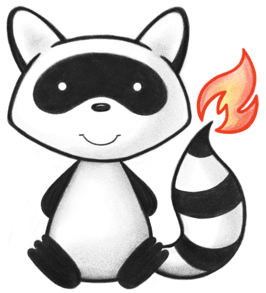
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A record of a request for a medication, substance or device used in the healthcare setting. 051 */ 052@ResourceDef(name="SupplyRequest", profile="http://hl7.org/fhir/Profile/SupplyRequest") 053public class SupplyRequest extends DomainResource { 054 055 public enum SupplyRequestStatus { 056 /** 057 * The request has been created but is not yet complete or ready for action 058 */ 059 DRAFT, 060 /** 061 * The request is ready to be acted upon 062 */ 063 ACTIVE, 064 /** 065 * The authorization/request to act has been temporarily withdrawn but is expected to resume in the future 066 */ 067 SUSPENDED, 068 /** 069 * The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur. 070 */ 071 CANCELLED, 072 /** 073 * Activity against the request has been sufficiently completed to the satisfaction of the requester 074 */ 075 COMPLETED, 076 /** 077 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be "cancelled" rather than "entered-in-error".) 078 */ 079 ENTEREDINERROR, 080 /** 081 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 082 */ 083 UNKNOWN, 084 /** 085 * added to help the parsers with the generic types 086 */ 087 NULL; 088 public static SupplyRequestStatus fromCode(String codeString) throws FHIRException { 089 if (codeString == null || "".equals(codeString)) 090 return null; 091 if ("draft".equals(codeString)) 092 return DRAFT; 093 if ("active".equals(codeString)) 094 return ACTIVE; 095 if ("suspended".equals(codeString)) 096 return SUSPENDED; 097 if ("cancelled".equals(codeString)) 098 return CANCELLED; 099 if ("completed".equals(codeString)) 100 return COMPLETED; 101 if ("entered-in-error".equals(codeString)) 102 return ENTEREDINERROR; 103 if ("unknown".equals(codeString)) 104 return UNKNOWN; 105 if (Configuration.isAcceptInvalidEnums()) 106 return null; 107 else 108 throw new FHIRException("Unknown SupplyRequestStatus code '"+codeString+"'"); 109 } 110 public String toCode() { 111 switch (this) { 112 case DRAFT: return "draft"; 113 case ACTIVE: return "active"; 114 case SUSPENDED: return "suspended"; 115 case CANCELLED: return "cancelled"; 116 case COMPLETED: return "completed"; 117 case ENTEREDINERROR: return "entered-in-error"; 118 case UNKNOWN: return "unknown"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getSystem() { 124 switch (this) { 125 case DRAFT: return "http://hl7.org/fhir/supplyrequest-status"; 126 case ACTIVE: return "http://hl7.org/fhir/supplyrequest-status"; 127 case SUSPENDED: return "http://hl7.org/fhir/supplyrequest-status"; 128 case CANCELLED: return "http://hl7.org/fhir/supplyrequest-status"; 129 case COMPLETED: return "http://hl7.org/fhir/supplyrequest-status"; 130 case ENTEREDINERROR: return "http://hl7.org/fhir/supplyrequest-status"; 131 case UNKNOWN: return "http://hl7.org/fhir/supplyrequest-status"; 132 case NULL: return null; 133 default: return "?"; 134 } 135 } 136 public String getDefinition() { 137 switch (this) { 138 case DRAFT: return "The request has been created but is not yet complete or ready for action"; 139 case ACTIVE: return "The request is ready to be acted upon"; 140 case SUSPENDED: return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future"; 141 case CANCELLED: return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 142 case COMPLETED: return "Activity against the request has been sufficiently completed to the satisfaction of the requester"; 143 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)"; 144 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 145 case NULL: return null; 146 default: return "?"; 147 } 148 } 149 public String getDisplay() { 150 switch (this) { 151 case DRAFT: return "Draft"; 152 case ACTIVE: return "Active"; 153 case SUSPENDED: return "Suspended"; 154 case CANCELLED: return "Cancelled"; 155 case COMPLETED: return "Completed"; 156 case ENTEREDINERROR: return "Entered in Error"; 157 case UNKNOWN: return "Unknown"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 } 163 164 public static class SupplyRequestStatusEnumFactory implements EnumFactory<SupplyRequestStatus> { 165 public SupplyRequestStatus fromCode(String codeString) throws IllegalArgumentException { 166 if (codeString == null || "".equals(codeString)) 167 if (codeString == null || "".equals(codeString)) 168 return null; 169 if ("draft".equals(codeString)) 170 return SupplyRequestStatus.DRAFT; 171 if ("active".equals(codeString)) 172 return SupplyRequestStatus.ACTIVE; 173 if ("suspended".equals(codeString)) 174 return SupplyRequestStatus.SUSPENDED; 175 if ("cancelled".equals(codeString)) 176 return SupplyRequestStatus.CANCELLED; 177 if ("completed".equals(codeString)) 178 return SupplyRequestStatus.COMPLETED; 179 if ("entered-in-error".equals(codeString)) 180 return SupplyRequestStatus.ENTEREDINERROR; 181 if ("unknown".equals(codeString)) 182 return SupplyRequestStatus.UNKNOWN; 183 throw new IllegalArgumentException("Unknown SupplyRequestStatus code '"+codeString+"'"); 184 } 185 public Enumeration<SupplyRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 186 if (code == null) 187 return null; 188 if (code.isEmpty()) 189 return new Enumeration<SupplyRequestStatus>(this); 190 String codeString = code.asStringValue(); 191 if (codeString == null || "".equals(codeString)) 192 return null; 193 if ("draft".equals(codeString)) 194 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.DRAFT); 195 if ("active".equals(codeString)) 196 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.ACTIVE); 197 if ("suspended".equals(codeString)) 198 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.SUSPENDED); 199 if ("cancelled".equals(codeString)) 200 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.CANCELLED); 201 if ("completed".equals(codeString)) 202 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.COMPLETED); 203 if ("entered-in-error".equals(codeString)) 204 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.ENTEREDINERROR); 205 if ("unknown".equals(codeString)) 206 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.UNKNOWN); 207 throw new FHIRException("Unknown SupplyRequestStatus code '"+codeString+"'"); 208 } 209 public String toCode(SupplyRequestStatus code) { 210 if (code == SupplyRequestStatus.NULL) 211 return null; 212 if (code == SupplyRequestStatus.DRAFT) 213 return "draft"; 214 if (code == SupplyRequestStatus.ACTIVE) 215 return "active"; 216 if (code == SupplyRequestStatus.SUSPENDED) 217 return "suspended"; 218 if (code == SupplyRequestStatus.CANCELLED) 219 return "cancelled"; 220 if (code == SupplyRequestStatus.COMPLETED) 221 return "completed"; 222 if (code == SupplyRequestStatus.ENTEREDINERROR) 223 return "entered-in-error"; 224 if (code == SupplyRequestStatus.UNKNOWN) 225 return "unknown"; 226 return "?"; 227 } 228 public String toSystem(SupplyRequestStatus code) { 229 return code.getSystem(); 230 } 231 } 232 233 public enum RequestPriority { 234 /** 235 * The request has normal priority 236 */ 237 ROUTINE, 238 /** 239 * The request should be actioned promptly - higher priority than routine 240 */ 241 URGENT, 242 /** 243 * The request should be actioned as soon as possible - higher priority than urgent 244 */ 245 ASAP, 246 /** 247 * The request should be actioned immediately - highest possible priority. E.g. an emergency 248 */ 249 STAT, 250 /** 251 * added to help the parsers with the generic types 252 */ 253 NULL; 254 public static RequestPriority fromCode(String codeString) throws FHIRException { 255 if (codeString == null || "".equals(codeString)) 256 return null; 257 if ("routine".equals(codeString)) 258 return ROUTINE; 259 if ("urgent".equals(codeString)) 260 return URGENT; 261 if ("asap".equals(codeString)) 262 return ASAP; 263 if ("stat".equals(codeString)) 264 return STAT; 265 if (Configuration.isAcceptInvalidEnums()) 266 return null; 267 else 268 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 269 } 270 public String toCode() { 271 switch (this) { 272 case ROUTINE: return "routine"; 273 case URGENT: return "urgent"; 274 case ASAP: return "asap"; 275 case STAT: return "stat"; 276 case NULL: return null; 277 default: return "?"; 278 } 279 } 280 public String getSystem() { 281 switch (this) { 282 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 283 case URGENT: return "http://hl7.org/fhir/request-priority"; 284 case ASAP: return "http://hl7.org/fhir/request-priority"; 285 case STAT: return "http://hl7.org/fhir/request-priority"; 286 case NULL: return null; 287 default: return "?"; 288 } 289 } 290 public String getDefinition() { 