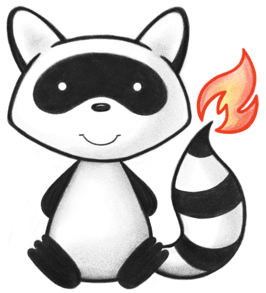
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.exceptions.FHIRFormatError; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * Record of delivery of what is supplied. 049 */ 050@ResourceDef(name="SupplyDelivery", profile="http://hl7.org/fhir/Profile/SupplyDelivery") 051public class SupplyDelivery extends DomainResource { 052 053 public enum SupplyDeliveryStatus { 054 /** 055 * Supply has been requested, but not delivered. 056 */ 057 INPROGRESS, 058 /** 059 * Supply has been delivered ("completed"). 060 */ 061 COMPLETED, 062 /** 063 * Delivery was not completed. 064 */ 065 ABANDONED, 066 /** 067 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 public static SupplyDeliveryStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("in-progress".equals(codeString)) 078 return INPROGRESS; 079 if ("completed".equals(codeString)) 080 return COMPLETED; 081 if ("abandoned".equals(codeString)) 082 return ABANDONED; 083 if ("entered-in-error".equals(codeString)) 084 return ENTEREDINERROR; 085 if (Configuration.isAcceptInvalidEnums()) 086 return null; 087 else 088 throw new FHIRException("Unknown SupplyDeliveryStatus code '"+codeString+"'"); 089 } 090 public String toCode() { 091 switch (this) { 092 case INPROGRESS: return "in-progress"; 093 case COMPLETED: return "completed"; 094 case ABANDONED: return "abandoned"; 095 case ENTEREDINERROR: return "entered-in-error"; 096 case NULL: return null; 097 default: return "?"; 098 } 099 } 100 public String getSystem() { 101 switch (this) { 102 case INPROGRESS: return "http://hl7.org/fhir/supplydelivery-status"; 103 case COMPLETED: return "http://hl7.org/fhir/supplydelivery-status"; 104 case ABANDONED: return "http://hl7.org/fhir/supplydelivery-status"; 105 case ENTEREDINERROR: return "http://hl7.org/fhir/supplydelivery-status"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getDefinition() { 111 switch (this) { 112 case INPROGRESS: return "Supply has been requested, but not delivered."; 113 case COMPLETED: return "Supply has been delivered (\"completed\")."; 114 case ABANDONED: return "Delivery was not completed."; 115 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getDisplay() { 121 switch (this) { 122 case INPROGRESS: return "In Progress"; 123 case COMPLETED: return "Delivered"; 124 case ABANDONED: return "Abandoned"; 125 case ENTEREDINERROR: return "Entered In Error"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 } 131 132 public static class SupplyDeliveryStatusEnumFactory implements EnumFactory<SupplyDeliveryStatus> { 133 public SupplyDeliveryStatus fromCode(String codeString) throws IllegalArgumentException { 134 if (codeString == null || "".equals(codeString)) 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("in-progress".equals(codeString)) 138 return SupplyDeliveryStatus.INPROGRESS; 139 if ("completed".equals(codeString)) 140 return SupplyDeliveryStatus.COMPLETED; 141 if ("abandoned".equals(codeString)) 142 return SupplyDeliveryStatus.ABANDONED; 143 if ("entered-in-error".equals(codeString)) 144 return SupplyDeliveryStatus.ENTEREDINERROR; 145 throw new IllegalArgumentException("Unknown SupplyDeliveryStatus code '"+codeString+"'"); 146 } 147 public Enumeration<SupplyDeliveryStatus> fromType(PrimitiveType<?> code) throws FHIRException { 148 if (code == null) 149 return null; 150 if (code.isEmpty()) 151 return new Enumeration<SupplyDeliveryStatus>(this); 152 String codeString = code.asStringValue(); 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("in-progress".equals(codeString)) 156 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.INPROGRESS); 157 if ("completed".equals(codeString)) 158 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.COMPLETED); 159 if ("abandoned".equals(codeString)) 160 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ABANDONED); 161 if ("entered-in-error".equals(codeString)) 162 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ENTEREDINERROR); 163 throw new FHIRException("Unknown SupplyDeliveryStatus code '"+codeString+"'"); 164 } 165 public String toCode(SupplyDeliveryStatus code) { 166 if (code == SupplyDeliveryStatus.NULL) 167 return null; 168 if (code == SupplyDeliveryStatus.INPROGRESS) 169 return "in-progress"; 170 if (code == SupplyDeliveryStatus.COMPLETED) 171 return "completed"; 172 if (code == SupplyDeliveryStatus.ABANDONED) 173 return "abandoned"; 174 if (code == SupplyDeliveryStatus.ENTEREDINERROR) 175 return "entered-in-error"; 176 return "?"; 177 } 178 public String toSystem(SupplyDeliveryStatus code) { 179 return code.getSystem(); 180 } 181 } 182 183 @Block() 184 public static class SupplyDeliverySuppliedItemComponent extends BackboneElement implements IBaseBackboneElement { 185 /** 186 * The amount of supply that has been dispensed. Includes unit of measure. 187 */ 188 @Child(name = "quantity", type = {SimpleQuantity.class}, order=1, min=0, max=1, modifier=false, summary=false) 189 @Description(shortDefinition="Amount dispensed", formalDefinition="The amount of supply that has been dispensed. Includes unit of measure." ) 190 protected SimpleQuantity quantity; 191 192 /** 193 * Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list. 194 */ 195 @Child(name = "item", type = {CodeableConcept.class, Medication.class, Substance.class, Device.class}, order=2, min=0, max=1, modifier=false, summary=false) 196 @Description(shortDefinition="Medication, Substance, or Device supplied", formalDefinition="Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list." ) 197 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supply-item") 198 protected Type item; 199 200 private static final long serialVersionUID = 80196045L; 201 202 /** 203 * Constructor 204 */ 205 public SupplyDeliverySuppliedItemComponent() { 206 super(); 207 } 208 209 /** 210 * @return {@link #quantity} (The amount of supply that has been dispensed. Includes unit of measure.) 211 */ 212 public SimpleQuantity getQuantity() { 213 if (this.quantity == null) 214 if (Configuration.errorOnAutoCreate()) 215 throw new Error("Attempt to auto-create SupplyDeliverySuppliedItemComponent.quantity"); 216 else if (Configuration.doAutoCreate()) 217 this.quantity = new SimpleQuantity(); // cc 218 return this.quantity; 219 } 220 221 public boolean hasQuantity() { 222 return this.quantity != null && !this.quantity.isEmpty(); 223 } 224 225 /** 226 * @param value {@link #quantity} (The amount of supply that has been dispensed. Includes unit of measure.) 