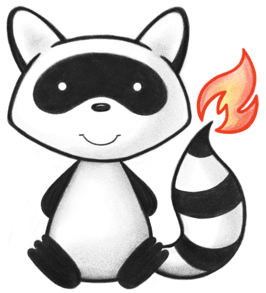
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A task to be performed. 051 */ 052@ResourceDef(name="Task", profile="http://hl7.org/fhir/Profile/Task") 053public class Task extends DomainResource { 054 055 public enum TaskStatus { 056 /** 057 * The task is not yet ready to be acted upon. 058 */ 059 DRAFT, 060 /** 061 * The task is ready to be acted upon and action is sought. 062 */ 063 REQUESTED, 064 /** 065 * A potential performer has claimed ownership of the task and is evaluating whether to perform it. 066 */ 067 RECEIVED, 068 /** 069 * The potential performer has agreed to execute the task but has not yet started work. 070 */ 071 ACCEPTED, 072 /** 073 * The potential performer who claimed ownership of the task has decided not to execute it prior to performing any action. 074 */ 075 REJECTED, 076 /** 077 * Task is ready to be performed, but no action has yet been taken. Used in place of requested/received/accepted/rejected when request assignment and acceptance is a given. 078 */ 079 READY, 080 /** 081 * The task was not completed. 082 */ 083 CANCELLED, 084 /** 085 * Task has been started but is not yet complete. 086 */ 087 INPROGRESS, 088 /** 089 * Task has been started but work has been paused. 090 */ 091 ONHOLD, 092 /** 093 * The task was attempted but could not be completed due to some error. 094 */ 095 FAILED, 096 /** 097 * The task has been completed. 098 */ 099 COMPLETED, 100 /** 101 * The task should never have existed and is retained only because of the possibility it may have used. 102 */ 103 ENTEREDINERROR, 104 /** 105 * added to help the parsers with the generic types 106 */ 107 NULL; 108 public static TaskStatus fromCode(String codeString) throws FHIRException { 109 if (codeString == null || "".equals(codeString)) 110 return null; 111 if ("draft".equals(codeString)) 112 return DRAFT; 113 if ("requested".equals(codeString)) 114 return REQUESTED; 115 if ("received".equals(codeString)) 116 return RECEIVED; 117 if ("accepted".equals(codeString)) 118 return ACCEPTED; 119 if ("rejected".equals(codeString)) 120 return REJECTED; 121 if ("ready".equals(codeString)) 122 return READY; 123 if ("cancelled".equals(codeString)) 124 return CANCELLED; 125 if ("in-progress".equals(codeString)) 126 return INPROGRESS; 127 if ("on-hold".equals(codeString)) 128 return ONHOLD; 129 if ("failed".equals(codeString)) 130 return FAILED; 131 if ("completed".equals(codeString)) 132 return COMPLETED; 133 if ("entered-in-error".equals(codeString)) 134 return ENTEREDINERROR; 135 if (Configuration.isAcceptInvalidEnums()) 136 return null; 137 else 138 throw new FHIRException("Unknown TaskStatus code '"+codeString+"'"); 139 } 140 public String toCode() { 141 switch (this) { 142 case DRAFT: return "draft"; 143 case REQUESTED: return "requested"; 144 case RECEIVED: return "received"; 145 case ACCEPTED: return "accepted"; 146 case REJECTED: return "rejected"; 147 case READY: return "ready"; 148 case CANCELLED: return "cancelled"; 149 case INPROGRESS: return "in-progress"; 150 case ONHOLD: return "on-hold"; 151 case FAILED: return "failed"; 152 case COMPLETED: return "completed"; 153 case ENTEREDINERROR: return "entered-in-error"; 154 case NULL: return null; 155 default: return "?"; 156 } 157 } 158 public String getSystem() { 159 switch (this) { 160 case DRAFT: return "http://hl7.org/fhir/task-status"; 161 case REQUESTED: return "http://hl7.org/fhir/task-status"; 162 case RECEIVED: return "http://hl7.org/fhir/task-status"; 163 case ACCEPTED: return "http://hl7.org/fhir/task-status"; 164 case REJECTED: return "http://hl7.org/fhir/task-status"; 165 case READY: return "http://hl7.org/fhir/task-status"; 166 case CANCELLED: return "http://hl7.org/fhir/task-status"; 167 case INPROGRESS: return "http://hl7.org/fhir/task-status"; 168 case ONHOLD: return "http://hl7.org/fhir/task-status"; 169 case FAILED: return "http://hl7.org/fhir/task-status"; 170 case COMPLETED: return "http://hl7.org/fhir/task-status"; 171 case ENTEREDINERROR: return "http://hl7.org/fhir/task-status"; 172 case NULL: return null; 173 default: return "?"; 174 } 175 } 176 public String getDefinition() { 177 switch (this) { 178 case DRAFT: return "The task is not yet ready to be acted upon."; 179 case REQUESTED: return "The task is ready to be acted upon and action is sought."; 180 case RECEIVED: return "A potential performer has claimed ownership of the task and is evaluating whether to perform it."; 181 case ACCEPTED: return "The potential performer has agreed to execute the task but has not yet started work."; 182 case REJECTED: return "The potential performer who claimed ownership of the task has decided not to execute it prior to performing any action."; 183 case READY: return "Task is ready to be performed, but no action has yet been taken. Used in place of requested/received/accepted/rejected when request assignment and acceptance is a given."; 184 case CANCELLED: return "The task was not completed."; 185 case INPROGRESS: return "Task has been started but is not yet complete."; 186 case ONHOLD: return "Task has been started but work has been paused."; 187 case FAILED: return "The task was attempted but could not be completed due to some error."; 188 case COMPLETED: return "The task has been completed."; 189 case ENTEREDINERROR: return "The task should never have existed and is retained only because of the possibility it may have used."; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getDisplay() { 195 switch (this) { 196 case DRAFT: return "Draft"; 197 case REQUESTED: return "Requested"; 198 case RECEIVED: return "Received"; 199 case ACCEPTED: return "Accepted"; 200 case REJECTED: return "Rejected"; 201 case READY: return "Ready"; 202 case CANCELLED: return "Cancelled"; 203 case INPROGRESS: return "In Progress"; 204 case ONHOLD: return "On Hold"; 205 case FAILED: return "Failed"; 206 case COMPLETED: return "Completed"; 207 case ENTEREDINERROR: return "Entered in Error"; 208 case NULL: return null; 209 default: return "?"; 210 } 211 } 212 } 213 214 public static class TaskStatusEnumFactory implements EnumFactory<TaskStatus> { 215 public TaskStatus fromCode(String codeString) throws IllegalArgumentException { 216 if (codeString == null || "".equals(codeString)) 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("draft".equals(codeString)) 220 return TaskStatus.DRAFT; 221 if ("requested".equals(codeString)) 222 return TaskStatus.REQUESTED; 223 if ("received".equals(codeString)) 224 return TaskStatus.RECEIVED; 225 if ("accepted".equals(codeString)) 226 return TaskStatus.ACCEPTED; 227 if ("rejected".equals(codeString)) 228 return TaskStatus.REJECTED; 229 if ("ready".equals(codeString)) 230 return TaskStatus.READY; 231 if ("cancelled".equals(codeString)) 232 return TaskStatus.CANCELLED; 233 if ("in-progress".equals(codeString)) 234 return TaskStatus.INPROGRESS; 235 if ("on-hold".equals(codeString)) 236 return TaskStatus.ONHOLD; 237 if ("failed".equals(codeString)) 238 return TaskStatus.FAILED; 239 if ("completed".equals(codeString)) 240 return TaskStatus.COMPLETED; 241 if ("entered-in-error".equals(codeString)) 242 return TaskStatus.ENTEREDINERROR; 243 throw new IllegalArgumentException("Unknown TaskStatus code '"+codeString+"'"); 244 } 245 public Enumeration<TaskStatus> fromType(PrimitiveType<?> code) throws FHIRException { 246 if (code == null) 247 return null; 248 if (code.isEmpty()) 249 return new Enumeration<TaskStatus>(this); 250 String codeString = code.asStringValue(); 251 if (codeString == null || "".equals(codeString)) 252 return null; 253 if ("draft".equals(codeString)) 254 return new Enumeration<TaskStatus>(this, TaskStatus.DRAFT); 255 if ("requested".equals(codeString)) 256 return new Enumeration<TaskStatus>(this, TaskStatus.REQUESTED); 257 if ("received".equals(codeString)) 258 return new Enumeration<TaskStatus>(this, TaskStatus.RECEIVED); 259 if ("accepted".equals(codeString)) 260 return new Enumeration<TaskStatus>(this, TaskStatus.ACCEPTED); 261 if ("rejected".equals(codeString)) 262 return new Enumeration<TaskStatus>(this, TaskStatus.REJECTED); 263 if ("ready".equals(codeString)) 264 return new Enumeration<TaskStatus>(this, TaskStatus.READY); 265 if ("cancelled".equals(codeString)) 266 return new Enumeration<TaskStatus>(this, TaskStatus.CANCELLED); 267 if ("in-progress".equals(codeString)) 268 return new Enumeration<TaskStatus>(this, TaskStatus.INPROGRESS); 269 if ("on-hold".equals(codeString)) 270 return new Enumeration<TaskStatus>(this, TaskStatus.ONHOLD); 271 if ("failed".equals(codeString)) 272 return new Enumeration<TaskStatus>(this, TaskStatus.FAILED); 273 if ("completed".equals(codeString)) 274 return new Enumeration<TaskStatus>(this, TaskStatus.COMPLETED); 275 if ("entered-in-error".equals(codeString)) 276 return new Enumeration<TaskStatus>(this, TaskStatus.ENTEREDINERROR); 277 throw new FHIRException("Unknown TaskStatus code '"+codeString+"'"); 278 } 279 public String toCode(TaskStatus code) { 280 if (code == TaskStatus.NULL) 281 return null; 282 if (code == TaskStatus.DRAFT) 283 return "draft"; 284 if (code == TaskStatus.REQUESTED) 285 return "requested"; 286 if (code == TaskStatus.RECEIVED) 287 return "received"; 288 if (code == TaskStatus.ACCEPTED) 289 return "accepted"; 290 if (code == TaskStatus.REJECTED) 291 return "rejected"; 292 if (code == TaskStatus.READY) 293 return "ready"; 294 if (code == TaskStatus.CANCELLED) 295 return "cancelled"; 296 if (code == TaskStatus.INPROGRESS) 297 return "in-progress"; 298 if (code == TaskStatus.ONHOLD) 299 return "on-hold"; 300 if (code == TaskStatus.FAILED) 301 return "failed"; 302 if (code == TaskStatus.COMPLETED) 303 return "completed"; 304 if (code == TaskStatus.ENTEREDINERROR) 305 return "entered-in-error"; 306 return "?"; 307 } 308 public String toSystem(TaskStatus code) { 309 return code.getSystem(); 310 } 311 } 312 313 public enum TaskIntent { 314 /** 315 * The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 316 */ 317 PROPOSAL, 318 /** 319 * The request represents an intension to ensure something occurs without providing an authorization for others to act 320 */ 321 PLAN, 322 /** 323 * The request represents a request/demand and authorization for action 324 */ 325 ORDER, 326 /** 327 * The request represents an original authorization for action 328 */ 329 ORIGINALORDER, 330 /** 331 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization 332 */ 333 REFLEXORDER, 334 /** 335 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order 336 */ 337 FILLERORDER, 338 /** 339 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 340 */ 341 INSTANCEORDER, 342 /** 343 * The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. 344 345Refer to [[[RequestGroup]]] for additional information on how this status is used 346 */ 347 OPTION, 348 /** 349 * added to help the parsers with the generic types 350 */ 351 NULL; 352 public static TaskIntent fromCode(String codeString) throws FHIRException { 353 if (codeString == null || "".equals(codeString)) 354 return null; 355 if ("proposal".equals(codeString)) 356 return PROPOSAL; 357 if ("plan".equals(codeString)) 358 return PLAN; 359 if ("order".equals(codeString)) 360 return ORDER; 361 if ("original-order".equals(codeString)) 362 return ORIGINALORDER; 363 if ("reflex-order".equals(codeString)) 364 return REFLEXORDER; 365 if ("filler-order".equals(codeString)) 366 return FILLERORDER; 367 if ("instance-order".equals(codeString)) 368 return INSTANCEORDER; 369 if ("option".equals(codeString)) 370 return OPTION; 371 if (Configuration.isAcceptInvalidEnums()) 372 return null; 373 else 374 throw new FHIRException("Unknown TaskIntent code '"+codeString+"'"); 375 } 376 public String toCode() { 377 switch (this) { 378 case PROPOSAL: return "proposal"; 379 case PLAN: return "plan"; 380 case ORDER: return "order"; 381 case ORIGINALORDER: return "original-order"; 382 case REFLEXORDER: return "reflex-order"; 383 case FILLERORDER: return "filler-order"; 384 case INSTANCEORDER: return "instance-order"; 385 case OPTION: return "option"; 386 case NULL: return null; 387 default: return "?"; 388 } 389 } 390 public String getSystem() { 391 switch (this) { 392 case PROPOSAL: return "http://hl7.org/fhir/request-intent"; 393 case PLAN: return "http://hl7.org/fhir/request-intent"; 394 case ORDER: return "http://hl7.org/fhir/request-intent"; 395 case ORIGINALORDER: return "http://hl7.org/fhir/request-intent"; 396 case REFLEXORDER: return "http://hl7.org/fhir/request-intent"; 397 case FILLERORDER: return "http://hl7.org/fhir/request-intent"; 398 case INSTANCEORDER: return "http://hl7.org/fhir/request-intent"; 399 case OPTION: return "http://hl7.org/fhir/request-intent"; 400 case NULL: return null; 401 default: return "?"; 402 } 403 } 404 public String getDefinition() { 405 switch (this) { 406 case PROPOSAL: return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 407 case PLAN: return "The request represents an intension to ensure something occurs without providing an authorization for others to act"; 408 case ORDER: return "The request represents a request/demand and authorization for action"; 409 case ORIGINALORDER: return "The request represents an original authorization for action"; 410 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization"; 411 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order"; 412 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 413 case OPTION: return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests.\n\nRefer to [[[RequestGroup]]] for additional information on how this status is used"; 414 case NULL: return null; 415 default: return "?"; 416 } 417 } 418 public String getDisplay() { 419 switch (this) { 420 case PROPOSAL: return "Proposal"; 421 case PLAN: return "Plan"; 422 case ORDER: return "Order"; 423 case ORIGINALORDER: return "Original Order"; 424 case REFLEXORDER: return "Reflex Order"; 425 case FILLERORDER: return "Filler Order"; 426 case INSTANCEORDER: return "Instance Order"; 427 case OPTION: return "Option"; 428 case NULL: return null; 429 default: return "?"; 430 } 431 } 432 } 433 434 public static class TaskIntentEnumFactory implements EnumFactory<TaskIntent> { 435 public TaskIntent fromCode(String codeString) throws IllegalArgumentException { 436 if (codeString == null || "".equals(codeString)) 437 if (codeString == null || "".equals(codeString)) 438 return null; 439 if ("proposal".equals(codeString)) 440 return TaskIntent.PROPOSAL; 441 if ("plan".equals(codeString)) 442 return TaskIntent.PLAN; 443 if ("order".equals(codeString)) 444 return TaskIntent.ORDER; 445 if ("original-order".equals(codeString)) 446 return TaskIntent.ORIGINALORDER; 447 if ("reflex-order".equals(codeString)) 448 return TaskIntent.REFLEXORDER; 449 if ("filler-order".equals(codeString)) 450 return TaskIntent.FILLERORDER; 451 if ("instance-order".equals(codeString)) 452 return TaskIntent.INSTANCEORDER; 453 if ("option".equals(codeString)) 454 return TaskIntent.OPTION; 455 throw new IllegalArgumentException("Unknown TaskIntent code '"+codeString+"'"); 456 } 457 public Enumeration<TaskIntent> fromType(PrimitiveType<?> code) throws FHIRException { 458 if (code == null) 459 return null; 460 if (code.isEmpty()) 461 return new Enumeration<TaskIntent>(this); 462 String codeString = code.asStringValue(); 463 if (codeString == null || "".equals(codeString)) 464 return null; 465 if ("proposal".equals(codeString)) 466 return new Enumeration<TaskIntent>(this, TaskIntent.PROPOSAL); 467 if ("plan".equals(codeString)) 468 return new Enumeration<TaskIntent>(this, TaskIntent.PLAN); 469 if ("order".equals(codeString)) 470 return new Enumeration<TaskIntent>(this, TaskIntent.ORDER); 471 if ("original-order".equals(codeString)) 472 return new Enumeration<TaskIntent>(this, TaskIntent.ORIGINALORDER); 473 if ("reflex-order".equals(codeString)) 474 return new Enumeration<TaskIntent>(this, TaskIntent.REFLEXORDER); 475 if ("filler-order".equals(codeString)) 476 return new Enumeration<TaskIntent>(this, TaskIntent.FILLERORDER); 477 if ("instance-order".equals(codeString)) 478 return new Enumeration<TaskIntent>(this, TaskIntent.INSTANCEORDER); 479 if ("option".equals(codeString)) 480 return new Enumeration<TaskIntent>(this, TaskIntent.OPTION); 481 throw new FHIRException("Unknown TaskIntent code '"+codeString+"'"); 482 } 483 public String toCode(TaskIntent code) { 484 if (code == TaskIntent.NULL) 485 return null; 486 if (code == TaskIntent.PROPOSAL) 487 return "proposal"; 488 if (code == TaskIntent.PLAN) 489 return "plan"; 490 if (code == TaskIntent.ORDER) 491 return "order"; 492 if (code == TaskIntent.ORIGINALORDER) 493 return "original-order"; 494 if (code == TaskIntent.REFLEXORDER) 495 return "reflex-order"; 496 if (code == TaskIntent.FILLERORDER) 497 return "filler-order"; 498 if (code == TaskIntent.INSTANCEORDER) 499 return "instance-order"; 500 if (code == TaskIntent.OPTION) 501 return "option"; 502 return "?"; 503 } 504 public String toSystem(TaskIntent code) { 505 return code.getSystem(); 506 } 507 } 508 509 public enum TaskPriority { 510 /** 511 * The request has normal priority 512 */ 513 ROUTINE, 514 /** 515 * The request should be actioned promptly - higher priority than routine 516 */ 517 URGENT, 518 /** 519 * The request should be actioned as soon as possible - higher priority than urgent 520 */ 521 ASAP, 522 /** 523 * The request should be actioned immediately - highest possible priority. E.g. an emergency 524 */ 525 STAT, 526 /** 527 * added to help the parsers with the generic types 528 */ 529 NULL; 530 public static TaskPriority fromCode(String codeString) throws FHIRException { 531 if (codeString == null || "".equals(codeString)) 532 return null; 533 if ("routine".equals(codeString)) 534 return ROUTINE; 535 if ("urgent".equals(codeString)) 536 return URGENT; 537 if ("asap".equals(codeString)) 538 return ASAP; 539 if ("stat".equals(codeString)) 540 return STAT; 541 if (Configuration.isAcceptInvalidEnums()) 542 return null; 543 else 544 throw new FHIRException("Unknown TaskPriority code '"+codeString+"'"); 545 } 546 public String toCode() { 547 switch (this) { 548 case ROUTINE: return "routine"; 549 case URGENT: return "urgent"; 550 case ASAP: return "asap"; 551 case STAT: return "stat"; 552 case NULL: return null; 553 default: return "?"; 554 } 555 } 556 public String getSystem() { 557 switch (this) { 558 case ROUTINE: return "http://hl7.org/fhir/request-priority"; 559 case URGENT: return "http://hl7.org/fhir/request-priority"; 560 case ASAP: return "http://hl7.org/fhir/request-priority"; 561 case STAT: return "http://hl7.org/fhir/request-priority"; 562 case NULL: return null; 563 default: return "?"; 564 } 565 } 566 public String getDefinition() { 567 switch (this) { 568 case ROUTINE: return "The request has normal priority"; 569 case URGENT: return "The request should be actioned promptly - higher priority than routine"; 570 case ASAP: return "The request should be actioned as soon as possible - higher priority than urgent"; 571 case STAT: return "The request should be actioned immediately - highest possible priority. E.g. an emergency"; 572 case NULL: return null; 573 default: return "?"; 574 } 575 } 576 public String getDisplay() { 577 switch (this) { 578 case ROUTINE: return "Routine"; 579 case URGENT: return "Urgent"; 580 case ASAP: return "ASAP"; 581 case STAT: return "STAT"; 582 case NULL: return null; 583 default: return "?"; 584 } 585 } 586 } 587 588 public static class TaskPriorityEnumFactory implements EnumFactory<TaskPriority> { 589 public TaskPriority fromCode(String codeString) throws IllegalArgumentException { 590 if (codeString == null || "".equals(codeString)) 591 if (codeString == null || "".equals(codeString)) 592 return null; 593 if ("routine".equals(codeString)) 594 return TaskPriority.ROUTINE; 595 if ("urgent".equals(codeString)) 596 return TaskPriority.URGENT; 597 if ("asap".equals(codeString)) 598 return TaskPriority.ASAP; 599 if ("stat".equals(codeString)) 600 return TaskPriority.STAT; 601 throw new IllegalArgumentException("Unknown TaskPriority code '"+codeString+"'"); 602 } 603 public Enumeration<TaskPriority> fromType(PrimitiveType<?