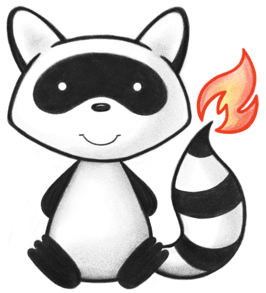
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * A homogeneous material with a definite composition. 052 */ 053@ResourceDef(name="Substance", profile="http://hl7.org/fhir/Profile/Substance") 054public class Substance extends DomainResource { 055 056 public enum FHIRSubstanceStatus { 057 /** 058 * The substance is considered for use or reference 059 */ 060 ACTIVE, 061 /** 062 * The substance is considered for reference, but not for use 063 */ 064 INACTIVE, 065 /** 066 * The substance was entered in error 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static FHIRSubstanceStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("inactive".equals(codeString)) 079 return INACTIVE; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown FHIRSubstanceStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case INACTIVE: return "inactive"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/substance-status"; 099 case INACTIVE: return "http://hl7.org/fhir/substance-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/substance-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The substance is considered for use or reference"; 108 case INACTIVE: return "The substance is considered for reference, but not for use"; 109 case ENTEREDINERROR: return "The substance was entered in error"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case INACTIVE: return "Inactive"; 118 case ENTEREDINERROR: return "Entered in Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class FHIRSubstanceStatusEnumFactory implements EnumFactory<FHIRSubstanceStatus> { 126 public FHIRSubstanceStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return FHIRSubstanceStatus.ACTIVE; 132 if ("inactive".equals(codeString)) 133 return FHIRSubstanceStatus.INACTIVE; 134 if ("entered-in-error".equals(codeString)) 135 return FHIRSubstanceStatus.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown FHIRSubstanceStatus code '"+codeString+"'"); 137 } 138 public Enumeration<FHIRSubstanceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<FHIRSubstanceStatus>(this); 143 String codeString = code.asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return null; 146 if ("active".equals(codeString)) 147 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.ACTIVE); 148 if ("inactive".equals(codeString)) 149 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.INACTIVE); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.ENTEREDINERROR); 152 throw new FHIRException("Unknown FHIRSubstanceStatus code '"+codeString+"'"); 153 } 154 public String toCode(FHIRSubstanceStatus code) { 155 if (code == FHIRSubstanceStatus.NULL) 156 return null; 157 if (code == FHIRSubstanceStatus.ACTIVE) 158 return "active"; 159 if (code == FHIRSubstanceStatus.INACTIVE) 160 return "inactive"; 161 if (code == FHIRSubstanceStatus.ENTEREDINERROR) 162 return "entered-in-error"; 163 return "?"; 164 } 165 public String toSystem(FHIRSubstanceStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class SubstanceInstanceComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * Identifier associated with the package/container (usually a label affixed directly). 174 */ 175 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="Identifier of the package/container", formalDefinition="Identifier associated with the package/container (usually a label affixed directly)." ) 177 protected Identifier identifier; 178 179 /** 180 * When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry. 181 */ 182 @Child(name = "expiry", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 183 @Description(shortDefinition="When no longer valid to use", formalDefinition="When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry." ) 184 protected DateTimeType expiry; 185 186 /** 187 * The amount of the substance. 188 */ 189 @Child(name = "quantity", type = {SimpleQuantity.class}, order=3, min=0, max=1, modifier=false, summary=true) 190 @Description(shortDefinition="Amount of substance in the package", formalDefinition="The amount of the substance." ) 191 protected SimpleQuantity quantity; 192 193 private static final long serialVersionUID = -794314734L; 194 195 /** 196 * Constructor 197 */ 198 public SubstanceInstanceComponent() { 199 super(); 200 } 201 202 /** 203 * @return {@link #identifier} (Identifier associated with the package/container (usually a label affixed directly).) 204 */ 205 public Identifier getIdentifier() { 206 if (this.identifier == null) 207 if (Configuration.errorOnAutoCreate()) 208 throw new Error("Attempt to auto-create SubstanceInstanceComponent.identifier"); 209 else if (Configuration.doAutoCreate()) 210 this.identifier = new Identifier(); // cc 211 return this.identifier; 212 } 213 214 public boolean hasIdentifier() { 215 return this.identifier != null && !this.identifier.isEmpty(); 216 } 217 218 /** 219 * @param value {@link #identifier} (Identifier associated with the package/container (usually a label affixed directly).) 220 */ 221 public SubstanceInstanceComponent setIdentifier(Identifier value) { 222 this.identifier = value; 223 return this; 224 } 225 226 /** 227 * @return {@link #expiry} (When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 228 */ 229 public DateTimeType getExpiryElement() { 230 if (this.expiry == null) 231 if (Configuration.errorOnAutoCreate()) 232 throw new Error("Attempt to auto-create SubstanceInstanceComponent.expiry"); 233 else if (Configuration.doAutoCreate()) 234 this.expiry = new DateTimeType(); // bb 235 return this.expiry; 236 } 237 238 public boolean hasExpiryElement() { 239 return this.expiry != null && !this.expiry.isEmpty(); 240 } 241 242 public boolean hasExpiry() { 243 return this.expiry != null && !this.expiry.isEmpty(); 244 } 245 246 /** 247 * @param value {@link #expiry} (When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 248 */ 249 public SubstanceInstanceComponent setExpiryElement(DateTimeType value) { 250 this.expiry = value; 251 return this; 252 } 253 254 /** 255 * @return When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry. 256 */ 257 public Date getExpiry() { 258 return this.expiry == null ? null : this.expiry.getValue(); 259 } 260 261 /** 262 * @param value When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry. 263 */ 264 public SubstanceInstanceComponent setExpiry(Date value) { 265 if (value == null) 266 this.expiry = null; 267 else { 268 if (this.expiry == null) 269 this.expiry = new DateTimeType(); 270 this.expiry.setValue(value); 271 } 272 return this; 273 } 274 275 /** 276 * @return {@link #quantity} (The amount of the substance.) 