291 switch (this) { 292 case ROUTINE: return "The request has normal priority"; 293 case URGENT: return "The request should be actioned promptly - higher priority than routine"; 294 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent"; 295 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency"; 296 case NULL: return null; 297 default: return "?"; 298 } 299 } 300 public String getDisplay() { 301 switch (this) { 302 case ROUTINE: return "Routine"; 303 case URGENT: return "Urgent"; 304 case ASAP: return "ASAP"; 305 case STAT: return "STAT"; 306 case NULL: return null; 307 default: return "?"; 308 } 309 } 310 } 311 312 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 313 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 314 if (codeString == null || "".equals(codeString)) 315 if (codeString == null || "".equals(codeString)) 316 return null; 317 if ("routine".equals(codeString)) 318 return RequestPriority.ROUTINE; 319 if ("urgent".equals(codeString)) 320 return RequestPriority.URGENT; 321 if ("asap".equals(codeString)) 322 return RequestPriority.ASAP; 323 if ("stat".equals(codeString)) 324 return RequestPriority.STAT; 325 throw new IllegalArgumentException("Unknown RequestPriority code '"+codeString+"'"); 326 } 327 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 328 if (code == null) 329 return null; 330 if (code.isEmpty()) 331 return new Enumeration<RequestPriority>(this); 332 String codeString = code.asStringValue(); 333 if (codeString == null || "".equals(codeString)) 334 return null; 335 if ("routine".equals(codeString)) 336 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE); 337 if ("urgent".equals(codeString)) 338 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT); 339 if ("asap".equals(codeString)) 340 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP); 341 if ("stat".equals(codeString)) 342 return new Enumeration<RequestPriority>(this, RequestPriority.STAT); 343 throw new FHIRException("Unknown RequestPriority code '"+codeString+"'"); 344 } 345 public String toCode(RequestPriority code) { 346 if (code == RequestPriority.NULL) 347 return null; 348 if (code == RequestPriority.ROUTINE) 349 return "routine"; 350 if (code == RequestPriority.URGENT) 351 return "urgent"; 352 if (code == RequestPriority.ASAP) 353 return "asap"; 354 if (code == RequestPriority.STAT) 355 return "stat"; 356 return "?"; 357 } 358 public String toSystem(RequestPriority code) { 359 return code.getSystem(); 360 } 361 } 362 363 @Block() 364 public static class SupplyRequestOrderedItemComponent extends BackboneElement implements IBaseBackboneElement { 365 /** 366 * The amount that is being ordered of the indicated item. 367 */ 368 @Child(name = "quantity", type = {Quantity.class}, order=1, min=1, max=1, modifier=false, summary=true) 369 @Description(shortDefinition="The requested amount of the item indicated", formalDefinition="The amount that is being ordered of the indicated item." ) 370 protected Quantity quantity; 371 372 /** 373 * The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list. 374 */ 375 @Child(name = "item", type = {CodeableConcept.class, Medication.class, Substance.class, Device.class}, order=2, min=0, max=1, modifier=false, summary=true) 376 @Description(shortDefinition="Medication, Substance, or Device requested to be supplied", formalDefinition="The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list." ) 377 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supply-item") 378 protected Type item; 379 380 private static final long serialVersionUID = 1628109307L; 381 382 /** 383 * Constructor 384 */ 385 public SupplyRequestOrderedItemComponent() { 386 super(); 387 } 388 389 /** 390 * Constructor 391 */ 392 public SupplyRequestOrderedItemComponent(Quantity quantity) { 393 super(); 394 this.quantity = quantity; 395 } 396 397 /** 398 * @return {@link #quantity} (The amount that is being ordered of the indicated item.) 399 */ 400 public Quantity getQuantity() { 401 if (this.quantity == null) 402 if (Configuration.errorOnAutoCreate()) 403 throw new Error("Attempt to auto-create SupplyRequestOrderedItemComponent.quantity"); 404 else if (Configuration.doAutoCreate()) 405 this.quantity = new Quantity(); // cc 406 return this.quantity; 407 } 408 409 public boolean hasQuantity() { 410 return this.quantity != null && !this.quantity.isEmpty(); 411 } 412 413 /** 414 * @param value {@link #quantity} (The amount that is being ordered of the indicated item.) 415 */ 416 public SupplyRequestOrderedItemComponent setQuantity(Quantity value) { 417 this.quantity = value; 418 return this; 419 } 420 421 /** 422 * @return {@link #item} (The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 423 */ 424 public Type getItem() { 425 return this.item; 426 } 427 428 /** 429 * @return {@link #item} (The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 430 */ 431 public CodeableConcept getItemCodeableConcept() throws FHIRException { 432 if (this.item == null) 433 return null; 434 if (!(this.item instanceof CodeableConcept)) 435 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 436 return (CodeableConcept) this.item; 437 } 438 439 public boolean hasItemCodeableConcept() { 440 return this != null && this.item instanceof CodeableConcept; 441 } 442 443 /** 444 * @return {@link #item} (The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 445 */ 446 public Reference getItemReference() throws FHIRException { 447 if (this.item == null) 448 return null; 449 if (!(this.item instanceof Reference)) 450 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 451 return (Reference) this.item; 452 } 453 454 public boolean hasItemReference() { 455 return this != null && this.item instanceof Reference; 456 } 457 458 public boolean hasItem() { 459 return this.item != null && !this.item.isEmpty(); 460 } 461 462 /** 463 * @param value {@link #item} (The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.) 464 */ 465 public SupplyRequestOrderedItemComponent setItem(Type value) throws FHIRFormatError { 466 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 467 throw new FHIRFormatError("Not the right type for SupplyRequest.orderedItem.item[x]: "+value.fhirType()); 468 this.item = value; 469 return this; 470 } 471 472 protected void listChildren(List<Property> children) { 473 super.listChildren(children); 474 children.add(new Property("quantity", "Quantity", "The amount that is being ordered of the indicated item.", 0, 1, quantity)); 475 children.add(new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item)); 476 } 477 478 @Override 479 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 480 switch (_hash) { 481 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount that is being ordered of the indicated item.", 0, 1, quantity); 482 case 2116201613: /*item[x]*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 483 case 3242771: /*item*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 484 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 485 case 1376364920: /*itemReference*/ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 0, 1, item); 486 default: return super.getNamedProperty(_hash, _name, _checkValid); 487 } 488 489 } 490 491 @Override 492 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 493 switch (hash) { 494 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 495 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // Type 496 default: return super.getProperty(hash, name, checkValid); 497 } 498 499 } 500 501 @Override 502 public Base setProperty(int hash, String name, Base value) throws FHIRException { 503 switch (hash) { 504 case -1285004149: // quantity 505 this.quantity = castToQuantity(value); // Quantity 506 return value; 507 case 3242771: // item 508 this.item = castToType(value); // Type 509 return value; 510 default: return super.setProperty(hash, name, value); 511 } 512 513 } 514 515 @Override 516 public Base setProperty(String name, Base value) throws FHIRException { 517 if (name.equals("quantity")) { 518 this.quantity = castToQuantity(value); // Quantity 519 } else if (name.equals("item[x]")) { 520 this.item = castToType(value); // Type 521 } else 522 return super.setProperty(name, value); 523 return value; 524 } 525 526 @Override 527 public Base makeProperty(int hash, String name) throws FHIRException { 528 switch (hash) { 529 case -1285004149: return getQuantity(); 530 case 2116201613: return getItem(); 531 case 3242771: return getItem(); 532 default: return super.makeProperty(hash, name); 533 } 534 535 } 536 537 @Override 538 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 539 switch (hash) { 540 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 541 case 3242771: /*item*/ return new String[] {"CodeableConcept", "Reference"}; 542 default: return super.getTypesForProperty(hash, name); 543 } 544 545 } 546 547 @Override 548 public Base addChild(String name) throws FHIRException { 549 if (name.equals("quantity")) { 550 this.quantity = new Quantity(); 551 return this.quantity; 552 } 553 else if (name.equals("itemCodeableConcept")) { 554 this.item = new CodeableConcept(); 555 return this.item; 556 } 557 else if (name.equals("itemReference")) { 558 this.item = new Reference(); 559 return this.item; 560 } 561 else 562 return super.addChild(name); 563 } 564 565 public SupplyRequestOrderedItemComponent copy() { 566 SupplyRequestOrderedItemComponent dst = new SupplyRequestOrderedItemComponent(); 567 copyValues(dst); 568 dst.quantity = quantity == null ? null : quantity.copy(); 569 dst.item = item == null ? null : item.copy(); 570 return dst; 571 } 572 573 @Override 574 public boolean equalsDeep(Base other_) { 575 if (!super.equalsDeep(other_)) 576 return false; 577 if (!