> code) throws FHIRException { 604 if (code == null) 605 return null; 606 if (code.isEmpty()) 607 return new Enumeration<TaskPriority>(this); 608 String codeString = code.asStringValue(); 609 if (codeString == null || "".equals(codeString)) 610 return null; 611 if ("routine".equals(codeString)) 612 return new Enumeration<TaskPriority>(this, TaskPriority.ROUTINE); 613 if ("urgent".equals(codeString)) 614 return new Enumeration<TaskPriority>(this, TaskPriority.URGENT); 615 if ("asap".equals(codeString)) 616 return new Enumeration<TaskPriority>(this, TaskPriority.ASAP); 617 if ("stat".equals(codeString)) 618 return new Enumeration<TaskPriority>(this, TaskPriority.STAT); 619 throw new FHIRException("Unknown TaskPriority code '"+codeString+"'"); 620 } 621 public String toCode(TaskPriority code) { 622 if (code == TaskPriority.NULL) 623 return null; 624 if (code == TaskPriority.ROUTINE) 625 return "routine"; 626 if (code == TaskPriority.URGENT) 627 return "urgent"; 628 if (code == TaskPriority.ASAP) 629 return "asap"; 630 if (code == TaskPriority.STAT) 631 return "stat"; 632 return "?"; 633 } 634 public String toSystem(TaskPriority code) { 635 return code.getSystem(); 636 } 637 } 638 639 @Block() 640 public static class TaskRequesterComponent extends BackboneElement implements IBaseBackboneElement { 641 /** 642 * The device, practitioner, etc. who initiated the task. 643 */ 644 @Child(name = "agent", type = {Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=1, min=1, max=1, modifier=false, summary=true) 645 @Description(shortDefinition="Individual asking for task", formalDefinition="The device, practitioner, etc. who initiated the task." ) 646 protected Reference agent; 647 648 /** 649 * The actual object that is the target of the reference (The device, practitioner, etc. who initiated the task.) 650 */ 651 protected Resource agentTarget; 652 653 /** 654 * The organization the device or practitioner was acting on behalf of when they initiated the task. 655 */ 656 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=false) 657 @Description(shortDefinition="Organization individual is acting for", formalDefinition="The organization the device or practitioner was acting on behalf of when they initiated the task." ) 658 protected Reference onBehalfOf; 659 660 /** 661 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of when they initiated the task.) 662 */ 663 protected Organization onBehalfOfTarget; 664 665 private static final long serialVersionUID = -71453027L; 666 667 /** 668 * Constructor 669 */ 670 public TaskRequesterComponent() { 671 super(); 672 } 673 674 /** 675 * Constructor 676 */ 677 public TaskRequesterComponent(Reference agent) { 678 super(); 679 this.agent = agent; 680 } 681 682 /** 683 * @return {@link #agent} (The device, practitioner, etc. who initiated the task.) 684 */ 685 public Reference getAgent() { 686 if (this.agent == null) 687 if (Configuration.errorOnAutoCreate()) 688 throw new Error("Attempt to auto-create TaskRequesterComponent.agent"); 689 else if (Configuration.doAutoCreate()) 690 this.agent = new Reference(); // cc 691 return this.agent; 692 } 693 694 public boolean hasAgent() { 695 return this.agent != null && !this.agent.isEmpty(); 696 } 697 698 /** 699 * @param value {@link #agent} (The device, practitioner, etc. who initiated the task.) 700 */ 701 public TaskRequesterComponent setAgent(Reference value) { 702 this.agent = value; 703 return this; 704 } 705 706 /** 707 * @return {@link #agent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the task.) 708 */ 709 public Resource getAgentTarget() { 710 return this.agentTarget; 711 } 712 713 /** 714 * @param value {@link #agent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who initiated the task.) 715 */ 716 public TaskRequesterComponent setAgentTarget(Resource value) { 717 this.agentTarget = value; 718 return this; 719 } 720 721 /** 722 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of when they initiated the task.) 723 */ 724 public Reference getOnBehalfOf() { 725 if (this.onBehalfOf == null) 726 if (Configuration.errorOnAutoCreate()) 727 throw new Error("Attempt to auto-create TaskRequesterComponent.onBehalfOf"); 728 else if (Configuration.doAutoCreate()) 729 this.onBehalfOf = new Reference(); // cc 730 return this.onBehalfOf; 731 } 732 733 public boolean hasOnBehalfOf() { 734 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 735 } 736 737 /** 738 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of when they initiated the task.) 739 */ 740 public TaskRequesterComponent setOnBehalfOf(Reference value) { 741 this.onBehalfOf = value; 742 return this; 743 } 744 745 /** 746 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of when they initiated the task.) 747 */ 748 public Organization getOnBehalfOfTarget() { 749 if (this.onBehalfOfTarget == null) 750 if (Configuration.errorOnAutoCreate()) 751 throw new Error("Attempt to auto-create TaskRequesterComponent.onBehalfOf"); 752 else if (Configuration.doAutoCreate()) 753 this.onBehalfOfTarget = new Organization(); // aa 754 return this.onBehalfOfTarget; 755 } 756 757 /** 758 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of when they initiated the task.) 759 */ 760 public TaskRequesterComponent setOnBehalfOfTarget(Organization value) { 761 this.onBehalfOfTarget = value; 762 return this; 763 } 764 765 protected void listChildren(List<Property> children) { 766 super.listChildren(children); 767 children.add(new Property("agent", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", "The device, practitioner, etc. who initiated the task.", 0, 1, agent)); 768 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of when they initiated the task.", 0, 1, onBehalfOf)); 769 } 770 771 @Override 772 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 773 switch (_hash) { 774 case 92750597: /*agent*/ return new Property("agent", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", "The device, practitioner, etc. who initiated the task.", 0, 1, agent); 775 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of when they initiated the task.", 0, 1, onBehalfOf); 776 default: return super.getNamedProperty(_hash, _name, _checkValid); 777 } 778 779 } 780 781 @Override 782 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 783 switch (hash) { 784 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : new Base[] {this.agent}; // Reference 785 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 786 default: return super.getProperty(hash, name, checkValid); 787 } 788 789 } 790 791 @Override 792 public Base setProperty(int hash, String name, Base value) throws FHIRException { 793 switch (hash) { 794 case 92750597: // agent 795 this.agent = castToReference(value); // Reference 796 return value; 797 case -14402964: // onBehalfOf 798 this.onBehalfOf = castToReference(value); // Reference 799 return value; 800 default: return super.setProperty(hash, name, value); 801 } 802 803 } 804 805 @Override 806 public Base setProperty(String name, Base value) throws FHIRException { 807 if (name.equals("agent")) { 808 this.agent = castToReference(value); // Reference 809 } else if (name.equals("onBehalfOf")) { 810 this.onBehalfOf = castToReference(value); // Reference 811 } else 812 return super.setProperty(name, value); 813 return value; 814 } 815 816 @Override 817 public Base makeProperty(int hash, String name) throws FHIRException { 818 switch (hash) { 819 case 92750597: return getAgent(); 820 case -14402964: return getOnBehalfOf(); 821 default: return super.makeProperty(hash, name); 822 } 823 824 } 825 826 @Override 827 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 828 switch (hash) { 829 case 92750597: /*agent*/ return new String[] {"Reference"}; 830 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 831 default: return super.getTypesForProperty(hash, name); 832 } 833 834 } 835 836 @Override 837 public Base addChild(String name) throws FHIRException { 838 if (name.equals("agent")) { 839 this.agent = new Reference(); 840 return this.agent; 841 } 842 else if (name.equals("onBehalfOf")) { 843 this.onBehalfOf = new Reference(); 844 return this.onBehalfOf; 845 } 846 else 847 return super.addChild(name); 848 } 849 850 public TaskRequesterComponent copy() { 851 TaskRequesterComponent dst = new TaskRequesterComponent(); 852 copyValues(dst); 853 dst.agent = agent == null ? null : agent.copy(); 854 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 855 return dst; 856 } 857 858 @Override 859 public boolean equalsDeep(Base other_) { 860 if (!super.equalsDeep(other_)) 861 return false; 862 if (!(other_ instanceof TaskRequesterComponent)) 863 return false; 864 TaskRequesterComponent o = (TaskRequesterComponent) other_; 865 return compareDeep(agent, o.agent, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 866 } 867 868 @Override 869 public boolean equalsShallow(Base other_) { 870 if (!super.equalsShallow(other_)) 871 return false; 872 if (!(other_ instanceof TaskRequesterComponent)) 873 return false; 874 TaskRequesterComponent o = (TaskRequesterComponent) other_; 875 return true; 876 } 877 878 public boolean isEmpty() { 879 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(agent, onBehalfOf); 880 } 881 882 public String fhirType() { 883 return "Task.requester"; 884 885 } 886 887 } 888 889 @Block() 890 public static class TaskRestrictionComponent extends BackboneElement implements IBaseBackboneElement { 891 /** 892 * Indicates the number of times the requested action should occur. 893 */ 894 @Child(name = "repetitions", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 895 @Description(shortDefinition="How many times to repeat", formalDefinition="Indicates the number of times the requested action should occur." ) 896 protected PositiveIntType repetitions; 897 898 /** 899 * Over what time-period is fulfillment sought. 900 */ 901 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 902 @Description(shortDefinition="When fulfillment sought", formalDefinition="Over what time-period is fulfillment sought." ) 903 protected Period period; 904 905 /** 906 * For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought? 907 */ 908 @Child(name = "recipient", type = {Patient.class, Practitioner.class, RelatedPerson.class, Group.class, Organization.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 909 @Description(shortDefinition="For whom is fulfillment sought?", formalDefinition="For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?" ) 910 protected List<Reference> recipient; 911 /** 912 * The actual objects that are the target of the reference (For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?) 913 */ 914 protected List<Resource> recipientTarget; 915 916 917 private static final long serialVersionUID = 1503908360L; 918 919 /** 920 * Constructor 921 */ 922 public TaskRestrictionComponent() { 923 super(); 924 } 925 926 /** 927 * @return {@link #repetitions} (Indicates the number of times the requested action should occur.). This is the underlying object with id, value and extensions. The accessor "getRepetitions" gives direct access to the value 928 */ 929 public PositiveIntType getRepetitionsElement() { 930 if (this.repetitions == null) 931 if (Configuration.errorOnAutoCreate()) 932 throw new Error("Attempt to auto-create TaskRestrictionComponent.repetitions"); 933 else if (Configuration.doAutoCreate()) 934 this.repetitions = new PositiveIntType(); // bb 935 return this.repetitions; 936 } 937 938 public boolean hasRepetitionsElement() { 939 return this.repetitions != null && !this.repetitions.isEmpty(); 940 } 941 942 public boolean hasRepetitions() { 943 return this.repetitions != null && !this.repetitions.isEmpty(); 944 } 945 946 /** 947 * @param value {@link #repetitions} (Indicates the number of times the requested action should occur.). This is the underlying object with id, value and extensions. The accessor "getRepetitions" gives direct access to the value 948 */ 949 public TaskRestrictionComponent setRepetitionsElement(PositiveIntType value) { 950 this.repetitions = value; 951 return this; 952 } 953 954 /** 955 * @return Indicates the number of times the requested action should occur. 956 */ 957 public int getRepetitions() { 958 return this.repetitions == null || this.repetitions.isEmpty() ? 0 : this.repetitions.getValue(); 959 } 960 961 /** 962 * @param value Indicates the number of times the requested action should occur. 963 */ 964 public TaskRestrictionComponent setRepetitions(int value) { 965 if (this.repetitions == null) 966 this.repetitions = new PositiveIntType(); 967 this.repetitions.setValue(value); 968 return this; 969 } 970 971 /** 972 * @return {@link #period} (Over what time-period is fulfillment sought.) 973 */ 974 public Period getPeriod() { 975 if (this.period == null) 976 if (Configuration.errorOnAutoCreate()) 977 throw new Error("Attempt to auto-create TaskRestrictionComponent.period"); 978 else if (Configuration.doAutoCreate()) 979 this.period = new Period(); // cc 980 return this.period; 981 } 982 983 public boolean hasPeriod() { 984 return this.period != null && !this.period.isEmpty(); 985 } 986 987 /** 988 * @param value {@link #period} (Over what time-period is fulfillment sought.) 989 */ 990 public TaskRestrictionComponent setPeriod(Period value) { 991 this.period = value; 992 return this; 993 } 994 995 /** 996 * @return {@link #recipient} (For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?) 997 */ 998 public List<Reference> getRecipient() { 999 if (this.recipient == null) 1000 this.recipient = new ArrayList<Reference>(); 1001 return this.recipient; 1002 } 1003 1004 /** 1005 * @return Returns a reference to <code>this</code> for easy method chaining 1006 */ 1007 public TaskRestrictionComponent setRecipient(List<Reference> theRecipient) { 1008 this.recipient = theRecipient; 1009 return this; 1010 } 1011 1012 public boolean hasRecipient() { 1013 if (this.recipient == null) 1014 return false; 1015 for (Reference item : this.recipient) 1016 if (!item.isEmpty()) 1017 return true; 1018 return false; 1019 } 1020 1021 public Reference addRecipient() { //3 1022 Reference t = new Reference(); 1023 if (this.recipient == null) 1024 this.recipient = new ArrayList<Reference>(); 1025 this.recipient.add(t); 1026 return t; 1027 } 1028 1029 public TaskRestrictionComponent addRecipient(Reference t) { //3 1030 if (t == null) 1031 return this; 1032 if (this.recipient == null) 1033 this.recipient = new ArrayList<Reference>(); 1034 this.recipient.add(t); 1035 return this; 1036 } 1037 1038 /** 1039 * @return The first repetition of repeating field {@link #recipient}, creating it if it does not already exist 1040 */ 1041 public Reference getRecipientFirstRep() { 1042 if (getRecipient().isEmpty()) { 1043 addRecipient(); 1044 } 1045 return getRecipient().get(0); 1046 } 1047 1048 /** 1049 * @deprecated Use Reference#setResource(IBaseResource) instead 1050 */ 1051 @Deprecated 1052 public List<Resource> getRecipientTarget() { 1053 if (this.recipientTarget == null) 1054 this.recipientTarget = new ArrayList<Resource>(); 1055 return this.recipientTarget; 1056 } 1057 1058 protected void listChildren(List<Property> children) { 1059 super.listChildren(children); 1060 children.add(new Property("repetitions", "positiveInt", "Indicates the number of times the requested action should occur.", 0, 1, repetitions)); 1061 children.add(new Property("period", "Period", "Over what time-period is fulfillment sought.", 0, 1, period)); 1062 children.add(new Property("recipient", "Reference(Patient|Practitioner|RelatedPerson|Group|Organization)", "For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?", 0, java.lang.Integer.MAX_VALUE, recipient)); 1063 } 1064 1065 @Override 1066 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1067 switch (_hash) { 1068 case 984367650: /*repetitions*/ return new Property("repetitions", "positiveInt", "Indicates the number of times the requested action should occur.", 0, 1, repetitions); 1069 case -991726143: /*period*/ return new Property("period", "Period", "Over what time-period is fulfillment sought.", 0, 1, period); 1070 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Patient|Practitioner|RelatedPerson|Group|Organization)", "For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?", 0, java.lang.Integer.MAX_VALUE, recipient); 1071 default: return super.getNamedProperty(_hash, _name, _checkValid); 1072 } 1073 1074 } 1075 1076 @Override 1077 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1078 switch (hash) { 1079 case 984367650: /*repetitions*/ return this.repetitions == null ? new Base[0] : new Base[] {this.repetitions}; // PositiveIntType 1080 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1081 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 1082 default: return super.getProperty(hash, name, checkValid); 1083 } 1084 1085 } 1086 1087 @Override 1088 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1089 switch (hash) { 1090 case 984367650: // repetitions 1091 this.repetitions = castToPositiveInt(value); // PositiveIntType 1092 return value; 1093 case -991726143: // period 1094 this.period = castToPeriod(value); // Period 1095 return value; 1096 case 820081177: // recipient 1097 this.getRecipient().add(castToReference(value)); // Reference 1098 return value; 1099 default: return super.setProperty(hash, name, value); 1100 } 1101 1102 } 1103 1104 @Override 1105 public Base setProperty(String name, Base value) throws FHIRException { 1106 if (name.equals("repetitions")) { 1107 this.repetitions = castToPositiveInt(value); // PositiveIntType 1108 } else if (name.equals("period")) { 1109 this.period = castToPeriod(value); // Period 1110 } else if (name.equals("recipient")) { 1111 this.getRecipient().add(castToReference(value)); 1112 } else 1113 return super.setProperty(name, value); 1114 return value; 1115 } 1116 1117 @Override 1118 public Base makeProperty(int hash, String name) throws FHIRException { 1119 switch (hash) { 1120 case 984367650: return getRepetitionsElement(); 1121 case -991726143: return getPeriod(); 1122 case 820081177: return addRecipient(); 1123 default: return super.makeProperty(hash, name); 1124 } 1125 1126 } 1127 1128 @Override 1129 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1130 switch (hash) { 1131 case 984367650: /*repetitions*/ return new String[] {"positiveInt"}; 1132 case -991726143: /*period*/ return new String[] {"Period"}; 1133 case 820081177: /*recipient*/ return new String[] {"Reference"}; 1134 default: return super.getTypesForProperty(hash, name); 1135 } 1136 1137 } 1138 1139 @Override 1140 public Base addChild(String name) throws FHIRException { 1141 if (name.equals("repetitions")) { 1142 throw new FHIRException("Cannot call addChild on a singleton property Task.repetitions"); 1143 } 1144 else if (name.equals("period")) { 1145 this.period = new Period(); 1146 return this.period; 1147 } 1148 else if (name.equals("recipient")) { 1149 return addRecipient(); 1150 } 1151 else 1152 return super.addChild(name); 1153 } 1154 1155 public TaskRestrictionComponent copy() { 1156 TaskRestrictionComponent dst = new TaskRestrictionComponent(); 1157 copyValues(dst); 1158 dst.repetitions = repetitions == null ? null : repetitions.copy(); 1159 dst.period = period == null ? null : period.copy(); 1160 if (recipient != null) { 1161 dst.recipient = new ArrayList<Reference>(); 1162 for (Reference i : recipient) 1163 dst.recipient.add(i.copy()); 1164 }; 1165 return dst; 1166 } 1167 1168 @Override 1169 public boolean equalsDeep(Base other_) { 1170 if (!super.equalsDeep(other_)) 1171 return false; 1172 if (!(other_ instanceof TaskRestrictionComponent)) 1173 return false; 1174 TaskRestrictionComponent o = (TaskRestrictionComponent) other_; 1175 return compareDeep(repetitions, o.repetitions, true) && compareDeep(period, o.period, true) && compareDeep(recipient, o.recipient, true) 1176 ; 1177 } 1178 1179 @Override 1180 public boolean equalsShallow(Base other_) { 1181 if (!super.equalsShallow(other_)) 1182 return false; 1183 if (!(other_ instanceof TaskRestrictionComponent)) 1184 return false; 1185 TaskRestrictionComponent o = (TaskRestrictionComponent) other_; 1186 return compareValues(repetitions, o.repetitions, true); 1187 } 1188 1189 public boolean isEmpty() { 1190 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(repetitions, period, recipient 1191 ); 1192 } 1193 1194 public String fhirType() { 1195 return "Task.restriction"; 1196 1197 } 1198 1199 } 1200 1201 @Block() 1202 public static class ParameterComponent extends BackboneElement implements IBaseBackboneElement { 1203 /** 1204 * A code or description indicating how the input is intended to be used as part of the task execution. 