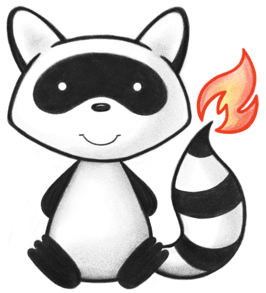
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * A homogeneous material with a definite composition. 052 */ 053@ResourceDef(name="Substance", profile="http://hl7.org/fhir/Profile/Substance") 054public class Substance extends DomainResource { 055 056 public enum FHIRSubstanceStatus { 057 /** 058 * The substance is considered for use or reference 059 */ 060 ACTIVE, 061 /** 062 * The substance is considered for reference, but not for use 063 */ 064 INACTIVE, 065 /** 066 * The substance was entered in error 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static FHIRSubstanceStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("inactive".equals(codeString)) 079 return INACTIVE; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown FHIRSubstanceStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case INACTIVE: return "inactive"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/substance-status"; 099 case INACTIVE: return "http://hl7.org/fhir/substance-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/substance-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The substance is considered for use or reference"; 108 case INACTIVE: return "The substance is considered for reference, but not for use"; 109 case ENTEREDINERROR: return "The substance was entered in error"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case INACTIVE: return "Inactive"; 118 case ENTEREDINERROR: return "Entered in Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class FHIRSubstanceStatusEnumFactory implements EnumFactory<FHIRSubstanceStatus> { 126 public FHIRSubstanceStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return FHIRSubstanceStatus.ACTIVE; 132 if ("inactive".equals(codeString)) 133 return FHIRSubstanceStatus.INACTIVE; 134 if ("entered-in-error".equals(codeString)) 135 return FHIRSubstanceStatus.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown FHIRSubstanceStatus code '"+codeString+"'"); 137 } 138 public Enumeration<FHIRSubstanceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<FHIRSubstanceStatus>(this); 143 String codeString = code.asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return null; 146 if ("active".equals(codeString)) 147 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.ACTIVE); 148 if ("inactive".equals(codeString)) 149 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.INACTIVE); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.ENTEREDINERROR); 152 throw new FHIRException("Unknown FHIRSubstanceStatus code '"+codeString+"'"); 153 } 154 public String toCode(FHIRSubstanceStatus code) { 155 if (code == FHIRSubstanceStatus.ACTIVE) 156 return "active"; 157 if (code == FHIRSubstanceStatus.INACTIVE) 158 return "inactive"; 159 if (code == FHIRSubstanceStatus.ENTEREDINERROR) 160 return "entered-in-error"; 161 return "?"; 162 } 163 public String toSystem(FHIRSubstanceStatus code) { 164 return code.getSystem(); 165 } 166 } 167 168 @Block() 169 public static class SubstanceInstanceComponent extends BackboneElement implements IBaseBackboneElement { 170 /** 171 * Identifier associated with the package/container (usually a label affixed directly). 172 */ 173 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 174 @Description(shortDefinition="Identifier of the package/container", formalDefinition="Identifier associated with the package/container (usually a label affixed directly)." ) 175 protected Identifier identifier; 176 177 /** 178 * When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry. 179 */ 180 @Child(name = "expiry", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 181 @Description(shortDefinition="When no longer valid to use", formalDefinition="When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry." ) 182 protected DateTimeType expiry; 183 184 /** 185 * The amount of the substance. 186 */ 187 @Child(name = "quantity", type = {SimpleQuantity.class}, order=3, min=0, max=1, modifier=false, summary=true) 188 @Description(shortDefinition="Amount of substance in the package", formalDefinition="The amount of the substance." ) 189 protected SimpleQuantity quantity; 190 191 private static final long serialVersionUID = -794314734L; 192 193 /** 194 * Constructor 195 */ 196 public SubstanceInstanceComponent() { 197 super(); 198 } 199 200 /** 201 * @return {@link #identifier} (Identifier associated with the package/container (usually a label affixed directly).) 202 */ 203 public Identifier getIdentifier() { 204 if (this.identifier == null) 205 if (Configuration.errorOnAutoCreate()) 206 throw new Error("Attempt to auto-create SubstanceInstanceComponent.identifier"); 207 else if (Configuration.doAutoCreate()) 208 this.identifier = new Identifier(); // cc 209 return this.identifier; 210 } 211 212 public boolean hasIdentifier() { 213 return this.identifier != null && !this.identifier.isEmpty(); 214 } 215 216 /** 217 * @param value {@link #identifier} (Identifier associated with the package/container (usually a label affixed directly).) 218 */ 219 public SubstanceInstanceComponent setIdentifier(Identifier value) { 220 this.identifier = value; 221 return this; 222 } 223 224 /** 225 * @return {@link #expiry} (When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 226 */ 227 public DateTimeType getExpiryElement() { 228 if (this.expiry == null) 229 if (Configuration.errorOnAutoCreate()) 230 throw new Error("Attempt to auto-create SubstanceInstanceComponent.expiry"); 231 else if (Configuration.doAutoCreate()) 232 this.expiry = new DateTimeType(); // bb 233 return this.expiry; 234 } 235 236 public boolean hasExpiryElement() { 237 return this.expiry != null && !this.expiry.isEmpty(); 238 } 239 240 public boolean hasExpiry() { 241 return this.expiry != null && !this.expiry.isEmpty(); 242 } 243 244 /** 245 * @param value {@link #expiry} (When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 246 */ 247 public SubstanceInstanceComponent setExpiryElement(DateTimeType value) { 248 this.expiry = value; 249 return this; 250 } 251 252 /** 253 * @return When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry. 254 */ 255 public Date getExpiry() { 256 return this.expiry == null ? null : this.expiry.getValue(); 257 } 258 259 /** 260 * @param value When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry. 261 */ 262 public SubstanceInstanceComponent setExpiry(Date value) { 263 if (value == null) 264 this.expiry = null; 265 else { 266 if (this.expiry == null) 267 this.expiry = new DateTimeType(); 268 this.expiry.setValue(value); 269 } 270 return this; 271 } 272 273 /** 274 * @return {@link #quantity} (The amount of the substance.) 