227 */ 228 public SupplyDeliverySuppliedItemComponent setQuantity(SimpleQuantity value) { 229 this.quantity = value; 230 return this; 231 } 232 233 /** 234 * @return {@link #item} (Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 235 */ 236 public Type getItem() { 237 return this.item; 238 } 239 240 /** 241 * @return {@link #item} (Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 242 */ 243 public CodeableConcept getItemCodeableConcept() throws FHIRException { 244 if (this.item == null) 245 return null; 246 if (!(this.item instanceof CodeableConcept)) 247 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 248 return (CodeableConcept) this.item; 249 } 250 251 public boolean hasItemCodeableConcept() { 252 return this != null && this.item instanceof CodeableConcept; 253 } 254 255 /** 256 * @return {@link #item} (Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 257 */ 258 public Reference getItemReference() throws FHIRException { 259 if (this.item == null) 260 return null; 261 if (!(this.item instanceof Reference)) 262 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 263 return (Reference) this.item; 264 } 265 266 public boolean hasItemReference() { 267 return this != null && this.item instanceof Reference; 268 } 269 270 public boolean hasItem() { 271 return this.item != null && !this.item.isEmpty(); 272 } 273 274 /** 275 * @param value {@link #item} (Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 276 */ 277 public SupplyDeliverySuppliedItemComponent setItem(Type value) throws FHIRFormatError { 278 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 279 throw new FHIRFormatError("Not the right type for SupplyDelivery.suppliedItem.item[x]: "+value.fhirType()); 280 this.item = value; 281 return this; 282 } 283 284 protected void listChildren(List<Property> children) { 285 super.listChildren(children); 286 children.add(new Property("quantity", "SimpleQuantity", "The amount of supply that has been dispensed. Includes unit of measure.", 0, 1, quantity)); 287 children.add(new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item)); 288 } 289 290 @Override 291 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 292 switch (_hash) { 293 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The amount of supply that has been dispensed. Includes unit of measure.", 0, 1, quantity); 294 case 2116201613: /*item[x]*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 295 case 3242771: /*item*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 296 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 297 case 1376364920: /*itemReference*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 298 default: return super.getNamedProperty(_hash, _name, _checkValid); 299 } 300 301 } 302 303 @Override 304 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 305 switch (hash) { 306 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 307 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // Type 308 default: return super.getProperty(hash, name, checkValid); 309 } 310 311 } 312 313 @Override 314 public Base setProperty(int hash, String name, Base value) throws FHIRException { 315 switch (hash) { 316 case -1285004149: // quantity 317 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 318 return value; 319 case 3242771: // item 320 this.item = castToType(value); // Type 321 return value; 322 default: return super.setProperty(hash, name, value); 323 } 324 325 } 326 327 @Override 328 public Base setProperty(String name, Base value) throws FHIRException { 329 if (name.equals("quantity")) { 330 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 331 } else if (name.equals("item[x]")) { 332 this.item = castToType(value); // Type 333 } else 334 return super.setProperty(name, value); 335 return value; 336 } 337 338 @Override 339 public Base makeProperty(int hash, String name) throws FHIRException { 340 switch (hash) { 341 case -1285004149: return getQuantity(); 342 case 2116201613: return getItem(); 343 case 3242771: return getItem(); 344 default: return super.makeProperty(hash, name); 345 } 346 347 } 348 349 @Override 350 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 351 switch (hash) { 352 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 353 case 3242771: /*item*/ return new String[] {"CodeableConcept", "Reference"}; 354 default: return super.getTypesForProperty(hash, name); 355 } 356 357 } 358 359 @Override 360 public Base addChild(String name) throws FHIRException { 361 if (name.equals("quantity")) { 362 this.quantity = new SimpleQuantity(); 363 return this.quantity; 364 } 365 else if (name.equals("itemCodeableConcept")) { 366 this.item = new CodeableConcept(); 367 return this.item; 368 } 369 else if (name.equals("itemReference")) { 370 this.item = new Reference(); 371 return this.item; 372 } 373 else 374 return super.addChild(name); 375 } 376 377 public SupplyDeliverySuppliedItemComponent copy() { 378 SupplyDeliverySuppliedItemComponent dst = new SupplyDeliverySuppliedItemComponent(); 379 copyValues(dst); 380 dst.quantity = quantity == null ? null : quantity.copy(); 381 dst.item = item == null ? null : item.copy(); 382 return dst; 383 } 384 385 @Override 386 public boolean equalsDeep(Base other_) { 387 if (!super.equalsDeep(other_)) 388 return false; 389 if (!(other_ instanceof SupplyDeliverySuppliedItemComponent)) 390 return false; 391 SupplyDeliverySuppliedItemComponent o = (SupplyDeliverySuppliedItemComponent) other_; 392 return compareDeep(quantity, o.quantity, true) && compareDeep(item, o.item, true); 393 } 394 395 @Override 396 public boolean equalsShallow(Base other_) { 397 if (!super.equalsShallow(other_)) 398 return false; 399 if (!(other_ instanceof SupplyDeliverySuppliedItemComponent)) 400 return false; 401 SupplyDeliverySuppliedItemComponent o = (SupplyDeliverySuppliedItemComponent) other_; 402 return true; 403 } 404 405 public boolean isEmpty() { 406 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, item); 407 } 408 409 public String fhirType() { 410 return "SupplyDelivery.suppliedItem"; 411 412 } 413 414 } 415 416 /** 417 * Identifier assigned by the dispensing facility when the item(s) is dispensed. 418 */ 419 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=false) 420 @Description(shortDefinition="External identifier", formalDefinition="Identifier assigned by the dispensing facility when the item(s) is dispensed." ) 421 protected Identifier identifier; 422 423 /** 424 * A plan, proposal or order that is fulfilled in whole or in part by this event. 