277 */ 278 public SimpleQuantity getQuantity() { 279 if (this.quantity == null) 280 if (Configuration.errorOnAutoCreate()) 281 throw new Error("Attempt to auto-create SubstanceInstanceComponent.quantity"); 282 else if (Configuration.doAutoCreate()) 283 this.quantity = new SimpleQuantity(); // cc 284 return this.quantity; 285 } 286 287 public boolean hasQuantity() { 288 return this.quantity != null && !this.quantity.isEmpty(); 289 } 290 291 /** 292 * @param value {@link #quantity} (The amount of the substance.) 293 */ 294 public SubstanceInstanceComponent setQuantity(SimpleQuantity value) { 295 this.quantity = value; 296 return this; 297 } 298 299 protected void listChildren(List<Property> children) { 300 super.listChildren(children); 301 children.add(new Property("identifier", "Identifier", "Identifier associated with the package/container (usually a label affixed directly).", 0, 1, identifier)); 302 children.add(new Property("expiry", "dateTime", "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.", 0, 1, expiry)); 303 children.add(new Property("quantity", "SimpleQuantity", "The amount of the substance.", 0, 1, quantity)); 304 } 305 306 @Override 307 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 308 switch (_hash) { 309 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier associated with the package/container (usually a label affixed directly).", 0, 1, identifier); 310 case -1289159373: /*expiry*/ return new Property("expiry", "dateTime", "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.", 0, 1, expiry); 311 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The amount of the substance.", 0, 1, quantity); 312 default: return super.getNamedProperty(_hash, _name, _checkValid); 313 } 314 315 } 316 317 @Override 318 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 319 switch (hash) { 320 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 321 case -1289159373: /*expiry*/ return this.expiry == null ? new Base[0] : new Base[] {this.expiry}; // DateTimeType 322 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 323 default: return super.getProperty(hash, name, checkValid); 324 } 325 326 } 327 328 @Override 329 public Base setProperty(int hash, String name, Base value) throws FHIRException { 330 switch (hash) { 331 case -1618432855: // identifier 332 this.identifier = castToIdentifier(value); // Identifier 333 return value; 334 case -1289159373: // expiry 335 this.expiry = castToDateTime(value); // DateTimeType 336 return value; 337 case -1285004149: // quantity 338 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 339 return value; 340 default: return super.setProperty(hash, name, value); 341 } 342 343 } 344 345 @Override 346 public Base setProperty(String name, Base value) throws FHIRException { 347 if (name.equals("identifier")) { 348 this.identifier = castToIdentifier(value); // Identifier 349 } else if (name.equals("expiry")) { 350 this.expiry = castToDateTime(value); // DateTimeType 351 } else if (name.equals("quantity")) { 352 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 353 } else 354 return super.setProperty(name, value); 355 return value; 356 } 357 358 @Override 359 public Base makeProperty(int hash, String name) throws FHIRException { 360 switch (hash) { 361 case -1618432855: return getIdentifier(); 362 case -1289159373: return getExpiryElement(); 363 case -1285004149: return getQuantity(); 364 default: return super.makeProperty(hash, name); 365 } 366 367 } 368 369 @Override 370 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 371 switch (hash) { 372 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 373 case -1289159373: /*expiry*/ return new String[] {"dateTime"}; 374 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 375 default: return super.getTypesForProperty(hash, name); 376 } 377 378 } 379 380 @Override 381 public Base addChild(String name) throws FHIRException { 382 if (name.equals("identifier")) { 383 this.identifier = new Identifier(); 384 return this.identifier; 385 } 386 else if (name.equals("expiry")) { 387 throw new FHIRException("Cannot call addChild on a singleton property Substance.expiry"); 388 } 389 else if (name.equals("quantity")) { 390 this.quantity = new SimpleQuantity(); 391 return this.quantity; 392 } 393 else 394 return super.addChild(name); 395 } 396 397 public SubstanceInstanceComponent copy() { 398 SubstanceInstanceComponent dst = new SubstanceInstanceComponent(); 399 copyValues(dst); 400 dst.identifier = identifier == null ? null : identifier.copy(); 401 dst.expiry = expiry == null ? null : expiry.copy(); 402 dst.quantity = quantity == null ? null : quantity.copy(); 403 return dst; 404 } 405 406 @Override 407 public boolean equalsDeep(Base other_) { 408 if (!super.equalsDeep(other_)) 409 return false; 410 if (!(other_ instanceof SubstanceInstanceComponent)) 411 return false; 412 SubstanceInstanceComponent o = (SubstanceInstanceComponent) other_; 413 return compareDeep(identifier, o.identifier, true) && compareDeep(expiry, o.expiry, true) && compareDeep(quantity, o.quantity, true) 414 ; 415 } 416 417 @Override 418 public boolean equalsShallow(Base other_) { 419 if (!super.equalsShallow(other_)) 420 return false; 421 if (!(other_ instanceof SubstanceInstanceComponent)) 422 return false; 423 SubstanceInstanceComponent o = (SubstanceInstanceComponent) other_; 424 return compareValues(expiry, o.expiry, true); 425 } 426 427 public boolean isEmpty() { 428 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, expiry, quantity 429 ); 430 } 431 432 public String fhirType() { 433 return "Substance.instance"; 434 435 } 436 437 } 438 439 @Block() 440 public static class SubstanceIngredientComponent extends BackboneElement implements IBaseBackboneElement { 441 /** 442 * The amount of the ingredient in the substance - a concentration ratio. 