275 */ 276 public SimpleQuantity getQuantity() { 277 if (this.quantity == null) 278 if (Configuration.errorOnAutoCreate()) 279 throw new Error("Attempt to auto-create SubstanceInstanceComponent.quantity"); 280 else if (Configuration.doAutoCreate()) 281 this.quantity = new SimpleQuantity(); // cc 282 return this.quantity; 283 } 284 285 public boolean hasQuantity() { 286 return this.quantity != null && !this.quantity.isEmpty(); 287 } 288 289 /** 290 * @param value {@link #quantity} (The amount of the substance.) 291 */ 292 public SubstanceInstanceComponent setQuantity(SimpleQuantity value) { 293 this.quantity = value; 294 return this; 295 } 296 297 protected void listChildren(List<Property> children) { 298 super.listChildren(children); 299 children.add(new Property("identifier", "Identifier", "Identifier associated with the package/container (usually a label affixed directly).", 0, 1, identifier)); 300 children.add(new Property("expiry", "dateTime", "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.", 0, 1, expiry)); 301 children.add(new Property("quantity", "SimpleQuantity", "The amount of the substance.", 0, 1, quantity)); 302 } 303 304 @Override 305 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 306 switch (_hash) { 307 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier associated with the package/container (usually a label affixed directly).", 0, 1, identifier); 308 case -1289159373: /*expiry*/ return new Property("expiry", "dateTime", "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.", 0, 1, expiry); 309 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The amount of the substance.", 0, 1, quantity); 310 default: return super.getNamedProperty(_hash, _name, _checkValid); 311 } 312 313 } 314 315 @Override 316 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 317 switch (hash) { 318 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 319 case -1289159373: /*expiry*/ return this.expiry == null ? new Base[0] : new Base[] {this.expiry}; // DateTimeType 320 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 321 default: return super.getProperty(hash, name, checkValid); 322 } 323 324 } 325 326 @Override 327 public Base setProperty(int hash, String name, Base value) throws FHIRException { 328 switch (hash) { 329 case -1618432855: // identifier 330 this.identifier = castToIdentifier(value); // Identifier 331 return value; 332 case -1289159373: // expiry 333 this.expiry = castToDateTime(value); // DateTimeType 334 return value; 335 case -1285004149: // quantity 336 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 337 return value; 338 default: return super.setProperty(hash, name, value); 339 } 340 341 } 342 343 @Override 344 public Base setProperty(String name, Base value) throws FHIRException { 345 if (name.equals("identifier")) { 346 this.identifier = castToIdentifier(value); // Identifier 347 } else if (name.equals("expiry")) { 348 this.expiry = castToDateTime(value); // DateTimeType 349 } else if (name.equals("quantity")) { 350 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 351 } else 352 return super.setProperty(name, value); 353 return value; 354 } 355 356 @Override 357 public Base makeProperty(int hash, String name) throws FHIRException { 358 switch (hash) { 359 case -1618432855: return getIdentifier(); 360 case -1289159373: return getExpiryElement(); 361 case -1285004149: return getQuantity(); 362 default: return super.makeProperty(hash, name); 363 } 364 365 } 366 367 @Override 368 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 369 switch (hash) { 370 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 371 case -1289159373: /*expiry*/ return new String[] {"dateTime"}; 372 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 373 default: return super.getTypesForProperty(hash, name); 374 } 375 376 } 377 378 @Override 379 public Base addChild(String name) throws FHIRException { 380 if (name.equals("identifier")) { 381 this.identifier = new Identifier(); 382 return this.identifier; 383 } 384 else if (name.equals("expiry")) { 385 throw new FHIRException("Cannot call addChild on a singleton property Substance.expiry"); 386 } 387 else if (name.equals("quantity")) { 388 this.quantity = new SimpleQuantity(); 389 return this.quantity; 390 } 391 else 392 return super.addChild(name); 393 } 394 395 public SubstanceInstanceComponent copy() { 396 SubstanceInstanceComponent dst = new SubstanceInstanceComponent(); 397 copyValues(dst); 398 dst.identifier = identifier == null ? null : identifier.copy(); 399 dst.expiry = expiry == null ? null : expiry.copy(); 400 dst.quantity = quantity == null ? null : quantity.copy(); 401 return dst; 402 } 403 404 @Override 405 public boolean equalsDeep(Base other_) { 406 if (!super.equalsDeep(other_)) 407 return false; 408 if (!(other_ instanceof SubstanceInstanceComponent)) 409 return false; 410 SubstanceInstanceComponent o = (SubstanceInstanceComponent) other_; 411 return compareDeep(identifier, o.identifier, true) && compareDeep(expiry, o.expiry, true) && compareDeep(quantity, o.quantity, true) 412 ; 413 } 414 415 @Override 416 public boolean equalsShallow(Base other_) { 417 if (!super.equalsShallow(other_)) 418 return false; 419 if (!(other_ instanceof SubstanceInstanceComponent)) 420 return false; 421 SubstanceInstanceComponent o = (SubstanceInstanceComponent) other_; 422 return compareValues(expiry, o.expiry, true); 423 } 424 425 public boolean isEmpty() { 426 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, expiry, quantity 427 ); 428 } 429 430 public String fhirType() { 431 return "Substance.instance"; 432 433 } 434 435 } 436 437 @Block() 438 public static class SubstanceIngredientComponent extends BackboneElement implements IBaseBackboneElement { 439 /** 440 * The amount of the ingredient in the substance - a concentration ratio. 