 2420 dst.documentation = documentation == null ? null : documentation.copy(); 2421 return dst; 2422 } 2423 2424 @Override 2425 public boolean equalsDeep(Base other_) { 2426 if (!super.equalsDeep(other_)) 2427 return false; 2428 if (!(other_ instanceof StructureMapGroupInputComponent)) 2429 return false; 2430 StructureMapGroupInputComponent o = (StructureMapGroupInputComponent) other_; 2431 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) && compareDeep(mode, o.mode, true) 2432 && compareDeep(documentation, o.documentation, true); 2433 } 2434 2435 @Override 2436 public boolean equalsShallow(Base other_) { 2437 if (!super.equalsShallow(other_)) 2438 return false; 2439 if (!(other_ instanceof StructureMapGroupInputComponent)) 2440 return false; 2441 StructureMapGroupInputComponent o = (StructureMapGroupInputComponent) other_; 2442 return compareValues(name, o.name, true) && compareValues(type, o.type, true) && compareValues(mode, o.mode, true) 2443 && compareValues(documentation, o.documentation, true); 2444 } 2445 2446 public boolean isEmpty() { 2447 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, mode, documentation 2448 ); 2449 } 2450 2451 public String fhirType() { 2452 return "StructureMap.group.input"; 2453 2454 } 2455 2456 } 2457 2458 @Block() 2459 public static class StructureMapGroupRuleComponent extends BackboneElement implements IBaseBackboneElement { 2460 /** 2461 * Name of the rule for internal references. 2462 */ 2463 @Child(name = "name", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2464 @Description(shortDefinition="Name of the rule for internal references", formalDefinition="Name of the rule for internal references." ) 2465 protected IdType name; 2466 2467 /** 2468 * Source inputs to the mapping. 2469 */ 2470 @Child(name = "source", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2471 @Description(shortDefinition="Source inputs to the mapping", formalDefinition="Source inputs to the mapping." ) 2472 protected List<StructureMapGroupRuleSourceComponent> source; 2473 2474 /** 2475 * Content to create because of this mapping rule. 2476 */ 2477 @Child(name = "target", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2478 @Description(shortDefinition="Content to create because of this mapping rule", formalDefinition="Content to create because of this mapping rule." ) 2479 protected List<StructureMapGroupRuleTargetComponent> target; 2480 2481 /** 2482 * Rules contained in this rule. 2483 */ 2484 @Child(name = "rule", type = {StructureMapGroupRuleComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2485 @Description(shortDefinition="Rules contained in this rule", formalDefinition="Rules contained in this rule." ) 2486 protected List<StructureMapGroupRuleComponent> rule; 2487 2488 /** 2489 * Which other rules to apply in the context of this rule. 2490 */ 2491 @Child(name = "dependent", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2492 @Description(shortDefinition="Which other rules to apply in the context of this rule", formalDefinition="Which other rules to apply in the context of this rule." ) 2493 protected List<StructureMapGroupRuleDependentComponent> dependent; 2494 2495 /** 2496 * Documentation for this instance of data. 2497 */ 2498 @Child(name = "documentation", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 2499 @Description(shortDefinition="Documentation for this instance of data", formalDefinition="Documentation for this instance of data." ) 2500 protected StringType documentation; 2501 2502 private static final long serialVersionUID = 773925517L; 2503 2504 /** 2505 * Constructor 2506 */ 2507 public StructureMapGroupRuleComponent() { 2508 super(); 2509 } 2510 2511 /** 2512 * Constructor 2513 */ 2514 public StructureMapGroupRuleComponent(IdType name) { 2515 super(); 2516 this.name = name; 2517 } 2518 2519 /** 2520 * @return {@link #name} (Name of the rule for internal references.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2521 */ 2522 public IdType getNameElement() { 2523 if (this.name == null) 2524 if (Configuration.errorOnAutoCreate()) 2525 throw new Error("Attempt to auto-create StructureMapGroupRuleComponent.name"); 2526 else if (Configuration.doAutoCreate()) 2527 this.name = new IdType(); // bb 2528 return this.name; 2529 } 2530 2531 public boolean hasNameElement() { 2532 return this.name != null && !this.name.isEmpty(); 2533 } 2534 2535 public boolean hasName() { 2536 return this.name != null && !this.name.isEmpty(); 2537 } 2538 2539 /** 2540 * @param value {@link #name} (Name of the rule for internal references.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2541 */ 2542 public StructureMapGroupRuleComponent setNameElement(IdType value) { 2543 this.name = value; 2544 return this; 2545 } 2546 2547 /** 2548 * @return Name of the rule for internal references. 2549 */ 2550 public String getName() { 2551 return this.name == null ? null : this.name.getValue(); 2552 } 2553 2554 /** 2555 * @param value Name of the rule for internal references. 2556 */ 2557 public StructureMapGroupRuleComponent setName(String value) { 2558 if (this.name == null) 2559 this.name = new IdType(); 2560 this.name.setValue(value); 2561 return this; 2562 } 2563 2564 /** 2565 * @return {@link #source} (Source inputs to the mapping.) 2566 */ 2567 public List<StructureMapGroupRuleSourceComponent> getSource() { 2568 if (this.source == null) 2569 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 2570 return this.source; 2571 } 2572 2573 /** 2574 * @return Returns a reference to <code>this</code> for easy method chaining 2575 */ 2576 public StructureMapGroupRuleComponent setSource(List<StructureMapGroupRuleSourceComponent> theSource) { 2577 this.source = theSource; 2578 return this; 2579 } 2580 2581 public boolean hasSource() { 2582 if (this.source == null) 2583 return false; 2584 for (StructureMapGroupRuleSourceComponent item : this.source) 2585 if (!item.isEmpty()) 2586 return true; 2587 return false; 2588 } 2589 2590 public StructureMapGroupRuleSourceComponent addSource() { //3 2591 StructureMapGroupRuleSourceComponent t = new StructureMapGroupRuleSourceComponent(); 2592 if (this.source == null) 2593 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 2594 this.source.add(t); 2595 return t; 2596 } 2597 2598 public StructureMapGroupRuleComponent addSource(StructureMapGroupRuleSourceComponent t) { //3 2599 if (t == null) 2600 return this; 2601 if (this.source == null) 2602 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 2603 this.source.add(t); 2604 return this; 2605 } 2606 2607 /** 2608 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist 2609 */ 2610 public StructureMapGroupRuleSourceComponent getSourceFirstRep() { 2611 if (getSource().isEmpty()) { 2612 addSource(); 2613 } 2614 return getSource().get(0); 2615 } 2616 2617 /** 2618 * @return {@link #target} (Content to create because of this mapping rule.) 2619 */ 2620 public List<StructureMapGroupRuleTargetComponent> getTarget() { 2621 if (this.target == null) 2622 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 2623 return this.target; 2624 } 2625 2626 /** 2627 * @return Returns a reference to <code>this</code> for easy method chaining 2628 */ 2629 public StructureMapGroupRuleComponent setTarget(List<StructureMapGroupRuleTargetComponent> theTarget) { 2630 this.target = theTarget; 2631 return this; 2632 } 2633 2634 public boolean hasTarget() { 2635 if (this.target == null) 2636 return false; 2637 for (StructureMapGroupRuleTargetComponent item : this.target) 2638 if (!item.isEmpty()) 2639 return true; 2640 return false; 2641 } 2642 2643 public StructureMapGroupRuleTargetComponent addTarget() { //3 2644 StructureMapGroupRuleTargetComponent t = new StructureMapGroupRuleTargetComponent(); 2645 if (this.target == null) 2646 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 2647 this.target.add(t); 2648 return t; 2649 } 2650 2651 public StructureMapGroupRuleComponent addTarget(StructureMapGroupRuleTargetComponent t) { //3 2652 if (t == null) 2653 return this; 2654 if (this.target == null) 2655 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 2656 this.target.add(t); 2657 return this; 2658 } 2659 2660 /** 2661 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist 2662 */ 2663 public StructureMapGroupRuleTargetComponent getTargetFirstRep() { 2664 if (getTarget().isEmpty()) { 2665 addTarget(); 2666 } 2667 return getTarget().get(0); 2668 } 2669 2670 /** 2671 * @return {@link #rule} (Rules contained in this rule.) 2672 */ 2673 public List<StructureMapGroupRuleComponent> getRule() { 2674 if (this.rule == null) 2675 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2676 return this.rule; 2677 } 2678 2679 /** 2680 * @return Returns a reference to <code>this</code> for easy method chaining 2681 */ 2682 public StructureMapGroupRuleComponent setRule(List<StructureMapGroupRuleComponent> theRule) { 2683 this.rule = theRule; 2684 return this; 2685 } 2686 2687 public boolean hasRule() { 2688 if (this.rule == null) 2689 return false; 2690 for (StructureMapGroupRuleComponent item : this.rule) 2691 if (!item.isEmpty()) 2692 return true; 2693 return false; 2694 } 2695 2696 public StructureMapGroupRuleComponent addRule() { //3 2697 StructureMapGroupRuleComponent t = new StructureMapGroupRuleComponent(); 2698 if (this.rule == null) 2699 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2700 this.rule.add(t); 2701 return t; 2702 } 2703 2704 public StructureMapGroupRuleComponent addRule(StructureMapGroupRuleComponent t) { //3 2705 if (t == null) 2706 return this; 2707 if (this.rule == null) 2708 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2709 this.rule.add(t); 2710 return this; 2711 } 2712 2713 /** 2714 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist 2715 */ 2716 public StructureMapGroupRuleComponent getRuleFirstRep() { 2717 if (getRule().isEmpty()) { 2718 addRule(); 2719 } 2720 return getRule().get(0); 2721 } 2722 2723 /** 2724 * @return {@link #dependent} (Which other rules to apply in the context of this rule.) 2725 */ 2726 public List<StructureMapGroupRuleDependentComponent> getDependent() { 2727 if (this.dependent == null) 2728 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 2729 return this.dependent; 2730 } 2731 2732 /** 2733 * @return Returns a reference to <code>this</code> for easy method chaining 2734 */ 2735 public StructureMapGroupRuleComponent setDependent(List<StructureMapGroupRuleDependentComponent> theDependent) { 2736 this.dependent = theDependent; 2737 return this; 2738 } 2739 2740 public boolean hasDependent() { 2741 if (this.dependent == null) 2742 return false; 2743 for (StructureMapGroupRuleDependentComponent item : this.dependent) 2744 if (!item.isEmpty()) 2745 return true; 2746 return false; 2747 } 2748 2749 public StructureMapGroupRuleDependentComponent addDependent() { //3 2750 StructureMapGroupRuleDependentComponent t = new StructureMapGroupRuleDependentComponent(); 2751 if (this.dependent == null) 2752 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 2753 this.dependent.add(t); 2754 return t; 2755 } 2756 2757 public StructureMapGroupRuleComponent addDependent(StructureMapGroupRuleDependentComponent t) { //3 2758 if (t == null) 2759 return this; 2760 if (this.dependent == null) 2761 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 2762 this.dependent.add(t); 2763 return this; 2764 } 2765 2766 /** 2767 * @return The first repetition of repeating field {@link #dependent}, creating it if it does not already exist 2768 */ 2769 public StructureMapGroupRuleDependentComponent getDependentFirstRep() { 2770 if (getDependent().isEmpty()) { 2771 addDependent(); 2772 } 2773 return getDependent().get(0); 2774 } 2775 2776 /** 2777 * @return {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2778 */ 2779 public StringType getDocumentationElement() { 2780 if (this.documentation == null) 2781 if (Configuration.errorOnAutoCreate()) 2782 throw new Error("Attempt to auto-create StructureMapGroupRuleComponent.documentation"); 2783 else if (Configuration.doAutoCreate()) 2784 this.documentation = new StringType(); // bb 2785 return this.documentation; 2786 } 2787 2788 public boolean hasDocumentationElement() { 2789 return this.documentation != null && !this.documentation.isEmpty(); 2790 } 2791 2792 public boolean hasDocumentation() { 2793 return this.documentation != null && !this.documentation.isEmpty(); 2794 } 2795 2796 /** 2797 * @param value {@link #documentation} (Documentation for this instance of data.). This is the underlying object with id, value and extensions. The accessor "getDocumentation" gives direct access to the value 2798 */ 2799 public StructureMapGroupRuleComponent setDocumentationElement(StringType value) { 2800 this.documentation = value; 2801 return this; 2802 } 2803 2804 /** 2805 * @return Documentation for this instance of data. 2806 */ 2807 public String getDocumentation() { 2808 return this.documentation == null ? null : this.documentation.getValue(); 2809 } 2810 2811 /** 2812 * @param value Documentation for this instance of data. 2813 */ 2814 public StructureMapGroupRuleComponent setDocumentation(String value) { 2815 if (Utilities.noString(value)) 2816 this.documentation = null; 2817 else { 2818 if (this.documentation == null) 2819 this.documentation = new StringType(); 2820 this.documentation.setValue(value); 2821 } 2822 return this; 2823 } 2824 2825 protected void listChildren(List<Property> children) { 2826 super.listChildren(children); 2827 children.add(new Property("name", "id", "Name of the rule for internal references.", 0, 1, name)); 2828 children.add(new Property("source", "", "Source inputs to the mapping.", 0, java.lang.Integer.MAX_VALUE, source)); 2829 children.add(new Property("target", "", "Content to create because of this mapping rule.", 0, java.lang.Integer.MAX_VALUE, target)); 2830 children.add(new Property("rule", "@StructureMap.group.rule", "Rules contained in this rule.", 0, java.lang.Integer.MAX_VALUE, rule)); 2831 children.add(new Property("dependent", "", "Which other rules to apply in the context of this rule.", 0, java.lang.Integer.MAX_VALUE, dependent)); 2832 children.add(new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation)); 2833 } 2834 2835 @Override 2836 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2837 switch (_hash) { 2838 case 3373707: /*name*/ return new Property("name", "id", "Name of the rule for internal references.", 0, 1, name); 2839 case -896505829: /*source*/ return new Property("source", "", "Source inputs to the mapping.", 0, java.lang.Integer.MAX_VALUE, source); 2840 case -880905839: /*target*/ return new Property("target", "", "Content to create because of this mapping rule.", 0, java.lang.Integer.MAX_VALUE, target); 2841 case 3512060: /*rule*/ return new Property("rule", "@StructureMap.group.rule", "Rules contained in this rule.", 0, java.lang.Integer.MAX_VALUE, rule); 2842 case -1109226753: /*dependent*/ return new Property("dependent", "", "Which other rules to apply in the context of this rule.", 0, java.lang.Integer.MAX_VALUE, dependent); 2843 case 1587405498: /*documentation*/ return new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation); 2844 default: return super.getNamedProperty(_hash, _name, _checkValid); 2845 } 2846 2847 } 2848 2849 @Override 2850 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2851 switch (hash) { 2852 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 2853 case -896505829: /*source*/ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // StructureMapGroupRuleSourceComponent 2854 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // StructureMapGroupRuleTargetComponent 2855 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // StructureMapGroupRuleComponent 2856 case -1109226753: /*dependent*/ return this.dependent == null ? new Base[0] : this.dependent.toArray(new Base[this.dependent.size()]); // StructureMapGroupRuleDependentComponent 2857 case 1587405498: /*documentation*/ return this.documentation == null ? new Base[0] : new Base[] {this.documentation}; // StringType 2858 default: return super.getProperty(hash, name, checkValid); 2859 } 2860 2861 } 2862 2863 @Override 2864 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2865 switch (hash) { 2866 case 3373707: // name 2867 this.name = castToId(value); // IdType 2868 return value; 2869 case -896505829: // source 2870 this.getSource().add((StructureMapGroupRuleSourceComponent) value); // StructureMapGroupRuleSourceComponent 2871 return value; 2872 case -880905839: // target 2873 this.getTarget().add((StructureMapGroupRuleTargetComponent) value); // StructureMapGroupRuleTargetComponent 2874 return value; 2875 case 3512060: // rule 2876 this.getRule().add((StructureMapGroupRuleComponent) value); // StructureMapGroupRuleComponent 2877 return value; 2878 case -1109226753: // dependent 2879 this.getDependent().add((StructureMapGroupRuleDependentComponent) value); // StructureMapGroupRuleDependentComponent 2880 return value; 2881 case 1587405498: // documentation 2882 this.documentation = castToString(value); // StringType 2883 return value; 2884 default: return super.setProperty(hash, name, value); 2885 } 2886 2887 } 2888 2889 @Override 2890 public Base setProperty(String name, Base value) throws FHIRException { 2891 if (name.equals("name")) { 2892 this.name = castToId(value); // IdType 2893 } else if (name.equals("source")) { 2894 this.getSource().add((StructureMapGroupRuleSourceComponent) value); 2895 } else if (name.equals("target")) { 2896 this.getTarget().add((StructureMapGroupRuleTargetComponent) value); 2897 } else if (name.equals("rule")) { 2898 this.getRule().add((StructureMapGroupRuleComponent) value); 2899 } else if (name.equals("dependent")) { 2900 this.getDependent().add((StructureMapGroupRuleDependentComponent) value); 2901 } else if (name.equals("documentation")) { 2902 this.documentation = castToString(value); // StringType 2903 } else 2904 return super.setProperty(name, value); 2905 return value; 2906 } 2907 2908 @Override 2909 public Base makeProperty(int hash, String name) throws FHIRException { 2910 switch (hash) { 2911 case 3373707: return getNameElement(); 2912 case -896505829: return addSource(); 2913 case -880905839: return addTarget(); 2914 case 3512060: return addRule(); 2915 case -1109226753: return addDependent(); 2916 case 1587405498: return getDocumentationElement(); 2917 default: return super.makeProperty(hash, name); 2918 } 2919 2920 } 2921 2922 @Override 2923 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2924 switch (hash) { 2925 case 3373707: /*name*/ return new String[] {"id"}; 2926 case -896505829: /*source*/ return new String[] {}; 2927 case -880905839: /*target*/ return new String[] {}; 2928 case 3512060: /*rule*/ return new String[] {"@StructureMap.group.rule"}; 2929 case -1109226753: /*dependent*/ return new String[] {}; 2930 case 1587405498: /*documentation*/ return new String[] {"string"}; 2931 default: return super.getTypesForProperty(hash, name); 2932 } 2933 2934 } 2935 2936 @Override 2937 public Base addChild(String name) throws FHIRException { 2938 if (name.equals("name")) { 2939 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 2940 } 2941 else if (name.equals("source")) { 2942 return addSource(); 2943 } 2944 else if (name.equals("target")) { 2945 return addTarget(); 2946 } 2947 else if (name.equals("rule")) { 2948 return addRule(); 2949 } 2950 else if (name.equals("dependent")) { 2951 return addDependent(); 2952 } 2953 else if (name.equals("documentation")) { 2954 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.documentation"); 2955 } 2956 else 2957 return super.addChild(name); 2958 } 2959 2960 public StructureMapGroupRuleComponent copy() { 2961 StructureMapGroupRuleComponent dst = new StructureMapGroupRuleComponent(); 2962 copyValues(dst); 2963 dst.name = name == null ? null : name.copy(); 2964 if (source != null) { 2965 dst.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 2966 for (StructureMapGroupRuleSourceComponent i : source) 2967 dst.source.add(i.copy()); 2968 }; 2969 if (target != null) { 2970 dst.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 2971 for (StructureMapGroupRuleTargetComponent i : target) 2972 dst.target.add(i.copy()); 2973 }; 2974 if (rule != null) { 2975 dst.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2976 for (StructureMapGroupRuleComponent i : rule) 2977 dst.rule.add(i.copy()); 2978 }; 2979 if (dependent != null) { 2980 dst.