1205 */ 1206 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1207 @Description(shortDefinition="Label for the input", formalDefinition="A code or description indicating how the input is intended to be used as part of the task execution." ) 1208 protected CodeableConcept type; 1209 1210 /** 1211 * The value of the input parameter as a basic type. 1212 */ 1213 @Child(name = "value", type = {}, order=2, min=1, max=1, modifier=false, summary=false) 1214 @Description(shortDefinition="Content to use in performing the task", formalDefinition="The value of the input parameter as a basic type." ) 1215 protected org.hl7.fhir.dstu3.model.Type value; 1216 1217 private static final long serialVersionUID = -852629026L; 1218 1219 /** 1220 * Constructor 1221 */ 1222 public ParameterComponent() { 1223 super(); 1224 } 1225 1226 /** 1227 * Constructor 1228 */ 1229 public ParameterComponent(CodeableConcept type, org.hl7.fhir.dstu3.model.Type value) { 1230 super(); 1231 this.type = type; 1232 this.value = value; 1233 } 1234 1235 /** 1236 * @return {@link #type} (A code or description indicating how the input is intended to be used as part of the task execution.) 1237 */ 1238 public CodeableConcept getType() { 1239 if (this.type == null) 1240 if (Configuration.errorOnAutoCreate()) 1241 throw new Error("Attempt to auto-create ParameterComponent.type"); 1242 else if (Configuration.doAutoCreate()) 1243 this.type = new CodeableConcept(); // cc 1244 return this.type; 1245 } 1246 1247 public boolean hasType() { 1248 return this.type != null && !this.type.isEmpty(); 1249 } 1250 1251 /** 1252 * @param value {@link #type} (A code or description indicating how the input is intended to be used as part of the task execution.) 1253 */ 1254 public ParameterComponent setType(CodeableConcept value) { 1255 this.type = value; 1256 return this; 1257 } 1258 1259 /** 1260 * @return {@link #value} (The value of the input parameter as a basic type.) 1261 */ 1262 public org.hl7.fhir.dstu3.model.Type getValue() { 1263 return this.value; 1264 } 1265 1266 public boolean hasValue() { 1267 return this.value != null && !this.value.isEmpty(); 1268 } 1269 1270 /** 1271 * @param value {@link #value} (The value of the input parameter as a basic type.) 1272 */ 1273 public ParameterComponent setValue(org.hl7.fhir.dstu3.model.Type value) { 1274 this.value = value; 1275 return this; 1276 } 1277 1278 protected void listChildren(List<Property> children) { 1279 super.listChildren(children); 1280 children.add(new Property("type", "CodeableConcept", "A code or description indicating how the input is intended to be used as part of the task execution.", 0, 1, type)); 1281 children.add(new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value)); 1282 } 1283 1284 @Override 1285 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1286 switch (_hash) { 1287 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code or description indicating how the input is intended to be used as part of the task execution.", 0, 1, type); 1288 case -1410166417: /*value[x]*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1289 case 111972721: /*value*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1290 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1291 case 733421943: /*valueBoolean*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1292 case -786218365: /*valueCanonical*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1293 case -766209282: /*valueCode*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1294 case -766192449: /*valueDate*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1295 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1296 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1297 case 231604844: /*valueId*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1298 case -1668687056: /*valueInstant*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1299 case -1668204915: /*valueInteger*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1300 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1301 case -1410178407: /*valueOid*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1302 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1303 case -1424603934: /*valueString*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1304 case -765708322: /*valueTime*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1305 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1306 case -1410172357: /*valueUri*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1307 case -1410172354: /*valueUrl*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1308 case -765667124: /*valueUuid*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1309 case -478981821: /*valueAddress*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1310 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1311 case -475566732: /*valueAttachment*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1312 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1313 case -1887705029: /*valueCoding*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1314 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1315 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1316 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1317 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1318 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1319 case 2030761548: /*valueRange*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1320 case 2030767386: /*valueRatio*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1321 case 1755241690: /*valueReference*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1322 case -962229101: /*valueSampledData*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1323 case -540985785: /*valueSignature*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1324 case -1406282469: /*valueTiming*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1325 case -1858636920: /*valueDosage*/ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value); 1326 default: return super.getNamedProperty(_hash, _name, _checkValid); 1327 } 1328 1329 } 1330 1331 @Override 1332 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1333 switch (hash) { 1334 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1335 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // org.hl7.fhir.dstu3.model.Type 1336 default: return super.getProperty(hash, name, checkValid); 1337 } 1338 1339 } 1340 1341 @Override 1342 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1343 switch (hash) { 1344 case 3575610: // type 1345 this.type = castToCodeableConcept(value); // CodeableConcept 1346 return value; 1347 case 111972721: // value 1348 this.value = castToType(value); // org.hl7.fhir.dstu3.model.Type 1349 return value; 1350 default: return super.setProperty(hash, name, value); 1351 } 1352 1353 } 1354 1355 @Override 1356 public Base setProperty(String name, Base value) throws FHIRException { 1357 if (name.equals("type")) { 1358 this.type = castToCodeableConcept(value); // CodeableConcept 1359 } else if (name.equals("value[x]")) { 1360 this.value = castToType(value); // org.hl7.fhir.dstu3.model.Type 1361 } else 1362 return super.setProperty(name, value); 1363 return value; 1364 } 1365 1366 @Override 1367 public Base makeProperty(int hash, String name) throws FHIRException { 1368 switch (hash) { 1369 case 3575610: return getType(); 1370 case -1410166417: return getValue(); 1371 case 111972721: return getValue(); 1372 default: return super.makeProperty(hash, name); 1373 } 1374 1375 } 1376 1377 @Override 1378 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1379 switch (hash) { 1380 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1381 case 111972721: /*value*/ return new String[] {"*"}; 1382 default: return super.getTypesForProperty(hash, name); 1383 } 1384 1385 } 1386 1387 @Override 1388 public Base addChild(String name) throws FHIRException { 1389 if (name.equals("type")) { 1390 this.type = new CodeableConcept(); 1391 return this.type; 1392 } 1393 else if (name.equals("valueBoolean")) { 1394 this.value = new BooleanType(); 1395 return this.value; 1396 } 1397 else if (name.equals("valueInteger")) { 1398 this.value = new IntegerType(); 1399 return this.value; 1400 } 1401 else if (name.equals("valueDecimal")) { 1402 this.value = new DecimalType(); 1403 return this.value; 1404 } 1405 else if (name.equals("valueBase64Binary")) { 1406 this.value = new Base64BinaryType(); 1407 return this.value; 1408 } 1409 else if (name.equals("valueInstant")) { 1410 this.value = new InstantType(); 1411 return this.value; 1412 } 1413 else if (name.equals("valueString")) { 1414 this.value = new StringType(); 1415 return this.value; 1416 } 1417 else if (name.equals("valueUri")) { 1418 this.value = new UriType(); 1419 return this.value; 1420 } 1421 else if (name.equals("valueDate")) { 1422 this.value = new DateType(); 1423 return this.value; 1424 } 1425 else if (name.equals("valueDateTime")) { 1426 this.value = new DateTimeType(); 1427 return this.value; 1428 } 1429 else if (name.equals("valueTime")) { 1430 this.value = new TimeType(); 1431 return this.value; 1432 } 1433 else if (name.equals("valueCode")) { 1434 this.value = new CodeType(); 1435 return this.value; 1436 } 1437 else if (name.equals("valueOid")) { 1438 this.value = new OidType(); 1439 return this.value; 1440 } 1441 else if (name.equals("valueId")) { 1442 this.value = new IdType(); 1443 return this.value; 1444 } 1445 else if (name.equals("valueUnsignedInt")) { 1446 this.value = new UnsignedIntType(); 1447 return this.value; 1448 } 1449 else if (name.equals("valuePositiveInt")) { 1450 this.value = new PositiveIntType(); 1451 return this.value; 1452 } 1453 else if (name.equals("valueMarkdown")) { 1454 this.value = new MarkdownType(); 1455 return this.value; 1456 } 1457 else if (name.equals("valueAnnotation")) { 1458 this.value = new Annotation(); 1459 return this.value; 1460 } 1461 else if (name.equals("valueAttachment")) { 1462 this.value = new Attachment(); 1463 return this.value; 1464 } 1465 else if (name.equals("valueIdentifier")) { 1466 this.value = new Identifier(); 1467 return this.value; 1468 } 1469 else if (name.equals("valueCodeableConcept")) { 1470 this.value = new CodeableConcept(); 1471 return this.value; 1472 } 1473 else if (name.equals("valueCoding")) { 1474 this.value = new Coding(); 1475 return this.value; 1476 } 1477 else if (name.equals("valueQuantity")) { 1478 this.value = new Quantity(); 1479 return this.value; 1480 } 1481 else if (name.equals("valueRange")) { 1482 this.value = new Range(); 1483 return this.value; 1484 } 1485 else if (name.equals("valuePeriod")) { 1486 this.value = new Period(); 1487 return this.value; 1488 } 1489 else if (name.equals("valueRatio")) { 1490 this.value = new Ratio(); 1491 return this.value; 1492 } 1493 else if (name.equals("valueSampledData")) { 1494 this.value = new SampledData(); 1495 return this.value; 1496 } 1497 else if (name.equals("valueSignature")) { 1498 this.value = new Signature(); 1499 return this.value; 1500 } 1501 else if (name.equals("valueHumanName")) { 1502 this.value = new HumanName(); 1503 return this.value; 1504 } 1505 else if (name.equals("valueAddress")) { 1506 this.value = new Address(); 1507 return this.value; 1508 } 1509 else if (name.equals("valueContactPoint")) { 1510 this.value = new ContactPoint(); 1511 return this.value; 1512 } 1513 else if (name.equals("valueTiming")) { 1514 this.value = new Timing(); 1515 return this.value; 1516 } 1517 else if (name.equals("valueReference")) { 1518 this.value = new Reference(); 1519 return this.value; 1520 } 1521 else if (name.equals("valueMeta")) { 1522 this.value = new Meta(); 1523 return this.value; 1524 } 1525 else 1526 return super.addChild(name); 1527 } 1528 1529 public ParameterComponent copy() { 1530 ParameterComponent dst = new ParameterComponent(); 1531 copyValues(dst); 1532 dst.type = type == null ? null : type.copy(); 1533 dst.value = value == null ? null : value.copy(); 1534 return dst; 1535 } 1536 1537 @Override 1538 public boolean equalsDeep(Base other_) { 1539 if (!super.equalsDeep(other_)) 1540 return false; 1541 if (!(other_ instanceof ParameterComponent)) 1542 return false; 1543 ParameterComponent o = (ParameterComponent) other_; 1544 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 1545 } 1546 1547 @Override 1548 public boolean equalsShallow(Base other_) { 1549 if (!super.equalsShallow(other_)) 1550 return false; 1551 if (!(other_ instanceof ParameterComponent)) 1552 return false; 1553 ParameterComponent o = (ParameterComponent) other_; 1554 return true; 1555 } 1556 1557 public boolean isEmpty() { 1558 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 1559 } 1560 1561 public String fhirType() { 1562 return "Task.input"; 1563 1564 } 1565 1566 } 1567 1568 @Block() 1569 public static class TaskOutputComponent extends BackboneElement implements IBaseBackboneElement { 1570 /** 1571 * The name of the Output parameter. 1572 */ 1573 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1574 @Description(shortDefinition="Label for output", formalDefinition="The name of the Output parameter." ) 1575 protected CodeableConcept type; 1576 1577 /** 1578 * The value of the Output parameter as a basic type. 1579 */ 1580 @Child(name = "value", type = {}, order=2, min=1, max=1, modifier=false, summary=false) 1581 @Description(shortDefinition="Result of output", formalDefinition="The value of the Output parameter as a basic type." ) 1582 protected org.hl7.fhir.dstu3.model.Type value; 1583 1584 private static final long serialVersionUID = -852629026L; 1585 1586 /** 1587 * Constructor 1588 */ 1589 public TaskOutputComponent() { 1590 super(); 1591 } 1592 1593 /** 1594 * Constructor 1595 */ 1596 public TaskOutputComponent(CodeableConcept type, org.hl7.fhir.dstu3.model.Type value) { 1597 super(); 1598 this.type = type; 1599 this.value = value; 1600 } 1601 1602 /** 1603 * @return {@link #type} (The name of the Output parameter.) 1604 */ 1605 public CodeableConcept getType() { 1606 if (this.type == null) 1607 if (Configuration.errorOnAutoCreate()) 1608 throw new Error("Attempt to auto-create TaskOutputComponent.type"); 1609 else if (Configuration.doAutoCreate()) 1610 this.type = new CodeableConcept(); // cc 1611 return this.type; 1612 } 1613 1614 public boolean hasType() { 1615 return this.type != null && !this.type.isEmpty(); 1616 } 1617 1618 /** 1619 * @param value {@link #type} (The name of the Output parameter.) 1620 */ 1621 public TaskOutputComponent setType(CodeableConcept value) { 1622 this.type = value; 1623 return this; 1624 } 1625 1626 /** 1627 * @return {@link #value} (The value of the Output parameter as a basic type.) 1628 */ 1629 public org.hl7.fhir.dstu3.model.Type getValue() { 1630 return this.value; 1631 } 1632 1633 public boolean hasValue() { 1634 return this.value != null && !this.value.isEmpty(); 1635 } 1636 1637 /** 1638 * @param value {@link #value} (The value of the Output parameter as a basic type.) 1639 */ 1640 public TaskOutputComponent setValue(org.hl7.fhir.dstu3.model.Type value) { 1641 this.value = value; 1642 return this; 1643 } 1644 1645 protected void listChildren(List<Property> children) { 1646 super.listChildren(children); 1647 children.add(new Property("type", "CodeableConcept", "The name of the Output parameter.", 0, 1, type)); 1648 children.add(new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value)); 1649 } 1650 1651 @Override 1652 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1653 switch (_hash) { 1654 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The name of the Output parameter.", 0, 1, type); 1655 case -1410166417: /*value[x]*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1656 case 111972721: /*value*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1657 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1658 case 733421943: /*valueBoolean*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1659 case -786218365: /*valueCanonical*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1660 case -766209282: /*valueCode*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1661 case -766192449: /*valueDate*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1662 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1663 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1664 case 231604844: /*valueId*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1665 case -1668687056: /*valueInstant*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1666 case -1668204915: /*valueInteger*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1667 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1668 case -1410178407: /*valueOid*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1669 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1670 case -1424603934: /*valueString*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1671 case -765708322: /*valueTime*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1672 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1673 case -1410172357: /*valueUri*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1674 case -1410172354: /*valueUrl*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1675 case -765667124: /*valueUuid*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1676 case -478981821: /*valueAddress*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1677 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1678 case -475566732: /*valueAttachment*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1679 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1680 case -1887705029: /*valueCoding*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1681 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1682 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1683 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1684 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1685 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1686 case 2030761548: /*valueRange*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1687 case 2030767386: /*valueRatio*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1688 case 1755241690: /*valueReference*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1689 case -962229101: /*valueSampledData*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1690 case -540985785: /*valueSignature*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1691 case -1406282469: /*valueTiming*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1692 case -1858636920: /*valueDosage*/ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value); 1693 default: return super.getNamedProperty(_hash, _name, _checkValid); 1694 } 1695 1696 } 1697 1698 @Override 1699 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1700 switch (hash) { 1701 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1702 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // org.hl7.fhir.dstu3.model.Type 1703 default: return super.getProperty(hash, name, checkValid); 1704 } 1705 1706 } 1707 1708 @Override 1709 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1710 switch (hash) { 1711 case 3575610: // type 1712 this.type = castToCodeableConcept(value); // CodeableConcept 1713 return value; 1714 case 111972721: // value 1715 this.value = castToType(value); // org.hl7.fhir.dstu3.model.Type 1716 return value; 1717 default: return super.setProperty(hash, name, value); 1718 } 1719 1720 } 1721 1722 @Override 1723 public Base setProperty(String name, Base value) throws FHIRException { 1724 if (name.equals("type")) { 1725 this.type = castToCodeableConcept(value); // CodeableConcept 1726 } else if (name.equals("value[x]")) { 1727 this.value = castToType(value); // org.hl7.fhir.dstu3.model.Type 1728 } else 1729 return super.setProperty(name, value); 1730 return value; 1731 } 1732 1733 @Override 1734 public Base makeProperty(int hash, String name) throws FHIRException { 1735 switch (hash) { 1736 case 3575610: return getType(); 1737 case -1410166417: return getValue(); 1738 case 111972721: return getValue(); 1739 default: return super.makeProperty(hash, name); 1740 } 1741 1742 } 1743 1744 @Override 1745 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1746 switch (hash) { 1747 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1748 case 111972721: /*value*/ return new String[] {"*"}; 1749 default: return super.getTypesForProperty(hash, name); 1750 } 1751 1752 } 1753 1754 @Override 1755 public Base addChild(String name) throws FHIRException { 1756 if (name.equals("type")) { 1757 this.type = new CodeableConcept(); 1758 return this.type; 1759 } 1760 else if (name.equals("valueBoolean")) { 1761 this.value = new BooleanType(); 1762 return this.value; 1763 } 1764 else if (name.equals("valueInteger")) { 1765 this.value = new IntegerType(); 1766 return this.value; 1767 } 1768 else if (name.equals("valueDecimal")) { 1769 this.value = new DecimalType(); 1770 return this.value; 1771 } 1772 else if (name.equals("valueBase64Binary")) { 1773 this.value = new Base64BinaryType(); 1774 return this.value; 1775 } 1776 else if (name.equals("valueInstant")) { 1777 this.value = new InstantType(); 1778 return this.value; 1779 } 1780 else if (name.equals("valueString")) { 1781 this.value = new StringType(); 1782 return this.value; 1783 } 1784 else if (name.equals("valueUri")) { 1785 this.value = new UriType(); 1786 return this.value; 1787 } 1788 else if (name.equals("valueDate")) { 1789 this.value = new DateType(); 1790 return this.value; 1791 } 1792 else if (name.equals("valueDateTime")) { 1793 this.value = new DateTimeType(); 1794 return this.value; 1795 } 1796 else if (name.equals("valueTime")) { 1797 this.value = new TimeType(); 1798 return this.value; 1799 } 1800 else if (name.equals("valueCode")) { 1801 this.value = new CodeType(); 1802 return this.value; 1803 } 1804 else if (name.equals("valueOid")) { 1805 this.value = new OidType(); 1806 return this.value; 1807 } 1808 else if (name.equals("valueId")) { 1809 this.value = new IdType(); 1810 return this.value; 1811 } 1812 else if (name.equals("valueUnsignedInt")) { 1813 this.value = new UnsignedIntType(); 1814 return this.value; 1815 } 1816 else if (name.equals("valuePositiveInt")) { 1817 this.value = new PositiveIntType(); 1818 return this.value; 1819 } 1820 else if (name.equals("valueMarkdown")) { 1821 this.value = new MarkdownType(); 1822 return this.value; 1823 } 1824 else if (name.equals("valueAnnotation")) { 1825 this.value = new Annotation(); 1826 return this.value; 1827 } 1828 else if (name.equals("valueAttachment")) { 1829 this.value = new Attachment(); 1830 return this.value; 1831 } 1832 else if (name.equals("valueIdentifier")) { 1833 this.value = new Identifier(); 1834 return this.value; 1835 } 1836 else if (name.equals("valueCodeableConcept")) { 1837 this.value = new CodeableConcept(); 1838 return this.value; 1839 } 1840 else if (name.equals("valueCoding")) { 1841 this.value = new Coding(); 1842 return this.value; 1843 } 1844 else if (name.equals("valueQuantity")) { 1845 this.value = new Quantity(); 1846 return this.value; 1847 } 1848 else if (name.equals("valueRange")) { 1849 this.value = new Range(); 1850 return this.value; 1851 } 1852 else if (name.equals("valuePeriod")) { 1853 this.value = new Period(); 1854 return this.value; 1855 } 1856 else if (name.equals("valueRatio")) { 1857 this.value = new Ratio(); 1858 return this.value; 1859 } 1860 else if (name.equals("valueSampledData")) { 1861 this.value = new SampledData(); 1862 return this.value; 1863 } 1864 else if (name.equals("valueSignature")) { 1865 this.value = new Signature(); 1866 return this.value; 1867 } 1868 else if (name.equals("valueHumanName")) { 1869 this.value = new HumanName(); 1870 return this.value; 1871 } 1872 else if (name.equals("valueAddress")) { 1873 this.value = new Address(); 1874 return this.value; 1875 } 1876 else if (name.equals("valueContactPoint")) { 1877 this.value = new ContactPoint(); 1878 return this.value; 1879 } 1880 else if (name.equals("valueTiming")) { 1881 this.value = new Timing(); 1882 return this.value; 1883 } 1884 else if (name.equals("valueReference")) { 1885 this.value = new Reference(); 1886 return this.value; 1887 } 1888 else if (name.equals("valueMeta")) { 1889 this.value = new Meta(); 1890 return this.value; 1891 } 1892 else 1893 return super.addChild(name); 1894 } 1895 1896 public TaskOutputComponent copy() { 1897 TaskOutputComponent dst = new TaskOutputComponent(); 1898 copyValues(dst); 1899 dst.type = type == null ? null : type.copy(); 1900 dst.value = value == null ? null : value.copy(); 1901 return dst; 1902 } 1903 1904 @Override 1905 public boolean equalsDeep(Base other_) { 1906 if (!super.equalsDeep(other_)) 1907 return false; 1908 if (!(other_ instanceof TaskOutputComponent)) 1909 return false; 1910 TaskOutputComponent o = (TaskOutputComponent) other_; 1911 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 1912 } 1913 1914 @Override 1915 public boolean equalsShallow(Base other_) { 1916 if (!super.equalsShallow(other_)) 1917 return false; 1918 if (!(other_ instanceof TaskOutputComponent)) 1919 return false; 1920 TaskOutputComponent o = (TaskOutputComponent) other_; 1921 return true; 1922 } 1923 1924 public boolean isEmpty() { 1925 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 1926 } 1927 1928 public String fhirType() { 1929 return "Task.output"; 1930 1931 } 1932 1933 } 1934 1935 /** 1936 * The business identifier for this task. 1937 */ 1938 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1939 @Description(shortDefinition="Task Instance Identifier", formalDefinition="The business identifier for this task." ) 1940 protected List<Identifier> identifier; 1941 1942 /** 1943 * A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc. 1944 */ 1945 @Child(name = "definition", type = {UriType.class, ActivityDefinition.class}, order=1, min=0, max=1, modifier=false, summary=true) 1946 @Description(shortDefinition="Formal definition of task", formalDefinition="A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc." ) 1947 protected Type definition; 1948 1949 /** 1950 * BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a "request" resource such as a ProcedureRequest, MedicationRequest, ProcedureRequest, CarePlan, etc. which is distinct from the "request" resource the task is seeking to fulfil. This latter resource is referenced by FocusOn. For example, based on a ProcedureRequest (= BasedOn), a task is created to fulfil a procedureRequest ( = FocusOn ) to collect a specimen from a patient. 1951 */ 1952 @Child(name = "basedOn", type = {Reference.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1953 @Description(shortDefinition="Request fulfilled by this task", formalDefinition="BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ProcedureRequest, MedicationRequest, ProcedureRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfil. This latter resource is referenced by FocusOn. For example, based on a ProcedureRequest (= BasedOn), a task is created to fulfil a procedureRequest ( = FocusOn ) to collect a specimen from a patient." ) 1954 protected List<Reference> basedOn; 1955 /** 1956 * The actual objects that are the target of the reference (BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a "request" resource such as a ProcedureRequest, MedicationRequest, ProcedureRequest, CarePlan, etc. which is distinct from the "request" resource the task is seeking to fulfil. This latter resource is referenced by FocusOn. For example, based on a ProcedureRequest (= BasedOn), a task is created to fulfil a procedureRequest ( = FocusOn ) to collect a specimen from a patient.) 1957 */ 1958 protected List<Resource> basedOnTarget; 1959 1960 1961 /** 1962 * An identifier that links together multiple tasks and other requests that were created in the same context. 1963 */ 1964 @Child(name = "groupIdentifier", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=true) 1965 @Description(shortDefinition="Requisition or grouper id", formalDefinition="An identifier that links together multiple tasks and other requests that were created in the same context." ) 1966 protected Identifier groupIdentifier; 1967 1968 /** 1969 * Task that this particular task is part of. 1970 */ 1971 @Child(name = "partOf", type = {Task.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1972 @Description(shortDefinition="Composite task", formalDefinition="Task that this particular task is part of." ) 1973 protected List<Reference> partOf; 1974 /** 1975 * The actual objects that are the target of the reference (Task that this particular task is part of.) 1976 */ 1977 protected List<Task> partOfTarget; 1978 1979 1980 /** 1981 * The current status of the task. 1982 */ 1983 @Child(name = "status", type = {CodeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 1984 @Description(shortDefinition="draft | requested | received | accepted | +", formalDefinition="The current status of the task." ) 1985 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/task-status") 1986 protected Enumeration<TaskStatus> status; 1987 1988 /** 1989 * An explanation as to why this task is held, failed, was refused, etc. 1990 */ 1991 @Child(name = "statusReason", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 1992 @Description(shortDefinition="Reason for current status", formalDefinition="An explanation as to why this task is held, failed, was refused, etc." ) 1993 protected CodeableConcept statusReason; 1994 1995 /** 1996 * Contains business-specific nuances of the business state. 1997 */ 1998 @Child(name = "businessStatus", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 1999 @Description(shortDefinition="E.g. \"Specimen collected\", \"IV prepped\"", formalDefinition="Contains business-specific nuances of the business state." ) 2000 protected CodeableConcept businessStatus; 2001 2002 /** 2003 * Indicates the "level" of actionability associated with the Task. I.e. Is this a proposed task, a planned task, an actionable task, etc. 2004 */ 2005 @Child(name = "intent", type = {CodeType.class}, order=8, min=1, max=1, modifier=false, summary=true) 2006 @Description(shortDefinition="proposal | plan | order +", formalDefinition="Indicates the \"level\" of actionability associated with the Task. I.e. Is this a proposed task, a planned task, an actionable task, etc." ) 2007 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-intent") 2008 protected Enumeration<TaskIntent> intent; 2009 2010 /** 2011 * Indicates how quickly the Task should be addressed with respect to other requests. 2012 */ 2013 @Child(name = "priority", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=false) 2014 @Description(shortDefinition="normal | urgent | asap | stat", formalDefinition="Indicates how quickly the Task should be addressed with respect to other requests." ) 2015 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/request-priority") 2016 protected Enumeration<TaskPriority> priority; 2017 2018 /** 2019 * A name or code (or both) briefly describing what the task involves. 2020 */ 2021 @Child(name = "code", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=true) 2022 @Description(shortDefinition="Task Type", formalDefinition="A name or code (or both) briefly describing what the task involves." ) 2023 protected CodeableConcept code; 2024 2025 /** 2026 * A free-text description of what is to be performed. 2027 */ 2028 @Child(name = "description", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 2029 @Description(shortDefinition="Human-readable explanation of task", formalDefinition="A free-text description of what is to be performed." ) 2030 protected StringType description; 2031 2032 /** 2033 * The request being actioned or the resource being manipulated by this task. 2034 */ 2035 @Child(name = "focus", type = {Reference.class}, order=12, min=0, max=1, modifier=false, summary=true) 2036 @Description(shortDefinition="What task is acting on", formalDefinition="The request being actioned or the resource being manipulated by this task." ) 2037 protected Reference focus; 2038 2039 /** 2040 * The actual object that is the target of the reference (The request being actioned or the resource being manipulated by this task.) 2041 */ 2042 protected Resource focusTarget; 2043 2044 /** 2045 * The entity who benefits from the performance of the service specified in the task (e.g., the patient). 2046 */ 2047 @Child(name = "for", type = {Reference.class}, order=13, min=0, max=1, modifier=false, summary=true) 2048 @Description(shortDefinition="Beneficiary of the Task", formalDefinition="The entity who benefits from the performance of the service specified in the task (e.g., the patient)." ) 2049 protected Reference for_; 2050 2051 /** 2052 * The actual object that is the target of the reference (The entity who benefits from the performance of the service specified in the task (e.g., the patient).) 2053 */ 2054 protected Resource for_Target; 2055 2056 /** 2057 * The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created. 2058 */ 2059 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=14, min=0, max=1, modifier=false, summary=true) 2060 @Description(shortDefinition="Healthcare event during which this task originated", formalDefinition="The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created." ) 2061 protected Reference context; 2062 2063 /** 2064 * The actual object that is the target of the reference (The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.) 2065 */ 2066 protected Resource contextTarget; 2067 2068 /** 2069 * Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end). 2070 */ 2071 @Child(name = "executionPeriod", type = {Period.class}, order=15, min=0, max=1, modifier=false, summary=true) 2072 @Description(shortDefinition="Start and end time of execution", formalDefinition="Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end)." ) 2073 protected Period executionPeriod; 2074 2075 /** 2076 * The date and time this task was created. 2077 */ 2078 @Child(name = "authoredOn", type = {DateTimeType.class}, order=16, min=0, max=1, modifier=false, summary=false) 2079 @Description(shortDefinition="Task Creation Date", formalDefinition="The date and time this task was created." ) 2080 protected DateTimeType authoredOn; 2081 2082 /** 2083 * The date and time of last modification to this task. 2084 */ 2085 @Child(name = "lastModified", type = {DateTimeType.class}, order=17, min=0, max=1, modifier=false, summary=true) 2086 @Description(shortDefinition="Task Last Modified Date", formalDefinition="The date and time of last modification to this task." ) 2087 protected DateTimeType lastModified; 2088 2089 /** 2090 * The creator of the task. 2091 */ 2092 @Child(name = "requester", type = {}, order=18, min=0, max=1, modifier=false, summary=true) 2093 @Description(shortDefinition="Who is asking for task to be done", formalDefinition="The creator of the task." ) 2094 protected TaskRequesterComponent requester; 2095 2096 /** 2097 * The type of participant that can execute the task. 2098 */ 2099 @Child(name = "performerType", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2100 @Description(shortDefinition="requester | dispatcher | scheduler | performer | monitor | manager | acquirer | reviewer", formalDefinition="The type of participant that can execute the task." ) 2101 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/task-performer-type") 2102 protected List<CodeableConcept> performerType; 2103 2104 /** 2105 * Individual organization or Device currently responsible for task execution. 2106 */ 2107 @Child(name = "owner", type = {Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class}, order=20, min=0, max=1, modifier=false, summary=true) 2108 @Description(shortDefinition="Responsible individual", formalDefinition="Individual organization or Device currently responsible for task execution." ) 2109 protected Reference owner; 2110 2111 /** 2112 * The actual object that is the target of the reference (Individual organization or Device currently responsible for task execution.) 2113 */ 2114 protected Resource ownerTarget; 2115 2116 /** 2117 * A description or code indicating why this task needs to be performed. 2118 */ 2119 @Child(name = "reason", type = {CodeableConcept.class}, order=21, min=0, max=1, modifier=false, summary=false) 2120 @Description(shortDefinition="Why task is needed", formalDefinition="A description or code indicating why this task needs to be performed." ) 2121 protected CodeableConcept reason; 2122 2123 /** 2124 * Free-text information captured about the task as it progresses. 2125 */ 2126 @Child(name = "note", type = {Annotation.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2127 @Description(shortDefinition="Comments made about the task", formalDefinition="Free-text information captured about the task as it progresses." ) 2128 protected List<Annotation> note; 2129 2130 /** 2131 * Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task. 2132 */ 2133 @Child(name = "relevantHistory", type = {Provenance.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2134 @Description(shortDefinition="Key events in history of the Task", formalDefinition="Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task." ) 2135 protected List<Reference> relevantHistory; 2136 /** 2137 * The actual objects that are the target of the reference (Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.) 2138 */ 2139 protected List<Provenance> relevantHistoryTarget; 2140 2141 2142 /** 2143 * If the Task.focus is a request resource and the task is seeking fulfillment (i.e is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned. 2144 */ 2145 @Child(name = "restriction", type = {}, order=24, min=0, max=1, modifier=false, summary=false) 2146 @Description(shortDefinition="Constraints on fulfillment tasks", formalDefinition="If the Task.focus is a request resource and the task is seeking fulfillment (i.e is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned." ) 2147 protected TaskRestrictionComponent restriction; 2148 2149 /** 2150 * Additional information that may be needed in the execution of the task. 2151 */ 2152 @Child(name = "input", type = {}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2153 @Description(shortDefinition="Information used to perform task", formalDefinition="Additional information that may be needed in the execution of the task." ) 2154 protected List<ParameterComponent> input; 2155 2156 /** 2157 * Outputs produced by the Task. 2158 */ 2159 @Child(name = "output", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2160 @Description(shortDefinition="Information produced as part of task", formalDefinition="Outputs produced by the Task." ) 2161 protected List<TaskOutputComponent> output; 2162 2163 private static final long serialVersionUID = 2060755798L; 2164 2165 /** 2166 * Constructor 2167 */ 2168 public Task() { 2169 super(); 2170 } 2171 2172 /** 2173 * Constructor 2174 */ 2175 public Task(Enumeration<TaskStatus> status, Enumeration<TaskIntent> intent) { 2176 super(); 2177 this.status = status; 2178 this.intent = intent; 2179 } 2180 2181 /** 2182 * @return {@link #identifier} (The business identifier for this task.) 2183 */ 2184 public List<Identifier> getIdentifier() { 2185 if (this.identifier == null) 2186 this.identifier = new ArrayList<Identifier>(); 2187 return this.identifier; 2188 } 2189 2190 /** 2191 * @return Returns a reference to <code>this</code> for easy method chaining 2192 */ 2193 public Task setIdentifier(List<Identifier> theIdentifier) { 2194 this.identifier = theIdentifier; 2195 return this; 2196 } 2197 2198 public boolean hasIdentifier() { 2199 if (this.identifier == null) 2200 return false; 2201 for (Identifier item : this.identifier) 2202 if (!item.isEmpty()) 2203 return true; 2204 return false; 2205 } 2206 2207 public Identifier addIdentifier() { //3 2208 Identifier t = new Identifier(); 2209 if (this.identifier == null) 2210 this.identifier = new ArrayList<Identifier>(); 2211 this.identifier.add(t); 2212 return t; 2213 } 2214 2215 public Task addIdentifier(Identifier t) { //3 2216 if (t == null) 2217 return this; 2218 if (this.identifier == null) 2219 this.identifier = new ArrayList<Identifier>(); 2220 this.identifier.add(t); 2221 return this; 2222 } 2223 2224 /** 2225 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 2226 */ 2227 public Identifier getIdentifierFirstRep() { 2228 if (getIdentifier().isEmpty()) { 2229 addIdentifier(); 2230 } 2231 return getIdentifier().get(0); 2232 } 2233 2234 /** 2235 * @return {@link #definition} (A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc.) 2236 */ 2237 public Type getDefinition() { 2238 return this.definition; 2239 } 2240 2241 /** 2242 * @return {@link #definition} (A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc.) 2243 */ 2244 public UriType getDefinitionUriType() throws FHIRException { 2245 if (this.definition == null) 2246 return null; 2247 if (!(this.definition instanceof UriType)) 2248 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.definition.getClass().getName()+" was encountered"); 2249 return (UriType) this.definition; 2250 } 2251 2252 public boolean hasDefinitionUriType() { 2253 return this != null && this.definition instanceof UriType; 2254 } 2255 2256 /** 2257 * @return {@link #definition} (A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc.) 2258 */ 2259 public Reference getDefinitionReference() throws FHIRException { 2260 if (this.definition == null) 2261 return null; 2262 if (!