441 */ 442 @Child(name = "quantity", type = {Ratio.class}, order=1, min=0, max=1, modifier=false, summary=true) 443 @Description(shortDefinition="Optional amount (concentration)", formalDefinition="The amount of the ingredient in the substance - a concentration ratio." ) 444 protected Ratio quantity; 445 446 /** 447 * Another substance that is a component of this substance. 448 */ 449 @Child(name = "substance", type = {CodeableConcept.class, Substance.class}, order=2, min=1, max=1, modifier=false, summary=true) 450 @Description(shortDefinition="A component of the substance", formalDefinition="Another substance that is a component of this substance." ) 451 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-code") 452 protected Type substance; 453 454 private static final long serialVersionUID = -469805322L; 455 456 /** 457 * Constructor 458 */ 459 public SubstanceIngredientComponent() { 460 super(); 461 } 462 463 /** 464 * Constructor 465 */ 466 public SubstanceIngredientComponent(Type substance) { 467 super(); 468 this.substance = substance; 469 } 470 471 /** 472 * @return {@link #quantity} (The amount of the ingredient in the substance - a concentration ratio.) 473 */ 474 public Ratio getQuantity() { 475 if (this.quantity == null) 476 if (Configuration.errorOnAutoCreate()) 477 throw new Error("Attempt to auto-create SubstanceIngredientComponent.quantity"); 478 else if (Configuration.doAutoCreate()) 479 this.quantity = new Ratio(); // cc 480 return this.quantity; 481 } 482 483 public boolean hasQuantity() { 484 return this.quantity != null && !this.quantity.isEmpty(); 485 } 486 487 /** 488 * @param value {@link #quantity} (The amount of the ingredient in the substance - a concentration ratio.) 489 */ 490 public SubstanceIngredientComponent setQuantity(Ratio value) { 491 this.quantity = value; 492 return this; 493 } 494 495 /** 496 * @return {@link #substance} (Another substance that is a component of this substance.) 497 */ 498 public Type getSubstance() { 499 return this.substance; 500 } 501 502 /** 503 * @return {@link #substance} (Another substance that is a component of this substance.) 504 */ 505 public CodeableConcept getSubstanceCodeableConcept() throws FHIRException { 506 if (this.substance == null) 507 return null; 508 if (!(this.substance instanceof CodeableConcept)) 509 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.substance.getClass().getName()+" was encountered"); 510 return (CodeableConcept) this.substance; 511 } 512 513 public boolean hasSubstanceCodeableConcept() { 514 return this != null && this.substance instanceof CodeableConcept; 515 } 516 517 /** 518 * @return {@link #substance} (Another substance that is a component of this substance.) 519 */ 520 public Reference getSubstanceReference() throws FHIRException { 521 if (this.substance == null) 522 return null; 523 if (!(this.substance instanceof Reference)) 524 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.substance.getClass().getName()+" was encountered"); 525 return (Reference) this.substance; 526 } 527 528 public boolean hasSubstanceReference() { 529 return this != null && this.substance instanceof Reference; 530 } 531 532 public boolean hasSubstance() { 533 return this.substance != null && !this.substance.isEmpty(); 534 } 535 536 /** 537 * @param value {@link #substance} (Another substance that is a component of this substance.) 538 */ 539 public SubstanceIngredientComponent setSubstance(Type value) throws FHIRFormatError { 540 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 541 throw new FHIRFormatError("Not the right type for Substance.ingredient.substance[x]: "+value.fhirType()); 542 this.substance = value; 543 return this; 544 } 545 546 protected void listChildren(List<Property> children) { 547 super.listChildren(children); 548 children.add(new Property("quantity", "Ratio", "The amount of the ingredient in the substance - a concentration ratio.", 0, 1, quantity)); 549 children.add(new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance)); 550 } 551 552 @Override 553 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 554 switch (_hash) { 555 case -1285004149: /*quantity*/ return new Property("quantity", "Ratio", "The amount of the ingredient in the substance - a concentration ratio.", 0, 1, quantity); 556 case 2127194384: /*substance[x]*/ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 557 case 530040176: /*substance*/ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 558 case -1974119407: /*substanceCodeableConcept*/ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 559 case 516208571: /*substanceReference*/ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 560 default: return super.getNamedProperty(_hash, _name, _checkValid); 561 } 562 563 } 564 565 @Override 566 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 567 switch (hash) { 568 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Ratio 569 case 530040176: /*substance*/ return this.substance == null ? new Base[0] : new Base[] {this.substance}; // Type 570 default: return super.getProperty(hash, name, checkValid); 571 } 572 573 } 574 575 @Override 576 public Base setProperty(int hash, String name, Base value) throws FHIRException { 577 switch (hash) { 578 case -1285004149: // quantity 579 this.quantity = castToRatio(value); // Ratio 580 return value; 581 case 530040176: // substance 582 this.substance = castToType(value); // Type 583 return value; 584 default: return super.setProperty(hash, name, value); 585 } 586 587 } 588 589 @Override 590 public Base setProperty(String name, Base value) throws FHIRException { 591 if (name.equals("quantity")) { 592 this.quantity = castToRatio(value); // Ratio 593 } else if (name.equals("substance[x]")) { 594 this.substance = castToType(value); // Type 595 } else 596 return super.setProperty(name, value); 597 return value; 598 } 599 600 @Override 601 public Base makeProperty(int hash, String name) throws FHIRException { 602 switch (hash) { 603 case -1285004149: return getQuantity(); 604 case 2127194384: return getSubstance(); 605 case 530040176: return getSubstance(); 606 default: return super.makeProperty(hash, name); 607 } 608 609 } 610 611 @Override 612 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 613 switch (hash) { 614 case -1285004149: /*quantity*/ return new String[] {"Ratio"}; 615 case 530040176: /*substance*/ return new String[] {"CodeableConcept", "Reference"}; 616 default: return super.getTypesForProperty(hash, name); 617 } 618 619 } 620 621 @Override 622 public Base addChild(String name) throws FHIRException { 623 if (name.equals("quantity")) { 624 this.quantity = new Ratio(); 625 return this.quantity; 626 } 627 else if (name.equals("substanceCodeableConcept")) { 628 this.substance = new CodeableConcept(); 629 return this.substance; 630 } 631 else if (name.equals("substanceReference")) { 632 this.substance = new Reference(); 633 return this.substance; 634 } 635 else 636 return super.addChild(name); 637 } 638 639 public SubstanceIngredientComponent copy() { 640 SubstanceIngredientComponent dst = new SubstanceIngredientComponent(); 641 copyValues(dst); 642 dst.quantity = quantity == null ? null : quantity.copy(); 643 dst.substance = substance == null ? null : substance.copy(); 644 return dst; 645 } 646 647 @Override 648 public boolean equalsDeep(Base other_) { 649 if (!super.equalsDeep(other_)) 650 return false; 651 if (!(other_ instanceof SubstanceIngredientComponent)) 652 return false; 653 SubstanceIngredientComponent o = (SubstanceIngredientComponent) other_; 654 return compareDeep(quantity, o.quantity, true) && compareDeep(substance, o.substance, true); 655 } 656 657 @Override 658 public boolean equalsShallow(Base other_) { 659 if (!super.equalsShallow(other_)) 660 return false; 661 if (!(other_ instanceof SubstanceIngredientComponent)) 662 return false; 663 SubstanceIngredientComponent o = (SubstanceIngredientComponent) other_; 664 return true; 665 } 666 667 public boolean isEmpty() { 668 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, substance); 669 } 670 671 public String fhirType() { 672 return "Substance.ingredient"; 673 674 } 675 676 } 677 678 /** 679 * Unique identifier for the substance. 680 */ 681 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 682 @Description(shortDefinition="Unique identifier", formalDefinition="Unique identifier for the substance." ) 683 protected List<Identifier> identifier; 684 685 /** 686 * A code to indicate if the substance is actively used. 687 */ 688 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 689 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="A code to indicate if the substance is actively used." ) 690 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-status") 691 protected Enumeration<FHIRSubstanceStatus> status; 692 693 /** 694 * A code that classifies the general type of substance. This is used for searching, sorting and display purposes. 695 */ 696 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 697 @Description(shortDefinition="What class/type of substance this is", formalDefinition="A code that classifies the general type of substance. This is used for searching, sorting and display purposes." ) 698 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-category") 699 protected List<CodeableConcept> category; 700 701 /** 702 * A code (or set of codes) that identify this substance. 703 */ 704 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 705 @Description(shortDefinition="What substance this is", formalDefinition="A code (or set of codes) that identify this substance." ) 706 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-code") 707 protected CodeableConcept code; 708 709 /** 710 * A description of the substance - its appearance, handling requirements, and other usage notes. 711 */ 712 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 713 @Description(shortDefinition="Textual description of the substance, comments", formalDefinition="A description of the substance - its appearance, handling requirements, and other usage notes." ) 714 protected StringType description; 715 716 /** 717 * Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance. 718 */ 719 @Child(name = "instance", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 720 @Description(shortDefinition="If this describes a specific package/container of the substance", formalDefinition="Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance." ) 721 protected List<SubstanceInstanceComponent> instance; 722 723 /** 724 * A substance can be composed of other substances. 725 */ 726 @Child(name = "ingredient", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 727 @Description(shortDefinition="Composition information about the substance", formalDefinition="A substance can be composed of other substances." ) 728 protected List<SubstanceIngredientComponent> ingredient; 729 730 private static final long serialVersionUID = -1467626602L; 731 732 /** 733 * Constructor 734 */ 735 public Substance() { 736 super(); 737 } 738 739 /** 740 * Constructor 741 */ 742 public Substance(CodeableConcept code) { 743 super(); 744 this.code = code; 745 } 746 747 /** 748 * @return {@link #identifier} (Unique identifier for the substance.) 749 */ 750 public List<Identifier> getIdentifier() { 751 if (this.identifier == null) 752 this.identifier = new ArrayList<Identifier>(); 753 return this.identifier; 754 } 755 756 /** 757 * @return Returns a reference to <code>this</code> for easy method chaining 758 */ 759 public Substance setIdentifier(List<Identifier> theIdentifier) { 760 this.identifier = theIdentifier; 761 return this; 762 } 763 764 public boolean hasIdentifier() { 765 if (this.identifier == null) 766 return false; 767 for (Identifier item : this.identifier) 768 if (!item.isEmpty()) 769 return true; 770 return false; 771 } 772 773 public Identifier addIdentifier() { //3 774 Identifier t = new Identifier(); 775 if (this.identifier == null) 776 this.identifier = new ArrayList<Identifier>(); 777 this.identifier.add(t); 778 return t; 779 } 780 781 public Substance addIdentifier(Identifier t) { //3 782 if (t == null) 783 return this; 784 if (this.identifier == null) 785 this.identifier = new ArrayList<Identifier>(); 786 this.identifier.add(t); 787 return this; 788 } 789 790 /** 791 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 792 */ 793 public Identifier getIdentifierFirstRep() { 794 if (getIdentifier().isEmpty()) { 795 addIdentifier(); 796 } 797 return getIdentifier().get(0); 798 } 799 800 /** 801 * @return {@link #status} (A code to indicate if the substance is actively used.