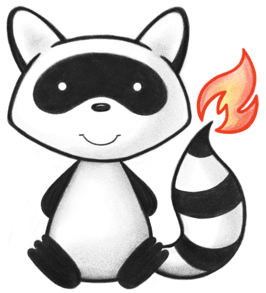
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined "channel" so that another system is able to take an appropriate action. 050 */ 051@ResourceDef(name="Subscription", profile="http://hl7.org/fhir/Profile/Subscription") 052public class Subscription extends DomainResource { 053 054 public enum SubscriptionStatus { 055 /** 056 * The client has requested the subscription, and the server has not yet set it up. 057 */ 058 REQUESTED, 059 /** 060 * The subscription is active. 061 */ 062 ACTIVE, 063 /** 064 * The server has an error executing the notification. 065 */ 066 ERROR, 067 /** 068 * Too many errors have occurred or the subscription has expired. 069 */ 070 OFF, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static SubscriptionStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("requested".equals(codeString)) 079 return REQUESTED; 080 if ("active".equals(codeString)) 081 return ACTIVE; 082 if ("error".equals(codeString)) 083 return ERROR; 084 if ("off".equals(codeString)) 085 return OFF; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown SubscriptionStatus code '"+codeString+"'"); 090 } 091 public String toCode() { 092 switch (this) { 093 case REQUESTED: return "requested"; 094 case ACTIVE: return "active"; 095 case ERROR: return "error"; 096 case OFF: return "off"; 097 case NULL: return null; 098 default: return "?"; 099 } 100 } 101 public String getSystem() { 102 switch (this) { 103 case REQUESTED: return "http://hl7.org/fhir/subscription-status"; 104 case ACTIVE: return "http://hl7.org/fhir/subscription-status"; 105 case ERROR: return "http://hl7.org/fhir/subscription-status"; 106 case OFF: return "http://hl7.org/fhir/subscription-status"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDefinition() { 112 switch (this) { 113 case REQUESTED: return "The client has requested the subscription, and the server has not yet set it up."; 114 case ACTIVE: return "The subscription is active."; 115 case ERROR: return "The server has an error executing the notification."; 116 case OFF: return "Too many errors have occurred or the subscription has expired."; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDisplay() { 122 switch (this) { 123 case REQUESTED: return "Requested"; 124 case ACTIVE: return "Active"; 125 case ERROR: return "Error"; 126 case OFF: return "Off"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 } 132 133 public static class SubscriptionStatusEnumFactory implements EnumFactory<SubscriptionStatus> { 134 public SubscriptionStatus fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("requested".equals(codeString)) 139 return SubscriptionStatus.REQUESTED; 140 if ("active".equals(codeString)) 141 return SubscriptionStatus.ACTIVE; 142 if ("error".equals(codeString)) 143 return SubscriptionStatus.ERROR; 144 if ("off".equals(codeString)) 145 return SubscriptionStatus.OFF; 146 throw new IllegalArgumentException("Unknown SubscriptionStatus code '"+codeString+"'"); 147 } 148 public Enumeration<SubscriptionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 149 if (code == null) 150 return null; 151 if (code.isEmpty()) 152 return new Enumeration<SubscriptionStatus>(this); 153 String codeString = code.asStringValue(); 154 if (codeString == null || "".equals(codeString)) 155 return null; 156 if ("requested".equals(codeString)) 157 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.REQUESTED); 158 if ("active".equals(codeString)) 159 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.ACTIVE); 160 if ("error".equals(codeString)) 161 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.ERROR); 162 if ("off".equals(codeString)) 163 return new Enumeration<SubscriptionStatus>(this, SubscriptionStatus.OFF); 164 throw new FHIRException("Unknown SubscriptionStatus code '"+codeString+"'"); 165 } 166 public String toCode(SubscriptionStatus code) { 167 if (code == SubscriptionStatus.NULL) 168 return null; 169 if (code == SubscriptionStatus.REQUESTED) 170 return "requested"; 171 if (code == SubscriptionStatus.ACTIVE) 172 return "active"; 173 if (code == SubscriptionStatus.ERROR) 174 return "error"; 175 if (code == SubscriptionStatus.OFF) 176 return "off"; 177 return "?"; 178 } 179 public String toSystem(SubscriptionStatus code) { 180 return code.getSystem(); 181 } 182 } 183 184 public enum SubscriptionChannelType { 185 /** 186 * The channel is executed by making a post to the URI. If a payload is included, the URL is interpreted as the service base, and an update (PUT) is made. 187 */ 188 RESTHOOK, 189 /** 190 * The channel is executed by sending a packet across a web socket connection maintained by the client. The URL identifies the websocket, and the client binds to this URL. 191 */ 192 WEBSOCKET, 193 /** 194 * The channel is executed by sending an email to the email addressed in the URI (which must be a mailto:). 195 */ 196 EMAIL, 197 /** 198 * The channel is executed by sending an SMS message to the phone number identified in the URL (tel:). 199 */ 200 SMS, 201 /** 202 * The channel is executed by sending a message (e.g. a Bundle with a MessageHeader resource etc.) to the application identified in the URI. 203 */ 204 MESSAGE, 205 /** 206 * added to help the parsers with the generic types 207 */ 208 NULL; 209 public static SubscriptionChannelType fromCode(String codeString) throws FHIRException { 210 if (codeString == null || "".equals(codeString)) 211 return null; 212 if ("rest-hook".equals(codeString)) 213 return RESTHOOK; 214 if ("websocket".equals(codeString)) 215 return WEBSOCKET; 216 if ("email".equals(codeString)) 217 return EMAIL; 218 if ("sms".equals(codeString)) 219 return SMS; 220 if ("message".equals(codeString)) 221 return MESSAGE; 222 if (Configuration.isAcceptInvalidEnums()) 223 return null; 224 else 225 throw new FHIRException("Unknown SubscriptionChannelType code '"+codeString+"'"); 226 } 227 public String toCode() { 228 switch (this) { 229 case RESTHOOK: return "rest-hook"; 230 case WEBSOCKET: return "websocket"; 231 case EMAIL: return "email"; 232 case SMS: return "sms"; 233 case MESSAGE: return "message"; 234 case NULL: return null; 235 default: return "?"; 236 } 237 } 238 public String getSystem() { 239 switch (this) { 240 case RESTHOOK: return "http://hl7.org/fhir/subscription-channel-type"; 241 case WEBSOCKET: return "http://hl7.org/fhir/subscription-channel-type"; 242 case EMAIL: return "http://hl7.org/fhir/subscription-channel-type"; 243 case SMS: return "http://hl7.org/fhir/subscription-channel-type"; 244 case MESSAGE: return "http://hl7.org/fhir/subscription-channel-type"; 245 case NULL: return null; 246 default: return "?"; 247 } 248 } 249 public String getDefinition() { 250 switch (this) { 251 case RESTHOOK: return "The channel is executed by making a post to the URI. If a payload is included, the URL is interpreted as the service base, and an update (PUT) is made."; 252 case WEBSOCKET: return "The channel is executed by sending a packet across a web socket connection maintained by the client. The URL identifies the websocket, and the client binds to this URL."; 253 case EMAIL: return "The channel is executed by sending an email to the email addressed in