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 2981 for (StructureMapGroupRuleDependentComponent i : dependent) 2982 dst.dependent.add(i.copy()); 2983 }; 2984 dst.documentation = documentation == null ? null : documentation.copy(); 2985 return dst; 2986 } 2987 2988 @Override 2989 public boolean equalsDeep(Base other_) { 2990 if (!super.equalsDeep(other_)) 2991 return false; 2992 if (!(other_ instanceof StructureMapGroupRuleComponent)) 2993 return false; 2994 StructureMapGroupRuleComponent o = (StructureMapGroupRuleComponent) other_; 2995 return compareDeep(name, o.name, true) && compareDeep(source, o.source, true) && compareDeep(target, o.target, true) 2996 && compareDeep(rule, o.rule, true) && compareDeep(dependent, o.dependent, true) && compareDeep(documentation, o.documentation, true) 2997 ; 2998 } 2999 3000 @Override 3001 public boolean equalsShallow(Base other_) { 3002 if (!super.equalsShallow(other_)) 3003 return false; 3004 if (!(other_ instanceof StructureMapGroupRuleComponent)) 3005 return false; 3006 StructureMapGroupRuleComponent o = (StructureMapGroupRuleComponent) other_; 3007 return compareValues(name, o.name, true) && compareValues(documentation, o.documentation, true); 3008 } 3009 3010 public boolean isEmpty() { 3011 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, source, target, rule 3012 , dependent, documentation); 3013 } 3014 3015 public String fhirType() { 3016 return "StructureMap.group.rule"; 3017 3018 } 3019 3020// added from java-adornments.txt: 3021 3022 public String toString() { 3023 return StructureMapUtilities.ruleToString(this); 3024 } 3025 3026 3027// end addition 3028 } 3029 3030 @Block() 3031 public static class StructureMapGroupRuleSourceComponent extends BackboneElement implements IBaseBackboneElement { 3032 /** 3033 * Type or variable this rule applies to. 3034 */ 3035 @Child(name = "context", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 3036 @Description(shortDefinition="Type or variable this rule applies to", formalDefinition="Type or variable this rule applies to." ) 3037 protected IdType context; 3038 3039 /** 3040 * Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content. 3041 */ 3042 @Child(name = "min", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3043 @Description(shortDefinition="Specified minimum cardinality", formalDefinition="Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content." ) 3044 protected IntegerType min; 3045 3046 /** 3047 * Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value). 3048 */ 3049 @Child(name = "max", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 3050 @Description(shortDefinition="Specified maximum cardinality (number or *)", formalDefinition="Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value)." ) 3051 protected StringType max; 3052 3053 /** 3054 * Specified type for the element. This works as a condition on the mapping - use for polymorphic elements. 3055 */ 3056 @Child(name = "type", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3057 @Description(shortDefinition="Rule only applies if source has this type", formalDefinition="Specified type for the element. This works as a condition on the mapping - use for polymorphic elements." ) 3058 protected StringType type; 3059 3060 /** 3061 * A value to use if there is no existing value in the source object. 3062 */ 3063 @Child(name = "defaultValue", type = {}, order=5, min=0, max=1, modifier=false, summary=true) 3064 @Description(shortDefinition="Default value if no value exists", formalDefinition="A value to use if there is no existing value in the source object." ) 3065 protected org.hl7.fhir.dstu3.model.Type defaultValue; 3066 3067 /** 3068 * Optional field for this source. 3069 */ 3070 @Child(name = "element", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 3071 @Description(shortDefinition="Optional field for this source", formalDefinition="Optional field for this source." ) 3072 protected StringType element; 3073 3074 /** 3075 * How to handle the list mode for this element. 3076 */ 3077 @Child(name = "listMode", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 3078 @Description(shortDefinition="first | not_first | last | not_last | only_one", formalDefinition="How to handle the list mode for this element." ) 3079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-source-list-mode") 3080 protected Enumeration<StructureMapSourceListMode> listMode; 3081 3082 /** 3083 * Named context for field, if a field is specified. 3084 */ 3085 @Child(name = "variable", type = {IdType.class}, order=8, min=0, max=1, modifier=false, summary=true) 3086 @Description(shortDefinition="Named context for field, if a field is specified", formalDefinition="Named context for field, if a field is specified." ) 3087 protected IdType variable; 3088 3089 /** 3090 * FHIRPath expression - must be true or the rule does not apply. 3091 */ 3092 @Child(name = "condition", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 3093 @Description(shortDefinition="FHIRPath expression - must be true or the rule does not apply", formalDefinition="FHIRPath expression - must be true or the rule does not apply." ) 3094 protected StringType condition; 3095 3096 /** 3097 * FHIRPath expression - must be true or the mapping engine throws an error instead of completing. 3098 */ 3099 @Child(name = "check", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=true) 3100 @Description(shortDefinition="FHIRPath expression - must be true or the mapping engine throws an error instead of completing", formalDefinition="FHIRPath expression - must be true or the mapping engine throws an error instead of completing." ) 3101 protected StringType check; 3102 3103 private static final long serialVersionUID = 1893118862L; 3104 3105 /** 3106 * Constructor 3107 */ 3108 public StructureMapGroupRuleSourceComponent() { 3109 super(); 3110 } 3111 3112 /** 3113 * Constructor 3114 */ 3115 public StructureMapGroupRuleSourceComponent(IdType context) { 3116 super(); 3117 this.context = context; 3118 } 3119 3120 /** 3121 * @return {@link #context} (Type or variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 3122 */ 3123 public IdType getContextElement() { 3124 if (this.context == null) 3125 if (Configuration.errorOnAutoCreate()) 3126 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.context"); 3127 else if (Configuration.doAutoCreate()) 3128 this.context = new IdType(); // bb 3129 return this.context; 3130 } 3131 3132 public boolean hasContextElement() { 3133 return this.context != null && !this.context.isEmpty(); 3134 } 3135 3136 public boolean hasContext() { 3137 return this.context != null && !this.context.isEmpty(); 3138 } 3139 3140 /** 3141 * @param value {@link #context} (Type or variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 3142 */ 3143 public StructureMapGroupRuleSourceComponent setContextElement(IdType value) { 3144 this.context = value; 3145 return this; 3146 } 3147 3148 /** 3149 * @return Type or variable this rule applies to. 3150 */ 3151 public String getContext() { 3152 return this.context == null ? null : this.context.getValue(); 3153 } 3154 3155 /** 3156 * @param value Type or variable this rule applies to. 3157 */ 3158 public StructureMapGroupRuleSourceComponent setContext(String value) { 3159 if (this.context == null) 3160 this.context = new IdType(); 3161 this.context.setValue(value); 3162 return this; 3163 } 3164 3165 /** 3166 * @return {@link #min} (Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 3167 */ 3168 public IntegerType getMinElement() { 3169 if (this.min == null) 3170 if (Configuration.errorOnAutoCreate()) 3171 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.min"); 3172 else if (Configuration.doAutoCreate()) 3173 this.min = new IntegerType(); // bb 3174 return this.min; 3175 } 3176 3177 public boolean hasMinElement() { 3178 return this.min != null && !this.min.isEmpty(); 3179 } 3180 3181 public boolean hasMin() { 3182 return this.min != null && !this.min.isEmpty(); 3183 } 3184 3185 /** 3186 * @param value {@link #min} (Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 3187 */ 3188 public StructureMapGroupRuleSourceComponent setMinElement(IntegerType value) { 3189 this.min = value; 3190 return this; 3191 } 3192 3193 /** 3194 * @return Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content. 3195 */ 3196 public int getMin() { 3197 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 3198 } 3199 3200 /** 3201 * @param value Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content. 3202 */ 3203 public StructureMapGroupRuleSourceComponent setMin(int value) { 3204 if (this.min == null) 3205 this.min = new IntegerType(); 3206 this.min.setValue(value); 3207 return this; 3208 } 3209 3210 /** 3211 * @return {@link #max} (Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 3212 */ 3213 public StringType getMaxElement() { 3214 if (this.max == null) 3215 if (Configuration.errorOnAutoCreate()) 3216 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.max"); 3217 else if (Configuration.doAutoCreate()) 3218 this.max = new StringType(); // bb 3219 return this.max; 3220 } 3221 3222 public boolean hasMaxElement() { 3223 return this.max != null && !this.max.isEmpty(); 3224 } 3225 3226 public boolean hasMax() { 3227 return this.max != null && !this.max.isEmpty(); 3228 } 3229 3230 /** 3231 * @param value {@link #max} (Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 3232 */ 3233 public StructureMapGroupRuleSourceComponent setMaxElement(StringType value) { 3234 this.max = value; 3235 return this; 3236 } 3237 3238 /** 3239 * @return Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value). 3240 */ 3241 public String getMax() { 3242 return this.max == null ? null : this.max.getValue(); 3243 } 3244 3245 /** 3246 * @param value Specified maximum cardinality for the element - a number or a "*". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value). 3247 */ 3248 public StructureMapGroupRuleSourceComponent setMax(String value) { 3249 if (Utilities.noString(value)) 3250 this.max = null; 3251 else { 3252 if (this.max == null) 3253 this.max = new StringType(); 3254 this.max.setValue(value); 3255 } 3256 return this; 3257 } 3258 3259 /** 3260 * @return {@link #type} (Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3261 */ 3262 public StringType getTypeElement() { 3263 if (this.type == null) 3264 if (Configuration.errorOnAutoCreate()) 3265 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.type"); 3266 else if (Configuration.doAutoCreate()) 3267 this.type = new StringType(); // bb 3268 return this.type; 3269 } 3270 3271 public boolean hasTypeElement() { 3272 return this.type != null && !this.type.isEmpty(); 3273 } 3274 3275 public boolean hasType() { 3276 return this.type != null && !this.type.isEmpty(); 3277 } 3278 3279 /** 3280 * @param value {@link #type} (Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3281 */ 3282 public StructureMapGroupRuleSourceComponent setTypeElement(StringType value) { 3283 this.type = value; 3284 return this; 3285 } 3286 3287 /** 3288 * @return Specified type for the element. This works as a condition on the mapping - use for polymorphic elements. 3289 */ 3290 public String getType() { 3291 return this.type == null ? null : this.type.getValue(); 3292 } 3293 3294 /** 3295 * @param value Specified type for the element. This works as a condition on the mapping - use for polymorphic elements. 3296 */ 3297 public StructureMapGroupRuleSourceComponent setType(String value) { 3298 if (Utilities.noString(value)) 3299 this.type = null; 3300 else { 3301 if (this.type == null) 3302 this.type = new StringType(); 3303 this.type.setValue(value); 3304 } 3305 return this; 3306 } 3307 3308 /** 3309 * @return {@link #defaultValue} (A value to use if there is no existing value in the source object.) 3310 */ 3311 public org.hl7.fhir.dstu3.model.Type getDefaultValue() { 3312 return this.defaultValue; 3313 } 3314 3315 public boolean hasDefaultValue() { 3316 return this.defaultValue != null && !this.defaultValue.isEmpty(); 3317 } 3318 3319 /** 3320 * @param value {@link #defaultValue} (A value to use if there is no existing value in the source object.) 3321 */ 3322 public StructureMapGroupRuleSourceComponent setDefaultValue(org.hl7.fhir.dstu3.model.Type value) { 3323 this.defaultValue = value; 3324 return this; 3325 } 3326 3327 /** 3328 * @return {@link #element} (Optional field for this source.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 3329 */ 3330 public StringType getElementElement() { 3331 if (this.element == null) 3332 if (Configuration.errorOnAutoCreate()) 3333 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.element"); 3334 else if (Configuration.doAutoCreate()) 3335 this.element = new StringType(); // bb 3336 return this.element; 3337 } 3338 3339 public boolean hasElementElement() { 3340 return this.element != null && !this.element.isEmpty(); 3341 } 3342 3343 public boolean hasElement() { 3344 return this.element != null && !this.element.isEmpty(); 3345 } 3346 3347 /** 3348 * @param value {@link #element} (Optional field for this source.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 3349 */ 3350 public StructureMapGroupRuleSourceComponent setElementElement(StringType value) { 3351 this.element = value; 3352 return this; 3353 } 3354 3355 /** 3356 * @return Optional field for this source. 3357 */ 3358 public String getElement() { 3359 return this.element == null ? null : this.element.getValue(); 3360 } 3361 3362 /** 3363 * @param value Optional field for this source. 3364 */ 3365 public StructureMapGroupRuleSourceComponent setElement(String value) { 3366 if (Utilities.noString(value)) 3367 this.element = null; 3368 else { 3369 if (this.element == null) 3370 this.element = new StringType(); 3371 this.element.setValue(value); 3372 } 3373 return this; 3374 } 3375 3376 /** 3377 * @return {@link #listMode} (How to handle the list mode for this element.). This is the underlying object with id, value and extensions. The accessor "getListMode" gives direct access to the value 3378 */ 3379 public Enumeration<StructureMapSourceListMode> getListModeElement() { 3380 if (this.listMode == null) 3381 if (Configuration.errorOnAutoCreate()) 3382 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.listMode"); 3383 else if (Configuration.doAutoCreate()) 3384 this.listMode = new Enumeration<StructureMapSourceListMode>(new StructureMapSourceListModeEnumFactory()); // bb 3385 return this.listMode; 3386 } 3387 3388 public boolean hasListModeElement() { 3389 return this.listMode != null && !this.listMode.isEmpty(); 3390 } 3391 3392 public boolean hasListMode() { 3393 return this.listMode != null && !this.listMode.isEmpty(); 3394 } 3395 3396 /** 3397 * @param value {@link #listMode} (How to handle the list mode for this element.). This is the underlying object with id, value and extensions. The accessor "getListMode" gives direct access to the value 3398 */ 3399 public StructureMapGroupRuleSourceComponent setListModeElement(Enumeration<StructureMapSourceListMode> value) { 3400 this.listMode = value; 3401 return this; 3402 } 3403 3404 /** 3405 * @return How to handle the list mode for this element. 3406 */ 3407 public StructureMapSourceListMode getListMode() { 3408 return this.listMode == null ? null : this.listMode.getValue(); 3409 } 3410 3411 /** 3412 * @param value How to handle the list mode for this element. 3413 */ 3414 public StructureMapGroupRuleSourceComponent setListMode(StructureMapSourceListMode value) { 3415 if (value == null) 3416 this.listMode = null; 3417 else { 3418 if (this.listMode == null) 3419 this.listMode = new Enumeration<StructureMapSourceListMode>(new StructureMapSourceListModeEnumFactory()); 3420 this.listMode.setValue(value); 3421 } 3422 return this; 3423 } 3424 3425 /** 3426 * @return {@link #variable} (Named context for field, if a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 3427 */ 3428 public IdType getVariableElement() { 3429 if (this.variable == null) 3430 if (Configuration.errorOnAutoCreate()) 3431 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.variable"); 3432 else if (Configuration.doAutoCreate()) 3433 this.variable = new IdType(); // bb 3434 return this.variable; 3435 } 3436 3437 public boolean hasVariableElement() { 3438 return this.variable != null && !this.variable.isEmpty(); 3439 } 3440 3441 public boolean hasVariable() { 3442 return this.variable != null && !this.variable.isEmpty(); 3443 } 3444 3445 /** 3446 * @param value {@link #variable} (Named context for field, if a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 3447 */ 3448 public StructureMapGroupRuleSourceComponent setVariableElement(IdType value) { 3449 this.variable = value; 3450 return this; 3451 } 3452 3453 /** 3454 * @return Named context for field, if a field is specified. 3455 */ 3456 public String getVariable() { 3457 return this.variable == null ? null : this.variable.getValue(); 3458 } 3459 3460 /** 3461 * @param value Named context for field, if a field is specified. 3462 */ 3463 public StructureMapGroupRuleSourceComponent setVariable(String value) { 3464 if (Utilities.noString(value)) 3465 this.variable = null; 3466 else { 3467 if (this.variable == null) 3468 this.variable = new IdType(); 3469 this.variable.setValue(value); 3470 } 3471 return this; 3472 } 3473 3474 /** 3475 * @return {@link #condition} (FHIRPath expression - must be true or the rule does not apply.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 3476 */ 3477 public StringType getConditionElement() { 3478 if (this.condition == null) 3479 if (Configuration.errorOnAutoCreate()) 3480 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.condition"); 3481 else if (Configuration.doAutoCreate()) 3482 this.condition = new StringType(); // bb 3483 return this.condition; 3484 } 3485 3486 public boolean hasConditionElement() { 3487 return this.condition != null && !this.condition.isEmpty(); 3488 } 3489 3490 public boolean hasCondition() { 3491 return this.condition != null && !this.condition.isEmpty(); 3492 } 3493 3494 /** 3495 * @param value {@link #condition} (FHIRPath expression - must be true or the rule does not apply.). This is the underlying object with id, value and extensions. The accessor "getCondition" gives direct access to the value 3496 */ 3497 public StructureMapGroupRuleSourceComponent setConditionElement(StringType value) { 3498 this.condition = value; 3499 return this; 3500 } 3501 3502 /** 3503 * @return FHIRPath expression - must be true or the rule does not apply. 3504 */ 3505 public String getCondition() { 3506 return this.condition == null ? null : this.condition.getValue(); 3507 } 3508 3509 /** 3510 * @param value FHIRPath expression - must be true or the rule does not apply. 3511 */ 3512 public StructureMapGroupRuleSourceComponent setCondition(String value) { 3513 if (Utilities.noString(value)) 3514 this.condition = null; 3515 else { 3516 if (this.condition == null) 3517 this.condition = new StringType(); 3518 this.condition.setValue(value); 3519 } 3520 return this; 3521 } 3522 3523 /** 3524 * @return {@link #check} (FHIRPath expression - must be true or the mapping engine throws an error instead of completing.). This is the underlying object with id, value and extensions. The accessor "getCheck" gives direct access to the value 3525 */ 3526 public StringType getCheckElement() { 3527 if (this.check == null) 3528 if (Configuration.errorOnAutoCreate()) 3529 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.check"); 3530 else if (Configuration.doAutoCreate()) 3531 this.check = new StringType(); // bb 3532 return this.check; 3533 } 3534 3535 public boolean hasCheckElement() { 3536 return this.check != null && !this.check.isEmpty(); 3537 } 3538 3539 public boolean hasCheck() { 3540 return this.check != null && !this.check.isEmpty(); 3541 } 3542 3543 /** 3544 * @param value {@link #check} (FHIRPath expression - must be true or the mapping engine throws an error instead of completing.). This is the underlying object with id, value and extensions. The accessor "getCheck" gives direct access to the value 3545 */ 3546 public StructureMapGroupRuleSourceComponent setCheckElement(StringType value) { 3547 this.check = value; 3548 return this; 3549 } 3550 3551 /** 3552 * @return FHIRPath expression - must be true or the mapping engine throws an error instead of completing. 3553 */ 3554 public String getCheck() { 3555 return this.check == null ? null : this.check.getValue(); 3556 } 3557 3558 /** 3559 * @param value FHIRPath expression - must be true or the mapping engine throws an error instead of completing. 