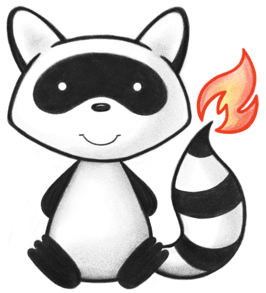
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A summary of information based on the results of executing a TestScript. 050 */ 051@ResourceDef(name="TestReport", profile="http://hl7.org/fhir/Profile/TestReport") 052public class TestReport extends DomainResource { 053 054 public enum TestReportStatus { 055 /** 056 * All test operations have completed 057 */ 058 COMPLETED, 059 /** 060 * A test operations is currently executing 061 */ 062 INPROGRESS, 063 /** 064 * A test operation is waiting for an external client request 065 */ 066 WAITING, 067 /** 068 * The test script execution was manually stopped 069 */ 070 STOPPED, 071 /** 072 * This test report was entered or created in error 073 */ 074 ENTEREDINERROR, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 public static TestReportStatus fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("completed".equals(codeString)) 083 return COMPLETED; 084 if ("in-progress".equals(codeString)) 085 return INPROGRESS; 086 if ("waiting".equals(codeString)) 087 return WAITING; 088 if ("stopped".equals(codeString)) 089 return STOPPED; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if (Configuration.isAcceptInvalidEnums()) 093 return null; 094 else 095 throw new FHIRException("Unknown TestReportStatus code '"+codeString+"'"); 096 } 097 public String toCode() { 098 switch (this) { 099 case COMPLETED: return "completed"; 100 case INPROGRESS: return "in-progress"; 101 case WAITING: return "waiting"; 102 case STOPPED: return "stopped"; 103 case ENTEREDINERROR: return "entered-in-error"; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getSystem() { 109 switch (this) { 110 case COMPLETED: return "http://hl7.org/fhir/report-status-codes"; 111 case INPROGRESS: return "http://hl7.org/fhir/report-status-codes"; 112 case WAITING: return "http://hl7.org/fhir/report-status-codes"; 113 case STOPPED: return "http://hl7.org/fhir/report-status-codes"; 114 case ENTEREDINERROR: return "http://hl7.org/fhir/report-status-codes"; 115 case NULL: return null; 116 default: return "?"; 117 } 118 } 119 public String getDefinition() { 120 switch (this) { 121 case COMPLETED: return "All test operations have completed"; 122 case INPROGRESS: return "A test operations is currently executing"; 123 case WAITING: return "A test operation is waiting for an external client request"; 124 case STOPPED: return "The test script execution was manually stopped"; 125 case ENTEREDINERROR: return "This test report was entered or created in error"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case COMPLETED: return "Completed"; 133 case INPROGRESS: return "In Progress"; 134 case WAITING: return "Waiting"; 135 case STOPPED: return "Stopped"; 136 case ENTEREDINERROR: return "Entered In Error"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 } 142 143 public static class TestReportStatusEnumFactory implements EnumFactory<TestReportStatus> { 144 public TestReportStatus fromCode(String codeString) throws IllegalArgumentException { 145 if (codeString == null || "".equals(codeString)) 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("completed".equals(codeString)) 149 return TestReportStatus.COMPLETED; 150 if ("in-progress".equals(codeString)) 151 return TestReportStatus.INPROGRESS; 152 if ("waiting".equals(codeString)) 153 return TestReportStatus.WAITING; 154 if ("stopped".equals(codeString)) 155 return TestReportStatus.STOPPED; 156 if ("entered-in-error".equals(codeString)) 157 return TestReportStatus.ENTEREDINERROR; 158 throw new IllegalArgumentException("Unknown TestReportStatus code '"+codeString+"'"); 159 } 160 public Enumeration<TestReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 161 if (code == null) 162 return null; 163 if (code.isEmpty()) 164 return new Enumeration<TestReportStatus>(this); 165 String codeString = code.asStringValue(); 