the URI (which must be a mailto:)."; 254 case SMS: return "The channel is executed by sending an SMS message to the phone number identified in the URL (tel:)."; 255 case MESSAGE: return "The channel is executed by sending a message (e.g. a Bundle with a MessageHeader resource etc.) to the application identified in the URI."; 256 case NULL: return null; 257 default: return "?"; 258 } 259 } 260 public String getDisplay() { 261 switch (this) { 262 case RESTHOOK: return "Rest Hook"; 263 case WEBSOCKET: return "Websocket"; 264 case EMAIL: return "Email"; 265 case SMS: return "SMS"; 266 case MESSAGE: return "Message"; 267 case NULL: return null; 268 default: return "?"; 269 } 270 } 271 } 272 273 public static class SubscriptionChannelTypeEnumFactory implements EnumFactory<SubscriptionChannelType> { 274 public SubscriptionChannelType fromCode(String codeString) throws IllegalArgumentException { 275 if (codeString == null || "".equals(codeString)) 276 if (codeString == null || "".equals(codeString)) 277 return null; 278 if ("rest-hook".equals(codeString)) 279 return SubscriptionChannelType.RESTHOOK; 280 if ("websocket".equals(codeString)) 281 return SubscriptionChannelType.WEBSOCKET; 282 if ("email".equals(codeString)) 283 return SubscriptionChannelType.EMAIL; 284 if ("sms".equals(codeString)) 285 return SubscriptionChannelType.SMS; 286 if ("message".equals(codeString)) 287 return SubscriptionChannelType.MESSAGE; 288 throw new IllegalArgumentException("Unknown SubscriptionChannelType code '"+codeString+"'"); 289 } 290 public Enumeration<SubscriptionChannelType> fromType(PrimitiveType<?> code) throws FHIRException { 291 if (code == null) 292 return null; 293 if (code.isEmpty()) 294 return new Enumeration<SubscriptionChannelType>(this); 295 String codeString = code.asStringValue(); 296 if (codeString == null || "".equals(codeString)) 297 return null; 298 if ("rest-hook".equals(codeString)) 299 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.RESTHOOK); 300 if ("websocket".equals(codeString)) 301 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.WEBSOCKET); 302 if ("email".equals(codeString)) 303 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.EMAIL); 304 if ("sms".equals(codeString)) 305 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.SMS); 306 if ("message".equals(codeString)) 307 return new Enumeration<SubscriptionChannelType>(this, SubscriptionChannelType.MESSAGE); 308 throw new FHIRException("Unknown SubscriptionChannelType code '"+codeString+"'"); 309 } 310 public String toCode(SubscriptionChannelType code) { 311 if (code == SubscriptionChannelType.NULL) 312 return null; 313 if (code == SubscriptionChannelType.RESTHOOK) 314 return "rest-hook"; 315 if (code == SubscriptionChannelType.WEBSOCKET) 316 return "websocket"; 317 if (code == SubscriptionChannelType.EMAIL) 318 return "email"; 319 if (code == SubscriptionChannelType.SMS) 320 return "sms"; 321 if (code == SubscriptionChannelType.MESSAGE) 322 return "message"; 323 return "?"; 324 } 325 public String toSystem(SubscriptionChannelType code) { 326 return code.getSystem(); 327 } 328 } 329 330 @Block() 331 public static class SubscriptionChannelComponent extends BackboneElement implements IBaseBackboneElement { 332 /** 333 * The type of channel to send notifications on. 334 */ 335 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 336 @Description(shortDefinition="rest-hook | websocket | email | sms | message", formalDefinition="The type of channel to send notifications on." ) 337 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-channel-type") 338 protected Enumeration<SubscriptionChannelType> type; 339 340 /** 341 * The uri that describes the actual end-point to send messages to. 342 */ 343 @Child(name = "endpoint", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 344 @Description(shortDefinition="Where the channel points to", formalDefinition="The uri that describes the actual end-point to send messages to." ) 345 protected UriType endpoint; 346 347 /** 348 * The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification. 349 */ 350 @Child(name = "payload", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 351 @Description(shortDefinition="Mimetype to send, or omit for no payload", formalDefinition="The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification." ) 352 protected StringType payload; 353 354 /** 355 * Additional headers / information to send as part of the notification. 356 */ 357 @Child(name = "header", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 358 @Description(shortDefinition="Usage depends on the channel type", formalDefinition="Additional headers / information to send as part of the notification." ) 359 protected List<StringType> header; 360 361 private static final long serialVersionUID = -854610293L; 362 363 /** 364 * Constructor 365 */ 366 public SubscriptionChannelComponent() { 367 super(); 368 } 369 370 /** 371 * Constructor 372 */ 373 public SubscriptionChannelComponent(Enumeration<SubscriptionChannelType> type) { 374 super(); 375 this.type = type; 376 } 377 378 /** 379 * @return {@link #type} (The type of channel to send notifications on.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 380 */ 381 public Enumeration<SubscriptionChannelType> getTypeElement() { 382 if (this.type == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create SubscriptionChannelComponent.type"); 385 else if (Configuration.doAutoCreate()) 386 this.type = new Enumeration<SubscriptionChannelType>(new SubscriptionChannelTypeEnumFactory()); // bb 387 return this.type; 388 } 389 390 public boolean hasTypeElement() { 391 return this.type != null && !this.type.isEmpty(); 392 } 393 394 public boolean hasType() { 395 return this.type != null && !this.type.isEmpty(); 396 } 397 398 /** 399 * @param value {@link #type} (The type of channel to send notifications on.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 400 */ 401 public SubscriptionChannelComponent setTypeElement(Enumeration<SubscriptionChannelType> value) { 402 this.type = value; 403 return this; 404 } 405 406 /** 407 * @return The type of channel to send notifications on. 408 */ 409 public SubscriptionChannelType getType() { 410 return this.type == null ? null : this.type.getValue(); 411 } 412 413 /** 414 * @param value The type of channel to send notifications on. 415 */ 416 public SubscriptionChannelComponent setType(SubscriptionChannelType value) { 417 if (this.type == null) 418 this.type = new Enumeration<SubscriptionChannelType>(new SubscriptionChannelTypeEnumFactory()); 419 this.type.setValue(value); 420 return this; 421 } 422 423 /** 424 * @return {@link #endpoint} (The uri that describes the actual end-point to send messages to.). This is the underlying object with id, value and extensions. The accessor "getEndpoint" gives direct access to the value 425 */ 426 public UriType getEndpointElement() { 427 if (this.endpoint == null) 428 if (Configuration.errorOnAutoCreate()) 429 throw new Error("Attempt to auto-create SubscriptionChannelComponent.endpoint"); 430 else if (Configuration.doAutoCreate()) 431 this.endpoint = new UriType(); // bb 432 return this.endpoint; 433 } 434 435 public