3560 */ 3561 public StructureMapGroupRuleSourceComponent setCheck(String value) { 3562 if (Utilities.noString(value)) 3563 this.check = null; 3564 else { 3565 if (this.check == null) 3566 this.check = new StringType(); 3567 this.check.setValue(value); 3568 } 3569 return this; 3570 } 3571 3572 protected void listChildren(List<Property> children) { 3573 super.listChildren(children); 3574 children.add(new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context)); 3575 children.add(new Property("min", "integer", "Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.", 0, 1, min)); 3576 children.add(new Property("max", "string", "Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).", 0, 1, max)); 3577 children.add(new Property("type", "string", "Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.", 0, 1, type)); 3578 children.add(new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue)); 3579 children.add(new Property("element", "string", "Optional field for this source.", 0, 1, element)); 3580 children.add(new Property("listMode", "code", "How to handle the list mode for this element.", 0, 1, listMode)); 3581 children.add(new Property("variable", "id", "Named context for field, if a field is specified.", 0, 1, variable)); 3582 children.add(new Property("condition", "string", "FHIRPath expression - must be true or the rule does not apply.", 0, 1, condition)); 3583 children.add(new Property("check", "string", "FHIRPath expression - must be true or the mapping engine throws an error instead of completing.", 0, 1, check)); 3584 } 3585 3586 @Override 3587 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3588 switch (_hash) { 3589 case 951530927: /*context*/ return new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context); 3590 case 108114: /*min*/ return new Property("min", "integer", "Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.", 0, 1, min); 3591 case 107876: /*max*/ return new Property("max", "string", "Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).", 0, 1, max); 3592 case 3575610: /*type*/ return new Property("type", "string", "Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.", 0, 1, type); 3593 case 587922128: /*defaultValue[x]*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3594 case -659125328: /*defaultValue*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3595 case 1470297600: /*defaultValueBase64Binary*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3596 case 600437336: /*defaultValueBoolean*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3597 case 264593188: /*defaultValueCanonical*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3598 case 1044993469: /*defaultValueCode*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3599 case 1045010302: /*defaultValueDate*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3600 case 1220374379: /*defaultValueDateTime*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3601 case 2077989249: /*defaultValueDecimal*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3602 case -2059245333: /*defaultValueId*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3603 case -1801671663: /*defaultValueInstant*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3604 case -1801189522: /*defaultValueInteger*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3605 case -325436225: /*defaultValueMarkdown*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3606 case 587910138: /*defaultValueOid*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3607 case -737344154: /*defaultValuePositiveInt*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3608 case -320515103: /*defaultValueString*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3609 case 1045494429: /*defaultValueTime*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3610 case 539117290: /*defaultValueUnsignedInt*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3611 case 587916188: /*defaultValueUri*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3612 case 587916191: /*defaultValueUrl*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3613 case 1045535627: /*defaultValueUuid*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3614 case -611966428: /*defaultValueAddress*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3615 case -1851689217: /*defaultValueAnnotation*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3616 case 2034820339: /*defaultValueAttachment*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3617 case -410434095: /*defaultValueCodeableConcept*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3618 case -783616198: /*defaultValueCoding*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3619 case -344740576: /*defaultValueContactPoint*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3620 case -975393912: /*defaultValueHumanName*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3621 case -1915078535: /*defaultValueIdentifier*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3622 case -420255343: /*defaultValuePeriod*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3623 case -1857379237: /*defaultValueQuantity*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3624 case -1951495315: /*defaultValueRange*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3625 case -1951489477: /*defaultValueRatio*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3626 case -1488914053: /*defaultValueReference*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3627 case -449641228: /*defaultValueSampledData*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3628 case 509825768: /*defaultValueSignature*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3629 case -302193638: /*defaultValueTiming*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3630 case -754548089: /*defaultValueDosage*/ return new Property("defaultValue[x]", "*", "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 3631 case -1662836996: /*element*/ return new Property("element", "string", "Optional field for this source.", 0, 1, element); 3632 case 1345445729: /*listMode*/ return new Property("listMode", "code", "How to handle the list mode for this element.", 0, 1, listMode); 3633 case -1249586564: /*variable*/ return new Property("variable", "id", "Named context for field, if a field is specified.", 0, 1, variable); 3634 case -861311717: /*condition*/ return new Property("condition", "string", "FHIRPath expression - must be true or the rule does not apply.", 0, 1, condition); 3635 case 94627080: /*check*/ return new Property("check", "string", "FHIRPath expression - must be true or the mapping engine throws an error instead of completing.", 0, 1, check); 3636 default: return super.getNamedProperty(_hash, _name, _checkValid); 3637 } 3638 3639 } 3640 3641 @Override 3642 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3643 switch (hash) { 3644 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // IdType 3645 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // IntegerType 3646 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 3647 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // StringType 3648 case -659125328: /*defaultValue*/ return this.defaultValue == null ? new Base[0] : new Base[] {this.defaultValue}; // org.hl7.fhir.dstu3.model.Type 3649 case -1662836996: /*element*/ return this.element == null ? new Base[0] : new Base[] {this.element}; // StringType 3650 case 1345445729: /*listMode*/ return this.listMode == null ? new Base[0] : new Base[] {this.listMode}; // Enumeration<StructureMapSourceListMode> 3651 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : new Base[] {this.variable}; // IdType 3652 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // StringType 3653 case 94627080: /*check*/ return this.check == null ? new Base[0] : new Base[] {this.check}; // StringType 3654 default: return super.getProperty(hash, name, checkValid); 3655 } 3656 3657 } 3658 3659 @Override 3660 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3661 switch (hash) { 3662 case 951530927: // context 3663 this.context = castToId(value); // IdType 3664 return value; 3665 case 108114: // min 3666 this.min = castToInteger(value); // IntegerType 3667 return value; 3668 case 107876: // max 3669 this.max = castToString(value); // StringType 3670 return value; 3671 case 3575610: // type 3672 this.type = castToString(value); // StringType 3673 return value; 3674 case -659125328: // defaultValue 3675 this.defaultValue = castToType(value); // org.hl7.fhir.dstu3.model.Type 3676 return value; 3677 case -1662836996: // element 3678 this.element = castToString(value); // StringType 3679 return value; 3680 case 1345445729: // listMode 3681 value = new StructureMapSourceListModeEnumFactory().fromType(castToCode(value)); 3682 this.listMode = (Enumeration) value; // Enumeration<StructureMapSourceListMode> 3683 return value; 3684 case -1249586564: // variable 3685 this.variable = castToId(value); // IdType 3686 return value; 3687 case -861311717: // condition 3688 this.condition = castToString(value); // StringType 3689 return value; 3690 case 94627080: // check 3691 this.check = castToString(value); // StringType 3692 return value; 3693 default: return super.setProperty(hash, name, value); 3694 } 3695 3696 } 3697 3698 @Override 3699 public Base setProperty(String name, Base value) throws FHIRException { 3700 if (name.equals("context")) { 3701 this.context = castToId(value); // IdType 3702 } else if (name.equals("min")) { 3703 this.min = castToInteger(value); // IntegerType 3704 } else if (name.equals("max")) { 3705 this.max = castToString(value); // StringType 3706 } else if (name.equals("type")) { 3707 this.type = castToString(value); // StringType 3708 } else if (name.equals("defaultValue[x]")) { 3709 this.defaultValue = castToType(value); // org.hl7.fhir.dstu3.model.Type 3710 } else if (name.equals("element")) { 3711 this.element = castToString(value); // StringType 3712 } else if (name.equals("listMode")) { 3713 value = new StructureMapSourceListModeEnumFactory().fromType(castToCode(value)); 3714 this.listMode = (Enumeration) value; // Enumeration<StructureMapSourceListMode> 3715 } else if (name.equals("variable")) { 3716 this.variable = castToId(value); // IdType 3717 } else if (name.equals("condition")) { 3718 this.condition = castToString(value); // StringType 3719 } else if (name.equals("check")) { 3720 this.check = castToString(value); // StringType 3721 } else 3722 return super.setProperty(name, value); 3723 return value; 3724 } 3725 3726 @Override 3727 public Base makeProperty(int hash, String name) throws FHIRException { 3728 switch (hash) { 3729 case 951530927: return getContextElement(); 3730 case 108114: return getMinElement(); 3731 case 107876: return getMaxElement(); 3732 case 3575610: return getTypeElement(); 3733 case 587922128: return getDefaultValue(); 3734 case -659125328: return getDefaultValue(); 3735 case -1662836996: return getElementElement(); 3736 case 1345445729: return getListModeElement(); 3737 case -1249586564: return getVariableElement(); 3738 case -861311717: return getConditionElement(); 3739 case 94627080: return getCheckElement(); 3740 default: return super.makeProperty(hash, name); 3741 } 3742 3743 } 3744 3745 @Override 3746 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3747 switch (hash) { 3748 case 951530927: /*context*/ return new String[] {"id"}; 3749 case 108114: /*min*/ return new String[] {"integer"}; 3750 case 107876: /*max*/ return new String[] {"string"}; 3751 case 3575610: /*type*/ return new String[] {"string"}; 3752 case -659125328: /*defaultValue*/ return new String[] {"*"}; 3753 case -1662836996: /*element*/ return new String[] {"string"}; 3754 case 1345445729: /*listMode*/ return new String[] {"code"}; 3755 case -1249586564: /*variable*/ return new String[] {"id"}; 3756 case -861311717: /*condition*/ return new String[] {"string"}; 3757 case 94627080: /*check*/ return new String[] {"string"}; 3758 default: return super.getTypesForProperty(hash, name); 3759 } 3760 3761 } 3762 3763 @Override 3764 public Base addChild(String name) throws FHIRException { 3765 if (name.equals("context")) { 3766 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.context"); 3767 } 3768 else if (name.equals("min")) { 3769 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.min"); 3770 } 3771 else if (name.equals("max")) { 3772 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.max"); 3773 } 3774 else if (name.equals("type")) { 3775 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.type"); 3776 } 3777 else if (name.equals("defaultValueBoolean")) { 3778 this.defaultValue = new BooleanType(); 3779 return this.defaultValue; 3780 } 3781 else if (name.equals("defaultValueInteger")) { 3782 this.defaultValue = new IntegerType(); 3783 return this.defaultValue; 3784 } 3785 else if (name.equals("defaultValueDecimal")) { 3786 this.defaultValue = new DecimalType(); 3787 return this.defaultValue; 3788 } 3789 else if (name.equals("defaultValueBase64Binary")) { 3790 this.defaultValue = new Base64BinaryType(); 3791 return this.defaultValue; 3792 } 3793 else if (name.equals("defaultValueInstant")) { 3794 this.defaultValue = new InstantType(); 3795 return this.defaultValue; 3796 } 3797 else if (name.equals("defaultValueString")) { 3798 this.defaultValue = new StringType(); 3799 return this.defaultValue; 3800 } 3801 else if (name.equals("defaultValueUri")) { 3802 this.defaultValue = new UriType(); 3803 return this.defaultValue; 3804 } 3805 else if (name.equals("defaultValueDate")) { 3806 this.defaultValue = new DateType(); 3807 return this.defaultValue; 3808 } 3809 else if (name.equals("defaultValueDateTime")) { 3810 this.defaultValue = new DateTimeType(); 3811 return this.defaultValue; 3812 } 3813 else if (name.equals("defaultValueTime")) { 3814 this.defaultValue = new TimeType(); 3815 return this.defaultValue; 3816 } 3817 else if (name.equals("defaultValueCode")) { 3818 this.defaultValue = new CodeType(); 3819 return this.defaultValue; 3820 } 3821 else if (name.equals("defaultValueOid")) { 3822 this.defaultValue = new OidType(); 3823 return this.defaultValue; 3824 } 3825 else if (name.equals("defaultValueId")) { 3826 this.defaultValue = new IdType(); 3827 return this.defaultValue; 3828 } 3829 else if (name.equals("defaultValueUnsignedInt")) { 3830 this.defaultValue = new UnsignedIntType(); 3831 return this.defaultValue; 3832 } 3833 else if (name.equals("defaultValuePositiveInt")) { 3834 this.defaultValue = new PositiveIntType(); 3835 return this.defaultValue; 3836 } 3837 else if (name.equals("defaultValueMarkdown")) { 3838 this.defaultValue = new MarkdownType(); 3839 return this.defaultValue; 3840 } 3841 else if (name.equals("defaultValueAnnotation")) { 3842 this.defaultValue = new Annotation(); 3843 return this.defaultValue; 3844 } 3845 else if (name.equals("defaultValueAttachment")) { 3846 this.defaultValue = new Attachment(); 3847 return this.defaultValue; 3848 } 3849 else if (name.equals("defaultValueIdentifier")) { 3850 this.defaultValue = new Identifier(); 3851 return this.defaultValue; 3852 } 3853 else if (name.equals("defaultValueCodeableConcept")) { 3854 this.defaultValue = new CodeableConcept(); 3855 return this.defaultValue; 3856 } 3857 else if (name.equals("defaultValueCoding")) { 3858 this.defaultValue = new Coding(); 3859 return this.defaultValue; 3860 } 3861 else if (name.equals("defaultValueQuantity")) { 3862 this.defaultValue = new Quantity(); 3863 return this.defaultValue; 3864 } 3865 else if (name.equals("defaultValueRange")) { 3866 this.defaultValue = new Range(); 3867 return this.defaultValue; 3868 } 3869 else if (name.equals("defaultValuePeriod")) { 3870 this.defaultValue = new Period(); 3871 return this.defaultValue; 3872 } 3873 else if (name.equals("defaultValueRatio")) { 3874 this.defaultValue = new Ratio(); 3875 return this.defaultValue; 3876 } 3877 else if (name.equals("defaultValueSampledData")) { 3878 this.defaultValue = new SampledData(); 3879 return this.defaultValue; 3880 } 3881 else if (name.equals("defaultValueSignature")) { 3882 this.defaultValue = new Signature(); 3883 return this.defaultValue; 3884 } 3885 else if (name.equals("defaultValueHumanName")) { 3886 this.defaultValue = new HumanName(); 3887 return this.defaultValue; 3888 } 3889 else if (name.equals("defaultValueAddress")) { 3890 this.defaultValue = new Address(); 3891 return this.defaultValue; 3892 } 3893 else if (name.equals("defaultValueContactPoint")) { 3894 this.defaultValue = new ContactPoint(); 3895 return this.defaultValue; 3896 } 3897 else if (name.equals("defaultValueTiming")) { 3898 this.defaultValue = new Timing(); 3899 return this.defaultValue; 3900 } 3901 else if (name.equals("defaultValueReference")) { 3902 this.defaultValue = new Reference(); 3903 return this.defaultValue; 3904 } 3905 else if (name.equals("defaultValueMeta")) { 3906 this.defaultValue = new Meta(); 3907 return this.defaultValue; 3908 } 3909 else if (name.equals("element")) { 3910 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.element"); 3911 } 3912 else if (name.equals("listMode")) { 3913 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.listMode"); 3914 } 3915 else if (name.equals("variable")) { 3916 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.variable"); 3917 } 3918 else if (name.equals("condition")) { 3919 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.condition"); 3920 } 3921 else if (name.equals("check")) { 3922 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.check"); 3923 } 3924 else 3925 return super.addChild(name); 3926 } 3927 3928 public StructureMapGroupRuleSourceComponent copy() { 3929 StructureMapGroupRuleSourceComponent dst = new StructureMapGroupRuleSourceComponent(); 3930 copyValues(dst); 3931 dst.context = context == null ? null : context.copy(); 3932 dst.min = min == null ? null : min.copy(); 3933 dst.max = max == null ? null : max.copy(); 3934 dst.type = type == null ? null : type.copy(); 3935 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 3936 dst.element = element == null ? null : element.copy(); 3937 dst.listMode = listMode == null ? null : listMode.copy(); 3938 dst.variable = variable == null ? null : variable.copy(); 3939 dst.condition = condition == null ? null : condition.copy(); 3940 dst.check = check == null ? null : check.copy(); 3941 return dst; 3942 } 3943 3944 @Override 3945 public boolean equalsDeep(Base other_) { 3946 if (!super.equalsDeep(other_)) 3947 return false; 3948 if (!(other_ instanceof StructureMapGroupRuleSourceComponent)) 3949 return false; 3950 StructureMapGroupRuleSourceComponent o = (StructureMapGroupRuleSourceComponent) other_; 3951 return compareDeep(context, o.context, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 3952 && compareDeep(type, o.type, true) && compareDeep(defaultValue, o.defaultValue, true) && compareDeep(element, o.element, true) 3953 && compareDeep(listMode, o.listMode, true) && compareDeep(variable, o.variable, true) && compareDeep(condition, o.condition, true) 3954 && compareDeep(check, o.check, true); 3955 } 3956 3957 @Override 3958 public boolean equalsShallow(Base other_) { 3959 if (!super.equalsShallow(other_)) 3960 return false; 3961 if (!(other_ instanceof StructureMapGroupRuleSourceComponent)) 3962 return false; 3963 StructureMapGroupRuleSourceComponent o = (StructureMapGroupRuleSourceComponent) other_; 3964 return compareValues(context, o.context, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 3965 && compareValues(type, o.type, true) && compareValues(element, o.element, true) && compareValues(listMode, o.listMode, true) 3966 && compareValues(variable, o.variable, true) && compareValues(condition, o.condition, true) && compareValues(check, o.check, true) 3967 ; 3968 } 3969 3970 public boolean isEmpty() { 3971 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(context, min, max, type 3972 , defaultValue, element, listMode, variable, condition, check); 3973 } 3974 3975 public String fhirType() { 3976 return "StructureMap.group.rule.source"; 3977 3978 } 3979 3980// added from java-adornments.txt: 3981 3982 public String toString() { 3983 return StructureMapUtilities.sourceToString(this); 3984 } 3985 3986 3987// end addition 3988 } 3989 3990 @Block() 3991 public static class StructureMapGroupRuleTargetComponent extends BackboneElement implements IBaseBackboneElement { 3992 /** 3993 * Type or variable this rule applies to. 3994 */ 3995 @Child(name = "context", type = {IdType.class}, order=1, min=0, max=1, modifier=false, summary=true) 3996 @Description(shortDefinition="Type or variable this rule applies to", formalDefinition="Type or variable this rule applies to." ) 3997 protected IdType context; 3998 3999 /** 4000 * How to interpret the context. 