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 802 */ 803 public Enumeration<FHIRSubstanceStatus> getStatusElement() { 804 if (this.status == null) 805 if (Configuration.errorOnAutoCreate()) 806 throw new Error("Attempt to auto-create Substance.status"); 807 else if (Configuration.doAutoCreate()) 808 this.status = new Enumeration<FHIRSubstanceStatus>(new FHIRSubstanceStatusEnumFactory()); // bb 809 return this.status; 810 } 811 812 public boolean hasStatusElement() { 813 return this.status != null && !this.status.isEmpty(); 814 } 815 816 public boolean hasStatus() { 817 return this.status != null && !this.status.isEmpty(); 818 } 819 820 /** 821 * @param value {@link #status} (A code to indicate if the substance is actively used.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 822 */ 823 public Substance setStatusElement(Enumeration<FHIRSubstanceStatus> value) { 824 this.status = value; 825 return this; 826 } 827 828 /** 829 * @return A code to indicate if the substance is actively used. 830 */ 831 public FHIRSubstanceStatus getStatus() { 832 return this.status == null ? null : this.status.getValue(); 833 } 834 835 /** 836 * @param value A code to indicate if the substance is actively used. 837 */ 838 public Substance setStatus(FHIRSubstanceStatus value) { 839 if (value == null) 840 this.status = null; 841 else { 842 if (this.status == null) 843 this.status = new Enumeration<FHIRSubstanceStatus>(new FHIRSubstanceStatusEnumFactory()); 844 this.status.setValue(value); 845 } 846 return this; 847 } 848 849 /** 850 * @return {@link #category} (A code that classifies the general type of substance. This is used for searching, sorting and display purposes.) 851 */ 852 public List<CodeableConcept> getCategory() { 853 if (this.category == null) 854 this.category = new ArrayList<CodeableConcept>(); 855 return this.category; 856 } 857 858 /** 859 * @return Returns a reference to <code>this</code> for easy method chaining 860 */ 861 public Substance setCategory(List<CodeableConcept> theCategory) { 862 this.category = theCategory; 863 return this; 864 } 865 866 public boolean hasCategory() { 867 if (this.category == null) 868 return false; 869 for (CodeableConcept item : this.category) 870 if (!item.isEmpty()) 871 return true; 872 return false; 873 } 874 875 public CodeableConcept addCategory() { //3 876 CodeableConcept t = new CodeableConcept(); 877 if (this.category == null) 878 this.category = new ArrayList<CodeableConcept>(); 879 this.category.add(t); 880 return t; 881 } 882 883 public Substance addCategory(CodeableConcept t) { //3 884 if (t == null) 885 return this; 886 if (this.category == null) 887 this.category = new ArrayList<CodeableConcept>(); 888 this.category.add(t); 889 return this; 890 } 891 892 /** 893 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 894 */ 895 public CodeableConcept getCategoryFirstRep() { 896 if (getCategory().isEmpty()) { 897 addCategory(); 898 } 899 return getCategory().get(0); 900 } 901 902 /** 903 * @return {@link #code} (A code (or set of codes) that identify this substance.) 904 */ 905 public CodeableConcept getCode() { 906 if (this.code == null) 907 if (Configuration.errorOnAutoCreate()) 908 throw new Error("Attempt to auto-create Substance.code"); 909 else if (Configuration.doAutoCreate()) 910 this.code = new CodeableConcept(); // cc 911 return this.code; 912 } 913 914 public boolean hasCode() { 915 return this.code != null && !this.code.isEmpty(); 916 } 917 918 /** 919 * @param value {@link #code} (A code (or set of codes) that identify this substance.) 920 */ 921 public Substance setCode(CodeableConcept value) { 922 this.code = value; 923 return this; 924 } 925 926 /** 927 * @return {@link #description} (A description of the substance - its appearance, handling requirements, and other usage notes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 928 */ 929 public StringType getDescriptionElement() { 930 if (this.description == null) 931 if (Configuration.errorOnAutoCreate()) 932 throw new Error("Attempt to auto-create Substance.description"); 933 else if (Configuration.doAutoCreate()) 934 this.description = new StringType(); // bb 935 return this.description; 936 } 937 938 public boolean hasDescriptionElement() { 939 return this.description != null && !this.description.isEmpty(); 940 } 941 942 public boolean hasDescription() { 943 return this.description != null && !this.description.isEmpty(); 944 } 945 946 /** 947 * @param value {@link #description} (A description of the substance - its appearance, handling requirements, and other usage notes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 948 */ 949 public Substance setDescriptionElement(StringType value) { 950 this.description = value; 951 return this; 952 } 953 954 /** 955 * @return A description of the substance - its appearance, handling requirements, and other usage notes. 956 */ 957 public String getDescription() { 958 return this.description == null ? null : this.description.getValue(); 959 } 960 961 /** 962 * @param value A description of the substance - its appearance, handling requirements, and other usage notes. 963 */ 964 public Substance setDescription(String value) { 965 if (Utilities.noString(value)) 966 this.description = null; 967 else { 968 if (this.description == null) 969 this.description = new StringType(); 970 this.description.setValue(value); 971 } 972 return this; 973 } 974 975 /** 976 * @return {@link #instance} (Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.) 