boolean hasEndpointElement() { 436 return this.endpoint != null && !this.endpoint.isEmpty(); 437 } 438 439 public boolean hasEndpoint() { 440 return this.endpoint != null && !this.endpoint.isEmpty(); 441 } 442 443 /** 444 * @param value {@link #endpoint} (The uri that describes the actual end-point to send messages to.). This is the underlying object with id, value and extensions. The accessor "getEndpoint" gives direct access to the value 445 */ 446 public SubscriptionChannelComponent setEndpointElement(UriType value) { 447 this.endpoint = value; 448 return this; 449 } 450 451 /** 452 * @return The uri that describes the actual end-point to send messages to. 453 */ 454 public String getEndpoint() { 455 return this.endpoint == null ? null : this.endpoint.getValue(); 456 } 457 458 /** 459 * @param value The uri that describes the actual end-point to send messages to. 460 */ 461 public SubscriptionChannelComponent setEndpoint(String value) { 462 if (Utilities.noString(value)) 463 this.endpoint = null; 464 else { 465 if (this.endpoint == null) 466 this.endpoint = new UriType(); 467 this.endpoint.setValue(value); 468 } 469 return this; 470 } 471 472 /** 473 * @return {@link #payload} (The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification.). This is the underlying object with id, value and extensions. The accessor "getPayload" gives direct access to the value 474 */ 475 public StringType getPayloadElement() { 476 if (this.payload == null) 477 if (Configuration.errorOnAutoCreate()) 478 throw new Error("Attempt to auto-create SubscriptionChannelComponent.payload"); 479 else if (Configuration.doAutoCreate()) 480 this.payload = new StringType(); // bb 481 return this.payload; 482 } 483 484 public boolean hasPayloadElement() { 485 return this.payload != null && !this.payload.isEmpty(); 486 } 487 488 public boolean hasPayload() { 489 return this.payload != null && !this.payload.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #payload} (The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification.). This is the underlying object with id, value and extensions. The accessor "getPayload" gives direct access to the value 494 */ 495 public SubscriptionChannelComponent setPayloadElement(StringType value) { 496 this.payload = value; 497 return this; 498 } 499 500 /** 501 * @return The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification. 502 */ 503 public String getPayload() { 504 return this.payload == null ? null : this.payload.getValue(); 505 } 506 507 /** 508 * @param value The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification. 509 */ 510 public SubscriptionChannelComponent setPayload(String value) { 511 if (Utilities.noString(value)) 512 this.payload = null; 513 else { 514 if (this.payload == null) 515 this.payload = new StringType(); 516 this.payload.setValue(value); 517 } 518 return this; 519 } 520 521 /** 522 * @return {@link #header} (Additional headers / information to send as part of the notification.) 523 */ 524 public List<StringType> getHeader() { 525 if (this.header == null) 526 this.header = new ArrayList<StringType>(); 527 return this.header; 528 } 529 530 /** 531 * @return Returns a reference to <code>this</code> for easy method chaining 532 */ 533 public SubscriptionChannelComponent setHeader(List<StringType> theHeader) { 534 this.header = theHeader; 535 return this; 536 } 537 538 public boolean hasHeader() { 539 if (this.header == null) 540 return false; 541 for (StringType item : this.header) 542 if (!item.isEmpty()) 543 return true; 544 return false; 545 } 546 547 /** 548 * @return {@link #header} (Additional headers / information to send as part of the notification.) 549 */ 550 public StringType addHeaderElement() {//2 551 StringType t = new StringType(); 552 if (this.header == null) 553 this.header = new ArrayList<StringType>(); 554 this.header.add(t); 555 return t; 556 } 557 558 /** 559 * @param value {@link #header} (Additional headers / information to send as part of the notification.) 560 */ 561 public SubscriptionChannelComponent addHeader(String value) { //1 562 StringType t = new StringType(); 563 t.setValue(value); 564 if (this.header == null) 565 this.header = new ArrayList<StringType>(); 566 this.header.add(t); 567 return this; 568 } 569 570 /** 571 * @param value {@link #header} (Additional headers / information to send as part of the notification.) 572 */ 573 public boolean hasHeader(String value) { 574 if (this.header == null) 575 return false; 576 for (StringType v : this.header) 577 if (v.getValue().equals(value)) // string 578 return true; 579 return false; 580 } 581 582 protected void listChildren(List<Property> children) { 583 super.listChildren(children); 584 children.add(new Property("type", "code", "The type of channel to send notifications on.", 0, 1, type)); 585 children.add(new Property("endpoint", "uri", "The uri that describes the actual end-point to send messages to.", 0, 1, endpoint)); 586 children.add(new Property("payload", "string", "The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification.", 0, 1, payload)); 587 children.add(new Property("header", "string", "Additional headers / information to send as part of the notification.", 0, java.lang.Integer.MAX_VALUE, header)); 588 } 589 590 @Override 591 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 592 switch (_hash) { 593 case 3575610: /*type*/ return new Property("type", "code", "The type of channel to send notifications on.", 0, 1, type); 594 case 1741102485: /*endpoint*/ return new Property("endpoint", "uri", "The uri that describes the actual end-point to send messages to.", 0, 1, endpoint); 595 case -786701938: /*payload*/ return new Property("payload", "string", "The mime type to send the payload in - either application/fhir+xml, or application/fhir+json. If the payload is not present, then there is no payload in the notification, just a notification.", 0, 1, payload); 596 case -1221270899: /*header*/ return new Property("header", "string", "Additional headers / information to send as part of the notification.", 0, java.lang.Integer.MAX_VALUE, header); 597 default: return super.getNamedProperty(_hash, _name, _checkValid); 598 } 599 600 } 601 602 @Override 603 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 604 switch (hash) { 605 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<SubscriptionChannelType> 606 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : new Base[] {this.endpoint}; // UriType 607 case -786701938: /*payload*/ return this.payload == null ? new Base[0] : new Base[] {this.payload}; // StringType 608 case -1221270899: /*header*/ return this.header == null ? new Base[0] : this.header.toArray(new Base[this.header.size()]); // StringType 609 default: return super.getProperty(hash, name, checkValid); 610 } 611 612 } 613 614 @Override 615 public Base setProperty(int hash, String name, Base value) throws FHIRException { 616 switch (hash) { 617 case 3575610: // type 618 value = new SubscriptionChannelTypeEnumFactory().fromType(castToCode(value)); 619 this.type = (Enumeration) value; // Enumeration<SubscriptionChannelType> 620 return value; 621 case 1741102485: // endpoint 622 