425 */ 426 @Child(name = "basedOn", type = {SupplyRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 427 @Description(shortDefinition="Fulfills plan, proposal or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this event." ) 428 protected List<Reference> basedOn; 429 /** 430 * The actual objects that are the target of the reference (A plan, proposal or order that is fulfilled in whole or in part by this event.) 431 */ 432 protected List<SupplyRequest> basedOnTarget; 433 434 435 /** 436 * A larger event of which this particular event is a component or step. 437 */ 438 @Child(name = "partOf", type = {SupplyDelivery.class, Contract.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 439 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular event is a component or step." ) 440 protected List<Reference> partOf; 441 /** 442 * The actual objects that are the target of the reference (A larger event of which this particular event is a component or step.) 443 */ 444 protected List<Resource> partOfTarget; 445 446 447 /** 448 * A code specifying the state of the dispense event. 449 */ 450 @Child(name = "status", type = {CodeType.class}, order=3, min=0, max=1, modifier=true, summary=true) 451 @Description(shortDefinition="in-progress | completed | abandoned | entered-in-error", formalDefinition="A code specifying the state of the dispense event." ) 452 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplydelivery-status") 453 protected Enumeration<SupplyDeliveryStatus> status; 454 455 /** 456 * A link to a resource representing the person whom the delivered item is for. 457 */ 458 @Child(name = "patient", type = {Patient.class}, order=4, min=0, max=1, modifier=false, summary=false) 459 @Description(shortDefinition="Patient for whom the item is supplied", formalDefinition="A link to a resource representing the person whom the delivered item is for." ) 460 protected Reference patient; 461 462 /** 463 * The actual object that is the target of the reference (A link to a resource representing the person whom the delivered item is for.) 464 */ 465 protected Patient patientTarget; 466 467 /** 468 * Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc. 469 */ 470 @Child(name = "type", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 471 @Description(shortDefinition="Category of dispense event", formalDefinition="Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc." ) 472 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplydelivery-type") 473 protected CodeableConcept type; 474 475 /** 476 * The item that is being delivered or has been supplied. 477 */ 478 @Child(name = "suppliedItem", type = {}, order=6, min=0, max=1, modifier=false, summary=false) 479 @Description(shortDefinition="The item that is delivered or supplied", formalDefinition="The item that is being delivered or has been supplied." ) 480 protected SupplyDeliverySuppliedItemComponent suppliedItem; 481 482 /** 483 * The date or time(s) the activity occurred. 484 */ 485 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=7, min=0, max=1, modifier=false, summary=true) 486 @Description(shortDefinition="When event occurred", formalDefinition="The date or time(s) the activity occurred." ) 487 protected Type occurrence; 488 489 /** 490 * The individual responsible for dispensing the medication, supplier or device. 491 */ 492 @Child(name = "supplier", type = {Practitioner.class, Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 493 @Description(shortDefinition="Dispenser", formalDefinition="The individual responsible for dispensing the medication, supplier or device." ) 494 protected Reference supplier; 495 496 /** 497 * The actual object that is the target of the reference (The individual responsible for dispensing the medication, supplier or device.) 498 */ 499 protected Resource supplierTarget; 500 501 /** 502 * Identification of the facility/location where the Supply was shipped to, as part of the dispense event. 503 */ 504 @Child(name = "destination", type = {Location.class}, order=9, min=0, max=1, modifier=false, summary=false) 505 @Description(shortDefinition="Where the Supply was sent", formalDefinition="Identification of the facility/location where the Supply was shipped to, as part of the dispense event." ) 506 protected Reference destination; 507 508 /** 509 * The actual object that is the target of the reference (Identification of the facility/location where the Supply was shipped to, as part of the dispense event.) 510 */ 511 protected Location destinationTarget; 512 513 /** 514 * Identifies the person who picked up the Supply. 515 */ 516 @Child(name = "receiver", type = {Practitioner.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 517 @Description(shortDefinition="Who collected the Supply", formalDefinition="Identifies the person who picked up the Supply." ) 518 protected List<Reference> receiver; 519 /** 520 * The actual objects that are the target of the reference (Identifies the person who picked up the Supply.) 521 */ 522 protected List<Practitioner> receiverTarget; 523 524 525 private static final long serialVersionUID = 2033462996L; 526 527 /** 528 * Constructor 529 */ 530 public SupplyDelivery() { 531 super(); 532 } 533 534 /** 535 * @return {@link #identifier} (Identifier assigned by the dispensing facility when the item(s) is dispensed.) 536 */ 537 public Identifier getIdentifier() { 538 if (this.identifier == null) 539 if (Configuration.errorOnAutoCreate()) 540 throw new Error("Attempt to auto-create SupplyDelivery.identifier"); 541 else if (Configuration.doAutoCreate()) 542 this.identifier = new Identifier(); // cc 543 return this.identifier; 544 } 545 546 public boolean hasIdentifier() { 547 return this.identifier != null && !this.identifier.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #identifier} (Identifier assigned by the dispensing facility when the item(s) is dispensed.) 552 */ 553 public SupplyDelivery setIdentifier(Identifier value) { 554 this.identifier = value; 555 return this; 556 } 557 558 /** 559 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this event.) 