(this.definition instanceof Reference)) 2263 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.definition.getClass().getName()+" was encountered"); 2264 return (Reference) this.definition; 2265 } 2266 2267 public boolean hasDefinitionReference() { 2268 return this != null && this.definition instanceof Reference; 2269 } 2270 2271 public boolean hasDefinition() { 2272 return this.definition != null && !this.definition.isEmpty(); 2273 } 2274 2275 /** 2276 * @param value {@link #definition} (A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc.) 2277 */ 2278 public Task setDefinition(Type value) throws FHIRFormatError { 2279 if (value != null && !(value instanceof UriType || value instanceof Reference)) 2280 throw new FHIRFormatError("Not the right type for Task.definition[x]: "+value.fhirType()); 2281 this.definition = value; 2282 return this; 2283 } 2284 2285 /** 2286 * @return {@link #basedOn} (BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a "request" resource such as a ProcedureRequest, MedicationRequest, ProcedureRequest, CarePlan, etc. which is distinct from the "request" resource the task is seeking to fulfil. This latter resource is referenced by FocusOn. For example, based on a ProcedureRequest (= BasedOn), a task is created to fulfil a procedureRequest ( = FocusOn ) to collect a specimen from a patient.) 2287 */ 2288 public List<Reference> getBasedOn() { 2289 if (this.basedOn == null) 2290 this.basedOn = new ArrayList<Reference>(); 2291 return this.basedOn; 2292 } 2293 2294 /** 2295 * @return Returns a reference to <code>this</code> for easy method chaining 2296 */ 2297 public Task setBasedOn(List<Reference> theBasedOn) { 2298 this.basedOn = theBasedOn; 2299 return this; 2300 } 2301 2302 public boolean hasBasedOn() { 2303 if (this.basedOn == null) 2304 return false; 2305 for (Reference item : this.basedOn) 2306 if (!item.isEmpty()) 2307 return true; 2308 return false; 2309 } 2310 2311 public Reference addBasedOn() { //3 2312 Reference t = new Reference(); 2313 if (this.basedOn == null) 2314 this.basedOn = new ArrayList<Reference>(); 2315 this.basedOn.add(t); 2316 return t; 2317 } 2318 2319 public Task addBasedOn(Reference t) { //3 2320 if (t == null) 2321 return this; 2322 if (this.basedOn == null) 2323 this.basedOn = new ArrayList<Reference>(); 2324 this.basedOn.add(t); 2325 return this; 2326 } 2327 2328 /** 2329 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 2330 */ 2331 public Reference getBasedOnFirstRep() { 2332 if (getBasedOn().isEmpty()) { 2333 addBasedOn(); 2334 } 2335 return getBasedOn().get(0); 2336 } 2337 2338 /** 2339 * @deprecated Use Reference#setResource(IBaseResource) instead 2340 */ 2341 @Deprecated 2342 public List<Resource> getBasedOnTarget() { 2343 if (this.basedOnTarget == null) 2344 this.basedOnTarget = new ArrayList<Resource>(); 2345 return this.basedOnTarget; 2346 } 2347 2348 /** 2349 * @return {@link #groupIdentifier} (An identifier that links together multiple tasks and other requests that were created in the same context.) 2350 */ 2351 public Identifier getGroupIdentifier() { 2352 if (this.groupIdentifier == null) 2353 if (Configuration.errorOnAutoCreate()) 2354 throw new Error("Attempt to auto-create Task.groupIdentifier"); 2355 else if (Configuration.doAutoCreate()) 2356 this.groupIdentifier = new Identifier(); // cc 2357 return this.groupIdentifier; 2358 } 2359 2360 public boolean hasGroupIdentifier() { 2361 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 2362 } 2363 2364 /** 2365 * @param value {@link #groupIdentifier} (An identifier that links together multiple tasks and other requests that were created in the same context.) 2366 */ 2367 public Task setGroupIdentifier(Identifier value) { 2368 this.groupIdentifier = value; 2369 return this; 2370 } 2371 2372 /** 2373 * @return {@link #partOf} (Task that this particular task is part of.) 2374 */ 2375 public List<Reference> getPartOf() { 2376 if (this.partOf == null) 2377 this.partOf = new ArrayList<Reference>(); 2378 return this.partOf; 2379 } 2380 2381 /** 2382 * @return Returns a reference to <code>this</code> for easy method chaining 2383 */ 2384 public Task setPartOf(List<Reference> thePartOf) { 2385 this.partOf = thePartOf; 2386 return this; 2387 } 2388 2389 public boolean hasPartOf() { 2390 if (this.partOf == null) 2391 return false; 2392 for (Reference item : this.partOf) 2393 if (!item.isEmpty()) 2394 return true; 2395 return false; 2396 } 2397 2398 public Reference addPartOf() { //3 2399 Reference t = new Reference(); 2400 if (this.partOf == null) 2401 this.partOf = new ArrayList<Reference>(); 2402 this.partOf.add(t); 2403 return t; 2404 } 2405 2406 public Task addPartOf(Reference t) { //3 2407 if (t == null) 2408 return this; 2409 if (this.partOf == null) 2410 this.partOf = new ArrayList<Reference>(); 2411 this.partOf.add(t); 2412 return this; 2413 } 2414 2415 /** 2416 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 2417 */ 2418 public Reference getPartOfFirstRep() { 2419 if (getPartOf().isEmpty()) { 2420 addPartOf(); 2421 } 2422 return getPartOf().get(0); 2423 } 2424 2425 /** 2426 * @deprecated Use Reference#setResource(IBaseResource) instead 2427 */ 2428 @Deprecated 2429 public List<Task> getPartOfTarget() { 2430 if (this.partOfTarget == null) 2431 this.partOfTarget = new ArrayList<Task>(); 2432 return this.partOfTarget; 2433 } 2434 2435 /** 2436 * @deprecated Use Reference#setResource(IBaseResource) instead 2437 */ 2438 @Deprecated 2439 public Task addPartOfTarget() { 2440 Task r = new Task(); 2441 if (this.partOfTarget == null) 2442 this.partOfTarget = new ArrayList<Task>(); 2443 this.partOfTarget.add(r); 2444 return r; 2445 } 2446 2447 /** 2448 * @return {@link #status} (The current status of the task.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2449 */ 2450 public Enumeration<TaskStatus> getStatusElement() { 2451 if (this.status == null) 2452 if (Configuration.errorOnAutoCreate()) 2453 throw new Error("Attempt to auto-create Task.status"); 2454 else if (Configuration.doAutoCreate()) 2455 this.status = new Enumeration<TaskStatus>(new TaskStatusEnumFactory()); // bb 2456 return this.status; 2457 } 2458 2459 public boolean hasStatusElement() { 2460 return this.status != null && !this.status.isEmpty(); 2461 } 2462 2463 public boolean hasStatus() { 2464 return this.status != null && !this.status.isEmpty(); 2465 } 2466 2467 /** 2468 * @param value {@link #status} (The current status of the task.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2469 */ 2470 public Task setStatusElement(Enumeration<TaskStatus> value) { 2471 this.status = value; 2472 return this; 2473 } 2474 2475 /** 2476 * @return The current status of the task. 2477 */ 2478 public TaskStatus getStatus() { 2479 return this.status == null ? null : this.status.getValue(); 2480 } 2481 2482 /** 2483 * @param value The current status of the task. 2484 */ 2485 public Task setStatus(TaskStatus value) { 2486 if (this.status == null) 2487 this.status = new Enumeration<TaskStatus>(new TaskStatusEnumFactory()); 2488 this.status.setValue(value); 2489 return this; 2490 } 2491 2492 /** 2493 * @return {@link #statusReason} (An explanation as to why this task is held, failed, was refused, etc.) 2494 */ 2495 public CodeableConcept getStatusReason() { 2496 if (this.statusReason == null) 2497 if (Configuration.errorOnAutoCreate()) 2498 throw new Error("Attempt to auto-create Task.statusReason"); 2499 else if (Configuration.doAutoCreate()) 2500 this.statusReason = new CodeableConcept(); // cc 2501 return this.statusReason; 2502 } 2503 2504 public boolean hasStatusReason() { 2505 return this.statusReason != null && !this.statusReason.isEmpty(); 2506 } 2507 2508 /** 2509 * @param value {@link #statusReason} (An explanation as to why this task is held, failed, was refused, etc.) 2510 */ 2511 public Task setStatusReason(CodeableConcept value) { 2512 this.statusReason = value; 2513 return this; 2514 } 2515 2516 /** 2517 * @return {@link #businessStatus} (Contains business-specific nuances of the business state.) 2518 */ 2519 public CodeableConcept getBusinessStatus() { 2520 if (this.businessStatus == null) 2521 if (Configuration.errorOnAutoCreate()) 2522 throw new Error("Attempt to auto-create Task.businessStatus"); 2523 else if (Configuration.doAutoCreate()) 2524 this.businessStatus = new CodeableConcept(); // cc 2525 return this.businessStatus; 2526 } 2527 2528 public boolean hasBusinessStatus() { 2529 return this.businessStatus != null && !this.businessStatus.isEmpty(); 2530 } 2531 2532 /** 2533 * @param value {@link #businessStatus} (Contains business-specific nuances of the business state.) 2534 */ 2535 public Task setBusinessStatus(CodeableConcept value) { 2536 this.businessStatus = value; 2537 return this; 2538 } 2539 2540 /** 2541 * @return {@link #intent} (Indicates the "level" of actionability associated with the Task. I.e. Is this a proposed task, a planned task, an actionable task, etc.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 2542 */ 2543 public Enumeration<TaskIntent> getIntentElement() { 2544 if (this.intent == null) 2545 if (Configuration.errorOnAutoCreate()) 2546 throw new Error("Attempt to auto-create Task.intent"); 2547 else if (Configuration.doAutoCreate()) 2548 this.intent = new Enumeration<TaskIntent>(new TaskIntentEnumFactory()); // bb 2549 return this.intent; 2550 } 2551 2552 public boolean hasIntentElement() { 2553 return this.intent != null && !this.intent.isEmpty(); 2554 } 2555 2556 public boolean hasIntent() { 2557 return this.intent != null && !this.intent.isEmpty(); 2558 } 2559 2560 /** 2561 * @param value {@link #intent} (Indicates the "level" of actionability associated with the Task. I.e. Is this a proposed task, a planned task, an actionable task, etc.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 2562 */ 2563 public Task setIntentElement(Enumeration<TaskIntent> value) { 2564 this.intent = value; 2565 return this; 2566 } 2567 2568 /** 2569 * @return Indicates the "level" of actionability associated with the Task. I.e. Is this a proposed task, a planned task, an actionable task, etc. 2570 */ 2571 public TaskIntent getIntent() { 2572 return this.intent == null ? null : this.intent.getValue(); 2573 } 2574 2575 /** 2576 * @param value Indicates the "level" of actionability associated with the Task. I.e. Is this a proposed task, a planned task, an actionable task, etc. 2577 */ 2578 public Task setIntent(TaskIntent value) { 2579 if (this.intent == null) 2580 this.intent = new Enumeration<TaskIntent>(new TaskIntentEnumFactory()); 2581 this.intent.setValue(value); 2582 return this; 2583 } 2584 2585 /** 2586 * @return {@link #priority} (Indicates how quickly the Task should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 2587 */ 2588 public Enumeration<TaskPriority> getPriorityElement() { 2589 if (this.priority == null) 2590 if (Configuration.errorOnAutoCreate()) 2591 throw new Error("Attempt to auto-create Task.priority"); 2592 else if (Configuration.doAutoCreate()) 2593 this.priority = new Enumeration<TaskPriority>(new TaskPriorityEnumFactory()); // bb 2594 return this.priority; 2595 } 2596 2597 public boolean hasPriorityElement() { 2598 return this.priority != null && !this.priority.isEmpty(); 2599 } 2600 2601 public boolean hasPriority() { 2602 return this.priority != null && !this.priority.isEmpty(); 2603 } 2604 2605 /** 2606 * @param value {@link #priority} (Indicates how quickly the Task should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 2607 */ 2608 public Task setPriorityElement(Enumeration<TaskPriority> value) { 2609 this.priority = value; 2610 return this; 2611 } 2612 2613 /** 2614 * @return Indicates how quickly the Task should be addressed with respect to other requests. 2615 */ 2616 public TaskPriority getPriority() { 2617 return this.priority == null ? null : this.priority.getValue(); 2618 } 2619 2620 /** 2621 * @param value Indicates how quickly the Task should be addressed with respect to other requests. 2622 */ 2623 public Task setPriority(TaskPriority value) { 2624 if (value == null) 2625 this.priority = null; 2626 else { 2627 if (this.priority == null) 2628 this.priority = new Enumeration<TaskPriority>(new TaskPriorityEnumFactory()); 2629 this.priority.setValue(value); 2630 } 2631 return this; 2632 } 2633 2634 /** 2635 * @return {@link #code} (A name or code (or both) briefly describing what the task involves.) 2636 */ 2637 public CodeableConcept getCode() { 2638 if (this.code == null) 2639 if (Configuration.errorOnAutoCreate()) 2640 throw new Error("Attempt to auto-create Task.code"); 2641 else if (Configuration.doAutoCreate()) 2642 this.code = new CodeableConcept(); // cc 2643 return this.code; 2644 } 2645 2646 public boolean hasCode() { 2647 return this.code != null && !this.code.isEmpty(); 2648 } 2649 2650 /** 2651 * @param value {@link #code} (A name or code (or both) briefly describing what the task involves.) 2652 */ 2653 public Task setCode(CodeableConcept value) { 2654 this.code = value; 2655 return this; 2656 } 2657 2658 /** 2659 * @return {@link #description} (A free-text description of what is to be performed.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2660 */ 2661 public StringType getDescriptionElement() { 2662 if (this.description == null) 2663 if (Configuration.errorOnAutoCreate()) 2664 throw new Error("Attempt to auto-create Task.description"); 2665 else if (Configuration.doAutoCreate()) 2666 this.description = new StringType(); // bb 2667 return this.description; 2668 } 2669 2670 public boolean hasDescriptionElement() { 2671 return this.description != null && !this.description.isEmpty(); 2672 } 2673 2674 public boolean hasDescription() { 2675 return this.description != null && !this.description.isEmpty(); 2676 } 2677 2678 /** 2679 * @param value {@link #description} (A free-text description of what is to be performed.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2680 */ 2681 public Task setDescriptionElement(StringType value) { 2682 this.description = value; 2683 return this; 2684 } 2685 2686 /** 2687 * @return A free-text description of what is to be performed. 2688 */ 2689 public String getDescription() { 2690 return this.description == null ? null : this.description.getValue(); 2691 } 2692 2693 /** 2694 * @param value A free-text description of what is to be performed. 2695 */ 2696 public Task setDescription(String value) { 2697 if (Utilities.noString(value)) 2698 this.description = null; 2699 else { 2700 if (this.description == null) 2701 this.description = new StringType(); 2702 this.description.setValue(value); 2703 } 2704 return this; 2705 } 2706 2707 /** 2708 * @return {@link #focus} (The request being actioned or the resource being manipulated by this task.) 2709 */ 2710 public Reference getFocus() { 2711 if (this.focus == null) 2712 if (Configuration.errorOnAutoCreate()) 2713 throw new Error("Attempt to auto-create Task.focus"); 2714 else if (Configuration.doAutoCreate()) 2715 this.focus = new Reference(); // cc 2716 return this.focus; 2717 } 2718 2719 public boolean hasFocus() { 2720 return this.focus != null && !this.focus.isEmpty(); 2721 } 2722 2723 /** 2724 * @param value {@link #focus} (The request being actioned or the resource being manipulated by this task.) 2725 */ 2726 public Task setFocus(Reference value) { 2727 this.focus = value; 2728 return this; 2729 } 2730 2731 /** 2732 * @return {@link #focus} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The request being actioned or the resource being manipulated by this task.) 2733 */ 2734 public Resource getFocusTarget() { 2735 return this.focusTarget; 2736 } 2737 2738 /** 2739 * @param value {@link #focus} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The request being actioned or the resource being manipulated by this task.) 2740 */ 2741 public Task setFocusTarget(Resource value) { 2742 this.focusTarget = value; 2743 return this; 2744 } 2745 2746 /** 2747 * @return {@link #for_} (The entity who benefits from the performance of the service specified in the task (e.g., the patient).) 2748 */ 2749 public Reference getFor() { 2750 if (this.for_ == null) 2751 if (Configuration.errorOnAutoCreate()) 2752 throw new Error("Attempt to auto-create Task.for_"); 2753 else if (Configuration.doAutoCreate()) 2754 this.for_ = new Reference(); // cc 2755 return this.for_; 2756 } 2757 2758 public boolean hasFor() { 2759 return this.for_ != null && !this.for_.isEmpty(); 2760 } 2761 2762 /** 2763 * @param value {@link #for_} (The entity who benefits from the performance of the service specified in the task (e.g., the patient).) 2764 */ 2765 public Task setFor(Reference value) { 2766 this.for_ = value; 2767 return this; 2768 } 2769 2770 /** 2771 * @return {@link #for_} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The entity who benefits from the performance of the service specified in the task (e.g., the patient).) 2772 */ 2773 public Resource getForTarget() { 2774 return this.for_Target; 2775 } 2776 2777 /** 2778 * @param value {@link #for_} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The entity who benefits from the performance of the service specified in the task (e.g., the patient).) 2779 */ 2780 public Task setForTarget(Resource value) { 2781 this.for_Target = value; 2782 return this; 2783 } 2784 2785 /** 2786 * @return {@link #context} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.) 2787 */ 2788 public Reference getContext() { 2789 if (this.context == null) 2790 if (Configuration.errorOnAutoCreate()) 2791 throw new Error("Attempt to auto-create Task.context"); 2792 else if (Configuration.doAutoCreate()) 2793 this.context = new Reference(); // cc 2794 return this.context; 2795 } 2796 2797 public boolean hasContext() { 2798 return this.context != null && !this.context.isEmpty(); 2799 } 2800 2801 /** 2802 * @param value {@link #context} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.) 2803 */ 2804 public Task setContext(Reference value) { 2805 this.context = value; 2806 return this; 2807 } 2808 2809 /** 2810 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.) 2811 */ 2812 public Resource getContextTarget() { 2813 return this.contextTarget; 2814 } 2815 2816 /** 2817 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.) 2818 */ 2819 public Task setContextTarget(Resource value) { 2820 this.contextTarget = value; 2821 return this; 2822 } 2823 2824 /** 2825 * @return {@link #executionPeriod} (Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).) 2826 */ 2827 public Period getExecutionPeriod() { 2828 if (this.executionPeriod == null) 2829 if (Configuration.errorOnAutoCreate()) 2830 throw new Error("Attempt to auto-create Task.executionPeriod"); 2831 else if (Configuration.doAutoCreate()) 2832 this.executionPeriod = new Period(); // cc 2833 return this.executionPeriod; 2834 } 2835 2836 public boolean hasExecutionPeriod() { 2837 return this.executionPeriod != null && !this.executionPeriod.isEmpty(); 2838 } 2839 2840 /** 2841 * @param value {@link #executionPeriod} (Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).) 2842 */ 2843 public Task setExecutionPeriod(Period value) { 2844 this.executionPeriod = value; 2845 return this; 2846 } 2847 2848 /** 2849 * @return {@link #authoredOn} (The date and time this task was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 2850 */ 2851 public DateTimeType getAuthoredOnElement() { 2852 if (this.authoredOn == null) 2853 if (Configuration.errorOnAutoCreate()) 2854 throw new Error("Attempt to auto-create Task.authoredOn"); 2855 else if (Configuration.doAutoCreate()) 2856 this.authoredOn = new DateTimeType(); // bb 2857 return this.authoredOn; 2858 } 2859 2860 public boolean hasAuthoredOnElement() { 2861 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2862 } 2863 2864 public boolean hasAuthoredOn() { 2865 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2866 } 2867 2868 /** 2869 * @param value {@link #authoredOn} (The date and time this task was created.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 2870 */ 2871 public Task setAuthoredOnElement(DateTimeType value) { 2872 this.authoredOn = value; 2873 return this; 2874 } 2875 2876 /** 2877 * @return The date and time this task was created. 2878 */ 2879 public Date getAuthoredOn() { 2880 return this.authoredOn == null ? null : this.authoredOn.getValue(); 2881 } 2882 2883 /** 2884 * @param value The date and time this task was created. 