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("completed".equals(codeString)) 169 return new Enumeration<TestReportStatus>(this, TestReportStatus.COMPLETED); 170 if ("in-progress".equals(codeString)) 171 return new Enumeration<TestReportStatus>(this, TestReportStatus.INPROGRESS); 172 if ("waiting".equals(codeString)) 173 return new Enumeration<TestReportStatus>(this, TestReportStatus.WAITING); 174 if ("stopped".equals(codeString)) 175 return new Enumeration<TestReportStatus>(this, TestReportStatus.STOPPED); 176 if ("entered-in-error".equals(codeString)) 177 return new Enumeration<TestReportStatus>(this, TestReportStatus.ENTEREDINERROR); 178 throw new FHIRException("Unknown TestReportStatus code '"+codeString+"'"); 179 } 180 public String toCode(TestReportStatus code) { 181 if (code == TestReportStatus.NULL) 182 return null; 183 if (code == TestReportStatus.COMPLETED) 184 return "completed"; 185 if (code == TestReportStatus.INPROGRESS) 186 return "in-progress"; 187 if (code == TestReportStatus.WAITING) 188 return "waiting"; 189 if (code == TestReportStatus.STOPPED) 190 return "stopped"; 191 if (code == TestReportStatus.ENTEREDINERROR) 192 return "entered-in-error"; 193 return "?"; 194 } 195 public String toSystem(TestReportStatus code) { 196 return code.getSystem(); 197 } 198 } 199 200 public enum TestReportResult { 201 /** 202 * All test operations successfully passed all asserts 203 */ 204 PASS, 205 /** 206 * One or more test operations failed one or more asserts 207 */ 208 FAIL, 209 /** 210 * One or more test operations is pending execution completion 211 */ 212 PENDING, 213 /** 214 * added to help the parsers with the generic types 215 */ 216 NULL; 217 public static TestReportResult fromCode(String codeString) throws FHIRException { 218 if (codeString == null || "".equals(codeString)) 219 return null; 220 if ("pass".equals(codeString)) 221 return PASS; 222 if ("fail".equals(codeString)) 223 return FAIL; 224 if ("pending".equals(codeString)) 225 return PENDING; 226 if (Configuration.isAcceptInvalidEnums()) 227 return null; 228 else 229 throw new FHIRException("Unknown TestReportResult code '"+codeString+"'"); 230 } 231 public String toCode() { 232 switch (this) { 233 case PASS: return "pass"; 234 case FAIL: return "fail"; 235 case PENDING: return "pending"; 236 case NULL: return null; 237 default: return "?"; 238 } 239 } 240 public String getSystem() { 241 switch (this) { 242 case PASS: return "http://hl7.org/fhir/report-result-codes"; 243 case FAIL: return "http://hl7.org/fhir/report-result-codes"; 244 case PENDING: return "http://hl7.org/fhir/report-result-codes"; 245 case NULL: return null; 246 default: return "?"; 247 } 248 } 249 public String getDefinition() { 250 switch (this) { 251 case PASS: return "All test operations successfully passed all asserts"; 252 case FAIL: return "One or more test operations failed one or more asserts"; 253 case PENDING: return "One or more test operations is pending execution completion"; 254 case NULL: return null; 255 default: return "?"; 256 } 257 } 258 public String getDisplay() { 259 switch (this) { 260 case PASS: return "Pass"; 261 case FAIL: return "Fail"; 262 case PENDING: return "Pending"; 263 case NULL: return null; 264 default: return "?"; 265 } 266 } 267 } 268 269 public static class TestReportResultEnumFactory implements EnumFactory<TestReportResult> { 270 public TestReportResult fromCode(String codeString) throws IllegalArgumentException { 271 if (codeString == null || "".equals(codeString)) 272 if (codeString == null || "".equals(codeString)) 273 return null; 274 if ("pass".equals(codeString)) 275 return TestReportResult.PASS; 276 if ("fail".equals(codeString)) 277 return TestReportResult.FAIL; 278 if ("pending".equals(codeString)) 279 return TestReportResult.PENDING; 280 throw new IllegalArgumentException("Unknown TestReportResult code '"+codeString+"'"); 281 } 282 public Enumeration<TestReportResult> fromType(PrimitiveType<?