560 */ 561 public List<Reference> getBasedOn() { 562 if (this.basedOn == null) 563 this.basedOn = new ArrayList<Reference>(); 564 return this.basedOn; 565 } 566 567 /** 568 * @return Returns a reference to <code>this</code> for easy method chaining 569 */ 570 public SupplyDelivery setBasedOn(List<Reference> theBasedOn) { 571 this.basedOn = theBasedOn; 572 return this; 573 } 574 575 public boolean hasBasedOn() { 576 if (this.basedOn == null) 577 return false; 578 for (Reference item : this.basedOn) 579 if (!item.isEmpty()) 580 return true; 581 return false; 582 } 583 584 public Reference addBasedOn() { //3 585 Reference t = new Reference(); 586 if (this.basedOn == null) 587 this.basedOn = new ArrayList<Reference>(); 588 this.basedOn.add(t); 589 return t; 590 } 591 592 public SupplyDelivery addBasedOn(Reference t) { //3 593 if (t == null) 594 return this; 595 if (this.basedOn == null) 596 this.basedOn = new ArrayList<Reference>(); 597 this.basedOn.add(t); 598 return this; 599 } 600 601 /** 602 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 603 */ 604 public Reference getBasedOnFirstRep() { 605 if (getBasedOn().isEmpty()) { 606 addBasedOn(); 607 } 608 return getBasedOn().get(0); 609 } 610 611 /** 612 * @deprecated Use Reference#setResource(IBaseResource) instead 613 */ 614 @Deprecated 615 public List<SupplyRequest> getBasedOnTarget() { 616 if (this.basedOnTarget == null) 617 this.basedOnTarget = new ArrayList<SupplyRequest>(); 618 return this.basedOnTarget; 619 } 620 621 /** 622 * @deprecated Use Reference#setResource(IBaseResource) instead 623 */ 624 @Deprecated 625 public SupplyRequest addBasedOnTarget() { 626 SupplyRequest r = new SupplyRequest(); 627 if (this.basedOnTarget == null) 628 this.basedOnTarget = new ArrayList<SupplyRequest>(); 629 this.basedOnTarget.add(r); 630 return r; 631 } 632 633 /** 634 * @return {@link #partOf} (A larger event of which this particular event is a component or step.) 635 */ 636 public List<Reference> getPartOf() { 637 if (this.partOf == null) 638 this.partOf = new ArrayList<Reference>(); 639 return this.partOf; 640 } 641 642 /** 643 * @return Returns a reference to <code>this</code> for easy method chaining 644 */ 645 public SupplyDelivery setPartOf(List<Reference> thePartOf) { 646 this.partOf = thePartOf; 647 return this; 648 } 649 650 public boolean hasPartOf() { 651 if (this.partOf == null) 652 return false; 653 for (Reference item : this.partOf) 654 if (!item.isEmpty()) 655 return true; 656 return false; 657 } 658 659 public Reference addPartOf() { //3 660 Reference t = new Reference(); 661 if (this.partOf == null) 662 this.partOf = new ArrayList<Reference>(); 663 this.partOf.add(t); 664 return t; 665 } 666 667 public SupplyDelivery addPartOf(Reference t) { //3 668 if (t == null) 669 return this; 670 if (this.partOf == null) 671 this.partOf = new ArrayList<Reference>(); 672 this.partOf.add(t); 673 return this; 674 } 675 676 /** 677 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 678 */ 679 public Reference getPartOfFirstRep() { 680 if (getPartOf().isEmpty()) { 681 addPartOf(); 682 } 683 return getPartOf().get(0); 684 } 685 686 /** 687 * @deprecated Use Reference#setResource(IBaseResource) instead 688 */ 689 @Deprecated 690 public List<Resource> getPartOfTarget() { 691 if (this.partOfTarget == null) 692 this.partOfTarget = new ArrayList<Resource>(); 693 return this.partOfTarget; 694 } 695 696 /** 697 * @return {@link #status} (A code specifying the state of the dispense event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 698 */ 699 public Enumeration<SupplyDeliveryStatus> getStatusElement() { 700 if (this.status == null) 701 if (Configuration.errorOnAutoCreate()) 702 throw new Error("Attempt to auto-create SupplyDelivery.status"); 703 else if (Configuration.doAutoCreate()) 704 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); // bb 705 return this.status; 706 } 707 708 public boolean hasStatusElement() { 709 return this.status != null && !this.status.isEmpty(); 710 } 711 712 public boolean hasStatus() { 713 return this.status != null && !this.status.isEmpty(); 714 } 715 716 /** 717 * @param value {@link #status} (A code specifying the state of the dispense event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 718 */ 719 public SupplyDelivery setStatusElement(Enumeration<SupplyDeliveryStatus> value) { 720 this.status = value; 721 return this; 722 } 723 724 /** 725 * @return A code specifying the state of the dispense event. 726 */ 727 public SupplyDeliveryStatus getStatus() { 728 return this.status == null ? null : this.status.getValue(); 729 } 730 731 /** 732 * @param value A code specifying the state of the dispense event. 733 */ 734 public SupplyDelivery setStatus(SupplyDeliveryStatus value) { 735 if (value == null) 736 this.status = null; 737 else { 738 if (this.status == null) 739 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); 740 this.status.setValue(value); 741 } 742 return this; 743 } 744 745 /** 746 * @return {@link #patient} (A link to a resource representing the person whom the delivered item is for.) 747 */ 748 public Reference getPatient() { 749 if (this.patient == null) 750 if (Configuration.errorOnAutoCreate()) 751 throw new Error("Attempt to auto-create SupplyDelivery.patient"); 752 else if (Configuration.doAutoCreate()) 753 this.patient = new Reference(); // cc 754 return this.patient; 755 } 756 757 public boolean hasPatient() { 758 return this.patient != null && !this.patient.isEmpty(); 759 } 760 761 /** 762 * @param value {@link #patient} (A link to a resource representing the person whom the delivered item is for.) 763 */ 764 public SupplyDelivery setPatient(Reference value) { 765 this.patient = value; 766 return this; 767 } 768 769 /** 770 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A link to a resource representing the person whom the delivered item is for.) 771 */ 772 public Patient getPatientTarget() { 773 if (this.patientTarget == null) 774 if (Configuration.errorOnAutoCreate()) 775 throw new Error("Attempt to auto-create SupplyDelivery.patient"); 776 else if (Configuration.doAutoCreate()) 777 this.patientTarget = new Patient(); // aa 778 return this.patientTarget; 779 } 780 781 /** 782 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A link to a resource representing the person whom the delivered item is for.) 783 */ 784 public SupplyDelivery setPatientTarget(Patient value) { 785 this.patientTarget = value; 786 return this; 787 } 788 789 /** 790 * @return {@link #type} (Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.) 791 */ 792 public CodeableConcept getType() { 793 if (this.type == null) 794 if (Configuration.errorOnAutoCreate()) 795 throw new Error("Attempt to auto-create SupplyDelivery.type"); 796 else if (Configuration.doAutoCreate()) 797 this.type = new CodeableConcept(); // cc 798 return this.type; 799 } 800 801 public boolean hasType() { 802 return this.type != null && !this.type.isEmpty(); 803 } 804 805 /** 806 * @param value {@link #type} (Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.) 