443 */ 444 @Child(name = "quantity", type = {Ratio.class}, order=1, min=0, max=1, modifier=false, summary=true) 445 @Description(shortDefinition="Optional amount (concentration)", formalDefinition="The amount of the ingredient in the substance - a concentration ratio." ) 446 protected Ratio quantity; 447 448 /** 449 * Another substance that is a component of this substance. 450 */ 451 @Child(name = "substance", type = {CodeableConcept.class, Substance.class}, order=2, min=1, max=1, modifier=false, summary=true) 452 @Description(shortDefinition="A component of the substance", formalDefinition="Another substance that is a component of this substance." ) 453 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-code") 454 protected Type substance; 455 456 private static final long serialVersionUID = -469805322L; 457 458 /** 459 * Constructor 460 */ 461 public SubstanceIngredientComponent() { 462 super(); 463 } 464 465 /** 466 * Constructor 467 */ 468 public SubstanceIngredientComponent(Type substance) { 469 super(); 470 this.substance = substance; 471 } 472 473 /** 474 * @return {@link #quantity} (The amount of the ingredient in the substance - a concentration ratio.) 475 */ 476 public Ratio getQuantity() { 477 if (this.quantity == null) 478 if (Configuration.errorOnAutoCreate()) 479 throw new Error("Attempt to auto-create SubstanceIngredientComponent.quantity"); 480 else if (Configuration.doAutoCreate()) 481 this.quantity = new Ratio(); // cc 482 return this.quantity; 483 } 484 485 public boolean hasQuantity() { 486 return this.quantity != null && !this.quantity.isEmpty(); 487 } 488 489 /** 490 * @param value {@link #quantity} (The amount of the ingredient in the substance - a concentration ratio.) 491 */ 492 public SubstanceIngredientComponent setQuantity(Ratio value) { 493 this.quantity = value; 494 return this; 495 } 496 497 /** 498 * @return {@link #substance} (Another substance that is a component of this substance.) 499 */ 500 public Type getSubstance() { 501 return this.substance; 502 } 503 504 /** 505 * @return {@link #substance} (Another substance that is a component of this substance.) 506 */ 507 public CodeableConcept getSubstanceCodeableConcept() throws FHIRException { 508 if (this.substance == null) 509 return null; 510 if (!(this.substance instanceof CodeableConcept)) 511 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.substance.getClass().getName()+" was encountered"); 512 return (CodeableConcept) this.substance; 513 } 514 515 public boolean hasSubstanceCodeableConcept() { 516 return this != null && this.substance instanceof CodeableConcept; 517 } 518 519 /** 520 * @return {@link #substance} (Another substance that is a component of this substance.) 521 */ 522 public Reference getSubstanceReference() throws FHIRException { 523 if (this.substance == null) 524 return null; 525 if (!(this.substance instanceof Reference)) 526 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.substance.getClass().getName()+" was encountered"); 527 return (Reference) this.substance; 528 } 529 530 public boolean hasSubstanceReference() { 531 return this != null && this.substance instanceof Reference; 532 } 533 534 public boolean hasSubstance() { 535 return this.substance != null && !this.substance.isEmpty(); 536 } 537 538 /** 539 * @param value {@link #substance} (Another substance that is a component of this substance.) 540 */ 541 public SubstanceIngredientComponent setSubstance(Type value) throws FHIRFormatError { 542 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 543 throw new FHIRFormatError("Not the right type for Substance.ingredient.substance[x]: "+value.fhirType()); 544 this.substance = value; 545 return this; 546 } 547 548 protected void listChildren(List<Property> children) { 549 super.listChildren(children); 550 children.add(new Property("quantity", "Ratio", "The amount of the ingredient in the substance - a concentration ratio.", 0, 1, quantity)); 551 children.add(new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance)); 552 } 553 554 @Override 555 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 556 switch (_hash) { 557 case -1285004149: /*quantity*/ return new Property("quantity", "Ratio", "The amount of the ingredient in the substance - a concentration ratio.", 0, 1, quantity); 558 case 2127194384: /*substance[x]*/ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 559 case 530040176: /*substance*/ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 560 case -1974119407: /*substanceCodeableConcept*/ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 561 case 516208571: /*substanceReference*/ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", "Another substance that is a component of this substance.", 0, 1, substance); 562 default: return super.getNamedProperty(_hash, _name, _checkValid); 563 } 564 565 } 566 567 @Override 568 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 569 switch (hash) { 570 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Ratio 571 case 530040176: /*substance*/ return this.substance == null ? new Base[0] : new Base[] {this.substance}; // Type 572 default: return super.getProperty(hash, name, checkValid); 573 } 574 575 } 576 577 @Override 578 public Base setProperty(int hash, String name, Base value) throws FHIRException { 579 switch (hash) { 580 case -1285004149: // quantity 581 this.quantity = castToRatio(value); // Ratio 582 return value; 583 case 530040176: // substance 584 this.substance = castToType(value); // Type 585 return value; 586 default: return super.setProperty(hash, name, value); 587 } 588 589 } 590 591 @Override 592 public Base setProperty(String name, Base value) throws FHIRException { 593 if (name.equals("quantity")) { 594 this.quantity = castToRatio(value); // Ratio 595 } else if (name.equals("substance[x]")) { 596 this.substance = castToType(value); // Type 597 } else 598 return super.setProperty(name, value); 599 return value; 600 } 601 602 @Override 603 public Base makeProperty(int hash, String name) throws FHIRException { 604 switch (hash) { 605 case -1285004149: return getQuantity(); 606 case 2127194384: return getSubstance(); 607 case 530040176: return getSubstance(); 608 default: return super.makeProperty(hash, name); 