(other_ instanceof SupplyRequestOrderedItemComponent)) 578 return false; 579 SupplyRequestOrderedItemComponent o = (SupplyRequestOrderedItemComponent) other_; 580 return compareDeep(quantity, o.quantity, true) && compareDeep(item, o.item, true); 581 } 582 583 @Override 584 public boolean equalsShallow(Base other_) { 585 if (!super.equalsShallow(other_)) 586 return false; 587 if (!(other_ instanceof SupplyRequestOrderedItemComponent)) 588 return false; 589 SupplyRequestOrderedItemComponent o = (SupplyRequestOrderedItemComponent) other_; 590 return true; 591 } 592 593 public boolean isEmpty() { 594 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, item); 595 } 596 597 public String fhirType() { 598 return "SupplyRequest.orderedItem"; 599 600 } 601 602 } 603 604 @Block() 605 public static class SupplyRequestRequesterComponent extends BackboneElement implements IBaseBackboneElement { 606 /** 607 * The device, practitioner, etc. who initiated the request. 608 */ 609 @Child(name = "agent", type = {Practitioner.class, Organization.class, Patient.class, RelatedPerson.class, Device.class}, order=1, min=1, max=1, modifier=false, summary=true) 610 @Description(shortDefinition="Individual making the request", formalDefinition="The device, practitioner, etc. who initiated the request." ) 611 protected Reference agent; 612 613 /** 614 * The actual object that is the target of the reference (The device, practitioner, etc. who initiated the request.) 615 */ 616 protected Resource agentTarget; 617 618 /** 619 * The organization the device or practitioner was acting on behalf of. 620 */ 621 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=false) 622 @Description(shortDefinition="Organization agent is acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 623 protected Reference onBehalfOf; 624 625 /** 626 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 627 */ 628 protected Organization onBehalfOfTarget; 629 630 private static final long serialVersionUID = -71453027L; 631 632 /** 633 * Constructor 634 */ 635 public SupplyRequestRequesterComponent() { 636 super(); 637 } 638 639 /** 640 * Constructor 641 */ 642 public SupplyRequestRequesterComponent(Reference agent) { 643 super(); 644 this.agent = agent; 645 } 646 647 /** 648 * @return {@link #agent} (The device, practitioner, etc. who initiated the request.) 649 */ 650 public Reference getAgent() { 651 if (this.agent == null) 652 if (Configuration.errorOnAutoCreate()) 653 throw new Error("Attempt to auto-create SupplyRequestRequesterComponent.agent"); 654 else if (Configuration.doAutoCreate()) 655 this.agent = new Reference(); // cc 656 return this.agent; 657 } 658 659 public boolean hasAgent() { 660 return this.agent != null && !this.agent.isEmpty(); 661 } 662 663 /** 664 * @param value {@link #agent} (The device, practitioner, etc. who initiated the request.) 665 */ 666 public SupplyRequestRequesterComponent setAgent(Reference value) { 667 this.agent = value; 668 return this; 669 } 670 671 /** 672 * @return {@link #agent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the request.) 673 */ 674 public Resource getAgentTarget() { 675 return this.agentTarget; 676 } 677 678 /** 679 * @param value {@link #agent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the request.) 680 */ 681 public SupplyRequestRequesterComponent setAgentTarget(Resource value) { 682 this.agentTarget = value; 683 return this; 684 } 685 686 /** 687 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 688 */ 689 public Reference getOnBehalfOf() { 690 if (this.onBehalfOf == null) 691 if (Configuration.errorOnAutoCreate()) 692 throw new Error("Attempt to auto-create SupplyRequestRequesterComponent.onBehalfOf"); 693 else if (Configuration.doAutoCreate()) 694 this.onBehalfOf = new Reference(); // cc 695 return this.onBehalfOf; 696 } 697 698 public boolean hasOnBehalfOf() { 699 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 700 } 701 702 /** 703 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 704 */ 705 public SupplyRequestRequesterComponent setOnBehalfOf(Reference value) { 706 this.onBehalfOf = value; 707 return this; 708 } 709 710 /** 711 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 712 */ 713 public Organization getOnBehalfOfTarget() { 714 if (this.onBehalfOfTarget == null) 715 if (Configuration.errorOnAutoCreate()) 716 throw new Error("Attempt to auto-create SupplyRequestRequesterComponent.onBehalfOf"); 717 else if (Configuration.doAutoCreate()) 718 this.onBehalfOfTarget = new Organization(); // aa 719 return this.onBehalfOfTarget; 720 } 721 722 /** 723 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 724 */ 725 public SupplyRequestRequesterComponent setOnBehalfOfTarget(Organization value) { 726 this.onBehalfOfTarget = value; 727 return this; 728 } 729 730 protected void listChildren(List<Property> children) { 731 super.listChildren(children); 732 children.add(new Property("agent", "Reference(Practitioner|Organization|Patient|RelatedPerson|Device)", "The device, practitioner, etc. who initiated the request.", 0, 1, agent)); 733 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 734 } 735 736 @Override 737 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 738 switch (_hash) { 739 case 92750597: /*agent*/ return new Property("agent", "Reference(Practitioner|Organization|Patient|RelatedPerson|Device)", "The device, practitioner, etc. who initiated the request.", 0, 1, agent); 740 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 741 default: return super.getNamedProperty(_hash, _name, _checkValid); 742 } 743 744 } 745 746 @Override 747 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 748 switch (hash) { 749 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : new Base[] {this.agent}; // Reference 750 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 751 default: return super.getProperty(hash, name, checkValid); 752 } 753 754 } 755 756 @Override 757 public Base setProperty(int hash, String name, Base value) throws FHIRException { 758 switch (hash) { 759 case 92750597: // agent 760 this.agent = castToReference(value); // Reference 761 return value; 762 case -14402964: // onBehalfOf 763 this.onBehalfOf = castToReference(value); // Reference 764 return value; 765 default: return super.setProperty(hash, name, value); 766 } 767 768 } 769 770 @Override 771 public Base setProperty(String name, Base value) throws FHIRException { 772 if (name.equals("agent")) { 773 this.agent = castToReference(value); // Reference 774 } else if (name.equals("onBehalfOf")) { 775 this.onBehalfOf = castToReference(value); // Reference 776 } else 777 return super.setProperty(name, value); 778 return value; 779 } 780 781 @Override 782 public Base makeProperty(int hash, String name) throws FHIRException { 783 switch (hash) { 784 case 92750597: return getAgent(); 785 case -14402964: return getOnBehalfOf(); 786 default: return super.makeProperty(hash, name); 787 } 788 789 } 790 791 @Override 792 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 793 switch (hash) { 794 case 92750597: /*agent*/ return new String[] {"Reference"}; 795 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 796 default: return super.getTypesForProperty(hash, name); 797 } 798 799 } 800 801 @Override 802 public Base addChild(String name) throws FHIRException { 803 if (name.equals("agent")) { 804 this.agent = new Reference(); 805 return this.agent; 806 } 807 else if (name.equals("onBehalfOf")) { 808 this.onBehalfOf = new Reference(); 809 return this.onBehalfOf; 810 } 811 else 812 return super.addChild(name); 813 } 814 815 public SupplyRequestRequesterComponent copy() { 816 SupplyRequestRequesterComponent dst = new SupplyRequestRequesterComponent(); 817 copyValues(dst); 818 dst.agent = agent == null ? null : agent.copy(); 819 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 820 return dst; 821 } 822 823 @Override 824 public boolean equalsDeep(Base other_) { 825 if (!super.equalsDeep(other_)) 826 return false; 827 if (!