807 */ 808 public SupplyDelivery setType(CodeableConcept value) { 809 this.type = value; 810 return this; 811 } 812 813 /** 814 * @return {@link #suppliedItem} (The item that is being delivered or has been supplied.) 815 */ 816 public SupplyDeliverySuppliedItemComponent getSuppliedItem() { 817 if (this.suppliedItem == null) 818 if (Configuration.errorOnAutoCreate()) 819 throw new Error("Attempt to auto-create SupplyDelivery.suppliedItem"); 820 else if (Configuration.doAutoCreate()) 821 this.suppliedItem = new SupplyDeliverySuppliedItemComponent(); // cc 822 return this.suppliedItem; 823 } 824 825 public boolean hasSuppliedItem() { 826 return this.suppliedItem != null && !this.suppliedItem.isEmpty(); 827 } 828 829 /** 830 * @param value {@link #suppliedItem} (The item that is being delivered or has been supplied.) 831 */ 832 public SupplyDelivery setSuppliedItem(SupplyDeliverySuppliedItemComponent value) { 833 this.suppliedItem = value; 834 return this; 835 } 836 837 /** 838 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 839 */ 840 public Type getOccurrence() { 841 return this.occurrence; 842 } 843 844 /** 845 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 846 */ 847 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 848 if (this.occurrence == null) 849 return null; 850 if (!(this.occurrence instanceof DateTimeType)) 851 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 852 return (DateTimeType) this.occurrence; 853 } 854 855 public boolean hasOccurrenceDateTimeType() { 856 return this != null && this.occurrence instanceof DateTimeType; 857 } 858 859 /** 860 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 861 */ 862 public Period getOccurrencePeriod() throws FHIRException { 863 if (this.occurrence == null) 864 return null; 865 if (!(this.occurrence instanceof Period)) 866 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 867 return (Period) this.occurrence; 868 } 869 870 public boolean hasOccurrencePeriod() { 871 return this != null && this.occurrence instanceof Period; 872 } 873 874 /** 875 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 876 */ 877 public Timing getOccurrenceTiming() throws FHIRException { 878 if (this.occurrence == null) 879 return null; 880 if (!(this.occurrence instanceof Timing)) 881 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 882 return (Timing) this.occurrence; 883 } 884 885 public boolean hasOccurrenceTiming() { 886 return this != null && this.occurrence instanceof Timing; 887 } 888 889 public boolean hasOccurrence() { 890 return this.occurrence != null && !this.occurrence.isEmpty(); 891 } 892 893 /** 894 * @param value {@link #occurrence} (The date or time(s) the activity occurred.) 895 */ 896 public SupplyDelivery setOccurrence(Type value) throws FHIRFormatError { 897 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 898 throw new FHIRFormatError("Not the right type for SupplyDelivery.occurrence[x]: "+value.fhirType()); 899 this.occurrence = value; 900 return this; 901 } 902 903 /** 904 * @return {@link #supplier} (The individual responsible for dispensing the medication, supplier or device.) 905 */ 906 public Reference getSupplier() { 907 if (this.supplier == null) 908 if (Configuration.errorOnAutoCreate()) 909 throw new Error("Attempt to auto-create SupplyDelivery.supplier"); 910 else if (Configuration.doAutoCreate()) 911 this.supplier = new Reference(); // cc 912 return this.supplier; 913 } 914 915 public boolean hasSupplier() { 916 return this.supplier != null && !this.supplier.isEmpty(); 917 } 918 919 /** 920 * @param value {@link #supplier} (The individual responsible for dispensing the medication, supplier or device.) 921 */ 922 public SupplyDelivery setSupplier(Reference value) { 923 this.supplier = value; 924 return this; 925 } 926 927 /** 928 * @return {@link #supplier} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The individual responsible for dispensing the medication, supplier or device.) 929 */ 930 public Resource getSupplierTarget() { 931 return this.supplierTarget; 932 } 933 934 /** 935 * @param value {@link #supplier} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The individual responsible for dispensing the medication, supplier or device.) 936 */ 937 public SupplyDelivery setSupplierTarget(Resource value) { 938 this.supplierTarget = value; 939 return this; 940 } 941 942 /** 943 * @return {@link #destination} (Identification of the facility/location where the Supply was shipped to, as part of the dispense event.) 944 */ 945 public Reference getDestination() { 946 if (this.destination == null) 947 if (Configuration.errorOnAutoCreate()) 948 throw new Error("Attempt to auto-create SupplyDelivery.destination"); 949 else if (Configuration.doAutoCreate()) 950 this.destination = new Reference(); // cc 951 return this.destination; 952 } 953 954 public boolean hasDestination() { 955 return this.destination != null && !this.destination.isEmpty(); 956 } 957 958 /** 959 * @param value {@link #destination} (Identification of the facility/location where the Supply was shipped to, as part of the dispense event.) 960 */ 961 public SupplyDelivery setDestination(Reference value) { 962 this.destination = value; 963 return this; 964 } 965 966 /** 967 * @return {@link #destination} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identification of the facility/location where the Supply was shipped to, as part of the dispense event.) 968 */ 969 public Location getDestinationTarget() { 970 if (this.destinationTarget == null) 971 if (Configuration.errorOnAutoCreate()) 972 throw new Error("Attempt to auto-create SupplyDelivery.destination"); 973 else if (Configuration.doAutoCreate()) 974 this.destinationTarget = new Location(); // aa 975 return this.destinationTarget; 976 } 977 978 /** 979 * @param value {@link #destination} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identification of the facility/location where the Supply was shipped to, as part of the dispense event.) 980 */ 981 public SupplyDelivery setDestinationTarget(Location value) { 982 this.destinationTarget = value; 983 return this; 984 } 985 986 /** 987 * @return {@link #receiver} (Identifies the person who picked up the Supply.) 