2885 */ 2886 public Task setAuthoredOn(Date value) { 2887 if (value == null) 2888 this.authoredOn = null; 2889 else { 2890 if (this.authoredOn == null) 2891 this.authoredOn = new DateTimeType(); 2892 this.authoredOn.setValue(value); 2893 } 2894 return this; 2895 } 2896 2897 /** 2898 * @return {@link #lastModified} (The date and time of last modification to this task.). This is the underlying object with id, value and extensions. The accessor "getLastModified" gives direct access to the value 2899 */ 2900 public DateTimeType getLastModifiedElement() { 2901 if (this.lastModified == null) 2902 if (Configuration.errorOnAutoCreate()) 2903 throw new Error("Attempt to auto-create Task.lastModified"); 2904 else if (Configuration.doAutoCreate()) 2905 this.lastModified = new DateTimeType(); // bb 2906 return this.lastModified; 2907 } 2908 2909 public boolean hasLastModifiedElement() { 2910 return this.lastModified != null && !this.lastModified.isEmpty(); 2911 } 2912 2913 public boolean hasLastModified() { 2914 return this.lastModified != null && !this.lastModified.isEmpty(); 2915 } 2916 2917 /** 2918 * @param value {@link #lastModified} (The date and time of last modification to this task.). This is the underlying object with id, value and extensions. The accessor "getLastModified" gives direct access to the value 2919 */ 2920 public Task setLastModifiedElement(DateTimeType value) { 2921 this.lastModified = value; 2922 return this; 2923 } 2924 2925 /** 2926 * @return The date and time of last modification to this task. 2927 */ 2928 public Date getLastModified() { 2929 return this.lastModified == null ? null : this.lastModified.getValue(); 2930 } 2931 2932 /** 2933 * @param value The date and time of last modification to this task. 2934 */ 2935 public Task setLastModified(Date value) { 2936 if (value == null) 2937 this.lastModified = null; 2938 else { 2939 if (this.lastModified == null) 2940 this.lastModified = new DateTimeType(); 2941 this.lastModified.setValue(value); 2942 } 2943 return this; 2944 } 2945 2946 /** 2947 * @return {@link #requester} (The creator of the task.) 2948 */ 2949 public TaskRequesterComponent getRequester() { 2950 if (this.requester == null) 2951 if (Configuration.errorOnAutoCreate()) 2952 throw new Error("Attempt to auto-create Task.requester"); 2953 else if (Configuration.doAutoCreate()) 2954 this.requester = new TaskRequesterComponent(); // cc 2955 return this.requester; 2956 } 2957 2958 public boolean hasRequester() { 2959 return this.requester != null && !this.requester.isEmpty(); 2960 } 2961 2962 /** 2963 * @param value {@link #requester} (The creator of the task.) 2964 */ 2965 public Task setRequester(TaskRequesterComponent value) { 2966 this.requester = value; 2967 return this; 2968 } 2969 2970 /** 2971 * @return {@link #performerType} (The type of participant that can execute the task.) 2972 */ 2973 public List<CodeableConcept> getPerformerType() { 2974 if (this.performerType == null) 2975 this.performerType = new ArrayList<CodeableConcept>(); 2976 return this.performerType; 2977 } 2978 2979 /** 2980 * @return Returns a reference to <code>this</code> for easy method chaining 2981 */ 2982 public Task setPerformerType(List<CodeableConcept> thePerformerType) { 2983 this.performerType = thePerformerType; 2984 return this; 2985 } 2986 2987 public boolean hasPerformerType() { 2988 if (this.performerType == null) 2989 return false; 2990 for (CodeableConcept item : this.performerType) 2991 if (!item.isEmpty()) 2992 return true; 2993 return false; 2994 } 2995 2996 public CodeableConcept addPerformerType() { //3 2997 CodeableConcept t = new CodeableConcept(); 2998 if (this.performerType == null) 2999 this.performerType = new ArrayList<CodeableConcept>(); 3000 this.performerType.add(t); 3001 return t; 3002 } 3003 3004 public Task addPerformerType(CodeableConcept t) { //3 3005 if (t == null) 3006 return this; 3007 if (this.performerType == null) 3008 this.performerType = new ArrayList<CodeableConcept>(); 3009 this.performerType.add(t); 3010 return this; 3011 } 3012 3013 /** 3014 * @return The first repetition of repeating field {@link #performerType}, creating it if it does not already exist 3015 */ 3016 public CodeableConcept getPerformerTypeFirstRep() { 3017 if (getPerformerType().isEmpty()) { 3018 addPerformerType(); 3019 } 3020 return getPerformerType().get(0); 3021 } 3022 3023 /** 3024 * @return {@link #owner} (Individual organization or Device currently responsible for task execution.) 3025 */ 3026 public Reference getOwner() { 3027 if (this.owner == null) 3028 if (Configuration.errorOnAutoCreate()) 3029 throw new Error("Attempt to auto-create Task.owner"); 3030 else if (Configuration.doAutoCreate()) 3031 this.owner = new Reference(); // cc 3032 return this.owner; 3033 } 3034 3035 public boolean hasOwner() { 3036 return this.owner != null && !this.owner.isEmpty(); 3037 } 3038 3039 /** 3040 * @param value {@link #owner} (Individual organization or Device currently responsible for task execution.) 3041 */ 3042 public Task setOwner(Reference value) { 3043 this.owner = value; 3044 return this; 3045 } 3046 3047 /** 3048 * @return {@link #owner} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Individual organization or Device currently responsible for task execution.) 3049 */ 3050 public Resource getOwnerTarget() { 3051 return this.ownerTarget; 3052 } 3053 3054 /** 3055 * @param value {@link #owner} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Individual organization or Device currently responsible for task execution.) 3056 */ 3057 public Task setOwnerTarget(Resource value) { 3058 this.ownerTarget = value; 3059 return this; 3060 } 3061 3062 /** 3063 * @return {@link #reason} (A description or code indicating why this task needs to be performed.) 3064 */ 3065 public CodeableConcept getReason() { 3066 if (this.reason == null) 3067 if (Configuration.errorOnAutoCreate()) 3068 throw new Error("Attempt to auto-create Task.reason"); 3069 else if (Configuration.doAutoCreate()) 3070 this.reason = new CodeableConcept(); // cc 3071 return this.reason; 3072 } 3073 3074 public boolean hasReason() { 3075 return this.reason != null && !this.reason.isEmpty(); 3076 } 3077 3078 /** 3079 * @param value {@link #reason} (A description or code indicating why this task needs to be performed.) 3080 */ 3081 public Task setReason(CodeableConcept value) { 3082 this.reason = value; 3083 return this; 3084 } 3085 3086 /** 3087 * @return {@link #note} (Free-text information captured about the task as it progresses.) 3088 */ 3089 public List<Annotation> getNote() { 3090 if (this.note == null) 3091 this.note = new ArrayList<Annotation>(); 3092 return this.note; 3093 } 3094 3095 /** 3096 * @return Returns a reference to <code>this</code> for easy method chaining 3097 */ 3098 public Task setNote(List<Annotation> theNote) { 3099 this.note = theNote; 3100 return this; 3101 } 3102 3103 public boolean hasNote() { 3104 if (this.note == null) 3105 return false; 3106 for (Annotation item : this.note) 3107 if (!item.isEmpty()) 3108 return true; 3109 return false; 3110 } 3111 3112 public Annotation addNote() { //3 3113 Annotation t = new Annotation(); 3114 if (this.note == null) 3115 this.note = new ArrayList<Annotation>(); 3116 this.note.add(t); 3117 return t; 3118 } 3119 3120 public Task addNote(Annotation t) { //3 3121 if (t == null) 3122 return this; 3123 if (this.note == null) 3124 this.note = new ArrayList<Annotation>(); 3125 this.note.add(t); 3126 return this; 3127 } 3128 3129 /** 3130 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 3131 */ 3132 public Annotation getNoteFirstRep() { 3133 if (getNote().isEmpty()) { 3134 addNote(); 3135 } 3136 return getNote().get(0); 3137 } 3138 3139 /** 3140 * @return {@link #relevantHistory} (Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.) 3141 */ 3142 public List<Reference> getRelevantHistory() { 3143 if (this.relevantHistory == null) 3144 this.relevantHistory = new ArrayList<Reference>(); 3145 return this.relevantHistory; 3146 } 3147 3148 /** 3149 * @return Returns a reference to <code>this</code> for easy method chaining 3150 */ 3151 public Task setRelevantHistory(List<Reference> theRelevantHistory) { 3152 this.relevantHistory = theRelevantHistory; 3153 return this; 3154 } 3155 3156 public boolean hasRelevantHistory() { 3157 if (this.relevantHistory == null) 3158 return false; 3159 for (Reference item : this.relevantHistory) 3160 if (!item.isEmpty()) 3161 return true; 3162 return false; 3163 } 3164 3165 public Reference addRelevantHistory() { //3 3166 Reference t = new Reference(); 3167 if (this.relevantHistory == null) 3168 this.relevantHistory = new ArrayList<Reference>(); 3169 this.relevantHistory.add(t); 3170 return t; 3171 } 3172 3173 public Task addRelevantHistory(Reference t) { //3 3174 if (t == null) 3175 return this; 3176 if (this.relevantHistory == null) 3177 this.relevantHistory = new ArrayList<Reference>(); 3178 this.relevantHistory.add(t); 3179 return this; 3180 } 3181 3182 /** 3183 * @return The first repetition of repeating field {@link #relevantHistory}, creating it if it does not already exist 3184 */ 3185 public Reference getRelevantHistoryFirstRep() { 3186 if (getRelevantHistory().isEmpty()) { 3187 addRelevantHistory(); 3188 } 3189 return getRelevantHistory().get(0); 3190 } 3191 3192 /** 3193 * @deprecated Use Reference#setResource(IBaseResource) instead 3194 */ 3195 @Deprecated 3196 public List<Provenance> getRelevantHistoryTarget() { 3197 if (this.relevantHistoryTarget == null) 3198 this.relevantHistoryTarget = new ArrayList<Provenance>(); 3199 return this.relevantHistoryTarget; 3200 } 3201 3202 /** 3203 * @deprecated Use Reference#setResource(IBaseResource) instead 3204 */ 3205 @Deprecated 3206 public Provenance addRelevantHistoryTarget() { 3207 Provenance r = new Provenance(); 3208 if (this.relevantHistoryTarget == null) 3209 this.relevantHistoryTarget = new ArrayList<Provenance>(); 3210 this.relevantHistoryTarget.add(r); 3211 return r; 3212 } 3213 3214 /** 3215 * @return {@link #restriction} (If the Task.focus is a request resource and the task is seeking fulfillment (i.e is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.) 3216 */ 3217 public TaskRestrictionComponent getRestriction() { 3218 if (this.restriction == null) 3219 if (Configuration.errorOnAutoCreate()) 3220 throw new Error("Attempt to auto-create Task.restriction"); 3221 else if (Configuration.doAutoCreate()) 3222 this.restriction = new TaskRestrictionComponent(); // cc 3223 return this.restriction; 3224 } 3225 3226 public boolean hasRestriction() { 3227 return this.restriction != null && !this.restriction.isEmpty(); 3228 } 3229 3230 /** 3231 * @param value {@link #restriction} (If the Task.focus is a request resource and the task is seeking fulfillment (i.e is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.) 3232 */ 3233 public Task setRestriction(TaskRestrictionComponent value) { 3234 this.restriction = value; 3235 return this; 3236 } 3237 3238 /** 3239 * @return {@link #input} (Additional information that may be needed in the execution of the task.) 3240 */ 3241 public List<ParameterComponent> getInput() { 3242 if (this.input == null) 3243 this.input = new ArrayList<ParameterComponent>(); 3244 return this.input; 3245 } 3246 3247 /** 3248 * @return Returns a reference to <code>this</code> for easy method chaining 3249 */ 3250 public Task setInput(List<ParameterComponent> theInput) { 3251 this.input = theInput; 3252 return this; 3253 } 3254 3255 public boolean hasInput() { 3256 if (this.input == null) 3257 return false; 3258 for (ParameterComponent item : this.input) 3259 if (!item.isEmpty()) 3260 return true; 3261 return false; 3262 } 3263 3264 public ParameterComponent addInput() { //3 3265 ParameterComponent t = new ParameterComponent(); 3266 if (this.input == null) 3267 this.input = new ArrayList<ParameterComponent>(); 3268 this.input.add(t); 3269 return t; 3270 } 3271 3272 public Task addInput(ParameterComponent t) { //3 3273 if (t == null) 3274 return this; 3275 if (this.input == null) 3276 this.input = new ArrayList<ParameterComponent>(); 3277 this.input.add(t); 3278 return this; 3279 } 3280 3281 /** 3282 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist 3283 */ 3284 public ParameterComponent getInputFirstRep() { 3285 if (getInput().isEmpty()) { 3286 addInput(); 3287 } 3288 return getInput().get(0); 3289 } 3290 3291 /** 3292 * @return {@link #output} (Outputs produced by the Task.) 3293 */ 3294 public List<TaskOutputComponent> getOutput() { 3295 if (this.output == null) 3296 this.output = new ArrayList<TaskOutputComponent>(); 3297 return this.output; 3298 } 3299 3300 /** 3301 * @return Returns a reference to <code>this</code> for easy method chaining 3302 */ 3303 public Task setOutput(List<TaskOutputComponent> theOutput) { 3304 this.output = theOutput; 3305 return this; 3306 } 3307 3308 public boolean hasOutput() { 3309 if (this.output == null) 3310 return false; 3311 for (TaskOutputComponent item : this.output) 3312 if (!item.isEmpty()) 3313 return true; 3314 return false; 3315 } 3316 3317 public TaskOutputComponent addOutput() { //3 3318 TaskOutputComponent t = new TaskOutputComponent(); 3319 if (this.output == null) 3320 this.output = new ArrayList<TaskOutputComponent>(); 3321 this.output.add(t); 3322 return t; 3323 } 3324 3325 public Task addOutput(TaskOutputComponent t) { //3 3326 if (t == null) 3327 return this; 3328 if (this.output == null) 3329 this.output = new ArrayList<TaskOutputComponent>(); 3330 this.output.add(t); 3331 return this; 3332 } 3333 3334 /** 3335 * @return The first repetition of repeating field {@link #output}, creating it if it does not already exist 3336 */ 3337 public TaskOutputComponent getOutputFirstRep() { 3338 if (getOutput().isEmpty()) { 3339 addOutput(); 3340 } 3341 return getOutput().get(0); 3342 } 3343 3344 protected void listChildren(List<Property> children) { 3345 super.listChildren(children); 3346 children.add(new Property("identifier", "Identifier", "The business identifier for this task.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3347 children.add(new Property("definition[x]", "uri|Reference(ActivityDefinition)", "A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc.", 0, 1, definition)); 3348 children.add(new Property("basedOn", "Reference(Any)", "BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ProcedureRequest, MedicationRequest, ProcedureRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfil. This latter resource is referenced by FocusOn. For example, based on a ProcedureRequest (= BasedOn), a task is created to fulfil a procedureRequest ( = FocusOn ) to collect a specimen from a patient.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 3349 children.add(new Property("groupIdentifier", "Identifier", "An identifier that links together multiple tasks and other requests that were created in the same context.", 0, 1, groupIdentifier)); 3350 children.add(new Property("partOf", "Reference(Task)", "Task that this particular task is part of.", 0, java.lang.Integer.MAX_VALUE, partOf)); 3351 children.add(new Property("status", "code", "The current status of the task.", 0, 1, status)); 3352 children.add(new Property("statusReason", "CodeableConcept", "An explanation as to why this task is held, failed, was refused, etc.", 0, 1, statusReason)); 3353 children.add(new Property("businessStatus", "CodeableConcept", "Contains business-specific nuances of the business state.", 0, 1, businessStatus)); 3354 children.add(new Property("intent", "code", "Indicates the \"level\" of actionability associated with the Task. I.e. Is this a proposed task, a planned task, an actionable task, etc.", 0, 1, intent)); 3355 children.add(new Property("priority", "code", "Indicates how quickly the Task should be addressed with respect to other requests.", 0, 1, priority)); 3356 children.add(new Property("code", "CodeableConcept", "A name or code (or both) briefly describing what the task involves.", 0, 1, code)); 3357 children.add(new Property("description", "string", "A free-text description of what is to be performed.", 0, 1, description)); 3358 children.add(new Property("focus", "Reference(Any)", "The request being actioned or the resource being manipulated by this task.", 0, 1, focus)); 3359 children.add(new Property("for", "Reference(Any)", "The entity who benefits from the performance of the service specified in the task (e.g., the patient).", 0, 1, for_)); 3360 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.", 0, 1, context)); 3361 children.add(new Property("executionPeriod", "Period", "Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).", 0, 1, executionPeriod)); 3362 children.add(new Property("authoredOn", "dateTime", "The date and time this task was created.", 0, 1, authoredOn)); 3363 children.add(new Property("lastModified", "dateTime", "The date and time of last modification to this task.", 0, 1, lastModified)); 3364 children.add(new Property("requester", "", "The creator of the task.", 0, 1, requester)); 3365 children.add(new Property("performerType", "CodeableConcept", "The type of participant that can execute the task.", 0, java.lang.Integer.MAX_VALUE, performerType)); 3366 children.add(new Property("owner", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", "Individual organization or Device currently responsible for task execution.", 0, 1, owner)); 3367 children.add(new Property("reason", "CodeableConcept", "A description or code indicating why this task needs to be performed.", 0, 1, reason)); 3368 children.add(new Property("note", "Annotation", "Free-text information captured about the task as it progresses.", 0, java.lang.Integer.MAX_VALUE, note)); 3369 children.add(new Property("relevantHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.", 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 3370 children.add(new Property("restriction", "", "If the Task.focus is a request resource and the task is seeking fulfillment (i.e is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.", 0, 1, restriction)); 3371 children.add(new Property("input", "", "Additional information that may be needed in the execution of the task.", 0, java.lang.Integer.MAX_VALUE, input)); 3372 children.add(new Property("output", "", "Outputs produced by the Task.", 0, java.lang.Integer.MAX_VALUE, output)); 3373 } 3374 3375 @Override 3376 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3377 switch (_hash) { 3378 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The business identifier for this task.", 0, java.lang.Integer.MAX_VALUE, identifier); 3379 case -1139422643: /*definition[x]*/ return new Property("definition[x]", "uri|Reference(ActivityDefinition)", "A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc.", 0, 1, definition); 3380 case -1014418093: /*definition*/ return new Property("definition[x]", "uri|Reference(ActivityDefinition)", "A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc.", 0, 1, definition); 3381 case -1139428583: /*definitionUri*/ return new Property("definition[x]", "uri|Reference(ActivityDefinition)", "A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc.", 0, 1, definition); 3382 case -820021448: /*definitionReference*/ return new Property("definition[x]", "uri|Reference(ActivityDefinition)", "A reference to a formal or informal definition of the task. For example, a protocol, a step within a defined workflow definition, etc.", 0, 1, definition); 3383 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(Any)", "BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ProcedureRequest, MedicationRequest, ProcedureRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfil. This latter resource is referenced by FocusOn. For example, based on a ProcedureRequest (= BasedOn), a task is created to fulfil a procedureRequest ( = FocusOn ) to collect a specimen from a patient.", 0, java.lang.Integer.MAX_VALUE, basedOn); 3384 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "An identifier that links together multiple tasks and other requests that were created in the same context.", 0, 1, groupIdentifier); 3385 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Task)", "Task that this particular task is part of.", 0, java.lang.Integer.MAX_VALUE, partOf); 3386 case -892481550: /*status*/ return new Property("status", "code", "The current status of the task.", 0, 1, status); 3387 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "An explanation as to why this task is held, failed, was refused, etc.", 0, 1, statusReason); 3388 case 2008591314: /*businessStatus*/ return new