(other_ instanceof SupplyRequestRequesterComponent)) 828 return false; 829 SupplyRequestRequesterComponent o = (SupplyRequestRequesterComponent) other_; 830 return compareDeep(agent, o.agent, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 831 } 832 833 @Override 834 public boolean equalsShallow(Base other_) { 835 if (!super.equalsShallow(other_)) 836 return false; 837 if (!(other_ instanceof SupplyRequestRequesterComponent)) 838 return false; 839 SupplyRequestRequesterComponent o = (SupplyRequestRequesterComponent) other_; 840 return true; 841 } 842 843 public boolean isEmpty() { 844 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(agent, onBehalfOf); 845 } 846 847 public String fhirType() { 848 return "SupplyRequest.requester"; 849 850 } 851 852 } 853 854 /** 855 * Unique identifier for this supply request. 856 */ 857 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 858 @Description(shortDefinition="Unique identifier", formalDefinition="Unique identifier for this supply request." ) 859 protected Identifier identifier; 860 861 /** 862 * Status of the supply request. 863 */ 864 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 865 @Description(shortDefinition="draft | active | suspended +", formalDefinition="Status of the supply request." ) 866 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplyrequest-status") 867 protected Enumeration<SupplyRequestStatus> status; 868 869 /** 870 * Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process. 871 */ 872 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 873 @Description(shortDefinition="The kind of supply (central, non-stock, etc.)", formalDefinition="Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process." ) 874 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplyrequest-kind") 875 protected CodeableConcept category; 876 877 /** 878 * Indicates how quickly this SupplyRequest should be addressed with respect to other requests. 879 */ 880 @Child(name = "priority", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 881 @Description(shortDefinition="routine | urgent | asap | stat", formalDefinition="Indicates how quickly this SupplyRequest should be addressed with respect to other requests." ) 882 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 883 protected Enumeration<RequestPriority> priority; 884 885 /** 886 * The item being requested. 887 */ 888 @Child(name = "orderedItem", type = {}, order=4, min=0, max=1, modifier=false, summary=true) 889 @Description(shortDefinition="The item being requested", formalDefinition="The item being requested." ) 890 protected SupplyRequestOrderedItemComponent orderedItem; 891 892 /** 893 * When the request should be fulfilled. 894 */ 895 @Child(name = "occurrence", type = {DateTimeType.class, Period.class, Timing.class}, order=5, min=0, max=1, modifier=false, summary=true) 896 @Description(shortDefinition="When the request should be fulfilled", formalDefinition="When the request should be fulfilled." ) 897 protected Type occurrence; 898 899 /** 900 * When the request was made. 901 */ 902 @Child(name = "authoredOn", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 903 @Description(shortDefinition="When the request was made", formalDefinition="When the request was made." ) 904 protected DateTimeType authoredOn; 905 906 /** 907 * The individual who initiated the request and has responsibility for its activation. 908 */ 909 @Child(name = "requester", type = {}, order=7, min=0, max=1, modifier=false, summary=true) 910 @Description(shortDefinition="Who/what is requesting service", formalDefinition="The individual who initiated the request and has responsibility for its activation." ) 911 protected SupplyRequestRequesterComponent requester; 912 913 /** 914 * Who is intended to fulfill the request. 915 */ 916 @Child(name = "supplier", type = {Organization.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 917 @Description(shortDefinition="Who is intended to fulfill the request", formalDefinition="Who is intended to fulfill the request." ) 918 protected List<Reference> supplier; 919 /** 920 * The actual objects that are the target of the reference (Who is intended to fulfill the request.) 921 */ 922 protected List<Organization> supplierTarget; 923 924 925 /** 926 * Why the supply item was requested. 927 */ 928 @Child(name = "reason", type = {CodeableConcept.class, Reference.class}, order=9, min=0, max=1, modifier=false, summary=false) 929 @Description(shortDefinition="Why the supply item was requested", formalDefinition="Why the supply item was requested." ) 930 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/supplyrequest-reason") 931 protected Type reason; 932 933 /** 934 * Where the supply is expected to come from. 935 */ 936 @Child(name = "deliverFrom", type = {Organization.class, Location.class}, order=10, min=0, max=1, modifier=false, summary=false) 937 @Description(shortDefinition="The origin of the supply", formalDefinition="Where the supply is expected to come from." ) 938 protected Reference deliverFrom; 939 940 /** 941 * The actual object that is the target of the reference (Where the supply is expected to come from.) 942 */ 943 protected Resource deliverFromTarget; 944 945 /** 946 * Where the supply is destined to go. 947 */ 948 @Child(name = "deliverTo", type = {Organization.class, Location.class, Patient.class}, order=11, min=0, max=1, modifier=false, summary=false) 949 @Description(shortDefinition="The destination of the supply", formalDefinition="Where the supply is destined to go." ) 950 protected Reference deliverTo; 951 952 /** 953 * The actual object that is the target of the reference (Where the supply is destined to go.) 954 */ 955 protected Resource deliverToTarget; 956 957 private static final long serialVersionUID = 43957782L; 958 959 /** 960 * Constructor 961 */ 962 public SupplyRequest() { 963 super(); 964 } 965 966 /** 967 * @return {@link #identifier} (Unique identifier for this supply request.) 968 */ 969 public Identifier getIdentifier() { 970 if (this.identifier == null) 971 if (Configuration.errorOnAutoCreate()) 972 throw new Error("Attempt to auto-create SupplyRequest.identifier"); 973 else if (Configuration.doAutoCreate()) 974 this.identifier = new Identifier(); // cc 975 return this.identifier; 976 } 977 978 public boolean hasIdentifier() { 979 return this.identifier != null && !this.identifier.isEmpty(); 980 } 981 982 /** 983 * @param value {@link #identifier} (Unique identifier for this supply request.) 984 */ 985 public SupplyRequest setIdentifier(Identifier value) { 986 this.identifier = value; 987 return this; 988 } 989 990 /** 991 * @return {@link #status} (Status of the supply request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 992 */ 993 public Enumeration<SupplyRequestStatus> getStatusElement() { 994 if (this.status == null) 995 if (Configuration.errorOnAutoCreate()) 996 throw new Error("Attempt to auto-create SupplyRequest.status"); 997 else if (Configuration.doAutoCreate()) 998 this.status = new Enumeration<SupplyRequestStatus>(new SupplyRequestStatusEnumFactory()); // bb 999 return this.status; 1000 } 1001 1002 public boolean hasStatusElement() { 1003 return this.status != null && !this.status.isEmpty(); 1004 } 1005 1006 public boolean hasStatus() { 1007 return this.status != null && !this.status.isEmpty(); 1008 } 1009 1010 /** 1011 * @param value {@link #status} (Status of the supply request.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1012 */ 1013 public SupplyRequest setStatusElement(Enumeration<SupplyRequestStatus> value) { 1014 this.status = value; 1015 return this; 1016 } 1017 1018 /** 1019 * @return Status of the supply request. 1020 */ 1021 public SupplyRequestStatus getStatus() { 1022 return this.status == null ? null : this.status.getValue(); 1023 } 1024 1025 /** 1026 * @param value Status of the supply request. 