988 */ 989 public List<Reference> getReceiver() { 990 if (this.receiver == null) 991 this.receiver = new ArrayList<Reference>(); 992 return this.receiver; 993 } 994 995 /** 996 * @return Returns a reference to <code>this</code> for easy method chaining 997 */ 998 public SupplyDelivery setReceiver(List<Reference> theReceiver) { 999 this.receiver = theReceiver; 1000 return this; 1001 } 1002 1003 public boolean hasReceiver() { 1004 if (this.receiver == null) 1005 return false; 1006 for (Reference item : this.receiver) 1007 if (!item.isEmpty()) 1008 return true; 1009 return false; 1010 } 1011 1012 public Reference addReceiver() { //3 1013 Reference t = new Reference(); 1014 if (this.receiver == null) 1015 this.receiver = new ArrayList<Reference>(); 1016 this.receiver.add(t); 1017 return t; 1018 } 1019 1020 public SupplyDelivery addReceiver(Reference t) { //3 1021 if (t == null) 1022 return this; 1023 if (this.receiver == null) 1024 this.receiver = new ArrayList<Reference>(); 1025 this.receiver.add(t); 1026 return this; 1027 } 1028 1029 /** 1030 * @return The first repetition of repeating field {@link #receiver}, creating it if it does not already exist 1031 */ 1032 public Reference getReceiverFirstRep() { 1033 if (getReceiver().isEmpty()) { 1034 addReceiver(); 1035 } 1036 return getReceiver().get(0); 1037 } 1038 1039 /** 1040 * @deprecated Use Reference#setResource(IBaseResource) instead 1041 */ 1042 @Deprecated 1043 public List<Practitioner> getReceiverTarget() { 1044 if (this.receiverTarget == null) 1045 this.receiverTarget = new ArrayList<Practitioner>(); 1046 return this.receiverTarget; 1047 } 1048 1049 /** 1050 * @deprecated Use Reference#setResource(IBaseResource) instead 1051 */ 1052 @Deprecated 1053 public Practitioner addReceiverTarget() { 1054 Practitioner r = new Practitioner(); 1055 if (this.receiverTarget == null) 1056 this.receiverTarget = new ArrayList<Practitioner>(); 1057 this.receiverTarget.add(r); 1058 return r; 1059 } 1060 1061 protected void listChildren(List<Property> children) { 1062 super.listChildren(children); 1063 children.add(new Property("identifier", "Identifier", "Identifier assigned by the dispensing facility when the item(s) is dispensed.", 0, 1, identifier)); 1064 children.add(new Property("basedOn", "Reference(SupplyRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1065 children.add(new Property("partOf", "Reference(SupplyDelivery|Contract)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1066 children.add(new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, status)); 1067 children.add(new Property("patient", "Reference(Patient)", "A link to a resource representing the person whom the delivered item is for.", 0, 1, patient)); 1068 children.add(new Property("type", "CodeableConcept", "Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 0, 1, type)); 1069 children.add(new Property("suppliedItem", "", "The item that is being delivered or has been supplied.", 0, 1, suppliedItem)); 1070 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence)); 1071 children.add(new Property("supplier", "Reference(Practitioner|Organization)", "The individual responsible for dispensing the medication, supplier or device.", 0, 1, supplier)); 1072 children.add(new Property("destination", "Reference(Location)", "Identification of the facility/location where the Supply was shipped to, as part of the dispense event.", 0, 1, destination)); 1073 children.add(new Property("receiver", "Reference(Practitioner)", "Identifies the person who picked up the Supply.", 0, java.lang.Integer.MAX_VALUE, receiver)); 1074 } 1075 1076 @Override 1077 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1078 switch (_hash) { 1079 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier assigned by the dispensing facility when the item(s) is dispensed.", 0, 1, identifier); 1080 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(SupplyRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1081 case -995410646: /*partOf*/ return new Property("partOf", "Reference(SupplyDelivery|Contract)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 1082 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, status); 1083 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "A link to a resource representing the person whom the delivered item is for.", 0, 1, patient); 1084 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 0, 1, type); 1085 case 1993333233: /*suppliedItem*/ return new Property("suppliedItem", "", "The item that is being delivered or has been supplied.", 0, 1, suppliedItem); 1086 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1087 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1088 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1089 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1090 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 0, 1, occurrence); 1091 case -1663305268: /*supplier*/ return new Property("supplier", "Reference(Practitioner|Organization)", "The individual responsible for dispensing the medication, supplier or device.", 0, 1, supplier); 1092 case -1429847026: /*destination*/ return new Property("destination", "Reference(Location)", "Identification of the facility/location where the Supply was shipped to, as part of the dispense event.", 0, 1, destination); 1093 case -808719889: /*receiver*/ return new Property("receiver", "Reference(Practitioner)", "Identifies the person who picked up the Supply.", 0, java.lang.Integer.MAX_VALUE, receiver); 1094 default: return super.getNamedProperty(_hash, _name, _checkValid); 1095 } 1096 1097 } 1098 1099 @Override 1100 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1101 switch (hash) { 1102 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1103 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1104 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1105 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SupplyDeliveryStatus> 1106 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1107 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1108 case 1993333233: /*suppliedItem*/ return this.suppliedItem == null ? new Base[0] : new Base[] {this.suppliedItem}; // SupplyDeliverySuppliedItemComponent 1109 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 1110 case -1663305268: /*supplier*/ return this.supplier == null ? new Base[0] : new Base[] {this.supplier}; // Reference 1111 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // Reference 1112 case -808719889: /*receiver*/ return this.receiver == null ? new Base[0] : this.receiver.toArray(new Base[this.receiver.size()]); // Reference 1113 default: return super.getProperty(hash, name, checkValid); 1114 } 1115 1116 } 1117 1118 @Override 1119 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1120 switch (hash) { 1121 case -1618432855: // identifier 1122 this.identifier = castToIdentifier(value); // Identifier 1123 return value; 1124 