this.endpoint = castToUri(value); // UriType 623 return value; 624 case -786701938: // payload 625 this.payload = castToString(value); // StringType 626 return value; 627 case -1221270899: // header 628 this.getHeader().add(castToString(value)); // StringType 629 return value; 630 default: return super.setProperty(hash, name, value); 631 } 632 633 } 634 635 @Override 636 public Base setProperty(String name, Base value) throws FHIRException { 637 if (name.equals("type")) { 638 value = new SubscriptionChannelTypeEnumFactory().fromType(castToCode(value)); 639 this.type = (Enumeration) value; // Enumeration<SubscriptionChannelType> 640 } else if (name.equals("endpoint")) { 641 this.endpoint = castToUri(value); // UriType 642 } else if (name.equals("payload")) { 643 this.payload = castToString(value); // StringType 644 } else if (name.equals("header")) { 645 this.getHeader().add(castToString(value)); 646 } else 647 return super.setProperty(name, value); 648 return value; 649 } 650 651 @Override 652 public Base makeProperty(int hash, String name) throws FHIRException { 653 switch (hash) { 654 case 3575610: return getTypeElement(); 655 case 1741102485: return getEndpointElement(); 656 case -786701938: return getPayloadElement(); 657 case -1221270899: return addHeaderElement(); 658 default: return super.makeProperty(hash, name); 659 } 660 661 } 662 663 @Override 664 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 665 switch (hash) { 666 case 3575610: /*type*/ return new String[] {"code"}; 667 case 1741102485: /*endpoint*/ return new String[] {"uri"}; 668 case -786701938: /*payload*/ return new String[] {"string"}; 669 case -1221270899: /*header*/ return new String[] {"string"}; 670 default: return super.getTypesForProperty(hash, name); 671 } 672 673 } 674 675 @Override 676 public Base addChild(String name) throws FHIRException { 677 if (name.equals("type")) { 678 throw new FHIRException("Cannot call addChild on a singleton property Subscription.type"); 679 } 680 else if (name.equals("endpoint")) { 681 throw new FHIRException("Cannot call addChild on a singleton property Subscription.endpoint"); 682 } 683 else if (name.equals("payload")) { 684 throw new FHIRException("Cannot call addChild on a singleton property Subscription.payload"); 685 } 686 else if (name.equals("header")) { 687 throw new FHIRException("Cannot call addChild on a singleton property Subscription.header"); 688 } 689 else 690 return super.addChild(name); 691 } 692 693 public SubscriptionChannelComponent copy() { 694 SubscriptionChannelComponent dst = new SubscriptionChannelComponent(); 695 copyValues(dst); 696 dst.type = type == null ? null : type.copy(); 697 dst.endpoint = endpoint == null ? null : endpoint.copy(); 698 dst.payload = payload == null ? null : payload.copy(); 699 if (header != null) { 700 dst.header = new ArrayList<StringType>(); 701 for (StringType i : header) 702 dst.header.add(i.copy()); 703 }; 704 return dst; 705 } 706 707 @Override 708 public boolean equalsDeep(Base other_) { 709 if (!super.equalsDeep(other_)) 710 return false; 711 if (!(other_ instanceof SubscriptionChannelComponent)) 712 return false; 713 SubscriptionChannelComponent o = (SubscriptionChannelComponent) other_; 714 return compareDeep(type, o.type, true) && compareDeep(endpoint, o.endpoint, true) && compareDeep(payload, o.payload, true) 715 && compareDeep(header, o.header, true); 716 } 717 718 @Override 719 public boolean equalsShallow(Base other_) { 720 if (!super.equalsShallow(other_)) 721 return false; 722 if (!(other_ instanceof SubscriptionChannelComponent)) 723 return false; 724 SubscriptionChannelComponent o = (SubscriptionChannelComponent) other_; 725 return compareValues(type, o.type, true) && compareValues(endpoint, o.endpoint, true) && compareValues(payload, o.payload, true) 726 && compareValues(header, o.header, true); 727 } 728 729 public boolean isEmpty() { 730 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, endpoint, payload 731 , header); 732 } 733 734 public String fhirType() { 735 return "Subscription.channel"; 736 737 } 738 739 } 740 741 /** 742 * The status of the subscription, which marks the server state for managing the subscription. 743 */ 744 @Child(name = "status", type = {CodeType.class}, order=0, min=1, max=1, modifier=true, summary=true) 745 @Description(shortDefinition="requested | active | error | off", formalDefinition="The status of the subscription, which marks the server state for managing the subscription." ) 746 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-status") 747 protected Enumeration<SubscriptionStatus> status; 748 749 /** 750 * Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting. 751 */ 752 @Child(name = "contact", type = {ContactPoint.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 753 @Description(shortDefinition="Contact details for source (e.g. troubleshooting)", formalDefinition="Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting." ) 754 protected List<ContactPoint> contact; 755 756 /** 757 * The time for the server to turn the subscription off. 758 */ 759 @Child(name = "end", type = {InstantType.class}, order=2, min=0, max=1, modifier=false, summary=true) 760 @Description(shortDefinition="When to automatically delete the subscription", formalDefinition="The time for the server to turn the subscription off." ) 761 protected InstantType end; 762 763 /** 764 * A description of why this subscription is defined. 765 */ 766 @Child(name = "reason", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 767 @Description(shortDefinition="Description of why this subscription was created", formalDefinition="A description of why this subscription is defined." ) 768 protected StringType reason; 769 770 /** 771 * The rules that the server should use to determine when to generate notifications for this subscription. 772 */ 773 @Child(name = "criteria", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 774 @Description(shortDefinition="Rule for server push criteria", formalDefinition="The rules that the server should use to determine when to generate notifications for this subscription." ) 775 protected StringType criteria; 776 777 /** 778 * A record of the last error that occurred when the server processed a notification. 779 */ 780 @Child(name = "error", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 781 @Description(shortDefinition="Latest error note", formalDefinition="A record of the last error that occurred when the server processed a notification." ) 782 protected StringType error; 783 784 /** 785 * Details where to send notifications when resources are received that meet the criteria. 786 */ 787 @Child(name = "channel", type = {}, order=6, min=1, max=1, modifier=false, summary=true) 788 @Description(shortDefinition="The channel on which to report matches to the criteria", formalDefinition="Details where to send notifications when resources are received that meet the criteria." ) 789 protected SubscriptionChannelComponent channel; 790 791 /** 792 * A tag to add to any resource that matches the criteria, after the subscription is processed. 793 */ 794 @Child(name = "tag", type = {Coding.