977 */ 978 public List<SubstanceInstanceComponent> getInstance() { 979 if (this.instance == null) 980 this.instance = new ArrayList<SubstanceInstanceComponent>(); 981 return this.instance; 982 } 983 984 /** 985 * @return Returns a reference to <code>this</code> for easy method chaining 986 */ 987 public Substance setInstance(List<SubstanceInstanceComponent> theInstance) { 988 this.instance = theInstance; 989 return this; 990 } 991 992 public boolean hasInstance() { 993 if (this.instance == null) 994 return false; 995 for (SubstanceInstanceComponent item : this.instance) 996 if (!item.isEmpty()) 997 return true; 998 return false; 999 } 1000 1001 public SubstanceInstanceComponent addInstance() { //3 1002 SubstanceInstanceComponent t = new SubstanceInstanceComponent(); 1003 if (this.instance == null) 1004 this.instance = new ArrayList<SubstanceInstanceComponent>(); 1005 this.instance.add(t); 1006 return t; 1007 } 1008 1009 public Substance addInstance(SubstanceInstanceComponent t) { //3 1010 if (t == null) 1011 return this; 1012 if (this.instance == null) 1013 this.instance = new ArrayList<SubstanceInstanceComponent>(); 1014 this.instance.add(t); 1015 return this; 1016 } 1017 1018 /** 1019 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist 1020 */ 1021 public SubstanceInstanceComponent getInstanceFirstRep() { 1022 if (getInstance().isEmpty()) { 1023 addInstance(); 1024 } 1025 return getInstance().get(0); 1026 } 1027 1028 /** 1029 * @return {@link #ingredient} (A substance can be composed of other substances.) 1030 */ 1031 public List<SubstanceIngredientComponent> getIngredient() { 1032 if (this.ingredient == null) 1033 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1034 return this.ingredient; 1035 } 1036 1037 /** 1038 * @return Returns a reference to <code>this</code> for easy method chaining 1039 */ 1040 public Substance setIngredient(List<SubstanceIngredientComponent> theIngredient) { 1041 this.ingredient = theIngredient; 1042 return this; 1043 } 1044 1045 public boolean hasIngredient() { 1046 if (this.ingredient == null) 1047 return false; 1048 for (SubstanceIngredientComponent item : this.ingredient) 1049 if (!item.isEmpty()) 1050 return true; 1051 return false; 1052 } 1053 1054 public SubstanceIngredientComponent addIngredient() { //3 1055 SubstanceIngredientComponent t = new SubstanceIngredientComponent(); 1056 if (this.ingredient == null) 1057 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1058 this.ingredient.add(t); 1059 return t; 1060 } 1061 1062 public Substance addIngredient(SubstanceIngredientComponent t) { //3 1063 if (t == null) 1064 return this; 1065 if (this.ingredient == null) 1066 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1067 this.ingredient.add(t); 1068 return this; 1069 } 1070 1071 /** 1072 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist 1073 */ 1074 public SubstanceIngredientComponent getIngredientFirstRep() { 1075 if (getIngredient().isEmpty()) { 1076 addIngredient(); 1077 } 1078 return getIngredient().get(0); 1079 } 1080 1081 protected void listChildren(List<Property> children) { 1082 super.listChildren(children); 1083 children.add(new Property("identifier", "Identifier", "Unique identifier for the substance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1084 children.add(new Property("status", "code", "A code to indicate if the substance is actively used.", 0, 1, status)); 1085 children.add(new Property("category", "CodeableConcept", "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.", 0, java.lang.Integer.MAX_VALUE, category)); 1086 children.add(new Property("code", "CodeableConcept", "A code (or set of codes) that identify this substance.", 0, 1, code)); 1087 children.add(new Property("description", "string", "A description of the substance - its appearance, handling requirements, and other usage notes.", 0, 1, description)); 1088 children.add(new Property("instance", "", "Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.", 0, java.lang.Integer.MAX_VALUE, instance)); 1089 children.add(new Property("ingredient", "", "A substance can be composed of other substances.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 1090 } 1091 1092 @Override 1093 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1094 switch (_hash) { 1095 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for the substance.", 0, java.lang.Integer.MAX_VALUE, identifier); 1096 case -892481550: /*status*/ return new Property("status", "code", "A code to indicate if the substance is actively used.", 0, 1, status); 1097 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.", 0, java.lang.Integer.MAX_VALUE, category); 1098 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code (or set of codes) that identify this substance.", 0, 1, code); 1099 case -1724546052: /*description*/ return new Property("description", "string", "A description of the substance - its appearance, handling requirements, and other usage notes.", 0, 1, description); 1100 case 555127957: /*instance*/ return new Property("instance", "", "Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.", 0, java.lang.Integer.MAX_VALUE, instance); 1101 case -206409263: /*ingredient*/ return new Property("ingredient", "", "A substance can be composed of other substances.", 0, java.lang.Integer.MAX_VALUE, ingredient); 1102 default: return super.getNamedProperty(_hash, _name, _checkValid); 1103 } 1104 1105 } 1106 1107 @Override 1108 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1109 switch (hash) { 1110 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1111 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FHIRSubstanceStatus> 1112 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1113 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1114 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1115 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // SubstanceInstanceComponent 1116 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // SubstanceIngredientComponent 1117 default: return super.getProperty(hash, name, checkValid); 1118 } 1119 1120 } 1121 1122 @Override 1123 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1124 switch (hash) { 1125 case -1618432855: // identifier 1126 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1127 return value; 1128 case -892481550: // status 1129 value = new FHIRSubstanceStatusEnumFactory().fromType(castToCode(value)); 1130 this.status = (Enumeration) value; // Enumeration<FHIRSubstanceStatus> 1131 return value; 1132 case 50511102: // category 1133 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1134 return value; 1135 case 3059181: // code 1136 this.code = castToCodeableConcept(value); // CodeableConcept 