4001 */ 4002 @Child(name = "contextType", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 4003 @Description(shortDefinition="type | variable", formalDefinition="How to interpret the context." ) 4004 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-context-type") 4005 protected Enumeration<StructureMapContextType> contextType; 4006 4007 /** 4008 * Field to create in the context. 4009 */ 4010 @Child(name = "element", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 4011 @Description(shortDefinition="Field to create in the context", formalDefinition="Field to create in the context." ) 4012 protected StringType element; 4013 4014 /** 4015 * Named context for field, if desired, and a field is specified. 4016 */ 4017 @Child(name = "variable", type = {IdType.class}, order=4, min=0, max=1, modifier=false, summary=true) 4018 @Description(shortDefinition="Named context for field, if desired, and a field is specified", formalDefinition="Named context for field, if desired, and a field is specified." ) 4019 protected IdType variable; 4020 4021 /** 4022 * If field is a list, how to manage the list. 4023 */ 4024 @Child(name = "listMode", type = {CodeType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4025 @Description(shortDefinition="first | share | last | collate", formalDefinition="If field is a list, how to manage the list." ) 4026 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-target-list-mode") 4027 protected List<Enumeration<StructureMapTargetListMode>> listMode; 4028 4029 /** 4030 * Internal rule reference for shared list items. 4031 */ 4032 @Child(name = "listRuleId", type = {IdType.class}, order=6, min=0, max=1, modifier=false, summary=true) 4033 @Description(shortDefinition="Internal rule reference for shared list items", formalDefinition="Internal rule reference for shared list items." ) 4034 protected IdType listRuleId; 4035 4036 /** 4037 * How the data is copied / created. 4038 */ 4039 @Child(name = "transform", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 4040 @Description(shortDefinition="create | copy +", formalDefinition="How the data is copied / created." ) 4041 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/map-transform") 4042 protected Enumeration<StructureMapTransform> transform; 4043 4044 /** 4045 * Parameters to the transform. 4046 */ 4047 @Child(name = "parameter", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4048 @Description(shortDefinition="Parameters to the transform", formalDefinition="Parameters to the transform." ) 4049 protected List<StructureMapGroupRuleTargetParameterComponent> parameter; 4050 4051 private static final long serialVersionUID = -1441766429L; 4052 4053 /** 4054 * Constructor 4055 */ 4056 public StructureMapGroupRuleTargetComponent() { 4057 super(); 4058 } 4059 4060 /** 4061 * @return {@link #context} (Type or variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 4062 */ 4063 public IdType getContextElement() { 4064 if (this.context == null) 4065 if (Configuration.errorOnAutoCreate()) 4066 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.context"); 4067 else if (Configuration.doAutoCreate()) 4068 this.context = new IdType(); // bb 4069 return this.context; 4070 } 4071 4072 public boolean hasContextElement() { 4073 return this.context != null && !this.context.isEmpty(); 4074 } 4075 4076 public boolean hasContext() { 4077 return this.context != null && !this.context.isEmpty(); 4078 } 4079 4080 /** 4081 * @param value {@link #context} (Type or variable this rule applies to.). This is the underlying object with id, value and extensions. The accessor "getContext" gives direct access to the value 4082 */ 4083 public StructureMapGroupRuleTargetComponent setContextElement(IdType value) { 4084 this.context = value; 4085 return this; 4086 } 4087 4088 /** 4089 * @return Type or variable this rule applies to. 4090 */ 4091 public String getContext() { 4092 return this.context == null ? null : this.context.getValue(); 4093 } 4094 4095 /** 4096 * @param value Type or variable this rule applies to. 4097 */ 4098 public StructureMapGroupRuleTargetComponent setContext(String value) { 4099 if (Utilities.noString(value)) 4100 this.context = null; 4101 else { 4102 if (this.context == null) 4103 this.context = new IdType(); 4104 this.context.setValue(value); 4105 } 4106 return this; 4107 } 4108 4109 /** 4110 * @return {@link #contextType} (How to interpret the context.). This is the underlying object with id, value and extensions. The accessor "getContextType" gives direct access to the value 4111 */ 4112 public Enumeration<StructureMapContextType> getContextTypeElement() { 4113 if (this.contextType == null) 4114 if (Configuration.errorOnAutoCreate()) 4115 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.contextType"); 4116 else if (Configuration.doAutoCreate()) 4117 this.contextType = new Enumeration<StructureMapContextType>(new StructureMapContextTypeEnumFactory()); // bb 4118 return this.contextType; 4119 } 4120 4121 public boolean hasContextTypeElement() { 4122 return this.contextType != null && !this.contextType.isEmpty(); 4123 } 4124 4125 public boolean hasContextType() { 4126 return this.contextType != null && !this.contextType.isEmpty(); 4127 } 4128 4129 /** 4130 * @param value {@link #contextType} (How to interpret the context.). This is the underlying object with id, value and extensions. The accessor "getContextType" gives direct access to the value 4131 */ 4132 public StructureMapGroupRuleTargetComponent setContextTypeElement(Enumeration<StructureMapContextType> value) { 4133 this.contextType = value; 4134 return this; 4135 } 4136 4137 /** 4138 * @return How to interpret the context. 4139 */ 4140 public StructureMapContextType getContextType() { 4141 return this.contextType == null ? null : this.contextType.getValue(); 4142 } 4143 4144 /** 4145 * @param value How to interpret the context. 4146 */ 4147 public StructureMapGroupRuleTargetComponent setContextType(StructureMapContextType value) { 4148 if (value == null) 4149 this.contextType = null; 4150 else { 4151 if (this.contextType == null) 4152 this.contextType = new Enumeration<StructureMapContextType>(new StructureMapContextTypeEnumFactory()); 4153 this.contextType.setValue(value); 4154 } 4155 return this; 4156 } 4157 4158 /** 4159 * @return {@link #element} (Field to create in the context.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 4160 */ 4161 public StringType getElementElement() { 4162 if (this.element == null) 4163 if (Configuration.errorOnAutoCreate()) 4164 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.element"); 4165 else if (Configuration.doAutoCreate()) 4166 this.element = new StringType(); // bb 4167 return this.element; 4168 } 4169 4170 public boolean hasElementElement() { 4171 return this.element != null && !this.element.isEmpty(); 4172 } 4173 4174 public boolean hasElement() { 4175 return this.element != null && !this.element.isEmpty(); 4176 } 4177 4178 /** 4179 * @param value {@link #element} (Field to create in the context.). This is the underlying object with id, value and extensions. The accessor "getElement" gives direct access to the value 4180 */ 4181 public StructureMapGroupRuleTargetComponent setElementElement(StringType value) { 4182 this.element = value; 4183 return this; 4184 } 4185 4186 /** 4187 * @return Field to create in the context. 4188 */ 4189 public String getElement() { 4190 return this.element == null ? null : this.element.getValue(); 4191 } 4192 4193 /** 4194 * @param value Field to create in the context. 4195 */ 4196 public StructureMapGroupRuleTargetComponent setElement(String value) { 4197 if (Utilities.noString(value)) 4198 this.element = null; 4199 else { 4200 if (this.element == null) 4201 this.element = new StringType(); 4202 this.element.setValue(value); 4203 } 4204 return this; 4205 } 4206 4207 /** 4208 * @return {@link #variable} (Named context for field, if desired, and a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 4209 */ 4210 public IdType getVariableElement() { 4211 if (this.variable == null) 4212 if (Configuration.errorOnAutoCreate()) 4213 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.variable"); 4214 else if (Configuration.doAutoCreate()) 4215 this.variable = new IdType(); // bb 4216 return this.variable; 4217 } 4218 4219 public boolean hasVariableElement() { 4220 return this.variable != null && !this.variable.isEmpty(); 4221 } 4222 4223 public boolean hasVariable() { 4224 return this.variable != null && !this.variable.isEmpty(); 4225 } 4226 4227 /** 4228 * @param value {@link #variable} (Named context for field, if desired, and a field is specified.). This is the underlying object with id, value and extensions. The accessor "getVariable" gives direct access to the value 4229 */ 4230 public StructureMapGroupRuleTargetComponent setVariableElement(IdType value) { 4231 this.variable = value; 4232 return this; 4233 } 4234 4235 /** 4236 * @return Named context for field, if desired, and a field is specified. 4237 */ 4238 public String getVariable() { 4239 return this.variable == null ? null : this.variable.getValue(); 4240 } 4241 4242 /** 4243 * @param value Named context for field, if desired, and a field is specified. 4244 */ 4245 public StructureMapGroupRuleTargetComponent setVariable(String value) { 4246 if (Utilities.noString(value)) 4247 this.variable = null; 4248 else { 4249 if (this.variable == null) 4250 this.variable = new IdType(); 4251 this.variable.setValue(value); 4252 } 4253 return this; 4254 } 4255 4256 /** 4257 * @return {@link #listMode} (If field is a list, how to manage the list.) 4258 */ 4259 public List<Enumeration<StructureMapTargetListMode>> getListMode() { 4260 if (this.listMode == null) 4261 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4262 return this.listMode; 4263 } 4264 4265 /** 4266 * @return Returns a reference to <code>this</code> for easy method chaining 4267 */ 4268 public StructureMapGroupRuleTargetComponent setListMode(List<Enumeration<StructureMapTargetListMode>> theListMode) { 4269 this.listMode = theListMode; 4270 return this; 4271 } 4272 4273 public boolean hasListMode() { 4274 if (this.listMode == null) 4275 return false; 4276 for (Enumeration<StructureMapTargetListMode> item : this.listMode) 4277 if (!item.isEmpty()) 4278 return true; 4279 return false; 4280 } 4281 4282 /** 4283 * @return {@link #listMode} (If field is a list, how to manage the list.) 4284 */ 4285 public Enumeration<StructureMapTargetListMode> addListModeElement() {//2 4286 Enumeration<StructureMapTargetListMode> t = new Enumeration<StructureMapTargetListMode>(new StructureMapTargetListModeEnumFactory()); 4287 if (this.listMode == null) 4288 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4289 this.listMode.add(t); 4290 return t; 4291 } 4292 4293 /** 4294 * @param value {@link #listMode} (If field is a list, how to manage the list.) 4295 */ 4296 public StructureMapGroupRuleTargetComponent addListMode(StructureMapTargetListMode value) { //1 4297 Enumeration<StructureMapTargetListMode> t = new Enumeration<StructureMapTargetListMode>(new StructureMapTargetListModeEnumFactory()); 4298 t.setValue(value); 4299 if (this.listMode == null) 4300 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4301 this.listMode.add(t); 4302 return this; 4303 } 4304 4305 /** 4306 * @param value {@link #listMode} (If field is a list, how to manage the list.) 4307 */ 4308 public boolean hasListMode(StructureMapTargetListMode value) { 4309 if (this.listMode == null) 4310 return false; 4311 for (Enumeration<StructureMapTargetListMode> v : this.listMode) 4312 if (v.getValue().equals(value)) // code 4313 return true; 4314 return false; 4315 } 4316 4317 /** 4318 * @return {@link #listRuleId} (Internal rule reference for shared list items.). This is the underlying object with id, value and extensions. The accessor "getListRuleId" gives direct access to the value 4319 */ 4320 public IdType getListRuleIdElement() { 4321 if (this.listRuleId == null) 4322 if (Configuration.errorOnAutoCreate()) 4323 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.listRuleId"); 4324 else if (Configuration.doAutoCreate()) 4325 this.listRuleId = new IdType(); // bb 4326 return this.listRuleId; 4327 } 4328 4329 public boolean hasListRuleIdElement() { 4330 return this.listRuleId != null && !this.listRuleId.isEmpty(); 4331 } 4332 4333 public boolean hasListRuleId() { 4334 return this.listRuleId != null && !this.listRuleId.isEmpty(); 4335 } 4336 4337 /** 4338 * @param value {@link #listRuleId} (Internal rule reference for shared list items.). This is the underlying object with id, value and extensions. The accessor "getListRuleId" gives direct access to the value 4339 */ 4340 public StructureMapGroupRuleTargetComponent setListRuleIdElement(IdType value) { 4341 this.listRuleId = value; 4342 return this; 4343 } 4344 4345 /** 4346 * @return Internal rule reference for shared list items. 4347 */ 4348 public String getListRuleId() { 4349 return this.listRuleId == null ? null : this.listRuleId.getValue(); 4350 } 4351 4352 /** 4353 * @param value Internal rule reference for shared list items. 4354 */ 4355 public StructureMapGroupRuleTargetComponent setListRuleId(String value) { 4356 if (Utilities.noString(value)) 4357 this.listRuleId = null; 4358 else { 4359 if (this.listRuleId == null) 4360 this.listRuleId = new IdType(); 4361 this.listRuleId.setValue(value); 4362 } 4363 return this; 4364 } 4365 4366 /** 4367 * @return {@link #transform} (How the data is copied / created.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 4368 */ 4369 public Enumeration<StructureMapTransform> getTransformElement() { 4370 if (this.transform == null) 4371 if (Configuration.errorOnAutoCreate()) 4372 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.transform"); 4373 else if (Configuration.doAutoCreate()) 4374 this.transform = new Enumeration<StructureMapTransform>(new StructureMapTransformEnumFactory()); // bb 4375 return this.transform; 4376 } 4377 4378 public boolean hasTransformElement() { 4379 return this.transform != null && !this.transform.isEmpty(); 4380 } 4381 4382 public boolean hasTransform() { 4383 return this.transform != null && !this.transform.isEmpty(); 4384 } 4385 4386 /** 4387 * @param value {@link #transform} (How the data is copied / created.). This is the underlying object with id, value and extensions. The accessor "getTransform" gives direct access to the value 4388 */ 4389 public StructureMapGroupRuleTargetComponent setTransformElement(Enumeration<StructureMapTransform> value) { 4390 this.transform = value; 4391 return this; 4392 } 4393 4394 /** 4395 * @return How the data is copied / created. 4396 */ 4397 public StructureMapTransform getTransform() { 4398 return this.transform == null ? null : this.transform.getValue(); 4399 } 4400 4401 /** 4402 * @param value How the data is copied / created. 4403 */ 4404 public StructureMapGroupRuleTargetComponent setTransform(StructureMapTransform value) { 4405 if (value == null) 4406 this.transform = null; 4407 else { 4408 if (this.transform == null) 4409 this.transform = new Enumeration<StructureMapTransform>(new StructureMapTransformEnumFactory()); 4410 this.transform.setValue(value); 4411 } 4412 return this; 4413 } 4414 4415 /** 4416 * @return {@link #parameter} (Parameters to the transform.) 4417 */ 4418 public List<StructureMapGroupRuleTargetParameterComponent> getParameter() { 4419 if (this.parameter == null) 4420 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4421 return this.parameter; 4422 } 4423 4424 /** 4425 * @return Returns a reference to <code>this</code> for easy method chaining 4426 */ 4427 public StructureMapGroupRuleTargetComponent setParameter(List<StructureMapGroupRuleTargetParameterComponent> theParameter) { 4428 this.parameter = theParameter; 4429 return this; 4430 } 4431 4432 public boolean hasParameter() { 4433 if (this.parameter == null) 4434 return false; 4435 for (StructureMapGroupRuleTargetParameterComponent item : this.parameter) 4436 if (!item.isEmpty()) 4437 return true; 4438 return false; 4439 } 4440 4441 public StructureMapGroupRuleTargetParameterComponent addParameter() { //3 4442 StructureMapGroupRuleTargetParameterComponent t = new StructureMapGroupRuleTargetParameterComponent(); 4443 if (this.parameter == null) 4444 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4445 this.parameter.add(t); 4446 return t; 4447 } 4448 4449 public StructureMapGroupRuleTargetComponent addParameter(StructureMapGroupRuleTargetParameterComponent t) { //3 4450 if (t == null) 4451 return this; 4452 if (this.parameter == null) 4453 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4454 this.parameter.add(t); 4455 return this; 4456 } 4457 4458 /** 4459 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist 4460 */ 4461 public StructureMapGroupRuleTargetParameterComponent getParameterFirstRep() { 4462 if (getParameter().isEmpty()) { 4463 addParameter(); 4464 } 4465 return getParameter().get(0); 4466 } 4467 4468 protected void listChildren(List<Property> children) { 4469 super.listChildren(children); 4470 children.add(new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context)); 4471 children.add(new Property("contextType", "code", "How to interpret the context.", 0, 1, contextType)); 4472 children.add(new Property("element", "string", "Field to create in the context.", 0, 1, element)); 4473 children.add(new Property("variable", "id", "Named context for field, if desired, and a field is specified.", 0, 1, variable)); 4474 children.add(new Property("listMode", "code", "If field is a list, how to manage the list.", 0, java.lang.Integer.MAX_VALUE, listMode)); 4475 children.add(new Property("listRuleId", "id", "Internal rule reference for shared list items.", 0, 1, listRuleId)); 4476 children.add(new Property("transform", "code", "How the data is copied / created.", 0, 1, transform)); 4477 children.add(new Property("parameter", "", "Parameters to the transform.", 0, java.lang.Integer.MAX_VALUE, parameter)); 4478 } 4479 4480 @Override 4481 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4482 switch (_hash) { 4483 case 951530927: /*context*/ return new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context); 4484 case -102839927: /*contextType*/ return new Property("contextType", "code", "How to interpret the context.", 0, 1, contextType); 4485 case -1662836996: /*element*/ return new Property("element", "string", "Field to create in the context.", 0, 1, element); 4486 case -1249586564: /*variable*/ return new Property("variable", "id", "Named context for field, if desired, and a field is specified.", 0, 1, variable); 4487 case 1345445729: /*listMode*/ return new Property("listMode", "code", "If field is a list, how to manage the list.", 0, java.lang.Integer.MAX_VALUE, listMode); 4488 case 337117045: /*listRuleId*/ return new Property("listRuleId", "id", "Internal rule reference for shared list items.", 0, 1, listRuleId); 4489 case 1052666732: /*transform*/ return new Property("transform", "code", "How the data is copied / created.", 0, 1, transform); 4490 case 1954460585: /*parameter*/ return new Property("parameter", "", "Parameters to the transform.", 0, java.lang.Integer.MAX_VALUE, parameter); 4491 default: return super.getNamedProperty(_hash, _name, _checkValid); 4492 } 4493 4494 } 4495 4496 @Override 4497 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4498 switch (hash) { 4499 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // IdType 4500 case -102839927: /*contextType*/ return this.contextType == null ? new Base[0] : new Base[] {this.contextType}; // Enumeration<StructureMapContextType> 4501 case -1662836996: /*element*/ return this.element == null ? new Base[0] : new Base[] {this.element}; // StringType 4502 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : new Base[] {this.variable}; // IdType 4503 case 1345445729: /*listMode*/ return this.listMode == null ? new Base[0] : this.listMode.toArray(new Base[this.listMode.size()]); // Enumeration<StructureMapTargetListMode> 4504 case 337117045: /*listRuleId*/ return this.listRuleId == null ? new Base[0] : new