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 795 @Description(shortDefinition="A tag to add to matching resources", formalDefinition="A tag to add to any resource that matches the criteria, after the subscription is processed." ) 796 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/subscription-tag") 797 protected List<Coding> tag; 798 799 private static final long serialVersionUID = 99986202L; 800 801 /** 802 * Constructor 803 */ 804 public Subscription() { 805 super(); 806 } 807 808 /** 809 * Constructor 810 */ 811 public Subscription(Enumeration<SubscriptionStatus> status, StringType reason, StringType criteria, SubscriptionChannelComponent channel) { 812 super(); 813 this.status = status; 814 this.reason = reason; 815 this.criteria = criteria; 816 this.channel = channel; 817 } 818 819 /** 820 * @return {@link #status} (The status of the subscription, which marks the server state for managing the subscription.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 821 */ 822 public Enumeration<SubscriptionStatus> getStatusElement() { 823 if (this.status == null) 824 if (Configuration.errorOnAutoCreate()) 825 throw new Error("Attempt to auto-create Subscription.status"); 826 else if (Configuration.doAutoCreate()) 827 this.status = new Enumeration<SubscriptionStatus>(new SubscriptionStatusEnumFactory()); // bb 828 return this.status; 829 } 830 831 public boolean hasStatusElement() { 832 return this.status != null && !this.status.isEmpty(); 833 } 834 835 public boolean hasStatus() { 836 return this.status != null && !this.status.isEmpty(); 837 } 838 839 /** 840 * @param value {@link #status} (The status of the subscription, which marks the server state for managing the subscription.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 841 */ 842 public Subscription setStatusElement(Enumeration<SubscriptionStatus> value) { 843 this.status = value; 844 return this; 845 } 846 847 /** 848 * @return The status of the subscription, which marks the server state for managing the subscription. 849 */ 850 public SubscriptionStatus getStatus() { 851 return this.status == null ? null : this.status.getValue(); 852 } 853 854 /** 855 * @param value The status of the subscription, which marks the server state for managing the subscription. 856 */ 857 public Subscription setStatus(SubscriptionStatus value) { 858 if (this.status == null) 859 this.status = new Enumeration<SubscriptionStatus>(new SubscriptionStatusEnumFactory()); 860 this.status.setValue(value); 861 return this; 862 } 863 864 /** 865 * @return {@link #contact} (Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.) 866 */ 867 public List<ContactPoint> getContact() { 868 if (this.contact == null) 869 this.contact = new ArrayList<ContactPoint>(); 870 return this.contact; 871 } 872 873 /** 874 * @return Returns a reference to <code>this</code> for easy method chaining 875 */ 876 public Subscription setContact(List<ContactPoint> theContact) { 877 this.contact = theContact; 878 return this; 879 } 880 881 public boolean hasContact() { 882 if (this.contact == null) 883 return false; 884 for (ContactPoint item : this.contact) 885 if (!item.isEmpty()) 886 return true; 887 return false; 888 } 889 890 public ContactPoint addContact() { //3 891 ContactPoint t = new ContactPoint(); 892 if (this.contact == null) 893 this.contact = new ArrayList<ContactPoint>(); 894 this.contact.add(t); 895 return t; 896 } 897 898 public Subscription addContact(ContactPoint t) { //3 899 if (t == null) 900 return this; 901 if (this.contact == null) 902 this.contact = new ArrayList<ContactPoint>(); 903 this.contact.add(t); 904 return this; 905 } 906 907 /** 908 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 909 */ 910 public ContactPoint getContactFirstRep() { 911 if (getContact().isEmpty()) { 912 addContact(); 913 } 914 return getContact().get(0); 915 } 916 917 /** 918 * @return {@link #end} (The time for the server to turn the subscription off.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 919 */ 920 public InstantType getEndElement() { 921 if (this.end == null) 922 if (Configuration.errorOnAutoCreate()) 923 throw new Error("Attempt to auto-create Subscription.end"); 924 else if (Configuration.doAutoCreate()) 925 this.end = new InstantType(); // bb 926 return this.end; 927 } 928 929 public boolean hasEndElement() { 930 return this.end != null && !this.end.isEmpty(); 931 } 932 933 public boolean hasEnd() { 934 return this.end != null && !this.end.isEmpty(); 935 } 936 937 /** 938 * @param value {@link #end} (The time for the server to turn the subscription off.). This is the underlying object with id, value and extensions. The accessor "getEnd" gives direct access to the value 939 */ 940 public Subscription setEndElement(InstantType value) { 941 this.end = value; 942 return this; 943 } 944 945 /** 946 * @return The time for the server to turn the subscription off. 947 */ 948 public Date getEnd() { 949 return this.end == null ? null : this.end.getValue(); 950 } 951 952 /** 953 * @param value The time for the server to turn the subscription off. 954 */ 955 public Subscription setEnd(Date value) { 956 if (value == null) 957 this.end = null; 958 else { 959 if (this.end == null) 960 this.end = new InstantType(); 961 this.end.setValue(value); 962 } 963 return this; 964 } 965 966 /** 967 * @return {@link #reason} (A description of why this subscription is defined.). This is the underlying object with id, value and extensions. The accessor "getReason" gives direct access to the value 968 */ 969 public StringType getReasonElement() { 970 if (this.reason == null) 971 if (Configuration.errorOnAutoCreate()) 972 throw new Error("Attempt to auto-create Subscription.reason"); 973 else if (Configuration.doAutoCreate()) 974 this.reason = new StringType(); // bb 975 return this.reason; 976 } 977 978 public boolean hasReasonElement() { 979 return this.reason != null && !this.reason.isEmpty(); 980 } 981 982 public boolean hasReason() { 983 return this.reason != null && !this.reason.isEmpty(); 984 } 985 986 /** 987 * @param value {@link #reason} (A description of why this subscription is defined.). This is the underlying object with id, value and extensions. The accessor "getReason" gives direct access to the value 988 */ 989 public Subscription setReasonElement(StringType value) { 990 this.reason = value; 991 return this; 992 } 993 994 /** 995 * @return A description of why this subscription is defined. 996 */ 997 public String getReason() { 998 return this.reason == null ? null : this.reason.getValue(); 999 } 1000 1001 /** 1002 * @param value A description of why this subscription is defined. 