> code) throws FHIRException { 283 if (code == null) 284 return null; 285 if (code.isEmpty()) 286 return new Enumeration<TestReportResult>(this); 287 String codeString = code.asStringValue(); 288 if (codeString == null || "".equals(codeString)) 289 return null; 290 if ("pass".equals(codeString)) 291 return new Enumeration<TestReportResult>(this, TestReportResult.PASS); 292 if ("fail".equals(codeString)) 293 return new Enumeration<TestReportResult>(this, TestReportResult.FAIL); 294 if ("pending".equals(codeString)) 295 return new Enumeration<TestReportResult>(this, TestReportResult.PENDING); 296 throw new FHIRException("Unknown TestReportResult code '"+codeString+"'"); 297 } 298 public String toCode(TestReportResult code) { 299 if (code == TestReportResult.NULL) 300 return null; 301 if (code == TestReportResult.PASS) 302 return "pass"; 303 if (code == TestReportResult.FAIL) 304 return "fail"; 305 if (code == TestReportResult.PENDING) 306 return "pending"; 307 return "?"; 308 } 309 public String toSystem(TestReportResult code) { 310 return code.getSystem(); 311 } 312 } 313 314 public enum TestReportParticipantType { 315 /** 316 * The test execution engine. 317 */ 318 TESTENGINE, 319 /** 320 * A FHIR Client 321 */ 322 CLIENT, 323 /** 324 * A FHIR Server 325 */ 326 SERVER, 327 /** 328 * added to help the parsers with the generic types 329 */ 330 NULL; 331 public static TestReportParticipantType fromCode(String codeString) throws FHIRException { 332 if (codeString == null || "".equals(codeString)) 333 return null; 334 if ("test-engine".equals(codeString)) 335 return TESTENGINE; 336 if ("client".equals(codeString)) 337 return CLIENT; 338 if ("server".equals(codeString)) 339 return SERVER; 340 if (Configuration.isAcceptInvalidEnums()) 341 return null; 342 else 343 throw new FHIRException("Unknown TestReportParticipantType code '"+codeString+"'"); 344 } 345 public String toCode() { 346 switch (this) { 347 case TESTENGINE: return "test-engine"; 348 case CLIENT: return "client"; 349 case SERVER: return "server"; 350 case NULL: return null; 351 default: return "?"; 352 } 353 } 354 public String getSystem() { 355 switch (this) { 356 case TESTENGINE: return "http://hl7.org/fhir/report-participant-type"; 357 case CLIENT: return "http://hl7.org/fhir/report-participant-type"; 358 case SERVER: return "http://hl7.org/fhir/report-participant-type"; 359 case NULL: return null; 360 default: return "?"; 361 } 362 } 363 public String getDefinition() { 364 switch (this) { 365 case TESTENGINE: return "The test execution engine."; 366 case CLIENT: return "A FHIR Client"; 367 case SERVER: return "A FHIR Server"; 368 case NULL: return null; 369 default: return "?"; 370 } 371 } 372 public String getDisplay() { 373 switch (this) { 374 case TESTENGINE: return "Test Engine"; 375 case CLIENT: return "Client"; 376 case SERVER: return "Server"; 377 case NULL: return null; 378 default: return "?"; 379 } 380 } 381 } 382 383 public static class TestReportParticipantTypeEnumFactory implements EnumFactory<TestReportParticipantType> { 384 public TestReportParticipantType fromCode(String codeString) throws IllegalArgumentException { 385 if (codeString == null || "".equals(codeString)) 386 if (codeString == null || "".equals(codeString)) 387 return null; 388 if ("test-engine".equals(codeString)) 389 return TestReportParticipantType.TESTENGINE; 390 if ("client".equals(codeString)) 391 return TestReportParticipantType.CLIENT; 392 if ("server".equals(codeString)) 393 return TestReportParticipantType.SERVER; 394 throw new IllegalArgumentException("Unknown TestReportParticipantType code '"+codeString+"'"); 395 } 396 public Enumeration<TestReportParticipantType> fromType(PrimitiveType<?