609 } 610 611 } 612 613 @Override 614 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 615 switch (hash) { 616 case -1285004149: /*quantity*/ return new String[] {"Ratio"}; 617 case 530040176: /*substance*/ return new String[] {"CodeableConcept", "Reference"}; 618 default: return super.getTypesForProperty(hash, name); 619 } 620 621 } 622 623 @Override 624 public Base addChild(String name) throws FHIRException { 625 if (name.equals("quantity")) { 626 this.quantity = new Ratio(); 627 return this.quantity; 628 } 629 else if (name.equals("substanceCodeableConcept")) { 630 this.substance = new CodeableConcept(); 631 return this.substance; 632 } 633 else if (name.equals("substanceReference")) { 634 this.substance = new Reference(); 635 return this.substance; 636 } 637 else 638 return super.addChild(name); 639 } 640 641 public SubstanceIngredientComponent copy() { 642 SubstanceIngredientComponent dst = new SubstanceIngredientComponent(); 643 copyValues(dst); 644 dst.quantity = quantity == null ? null : quantity.copy(); 645 dst.substance = substance == null ? null : substance.copy(); 646 return dst; 647 } 648 649 @Override 650 public boolean equalsDeep(Base other_) { 651 if (!super.equalsDeep(other_)) 652 return false; 653 if (!(other_ instanceof SubstanceIngredientComponent)) 654 return false; 655 SubstanceIngredientComponent o = (SubstanceIngredientComponent) other_; 656 return compareDeep(quantity, o.quantity, true) && compareDeep(substance, o.substance, true); 657 } 658 659 @Override 660 public boolean equalsShallow(Base other_) { 661 if (!super.equalsShallow(other_)) 662 return false; 663 if (!(other_ instanceof SubstanceIngredientComponent)) 664 return false; 665 SubstanceIngredientComponent o = (SubstanceIngredientComponent) other_; 666 return true; 667 } 668 669 public boolean isEmpty() { 670 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, substance); 671 } 672 673 public String fhirType() { 674 return "Substance.ingredient"; 675 676 } 677 678 } 679 680 /** 681 * Unique identifier for the substance. 682 */ 683 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 684 @Description(shortDefinition="Unique identifier", formalDefinition="Unique identifier for the substance." ) 685 protected List<Identifier> identifier; 686 687 /** 688 * A code to indicate if the substance is actively used. 689 */ 690 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 691 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="A code to indicate if the substance is actively used." ) 692 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-status") 693 protected Enumeration<FHIRSubstanceStatus> status; 694 695 /** 696 * A code that classifies the general type of substance. This is used for searching, sorting and display purposes. 697 */ 698 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 699 @Description(shortDefinition="What class/type of substance this is", formalDefinition="A code that classifies the general type of substance. This is used for searching, sorting and display purposes." ) 700 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-category") 701 protected List<CodeableConcept> category; 702 703 /** 704 * A code (or set of codes) that identify this substance. 705 */ 706 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 707 @Description(shortDefinition="What substance this is", formalDefinition="A code (or set of codes) that identify this substance." ) 708 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-code") 709 protected CodeableConcept code; 710 711 /** 712 * A description of the substance - its appearance, handling requirements, and other usage notes. 713 */ 714 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 715 @Description(shortDefinition="Textual description of the substance, comments", formalDefinition="A description of the substance - its appearance, handling requirements, and other usage notes." ) 716 protected StringType description; 717 718 /** 719 * Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance. 720 */ 721 @Child(name = "instance", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 722 @Description(shortDefinition="If this describes a specific package/container of the substance", formalDefinition="Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance." ) 723 protected List<SubstanceInstanceComponent> instance; 724 725 /** 726 * A substance can be composed of other substances. 727 */ 728 @Child(name = "ingredient", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 729 @Description(shortDefinition="Composition information about the substance", formalDefinition="A substance can be composed of other substances." ) 730 protected List<SubstanceIngredientComponent> ingredient; 731 732 private static final long serialVersionUID = -1467626602L; 733 734 /** 735 * Constructor 736 */ 737 public Substance() { 738 super(); 739 } 740 741 /** 742 * Constructor 743 */ 744 public Substance(CodeableConcept code) { 745 super(); 746 this.code = code; 747 } 748 749 /** 750 * @return {@link #identifier} (Unique identifier for the substance.) 751 */ 752 public List<Identifier> getIdentifier() { 753 if (this.identifier == null) 754 this.identifier = new ArrayList<Identifier>(); 755 return this.identifier; 756 } 757 758 /** 759 * @return Returns a reference to <code>this</code> for easy method chaining 760 */ 761 public Substance setIdentifier(List<Identifier> theIdentifier) { 762 this.identifier = theIdentifier; 763 return this; 764 } 765 766 public boolean hasIdentifier() { 767 if (this.identifier == null) 768 return false; 769 for (Identifier item : this.identifier) 770 if (!item.isEmpty()) 771 return true; 772 return false; 773 } 774 775 public Identifier addIdentifier() { //3 776 Identifier t = new Identifier(); 777 if (this.identifier == null) 778 this.identifier = new ArrayList<Identifier>(); 779 this.identifier.add(t); 780 return t; 781 } 782 783 public Substance addIdentifier(Identifier t) { //3 784 if (t == null) 785 return this; 786 if (this.identifier == null) 787 this.identifier = new ArrayList<Identifier>(); 788 this.identifier.add(t); 789 return this; 790 } 791 792 /** 793 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 794 */ 795 public Identifier getIdentifierFirstRep() { 796 if (getIdentifier().isEmpty()) { 797 addIdentifier(); 798 } 799 return getIdentifier().get(0); 800 } 801 802 /** 803 * @return {@link #status} (A code to indicate if the substance is actively used.