1003 */ 1004 public Subscription setReason(String value) { 1005 if (this.reason == null) 1006 this.reason = new StringType(); 1007 this.reason.setValue(value); 1008 return this; 1009 } 1010 1011 /** 1012 * @return {@link #criteria} (The rules that the server should use to determine when to generate notifications for this subscription.). This is the underlying object with id, value and extensions. The accessor "getCriteria" gives direct access to the value 1013 */ 1014 public StringType getCriteriaElement() { 1015 if (this.criteria == null) 1016 if (Configuration.errorOnAutoCreate()) 1017 throw new Error("Attempt to auto-create Subscription.criteria"); 1018 else if (Configuration.doAutoCreate()) 1019 this.criteria = new StringType(); // bb 1020 return this.criteria; 1021 } 1022 1023 public boolean hasCriteriaElement() { 1024 return this.criteria != null && !this.criteria.isEmpty(); 1025 } 1026 1027 public boolean hasCriteria() { 1028 return this.criteria != null && !this.criteria.isEmpty(); 1029 } 1030 1031 /** 1032 * @param value {@link #criteria} (The rules that the server should use to determine when to generate notifications for this subscription.). This is the underlying object with id, value and extensions. The accessor "getCriteria" gives direct access to the value 1033 */ 1034 public Subscription setCriteriaElement(StringType value) { 1035 this.criteria = value; 1036 return this; 1037 } 1038 1039 /** 1040 * @return The rules that the server should use to determine when to generate notifications for this subscription. 1041 */ 1042 public String getCriteria() { 1043 return this.criteria == null ? null : this.criteria.getValue(); 1044 } 1045 1046 /** 1047 * @param value The rules that the server should use to determine when to generate notifications for this subscription. 1048 */ 1049 public Subscription setCriteria(String value) { 1050 if (this.criteria == null) 1051 this.criteria = new StringType(); 1052 this.criteria.setValue(value); 1053 return this; 1054 } 1055 1056 /** 1057 * @return {@link #error} (A record of the last error that occurred when the server processed a notification.). This is the underlying object with id, value and extensions. The accessor "getError" gives direct access to the value 1058 */ 1059 public StringType getErrorElement() { 1060 if (this.error == null) 1061 if (Configuration.errorOnAutoCreate()) 1062 throw new Error("Attempt to auto-create Subscription.error"); 1063 else if (Configuration.doAutoCreate()) 1064 this.error = new StringType(); // bb 1065 return this.error; 1066 } 1067 1068 public boolean hasErrorElement() { 1069 return this.error != null && !this.error.isEmpty(); 1070 } 1071 1072 public boolean hasError() { 1073 return this.error != null && !this.error.isEmpty(); 1074 } 1075 1076 /** 1077 * @param value {@link #error} (A record of the last error that occurred when the server processed a notification.). This is the underlying object with id, value and extensions. The accessor "getError" gives direct access to the value 1078 */ 1079 public Subscription setErrorElement(StringType value) { 1080 this.error = value; 1081 return this; 1082 } 1083 1084 /** 1085 * @return A record of the last error that occurred when the server processed a notification. 1086 */ 1087 public String getError() { 1088 return this.error == null ? null : this.error.getValue(); 1089 } 1090 1091 /** 1092 * @param value A record of the last error that occurred when the server processed a notification. 1093 */ 1094 public Subscription setError(String value) { 1095 if (Utilities.noString(value)) 1096 this.error = null; 1097 else { 1098 if (this.error == null) 1099 this.error = new StringType(); 1100 this.error.setValue(value); 1101 } 1102 return this; 1103 } 1104 1105 /** 1106 * @return {@link #channel} (Details where to send notifications when resources are received that meet the criteria.) 1107 */ 1108 public SubscriptionChannelComponent getChannel() { 1109 if (this.channel == null) 1110 if (Configuration.errorOnAutoCreate()) 1111 throw new Error("Attempt to auto-create Subscription.channel"); 1112 else if (Configuration.doAutoCreate()) 1113 this.channel = new SubscriptionChannelComponent(); // cc 1114 return this.channel; 1115 } 1116 1117 public boolean hasChannel() { 1118 return this.channel != null && !this.channel.isEmpty(); 1119 } 1120 1121 /** 1122 * @param value {@link #channel} (Details where to send notifications when resources are received that meet the criteria.) 1123 */ 1124 public Subscription setChannel(SubscriptionChannelComponent value) { 1125 this.channel = value; 1126 return this; 1127 } 1128 1129 /** 1130 * @return {@link #tag} (A tag to add to any resource that matches the criteria, after the subscription is processed.) 1131 */ 1132 public List<Coding> getTag() { 1133 if (this.tag == null) 1134 this.tag = new ArrayList<Coding>(); 1135 return this.tag; 1136 } 1137 1138 /** 1139 * @return Returns a reference to <code>this</code> for easy method chaining 1140 */ 1141 public Subscription setTag(List<Coding> theTag) { 1142 this.tag = theTag; 1143 return this; 1144 } 1145 1146 public boolean hasTag() { 1147 if (this.tag == null) 1148 return false; 1149 for (Coding item : this.tag) 1150 if (!item.isEmpty()) 1151 return true; 1152 return false; 1153 } 1154 1155 public Coding addTag() { //3 1156 Coding t = new Coding(); 1157 if (this.tag == null) 1158 this.tag = new ArrayList<Coding>(); 1159 this.tag.add(t); 1160 return t; 1161 } 1162 1163 public Subscription addTag(Coding t) { //3 1164 if (t == null) 1165 return this; 1166 if (this.tag == null) 1167 this.tag = new ArrayList<Coding>(); 1168 this.tag.add(t); 1169 return this; 1170 } 1171 1172 /** 1173 * @return The first repetition of repeating field {@link #tag}, creating it if it does not already exist 1174 */ 1175 public Coding getTagFirstRep() { 1176 if (getTag().isEmpty()) { 1177 addTag(); 1178 } 1179 return getTag().get(0); 1180 } 1181 1182 protected void listChildren(List<Property> children) { 1183 super.listChildren(children); 1184 children.add(new Property("status", "code", "The status of the subscription, which marks the server state for managing the subscription.", 0, 1, status)); 1185 children.add(new Property("contact", "ContactPoint", "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 0, java.lang.Integer.MAX_VALUE, contact)); 1186 children.add(new Property("end", "instant", "The time for the server to turn the subscription off.", 0, 1, end)); 1187 children.add(new Property("reason", "string", "A description of why this subscription is defined.", 0, 1, reason)); 1188 children.add(new Property("criteria", "string", "The rules that the server should use to determine when to generate notifications for this subscription.", 0, 1, criteria)); 1189 children.add(new Property("error", "string", "A record of the last error that occurred when the server processed a notification.", 0, 1, error)); 1190 children.add(new Property("channel", "", "Details where to send notifications when resources are received that meet the criteria.", 0, 1, channel)); 1191 children.add(new Property("tag", "Coding", "A tag to add to any resource that matches the criteria, after the subscription is processed.", 0, java.lang.Integer.MAX_VALUE, tag)); 1192 } 1193 1194 @Override 1195 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1196 switch (_hash) { 1197 case -892481550: /*status*/ return new Property("status", "code", "The status of the subscription, which marks the server state for managing the subscription.", 0, 1, status); 1198 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 0, java.lang.Integer.MAX_VALUE, contact); 1199 case 100571: /*end*/ return new Property("end", "instant", "The time for the server to turn the subscription off.", 0, 1, end); 