> code) throws FHIRException { 397 if (code == null) 398 return null; 399 if (code.isEmpty()) 400 return new Enumeration<TestReportParticipantType>(this); 401 String codeString = code.asStringValue(); 402 if (codeString == null || "".equals(codeString)) 403 return null; 404 if ("test-engine".equals(codeString)) 405 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.TESTENGINE); 406 if ("client".equals(codeString)) 407 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.CLIENT); 408 if ("server".equals(codeString)) 409 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.SERVER); 410 throw new FHIRException("Unknown TestReportParticipantType code '"+codeString+"'"); 411 } 412 public String toCode(TestReportParticipantType code) { 413 if (code == TestReportParticipantType.NULL) 414 return null; 415 if (code == TestReportParticipantType.TESTENGINE) 416 return "test-engine"; 417 if (code == TestReportParticipantType.CLIENT) 418 return "client"; 419 if (code == TestReportParticipantType.SERVER) 420 return "server"; 421 return "?"; 422 } 423 public String toSystem(TestReportParticipantType code) { 424 return code.getSystem(); 425 } 426 } 427 428 public enum TestReportActionResult { 429 /** 430 * The action was successful. 431 */ 432 PASS, 433 /** 434 * The action was skipped. 435 */ 436 SKIP, 437 /** 438 * The action failed. 439 */ 440 FAIL, 441 /** 442 * The action passed but with warnings. 443 */ 444 WARNING, 445 /** 446 * The action encountered a fatal error and the engine was unable to process. 447 */ 448 ERROR, 449 /** 450 * added to help the parsers with the generic types 451 */ 452 NULL; 453 public static TestReportActionResult fromCode(String codeString) throws FHIRException { 454 if (codeString == null || "".equals(codeString)) 455 return null; 456 if ("pass".equals(codeString)) 457 return PASS; 458 if ("skip".equals(codeString)) 459 return SKIP; 460 if ("fail".equals(codeString)) 461 return FAIL; 462 if ("warning".equals(codeString)) 463 return WARNING; 464 if ("error".equals(codeString)) 465 return ERROR; 466 if (Configuration.isAcceptInvalidEnums()) 467 return null; 468 else 469 throw new FHIRException("Unknown TestReportActionResult code '"+codeString+"'"); 470 } 471 public String toCode() { 472 switch (this) { 473 case PASS: return "pass"; 474 case SKIP: return "skip"; 475 case FAIL: return "fail"; 476 case WARNING: return "warning"; 477 case ERROR: return "error"; 478 case NULL: return null; 479 default: return "?"; 480 } 481 } 482 public String getSystem() { 483 switch (this) { 484 case PASS: return "http://hl7.org/fhir/report-action-result-codes"; 485 case SKIP: return "http://hl7.org/fhir/report-action-result-codes"; 486 case FAIL: return "http://hl7.org/fhir/report-action-result-codes"; 487 case WARNING: return "http://hl7.org/fhir/report-action-result-codes"; 488 case ERROR: return "http://hl7.org/fhir/report-action-result-codes"; 489 case NULL: return null; 490 default: return "?"; 491 } 492 } 493 public String getDefinition() { 494 switch (this) { 495 case PASS: return "The action was successful."; 496 case SKIP: return "The action was skipped."; 497 case FAIL: return "The action failed."; 498 case WARNING: return "The action passed but with warnings."; 499 case ERROR: return "The action encountered a fatal error and the engine was unable to process."; 500 case NULL: return null; 501 default: return "?"; 502 } 503 } 504 public String getDisplay() { 505 switch (this) { 506 case PASS: return "Pass"; 507 case SKIP: return "Skip"; 508 case FAIL: return "Fail"; 509 case WARNING: return "Warning"; 510 case ERROR: return "Error"; 511 case NULL: return null; 512 default: return "?"; 513 } 514 } 515 } 516 517 public static class TestReportActionResultEnumFactory implements EnumFactory<TestReportActionResult> { 518 public TestReportActionResult fromCode(String codeString) throws IllegalArgumentException { 519 if (codeString == null || "".equals(codeString)) 520 if (codeString == null || "".equals(codeString)) 521 return null; 522 if ("pass".equals(codeString)) 523 return TestReportActionResult.PASS; 524 if ("skip".equals(codeString)) 525 return TestReportActionResult.SKIP; 526 if ("fail".equals(codeString)) 527 return TestReportActionResult.FAIL; 528 if ("warning".equals(codeString)) 529 return TestReportActionResult.WARNING; 530 if ("error".equals(codeString)) 531 return TestReportActionResult.ERROR; 532 throw new IllegalArgumentException("Unknown TestReportActionResult code '"+codeString+"'"); 533 } 534 public Enumeration<TestReportActionResult> fromType(PrimitiveType<?