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 804 */ 805 public Enumeration<FHIRSubstanceStatus> getStatusElement() { 806 if (this.status == null) 807 if (Configuration.errorOnAutoCreate()) 808 throw new Error("Attempt to auto-create Substance.status"); 809 else if (Configuration.doAutoCreate()) 810 this.status = new Enumeration<FHIRSubstanceStatus>(new FHIRSubstanceStatusEnumFactory()); // bb 811 return this.status; 812 } 813 814 public boolean hasStatusElement() { 815 return this.status != null && !this.status.isEmpty(); 816 } 817 818 public boolean hasStatus() { 819 return this.status != null && !this.status.isEmpty(); 820 } 821 822 /** 823 * @param value {@link #status} (A code to indicate if the substance is actively used.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 824 */ 825 public Substance setStatusElement(Enumeration<FHIRSubstanceStatus> value) { 826 this.status = value; 827 return this; 828 } 829 830 /** 831 * @return A code to indicate if the substance is actively used. 832 */ 833 public FHIRSubstanceStatus getStatus() { 834 return this.status == null ? null : this.status.getValue(); 835 } 836 837 /** 838 * @param value A code to indicate if the substance is actively used. 839 */ 840 public Substance setStatus(FHIRSubstanceStatus value) { 841 if (value == null) 842 this.status = null; 843 else { 844 if (this.status == null) 845 this.status = new Enumeration<FHIRSubstanceStatus>(new FHIRSubstanceStatusEnumFactory()); 846 this.status.setValue(value); 847 } 848 return this; 849 } 850 851 /** 852 * @return {@link #category} (A code that classifies the general type of substance. This is used for searching, sorting and display purposes.) 853 */ 854 public List<CodeableConcept> getCategory() { 855 if (this.category == null) 856 this.category = new ArrayList<CodeableConcept>(); 857 return this.category; 858 } 859 860 /** 861 * @return Returns a reference to <code>this</code> for easy method chaining 862 */ 863 public Substance setCategory(List<CodeableConcept> theCategory) { 864 this.category = theCategory; 865 return this; 866 } 867 868 public boolean hasCategory() { 869 if (this.category == null) 870 return false; 871 for (CodeableConcept item : this.category) 872 if (!item.isEmpty()) 873 return true; 874 return false; 875 } 876 877 public CodeableConcept addCategory() { //3 878 CodeableConcept t = new CodeableConcept(); 879 if (this.category == null) 880 this.category = new ArrayList<CodeableConcept>(); 881 this.category.add(t); 882 return t; 883 } 884 885 public Substance addCategory(CodeableConcept t) { //3 886 if (t == null) 887 return this; 888 if (this.category == null) 889 this.category = new ArrayList<CodeableConcept>(); 890 this.category.add(t); 891 return this; 892 } 893 894 /** 895 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 896 */ 897 public CodeableConcept getCategoryFirstRep() { 898 if (getCategory().isEmpty()) { 899 addCategory(); 900 } 901 return getCategory().get(0); 902 } 903 904 /** 905 * @return {@link #code} (A code (or set of codes) that identify this substance.) 906 */ 907 public CodeableConcept getCode() { 908 if (this.code == null) 909 if (Configuration.errorOnAutoCreate()) 910 throw new Error("Attempt to auto-create Substance.code"); 911 else if (Configuration.doAutoCreate()) 912 this.code = new CodeableConcept(); // cc 913 return this.code; 914 } 915 916 public boolean hasCode() { 917 return this.code != null && !this.code.isEmpty(); 918 } 919 920 /** 921 * @param value {@link #code} (A code (or set of codes) that identify this substance.) 922 */ 923 public Substance setCode(CodeableConcept value) { 924 this.code = value; 925 return this; 926 } 927 928 /** 929 * @return {@link #description} (A description of the substance - its appearance, handling requirements, and other usage notes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 930 */ 931 public StringType getDescriptionElement() { 932 if (this.description == null) 933 if (Configuration.errorOnAutoCreate()) 934 throw new Error("Attempt to auto-create Substance.description"); 935 else if (Configuration.doAutoCreate()) 936 this.description = new StringType(); // bb 937 return this.description; 938 } 939 940 public boolean hasDescriptionElement() { 941 return this.description != null && !this.description.isEmpty(); 942 } 943 944 public boolean hasDescription() { 945 return this.description != null && !this.description.isEmpty(); 946 } 947 948 /** 949 * @param value {@link #description} (A description of the substance - its appearance, handling requirements, and other usage notes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 950 */ 951 public Substance setDescriptionElement(StringType value) { 952 this.description = value; 953 return this; 954 } 955 956 /** 957 * @return A description of the substance - its appearance, handling requirements, and other usage notes. 958 */ 959 public String getDescription() { 960 return this.description == null ? null : this.description.getValue(); 961 } 962 963 /** 964 * @param value A description of the substance - its appearance, handling requirements, and other usage notes. 965 */ 966 public Substance setDescription(String value) { 967 if (Utilities.noString(value)) 968 this.description = null; 969 else { 970 if (this.description == null) 971 this.description = new StringType(); 972 this.description.setValue(value); 973 } 974 return this; 975 } 976 977 /** 978 * @return {@link #instance} (Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.) 