Property("businessStatus", "CodeableConcept", "Contains business-specific nuances of the business state.", 0, 1, businessStatus); 3389 case -1183762788: /*intent*/ return new Property("intent", "code", "Indicates the \"level\" of actionability associated with the Task. I.e. Is this a proposed task, a planned task, an actionable task, etc.", 0, 1, intent); 3390 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the Task should be addressed with respect to other requests.", 0, 1, priority); 3391 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A name or code (or both) briefly describing what the task involves.", 0, 1, code); 3392 case -1724546052: /*description*/ return new Property("description", "string", "A free-text description of what is to be performed.", 0, 1, description); 3393 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The request being actioned or the resource being manipulated by this task.", 0, 1, focus); 3394 case 101577: /*for*/ return new Property("for", "Reference(Any)", "The entity who benefits from the performance of the service specified in the task (e.g., the patient).", 0, 1, for_); 3395 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.", 0, 1, context); 3396 case 1218624249: /*executionPeriod*/ return new Property("executionPeriod", "Period", "Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).", 0, 1, executionPeriod); 3397 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "The date and time this task was created.", 0, 1, authoredOn); 3398 case 1959003007: /*lastModified*/ return new Property("lastModified", "dateTime", "The date and time of last modification to this task.", 0, 1, lastModified); 3399 case 693933948: /*requester*/ return new Property("requester", "", "The creator of the task.", 0, 1, requester); 3400 case -901444568: /*performerType*/ return new Property("performerType", "CodeableConcept", "The type of participant that can execute the task.", 0, java.lang.Integer.MAX_VALUE, performerType); 3401 case 106164915: /*owner*/ return new Property("owner", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", "Individual organization or Device currently responsible for task execution.", 0, 1, owner); 3402 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "A description or code indicating why this task needs to be performed.", 0, 1, reason); 3403 case 3387378: /*note*/ return new Property("note", "Annotation", "Free-text information captured about the task as it progresses.", 0, java.lang.Integer.MAX_VALUE, note); 3404 case 1538891575: /*relevantHistory*/ return new Property("relevantHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 3405 case -1561062452: /*restriction*/ return new Property("restriction", "", "If the Task.focus is a request resource and the task is seeking fulfillment (i.e is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.", 0, 1, restriction); 3406 case 100358090: /*input*/ return new Property("input", "", "Additional information that may be needed in the execution of the task.", 0, java.lang.Integer.MAX_VALUE, input); 3407 case -1005512447: /*output*/ return new Property("output", "", "Outputs produced by the Task.", 0, java.lang.Integer.MAX_VALUE, output); 3408 default: return super.getNamedProperty(_hash, _name, _checkValid); 3409 } 3410 3411 } 3412 3413 @Override 3414 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3415 switch (hash) { 3416 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3417 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // Type 3418 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3419 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 3420 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 3421 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<TaskStatus> 3422 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableConcept 3423 case 2008591314: /*businessStatus*/ return this.businessStatus == null ? new Base[0] : new Base[] {this.businessStatus}; // CodeableConcept 3424 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<TaskIntent> 3425 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<TaskPriority> 3426 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3427 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 3428 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : new Base[] {this.focus}; // Reference 3429 case 101577: /*for*/ return this.for_ == null ? new Base[0] : new Base[] {this.for_}; // Reference 3430 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 3431 case 1218624249: /*executionPeriod*/ return this.executionPeriod == null ? new Base[0] : new Base[] {this.executionPeriod}; // Period 3432 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 3433 case 1959003007: /*lastModified*/ return this.lastModified == null ? new Base[0] : new Base[] {this.lastModified}; // DateTimeType 3434 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // TaskRequesterComponent 3435 case -901444568: /*performerType*/ return this.performerType == null ? new Base[0] : this.performerType.toArray(new Base[this.performerType.size()]); // CodeableConcept 3436 case 106164915: /*owner*/ return this.owner == null ? new Base[0] : new Base[] {this.owner}; // Reference 3437 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 3438 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3439 case 1538891575: /*relevantHistory*/ return this.relevantHistory == null ? new Base[0] : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 3440 case -1561062452: /*restriction*/ return this.restriction == null ? new Base[0] : new Base[] {this.restriction}; // TaskRestrictionComponent 3441 case 100358090: /*input*/ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // ParameterComponent 3442 case -1005512447: /*output*/ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // TaskOutputComponent 3443 default: return super.getProperty(hash, name, checkValid); 3444 } 3445 3446 } 3447 3448 @Override 3449 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3450 switch (hash) { 3451 case -1618432855: // identifier 3452 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3453 return value; 3454 case -1014418093: // definition 3455 this.definition = castToType(value); // Type 3456 return value; 3457 case -332612366: // basedOn 3458 this.getBasedOn().add(castToReference(value)); // Reference 3459 return value; 3460 case -445338488: // groupIdentifier 3461 this.groupIdentifier = castToIdentifier(value); // Identifier 3462 return value; 3463 case -995410646: // partOf 3464 this.getPartOf().add(castToReference(value)); // Reference 3465 return value; 3466 case -892481550: // status 3467 value = new TaskStatusEnumFactory().fromType(castToCode(value)); 3468 this.status = (Enumeration) value; // Enumeration<TaskStatus> 3469 return value; 3470 case 2051346646: // statusReason 3471 this.statusReason = castToCodeableConcept(value); // CodeableConcept 3472 return value; 3473 case 2008591314: // businessStatus 3474 this.businessStatus = castToCodeableConcept(value); // CodeableConcept 3475 return value; 3476 case -1183762788: // intent 3477 value = new TaskIntentEnumFactory().fromType(castToCode(value)); 3478 this.intent = (Enumeration) value; // Enumeration<TaskIntent> 3479 return value; 3480 case -1165461084: // priority 3481 value = new TaskPriorityEnumFactory().fromType(castToCode(value)); 3482 this.priority = (Enumeration) value; // Enumeration<TaskPriority> 3483 return value; 3484 case 3059181: // code 3485 this.code = castToCodeableConcept(value); // CodeableConcept 3486 return value; 3487 case -1724546052: // description 3488 this.description = castToString(value); // StringType 3489 return value; 3490 case 97604824: // focus 3491 this.focus = castToReference(value); // Reference 3492 return value; 3493 case 101577: // for 3494 this.for_ = castToReference(value); // Reference 3495 return value; 3496 case 951530927: // context 3497 this.context = castToReference(value); // Reference 3498 return value; 3499 case 1218624249: // executionPeriod 3500 this.executionPeriod = castToPeriod(value); // Period 3501 return value; 3502 case -1500852503: // authoredOn 3503 this.authoredOn = castToDateTime(value); // DateTimeType 3504 return value; 3505 case 1959003007: // lastModified 3506 this.lastModified = castToDateTime(value); // DateTimeType 3507 return value; 3508 case 693933948: // requester 3509 this.requester = (TaskRequesterComponent) value; // TaskRequesterComponent 3510 return value; 3511 case -901444568: // performerType 3512 this.getPerformerType().add(castToCodeableConcept(value)); // CodeableConcept 3513 return value; 3514 case 106164915: // owner 3515 this.owner = castToReference(value); // Reference 3516 return value; 3517 case -934964668: // reason 3518 this.reason = castToCodeableConcept(value); // CodeableConcept 3519 return value; 3520 case 3387378: // note 3521 this.getNote().add(castToAnnotation(value)); // Annotation 3522 return value; 3523 case 1538891575: // relevantHistory 3524 this.getRelevantHistory().add(castToReference(value)); // Reference 3525 return value; 3526 case -1561062452: // restriction 3527 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 3528 return value; 3529 case 100358090: // input 3530 this.getInput().add((ParameterComponent) value); // ParameterComponent 3531 return value; 3532 case -1005512447: // output 3533 this.getOutput().add((TaskOutputComponent) value); // TaskOutputComponent 3534 return value; 3535 default: return super.setProperty(hash, name, value); 3536 } 3537 3538 } 3539 3540 @Override 3541 public Base setProperty(String name, Base value) throws FHIRException { 3542 if (name.equals("identifier")) { 3543 this.getIdentifier().add(castToIdentifier(value)); 3544 } else if (name.equals("definition[x]")) { 3545 this.definition = castToType(value); // Type 3546 } else if (name.equals("basedOn")) { 3547 this.getBasedOn().add(castToReference(value)); 3548 } else if (name.equals("groupIdentifier")) { 3549 this.groupIdentifier = castToIdentifier(value); // Identifier 3550 } else if (name.equals("partOf")) { 3551 this.getPartOf().add(castToReference(value)); 3552 } else if (name.equals("status")) { 3553 value = new TaskStatusEnumFactory().fromType(castToCode(value)); 3554 this.status = (Enumeration) value; // Enumeration<TaskStatus> 3555 } else if (name.equals("statusReason")) { 3556 this.statusReason = castToCodeableConcept(value); // CodeableConcept 3557 } else if (name.equals("businessStatus")) { 3558 this.businessStatus = castToCodeableConcept(value); // CodeableConcept 3559 } else if (name.equals("intent")) { 3560 value = new TaskIntentEnumFactory().fromType(castToCode(value)); 3561 this.intent = (Enumeration) value; // Enumeration<TaskIntent> 3562 } else if (name.equals("priority")) { 3563 value = new TaskPriorityEnumFactory().fromType(castToCode(value)); 3564 this.priority = (Enumeration) value; // Enumeration<TaskPriority> 3565 } else if (name.equals("code")) { 3566 this.code = castToCodeableConcept(value); // CodeableConcept 3567 } else if (name.equals("description")) { 3568 this.description = castToString(value); // StringType 3569 } else if (name.equals("focus")) { 3570 this.focus = castToReference(value); // Reference 3571 } else if (name.equals("for")) { 3572 this.for_ = castToReference(value); // Reference 3573 } else if (name.equals("context")) { 3574 this.context = castToReference(value); // Reference 3575 } else if (name.equals("executionPeriod")) { 3576 this.executionPeriod = castToPeriod(value); // Period 3577 } else if (name.equals("authoredOn")) { 3578 this.authoredOn = castToDateTime(value); // DateTimeType 3579 } else if (name.equals("lastModified")) { 3580 this.lastModified = castToDateTime(value); // DateTimeType 3581 } else if (name.equals("requester")) { 3582 this.requester = (TaskRequesterComponent) value; // TaskRequesterComponent 3583 } else if (name.equals("performerType")) { 3584 this.getPerformerType().add(castToCodeableConcept(value)); 3585 } else if (name.equals("owner")) { 3586 this.owner = castToReference(value); // Reference 3587 } else if (name.equals("reason")) { 3588 this.reason = castToCodeableConcept(value); // CodeableConcept 3589 } else if (name.equals("note")) { 3590 this.getNote().add(castToAnnotation(value)); 3591 } else if (name.equals("relevantHistory")) { 3592 this.getRelevantHistory().add(castToReference(value)); 3593 } else if (name.equals("restriction")) { 3594 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 3595 } else if (name.equals("input")) { 3596 this.getInput().add((ParameterComponent) value); 3597 } else if (name.equals("output")) { 3598 this.getOutput().add((TaskOutputComponent) value); 3599 } else 3600 return super.setProperty(name, value); 3601 return value; 3602 } 3603 3604 @Override 3605 public Base makeProperty(int hash, String name) throws FHIRException { 3606 switch (hash) { 3607 case -1618432855: return addIdentifier(); 3608 case -1139422643: return getDefinition(); 3609 case -1014418093: return getDefinition(); 3610 case -332612366: return addBasedOn(); 3611 case -445338488: return getGroupIdentifier(); 3612 case -995410646: return addPartOf(); 3613 case -892481550: return getStatusElement(); 3614 case 2051346646: return getStatusReason(); 3615 case 2008591314: return getBusinessStatus(); 3616 case -1183762788: return getIntentElement(); 3617 case -1165461084: return getPriorityElement(); 3618 case 3059181: return getCode(); 3619 case -1724546052: return getDescriptionElement(); 3620 case 97604824: return getFocus(); 3621 case 101577: return getFor(); 3622 case 951530927: return getContext(); 3623 case 1218624249: return getExecutionPeriod(); 3624 case -1500852503: return getAuthoredOnElement(); 3625 case 1959003007: return getLastModifiedElement(); 3626 case 693933948: return getRequester(); 3627 case -901444568: return addPerformerType(); 3628 case 106164915: return getOwner(); 3629 case -934964668: return getReason(); 3630 case 3387378: return addNote(); 3631 case 1538891575: return addRelevantHistory(); 3632 case -1561062452: return getRestriction(); 3633 case 100358090: return addInput(); 3634 case -1005512447: return addOutput(); 3635 default: return super.makeProperty(hash, name); 3636 } 3637 3638 } 3639 3640 @Override 3641 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3642 switch (hash) { 3643 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3644 case -1014418093: /*definition*/ return new String[] {"uri", "Reference"}; 3645 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3646 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 3647 case -995410646: /*partOf*/ return new String[] {"Reference"}; 3648 case -892481550: /*status*/ return new String[] {"code"}; 3649 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 3650 case 2008591314: /*businessStatus*/ return new String[] {"CodeableConcept"}; 3651 case -1183762788: /*intent*/ return new String[] {"code"}; 3652 case -1165461084: /*priority*/ return new String[] {"code"}; 3653 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3654 case -1724546052: /*description*/ return new String[] {"string"}; 3655 case 97604824: /*focus*/ return new String[] {"Reference"}; 3656 case 101577: /*for*/ return new String[] {"Reference"}; 3657 case 951530927: /*context*/ return new String[] {"Reference"}; 3658 case 1218624249: /*executionPeriod*/ return new String[] {"Period"}; 3659 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 3660 case 1959003007: /*lastModified*/ return new String[] {"dateTime"}; 3661 case 693933948: /*requester*/ return new String[] {}; 3662 case -901444568: /*performerType*/ return new String[] {"CodeableConcept"}; 3663 case 106164915: /*owner*/ return new String[] {"Reference"}; 3664 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 3665 case 3387378: /*note*/ return new String[] {"Annotation"}; 3666 case 1538891575: /*relevantHistory*/ return new String[] {"Reference"}; 3667 case -1561062452: /*restriction*/ return new String[] {}; 3668 case 100358090: /*input*/ return new String[] {}; 3669 case -1005512447: /*output*/ return new String[] {}; 3670 default: return super.getTypesForProperty(hash, name); 3671 } 3672 3673 } 3674 3675 @Override 3676 public Base addChild(String name) throws FHIRException { 3677 if (name.equals("identifier")) { 3678 return addIdentifier(); 3679 } 3680 else if (name.equals("definitionUri")) { 3681 this.definition = new UriType(); 3682 return this.definition; 3683 } 3684 else if (name.equals("definitionReference")) { 3685 this.definition = new Reference(); 3686 return this.definition; 3687 } 3688 else if (name.equals("basedOn")) { 3689 return addBasedOn(); 3690 } 3691 else if (name.equals("groupIdentifier")) { 3692 this.groupIdentifier = new Identifier(); 3693 return this.groupIdentifier; 3694 } 3695 else if (name.equals("partOf")) { 3696 return addPartOf(); 3697 } 3698 else if (name.equals("status")) { 3699 throw new FHIRException("Cannot call addChild on a singleton property Task.status"); 3700 } 3701 else if (name.equals("statusReason")) { 3702 this.statusReason = new CodeableConcept(); 3703 return this.statusReason; 3704 } 3705 else if (name.equals("businessStatus")) { 3706 this.businessStatus = new CodeableConcept(); 3707 return this.businessStatus; 3708 } 3709 else if (name.equals("intent")) { 3710 throw new FHIRException("Cannot call addChild on a singleton property Task.intent"); 3711 } 3712 else if (name.equals("priority")) { 3713 throw new FHIRException("Cannot call addChild on a singleton property Task.priority"); 3714 } 3715 else if (name.equals("code")) { 3716 this.code = new CodeableConcept(); 3717 return this.code; 3718 } 3719 else if (name.equals("description")) { 3720 throw new FHIRException("Cannot call addChild on a singleton property Task.description"); 3721 } 3722 else if (name.equals("focus")) { 3723 this.focus = new Reference(); 3724 return this.focus; 3725 } 3726 else if (name.equals("for")) { 3727 this.for_ = new Reference(); 3728 return this.for_; 3729 } 3730 else if (name.equals("context")) { 3731 this.context = new Reference(); 3732 return this.context; 3733 } 3734 else if (name.equals("executionPeriod")) { 3735 this.executionPeriod = new Period(); 3736 return this.executionPeriod; 3737 } 3738 else if (name.equals("authoredOn")) { 3739 throw new FHIRException("Cannot call addChild on a singleton property Task.authoredOn"); 3740 } 3741 else if (name.equals("lastModified")) { 3742 throw new FHIRException("Cannot call addChild on a singleton property Task.lastModified"); 3743 } 3744 else if (name.equals("requester")) { 3745 this.requester = new TaskRequesterComponent(); 3746 return this.requester; 3747 } 3748 else if (name.equals("performerType")) { 3749 return addPerformerType(); 3750 } 3751 else if (name.equals("owner")) { 3752 this.owner = new Reference(); 3753 return this.owner; 3754 } 3755 else if (name.equals("reason")) { 3756 this.reason = new CodeableConcept(); 3757 return this.reason; 3758 } 3759 else if (name.equals("note")) { 3760 return addNote(); 3761 } 3762 else if (name.equals("relevantHistory")) { 3763 return addRelevantHistory(); 3764 } 3765 else if (name.equals("restriction")) { 3766 this.restriction = new TaskRestrictionComponent(); 3767 return this.restriction; 3768 } 3769 else if (name.equals("input")) { 3770 return addInput(); 3771 } 3772 else if (name.equals("output")) { 3773 return addOutput(); 3774 } 3775 else 3776 return super.addChild(name); 3777 } 3778 3779 public String fhirType() { 3780 return "Task"; 3781 3782 } 3783 3784 public Task copy() { 3785 Task dst = new Task(); 3786 copyValues(dst); 3787 if (identifier != null) { 3788 dst.identifier = new ArrayList<Identifier>(); 3789 for (Identifier i : identifier) 3790 dst.identifier.add(i.copy()); 3791 }; 3792 dst.definition = definition == null ? null : definition.copy(); 3793 if (basedOn != null) { 3794 dst.basedOn = new ArrayList<Reference>(); 3795 for (Reference i : basedOn) 3796 dst.basedOn.add(i.copy()); 3797 }; 3798 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 3799 if (partOf != null) { 3800 dst.partOf = new ArrayList<Reference>(); 3801 for (Reference i : partOf) 3802 dst.partOf.add(i.copy()); 3803 }; 3804 dst.status = status == null ? null : status.copy(); 3805 dst.statusReason = statusReason == null ? null : statusReason.copy(); 3806 dst.businessStatus = businessStatus == null ? null : businessStatus.copy(); 3807 dst.intent = intent == null ? null : intent.copy(); 3808 dst.priority = priority == null ? null : priority.copy(); 3809 dst.code = code == null ? null : code.copy(); 3810 dst.description = description == null ? null : description.copy(); 3811 dst.focus = focus == null ? null : focus.copy(); 3812 dst.for_ = for_ == null ? null : for_.copy(); 3813 dst.context = context == null ? null : context.copy(); 3814 dst.executionPeriod = executionPeriod == null ? null : executionPeriod.copy(); 3815 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 3816 dst.lastModified = lastModified == null ? null : lastModified.copy(); 3817 dst.requester = requester == null ? null : requester.copy(); 3818 if (performerType != null) { 3819 dst.performerType = new ArrayList<CodeableConcept>(); 3820 for (CodeableConcept i : performerType) 3821 dst.performerType.add(i.copy()); 3822 }; 3823 dst.owner = owner == null ? null : owner.copy(); 3824 dst.reason = reason == null ? null : reason.copy(); 3825 if (note != null) { 3826 dst.note = new ArrayList<Annotation>(); 3827 for (Annotation i : note) 3828 dst.note.add(i.copy()); 3829 }; 3830 if (relevantHistory != null) { 3831 dst.relevantHistory = new ArrayList<Reference>(); 3832 for (Reference i : relevantHistory) 3833 dst.relevantHistory.add(i.copy()); 3834 }; 3835 dst.restriction = restriction == null ? null : restriction.copy(); 3836 if (input != null) { 3837 dst.input = new ArrayList<ParameterComponent>(); 3838 for (ParameterComponent i : input) 3839 dst.input.add(i.copy()); 3840 }; 3841 if (output != null) { 3842 dst.output = new ArrayList<TaskOutputComponent>(); 3843 for (TaskOutputComponent i : output) 3844 dst.output.add(i.copy()); 3845 }; 3846 return dst; 3847 } 3848 3849 protected Task typedCopy() { 3850 return copy(); 3851 } 3852 3853 @Override 3854 public boolean equalsDeep(Base other_) { 3855 if (!super.equalsDeep(other_)) 3856 return false; 3857 if (!