1027 */ 1028 public SupplyRequest setStatus(SupplyRequestStatus value) { 1029 if (value == null) 1030 this.status = null; 1031 else { 1032 if (this.status == null) 1033 this.status = new Enumeration<SupplyRequestStatus>(new SupplyRequestStatusEnumFactory()); 1034 this.status.setValue(value); 1035 } 1036 return this; 1037 } 1038 1039 /** 1040 * @return {@link #category} (Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.) 1041 */ 1042 public CodeableConcept getCategory() { 1043 if (this.category == null) 1044 if (Configuration.errorOnAutoCreate()) 1045 throw new Error("Attempt to auto-create SupplyRequest.category"); 1046 else if (Configuration.doAutoCreate()) 1047 this.category = new CodeableConcept(); // cc 1048 return this.category; 1049 } 1050 1051 public boolean hasCategory() { 1052 return this.category != null && !this.category.isEmpty(); 1053 } 1054 1055 /** 1056 * @param value {@link #category} (Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.) 1057 */ 1058 public SupplyRequest setCategory(CodeableConcept value) { 1059 this.category = value; 1060 return this; 1061 } 1062 1063 /** 1064 * @return {@link #priority} (Indicates how quickly this SupplyRequest should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1065 */ 1066 public Enumeration<RequestPriority> getPriorityElement() { 1067 if (this.priority == null) 1068 if (Configuration.errorOnAutoCreate()) 1069 throw new Error("Attempt to auto-create SupplyRequest.priority"); 1070 else if (Configuration.doAutoCreate()) 1071 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 1072 return this.priority; 1073 } 1074 1075 public boolean hasPriorityElement() { 1076 return this.priority != null && !this.priority.isEmpty(); 1077 } 1078 1079 public boolean hasPriority() { 1080 return this.priority != null && !this.priority.isEmpty(); 1081 } 1082 1083 /** 1084 * @param value {@link #priority} (Indicates how quickly this SupplyRequest should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1085 */ 1086 public SupplyRequest setPriorityElement(Enumeration<RequestPriority> value) { 1087 this.priority = value; 1088 return this; 1089 } 1090 1091 /** 1092 * @return Indicates how quickly this SupplyRequest should be addressed with respect to other requests. 1093 */ 1094 public RequestPriority getPriority() { 1095 return this.priority == null ? null : this.priority.getValue(); 1096 } 1097 1098 /** 1099 * @param value Indicates how quickly this SupplyRequest should be addressed with respect to other requests. 1100 */ 1101 public SupplyRequest setPriority(RequestPriority value) { 1102 if (value == null) 1103 this.priority = null; 1104 else { 1105 if (this.priority == null) 1106 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 1107 this.priority.setValue(value); 1108 } 1109 return this; 1110 } 1111 1112 /** 1113 * @return {@link #orderedItem} (The item being requested.) 1114 */ 1115 public SupplyRequestOrderedItemComponent getOrderedItem() { 1116 if (this.orderedItem == null) 1117 if (Configuration.errorOnAutoCreate()) 1118 throw new Error("Attempt to auto-create SupplyRequest.orderedItem"); 1119 else if (Configuration.doAutoCreate()) 1120 this.orderedItem = new SupplyRequestOrderedItemComponent(); // cc 1121 return this.orderedItem; 1122 } 1123 1124 public boolean hasOrderedItem() { 1125 return this.orderedItem != null && !this.orderedItem.isEmpty(); 1126 } 1127 1128 /** 1129 * @param value {@link #orderedItem} (The item being requested.) 1130 */ 1131 public SupplyRequest setOrderedItem(SupplyRequestOrderedItemComponent value) { 1132 this.orderedItem = value; 1133 return this; 1134 } 1135 1136 /** 1137 * @return {@link #occurrence} (When the request should be fulfilled.) 1138 */ 1139 public Type getOccurrence() { 1140 return this.occurrence; 1141 } 1142 1143 /** 1144 * @return {@link #occurrence} (When the request should be fulfilled.) 1145 */ 1146 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1147 if (this.occurrence == null) 1148 return null; 1149 if (!(this.occurrence instanceof DateTimeType)) 1150 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1151 return (DateTimeType) this.occurrence; 1152 } 1153 1154 public boolean hasOccurrenceDateTimeType() { 1155 return this != null && this.occurrence instanceof DateTimeType; 1156 } 1157 1158 /** 1159 * @return {@link #occurrence} (When the request should be fulfilled.) 1160 */ 1161 public Period getOccurrencePeriod() throws FHIRException { 1162 if (this.occurrence == null) 1163 return null; 1164 if (!(this.occurrence instanceof Period)) 1165 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1166 return (Period) this.occurrence; 1167 } 1168 1169 public boolean hasOccurrencePeriod() { 1170 return this != null && this.occurrence instanceof Period; 1171 } 1172 1173 /** 1174 * @return {@link #occurrence} (When the request should be fulfilled.) 1175 */ 1176 public Timing getOccurrenceTiming() throws FHIRException { 1177 if (this.occurrence == null) 1178 return null; 1179 if (!(this.occurrence instanceof Timing)) 1180 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 1181 return (Timing) this.occurrence; 1182 } 1183 1184 public boolean hasOccurrenceTiming() { 1185 return this != null && this.occurrence instanceof Timing; 1186 } 1187 1188 public boolean hasOccurrence() { 1189 return this.occurrence != null && !this.occurrence.isEmpty(); 1190 } 1191 1192 /** 1193 * @param value {@link #occurrence} (When the request should be fulfilled.) 1194 */ 1195 public SupplyRequest setOccurrence(Type value) throws FHIRFormatError { 1196 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1197 throw new FHIRFormatError("Not the right type for SupplyRequest.occurrence[x]: "+value.fhirType()); 1198 this.occurrence = value; 1199 return this; 1200 } 1201 1202 /** 1203 * @return {@link #authoredOn} (When the request was made.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1204 */ 1205 public DateTimeType getAuthoredOnElement() { 1206 if (this.authoredOn == null) 1207 if (Configuration.errorOnAutoCreate()) 1208 throw new Error("Attempt to auto-create SupplyRequest.authoredOn"); 1209 else if (Configuration.doAutoCreate()) 1210 this.authoredOn = new DateTimeType(); // bb 1211 return this.authoredOn; 1212 } 1213 1214 public boolean hasAuthoredOnElement() { 1215 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1216 } 1217 1218 public boolean hasAuthoredOn() { 1219 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1220 } 1221 1222 /** 1223 * @param value {@link #authoredOn} (When the request was made.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 1224 */ 1225 public SupplyRequest setAuthoredOnElement(DateTimeType value) { 1226 this.authoredOn = value; 1227 return this; 1228 } 1229 1230 /** 1231 * @return When the request was made. 1232 */ 1233 public Date getAuthoredOn() { 1234 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1235 } 1236 1237 /** 1238 * @param value When the request was made. 1239 */ 1240 public SupplyRequest setAuthoredOn(Date value) { 1241 if (value == null) 1242 this.authoredOn = null; 1243 else { 1244 if (this.authoredOn == null) 1245 this.authoredOn = new DateTimeType(); 1246 this.authoredOn.setValue(value); 1247 } 1248 return this; 1249 } 1250 1251 /** 1252 * @return {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1253 */ 1254 public SupplyRequestRequesterComponent getRequester() { 1255 if (this.requester == null) 1256 if (Configuration.errorOnAutoCreate()) 1257 throw new Error("Attempt to auto-create SupplyRequest.requester"); 1258 else if (Configuration.doAutoCreate()) 1259 this.requester = new SupplyRequestRequesterComponent(); // cc 1260 return this.requester; 1261 } 1262 1263 public boolean hasRequester() { 1264 return this.requester != null && !this.requester.isEmpty(); 1265 } 1266 1267 /** 1268 * @param value {@link #requester} (The individual who initiated the request and has responsibility for its activation.) 1269 */ 1270 public SupplyRequest setRequester(SupplyRequestRequesterComponent value) { 1271 this.requester = value; 1272 return this; 1273 } 1274 1275 /** 1276 * @return {@link #supplier} (Who is intended to fulfill the request.) 