979 */ 980 public List<SubstanceInstanceComponent> getInstance() { 981 if (this.instance == null) 982 this.instance = new ArrayList<SubstanceInstanceComponent>(); 983 return this.instance; 984 } 985 986 /** 987 * @return Returns a reference to <code>this</code> for easy method chaining 988 */ 989 public Substance setInstance(List<SubstanceInstanceComponent> theInstance) { 990 this.instance = theInstance; 991 return this; 992 } 993 994 public boolean hasInstance() { 995 if (this.instance == null) 996 return false; 997 for (SubstanceInstanceComponent item : this.instance) 998 if (!item.isEmpty()) 999 return true; 1000 return false; 1001 } 1002 1003 public SubstanceInstanceComponent addInstance() { //3 1004 SubstanceInstanceComponent t = new SubstanceInstanceComponent(); 1005 if (this.instance == null) 1006 this.instance = new ArrayList<SubstanceInstanceComponent>(); 1007 this.instance.add(t); 1008 return t; 1009 } 1010 1011 public Substance addInstance(SubstanceInstanceComponent t) { //3 1012 if (t == null) 1013 return this; 1014 if (this.instance == null) 1015 this.instance = new ArrayList<SubstanceInstanceComponent>(); 1016 this.instance.add(t); 1017 return this; 1018 } 1019 1020 /** 1021 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist 1022 */ 1023 public SubstanceInstanceComponent getInstanceFirstRep() { 1024 if (getInstance().isEmpty()) { 1025 addInstance(); 1026 } 1027 return getInstance().get(0); 1028 } 1029 1030 /** 1031 * @return {@link #ingredient} (A substance can be composed of other substances.) 1032 */ 1033 public List<SubstanceIngredientComponent> getIngredient() { 1034 if (this.ingredient == null) 1035 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1036 return this.ingredient; 1037 } 1038 1039 /** 1040 * @return Returns a reference to <code>this</code> for easy method chaining 1041 */ 1042 public Substance setIngredient(List<SubstanceIngredientComponent> theIngredient) { 1043 this.ingredient = theIngredient; 1044 return this; 1045 } 1046 1047 public boolean hasIngredient() { 1048 if (this.ingredient == null) 1049 return false; 1050 for (SubstanceIngredientComponent item : this.ingredient) 1051 if (!item.isEmpty()) 1052 return true; 1053 return false; 1054 } 1055 1056 public SubstanceIngredientComponent addIngredient() { //3 1057 SubstanceIngredientComponent t = new SubstanceIngredientComponent(); 1058 if (this.ingredient == null) 1059 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1060 this.ingredient.add(t); 1061 return t; 1062 } 1063 1064 public Substance addIngredient(SubstanceIngredientComponent t) { //3 1065 if (t == null) 1066 return this; 1067 if (this.ingredient == null) 1068 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1069 this.ingredient.add(t); 1070 return this; 1071 } 1072 1073 /** 1074 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist 1075 */ 1076 public SubstanceIngredientComponent getIngredientFirstRep() { 1077 if (getIngredient().isEmpty()) { 1078 addIngredient(); 1079 } 1080 return getIngredient().get(0); 1081 } 1082 1083 protected void listChildren(List<Property> children) { 1084 super.listChildren(children); 1085 children.add(new Property("identifier", "Identifier", "Unique identifier for the substance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1086 children.add(new Property("status", "code", "A code to indicate if the substance is actively used.", 0, 1, status)); 1087 children.add(new Property("category", "CodeableConcept", "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.", 0, java.lang.Integer.MAX_VALUE, category)); 1088 children.add(new Property("code", "CodeableConcept", "A code (or set of codes) that identify this substance.", 0, 1, code)); 1089 children.add(new Property("description", "string", "A description of the substance - its appearance, handling requirements, and other usage notes.", 0, 1, description)); 1090 children.add(new Property("instance", "", "Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.", 0, java.lang.Integer.MAX_VALUE, instance)); 1091 children.add(new Property("ingredient", "", "A substance can be composed of other substances.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 1092 } 1093 1094 @Override 1095 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1096 switch (_hash) { 1097 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for the substance.", 0, java.lang.Integer.MAX_VALUE, identifier); 1098 case -892481550: /*status*/ return new Property("status", "code", "A code to indicate if the substance is actively used.", 0, 1, status); 1099 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.", 0, java.lang.Integer.MAX_VALUE, category); 1100 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code (or set of codes) that identify this substance.", 0, 1, code); 1101 case -1724546052: /*description*/ return new Property("description", "string", "A description of the substance - its appearance, handling requirements, and other usage notes.", 0, 1, description); 1102 case 555127957: /*instance*/ return new Property("instance", "", "Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.", 0, java.lang.Integer.MAX_VALUE, instance); 1103 case -206409263: /*ingredient*/ return new Property("ingredient", "", "A substance can be composed of other substances.", 0, java.lang.Integer.MAX_VALUE, ingredient); 1104 default: return super.getNamedProperty(_hash, _name, _checkValid); 1105 } 1106 1107 } 1108 1109 @Override 1110 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1111 switch (hash) { 1112 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1113 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FHIRSubstanceStatus> 1114 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1115 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1116 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1117 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // SubstanceInstanceComponent 1118 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // SubstanceIngredientComponent 1119 default: return super.getProperty(hash, name, checkValid); 1120 } 1121 1122 } 1123 1124 @Override 1125 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1126 switch (hash) { 1127 case -1618432855: // identifier 1128 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1129 return value; 1130 case -892481550: // status 1131 value = new FHIRSubstanceStatusEnumFactory().fromType(castToCode(value)); 1132 this.status = (Enumeration) value; // Enumeration<FHIRSubstanceStatus> 1133 return value; 1134 case 50511102: // category 1135 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1136 return value; 1137 case 3059181: // code 1138 this.code = castToCodeableConcept(value); // CodeableConcept 