case -332612366: // basedOn 1125 this.getBasedOn().add(castToReference(value)); // Reference 1126 return value; 1127 case -995410646: // partOf 1128 this.getPartOf().add(castToReference(value)); // Reference 1129 return value; 1130 case -892481550: // status 1131 value = new SupplyDeliveryStatusEnumFactory().fromType(castToCode(value)); 1132 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1133 return value; 1134 case -791418107: // patient 1135 this.patient = castToReference(value); // Reference 1136 return value; 1137 case 3575610: // type 1138 this.type = castToCodeableConcept(value); // CodeableConcept 1139 return value; 1140 case 1993333233: // suppliedItem 1141 this.suppliedItem = (SupplyDeliverySuppliedItemComponent) value; // SupplyDeliverySuppliedItemComponent 1142 return value; 1143 case 1687874001: // occurrence 1144 this.occurrence = castToType(value); // Type 1145 return value; 1146 case -1663305268: // supplier 1147 this.supplier = castToReference(value); // Reference 1148 return value; 1149 case -1429847026: // destination 1150 this.destination = castToReference(value); // Reference 1151 return value; 1152 case -808719889: // receiver 1153 this.getReceiver().add(castToReference(value)); // Reference 1154 return value; 1155 default: return super.setProperty(hash, name, value); 1156 } 1157 1158 } 1159 1160 @Override 1161 public Base setProperty(String name, Base value) throws FHIRException { 1162 if (name.equals("identifier")) { 1163 this.identifier = castToIdentifier(value); // Identifier 1164 } else if (name.equals("basedOn")) { 1165 this.getBasedOn().add(castToReference(value)); 1166 } else if (name.equals("partOf")) { 1167 this.getPartOf().add(castToReference(value)); 1168 } else if (name.equals("status")) { 1169 value = new SupplyDeliveryStatusEnumFactory().fromType(castToCode(value)); 1170 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1171 } else if (name.equals("patient")) { 1172 this.patient = castToReference(value); // Reference 1173 } else if (name.equals("type")) { 1174 this.type = castToCodeableConcept(value); // CodeableConcept 1175 } else if (name.equals("suppliedItem")) { 1176 this.suppliedItem = (SupplyDeliverySuppliedItemComponent) value; // SupplyDeliverySuppliedItemComponent 1177 } else if (name.equals("occurrence[x]")) { 1178 this.occurrence = castToType(value); // Type 1179 } else if (name.equals("supplier")) { 1180 this.supplier = castToReference(value); // Reference 1181 } else if (name.equals("destination")) { 1182 this.destination = castToReference(value); // Reference 1183 } else if (name.equals("receiver")) { 1184 this.getReceiver().add(castToReference(value)); 1185 } else 1186 return super.setProperty(name, value); 1187 return value; 1188 } 1189 1190 @Override 1191 public Base makeProperty(int hash, String name) throws FHIRException { 1192 switch (hash) { 1193 case -1618432855: return getIdentifier(); 1194 case -332612366: return addBasedOn(); 1195 case -995410646: return addPartOf(); 1196 case -892481550: return getStatusElement(); 1197 case -791418107: return getPatient(); 1198 case 3575610: return getType(); 1199 case 1993333233: return getSuppliedItem(); 1200 case -2022646513: return getOccurrence(); 1201 case 1687874001: return getOccurrence(); 1202 case -1663305268: return getSupplier(); 1203 case -1429847026: return getDestination(); 1204 case -808719889: return addReceiver(); 1205 default: return super.makeProperty(hash, name); 1206 } 1207 1208 } 1209 1210 @Override 1211 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1212 switch (hash) { 1213 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1214 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1215 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1216 case -892481550: /*status*/ return new String[] {"code"}; 1217 case -791418107: /*patient*/ return new String[] {"Reference"}; 1218 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1219 case 1993333233: /*suppliedItem*/ return new String[] {}; 1220 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 1221 case -1663305268: /*supplier*/ return new String[] {"Reference"}; 1222 case -1429847026: /*destination*/ return new String[] {"Reference"}; 1223 case -808719889: /*receiver*/ return new String[] {"Reference"}; 1224 default: return super.getTypesForProperty(hash, name); 1225 } 1226 1227 } 1228 1229 @Override 1230 public Base addChild(String name) throws FHIRException { 1231 if (name.equals("identifier")) { 1232 this.identifier = new Identifier(); 1233 return this.identifier; 1234 } 1235 else if (name.equals("basedOn")) { 1236 return addBasedOn(); 1237 } 1238 else if (name.equals("partOf")) { 1239 return addPartOf(); 1240 } 1241 else if (name.equals("status")) { 1242 throw new FHIRException("Cannot call addChild on a singleton property SupplyDelivery.status"); 1243 } 1244 else if (name.equals("patient")) { 1245 this.patient = new Reference(); 1246 return this.patient; 1247 } 1248 else if (name.equals("type")) { 1249 this.type = new CodeableConcept(); 1250 return this.type; 1251 } 1252 else if (name.equals("suppliedItem")) { 1253 this.suppliedItem = new SupplyDeliverySuppliedItemComponent(); 1254 return this.suppliedItem; 1255 } 1256 else if (name.equals("occurrenceDateTime")) { 1257 this.occurrence = new DateTimeType(); 1258 return this.occurrence; 1259 } 1260 else if (name.equals("occurrencePeriod")) { 1261 this.occurrence = new Period(); 1262 return this.occurrence; 1263 } 1264 else if (name.equals("occurrenceTiming")) { 1265 this.occurrence = new Timing(); 1266 return this.occurrence; 1267 } 1268 else if (name.equals("supplier")) { 1269 this.supplier = new Reference(); 1270 return this.supplier; 1271 } 1272 else if (name.equals("destination")) { 1273 this.destination = new Reference(); 1274 return this.destination; 1275 } 1276 else if (name.equals("receiver")) { 1277 return addReceiver(); 1278 } 1279 else 1280 return super.addChild(name); 1281 } 1282 1283 public String fhirType() { 1284 return "SupplyDelivery"; 1285 1286 } 1287 1288 public SupplyDelivery copy() { 1289 SupplyDelivery dst = new SupplyDelivery(); 1290 copyValues(dst); 1291 dst.identifier = identifier == null ? null : identifier.copy(); 1292 if (basedOn != null) { 1293 dst.basedOn = new ArrayList<Reference>(); 1294 for (Reference i : basedOn) 1295 dst.basedOn.add(i.copy()); 1296 }; 1297 if (partOf != null) { 1298 dst.partOf = new ArrayList<Reference>(); 1299 for (Reference i : partOf) 1300 dst.partOf.add(i.copy()); 1301 }; 1302 dst.status = status == null ? null : status.copy(); 1303 dst.patient = patient == null ? null : patient.copy(); 1304 dst.type = type == null ? null : type.copy(); 1305 dst.suppliedItem = suppliedItem == null ? null : suppliedItem.copy(); 1306 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1307 dst.supplier = supplier == null ? null : supplier.copy(); 1308 dst.destination = destination == null ? null : destination.copy(); 1309 if (receiver != null) { 1310 dst.receiver = new ArrayList<Reference>(); 1311 for (Reference i : receiver) 1312 dst.receiver.add(i.copy()); 1313 }; 1314 return dst; 1315 } 1316 1317 protected SupplyDelivery typedCopy() { 1318 return copy(); 1319 } 1320 1321 @Override 1322 public boolean equalsDeep(Base other_) { 1323 if (!super.equalsDeep(other_)) 1324 return false; 1325 if (!