1200 case -934964668: /*reason*/ return new Property("reason", "string", "A description of why this subscription is defined.", 0, 1, reason); 1201 case 1952046943: /*criteria*/ return new Property("criteria", "string", "The rules that the server should use to determine when to generate notifications for this subscription.", 0, 1, criteria); 1202 case 96784904: /*error*/ return new Property("error", "string", "A record of the last error that occurred when the server processed a notification.", 0, 1, error); 1203 case 738950403: /*channel*/ return new Property("channel", "", "Details where to send notifications when resources are received that meet the criteria.", 0, 1, channel); 1204 case 114586: /*tag*/ return new Property("tag", "Coding", "A tag to add to any resource that matches the criteria, after the subscription is processed.", 0, java.lang.Integer.MAX_VALUE, tag); 1205 default: return super.getNamedProperty(_hash, _name, _checkValid); 1206 } 1207 1208 } 1209 1210 @Override 1211 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1212 switch (hash) { 1213 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SubscriptionStatus> 1214 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 1215 case 100571: /*end*/ return this.end == null ? new Base[0] : new Base[] {this.end}; // InstantType 1216 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // StringType 1217 case 1952046943: /*criteria*/ return this.criteria == null ? new Base[0] : new Base[] {this.criteria}; // StringType 1218 case 96784904: /*error*/ return this.error == null ? new Base[0] : new Base[] {this.error}; // StringType 1219 case 738950403: /*channel*/ return this.channel == null ? new Base[0] : new Base[] {this.channel}; // SubscriptionChannelComponent 1220 case 114586: /*tag*/ return this.tag == null ? new Base[0] : this.tag.toArray(new Base[this.tag.size()]); // Coding 1221 default: return super.getProperty(hash, name, checkValid); 1222 } 1223 1224 } 1225 1226 @Override 1227 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1228 switch (hash) { 1229 case -892481550: // status 1230 value = new SubscriptionStatusEnumFactory().fromType(castToCode(value)); 1231 this.status = (Enumeration) value; // Enumeration<SubscriptionStatus> 1232 return value; 1233 case 951526432: // contact 1234 this.getContact().add(castToContactPoint(value)); // ContactPoint 1235 return value; 1236 case 100571: // end 1237 this.end = castToInstant(value); // InstantType 1238 return value; 1239 case -934964668: // reason 1240 this.reason = castToString(value); // StringType 1241 return value; 1242 case 1952046943: // criteria 1243 this.criteria = castToString(value); // StringType 1244 return value; 1245 case 96784904: // error 1246 this.error = castToString(value); // StringType 1247 return value; 1248 case 738950403: // channel 1249 this.channel = (SubscriptionChannelComponent) value; // SubscriptionChannelComponent 1250 return value; 1251 case 114586: // tag 1252 this.getTag().add(castToCoding(value)); // Coding 1253 return value; 1254 default: return super.setProperty(hash, name, value); 1255 } 1256 1257 } 1258 1259 @Override 1260 public Base setProperty(String name, Base value) throws FHIRException { 1261 if (name.equals("status")) { 1262 value = new SubscriptionStatusEnumFactory().fromType(castToCode(value)); 1263 this.status = (Enumeration) value; // Enumeration<SubscriptionStatus> 1264 } else if (name.equals("contact")) { 1265 this.getContact().add(castToContactPoint(value)); 1266 } else if (name.equals("end")) { 1267 this.end = castToInstant(value); // InstantType 1268 } else if (name.equals("reason")) { 1269 this.reason = castToString(value); // StringType 1270 } else if (name.equals("criteria")) { 1271 this.criteria = castToString(value); // StringType 1272 } else if (name.equals("error")) { 1273 this.error = castToString(value); // StringType 1274 } else if (name.equals("channel")) { 1275 this.channel = (SubscriptionChannelComponent) value; // SubscriptionChannelComponent 1276 } else if (name.equals("tag")) { 1277 this.getTag().add(castToCoding(value)); 1278 } else 1279 return super.setProperty(name, value); 1280 return value; 1281 } 1282 1283 @Override 1284 public Base makeProperty(int hash, String name) throws FHIRException { 1285 switch (hash) { 1286 case -892481550: return getStatusElement(); 1287 case 951526432: return addContact(); 1288 case 100571: return getEndElement(); 1289 case -934964668: return getReasonElement(); 1290 case 1952046943: return getCriteriaElement(); 1291 case 96784904: return getErrorElement(); 1292 case 738950403: return getChannel(); 1293 case 114586: return addTag(); 1294 default: return super.makeProperty(hash, name); 1295 } 1296 1297 } 1298 1299 @Override 1300 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1301 switch (hash) { 1302 case -892481550: /*status*/ return new String[] {"code"}; 1303 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 1304 case 100571: /*end*/ return new String[] {"instant"}; 1305 case -934964668: /*reason*/ return new String[] {"string"}; 1306 case 1952046943: /*criteria*/ return new String[] {"string"}; 1307 case 96784904: /*error*/ return new String[] {"string"}; 1308 case 738950403: /*channel*/ return new String[] {}; 1309 case 114586: /*tag*/ return new String[] {"Coding"}; 1310 default: return super.getTypesForProperty(hash, name); 1311 } 1312 1313 } 1314 1315 @Override 1316 public Base addChild(String name) throws FHIRException { 1317 if (name.equals("status")) { 1318 throw new FHIRException("Cannot call addChild on a singleton property Subscription.status"); 1319 } 1320 else if (name.equals("contact")) { 1321 return addContact(); 1322 } 1323 else if (name.equals("end")) { 1324 throw new FHIRException("Cannot call addChild on a singleton property Subscription.end"); 1325 } 1326 else if (name.equals("reason")) { 1327 throw new FHIRException("Cannot call addChild on a singleton property Subscription.reason"); 1328 } 1329 else if (name.equals("criteria")) { 1330 throw new FHIRException("Cannot call addChild on a singleton property Subscription.criteria"); 1331 } 1332 else if (name.equals("error")) { 1333 throw new FHIRException("Cannot call addChild on a singleton property Subscription.error"); 1334 } 1335 else if (name.equals("channel")) { 1336 this.channel = new SubscriptionChannelComponent(); 1337 return this.channel; 1338 } 1339 else if (name.equals("tag")) { 1340 return addTag(); 1341 } 1342 else 1343 return super.addChild(name); 1344 } 1345 1346 public String fhirType() { 1347 return "Subscription"; 1348 1349 } 1350 1351 public Subscription copy() { 1352 Subscription dst = new Subscription(); 1353 copyValues(dst); 1354 dst.status = status == null ? null : status.copy(); 1355 if (contact != null) { 1356 dst.contact = new ArrayList<ContactPoint>(); 1357 for (ContactPoint i : contact) 1358 dst.contact.add(i.copy()); 1359 }; 1360 dst.end = end == null ? null : end.copy(); 1361 dst.reason = reason == null ? null : reason.copy(); 1362 dst.criteria = criteria == null ? null : criteria.copy(); 1363 dst.error = error == null ? null : error.copy(); 1364 dst.channel = channel == null ? null : channel.copy(); 1365 if (tag != null) { 1366 dst.tag = new ArrayList<Coding>(); 1367 for (Coding i : tag) 1368 dst.tag.add(i.copy()); 1369 }; 1370 return dst; 1371 } 1372 1373 protected Subscription typedCopy() { 1374 return copy(); 1375 } 1376 1377 @Override 1378 public boolean equalsDeep(Base other_) { 1379 if (!super.equalsDeep(other_)) 1380 return false; 1381 if (!