1139 return value; 1140 case -1724546052: // description 1141 this.description = castToString(value); // StringType 1142 return value; 1143 case 555127957: // instance 1144 this.getInstance().add((SubstanceInstanceComponent) value); // SubstanceInstanceComponent 1145 return value; 1146 case -206409263: // ingredient 1147 this.getIngredient().add((SubstanceIngredientComponent) value); // SubstanceIngredientComponent 1148 return value; 1149 default: return super.setProperty(hash, name, value); 1150 } 1151 1152 } 1153 1154 @Override 1155 public Base setProperty(String name, Base value) throws FHIRException { 1156 if (name.equals("identifier")) { 1157 this.getIdentifier().add(castToIdentifier(value)); 1158 } else if (name.equals("status")) { 1159 value = new FHIRSubstanceStatusEnumFactory().fromType(castToCode(value)); 1160 this.status = (Enumeration) value; // Enumeration<FHIRSubstanceStatus> 1161 } else if (name.equals("category")) { 1162 this.getCategory().add(castToCodeableConcept(value)); 1163 } else if (name.equals("code")) { 1164 this.code = castToCodeableConcept(value); // CodeableConcept 1165 } else if (name.equals("description")) { 1166 this.description = castToString(value); // StringType 1167 } else if (name.equals("instance")) { 1168 this.getInstance().add((SubstanceInstanceComponent) value); 1169 } else if (name.equals("ingredient")) { 1170 this.getIngredient().add((SubstanceIngredientComponent) value); 1171 } else 1172 return super.setProperty(name, value); 1173 return value; 1174 } 1175 1176 @Override 1177 public Base makeProperty(int hash, String name) throws FHIRException { 1178 switch (hash) { 1179 case -1618432855: return addIdentifier(); 1180 case -892481550: return getStatusElement(); 1181 case 50511102: return addCategory(); 1182 case 3059181: return getCode(); 1183 case -1724546052: return getDescriptionElement(); 1184 case 555127957: return addInstance(); 1185 case -206409263: return addIngredient(); 1186 default: return super.makeProperty(hash, name); 1187 } 1188 1189 } 1190 1191 @Override 1192 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1193 switch (hash) { 1194 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1195 case -892481550: /*status*/ return new String[] {"code"}; 1196 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1197 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1198 case -1724546052: /*description*/ return new String[] {"string"}; 1199 case 555127957: /*instance*/ return new String[] {}; 1200 case -206409263: /*ingredient*/ return new String[] {}; 1201 default: return super.getTypesForProperty(hash, name); 1202 } 1203 1204 } 1205 1206 @Override 1207 public Base addChild(String name) throws FHIRException { 1208 if (name.equals("identifier")) { 1209 return addIdentifier(); 1210 } 1211 else if (name.equals("status")) { 1212 throw new FHIRException("Cannot call addChild on a singleton property Substance.status"); 1213 } 1214 else if (name.equals("category")) { 1215 return addCategory(); 1216 } 1217 else if (name.equals("code")) { 1218 this.code = new CodeableConcept(); 1219 return this.code; 1220 } 1221 else if (name.equals("description")) { 1222 throw new FHIRException("Cannot call addChild on a singleton property Substance.description"); 1223 } 1224 else if (name.equals("instance")) { 1225 return addInstance(); 1226 } 1227 else if (name.equals("ingredient")) { 1228 return addIngredient(); 1229 } 1230 else 1231 return super.addChild(name); 1232 } 1233 1234 public String fhirType() { 1235 return "Substance"; 1236 1237 } 1238 1239 public Substance copy() { 1240 Substance dst = new Substance(); 1241 copyValues(dst); 1242 if (identifier != null) { 1243 dst.identifier = new ArrayList<Identifier>(); 1244 for (Identifier i : identifier) 1245 dst.identifier.add(i.copy()); 1246 }; 1247 dst.status = status == null ? null : status.copy(); 1248 if (category != null) { 1249 dst.category = new ArrayList<CodeableConcept>(); 1250 for (CodeableConcept i : category) 1251 dst.category.add(i.copy()); 1252 }; 1253 dst.code = code == null ? null : code.copy(); 1254 dst.description = description == null ? null : description.copy(); 1255 if (instance != null) { 1256 dst.instance = new ArrayList<SubstanceInstanceComponent>(); 1257 for (SubstanceInstanceComponent i : instance) 1258 dst.instance.add(i.copy()); 1259 }; 1260 if (ingredient != null) { 1261 dst.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1262 for (SubstanceIngredientComponent i : ingredient) 1263 dst.ingredient.add(i.copy()); 1264 }; 1265 return dst; 1266 } 1267 1268 protected Substance typedCopy() { 1269 return copy(); 1270 } 1271 1272 @Override 1273 public boolean equalsDeep(Base other_) { 1274 if (!super.equalsDeep(other_)) 1275 return false; 1276 if (!(other_ instanceof Substance)) 1277 return false; 1278 Substance o = (Substance) other_; 1279 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1280 && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) && compareDeep(instance, o.instance, true) 1281 && compareDeep(ingredient, o.ingredient, true); 1282 } 1283 1284 @Override 1285 public boolean equalsShallow(Base other_) { 1286 if (!super.equalsShallow(other_)) 1287 return false; 1288 if (!(other_ instanceof Substance)) 1289 return false; 1290 Substance o = (Substance) other_; 1291 return compareValues(status, o.status, true) && compareValues(description, o.description, true); 1292 } 1293 1294 public boolean isEmpty() { 1295 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 1296 , code, description, instance, ingredient); 1297 } 1298 1299 @Override 1300 public ResourceType getResourceType() { 1301 return ResourceType.Substance; 1302 } 1303 1304 /** 1305 * Search parameter: <b>identifier</b> 1306 * <p> 1307 * Description: <b>Unique identifier for the substance</b><br> 1308 * Type: <b>token</b><br> 1309 * Path: <b>Substance.identifier</b><br> 1310 * </p> 1311 */ 1312 @SearchParamDefinition(name="identifier", path="Substance.identifier", description="Unique identifier for the substance", type="token" ) 1313 public static final String SP_IDENTIFIER = "identifier"; 1314 /** 1315 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1316 * <p> 1317 * Description: <b>Unique