1137 return value; 1138 case -1724546052: // description 1139 this.description = castToString(value); // StringType 1140 return value; 1141 case 555127957: // instance 1142 this.getInstance().add((SubstanceInstanceComponent) value); // SubstanceInstanceComponent 1143 return value; 1144 case -206409263: // ingredient 1145 this.getIngredient().add((SubstanceIngredientComponent) value); // SubstanceIngredientComponent 1146 return value; 1147 default: return super.setProperty(hash, name, value); 1148 } 1149 1150 } 1151 1152 @Override 1153 public Base setProperty(String name, Base value) throws FHIRException { 1154 if (name.equals("identifier")) { 1155 this.getIdentifier().add(castToIdentifier(value)); 1156 } else if (name.equals("status")) { 1157 value = new FHIRSubstanceStatusEnumFactory().fromType(castToCode(value)); 1158 this.status = (Enumeration) value; // Enumeration<FHIRSubstanceStatus> 1159 } else if (name.equals("category")) { 1160 this.getCategory().add(castToCodeableConcept(value)); 1161 } else if (name.equals("code")) { 1162 this.code = castToCodeableConcept(value); // CodeableConcept 1163 } else if (name.equals("description")) { 1164 this.description = castToString(value); // StringType 1165 } else if (name.equals("instance")) { 1166 this.getInstance().add((SubstanceInstanceComponent) value); 1167 } else if (name.equals("ingredient")) { 1168 this.getIngredient().add((SubstanceIngredientComponent) value); 1169 } else 1170 return super.setProperty(name, value); 1171 return value; 1172 } 1173 1174 @Override 1175 public Base makeProperty(int hash, String name) throws FHIRException { 1176 switch (hash) { 1177 case -1618432855: return addIdentifier(); 1178 case -892481550: return getStatusElement(); 1179 case 50511102: return addCategory(); 1180 case 3059181: return getCode(); 1181 case -1724546052: return getDescriptionElement(); 1182 case 555127957: return addInstance(); 1183 case -206409263: return addIngredient(); 1184 default: return super.makeProperty(hash, name); 1185 } 1186 1187 } 1188 1189 @Override 1190 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1191 switch (hash) { 1192 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1193 case -892481550: /*status*/ return new String[] {"code"}; 1194 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1195 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1196 case -1724546052: /*description*/ return new String[] {"string"}; 1197 case 555127957: /*instance*/ return new String[] {}; 1198 case -206409263: /*ingredient*/ return new String[] {}; 1199 default: return super.getTypesForProperty(hash, name); 1200 } 1201 1202 } 1203 1204 @Override 1205 public Base addChild(String name) throws FHIRException { 1206 if (name.equals("identifier")) { 1207 return addIdentifier(); 1208 } 1209 else if (name.equals("status")) { 1210 throw new FHIRException("Cannot call addChild on a singleton property Substance.status"); 1211 } 1212 else if (name.equals("category")) { 1213 return addCategory(); 1214 } 1215 else if (name.equals("code")) { 1216 this.code = new CodeableConcept(); 1217 return this.code; 1218 } 1219 else if (name.equals("description")) { 1220 throw new FHIRException("Cannot call addChild on a singleton property Substance.description"); 1221 } 1222 else if (name.equals("instance")) { 1223 return addInstance(); 1224 } 1225 else if (name.equals("ingredient")) { 1226 return addIngredient(); 1227 } 1228 else 1229 return super.addChild(name); 1230 } 1231 1232 public String fhirType() { 1233 return "Substance"; 1234 1235 } 1236 1237 public Substance copy() { 1238 Substance dst = new Substance(); 1239 copyValues(dst); 1240 if (identifier != null) { 1241 dst.identifier = new ArrayList<Identifier>(); 1242 for (Identifier i : identifier) 1243 dst.identifier.add(i.copy()); 1244 }; 1245 dst.status = status == null ? null : status.copy(); 1246 if (category != null) { 1247 dst.category = new ArrayList<CodeableConcept>(); 1248 for (CodeableConcept i : category) 1249 dst.category.add(i.copy()); 1250 }; 1251 dst.code = code == null ? null : code.copy(); 1252 dst.description = description == null ? null : description.copy(); 1253 if (instance != null) { 1254 dst.instance = new ArrayList<SubstanceInstanceComponent>(); 1255 for (SubstanceInstanceComponent i : instance) 1256 dst.instance.add(i.copy()); 1257 }; 1258 if (ingredient != null) { 1259 dst.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1260 for (SubstanceIngredientComponent i : ingredient) 1261 dst.ingredient.add(i.copy()); 1262 }; 1263 return dst; 1264 } 1265 1266 protected Substance typedCopy() { 1267 return copy(); 1268 } 1269 1270 @Override 1271 public boolean equalsDeep(Base other_) { 1272 if (!super.equalsDeep(other_)) 1273 return false; 1274 if (!(other_ instanceof Substance)) 1275 return false; 1276 Substance o = (Substance) other_; 1277 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1278 && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) && compareDeep(instance, o.instance, true) 1279 && compareDeep(ingredient, o.ingredient, true); 1280 } 1281 1282 @Override 1283 public boolean equalsShallow(Base other_) { 1284 if (!super.equalsShallow(other_)) 1285 return false; 1286 if (!(other_ instanceof Substance)) 1287 return false; 1288 Substance o = (Substance) other_; 1289 return compareValues(status, o.status, true) && compareValues(description, o.description, true); 1290 } 1291 1292 public boolean isEmpty() { 1293 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 1294 , code, description, instance, ingredient); 1295 } 1296 1297 @Override 1298 public ResourceType getResourceType() { 1299 return ResourceType.Substance; 1300 } 1301 1302 /** 1303 * Search parameter: <b>identifier</b> 1304 * <p> 1305 * Description: <b>Unique identifier for the substance</b><br> 1306 * Type: <b>token</b><br> 1307 * Path: <b>Substance.identifier</b><br> 1308 * </p> 1309 */ 1310 @SearchParamDefinition(name="identifier", path="Substance.identifier", description="Unique identifier for the substance", type="token" ) 1311 public static final String SP_IDENTIFIER = "identifier"; 1312 /** 1313 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1314 * <p> 1315 * Description: <b>Unique identifier