(other_ instanceof Subscription)) 1382 return false; 1383 Subscription o = (Subscription) other_; 1384 return compareDeep(status, o.status, true) && compareDeep(contact, o.contact, true) && compareDeep(end, o.end, true) 1385 && compareDeep(reason, o.reason, true) && compareDeep(criteria, o.criteria, true) && compareDeep(error, o.error, true) 1386 && compareDeep(channel, o.channel, true) && compareDeep(tag, o.tag, true); 1387 } 1388 1389 @Override 1390 public boolean equalsShallow(Base other_) { 1391 if (!super.equalsShallow(other_)) 1392 return false; 1393 if (!(other_ instanceof Subscription)) 1394 return false; 1395 Subscription o = (Subscription) other_; 1396 return compareValues(status, o.status, true) && compareValues(end, o.end, true) && compareValues(reason, o.reason, true) 1397 && compareValues(criteria, o.criteria, true) && compareValues(error, o.error, true); 1398 } 1399 1400 public boolean isEmpty() { 1401 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, contact, end, reason 1402 , criteria, error, channel, tag); 1403 } 1404 1405 @Override 1406 public ResourceType getResourceType() { 1407 return ResourceType.Subscription; 1408 } 1409 1410 /** 1411 * Search parameter: <b>payload</b> 1412 * <p> 1413 * Description: <b>The mime-type of the notification payload</b><br> 1414 * Type: <b>string</b><br> 1415 * Path: <b>Subscription.channel.payload</b><br> 1416 * </p> 1417 */ 1418 @SearchParamDefinition(name="payload", path="Subscription.channel.payload", description="The mime-type of the notification payload", type="string" ) 1419 public static final String SP_PAYLOAD = "payload"; 1420 /** 1421 * <b>Fluent Client</b> search parameter constant for <b>payload</b> 1422 * <p> 1423 * Description: <b>The mime-type of the notification payload</b><br> 1424 * Type: <b>string</b><br> 1425 * Path: <b>Subscription.channel.payload</b><br> 1426 * </p> 1427 */ 1428 public static final ca.uhn.fhir.rest.gclient.StringClientParam PAYLOAD = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PAYLOAD); 1429 1430 /** 1431 * Search parameter: <b>criteria</b> 1432 * <p> 1433 * Description: <b>The search rules used to determine when to send a notification</b><br> 1434 * Type: <b>string</b><br> 1435 * Path: <b>Subscription.criteria</b><br> 1436 * </p> 1437 */ 1438 @SearchParamDefinition(name="criteria", path="Subscription.criteria", description="The search rules used to determine when to send a notification", type="string" ) 1439 public static final String SP_CRITERIA = "criteria"; 1440 /** 1441 * <b>Fluent Client</b> search parameter constant for <b>criteria</b> 1442 * <p> 1443 * Description: <b>The search rules used to determine when to send a notification</b><br> 1444 * Type: <b>string</b><br> 1445 * Path: <b>Subscription.criteria</b><br> 1446 * </p> 1447 */ 1448 public static final ca.uhn.fhir.rest.gclient.StringClientParam CRITERIA = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_CRITERIA); 1449 1450 /** 1451 * Search parameter: <b>add-tag</b> 1452 * <p> 1453 * Description: <b>A tag to be added to the resource matching the criteria</b><br> 1454 * Type: <b>token</b><br> 1455 * Path: <b>Subscription.tag</b><br> 1456 * </p> 1457 */ 1458 @SearchParamDefinition(name="add-tag", path="Subscription.tag", description="A tag to be added to the resource matching the criteria", type="token" ) 1459 public static final String SP_ADD_TAG = "add-tag"; 1460 /** 1461 * <b>Fluent Client</b> search parameter constant for <b>add-tag</b> 1462 * <p> 1463 * Description: <b>A tag to be added to the resource matching the criteria</b><br> 1464 * Type: <b>token</b><br> 1465 * Path: <b>Subscription.tag</b><br> 1466 * </p> 1467 */ 1468 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADD_TAG = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADD_TAG); 1469 1470 /** 1471 * Search parameter: <b>contact</b> 1472 * <p> 1473 * Description: <b>Contact details for the subscription</b><br> 1474 * Type: <b>token</b><br> 1475 * Path: <b>Subscription.contact</b><br> 1476 * </p> 1477 */ 1478 @SearchParamDefinition(name="contact", path="Subscription.contact", description="Contact details for the subscription", type="token" ) 1479 public static final String SP_CONTACT = "contact"; 1480 /** 1481 * <b>Fluent Client</b> search parameter constant for <b>contact</b> 1482 * <p> 1483 * Description: <b>Contact details for the subscription</b><br> 1484 * Type: <b>token</b><br> 1485 * Path: <b>Subscription.contact</b><br> 1486 * </p> 1487 */ 1488 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTACT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTACT); 1489 1490 /** 1491 * Search parameter: <b>type</b> 1492 * <p> 1493 * Description: <b>The type of channel for the sent notifications</b><br> 1494 * Type: <b>token</b><br> 1495 * Path: <b>Subscription.channel.type</b><br> 1496 * </p> 1497 */ 1498 @SearchParamDefinition(name="type", path="Subscription.channel.type", description="The type of channel for the sent notifications", type="token" ) 1499 public static final String SP_TYPE = "type"; 1500 /** 1501 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1502 * <p> 1503 * Description: <b>The type of channel for the sent notifications</b><br> 1504 * Type: <b>token</b><br> 1505 * Path: <b>Subscription.channel.type</b><br> 1506 * </p> 1507 */ 1508 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1509 1510 /** 1511 * Search parameter: <b>url</b> 1512 * <p> 1513 * Description: <b>The uri that will receive the notifications</b><br> 1514 * Type: <b>uri</b><br> 1515 * Path: <b>Subscription.channel.endpoint</b><br> 1516 * </p> 1517 */ 1518 @SearchParamDefinition(name="url", path="Subscription.channel.endpoint", description="The uri that will receive the notifications", type="uri" ) 1519 public static final String SP_URL = "url"; 1520 /** 1521 * <b>Fluent Client</b> search parameter constant for <b>url</b> 1522 * <p> 1523 * Description: <b>The uri that will receive the notifications</b><br> 1524 * Type: <b>uri</b><br> 1525 * Path: <b>Subscription.channel.endpoint</b><br> 1526 * </p> 1527 */ 1528 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 1529 1530 /** 1531 * Search parameter: <b>status</b> 1532 * <p> 1533 * Description: <b>The current state of the subscription</b><br> 1534 * Type: <b>token</b><br> 1535 * Path: <b>Subscription.status</b><br> 1536 * </p> 1537 */ 1538 @SearchParamDefinition(name="status", path="Subscription.status", description="The current state of the subscription", type="token" ) 1539 public static final String SP_STATUS = "status"; 1540 /** 1541 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1542 * <p> 1543 * Description: <b>The current state of the subscription</b><br> 1544 * Type: <b>token</b><br> 1545 * Path: <b>Subscription.status</b><br> 1546 * </p> 1547 */ 1548 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1549 1550 1551}