Base[] {this.listRuleId}; // IdType 4505 case 1052666732: /*transform*/ return this.transform == null ? new Base[0] : new Base[] {this.transform}; // Enumeration<StructureMapTransform> 4506 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // StructureMapGroupRuleTargetParameterComponent 4507 default: return super.getProperty(hash, name, checkValid); 4508 } 4509 4510 } 4511 4512 @Override 4513 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4514 switch (hash) { 4515 case 951530927: // context 4516 this.context = castToId(value); // IdType 4517 return value; 4518 case -102839927: // contextType 4519 value = new StructureMapContextTypeEnumFactory().fromType(castToCode(value)); 4520 this.contextType = (Enumeration) value; // Enumeration<StructureMapContextType> 4521 return value; 4522 case -1662836996: // element 4523 this.element = castToString(value); // StringType 4524 return value; 4525 case -1249586564: // variable 4526 this.variable = castToId(value); // IdType 4527 return value; 4528 case 1345445729: // listMode 4529 value = new StructureMapTargetListModeEnumFactory().fromType(castToCode(value)); 4530 this.getListMode().add((Enumeration) value); // Enumeration<StructureMapTargetListMode> 4531 return value; 4532 case 337117045: // listRuleId 4533 this.listRuleId = castToId(value); // IdType 4534 return value; 4535 case 1052666732: // transform 4536 value = new StructureMapTransformEnumFactory().fromType(castToCode(value)); 4537 this.transform = (Enumeration) value; // Enumeration<StructureMapTransform> 4538 return value; 4539 case 1954460585: // parameter 4540 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); // StructureMapGroupRuleTargetParameterComponent 4541 return value; 4542 default: return super.setProperty(hash, name, value); 4543 } 4544 4545 } 4546 4547 @Override 4548 public Base setProperty(String name, Base value) throws FHIRException { 4549 if (name.equals("context")) { 4550 this.context = castToId(value); // IdType 4551 } else if (name.equals("contextType")) { 4552 value = new StructureMapContextTypeEnumFactory().fromType(castToCode(value)); 4553 this.contextType = (Enumeration) value; // Enumeration<StructureMapContextType> 4554 } else if (name.equals("element")) { 4555 this.element = castToString(value); // StringType 4556 } else if (name.equals("variable")) { 4557 this.variable = castToId(value); // IdType 4558 } else if (name.equals("listMode")) { 4559 value = new StructureMapTargetListModeEnumFactory().fromType(castToCode(value)); 4560 this.getListMode().add((Enumeration) value); 4561 } else if (name.equals("listRuleId")) { 4562 this.listRuleId = castToId(value); // IdType 4563 } else if (name.equals("transform")) { 4564 value = new StructureMapTransformEnumFactory().fromType(castToCode(value)); 4565 this.transform = (Enumeration) value; // Enumeration<StructureMapTransform> 4566 } else if (name.equals("parameter")) { 4567 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); 4568 } else 4569 return super.setProperty(name, value); 4570 return value; 4571 } 4572 4573 @Override 4574 public Base makeProperty(int hash, String name) throws FHIRException { 4575 switch (hash) { 4576 case 951530927: return getContextElement(); 4577 case -102839927: return getContextTypeElement(); 4578 case -1662836996: return getElementElement(); 4579 case -1249586564: return getVariableElement(); 4580 case 1345445729: return addListModeElement(); 4581 case 337117045: return getListRuleIdElement(); 4582 case 1052666732: return getTransformElement(); 4583 case 1954460585: return addParameter(); 4584 default: return super.makeProperty(hash, name); 4585 } 4586 4587 } 4588 4589 @Override 4590 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4591 switch (hash) { 4592 case 951530927: /*context*/ return new String[] {"id"}; 4593 case -102839927: /*contextType*/ return new String[] {"code"}; 4594 case -1662836996: /*element*/ return new String[] {"string"}; 4595 case -1249586564: /*variable*/ return new String[] {"id"}; 4596 case 1345445729: /*listMode*/ return new String[] {"code"}; 4597 case 337117045: /*listRuleId*/ return new String[] {"id"}; 4598 case 1052666732: /*transform*/ return new String[] {"code"}; 4599 case 1954460585: /*parameter*/ return new String[] {}; 4600 default: return super.getTypesForProperty(hash, name); 4601 } 4602 4603 } 4604 4605 @Override 4606 public Base addChild(String name) throws FHIRException { 4607 if (name.equals("context")) { 4608 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.context"); 4609 } 4610 else if (name.equals("contextType")) { 4611 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.contextType"); 4612 } 4613 else if (name.equals("element")) { 4614 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.element"); 4615 } 4616 else if (name.equals("variable")) { 4617 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.variable"); 4618 } 4619 else if (name.equals("listMode")) { 4620 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.listMode"); 4621 } 4622 else if (name.equals("listRuleId")) { 4623 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.listRuleId"); 4624 } 4625 else if (name.equals("transform")) { 4626 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.transform"); 4627 } 4628 else if (name.equals("parameter")) { 4629 return addParameter(); 4630 } 4631 else 4632 return super.addChild(name); 4633 } 4634 4635 public StructureMapGroupRuleTargetComponent copy() { 4636 StructureMapGroupRuleTargetComponent dst = new StructureMapGroupRuleTargetComponent(); 4637 copyValues(dst); 4638 dst.context = context == null ? null : context.copy(); 4639 dst.contextType = contextType == null ? null : contextType.copy(); 4640 dst.element = element == null ? null : element.copy(); 4641 dst.variable = variable == null ? null : variable.copy(); 4642 if (listMode != null) { 4643 dst.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 4644 for (Enumeration<StructureMapTargetListMode> i : listMode) 4645 dst.listMode.add(i.copy()); 4646 }; 4647 dst.listRuleId = listRuleId == null ? null : listRuleId.copy(); 4648 dst.transform = transform == null ? null : transform.copy(); 4649 if (parameter != null) { 4650 dst.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 4651 for (StructureMapGroupRuleTargetParameterComponent i : parameter) 4652 dst.parameter.add(i.copy()); 4653 }; 4654 return dst; 4655 } 4656 4657 @Override 4658 public boolean equalsDeep(Base other_) { 4659 if (!super.equalsDeep(other_)) 4660 return false; 4661 if (!(other_ instanceof StructureMapGroupRuleTargetComponent)) 4662 return false; 4663 StructureMapGroupRuleTargetComponent o = (StructureMapGroupRuleTargetComponent) other_; 4664 return compareDeep(context, o.context, true) && compareDeep(contextType, o.contextType, true) && compareDeep(element, o.element, true) 4665 && compareDeep(variable, o.variable, true) && compareDeep(listMode, o.listMode, true) && compareDeep(listRuleId, o.listRuleId, true) 4666 && compareDeep(transform, o.transform, true) && compareDeep(parameter, o.parameter, true); 4667 } 4668 4669 @Override 4670 public boolean equalsShallow(Base other_) { 4671 if (!super.equalsShallow(other_)) 4672 return false; 4673 if (!(other_ instanceof StructureMapGroupRuleTargetComponent)) 4674 return false; 4675 StructureMapGroupRuleTargetComponent o = (StructureMapGroupRuleTargetComponent) other_; 4676 return compareValues(context, o.context, true) && compareValues(contextType, o.contextType, true) && compareValues(element, o.element, true) 4677 && compareValues(variable, o.variable, true) && compareValues(listMode, o.listMode, true) && compareValues(listRuleId, o.listRuleId, true) 4678 && compareValues(transform, o.transform, true); 4679 } 4680 4681 public boolean isEmpty() { 4682 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(context, contextType, element 4683 , variable, listMode, listRuleId, transform, parameter); 4684 } 4685 4686 public String fhirType() { 4687 return "StructureMap.group.rule.target"; 4688 4689 } 4690 4691// added from java-adornments.txt: 4692 4693 public String toString() { 4694 return StructureMapUtilities.targetToString(this); 4695 } 4696 4697 4698// end addition 4699 } 4700 4701 @Block() 4702 public static class StructureMapGroupRuleTargetParameterComponent extends BackboneElement implements IBaseBackboneElement { 4703 /** 4704 * Parameter value - variable or literal. 4705 */ 4706 @Child(name = "value", type = {IdType.class, StringType.class, BooleanType.class, IntegerType.class, DecimalType.class}, order=1, min=1, max=1, modifier=false, summary=true) 4707 @Description(shortDefinition="Parameter value - variable or literal", formalDefinition="Parameter value - variable or literal." ) 4708 protected Type value; 4709 4710 private static final long serialVersionUID = -732981989L; 4711 4712 /** 4713 * Constructor 4714 */ 4715 public StructureMapGroupRuleTargetParameterComponent() { 4716 super(); 4717 } 4718 4719 /** 4720 * Constructor 4721 */ 4722 public StructureMapGroupRuleTargetParameterComponent(Type value) { 4723 super(); 4724 this.value = value; 4725 } 4726 4727 /** 4728 * @return {@link #value} (Parameter value - variable or literal.) 4729 */ 4730 public Type getValue() { 4731 return this.value; 4732 } 4733 4734 /** 4735 * @return {@link #value} (Parameter value - variable or literal.) 4736 */ 4737 public IdType getValueIdType() throws FHIRException { 4738 if (this.value == null) 4739 return null; 4740 if (!(this.value instanceof IdType)) 4741 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 4742 return (IdType) this.value; 4743 } 4744 4745 public boolean hasValueIdType() { 4746 return this != null && this.value instanceof IdType; 4747 } 4748 4749 /** 4750 * @return {@link #value} (Parameter value - variable or literal.) 4751 */ 4752 public StringType getValueStringType() throws FHIRException { 4753 if (this.value == null) 4754 return null; 4755 if (!(this.value instanceof StringType)) 4756 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 4757 return (StringType) this.value; 4758 } 4759 4760 public boolean hasValueStringType() { 4761 return this != null && this.value instanceof StringType; 4762 } 4763 4764 /** 4765 * @return {@link #value} (Parameter value - variable or literal.) 4766 */ 4767 public BooleanType getValueBooleanType() throws FHIRException { 4768 if (this.value == null) 4769 return null; 4770 if (!(this.value instanceof BooleanType)) 4771 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 4772 return (BooleanType) this.value; 4773 } 4774 4775 public boolean hasValueBooleanType() { 4776 return this != null && this.value instanceof BooleanType; 4777 } 4778 4779 /** 4780 * @return {@link #value} (Parameter value - variable or literal.) 4781 */ 4782 public IntegerType getValueIntegerType() throws FHIRException { 4783 if (this.value == null) 4784 return null; 4785 if (!(this.value instanceof IntegerType)) 4786 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 4787 return (IntegerType) this.value; 4788 } 4789 4790 public boolean hasValueIntegerType() { 4791 return this != null && this.value instanceof IntegerType; 4792 } 4793 4794 /** 4795 * @return {@link #value} (Parameter value - variable or literal.) 4796 */ 4797 public DecimalType getValueDecimalType() throws FHIRException { 4798 if (this.value == null) 4799 return null; 4800 if (!(this.value instanceof DecimalType)) 4801 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 4802 return (DecimalType) this.value; 4803 } 4804 4805 public boolean hasValueDecimalType() { 4806 return this != null && this.value instanceof DecimalType; 4807 } 4808 4809 public boolean hasValue() { 4810 return this.value != null && !this.value.isEmpty(); 4811 } 4812 4813 /** 4814 * @param value {@link #value} (Parameter value - variable or literal.) 4815 */ 4816 public StructureMapGroupRuleTargetParameterComponent setValue(Type value) throws FHIRFormatError { 4817 if (value != null && !(value instanceof IdType || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof DecimalType)) 4818 throw new FHIRFormatError("Not the right type for StructureMap.group.rule.target.parameter.value[x]: "+value.fhirType()); 4819 this.value = value; 4820 return this; 4821 } 4822 4823 protected void listChildren(List<Property> children) { 4824 super.listChildren(children); 4825 children.add(new Property("value[x]", "id|string|boolean|integer|decimal", "Parameter value - variable or literal.", 0, 1, value)); 4826 } 4827 4828 @Override 4829 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4830 switch (_hash) { 4831 case -1410166417: /*value[x]*/ return new Property("value[x]", "id|string|boolean|integer|decimal", "Parameter value - variable or literal.", 0, 1, value); 4832 case 111972721: /*value*/ return new Property("value[x]", "id|string|boolean|integer|decimal", "Parameter value - variable or literal.", 0, 1, value); 4833 case 231604844: /*valueId*/ return new Property("value[x]", "id|string|boolean|integer|decimal", "Parameter value - variable or literal.", 0, 1, value); 4834 case -1424603934: /*valueString*/ return new Property("value[x]", "id|string|boolean|integer|decimal", "Parameter value - variable or literal.", 0, 1, value); 4835 case 733421943: /*valueBoolean*/ return new Property("value[x]", "id|string|boolean|integer|decimal", "Parameter value - variable or literal.", 0, 1, value); 4836 case -1668204915: /*valueInteger*/ return new Property("value[x]", "id|string|boolean|integer|decimal", "Parameter value - variable or literal.", 0, 1, value); 4837 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "id|string|boolean|integer|decimal", "Parameter value - variable or literal.", 0, 1, value); 4838 default: return super.getNamedProperty(_hash, _name, _checkValid); 4839 } 4840 4841 } 4842 4843 @Override 4844 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4845 switch (hash) { 4846 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 4847 default: return super.getProperty(hash, name, checkValid); 4848 } 4849 4850 } 4851 4852 @Override 4853 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4854 switch (hash) { 4855 case 111972721: // value 4856 this.value = castToType(value); // Type 4857 return value; 4858 default: return super.setProperty(hash, name, value); 4859 } 4860 4861 } 4862 4863 @Override 4864 public Base setProperty(String name, Base value) throws FHIRException { 4865 if (name.equals("value[x]")) { 4866 this.value = castToType(value); // Type 4867 } else 4868 return super.setProperty(name, value); 4869 return value; 4870 } 4871 4872 @Override 4873 public Base makeProperty(int hash, String name) throws FHIRException { 4874 switch (hash) { 4875 case -1410166417: return getValue(); 4876 case 111972721: return getValue(); 4877 default: return super.makeProperty(hash, name); 4878 } 4879 4880 } 4881 4882 @Override 4883 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4884 switch (hash) { 4885 case 111972721: /*value*/ return new String[] {"id", "string", "boolean", "integer", "decimal"}; 4886 default: return super.getTypesForProperty(hash, name); 4887 } 4888 4889 } 4890 4891 @Override 4892 public Base addChild(String name) throws FHIRException { 4893 if (name.equals("valueId")) { 4894 this.value = new IdType(); 4895 return this.value; 4896 } 4897 else if (name.equals("valueString")) { 4898 this.value = new StringType(); 4899 return this.value; 4900 } 4901 else if (name.equals("valueBoolean")) { 4902 this.value = new BooleanType(); 4903 return this.value; 4904 } 4905 else if (name.equals("valueInteger")) { 4906 this.value = new IntegerType(); 4907 return this.value; 4908 } 4909 else if (name.equals("valueDecimal")) { 4910 this.value = new DecimalType(); 4911 return this.value; 4912 } 4913 else 4914 return super.addChild(name); 4915 } 4916 4917 public StructureMapGroupRuleTargetParameterComponent copy() { 4918 StructureMapGroupRuleTargetParameterComponent dst = new StructureMapGroupRuleTargetParameterComponent(); 4919 copyValues(dst); 4920 dst.value = value == null ? null : value.copy(); 4921 return dst; 4922 } 4923 4924 @Override 4925 public boolean equalsDeep(Base other_) { 4926 if (!super.equalsDeep(other_)) 4927 return false; 4928 if (!(other_ instanceof StructureMapGroupRuleTargetParameterComponent)) 4929 return false; 4930 StructureMapGroupRuleTargetParameterComponent o = (StructureMapGroupRuleTargetParameterComponent) other_; 4931 return compareDeep(value, o.value, true); 4932 } 4933 4934 @Override 4935 public boolean equalsShallow(Base other_) { 4936 if (!super.equalsShallow(other_)) 4937 return false; 4938 if (!(other_ instanceof StructureMapGroupRuleTargetParameterComponent)) 4939 return false; 4940 StructureMapGroupRuleTargetParameterComponent o = (StructureMapGroupRuleTargetParameterComponent) other_; 4941 return true; 4942 } 4943 4944 public boolean isEmpty() { 4945 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 4946 } 4947 4948 public String fhirType() { 4949 return "StructureMap.group.rule.target.parameter"; 4950 4951 } 4952 4953// added from java-adornments.txt: 4954 4955 public String toString() { 4956 return value == null ? "null!" : value.toString(); 4957 } 4958 4959 4960 4961// end addition 4962 } 4963 4964 @Block() 4965 public static class StructureMapGroupRuleDependentComponent extends BackboneElement implements IBaseBackboneElement { 4966 /** 4967 * Name of a rule or group to apply. 4968 */ 4969 @Child(name = "name", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 4970 @Description(shortDefinition="Name of a rule or group to apply", formalDefinition="Name of a rule or group to apply." ) 4971 protected IdType name; 4972 4973 /** 4974 * Variable to pass to the rule or group. 4975 */ 4976 @Child(name = "variable", type = {StringType.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4977 @Description(shortDefinition="Variable to pass to the rule or group", formalDefinition="Variable to pass to the rule or group." ) 4978 protected List<StringType> variable; 4979 4980 private static final long serialVersionUID = 1021661591L; 4981 4982 /** 4983 * Constructor 4984 */ 4985 public StructureMapGroupRuleDependentComponent() { 4986 super(); 4987 } 4988 4989 /** 4990 * Constructor 4991 */ 4992 public StructureMapGroupRuleDependentComponent(IdType name) { 4993 super(); 4994 this.name = name; 4995 } 4996 4997 /** 4998 * @return {@link #name} (Name of a rule or group to apply.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4999 */ 5000 public IdType getNameElement() { 5001 if (this.name == null) 5002 if (Configuration.errorOnAutoCreate()) 5003 throw new Error("Attempt to auto-create StructureMapGroupRuleDependentComponent.name"); 5004 else if (Configuration.doAutoCreate()) 5005 this.name = new IdType(); // bb 5006 return this.name; 5007 } 5008 5009 public boolean hasNameElement() { 5010 return this.name != null && !this.name.isEmpty(); 5011 } 5012 5013 public boolean hasName() { 5014 return this.name != null && !this.name.isEmpty(); 5015 } 5016 5017 /** 5018 * @param value {@link #name} (Name of a rule or group to apply.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5019 */ 5020 public StructureMapGroupRuleDependentComponent setNameElement(IdType value) { 5021 this.name = value; 5022 return this; 5023 } 5024 5025 /** 5026 * @return Name of a rule or group to apply. 5027 */ 5028 public String getName() { 5029 return this.name == null ? null : this.name.getValue(); 5030 } 5031 5032 /** 5033 * @param value Name of a rule or group to apply. 5034 */ 5035 public StructureMapGroupRuleDependentComponent setName(String value) { 5036 if (this.name == null) 5037 this.name = new IdType(); 5038 this.name.setValue(value); 5039 return this; 5040 } 5041 5042 /** 5043 * @return {@link #variable} (Variable to pass to the rule or group.) 5044 */ 5045 public List<StringType> getVariable() { 5046 if (this.variable == null) 5047 this.variable = new ArrayList<StringType>(); 5048 return this.variable; 5049 } 5050 5051 /** 5052 * @return Returns a reference to <code>this</code> for easy method chaining 5053 */ 5054 public StructureMapGroupRuleDependentComponent setVariable(List<StringType> theVariable) { 5055 this.variable = theVariable; 5056 return this; 5057 } 5058 5059 public boolean hasVariable() { 5060 if (this.variable == null) 5061 return false; 5062 for (StringType item : this.variable) 5063 if (!item.isEmpty()) 5064 return true; 5065 return false; 5066 } 5067 5068 /** 5069 * @return {@link #variable} (Variable to pass to the rule or group.) 