(other_ instanceof SupplyDelivery)) 1326 return false; 1327 SupplyDelivery o = (SupplyDelivery) other_; 1328 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 1329 && compareDeep(status, o.status, true) && compareDeep(patient, o.patient, true) && compareDeep(type, o.type, true) 1330 && compareDeep(suppliedItem, o.suppliedItem, true) && compareDeep(occurrence, o.occurrence, true) 1331 && compareDeep(supplier, o.supplier, true) && compareDeep(destination, o.destination, true) && compareDeep(receiver, o.receiver, true) 1332 ; 1333 } 1334 1335 @Override 1336 public boolean equalsShallow(Base other_) { 1337 if (!super.equalsShallow(other_)) 1338 return false; 1339 if (!(other_ instanceof SupplyDelivery)) 1340 return false; 1341 SupplyDelivery o = (SupplyDelivery) other_; 1342 return compareValues(status, o.status, true); 1343 } 1344 1345 public boolean isEmpty() { 1346 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 1347 , status, patient, type, suppliedItem, occurrence, supplier, destination, receiver 1348 ); 1349 } 1350 1351 @Override 1352 public ResourceType getResourceType() { 1353 return ResourceType.SupplyDelivery; 1354 } 1355 1356 /** 1357 * Search parameter: <b>identifier</b> 1358 * <p> 1359 * Description: <b>External identifier</b><br> 1360 * Type: <b>token</b><br> 1361 * Path: <b>SupplyDelivery.identifier</b><br> 1362 * </p> 1363 */ 1364 @SearchParamDefinition(name="identifier", path="SupplyDelivery.identifier", description="External identifier", type="token" ) 1365 public static final String SP_IDENTIFIER = "identifier"; 1366 /** 1367 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1368 * <p> 1369 * Description: <b>External identifier</b><br> 1370 * Type: <b>token</b><br> 1371 * Path: <b>SupplyDelivery.identifier</b><br> 1372 * </p> 1373 */ 1374 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1375 1376 /** 1377 * Search parameter: <b>receiver</b> 1378 * <p> 1379 * Description: <b>Who collected the Supply</b><br> 1380 * Type: <b>reference</b><br> 1381 * Path: <b>SupplyDelivery.receiver</b><br> 1382 * </p> 1383 */ 1384 @SearchParamDefinition(name="receiver", path="SupplyDelivery.receiver", description="Who collected the Supply", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 1385 public static final String SP_RECEIVER = "receiver"; 1386 /** 1387 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 1388 * <p> 1389 * Description: <b>Who collected the Supply</b><br> 1390 * Type: <b>reference</b><br> 1391 * Path: <b>SupplyDelivery.receiver</b><br> 1392 * </p> 1393 */ 1394 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECEIVER); 1395 1396/** 1397 * Constant for fluent queries to be used to add include statements. Specifies 1398 * the path value of "<b>SupplyDelivery:receiver</b>". 1399 */ 1400 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include("SupplyDelivery:receiver").toLocked(); 1401 1402 /** 1403 * Search parameter: <b>patient</b> 1404 * <p> 1405 * Description: <b>Patient for whom the item is supplied</b><br> 1406 * Type: <b>reference</b><br> 1407 * Path: <b>SupplyDelivery.patient</b><br> 1408 * </p> 1409 */ 1410 @SearchParamDefinition(name="patient", path="SupplyDelivery.patient", description="Patient for whom the item is supplied", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1411 public static final String SP_PATIENT = "patient"; 1412 /** 1413 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1414 * <p> 1415 * Description: <b>Patient for whom the item is supplied</b><br> 1416 * Type: <b>reference</b><br> 1417 * Path: <b>SupplyDelivery.patient</b><br> 1418 * </p> 1419 */ 1420 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1421 1422/** 1423 * Constant for fluent queries to be used to add include statements. Specifies 1424 * the path value of "<b>SupplyDelivery:patient</b>". 1425 */ 1426 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("SupplyDelivery:patient").toLocked(); 1427 1428 /** 1429 * Search parameter: <b>supplier</b> 1430 * <p> 1431 * Description: <b>Dispenser</b><br> 1432 * Type: <b>reference</b><br> 1433 * Path: <b>SupplyDelivery.supplier</b><br> 1434 * </p> 1435 */ 1436 @SearchParamDefinition(name="supplier", path="SupplyDelivery.supplier", description="Dispenser", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 1437 public static final String SP_SUPPLIER = "supplier"; 1438 /** 1439 * <b>Fluent Client</b> search parameter constant for <b>supplier</b> 1440 * <p> 1441 * Description: <b>Dispenser</b><br> 1442 * Type: <b>reference</b><br> 1443 * Path: <b>SupplyDelivery.supplier</b><br> 1444 * </p> 1445 */ 1446 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLIER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPLIER); 1447 1448/** 1449 * Constant for fluent queries to be used to add include statements. Specifies 1450 * the path value of "<b>SupplyDelivery:supplier</b>". 1451 */ 1452 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLIER = new ca.uhn.fhir.model.api.Include("SupplyDelivery:supplier").toLocked(); 1453 1454 /** 1455 * Search parameter: <b>status</b> 1456 * <p> 1457 * Description: <b>in-progress | completed | abandoned | entered-in-error</b><br> 1458 * Type: <b>token</b><br> 1459 * Path: <b>SupplyDelivery.status</b><br> 1460 * </p> 1461 */ 1462 @SearchParamDefinition(name="status", path="SupplyDelivery.status", description="in-progress | completed | abandoned | entered-in-error", type="token" ) 1463 public static final String SP_STATUS = "status"; 1464 /** 1465 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1466 * <p> 1467 * Description: <b>in-progress | completed | abandoned | entered-in-error</b><br> 1468 * Type: <b>token</b><br> 1469 * Path: <b>SupplyDelivery.status</b><br> 1470 * </p> 1471 */ 1472 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1473 1474 1475}