for the substance</b><br> 1316 * Type: <b>token</b><br> 1317 * Path: <b>Substance.identifier</b><br> 1318 * </p> 1319 */ 1320 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1321 1322 /** 1323 * Search parameter: <b>container-identifier</b> 1324 * <p> 1325 * Description: <b>Identifier of the package/container</b><br> 1326 * Type: <b>token</b><br> 1327 * Path: <b>Substance.instance.identifier</b><br> 1328 * </p> 1329 */ 1330 @SearchParamDefinition(name="container-identifier", path="Substance.instance.identifier", description="Identifier of the package/container", type="token" ) 1331 public static final String SP_CONTAINER_IDENTIFIER = "container-identifier"; 1332 /** 1333 * <b>Fluent Client</b> search parameter constant for <b>container-identifier</b> 1334 * <p> 1335 * Description: <b>Identifier of the package/container</b><br> 1336 * Type: <b>token</b><br> 1337 * Path: <b>Substance.instance.identifier</b><br> 1338 * </p> 1339 */ 1340 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTAINER_IDENTIFIER); 1341 1342 /** 1343 * Search parameter: <b>code</b> 1344 * <p> 1345 * Description: <b>The code of the substance or ingredient</b><br> 1346 * Type: <b>token</b><br> 1347 * Path: <b>Substance.code, Substance.ingredient.substanceCodeableConcept</b><br> 1348 * </p> 1349 */ 1350 @SearchParamDefinition(name="code", path="Substance.code | Substance.ingredient.substance.as(CodeableConcept)", description="The code of the substance or ingredient", type="token" ) 1351 public static final String SP_CODE = "code"; 1352 /** 1353 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1354 * <p> 1355 * Description: <b>The code of the substance or ingredient</b><br> 1356 * Type: <b>token</b><br> 1357 * Path: <b>Substance.code, Substance.ingredient.substanceCodeableConcept</b><br> 1358 * </p> 1359 */ 1360 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1361 1362 /** 1363 * Search parameter: <b>quantity</b> 1364 * <p> 1365 * Description: <b>Amount of substance in the package</b><br> 1366 * Type: <b>quantity</b><br> 1367 * Path: <b>Substance.instance.quantity</b><br> 1368 * </p> 1369 */ 1370 @SearchParamDefinition(name="quantity", path="Substance.instance.quantity", description="Amount of substance in the package", type="quantity" ) 1371 public static final String SP_QUANTITY = "quantity"; 1372 /** 1373 * <b>Fluent Client</b> search parameter constant for <b>quantity</b> 1374 * <p> 1375 * Description: <b>Amount of substance in the package</b><br> 1376 * Type: <b>quantity</b><br> 1377 * Path: <b>Substance.instance.quantity</b><br> 1378 * </p> 1379 */ 1380 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_QUANTITY); 1381 1382 /** 1383 * Search parameter: <b>substance-reference</b> 1384 * <p> 1385 * Description: <b>A component of the substance</b><br> 1386 * Type: <b>reference</b><br> 1387 * Path: <b>Substance.ingredient.substanceReference</b><br> 1388 * </p> 1389 */ 1390 @SearchParamDefinition(name="substance-reference", path="Substance.ingredient.substance.as(Reference)", description="A component of the substance", type="reference", target={Substance.class } ) 1391 public static final String SP_SUBSTANCE_REFERENCE = "substance-reference"; 1392 /** 1393 * <b>Fluent Client</b> search parameter constant for <b>substance-reference</b> 1394 * <p> 1395 * Description: <b>A component of the substance</b><br> 1396 * Type: <b>reference</b><br> 1397 * Path: <b>Substance.ingredient.substanceReference</b><br> 1398 * </p> 1399 */ 1400 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSTANCE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSTANCE_REFERENCE); 1401 1402/** 1403 * Constant for fluent queries to be used to add include statements. Specifies 1404 * the path value of "<b>Substance:substance-reference</b>". 1405 */ 1406 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSTANCE_REFERENCE = new ca.uhn.fhir.model.api.Include("Substance:substance-reference").toLocked(); 1407 1408 /** 1409 * Search parameter: <b>expiry</b> 1410 * <p> 1411 * Description: <b>Expiry date of package or container of substance</b><br> 1412 * Type: <b>date</b><br> 1413 * Path: <b>Substance.instance.expiry</b><br> 1414 * </p> 1415 */ 1416 @SearchParamDefinition(name="expiry", path="Substance.instance.expiry", description="Expiry date of package or container of substance", type="date" ) 1417 public static final String SP_EXPIRY = "expiry"; 1418 /** 1419 * <b>Fluent Client</b> search parameter constant for <b>expiry</b> 1420 * <p> 1421 * Description: <b>Expiry date of package or container of substance</b><br> 1422 * Type: <b>date</b><br> 1423 * Path: <b>Substance.instance.expiry</b><br> 1424 * </p> 1425 */ 1426 public static final ca.uhn.fhir.rest.gclient.DateClientParam EXPIRY = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EXPIRY); 1427 1428 /** 1429 * Search parameter: <b>category</b> 1430 * <p> 1431 * Description: <b>The category of the substance</b><br> 1432 * Type: <b>token</b><br> 1433 * Path: <b>Substance.category</b><br> 1434 * </p> 1435 */ 1436 @SearchParamDefinition(name="category", path="Substance.category", description="The category of the substance", type="token" ) 1437 public static final String SP_CATEGORY = "category"; 1438 /** 1439 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1440 * <p> 1441 * Description: <b>The category of the substance</b><br> 1442 * Type: <b>token</b><br> 1443 * Path: <b>Substance.category</b><br> 1444 * </p> 1445 */ 1446 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1447 1448 /** 1449 * Search parameter: <b>status</b> 1450 * <p> 1451 * Description: <b>active | inactive | entered-in-error</b><br> 1452 * Type: <b>token</b><br> 1453 * Path: <b>Substance.status</b><br> 1454 * </p> 1455 */ 1456 @SearchParamDefinition(name="status", path="Substance.status", description="active | inactive | entered-in-error", type="token" ) 1457 public static final String SP_STATUS = "status"; 1458 /** 1459 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1460 * <p> 1461 * Description: <b>active | inactive | entered-in-error</b><br> 1462 * Type: <b>token</b><br> 1463 * Path: <b>Substance.status</b><br> 1464 * </p> 1465 */ 1466 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1467 1468 1469}