(other_ instanceof Task)) 3858 return false; 3859 Task o = (Task) other_; 3860 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 3861 && compareDeep(basedOn, o.basedOn, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 3862 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 3863 && compareDeep(businessStatus, o.businessStatus, true) && compareDeep(intent, o.intent, true) && compareDeep(priority, o.priority, true) 3864 && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) && compareDeep(focus, o.focus, true) 3865 && compareDeep(for_, o.for_, true) && compareDeep(context, o.context, true) && compareDeep(executionPeriod, o.executionPeriod, true) 3866 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(lastModified, o.lastModified, true) 3867 && compareDeep(requester, o.requester, true) && compareDeep(performerType, o.performerType, true) 3868 && compareDeep(owner, o.owner, true) && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true) 3869 && compareDeep(relevantHistory, o.relevantHistory, true) && compareDeep(restriction, o.restriction, true) 3870 && compareDeep(input, o.input, true) && compareDeep(output, o.output, true); 3871 } 3872 3873 @Override 3874 public boolean equalsShallow(Base other_) { 3875 if (!super.equalsShallow(other_)) 3876 return false; 3877 if (!(other_ instanceof Task)) 3878 return false; 3879 Task o = (Task) other_; 3880 return compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 3881 && compareValues(description, o.description, true) && compareValues(authoredOn, o.authoredOn, true) 3882 && compareValues(lastModified, o.lastModified, true); 3883 } 3884 3885 public boolean isEmpty() { 3886 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 3887 , groupIdentifier, partOf, status, statusReason, businessStatus, intent, priority 3888 , code, description, focus, for_, context, executionPeriod, authoredOn, lastModified 3889 , requester, performerType, owner, reason, note, relevantHistory, restriction 3890 , input, output); 3891 } 3892 3893 @Override 3894 public ResourceType getResourceType() { 3895 return ResourceType.Task; 3896 } 3897 3898 /** 3899 * Search parameter: <b>owner</b> 3900 * <p> 3901 * Description: <b>Search by task owner</b><br> 3902 * Type: <b>reference</b><br> 3903 * Path: <b>Task.owner</b><br> 3904 * </p> 3905 */ 3906 @SearchParamDefinition(name="owner", path="Task.owner", description="Search by task owner", type="reference", target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 3907 public static final String SP_OWNER = "owner"; 3908 /** 3909 * <b>Fluent Client</b> search parameter constant for <b>owner</b> 3910 * <p> 3911 * Description: <b>Search by task owner</b><br> 3912 * Type: <b>reference</b><br> 3913 * Path: <b>Task.owner</b><br> 3914 * </p> 3915 */ 3916 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OWNER); 3917 3918/** 3919 * Constant for fluent queries to be used to add include statements. Specifies 3920 * the path value of "<b>Task:owner</b>". 3921 */ 3922 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNER = new ca.uhn.fhir.model.api.Include("Task:owner").toLocked(); 3923 3924 /** 3925 * Search parameter: <b>requester</b> 3926 * <p> 3927 * Description: <b>Search by task requester</b><br> 3928 * Type: <b>reference</b><br> 3929 * Path: <b>Task.requester.agent</b><br> 3930 * </p> 3931 */ 3932 @SearchParamDefinition(name="requester", path="Task.requester.agent", description="Search by task requester", type="reference", target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 3933 public static final String SP_REQUESTER = "requester"; 3934 /** 3935 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 3936 * <p> 3937 * Description: <b>Search by task requester</b><br> 3938 * Type: <b>reference</b><br> 3939 * Path: <b>Task.requester.agent</b><br> 3940 * </p> 3941 */ 3942 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 3943 3944/** 3945 * Constant for fluent queries to be used to add include statements. Specifies 3946 * the path value of "<b>Task:requester</b>". 3947 */ 3948 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("Task:requester").toLocked(); 3949 3950 /** 3951 * Search parameter: <b>identifier</b> 3952 * <p> 3953 * Description: <b>Search for a task instance by its business identifier</b><br> 3954 * Type: <b>token</b><br> 3955 * Path: <b>Task.identifier</b><br> 3956 * </p> 3957 */ 3958 @SearchParamDefinition(name="identifier", path="Task.identifier", description="Search for a task instance by its business identifier", type="token" ) 3959 public static final String SP_IDENTIFIER = "identifier"; 3960 /** 3961 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3962 * <p> 3963 * Description: <b>Search for a task instance by its business identifier</b><br> 3964 * Type: <b>token</b><br> 3965 * Path: <b>Task.identifier</b><br> 3966 * </p> 3967 */ 3968 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3969 3970 /** 3971 * Search parameter: <b>business-status</b> 3972 * <p> 3973 * Description: <b>Search by business status</b><br> 3974 * Type: <b>token</b><br> 3975 * Path: <b>Task.businessStatus</b><br> 3976 * </p> 3977 */ 3978 @SearchParamDefinition(name="business-status", path="Task.businessStatus", description="Search by business status", type="token" ) 3979 public static final String SP_BUSINESS_STATUS = "business-status"; 3980 /** 3981 * <b>Fluent Client</b> search parameter constant for <b>business-status</b> 3982 * <p> 3983 * Description: <b>Search by business status</b><br> 3984 * Type: <b>token</b><br> 3985 * Path: <b>Task.businessStatus</b><br> 3986 * </p> 3987 */ 3988 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BUSINESS_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BUSINESS_STATUS); 3989 3990 /** 3991 * Search parameter: <b>period</b> 3992 * <p> 3993 * Description: <b>Search by period Task is/was underway</b><br> 3994 * Type: <b>date</b><br> 3995 * Path: <b>Task.executionPeriod</b><br> 3996 * </p> 3997 */ 3998 @SearchParamDefinition(name="period", path="Task.executionPeriod", description="Search by period Task is/was underway", type="date" ) 3999 public static final String SP_PERIOD = "period"; 4000 /** 4001 * <b>Fluent Client</b> search parameter constant for <b>period</b> 4002 * <p> 4003 * Description: <b>Search by period Task is/was underway</b><br> 4004 * Type: <b>date</b><br> 4005 * Path: <b>Task.executionPeriod</b><br> 4006 * </p> 4007 */ 4008 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 4009 4010 /** 4011 * Search parameter: <b>code</b> 4012 * <p> 4013 * Description: <b>Search by task code</b><br> 4014 * Type: <b>token</b><br> 4015 * Path: <b>Task.code</b><br> 4016 * </p> 4017 */ 4018 @SearchParamDefinition(name="code", path="Task.code", description="Search by task code", type="token" ) 4019 public static final String SP_CODE = "code"; 4020 /** 4021 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4022 * <p> 4023 * Description: <b>Search by task code</b><br> 4024 * Type: <b>token</b><br> 4025 * Path: <b>Task.code</b><br> 4026 * </p> 4027 */ 4028 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 4029 4030 /** 4031 * Search parameter: <b>performer</b> 4032 * <p> 4033 * Description: <b>Search by recommended type of performer (e.g., Requester, Performer, Scheduler).</b><br> 4034 * Type: <b>token</b><br> 4035 * Path: <b>Task.performerType</b><br> 4036 * </p> 4037 */ 4038 @SearchParamDefinition(name="performer", path="Task.performerType", description="Search by recommended type of performer (e.g., Requester, Performer, Scheduler).", type="token" ) 4039 public static final String SP_PERFORMER = "performer"; 4040 /** 4041 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 4042 * <p> 4043 * Description: <b>Search by recommended type of performer (e.g., Requester, Performer, Scheduler).</b><br> 4044 * Type: <b>token</b><br> 4045 * Path: <b>Task.performerType</b><br> 4046 * </p> 4047 */ 4048 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PERFORMER); 4049 4050 /** 4051 * Search parameter: <b>subject</b> 4052 * <p> 4053 * Description: <b>Search by subject</b><br> 4054 * Type: <b>reference</b><br> 4055 * Path: <b>Task.for</b><br> 4056 * </p> 4057 */ 4058 @SearchParamDefinition(name="subject", path="Task.for", description="Search by subject", type="reference" ) 4059 public static final String SP_SUBJECT = "subject"; 4060 /** 4061 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4062 * <p> 4063 * Description: <b>Search by subject</b><br> 4064 * Type: <b>reference</b><br> 4065 * Path: <b>Task.for</b><br> 4066 * </p> 4067 */ 4068 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4069 4070/** 4071 * Constant for fluent queries to be used to add include statements. Specifies 4072 * the path value of "<b>Task:subject</b>". 4073 */ 4074 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Task:subject").toLocked(); 4075 4076 /** 4077 * Search parameter: <b>focus</b> 4078 * <p> 4079 * Description: <b>Search by task focus</b><br> 4080 * Type: <b>reference</b><br> 4081 * Path: <b>Task.focus</b><br> 4082 * </p> 4083 */ 4084 @SearchParamDefinition(name="focus", path="Task.focus", description="Search by task focus", type="reference" ) 4085 public static final String SP_FOCUS = "focus"; 4086 /** 4087 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 4088 * <p> 4089 * Description: <b>Search by task focus</b><br> 4090 * Type: <b>reference</b><br> 4091 * Path: <b>Task.focus</b><br> 4092 * </p> 4093 */ 4094 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOCUS); 4095 4096/** 4097 * Constant for fluent queries to be used to add include statements. Specifies 4098 * the path value of "<b>Task:focus</b>". 4099 */ 4100 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include("Task:focus").toLocked(); 4101 4102 /** 4103 * Search parameter: <b>part-of</b> 4104 * <p> 4105 * Description: <b>Search by task this task is part of</b><br> 4106 * Type: <b>reference</b><br> 4107 * Path: <b>Task.partOf</b><br> 4108 * </p> 4109 */ 4110 @SearchParamDefinition(name="part-of", path="Task.partOf", description="Search by task this task is part of", type="reference", target={Task.class } ) 4111 public static final String SP_PART_OF = "part-of"; 4112 /** 4113 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 4114 * <p> 4115 * Description: <b>Search by task this task is part of</b><br> 4116 * Type: <b>reference</b><br> 4117 * Path: <b>Task.partOf</b><br> 4118 * </p> 4119 */ 4120 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 4121 4122/** 4123 * Constant for fluent queries to be used to add include statements. Specifies 4124 * the path value of "<b>Task:part-of</b>". 4125 */ 4126 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Task:part-of").toLocked(); 4127 4128 /** 4129 * Search parameter: <b>priority</b> 4130 * <p> 4131 * Description: <b>Search by task priority</b><br> 4132 * Type: <b>token</b><br> 4133 * Path: <b>Task.priority</b><br> 4134 * </p> 4135 */ 4136 @SearchParamDefinition(name="priority", path="Task.priority", description="Search by task priority", type="token" ) 4137 public static final String SP_PRIORITY = "priority"; 4138 /** 4139 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 4140 * <p> 4141 * Description: <b>Search by task priority</b><br> 4142 * Type: <b>token</b><br> 4143 * Path: <b>Task.priority</b><br> 4144 * </p> 4145 */ 4146 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 4147 4148 /** 4149 * Search parameter: <b>authored-on</b> 4150 * <p> 4151 * Description: <b>Search by creation date</b><br> 4152 * Type: <b>date</b><br> 4153 * Path: <b>Task.authoredOn</b><br> 4154 * </p> 4155 */ 4156 @SearchParamDefinition(name="authored-on", path="Task.authoredOn", description="Search by creation date", type="date" ) 4157 public static final String SP_AUTHORED_ON = "authored-on"; 4158 /** 4159 * <b>Fluent Client</b> search parameter constant for <b>authored-on</b> 4160 * <p> 4161 * Description: <b>Search by creation date</b><br> 4162 * Type: <b>date</b><br> 4163 * Path: <b>Task.authoredOn</b><br> 4164 * </p> 4165 */ 4166 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED_ON = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORED_ON); 4167 4168 /** 4169 * Search parameter: <b>intent</b> 4170 * <p> 4171 * Description: <b>Search by task intent</b><br> 4172 * Type: <b>token</b><br> 4173 * Path: <b>Task.intent</b><br> 4174 * </p> 4175 */ 4176 @SearchParamDefinition(name="intent", path="Task.intent", description="Search by task intent", type="token" ) 4177 public static final String SP_INTENT = "intent"; 4178 /** 4179 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 4180 * <p> 4181 * Description: <b>Search by task intent</b><br> 4182 * Type: <b>token</b><br> 4183 * Path: <b>Task.intent</b><br> 4184 * </p> 4185 */ 4186 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 4187 4188 /** 4189 * Search parameter: <b>group-identifier</b> 4190 * <p> 4191 * Description: <b>Search by group identifier</b><br> 4192 * Type: <b>token</b><br> 4193 * Path: <b>Task.groupIdentifier</b><br> 4194 * </p> 4195 */ 4196 @SearchParamDefinition(name="group-identifier", path="Task.groupIdentifier", description="Search by group identifier", type="token" ) 4197 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 4198 /** 4199 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 4200 * <p> 4201 * Description: <b>Search by group identifier</b><br> 4202 * Type: <b>token</b><br> 4203 * Path: <b>Task.groupIdentifier</b><br> 4204 * </p> 4205 */ 4206 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GROUP_IDENTIFIER); 4207 4208 /** 4209 * Search parameter: <b>based-on</b> 4210 * <p> 4211 * Description: <b>Search by requests this task is based on</b><br> 4212 * Type: <b>reference</b><br> 4213 * Path: <b>Task.basedOn</b><br> 4214 * </p> 4215 */ 4216 @SearchParamDefinition(name="based-on", path="Task.basedOn", description="Search by requests this task is based on", type="reference" ) 4217 public static final String SP_BASED_ON = "based-on"; 4218 /** 4219 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 4220 * <p> 4221 * Description: <b>Search by requests this task is based on</b><br> 4222 * Type: <b>reference</b><br> 4223 * Path: <b>Task.basedOn</b><br> 4224 * </p> 4225 */ 4226 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 4227 4228/** 4229 * Constant for fluent queries to be used to add include statements. Specifies 4230 * the path value of "<b>Task:based-on</b>". 4231 */ 4232 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Task:based-on").toLocked(); 4233 4234 /** 4235 * Search parameter: <b>patient</b> 4236 * <p> 4237 * Description: <b>Search by patient</b><br> 4238 * Type: <b>reference</b><br> 4239 * Path: <b>Task.for</b><br> 4240 * </p> 4241 */ 4242 @SearchParamDefinition(name="patient", path="Task.for", description="Search by patient", type="reference", target={Patient.class } ) 4243 public static final String SP_PATIENT = "patient"; 4244 /** 4245 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4246 * <p> 4247 * Description: <b>Search by patient</b><br> 4248 * Type: <b>reference</b><br> 4249 * Path: <b>Task.for</b><br> 4250 * </p> 4251 */ 4252 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4253 4254/** 4255 * Constant for fluent queries to be used to add include statements. Specifies 4256 * the path value of "<b>Task:patient</b>". 4257 */ 4258 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Task:patient").toLocked(); 4259 4260 /** 4261 * Search parameter: <b>organization</b> 4262 * <p> 4263 * Description: <b>Search by responsible organization</b><br> 4264 * Type: <b>reference</b><br> 4265 * Path: <b>Task.requester.onBehalfOf</b><br> 4266 * </p> 4267 */ 4268 @SearchParamDefinition(name="organization", path="Task.requester.onBehalfOf", description="Search by responsible organization", type="reference", target={Organization.class } ) 4269 public static final String SP_ORGANIZATION = "organization"; 4270 /** 4271 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 4272 * <p> 4273 * Description: <b>Search by responsible organization</b><br> 4274 * Type: <b>reference</b><br> 4275 * Path: <b>Task.requester.onBehalfOf</b><br> 4276 * </p> 4277 */ 4278 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 4279 4280/** 4281 * Constant for fluent queries to be used to add include statements. Specifies 4282 * the path value of "<b>Task:organization</b>". 4283 */ 4284 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Task:organization").toLocked(); 4285 4286 /** 4287 * Search parameter: <b>context</b> 4288 * <p> 4289 * Description: <b>Search by encounter or episode</b><br> 4290 * Type: <b>reference</b><br> 4291 * Path: <b>Task.context</b><br> 4292 * </p> 4293 */ 4294 @SearchParamDefinition(name="context", path="Task.context", description="Search by encounter or episode", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 4295 public static final String SP_CONTEXT = "context"; 4296 /** 4297 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4298 * <p> 4299 * Description: <b>Search by encounter or episode</b><br> 4300 * Type: <b>reference</b><br> 4301 * Path: <b>Task.context</b><br> 4302 * </p> 4303 */ 4304 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 4305 4306/** 4307 * Constant for fluent queries to be used to add include statements. Specifies 4308 * the path value of "<b>Task:context</b>". 4309 */ 4310 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("Task:context").toLocked(); 4311 4312 /** 4313 * Search parameter: <b>modified</b> 4314 * <p> 4315 * Description: <b>Search by last modification date</b><br> 4316 * Type: <b>date</b><br> 4317 * Path: <b>Task.lastModified</b><br> 4318 * </p> 4319 */ 4320 @SearchParamDefinition(name="modified", path="Task.lastModified", description="Search by last modification date", type="date" ) 4321 public static final String SP_MODIFIED = "modified"; 4322 /** 4323 * <b>Fluent Client</b> search parameter constant for <b>modified</b> 4324 * <p> 4325 * Description: <b>Search by last modification date</b><br> 4326 * Type: <b>date</b><br> 4327 * Path: <b>Task.lastModified</b><br> 4328 * </p> 4329 */ 4330 public static final ca.uhn.fhir.rest.gclient.DateClientParam MODIFIED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_MODIFIED); 4331 4332 /** 4333 * Search parameter: <b>status</b> 4334 * <p> 4335 * Description: <b>Search by task status</b><br> 4336 * Type: <b>token</b><br> 4337 * Path: <b>Task.status</b><br> 4338 * </p> 4339 */ 4340 @SearchParamDefinition(name="status", path="Task.status", description="Search by task status", type="token" ) 4341 public static final String SP_STATUS = "status"; 4342 /** 4343 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4344 * <p> 4345 * Description: <b>Search by task status</b><br> 4346 * Type: <b>token</b><br> 4347 * Path: <b>Task.status</b><br> 4348 * </p> 4349 */ 4350 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4351 4352 4353}