1277 */ 1278 public List<Reference> getSupplier() { 1279 if (this.supplier == null) 1280 this.supplier = new ArrayList<Reference>(); 1281 return this.supplier; 1282 } 1283 1284 /** 1285 * @return Returns a reference to <code>this</code> for easy method chaining 1286 */ 1287 public SupplyRequest setSupplier(List<Reference> theSupplier) { 1288 this.supplier = theSupplier; 1289 return this; 1290 } 1291 1292 public boolean hasSupplier() { 1293 if (this.supplier == null) 1294 return false; 1295 for (Reference item : this.supplier) 1296 if (!item.isEmpty()) 1297 return true; 1298 return false; 1299 } 1300 1301 public Reference addSupplier() { //3 1302 Reference t = new Reference(); 1303 if (this.supplier == null) 1304 this.supplier = new ArrayList<Reference>(); 1305 this.supplier.add(t); 1306 return t; 1307 } 1308 1309 public SupplyRequest addSupplier(Reference t) { //3 1310 if (t == null) 1311 return this; 1312 if (this.supplier == null) 1313 this.supplier = new ArrayList<Reference>(); 1314 this.supplier.add(t); 1315 return this; 1316 } 1317 1318 /** 1319 * @return The first repetition of repeating field {@link #supplier}, creating it if it does not already exist 1320 */ 1321 public Reference getSupplierFirstRep() { 1322 if (getSupplier().isEmpty()) { 1323 addSupplier(); 1324 } 1325 return getSupplier().get(0); 1326 } 1327 1328 /** 1329 * @deprecated Use Reference#setResource(IBaseResource) instead 1330 */ 1331 @Deprecated 1332 public List<Organization> getSupplierTarget() { 1333 if (this.supplierTarget == null) 1334 this.supplierTarget = new ArrayList<Organization>(); 1335 return this.supplierTarget; 1336 } 1337 1338 /** 1339 * @deprecated Use Reference#setResource(IBaseResource) instead 1340 */ 1341 @Deprecated 1342 public Organization addSupplierTarget() { 1343 Organization r = new Organization(); 1344 if (this.supplierTarget == null) 1345 this.supplierTarget = new ArrayList<Organization>(); 1346 this.supplierTarget.add(r); 1347 return r; 1348 } 1349 1350 /** 1351 * @return {@link #reason} (Why the supply item was requested.) 1352 */ 1353 public Type getReason() { 1354 return this.reason; 1355 } 1356 1357 /** 1358 * @return {@link #reason} (Why the supply item was requested.) 1359 */ 1360 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 1361 if (this.reason == null) 1362 return null; 1363 if (!(this.reason instanceof CodeableConcept)) 1364 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.reason.getClass().getName()+" was encountered"); 1365 return (CodeableConcept) this.reason; 1366 } 1367 1368 public boolean hasReasonCodeableConcept() { 1369 return this != null && this.reason instanceof CodeableConcept; 1370 } 1371 1372 /** 1373 * @return {@link #reason} (Why the supply item was requested.) 1374 */ 1375 public Reference getReasonReference() throws FHIRException { 1376 if (this.reason == null) 1377 return null; 1378 if (!(this.reason instanceof Reference)) 1379 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.reason.getClass().getName()+" was encountered"); 1380 return (Reference) this.reason; 1381 } 1382 1383 public boolean hasReasonReference() { 1384 return this != null && this.reason instanceof Reference; 1385 } 1386 1387 public boolean hasReason() { 1388 return this.reason != null && !this.reason.isEmpty(); 1389 } 1390 1391 /** 1392 * @param value {@link #reason} (Why the supply item was requested.) 1393 */ 1394 public SupplyRequest setReason(Type value) throws FHIRFormatError { 1395 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1396 throw new FHIRFormatError("Not the right type for SupplyRequest.reason[x]: "+value.fhirType()); 1397 this.reason = value; 1398 return this; 1399 } 1400 1401 /** 1402 * @return {@link #deliverFrom} (Where the supply is expected to come from.) 1403 */ 1404 public Reference getDeliverFrom() { 1405 if (this.deliverFrom == null) 1406 if (Configuration.errorOnAutoCreate()) 1407 throw new Error("Attempt to auto-create SupplyRequest.deliverFrom"); 1408 else if (Configuration.doAutoCreate()) 1409 this.deliverFrom = new Reference(); // cc 1410 return this.deliverFrom; 1411 } 1412 1413 public boolean hasDeliverFrom() { 1414 return this.deliverFrom != null && !this.deliverFrom.isEmpty(); 1415 } 1416 1417 /** 1418 * @param value {@link #deliverFrom} (Where the supply is expected to come from.) 1419 */ 1420 public SupplyRequest setDeliverFrom(Reference value) { 1421 this.deliverFrom = value; 1422 return this; 1423 } 1424 1425 /** 1426 * @return {@link #deliverFrom} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Where the supply is expected to come from.) 1427 */ 1428 public Resource getDeliverFromTarget() { 1429 return this.deliverFromTarget; 1430 } 1431 1432 /** 1433 * @param value {@link #deliverFrom} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Where the supply is expected to come from.) 1434 */ 1435 public SupplyRequest setDeliverFromTarget(Resource value) { 1436 this.deliverFromTarget = value; 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #deliverTo} (Where the supply is destined to go.) 1442 */ 1443 public Reference getDeliverTo() { 1444 if (this.deliverTo == null) 1445 if (Configuration.errorOnAutoCreate()) 1446 throw new Error("Attempt to auto-create SupplyRequest.deliverTo"); 1447 else if (Configuration.doAutoCreate()) 1448 this.deliverTo = new Reference(); // cc 1449 return this.deliverTo; 1450 } 1451 1452 public boolean hasDeliverTo() { 1453 return this.deliverTo != null && !this.deliverTo.isEmpty(); 1454 } 1455 1456 /** 1457 * @param value {@link #deliverTo} (Where the supply is destined to go.) 1458 */ 1459 public SupplyRequest setDeliverTo(Reference value) { 1460 this.deliverTo = value; 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #deliverTo} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Where the supply is destined to go.) 1466 */ 1467 public Resource getDeliverToTarget() { 1468 return this.deliverToTarget; 1469 } 1470 1471 /** 1472 * @param value {@link #deliverTo} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Where the supply is destined to go.) 1473 */ 1474 public SupplyRequest setDeliverToTarget(Resource value) { 1475 this.deliverToTarget = value; 1476 return this; 1477 } 1478 1479 protected void listChildren(List<Property> children) { 1480 super.listChildren(children); 1481 children.add(new Property("identifier", "Identifier", "Unique identifier for this supply request.", 0, 1, identifier)); 1482 children.add(new Property("status", "code", "Status of the supply request.", 0, 1, status)); 1483 children.add(new Property("category", "CodeableConcept", "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.", 0, 1, category)); 1484 children.add(new Property("priority", "code", "Indicates how quickly this SupplyRequest should be addressed with respect to other requests.", 0, 1, priority)); 1485 children.add(new Property("orderedItem", "", "The item being requested.", 0, 1, orderedItem)); 1486 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, occurrence)); 1487 children.add(new Property("authoredOn", "dateTime", "When the request was made.", 0, 1, authoredOn)); 1488 children.add(new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester)); 1489 children.add(new Property("supplier", "Reference(Organization)", "Who is intended to fulfill the request.", 0, java.lang.Integer.MAX_VALUE, supplier)); 1490 children.add(new Property("reason[x]", "CodeableConcept|Reference(Any)", "Why the supply item was requested.", 0, 1, reason)); 1491 children.add(new Property("deliverFrom", "Reference(Organization|Location)", "Where the supply is expected to come from.", 0, 1, deliverFrom)); 1492 children.add(new Property("deliverTo", "Reference(Organization|Location|Patient)", "Where the supply is destined to go.", 0, 1, deliverTo)); 1493 } 1494 1495 @Override 1496 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1497 switch (_hash) { 1498 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier for this supply request.", 0, 1, identifier); 1499 case -892481550: /*status*/ return new Property("status", "code", "Status of the supply request.", 0, 1, status); 1500 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.", 0, 1, category); 1501 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly this SupplyRequest should be addressed with respect to other requests.", 0, 1, priority); 1502 case 2129914144: /*orderedItem*/ return new Property("orderedItem", "", "The item being requested.", 0, 1, orderedItem); 1503 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, occurrence); 1504 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, occurrence); 1505 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, occurrence); 1506 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, occurrence); 1507 case 1515218299: /*occurrenceTiming*/ return new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, occurrence); 1508 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "When the request was made.", 0, 1, authoredOn); 1509 case 693933948: /*requester*/ return new Property("requester", "", "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester); 1510 case -1663305268: /*supplier*/ return new Property("supplier", "Reference(Organization)", "Who