5070 */ 5071 public StringType addVariableElement() {//2 5072 StringType t = new StringType(); 5073 if (this.variable == null) 5074 this.variable = new ArrayList<StringType>(); 5075 this.variable.add(t); 5076 return t; 5077 } 5078 5079 /** 5080 * @param value {@link #variable} (Variable to pass to the rule or group.) 5081 */ 5082 public StructureMapGroupRuleDependentComponent addVariable(String value) { //1 5083 StringType t = new StringType(); 5084 t.setValue(value); 5085 if (this.variable == null) 5086 this.variable = new ArrayList<StringType>(); 5087 this.variable.add(t); 5088 return this; 5089 } 5090 5091 /** 5092 * @param value {@link #variable} (Variable to pass to the rule or group.) 5093 */ 5094 public boolean hasVariable(String value) { 5095 if (this.variable == null) 5096 return false; 5097 for (StringType v : this.variable) 5098 if (v.getValue().equals(value)) // string 5099 return true; 5100 return false; 5101 } 5102 5103 protected void listChildren(List<Property> children) { 5104 super.listChildren(children); 5105 children.add(new Property("name", "id", "Name of a rule or group to apply.", 0, 1, name)); 5106 children.add(new Property("variable", "string", "Variable to pass to the rule or group.", 0, java.lang.Integer.MAX_VALUE, variable)); 5107 } 5108 5109 @Override 5110 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5111 switch (_hash) { 5112 case 3373707: /*name*/ return new Property("name", "id", "Name of a rule or group to apply.", 0, 1, name); 5113 case -1249586564: /*variable*/ return new Property("variable", "string", "Variable to pass to the rule or group.", 0, java.lang.Integer.MAX_VALUE, variable); 5114 default: return super.getNamedProperty(_hash, _name, _checkValid); 5115 } 5116 5117 } 5118 5119 @Override 5120 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5121 switch (hash) { 5122 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // IdType 5123 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : this.variable.toArray(new Base[this.variable.size()]); // StringType 5124 default: return super.getProperty(hash, name, checkValid); 5125 } 5126 5127 } 5128 5129 @Override 5130 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5131 switch (hash) { 5132 case 3373707: // name 5133 this.name = castToId(value); // IdType 5134 return value; 5135 case -1249586564: // variable 5136 this.getVariable().add(castToString(value)); // StringType 5137 return value; 5138 default: return super.setProperty(hash, name, value); 5139 } 5140 5141 } 5142 5143 @Override 5144 public Base setProperty(String name, Base value) throws FHIRException { 5145 if (name.equals("name")) { 5146 this.name = castToId(value); // IdType 5147 } else if (name.equals("variable")) { 5148 this.getVariable().add(castToString(value)); 5149 } else 5150 return super.setProperty(name, value); 5151 return value; 5152 } 5153 5154 @Override 5155 public Base makeProperty(int hash, String name) throws FHIRException { 5156 switch (hash) { 5157 case 3373707: return getNameElement(); 5158 case -1249586564: return addVariableElement(); 5159 default: return super.makeProperty(hash, name); 5160 } 5161 5162 } 5163 5164 @Override 5165 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5166 switch (hash) { 5167 case 3373707: /*name*/ return new String[] {"id"}; 5168 case -1249586564: /*variable*/ return new String[] {"string"}; 5169 default: return super.getTypesForProperty(hash, name); 5170 } 5171 5172 } 5173 5174 @Override 5175 public Base addChild(String name) throws FHIRException { 5176 if (name.equals("name")) { 5177 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 5178 } 5179 else if (name.equals("variable")) { 5180 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.variable"); 5181 } 5182 else 5183 return super.addChild(name); 5184 } 5185 5186 public StructureMapGroupRuleDependentComponent copy() { 5187 StructureMapGroupRuleDependentComponent dst = new StructureMapGroupRuleDependentComponent(); 5188 copyValues(dst); 5189 dst.name = name == null ? null : name.copy(); 5190 if (variable != null) { 5191 dst.variable = new ArrayList<StringType>(); 5192 for (StringType i : variable) 5193 dst.variable.add(i.copy()); 5194 }; 5195 return dst; 5196 } 5197 5198 @Override 5199 public boolean equalsDeep(Base other_) { 5200 if (!super.equalsDeep(other_)) 5201 return false; 5202 if (!(other_ instanceof StructureMapGroupRuleDependentComponent)) 5203 return false; 5204 StructureMapGroupRuleDependentComponent o = (StructureMapGroupRuleDependentComponent) other_; 5205 return compareDeep(name, o.name, true) && compareDeep(variable, o.variable, true); 5206 } 5207 5208 @Override 5209 public boolean equalsShallow(Base other_) { 5210 if (!super.equalsShallow(other_)) 5211 return false; 5212 if (!(other_ instanceof StructureMapGroupRuleDependentComponent)) 5213 return false; 5214 StructureMapGroupRuleDependentComponent o = (StructureMapGroupRuleDependentComponent) other_; 5215 return compareValues(name, o.name, true) && compareValues(variable, o.variable, true); 5216 } 5217 5218 public boolean isEmpty() { 5219 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, variable); 5220 } 5221 5222 public String fhirType() { 5223 return "StructureMap.group.rule.dependent"; 5224 5225 } 5226 5227 } 5228 5229 /** 5230 * A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance. 5231 */ 5232 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5233 @Description(shortDefinition="Additional identifier for the structure map", formalDefinition="A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 5234 protected List<Identifier> identifier; 5235 5236 /** 5237 * Explaination of why this structure map is needed and why it has been designed as it has. 5238 */ 5239 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 5240 @Description(shortDefinition="Why this structure map is defined", formalDefinition="Explaination of why this structure map is needed and why it has been designed as it has." ) 5241 protected MarkdownType purpose; 5242 5243 /** 5244 * A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map. 5245 */ 5246 @Child(name = "copyright", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5247 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map." ) 5248 protected MarkdownType copyright; 5249 5250 /** 5251 * A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced. 5252 */ 5253 @Child(name = "structure", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5254 @Description(shortDefinition="Structure Definition used by this map", formalDefinition="A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced." ) 5255 protected List<StructureMapStructureComponent> structure; 5256 5257 /** 5258 * Other maps used by this map (canonical URLs). 5259 */ 5260 @Child(name = "import", type = {UriType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5261 @Description(shortDefinition="Other maps used by this map (canonical URLs)", formalDefinition="Other maps used by this map (canonical URLs)." ) 5262 protected List<UriType> import_; 5263 5264 /** 5265 * Organizes the mapping into managable chunks for human review/ease of maintenance. 5266 */ 5267 @Child(name = "group", type = {}, order=5, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5268 @Description(shortDefinition="Named sections for reader convenience", formalDefinition="Organizes the mapping into managable chunks for human review/ease of maintenance." ) 5269 protected List<StructureMapGroupComponent> group; 5270 5271 private static final long serialVersionUID = 952506557L; 5272 5273 /** 5274 * Constructor 5275 */ 5276 public StructureMap() { 5277 super(); 5278 } 5279 5280 /** 5281 * Constructor 5282 */ 5283 public StructureMap(UriType url, StringType name, Enumeration<PublicationStatus> status) { 5284 super(); 5285 this.url = url; 5286 this.name = name; 5287 this.status = status; 5288 } 5289 5290 /** 5291 * @return {@link #url} (An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure map is (or will be) published. The URL SHOULD include the major version of the structure map. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5292 */ 5293 public UriType getUrlElement() { 5294 if (this.url == null) 5295 if (Configuration.errorOnAutoCreate()) 5296 throw new Error("Attempt to auto-create StructureMap.url"); 5297 else if (Configuration.doAutoCreate()) 5298 this.url = new UriType(); // bb 5299 return this.url; 5300 } 5301 5302 public boolean hasUrlElement() { 5303 return this.url != null && !this.url.isEmpty(); 5304 } 5305 5306 public boolean hasUrl() { 5307 return this.url != null && !this.url.isEmpty(); 5308 } 5309 5310 /** 5311 * @param value {@link #url} (An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure map is (or will be) published. The URL SHOULD include the major version of the structure map. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 5312 */ 5313 public StructureMap setUrlElement(UriType value) { 5314 this.url = value; 5315 return this; 5316 } 5317 5318 /** 5319 * @return An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure map is (or will be) published. The URL SHOULD include the major version of the structure map. For more information see [Technical and Business Versions](resource.html#versions). 5320 */ 5321 public String getUrl() { 5322 return this.url == null ? null : this.url.getValue(); 5323 } 5324 5325 /** 5326 * @param value An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure map is (or will be) published. The URL SHOULD include the major version of the structure map. For more information see [Technical and Business Versions](resource.html#versions). 5327 */ 5328 public StructureMap setUrl(String value) { 5329 if (this.url == null) 5330 this.url = new UriType(); 5331 this.url.setValue(value); 5332 return this; 5333 } 5334 5335 /** 5336 * @return {@link #identifier} (A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.) 5337 */ 5338 public List<Identifier> getIdentifier() { 5339 if (this.identifier == null) 5340 this.identifier = new ArrayList<Identifier>(); 5341 return this.identifier; 5342 } 5343 5344 /** 5345 * @return Returns a reference to <code>this</code> for easy method chaining 5346 */ 5347 public StructureMap setIdentifier(List<Identifier> theIdentifier) { 5348 this.identifier = theIdentifier; 5349 return this; 5350 } 5351 5352 public boolean hasIdentifier() { 5353 if (this.identifier == null) 5354 return false; 5355 for (Identifier item : this.identifier) 5356 if (!item.isEmpty()) 5357 return true; 5358 return false; 5359 } 5360 5361 public Identifier addIdentifier() { //3 5362 Identifier t = new Identifier(); 5363 if (this.identifier == null) 5364 this.identifier = new ArrayList<Identifier>(); 5365 this.identifier.add(t); 5366 return t; 5367 } 5368 5369 public StructureMap addIdentifier(Identifier t) { //3 5370 if (t == null) 5371 return this; 5372 if (this.identifier == null) 5373 this.identifier = new ArrayList<Identifier>(); 5374 this.identifier.add(t); 5375 return this; 5376 } 5377 5378 /** 5379 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 5380 */ 5381 public Identifier getIdentifierFirstRep() { 5382 if (getIdentifier().isEmpty()) { 5383 addIdentifier(); 5384 } 5385 return getIdentifier().get(0); 5386 } 5387 5388 /** 5389 * @return {@link #version} (The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5390 */ 5391 public StringType getVersionElement() { 5392 if (this.version == null) 5393 if (Configuration.errorOnAutoCreate()) 5394 throw new Error("Attempt to auto-create StructureMap.version"); 5395 else if (Configuration.doAutoCreate()) 5396 this.version = new StringType(); // bb 5397 return this.version; 5398 } 5399 5400 public boolean hasVersionElement() { 5401 return this.version != null && !this.version.isEmpty(); 5402 } 5403 5404 public boolean hasVersion() { 5405 return this.version != null && !this.version.isEmpty(); 5406 } 5407 5408 /** 5409 * @param value {@link #version} (The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 5410 */ 5411 public StructureMap setVersionElement(StringType value) { 5412 this.version = value; 5413 return this; 5414 } 5415 5416 /** 5417 * @return The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5418 */ 5419 public String getVersion() { 5420 return this.version == null ? null : this.version.getValue(); 5421 } 5422 5423 /** 5424 * @param value The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 5425 */ 5426 public StructureMap setVersion(String value) { 5427 if (Utilities.noString(value)) 5428 this.version = null; 5429 else { 5430 if (this.version == null) 5431 this.version = new StringType(); 5432 this.version.setValue(value); 5433 } 5434 return this; 5435 } 5436 5437 /** 5438 * @return {@link #name} (A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5439 */ 5440 public StringType getNameElement() { 5441 if (this.name == null) 5442 if (Configuration.errorOnAutoCreate()) 5443 throw new Error("Attempt to auto-create StructureMap.name"); 5444 else if (Configuration.doAutoCreate()) 5445 this.name = new StringType(); // bb 5446 return this.name; 5447 } 5448 5449 public boolean hasNameElement() { 5450 return this.name != null && !this.name.isEmpty(); 5451 } 5452 5453 public boolean hasName() { 5454 return this.name != null && !this.name.isEmpty(); 5455 } 5456 5457 /** 5458 * @param value {@link #name} (A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 5459 */ 5460 public StructureMap setNameElement(StringType value) { 5461 this.name = value; 5462 return this; 5463 } 5464 5465 /** 5466 * @return A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5467 */ 5468 public String getName() { 5469 return this.name == null ? null : this.name.getValue(); 5470 } 5471 5472 /** 5473 * @param value A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation. 5474 */ 5475 public StructureMap setName(String value) { 5476 if (this.name == null) 5477 this.name = new StringType(); 5478 this.name.setValue(value); 5479 return this; 5480 } 5481 5482 /** 5483 * @return {@link #title} (A short, descriptive, user-friendly title for the structure map.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 5484 */ 5485 public StringType getTitleElement() { 5486 if (this.title == null) 5487 if (Configuration.errorOnAutoCreate()) 5488 throw new Error("Attempt to auto-create StructureMap.title"); 5489 else if (Configuration.doAutoCreate()) 5490 this.title = new StringType(); // bb 5491 return this.title; 5492 } 5493 5494 public boolean hasTitleElement() { 5495 return this.title != null && !this.title.isEmpty(); 5496 } 5497 5498 public boolean hasTitle() { 5499 return this.title != null && !this.title.isEmpty(); 5500 } 5501 5502 /** 5503 * @param value {@link #title} (A short, descriptive, user-friendly title for the structure map.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 5504 */ 5505 public StructureMap setTitleElement(StringType value) { 5506 this.title = value; 5507 return this; 5508 } 5509 5510 /** 5511 * @return A short, descriptive, user-friendly title for the structure map. 5512 */ 5513 public String getTitle() { 5514 return this.title == null ? null : this.title.getValue(); 5515 } 5516 5517 /** 5518 * @param value A short, descriptive, user-friendly title for the structure map. 5519 */ 5520 public StructureMap setTitle(String value) { 5521 if (Utilities.noString(value)) 5522 this.title = null; 5523 else { 5524 if (this.title == null) 5525 this.title = new StringType(); 5526 this.title.setValue(value); 5527 } 5528 return this; 5529 } 5530 5531 /** 5532 * @return {@link #status} (The status of this structure map. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5533 */ 5534 public Enumeration<PublicationStatus> getStatusElement() { 5535 if (this.status == null) 5536 if (Configuration.errorOnAutoCreate()) 5537 throw new Error("Attempt to auto-create StructureMap.status"); 5538 else if (Configuration.doAutoCreate()) 5539 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 5540 return this.status; 5541 } 5542 5543 public boolean hasStatusElement() { 5544 return this.status != null && !this.status.isEmpty(); 5545 } 5546 5547 public boolean hasStatus() { 5548 return this.status != null && !this.status.isEmpty(); 5549 } 5550 5551 /** 5552 * @param value {@link #status} (The status of this structure map. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5553 */ 5554 public StructureMap setStatusElement(Enumeration<PublicationStatus> value) { 5555 this.status = value; 5556 return this; 5557 } 5558 5559 /** 5560 * @return The status of this structure map. Enables tracking the life-cycle of the content. 5561 */ 5562 public PublicationStatus getStatus() { 5563 return this.status == null ? null : this.status.getValue(); 5564 } 5565 5566 /** 5567 * @param value The status of this structure map. Enables tracking the life-cycle of the content. 5568 */ 5569 public StructureMap setStatus(PublicationStatus value) { 5570 if (this.status == null) 5571 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 5572 this.status.setValue(value); 5573 return this; 5574 } 5575 5576 /** 5577 * @return {@link #experimental} (A boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 5578 */ 5579 public BooleanType getExperimentalElement() { 5580 if (this.experimental == null) 5581 if (Configuration.errorOnAutoCreate()) 5582 throw new Error("Attempt to auto-create StructureMap.experimental"); 5583 else if (Configuration.doAutoCreate()) 5584 this.experimental = new BooleanType(); // bb 5585 return this.experimental; 5586 } 5587 5588 public boolean hasExperimentalElement() { 5589 return this.experimental != null && !this.experimental.isEmpty(); 5590 } 5591 5592 public boolean hasExperimental() { 5593 return this.experimental != null && !this.experimental.isEmpty(); 5594 } 5595 5596 /** 5597 * @param value {@link #experimental} (A boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 5598 */ 5599 public StructureMap setExperimentalElement(BooleanType value) { 5600 this.experimental = value; 5601 return this; 5602 } 5603 5604 /** 5605 * @return A boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 5606 */ 5607 public boolean getExperimental() { 5608 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 5609 } 5610 5611 /** 5612 * @param value A boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 5613 */ 5614 public StructureMap setExperimental(boolean value) { 5615 if (this.experimental == null) 5616 this.experimental = new BooleanType(); 5617 this.experimental.setValue(value); 5618 return this; 5619 } 5620 5621 /** 5622 * @return {@link #date} (The date (and optionally time) when the structure map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 5623 */ 5624 public DateTimeType getDateElement() { 5625 if (this.date == null) 5626 if (Configuration.errorOnAutoCreate()) 5627 throw new Error("Attempt to auto-create StructureMap.date"); 5628 else if (Configuration.doAutoCreate()) 5629 this.date = new DateTimeType(); // bb 5630 return this.date; 5631 } 5632 5633 public boolean hasDateElement() { 5634 return this.date != null && !this.date.isEmpty(); 5635 } 5636 5637 public boolean hasDate() { 5638 return this.date != null && !this.date.isEmpty(); 5639 } 5640 5641 /** 5642 * @param value {@link #date} (The date (and optionally time) when the structure map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 5643 */ 5644 public StructureMap setDateElement(DateTimeType value) { 5645 this.date = value; 5646 return this; 5647 } 5648 5649 /** 5650 * @return The date (and optionally time) when the structure map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes. 5651 */ 5652 public Date getDate() { 5653 return this.date == null ? null : this.date.getValue(); 5654 } 5655 5656 /** 5657 * @param value The date (and optionally time) when the structure map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes. 5658 */ 5659 public StructureMap setDate(Date value) { 5660 if (value == null) 5661 this.date = null; 5662 else { 5663 if (this.date == null) 5664 this.date = new DateTimeType(); 5665 this.date.setValue(value); 5666 } 5667 return this; 5668 } 5669 5670 /** 5671 * @return {@link #publisher} (The name of the individual or organization that published the structure map.