identifier for the substance</b><br> 1318 * Type: <b>token</b><br> 1319 * Path: <b>Substance.identifier</b><br> 1320 * </p> 1321 */ 1322 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1323 1324 /** 1325 * Search parameter: <b>container-identifier</b> 1326 * <p> 1327 * Description: <b>Identifier of the package/container</b><br> 1328 * Type: <b>token</b><br> 1329 * Path: <b>Substance.instance.identifier</b><br> 1330 * </p> 1331 */ 1332 @SearchParamDefinition(name="container-identifier", path="Substance.instance.identifier", description="Identifier of the package/container", type="token" ) 1333 public static final String SP_CONTAINER_IDENTIFIER = "container-identifier"; 1334 /** 1335 * <b>Fluent Client</b> search parameter constant for <b>container-identifier</b> 1336 * <p> 1337 * Description: <b>Identifier of the package/container</b><br> 1338 * Type: <b>token</b><br> 1339 * Path: <b>Substance.instance.identifier</b><br> 1340 * </p> 1341 */ 1342 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTAINER_IDENTIFIER); 1343 1344 /** 1345 * Search parameter: <b>code</b> 1346 * <p> 1347 * Description: <b>The code of the substance or ingredient</b><br> 1348 * Type: <b>token</b><br> 1349 * Path: <b>Substance.code, Substance.ingredient.substanceCodeableConcept</b><br> 1350 * </p> 1351 */ 1352 @SearchParamDefinition(name="code", path="Substance.code | Substance.ingredient.substance.as(CodeableConcept)", description="The code of the substance or ingredient", type="token" ) 1353 public static final String SP_CODE = "code"; 1354 /** 1355 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1356 * <p> 1357 * Description: <b>The code of the substance or ingredient</b><br> 1358 * Type: <b>token</b><br> 1359 * Path: <b>Substance.code, Substance.ingredient.substanceCodeableConcept</b><br> 1360 * </p> 1361 */ 1362 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1363 1364 /** 1365 * Search parameter: <b>quantity</b> 1366 * <p> 1367 * Description: <b>Amount of substance in the package</b><br> 1368 * Type: <b>quantity</b><br> 1369 * Path: <b>Substance.instance.quantity</b><br> 1370 * </p> 1371 */ 1372 @SearchParamDefinition(name="quantity", path="Substance.instance.quantity", description="Amount of substance in the package", type="quantity" ) 1373 public static final String SP_QUANTITY = "quantity"; 1374 /** 1375 * <b>Fluent Client</b> search parameter constant for <b>quantity</b> 1376 * <p> 1377 * Description: <b>Amount of substance in the package</b><br> 1378 * Type: <b>quantity</b><br> 1379 * Path: <b>Substance.instance.quantity</b><br> 1380 * </p> 1381 */ 1382 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_QUANTITY); 1383 1384 /** 1385 * Search parameter: <b>substance-reference</b> 1386 * <p> 1387 * Description: <b>A component of the substance</b><br> 1388 * Type: <b>reference</b><br> 1389 * Path: <b>Substance.ingredient.substanceReference</b><br> 1390 * </p> 1391 */ 1392 @SearchParamDefinition(name="substance-reference", path="Substance.ingredient.substance.as(Reference)", description="A component of the substance", type="reference", target={Substance.class } ) 1393 public static final String SP_SUBSTANCE_REFERENCE = "substance-reference"; 1394 /** 1395 * <b>Fluent Client</b> search parameter constant for <b>substance-reference</b> 1396 * <p> 1397 * Description: <b>A component of the substance</b><br> 1398 * Type: <b>reference</b><br> 1399 * Path: <b>Substance.ingredient.substanceReference</b><br> 1400 * </p> 1401 */ 1402 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSTANCE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSTANCE_REFERENCE); 1403 1404/** 1405 * Constant for fluent queries to be used to add include statements. Specifies 1406 * the path value of "<b>Substance:substance-reference</b>". 1407 */ 1408 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSTANCE_REFERENCE = new ca.uhn.fhir.model.api.Include("Substance:substance-reference").toLocked(); 1409 1410 /** 1411 * Search parameter: <b>expiry</b> 1412 * <p> 1413 * Description: <b>Expiry date of package or container of substance</b><br> 1414 * Type: <b>date</b><br> 1415 * Path: <b>Substance.instance.expiry</b><br> 1416 * </p> 1417 */ 1418 @SearchParamDefinition(name="expiry", path="Substance.instance.expiry", description="Expiry date of package or container of substance", type="date" ) 1419 public static final String SP_EXPIRY = "expiry"; 1420 /** 1421 * <b>Fluent Client</b> search parameter constant for <b>expiry</b> 1422 * <p> 1423 * Description: <b>Expiry date of package or container of substance</b><br> 1424 * Type: <b>date</b><br> 1425 * Path: <b>Substance.instance.expiry</b><br> 1426 * </p> 1427 */ 1428 public static final ca.uhn.fhir.rest.gclient.DateClientParam EXPIRY = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EXPIRY); 1429 1430 /** 1431 * Search parameter: <b>category</b> 1432 * <p> 1433 * Description: <b>The category of the substance</b><br> 1434 * Type: <b>token</b><br> 1435 * Path: <b>Substance.category</b><br> 1436 * </p> 1437 */ 1438 @SearchParamDefinition(name="category", path="Substance.category", description="The category of the substance", type="token" ) 1439 public static final String SP_CATEGORY = "category"; 1440 /** 1441 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1442 * <p> 1443 * Description: <b>The category of the substance</b><br> 1444 * Type: <b>token</b><br> 1445 * Path: <b>Substance.category</b><br> 1446 * </p> 1447 */ 1448 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1449 1450 /** 1451 * Search parameter: <b>status</b> 1452 * <p> 1453 * Description: <b>active | inactive | entered-in-error</b><br> 1454 * Type: <b>token</b><br> 1455 * Path: <b>Substance.status</b><br> 1456 * </p> 1457 */ 1458 @SearchParamDefinition(name="status", path="Substance.status", description="active | inactive | entered-in-error", type="token" ) 1459 public static final String SP_STATUS = "status"; 1460 /** 1461 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1462 * <p> 1463 * Description: <b>active | inactive | entered-in-error</b><br> 1464 * Type: <b>token</b><br> 1465 * Path: <b>Substance.status</b><br> 1466 * </p> 1467 */ 1468 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1469 1470 1471}