is intended to fulfill the request.", 0, java.lang.Integer.MAX_VALUE, supplier); 1511 case -669418564: /*reason[x]*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Why the supply item was requested.", 0, 1, reason); 1512 case -934964668: /*reason*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Why the supply item was requested.", 0, 1, reason); 1513 case -610155331: /*reasonCodeableConcept*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Why the supply item was requested.", 0, 1, reason); 1514 case -1146218137: /*reasonReference*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Why the supply item was requested.", 0, 1, reason); 1515 case -949323153: /*deliverFrom*/ return new Property("deliverFrom", "Reference(Organization|Location)", "Where the supply is expected to come from.", 0, 1, deliverFrom); 1516 case -242327936: /*deliverTo*/ return new Property("deliverTo", "Reference(Organization|Location|Patient)", "Where the supply is destined to go.", 0, 1, deliverTo); 1517 default: return super.getNamedProperty(_hash, _name, _checkValid); 1518 } 1519 1520 } 1521 1522 @Override 1523 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1524 switch (hash) { 1525 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1526 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<SupplyRequestStatus> 1527 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1528 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<RequestPriority> 1529 case 2129914144: /*orderedItem*/ return this.orderedItem == null ? new Base[0] : new Base[] {this.orderedItem}; // SupplyRequestOrderedItemComponent 1530 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 1531 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 1532 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // SupplyRequestRequesterComponent 1533 case -1663305268: /*supplier*/ return this.supplier == null ? new Base[0] : this.supplier.toArray(new Base[this.supplier.size()]); // Reference 1534 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // Type 1535 case -949323153: /*deliverFrom*/ return this.deliverFrom == null ? new Base[0] : new Base[] {this.deliverFrom}; // Reference 1536 case -242327936: /*deliverTo*/ return this.deliverTo == null ? new Base[0] : new Base[] {this.deliverTo}; // Reference 1537 default: return super.getProperty(hash, name, checkValid); 1538 } 1539 1540 } 1541 1542 @Override 1543 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1544 switch (hash) { 1545 case -1618432855: // identifier 1546 this.identifier = castToIdentifier(value); // Identifier 1547 return value; 1548 case -892481550: // status 1549 value = new SupplyRequestStatusEnumFactory().fromType(castToCode(value)); 1550 this.status = (Enumeration) value; // Enumeration<SupplyRequestStatus> 1551 return value; 1552 case 50511102: // category 1553 this.category = castToCodeableConcept(value); // CodeableConcept 1554 return value; 1555 case -1165461084: // priority 1556 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 1557 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1558 return value; 1559 case 2129914144: // orderedItem 1560 this.orderedItem = (SupplyRequestOrderedItemComponent) value; // SupplyRequestOrderedItemComponent 1561 return value; 1562 case 1687874001: // occurrence 1563 this.occurrence = castToType(value); // Type 1564 return value; 1565 case -1500852503: // authoredOn 1566 this.authoredOn = castToDateTime(value); // DateTimeType 1567 return value; 1568 case 693933948: // requester 1569 this.requester = (SupplyRequestRequesterComponent) value; // SupplyRequestRequesterComponent 1570 return value; 1571 case -1663305268: // supplier 1572 this.getSupplier().add(castToReference(value)); // Reference 1573 return value; 1574 case -934964668: // reason 1575 this.reason = castToType(value); // Type 1576 return value; 1577 case -949323153: // deliverFrom 1578 this.deliverFrom = castToReference(value); // Reference 1579 return value; 1580 case -242327936: // deliverTo 1581 this.deliverTo = castToReference(value); // Reference 1582 return value; 1583 default: return super.setProperty(hash, name, value); 1584 } 1585 1586 } 1587 1588 @Override 1589 public Base setProperty(String name, Base value) throws FHIRException { 1590 if (name.equals("identifier")) { 1591 this.identifier = castToIdentifier(value); // Identifier 1592 } else if (name.equals("status")) { 1593 value = new SupplyRequestStatusEnumFactory().fromType(castToCode(value)); 1594 this.status = (Enumeration) value; // Enumeration<SupplyRequestStatus> 1595 } else if (name.equals("category")) { 1596 this.category = castToCodeableConcept(value); // CodeableConcept 1597 } else if (name.equals("priority")) { 1598 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 1599 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1600 } else if (name.equals("orderedItem")) { 1601 this.orderedItem = (SupplyRequestOrderedItemComponent) value; // SupplyRequestOrderedItemComponent 1602 } else if (name.equals("occurrence[x]")) { 1603 this.occurrence = castToType(value); // Type 1604 } else if (name.equals("authoredOn")) { 1605 this.authoredOn = castToDateTime(value); // DateTimeType 1606 } else if (name.equals("requester")) { 1607 this.requester = (SupplyRequestRequesterComponent) value; // SupplyRequestRequesterComponent 1608 } else if (name.equals("supplier")) { 1609 this.getSupplier().add(castToReference(value)); 1610 } else if (name.equals("reason[x]")) { 1611 this.reason = castToType(value); // Type 1612 } else if (name.equals("deliverFrom")) { 1613 this.deliverFrom = castToReference(value); // Reference 1614 } else if (name.equals("deliverTo")) { 1615 this.deliverTo = castToReference(value); // Reference 1616 } else 1617 return super.setProperty(name, value); 1618 return value; 1619 } 1620 1621 @Override 1622 public Base makeProperty(int hash, String name) throws FHIRException { 1623 switch (hash) { 1624 case -1618432855: return getIdentifier(); 1625 case -892481550: return getStatusElement(); 1626 case 50511102: return getCategory(); 1627 case -1165461084: return getPriorityElement(); 1628 case 2129914144: return getOrderedItem(); 1629 case -2022646513: return getOccurrence(); 1630 case 1687874001: return getOccurrence(); 1631 case -1500852503: return getAuthoredOnElement(); 1632 case 693933948: return getRequester(); 1633 case -1663305268: return addSupplier(); 1634 case -669418564: return getReason(); 1635 case -934964668: return getReason(); 1636 case -949323153: return getDeliverFrom(); 1637 case -242327936: return getDeliverTo(); 1638 default: return super.makeProperty(hash, name); 1639 } 1640 1641 } 1642 1643 @Override 1644 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1645 switch (hash) { 1646 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1647 case -892481550: /*status*/ return new String[] {"code"}; 1648 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1649 case -1165461084: /*priority*/ return new String[] {"code"}; 1650 case 2129914144: /*orderedItem*/ return new String[] {}; 1651 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period", "Timing"}; 1652 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 1653 case 693933948: /*requester*/ return new String[] {}; 1654 case -1663305268: /*supplier*/ return new String[] {"Reference"}; 1655 case -934964668: /*reason*/ return new String[] {"CodeableConcept", "Reference"}; 1656 case -949323153: /*deliverFrom*/ return new String[] {"Reference"}; 1657 case -242327936: /*deliverTo*/ return new String[] {"Reference"}; 1658 default: return super.getTypesForProperty(hash, name); 1659 } 1660 1661 } 1662 1663 @Override 1664 public Base addChild(String name) throws FHIRException { 1665 if (name.equals("identifier")) { 1666 this.identifier = new Identifier(); 1667 return this.identifier; 1668 } 1669 else if (name.equals("status")) { 1670 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.status"); 1671 } 1672 else if (name.equals("category")) { 1673 this.category = new CodeableConcept(); 1674 return this.category; 1675 } 1676 else if (name.equals("priority")) { 1677 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.priority"); 1678 } 1679 else if (name.equals("orderedItem")) { 1680 this.orderedItem = new SupplyRequestOrderedItemComponent(); 1681 return this.orderedItem; 1682 } 1683 else if (name.equals("occurrenceDateTime")) { 1684 this.occurrence = new DateTimeType(); 1685 return this.occurrence; 1686 } 1687 else if (name.equals("occurrencePeriod")) { 1688 this.occurrence = new Period(); 1689 return this.occurrence; 