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 5672 */ 5673 public StringType getPublisherElement() { 5674 if (this.publisher == null) 5675 if (Configuration.errorOnAutoCreate()) 5676 throw new Error("Attempt to auto-create StructureMap.publisher"); 5677 else if (Configuration.doAutoCreate()) 5678 this.publisher = new StringType(); // bb 5679 return this.publisher; 5680 } 5681 5682 public boolean hasPublisherElement() { 5683 return this.publisher != null && !this.publisher.isEmpty(); 5684 } 5685 5686 public boolean hasPublisher() { 5687 return this.publisher != null && !this.publisher.isEmpty(); 5688 } 5689 5690 /** 5691 * @param value {@link #publisher} (The name of the individual or organization that published the structure map.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 5692 */ 5693 public StructureMap setPublisherElement(StringType value) { 5694 this.publisher = value; 5695 return this; 5696 } 5697 5698 /** 5699 * @return The name of the individual or organization that published the structure map. 5700 */ 5701 public String getPublisher() { 5702 return this.publisher == null ? null : this.publisher.getValue(); 5703 } 5704 5705 /** 5706 * @param value The name of the individual or organization that published the structure map. 5707 */ 5708 public StructureMap setPublisher(String value) { 5709 if (Utilities.noString(value)) 5710 this.publisher = null; 5711 else { 5712 if (this.publisher == null) 5713 this.publisher = new StringType(); 5714 this.publisher.setValue(value); 5715 } 5716 return this; 5717 } 5718 5719 /** 5720 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 5721 */ 5722 public List<ContactDetail> getContact() { 5723 if (this.contact == null) 5724 this.contact = new ArrayList<ContactDetail>(); 5725 return this.contact; 5726 } 5727 5728 /** 5729 * @return Returns a reference to <code>this</code> for easy method chaining 5730 */ 5731 public StructureMap setContact(List<ContactDetail> theContact) { 5732 this.contact = theContact; 5733 return this; 5734 } 5735 5736 public boolean hasContact() { 5737 if (this.contact == null) 5738 return false; 5739 for (ContactDetail item : this.contact) 5740 if (!item.isEmpty()) 5741 return true; 5742 return false; 5743 } 5744 5745 public ContactDetail addContact() { //3 5746 ContactDetail t = new ContactDetail(); 5747 if (this.contact == null) 5748 this.contact = new ArrayList<ContactDetail>(); 5749 this.contact.add(t); 5750 return t; 5751 } 5752 5753 public StructureMap addContact(ContactDetail t) { //3 5754 if (t == null) 5755 return this; 5756 if (this.contact == null) 5757 this.contact = new ArrayList<ContactDetail>(); 5758 this.contact.add(t); 5759 return this; 5760 } 5761 5762 /** 5763 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 5764 */ 5765 public ContactDetail getContactFirstRep() { 5766 if (getContact().isEmpty()) { 5767 addContact(); 5768 } 5769 return getContact().get(0); 5770 } 5771 5772 /** 5773 * @return {@link #description} (A free text natural language description of the structure map from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5774 */ 5775 public MarkdownType getDescriptionElement() { 5776 if (this.description == null) 5777 if (Configuration.errorOnAutoCreate()) 5778 throw new Error("Attempt to auto-create StructureMap.description"); 5779 else if (Configuration.doAutoCreate()) 5780 this.description = new MarkdownType(); // bb 5781 return this.description; 5782 } 5783 5784 public boolean hasDescriptionElement() { 5785 return this.description != null && !this.description.isEmpty(); 5786 } 5787 5788 public boolean hasDescription() { 5789 return this.description != null && !this.description.isEmpty(); 5790 } 5791 5792 /** 5793 * @param value {@link #description} (A free text natural language description of the structure map from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5794 */ 5795 public StructureMap setDescriptionElement(MarkdownType value) { 5796 this.description = value; 5797 return this; 5798 } 5799 5800 /** 5801 * @return A free text natural language description of the structure map from a consumer's perspective. 5802 */ 5803 public String getDescription() { 5804 return this.description == null ? null : this.description.getValue(); 5805 } 5806 5807 /** 5808 * @param value A free text natural language description of the structure map from a consumer's perspective. 5809 */ 5810 public StructureMap setDescription(String value) { 5811 if (value == null) 5812 this.description = null; 5813 else { 5814 if (this.description == null) 5815 this.description = new MarkdownType(); 5816 this.description.setValue(value); 5817 } 5818 return this; 5819 } 5820 5821 /** 5822 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate structure map instances.) 5823 */ 5824 public List<UsageContext> getUseContext() { 5825 if (this.useContext == null) 5826 this.useContext = new ArrayList<UsageContext>(); 5827 return this.useContext; 5828 } 5829 5830 /** 5831 * @return Returns a reference to <code>this</code> for easy method chaining 5832 */ 5833 public StructureMap setUseContext(List<UsageContext> theUseContext) { 5834 this.useContext = theUseContext; 5835 return this; 5836 } 5837 5838 public boolean hasUseContext() { 5839 if (this.useContext == null) 5840 return false; 5841 for (UsageContext item : this.useContext) 5842 if (!item.isEmpty()) 5843 return true; 5844 return false; 5845 } 5846 5847 public UsageContext addUseContext() { //3 5848 UsageContext t = new UsageContext(); 5849 if (this.useContext == null) 5850 this.useContext = new ArrayList<UsageContext>(); 5851 this.useContext.add(t); 5852 return t; 5853 } 5854 5855 public StructureMap addUseContext(UsageContext t) { //3 5856 if (t == null) 5857 return this; 5858 if (this.useContext == null) 5859 this.useContext = new ArrayList<UsageContext>(); 5860 this.useContext.add(t); 5861 return this; 5862 } 5863 5864 /** 5865 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 5866 */ 5867 public UsageContext getUseContextFirstRep() { 5868 if (getUseContext().isEmpty()) { 5869 addUseContext(); 5870 } 5871 return getUseContext().get(0); 5872 } 5873 5874 /** 5875 * @return {@link #jurisdiction} (A legal or geographic region in which the structure map is intended to be used.) 5876 */ 5877 public List<CodeableConcept> getJurisdiction() { 5878 if (this.jurisdiction == null) 5879 this.jurisdiction = new ArrayList<CodeableConcept>(); 5880 return this.jurisdiction; 5881 } 5882 5883 /** 5884 * @return Returns a reference to <code>this</code> for easy method chaining 5885 */ 5886 public StructureMap setJurisdiction(List<CodeableConcept> theJurisdiction) { 5887 this.jurisdiction = theJurisdiction; 5888 return this; 5889 } 5890 5891 public boolean hasJurisdiction() { 5892 if (this.jurisdiction == null) 5893 return false; 5894 for (CodeableConcept item : this.jurisdiction) 5895 if (!item.isEmpty()) 5896 return true; 5897 return false; 5898 } 5899 5900 public CodeableConcept addJurisdiction() { //3 5901 CodeableConcept t = new CodeableConcept(); 5902 if (this.jurisdiction == null) 5903 this.jurisdiction = new ArrayList<CodeableConcept>(); 5904 this.jurisdiction.add(t); 5905 return t; 5906 } 5907 5908 public StructureMap addJurisdiction(CodeableConcept t) { //3 5909 if (t == null) 5910 return this; 5911 if (this.jurisdiction == null) 5912 this.jurisdiction = new ArrayList<CodeableConcept>(); 5913 this.jurisdiction.add(t); 5914 return this; 5915 } 5916 5917 /** 5918 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 5919 */ 5920 public CodeableConcept getJurisdictionFirstRep() { 5921 if (getJurisdiction().isEmpty()) { 5922 addJurisdiction(); 5923 } 5924 return getJurisdiction().get(0); 5925 } 5926 5927 /** 5928 * @return {@link #purpose} (Explaination of why this structure map is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 5929 */ 5930 public MarkdownType getPurposeElement() { 5931 if (this.purpose == null) 5932 if (Configuration.errorOnAutoCreate()) 5933 throw new Error("Attempt to auto-create StructureMap.purpose"); 5934 else if (Configuration.doAutoCreate()) 5935 this.purpose = new MarkdownType(); // bb 5936 return this.purpose; 5937 } 5938 5939 public boolean hasPurposeElement() { 5940 return this.purpose != null && !this.purpose.isEmpty(); 5941 } 5942 5943 public boolean hasPurpose() { 5944 return this.purpose != null && !this.purpose.isEmpty(); 5945 } 5946 5947 /** 5948 * @param value {@link #purpose} (Explaination of why this structure map is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 5949 */ 5950 public StructureMap setPurposeElement(MarkdownType value) { 5951 this.purpose = value; 5952 return this; 5953 } 5954 5955 /** 5956 * @return Explaination of why this structure map is needed and why it has been designed as it has. 5957 */ 5958 public String getPurpose() { 5959 return this.purpose == null ? null : this.purpose.getValue(); 5960 } 5961 5962 /** 5963 * @param value Explaination of why this structure map is needed and why it has been designed as it has. 5964 */ 5965 public StructureMap setPurpose(String value) { 5966 if (value == null) 5967 this.purpose = null; 5968 else { 5969 if (this.purpose == null) 5970 this.purpose = new MarkdownType(); 5971 this.purpose.setValue(value); 5972 } 5973 return this; 5974 } 5975 5976 /** 5977 * @return {@link #copyright} (A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 5978 */ 5979 public MarkdownType getCopyrightElement() { 5980 if (this.copyright == null) 5981 if (Configuration.errorOnAutoCreate()) 5982 throw new Error("Attempt to auto-create StructureMap.copyright"); 5983 else if (Configuration.doAutoCreate()) 5984 this.copyright = new MarkdownType(); // bb 5985 return this.copyright; 5986 } 5987 5988 public boolean hasCopyrightElement() { 5989 return this.copyright != null && !this.copyright.isEmpty(); 5990 } 5991 5992 public boolean hasCopyright() { 5993 return this.copyright != null && !this.copyright.isEmpty(); 5994 } 5995 5996 /** 5997 * @param value {@link #copyright} (A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 5998 */ 5999 public StructureMap setCopyrightElement(MarkdownType value) { 6000 this.copyright = value; 6001 return this; 6002 } 6003 6004 /** 6005 * @return A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map. 6006 */ 6007 public String getCopyright() { 6008 return this.copyright == null ? null : this.copyright.getValue(); 6009 } 6010 6011 /** 6012 * @param value A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map. 6013 */ 6014 public StructureMap setCopyright(String value) { 6015 if (value == null) 6016 this.copyright = null; 6017 else { 6018 if (this.copyright == null) 6019 this.copyright = new MarkdownType(); 6020 this.copyright.setValue(value); 6021 } 6022 return this; 6023 } 6024 6025 /** 6026 * @return {@link #structure} (A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.) 6027 */ 6028 public List<StructureMapStructureComponent> getStructure() { 6029 if (this.structure == null) 6030 this.structure = new ArrayList<StructureMapStructureComponent>(); 6031 return this.structure; 6032 } 6033 6034 /** 6035 * @return Returns a reference to <code>this</code> for easy method chaining 6036 */ 6037 public StructureMap setStructure(List<StructureMapStructureComponent> theStructure) { 6038 this.structure = theStructure; 6039 return this; 6040 } 6041 6042 public boolean hasStructure() { 6043 if (this.structure == null) 6044 return false; 6045 for (StructureMapStructureComponent item : this.structure) 6046 if (!item.isEmpty()) 6047 return true; 6048 return false; 6049 } 6050 6051 public StructureMapStructureComponent addStructure() { //3 6052 StructureMapStructureComponent t = new StructureMapStructureComponent(); 6053 if (this.structure == null) 6054 this.structure = new ArrayList<StructureMapStructureComponent>(); 6055 this.structure.add(t); 6056 return t; 6057 } 6058 6059 public StructureMap addStructure(StructureMapStructureComponent t) { //3 6060 if (t == null) 6061 return this; 6062 if (this.structure == null) 6063 this.structure = new ArrayList<StructureMapStructureComponent>(); 6064 this.structure.add(t); 6065 return this; 6066 } 6067 6068 /** 6069 * @return The first repetition of repeating field {@link #structure}, creating it if it does not already exist 6070 */ 6071 public StructureMapStructureComponent getStructureFirstRep() { 6072 if (getStructure().isEmpty()) { 6073 addStructure(); 6074 } 6075 return getStructure().get(0); 6076 } 6077 6078 /** 6079 * @return {@link #import_} (Other maps used by this map (canonical URLs).) 6080 */ 6081 public List<UriType> getImport() { 6082 if (this.import_ == null) 6083 this.import_ = new ArrayList<UriType>(); 6084 return this.import_; 6085 } 6086 6087 /** 6088 * @return Returns a reference to <code>this</code> for easy method chaining 6089 */ 6090 public StructureMap setImport(List<UriType> theImport) { 6091 this.import_ = theImport; 6092 return this; 6093 } 6094 6095 public boolean hasImport() { 6096 if (this.import_ == null) 6097 return false; 6098 for (UriType item : this.import_) 6099 if (!item.isEmpty()) 6100 return true; 6101 return false; 6102 } 6103 6104 /** 6105 * @return {@link #import_} (Other maps used by this map (canonical URLs).) 6106 */ 6107 public UriType addImportElement() {//2 6108 UriType t = new UriType(); 6109 if (this.import_ == null) 6110 this.import_ = new ArrayList<UriType>(); 6111 this.import_.add(t); 6112 return t; 6113 } 6114 6115 /** 6116 * @param value {@link #import_} (Other maps used by this map (canonical URLs).) 6117 */ 6118 public StructureMap addImport(String value) { //1 6119 UriType t = new UriType(); 6120 t.setValue(value); 6121 if (this.import_ == null) 6122 this.import_ = new ArrayList<UriType>(); 6123 this.import_.add(t); 6124 return this; 6125 } 6126 6127 /** 6128 * @param value {@link #import_} (Other maps used by this map (canonical URLs).) 6129 */ 6130 public boolean hasImport(String value) { 6131 if (this.import_ == null) 6132 return false; 6133 for (UriType v : this.import_) 6134 if (v.getValue().equals(value)) // uri 6135 return true; 6136 return false; 6137 } 6138 6139 /** 6140 * @return {@link #group} (Organizes the mapping into managable chunks for human review/ease of maintenance.) 6141 */ 6142 public List<StructureMapGroupComponent> getGroup() { 6143 if (this.group == null) 6144 this.group = new ArrayList<StructureMapGroupComponent>(); 6145 return this.group; 6146 } 6147 6148 /** 6149 * @return Returns a reference to <code>this</code> for easy method chaining 6150 */ 6151 public StructureMap setGroup(List<StructureMapGroupComponent> theGroup) { 6152 this.group = theGroup; 6153 return this; 6154 } 6155 6156 public boolean hasGroup() { 6157 if (this.group == null) 6158 return false; 6159 for (StructureMapGroupComponent item : this.group) 6160 if (!item.isEmpty()) 6161 return true; 6162 return false; 6163 } 6164 6165 public StructureMapGroupComponent addGroup() { //3 6166 StructureMapGroupComponent t = new StructureMapGroupComponent(); 6167 if (this.group == null) 6168 this.group = new ArrayList<StructureMapGroupComponent>(); 6169 this.group.add(t); 6170 return t; 6171 } 6172 6173 public StructureMap addGroup(StructureMapGroupComponent t) { //3 6174 if (t == null) 6175 return this; 6176 if (this.group == null) 6177 this.group = new ArrayList<StructureMapGroupComponent>(); 6178 this.group.add(t); 6179 return this; 6180 } 6181 6182 /** 6183 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist 6184 */ 6185 public StructureMapGroupComponent getGroupFirstRep() { 6186 if (getGroup().isEmpty()) { 6187 addGroup(); 6188 } 6189 return getGroup().get(0); 6190 } 6191 6192 protected void listChildren(List<Property> children) { 6193 super.listChildren(children); 6194 children.add(new Property("url", "uri", "An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure map is (or will be) published. The URL SHOULD include the major version of the structure map. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 6195 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 6196 children.add(new Property("version", "string", "The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 6197 children.add(new Property("name", "string", "A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 6198 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the structure map.", 0, 1, title)); 6199 children.add(new Property("status", "code", "The status of this structure map. Enables tracking the life-cycle of the content.", 0, 1, status)); 6200 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 6201 children.add(new Property("date", "dateTime", "The date (and optionally time) when the structure map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.", 0, 1, date)); 6202 children.add(new Property("publisher", "string", "The name of the individual or organization that published the structure map.", 0, 1, publisher)); 6203 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 6204 children.add(new Property("description", "markdown", "A free text natural language description of the structure map from a consumer's perspective.", 0, 1, description)); 6205 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate structure map instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 6206 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 6207 children.add(new Property("purpose", "markdown", "Explaination of why this structure map is needed and why it has been designed as it has.", 0, 1, purpose)); 6208 children.add(new Property("copyright", "markdown", "A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.", 0, 1, copyright)); 6209 children.add(new Property("structure", "", "A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.", 0, java.lang.Integer.MAX_VALUE, structure)); 6210 children.add(new Property("import", "uri", "Other maps used by this map (canonical URLs).", 0, java.lang.Integer.MAX_VALUE, import_)); 6211 children.add(new Property("group", "", "Organizes the mapping into managable chunks for human review/ease of maintenance.", 0, java.lang.Integer.MAX_VALUE, group)); 6212 } 6213 6214 @Override 6215 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6216 switch (_hash) { 6217 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this structure map is (or will be) published. The URL SHOULD include the major version of the structure map. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 6218 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 6219 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 6220 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 6221 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the structure map.", 0, 1, title); 6222 case -892481550: /*status*/ return new Property("status", "code", "The status of this structure map. Enables tracking the life-cycle of the content.", 0, 1, status); 6223 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 6224 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the structure map was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.", 0, 1, date); 6225 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the structure map.", 0, 1, publisher); 6226 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 6227 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the structure map from a consumer's perspective.", 0, 1, description); 6228 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate structure map instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 6229 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the structure map is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 6230 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this structure map is needed and why it has been designed as it has.", 0, 1, purpose); 6231 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.", 0, 1, copyright); 6232 case 144518515: /*structure*/ return new Property("structure", "", "A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.", 0, java.lang.Integer.MAX_VALUE, structure); 6233 case -1184795739: /*import*/ return new Property("import", "uri", "Other maps used by this map (canonical URLs).", 0, java.lang.Integer.MAX_VALUE, import_); 6234 case 98629247: /*group*/ return new Property("group", "", "Organizes the mapping into managable chunks for human review/ease of maintenance.", 0, java.lang.Integer.MAX_VALUE, group); 6235 default: return super.getNamedProperty(_hash, _name, _checkValid); 6236 } 6237 6238 } 6239 6240 @Override 6241 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6242 switch (hash) { 6243 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 6244 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 6245 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 6246 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 6247 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 6248 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 6249 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 6250 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 6251 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 6252 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 6253 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 6254 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 6255 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 6256 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 6257 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 6258 case 144518515: /*structure*/ return this.structure == null ? new Base[0] : this.structure.toArray(new Base[this.structure.size()]); // StructureMapStructureComponent 6259 case -1184795739: /*import*/ return this.import_ == null ? new Base[0] : this.import_.toArray(new Base[this.import_.size()]); // UriType 6260 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // StructureMapGroupComponent 6261 default: return super.getProperty(hash, name, checkValid); 6262 } 6263 6264 } 6265 6266 @Override 6267 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6268 switch (hash) { 6269 case 116079: // url 6270 this.url = castToUri(value); // UriType 6271 return value; 6272 case -1618432855: // identifier 6273 this.getIdentifier().add(castToIdentifier(value)); // Identifier 6274 return value; 6275 case 351608024: // version 6276 this.version = castToString(value); // StringType 6277 return value; 6278 case 3373707: // name 6279 this.name = castToString(value); // StringType 6280 return value; 6281 case 110371416: // title 6282 this.title = castToString(value); // StringType 6283 return value; 6284 case -892481550: // status 6285 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 6286 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6287 return value; 6288 case -404562712: // experimental 6289 this.experimental = castToBoolean(value); // BooleanType 6290 return value; 6291 case 3076014: // date 6292 this.date = castToDateTime(value); // DateTimeType 6293 return value; 6294 case 1447404028: // publisher 6295 this.publisher = castToString(value); // StringType 6296 return value; 6297 case 951526432: // contact 6298 this.getContact().add(castToContactDetail(value)); // ContactDetail 6299 return value; 6300 case -1724546052: // description 6301 this.description = castToMarkdown(value); // MarkdownType 6302 return value; 6303 case -669707736: // useContext 6304 this.getUseContext().add(castToUsageContext(value)); // UsageContext 6305 return value; 6306 case -507075711: // jurisdiction 6307 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 6308 return value; 6309 case -220463842: // purpose 6310 this.purpose = castToMarkdown(value); // MarkdownType 6311 return value; 6312 case 1522889671: // copyright 6313 this.copyright = castToMarkdown(value); // MarkdownType 6314 return value; 6315 case 144518515: // structure 6316 this.getStructure().add((StructureMapStructureComponent) value); // StructureMapStructureComponent 6317 return value; 6318 case -1184795739: // import 6319 this.getImport().add(castToUri(value)); // UriType 6320 return value; 6321 case 98629247: // group 6322 this.getGroup().add((StructureMapGroupComponent) value); // StructureMapGroupComponent 6323 return value; 6324 default: return super.setProperty(hash, name, value); 6325 } 6326 6327 } 6328 6329 @Override 6330 public Base setProperty(String name, Base value) throws FHIRException { 6331 if (name.equals("url")) { 6332 this.url = castToUri(value); // UriType 6333 } else if (name.equals("identifier")) { 6334 this.getIdentifier().add(castToIdentifier(value)); 6335 } else if (name.equals("version")) { 6336 this.version = castToString(value); // StringType 6337 } else if (name.equals("name")) { 6338 this.name = castToString(value); // StringType 6339 } else if (name.equals("title")) { 6340 this.title = castToString(value); // StringType 6341 } else if (name.equals("status")) { 6342 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 6343 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6344 } else if (name.equals("experimental")) { 6345 this.experimental = castToBoolean(value); // BooleanType 6346 } else if (name.equals("date")) { 6347 this.date = castToDateTime(value); // DateTimeType 6348 } else if (name.equals("publisher")) { 6349 this.publisher = castToString(value); // StringType 6350 } else if (name.equals("contact")) { 6351 this.getContact().add(castToContactDetail(value)); 6352 } else if (name.equals("description")) { 6353 this.description = castToMarkdown(value); // MarkdownType 6354 } else if (name.equals("useContext")) { 6355 this.getUseContext().add(castToUsageContext(value)); 6356 } else if (name.equals("jurisdiction")) { 6357 this.getJurisdiction().add(castToCodeableConcept(value)); 6358 } else if (name.equals("purpose")) { 6359 this.purpose = castToMarkdown(value); // MarkdownType 6360 } else if (name.equals("copyright")) { 6361 this.copyright = castToMarkdown(value); // MarkdownType 6362 } else if (name.equals("structure")) { 6363 this.getStructure().add((StructureMapStructureComponent) value); 6364 } else if (name.equals("import")) { 6365 this.getImport().add(castToUri(value)); 6366 } else if (name.equals("group")) { 6367 this.getGroup().add((StructureMapGroupComponent) value); 6368 } else 6369 return super.setProperty(name, value); 6370 return value; 6371 } 6372 6373 @Override 6374 public Base makeProperty(int hash, String name) throws FHIRException { 6375 switch (hash) { 6376 case 116079: return getUrlElement(); 6377 case -1618432855: return addIdentifier(); 6378 case 351608024: return getVersionElement(); 6379 case 3373707: return getNameElement(); 6380 case 110371416: return getTitleElement(); 6381 case -892481550: return getStatusElement(); 6382 case -404562712: return getExperimentalElement(); 6383 case 3076014: return getDateElement(); 6384 case 1447404028: return getPublisherElement(); 6385 case 951526432: return addContact(); 6386 case -1724546052: return getDescriptionElement(); 6387 case -669707736: return addUseContext(); 6388 case -507075711: return addJurisdiction(); 6389 case -220463842: return getPurposeElement(); 6390 case 1522889671: return getCopyrightElement(); 6391 case 144518515: return addStructure(); 6392 case -1184795739: return addImportElement(); 6393 case 98629247: return addGroup(); 6394 default: return super.makeProperty(hash, name); 6395 } 6396 6397 } 6398 6399 @Override 6400 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6401 switch (hash) { 6402 case 116079: /*url*/ return new String[] {"uri"}; 6403 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6404 case 351608024: /*version*/ return new String[] {"string"}; 6405 case 3373707: /*name*/ return new String[] {"string"}; 6406 case 110371416: /*title*/ return new String[] {"string"}; 6407 case -892481550: /*status*/ return new String[] {"code"}; 6408 case -404562712: /*experimental*/ return new String[] {"boolean"}; 6409 case 3076014: /*date*/ return new String[] {"dateTime"}; 6410 case 1447404028: /*publisher*/ return new String[] {"string"}; 6411 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 6412 case -1724546052: /*description*/ return new String[] {"markdown"}; 6413 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 6414 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 6415 case -220463842: /*purpose*/ return new String[] {"markdown"}; 6416 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 6417 case 144518515: /*structure*/ return new String[] {}; 6418 case -1184795739: /*import*/ return new String[] {"uri"}; 6419 case 98629247: /*group*/ return new String[] {}; 6420 default: return super.getTypesForProperty(hash, name); 6421 } 6422 6423 } 6424 6425 @Override 6426 public Base addChild(String name) throws FHIRException { 6427 if (name.equals("url")) { 6428 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.url"); 6429 } 6430 else if (name.equals("identifier")) { 6431 return addIdentifier(); 6432 } 6433 else if (name.equals("version")) { 6434 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.version"); 6435 } 6436 else if (name.equals("name")) { 6437 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 6438 } 6439 else if (name.equals("title")) { 6440 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.title"); 6441 } 6442 else if (name.equals("status")) { 6443 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.status"); 6444 } 6445 else if (name.equals("experimental")) { 6446 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.experimental"); 6447 } 6448 else if (name.equals("date")) { 6449 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.date"); 6450 } 6451 else if (name.equals("publisher")) { 6452 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.publisher"); 6453 } 6454 else if (name.equals("contact")) { 6455 return addContact(); 6456 } 6457 else if (name.equals("description")) { 6458 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.description"); 6459 } 6460 else if (name.equals("useContext")) { 6461 return addUseContext(); 6462 } 6463 else if (name.equals("jurisdiction")) { 6464 return addJurisdiction(); 6465 } 6466 else if (name.equals("purpose")) { 6467 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.purpose"); 6468 } 6469 else if (name.equals("copyright")) { 6470 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.copyright"); 6471 } 6472 else if (name.equals("structure")) { 6473 return addStructure(); 6474 } 6475 else if (name.equals("import")) { 6476 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.import"); 6477 } 6478 else if (name.equals("group")) { 6479 return addGroup(); 6480 } 6481 else 6482 return super.addChild(name); 6483 } 6484 6485 public String fhirType() { 6486 return "StructureMap"; 6487 6488 } 6489 6490 public StructureMap copy() { 6491 StructureMap dst = new StructureMap(); 6492 copyValues(dst); 6493 dst.url = url == null ? null : url.copy(); 6494 if (identifier != null) { 6495 dst.identifier = new ArrayList<Identifier>(); 6496 for (Identifier i : identifier) 6497 dst.identifier.add(i.copy()); 6498 }; 6499 dst.version = version == null ? null : version.copy(); 6500 dst.name = name == null ? null : name.copy(); 6501 dst.title = title == null ? null : title.copy(); 6502 dst.status = status == null ? null : status.copy(); 6503 dst.experimental = experimental == null ? null : experimental.copy(); 6504 dst.date = date == null ? null : date.copy(); 6505 dst.publisher = publisher == null ? null : publisher.copy(); 6506 if (contact != null) { 6507 dst.contact = new ArrayList<ContactDetail>(); 6508 for (ContactDetail i : contact) 6509 dst.contact.add(i.copy()); 6510 }; 6511 dst.description = description == null ? null : description.copy(); 6512 if (useContext != null) { 6513 dst.useContext = new ArrayList<UsageContext>(); 6514 for (UsageContext i : useContext) 6515 dst.useContext.add(i.copy()); 6516 }; 6517 if (jurisdiction != null) { 6518 dst.jurisdiction = new ArrayList<CodeableConcept>(); 6519 for (CodeableConcept i : jurisdiction) 6520 dst.jurisdiction.add(i.copy()); 6521 }; 6522 dst.purpose = purpose == null ? null : purpose.copy(); 6523 dst.copyright = copyright == null ? null : copyright.copy(); 6524 if (structure != null) { 6525 dst.structure = new ArrayList<StructureMapStructureComponent>(); 6526 for (StructureMapStructureComponent i : structure) 6527 dst.structure.add(i.copy()); 6528 }; 6529 if (import_ != null) { 6530 dst.import_ = new ArrayList<UriType>(); 6531 for (UriType i : import_) 6532 dst.import_.add(i.copy()); 6533 }; 6534 if (group != null) { 6535 dst.group = new ArrayList<StructureMapGroupComponent>(); 6536 for (StructureMapGroupComponent i : group) 6537 dst.group.add(i.copy()); 6538 }; 6539 return dst; 6540 } 6541 6542 protected StructureMap typedCopy() { 6543 return copy(); 6544 } 6545 6546 @Override 6547 public boolean equalsDeep(Base other_) { 6548 if (!super.equalsDeep(other_)) 6549 return false; 6550 if (!(other_ instanceof StructureMap)) 6551 return false; 6552 StructureMap o = (StructureMap) other_; 6553 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 6554 && compareDeep(structure, o.structure, true) && compareDeep(import_, o.import_, true) && compareDeep(group, o.group, true) 6555 ; 6556 } 6557 6558 @Override 6559 public boolean equalsShallow(Base other_) { 6560 if (!super.equalsShallow(other_)) 6561 return false; 6562 if (!(other_ instanceof StructureMap)) 6563 return false; 6564 StructureMap o = (StructureMap) other_; 6565 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(import_, o.import_, true) 6566 ; 6567 } 6568 6569 public boolean isEmpty() { 6570 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright 6571 , structure, import_, group); 6572 } 6573 6574 @Override 6575 public ResourceType getResourceType() { 6576 return ResourceType.StructureMap; 6577 } 6578 6579 /** 6580 * Search parameter: <b>date</b> 6581 * <p> 6582 * Description: <b>The structure map publication date</b><br> 6583 * Type: <b>date</b><br> 6584 * Path: <b>StructureMap.date</b><br> 6585 * </p> 6586 */ 6587 @SearchParamDefinition(name="date", path="StructureMap.date", description="The structure map publication date", type="date" ) 6588 public static final String SP_DATE = "date"; 6589 /** 6590 * <b>Fluent Client</b> search parameter constant for <b>date</b> 6591 * <p> 6592 * Description: <b>The structure map publication date</b><br> 6593 * Type: <b>date</b><br> 6594 * Path: <b>StructureMap.date</b><br> 6595 * </p> 6596 */ 6597 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 6598 6599 /** 6600 * Search parameter: <b>identifier</b> 6601 * <p> 6602 * Description: <b>External identifier for the structure map</b><br> 6603 * Type: <b>token</b><br> 6604 * Path: <b>StructureMap.identifier</b><br> 6605 * </p> 6606 */ 6607 @SearchParamDefinition(name="identifier", path="StructureMap.identifier", description="External identifier for the structure map", type="token" ) 6608 public static final String SP_IDENTIFIER = "identifier"; 6609 /** 6610 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6611 * <p> 6612 * Description: <b>External identifier for the structure map</b><br> 6613 * Type: <b>token</b><br> 6614 * Path: <b>StructureMap.identifier</b><br> 6615 * </p> 6616 */ 6617 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 6618 6619 /** 6620 * Search parameter: <b>jurisdiction</b> 6621 * <p> 6622 * Description: <b>Intended jurisdiction for the structure map</b><br> 6623 * Type: <b>token</b><br> 6624 * Path: <b>StructureMap.jurisdiction</b><br> 6625 * </p> 6626 */ 6627 @SearchParamDefinition(name="jurisdiction", path="StructureMap.jurisdiction", description="Intended jurisdiction for the structure map", type="token" ) 6628 public static final String SP_JURISDICTION = "jurisdiction"; 6629 /** 6630 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 6631 * <p> 6632 * Description: <b>Intended jurisdiction for the structure map</b><br> 6633 * Type: <b>token</b><br> 6634 * Path: <b>StructureMap.jurisdiction</b><br> 6635 * </p> 6636 */ 6637 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 6638 6639 /** 6640 * Search parameter: <b>name</b> 6641 * <p> 6642 * Description: <b>Computationally friendly name of the structure map</b><br> 6643 * Type: <b>string</b><br> 6644 * Path: <b>StructureMap.name</b><br> 6645 * </p> 6646 */ 6647 @SearchParamDefinition(name="name", path="StructureMap.name", description="Computationally friendly name of the structure map", type="string" ) 6648 public static final String SP_NAME = "name"; 6649 /** 6650 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6651 * <p> 6652 * Description: <b>Computationally friendly name of the structure map</b><br> 6653 * Type: <b>string</b><br> 6654 * Path: <b>StructureMap.name</b><br> 6655 * </p> 6656 */ 6657 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 6658 6659 /** 6660 * Search parameter: <b>description</b> 6661 * <p> 6662 * Description: <b>The description of the structure map</b><br> 6663 * Type: <b>string</b><br> 6664 * Path: <b>StructureMap.description</b><br> 6665 * </p> 6666 */ 6667 @SearchParamDefinition(name="description", path="StructureMap.description", description="The description of the structure map", type="string" ) 6668 public static final String SP_DESCRIPTION = "description"; 6669 /** 6670 * <b>Fluent Client</b> search parameter constant for <b>description</b> 6671 * <p> 6672 * Description: <b>The description of the structure map</b><br> 6673 * Type: <b>string</b><br> 6674 * Path: <b>StructureMap.description</b><br> 6675 * </p> 6676 */ 6677 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 6678 6679 /** 6680 * Search parameter: <b>publisher</b> 6681 * <p> 6682 * Description: <b>Name of the publisher of the structure map</b><br> 6683 * Type: <b>string</b><br> 6684 * Path: <b>StructureMap.publisher</b><br> 6685 * </p> 6686 */ 6687 @SearchParamDefinition(name="publisher", path="StructureMap.publisher", description="Name of the publisher of the structure map", type="string" ) 6688 public static final String SP_PUBLISHER = "publisher"; 6689 /** 6690 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6691 * <p> 6692 * Description: <b>Name of the publisher of the structure map</b><br> 6693 * Type: <b>string</b><br> 6694 * Path: <b>StructureMap.publisher</b><br> 6695 * </p> 6696 */ 6697 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 6698 6699 /** 6700 * Search parameter: <b>title</b> 6701 * <p> 6702 * Description: <b>The human-friendly name of the structure map</b><br> 6703 * Type: <b>string</b><br> 6704 * Path: <b>StructureMap.title</b><br> 6705 * </p> 6706 */ 6707 @SearchParamDefinition(name="title", path="StructureMap.title", description="The human-friendly name of the structure map", type="string" ) 6708 public static final String SP_TITLE = "title"; 6709 /** 6710 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6711 * <p> 6712 * Description: <b>The human-friendly name of the structure map</b><br> 6713 * Type: <b>string</b><br> 6714 * Path: <b>StructureMap.title</b><br> 6715 * </p> 6716 */ 6717 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 6718 6719 /** 6720 * Search parameter: <b>version</b> 6721 * <p> 6722 * Description: <b>The business version of the structure map</b><br> 6723 * Type: <b>token</b><br> 6724 * Path: <b>StructureMap.version</b><br> 6725 * </p> 6726 */ 6727 @SearchParamDefinition(name="version", path="StructureMap.version", description="The business version of the structure map", type="token" ) 6728 public static final String SP_VERSION = "version"; 6729 /** 6730 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6731 * <p> 6732 * Description: <b>The business version of the structure map</b><br> 6733 * Type: <b>token</b><br> 6734 * Path: <b>StructureMap.version</b><br> 6735 * </p> 6736 */ 6737 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 6738 6739 /** 6740 * Search parameter: <b>url</b> 6741 * <p> 6742 * Description: <b>The uri that identifies the structure map</b><br> 6743 * Type: <b>uri</b><br> 6744 * Path: <b>StructureMap.url</b><br> 6745 * </p> 6746 */ 6747 @SearchParamDefinition(name="url", path="StructureMap.url", description="The uri that identifies the structure map", type="uri" ) 6748 public static final String SP_URL = "url"; 6749 /** 6750 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6751 * <p> 6752 * Description: <b>The uri that identifies the structure map</b><br> 6753 * Type: <b>uri</b><br> 6754 * Path: <b>StructureMap.url</b><br> 6755 * </p> 6756 */ 6757 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6758 6759 /** 6760 * Search parameter: <b>status</b> 6761 * <p> 6762 * Description: <b>The current status of the structure map</b><br> 6763 * Type: <b>token</b><br> 6764 * Path: <b>StructureMap.status</b><br> 6765 * </p> 6766 */ 6767 @SearchParamDefinition(name="status", path="StructureMap.status", description="The current status of the structure map", type="token" ) 6768 public static final String SP_STATUS = "status"; 6769 /** 6770 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6771 * <p> 6772 * Description: <b>The current status of the structure map</b><br> 6773 * Type: <b>token</b><br> 6774 * Path: <b>StructureMap.status</b><br> 6775 * </p> 6776 */ 6777 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 6778 6779// added from java-adornments.txt: 6780 6781 public String toString() { 6782 return StructureMapUtilities.render(this); 6783 } 6784 6785 6786// end addition 6787 6788}