1690 } 1691 else if (name.equals("occurrenceTiming")) { 1692 this.occurrence = new Timing(); 1693 return this.occurrence; 1694 } 1695 else if (name.equals("authoredOn")) { 1696 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.authoredOn"); 1697 } 1698 else if (name.equals("requester")) { 1699 this.requester = new SupplyRequestRequesterComponent(); 1700 return this.requester; 1701 } 1702 else if (name.equals("supplier")) { 1703 return addSupplier(); 1704 } 1705 else if (name.equals("reasonCodeableConcept")) { 1706 this.reason = new CodeableConcept(); 1707 return this.reason; 1708 } 1709 else if (name.equals("reasonReference")) { 1710 this.reason = new Reference(); 1711 return this.reason; 1712 } 1713 else if (name.equals("deliverFrom")) { 1714 this.deliverFrom = new Reference(); 1715 return this.deliverFrom; 1716 } 1717 else if (name.equals("deliverTo")) { 1718 this.deliverTo = new Reference(); 1719 return this.deliverTo; 1720 } 1721 else 1722 return super.addChild(name); 1723 } 1724 1725 public String fhirType() { 1726 return "SupplyRequest"; 1727 1728 } 1729 1730 public SupplyRequest copy() { 1731 SupplyRequest dst = new SupplyRequest(); 1732 copyValues(dst); 1733 dst.identifier = identifier == null ? null : identifier.copy(); 1734 dst.status = status == null ? null : status.copy(); 1735 dst.category = category == null ? null : category.copy(); 1736 dst.priority = priority == null ? null : priority.copy(); 1737 dst.orderedItem = orderedItem == null ? null : orderedItem.copy(); 1738 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1739 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 1740 dst.requester = requester == null ? null : requester.copy(); 1741 if (supplier != null) { 1742 dst.supplier = new ArrayList<Reference>(); 1743 for (Reference i : supplier) 1744 dst.supplier.add(i.copy()); 1745 }; 1746 dst.reason = reason == null ? null : reason.copy(); 1747 dst.deliverFrom = deliverFrom == null ? null : deliverFrom.copy(); 1748 dst.deliverTo = deliverTo == null ? null : deliverTo.copy(); 1749 return dst; 1750 } 1751 1752 protected SupplyRequest typedCopy() { 1753 return copy(); 1754 } 1755 1756 @Override 1757 public boolean equalsDeep(Base other_) { 1758 if (!super.equalsDeep(other_)) 1759 return false; 1760 if (!(other_ instanceof SupplyRequest)) 1761 return false; 1762 SupplyRequest o = (SupplyRequest) other_; 1763 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1764 && compareDeep(priority, o.priority, true) && compareDeep(orderedItem, o.orderedItem, true) && compareDeep(occurrence, o.occurrence, true) 1765 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) && compareDeep(supplier, o.supplier, true) 1766 && compareDeep(reason, o.reason, true) && compareDeep(deliverFrom, o.deliverFrom, true) && compareDeep(deliverTo, o.deliverTo, true) 1767 ; 1768 } 1769 1770 @Override 1771 public boolean equalsShallow(Base other_) { 1772 if (!super.equalsShallow(other_)) 1773 return false; 1774 if (!(other_ instanceof SupplyRequest)) 1775 return false; 1776 SupplyRequest o = (SupplyRequest) other_; 1777 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) && compareValues(authoredOn, o.authoredOn, true) 1778 ; 1779 } 1780 1781 public boolean isEmpty() { 1782 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 1783 , priority, orderedItem, occurrence, authoredOn, requester, supplier, reason, deliverFrom 1784 , deliverTo); 1785 } 1786 1787 @Override 1788 public ResourceType getResourceType() { 1789 return ResourceType.SupplyRequest; 1790 } 1791 1792 /** 1793 * Search parameter: <b>requester</b> 1794 * <p> 1795 * Description: <b>Individual making the request</b><br> 1796 * Type: <b>reference</b><br> 1797 * Path: <b>SupplyRequest.requester.agent</b><br> 1798 * </p> 1799 */ 1800 @SearchParamDefinition(name="requester", path="SupplyRequest.requester.agent", description="Individual making the request", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 1801 public static final String SP_REQUESTER = "requester"; 1802 /** 1803 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 1804 * <p> 1805 * Description: <b>Individual making the request</b><br> 1806 * Type: <b>reference</b><br> 1807 * Path: <b>SupplyRequest.requester.agent</b><br> 1808 * </p> 1809 */ 1810 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 1811 1812/** 1813 * Constant for fluent queries to be used to add include statements. Specifies 1814 * the path value of "<b>SupplyRequest:requester</b>". 1815 */ 1816 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("SupplyRequest:requester").toLocked(); 1817 1818 /** 1819 * Search parameter: <b>date</b> 1820 * <p> 1821 * Description: <b>When the request was made</b><br> 1822 * Type: <b>date</b><br> 1823 * Path: <b>SupplyRequest.authoredOn</b><br> 1824 * </p> 1825 */ 1826 @SearchParamDefinition(name="date", path="SupplyRequest.authoredOn", description="When the request was made", type="date" ) 1827 public static final String SP_DATE = "date"; 1828 /** 1829 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1830 * <p> 1831 * Description: <b>When the request was made</b><br> 1832 * Type: <b>date</b><br> 1833 * Path: <b>SupplyRequest.authoredOn</b><br> 1834 * </p> 1835 */ 1836 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1837 1838 /** 1839 * Search parameter: <b>identifier</b> 1840 * <p> 1841 * Description: <b>Unique identifier</b><br> 1842 * Type: <b>token</b><br> 1843 * Path: <b>SupplyRequest.identifier</b><br> 1844 * </p> 1845 */ 1846 @SearchParamDefinition(name="identifier", path="SupplyRequest.identifier", description="Unique identifier", type="token" ) 1847 public static final String SP_IDENTIFIER = "identifier"; 1848 /** 1849 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1850 * <p> 1851 * Description: <b>Unique identifier</b><br> 1852 * Type: <b>token</b><br> 1853 * Path: <b>SupplyRequest.identifier</b><br> 1854 * </p> 1855 */ 1856 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1857 1858 /** 1859 * Search parameter: <b>supplier</b> 1860 * <p> 1861 * Description: <b>Who is intended to fulfill the request</b><br> 1862 * Type: <b>reference</b><br> 1863 * Path: <b>SupplyRequest.supplier</b><br> 1864 * </p> 1865 */ 1866 @SearchParamDefinition(name="supplier", path="SupplyRequest.supplier", description="Who is intended to fulfill the request", type="reference", target={Organization.class } ) 1867 public static final String SP_SUPPLIER = "supplier"; 1868 /** 1869 * <b>Fluent Client</b> search parameter constant for <b>supplier</b> 1870 * <p> 1871 * Description: <b>Who is intended to fulfill the request</b><br> 1872 * Type: <b>reference</b><br> 1873 * Path: <b>SupplyRequest.supplier</b><br> 1874 * </p> 1875 */ 1876 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLIER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPLIER); 1877 1878/** 1879 * Constant for fluent queries to be used to add include statements. Specifies 1880 * the path value of "<b>SupplyRequest:supplier</b>". 1881 */ 1882 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLIER = new ca.uhn.fhir.model.api.Include("SupplyRequest:supplier").toLocked(); 1883 1884 /** 1885 * Search parameter: <b>category</b> 1886 * <p> 1887 * Description: <b>The kind of supply (central, non-stock, etc.)</b><br> 1888 * Type: <b>token</b><br> 1889 * Path: <b>SupplyRequest.category</b><br> 1890 * </p> 1891 */ 1892 @SearchParamDefinition(name="category", path="SupplyRequest.category", description="The kind of supply (central, non-stock, etc.)", type="token" ) 1893 public static final String SP_CATEGORY = "category"; 1894 /** 1895 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1896 * <p> 1897 * Description: <b>The kind of supply (central, non-stock, etc.)</b><br> 1898 * Type: <b>token</b><br> 1899 * Path: <b>SupplyRequest.category</b><br> 1900 * </p> 1901 */ 1902 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1903 1904 /** 1905 * Search parameter: <b>status</b> 1906 * <p> 1907 * Description: <b>draft | active | suspended +</b><br> 1908 * Type: <b>token</b><br> 1909 * Path: <b>SupplyRequest.status</b><br> 1910 * </p> 1911 */ 1912 @SearchParamDefinition(name="status", path="SupplyRequest.status", description="draft | active | suspended +", type="token" ) 1913 public static final String SP_STATUS = "status"; 1914 /** 1915 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1916 * <p> 1917 * Description: <b>draft | active | suspended +</b><br> 1918 * Type: <b>token</b><br> 1919 * Path: <b>SupplyRequest.status</b><br> 1920 * </p> 1921 */ 1922 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1923 1924 1925}