> code) throws FHIRException { 535 if (code == null) 536 return null; 537 if (code.isEmpty()) 538 return new Enumeration<TestReportActionResult>(this); 539 String codeString = code.asStringValue(); 540 if (codeString == null || "".equals(codeString)) 541 return null; 542 if ("pass".equals(codeString)) 543 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.PASS); 544 if ("skip".equals(codeString)) 545 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.SKIP); 546 if ("fail".equals(codeString)) 547 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.FAIL); 548 if ("warning".equals(codeString)) 549 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.WARNING); 550 if ("error".equals(codeString)) 551 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.ERROR); 552 throw new FHIRException("Unknown TestReportActionResult code '"+codeString+"'"); 553 } 554 public String toCode(TestReportActionResult code) { 555 if (code == TestReportActionResult.NULL) 556 return null; 557 if (code == TestReportActionResult.PASS) 558 return "pass"; 559 if (code == TestReportActionResult.SKIP) 560 return "skip"; 561 if (code == TestReportActionResult.FAIL) 562 return "fail"; 563 if (code == TestReportActionResult.WARNING) 564 return "warning"; 565 if (code == TestReportActionResult.ERROR) 566 return "error"; 567 return "?"; 568 } 569 public String toSystem(TestReportActionResult code) { 570 return code.getSystem(); 571 } 572 } 573 574 @Block() 575 public static class TestReportParticipantComponent extends BackboneElement implements IBaseBackboneElement { 576 /** 577 * The type of participant. 578 */ 579 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 580 @Description(shortDefinition="test-engine | client | server", formalDefinition="The type of participant." ) 581 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-participant-type") 582 protected Enumeration<TestReportParticipantType> type; 583 584 /** 585 * The uri of the participant. An absolute URL is preferred. 586 */ 587 @Child(name = "uri", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=false) 588 @Description(shortDefinition="The uri of the participant. An absolute URL is preferred", formalDefinition="The uri of the participant. An absolute URL is preferred." ) 589 protected UriType uri; 590 591 /** 592 * The display name of the participant. 593 */ 594 @Child(name = "display", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 595 @Description(shortDefinition="The display name of the participant", formalDefinition="The display name of the participant." ) 596 protected StringType display; 597 598 private static final long serialVersionUID = 577488357L; 599 600 /** 601 * Constructor 602 */ 603 public TestReportParticipantComponent() { 604 super(); 605 } 606 607 /** 608 * Constructor 609 */ 610 public TestReportParticipantComponent(Enumeration<TestReportParticipantType> type, UriType uri) { 611 super(); 612 this.type = type; 613 this.uri = uri; 614 } 615 616 /** 617 * @return {@link #type} (The type of participant.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 618 */ 619 public Enumeration<TestReportParticipantType> getTypeElement() { 620 if (this.type == null) 621 if (Configuration.errorOnAutoCreate()) 622 throw new Error("Attempt to auto-create TestReportParticipantComponent.type"); 623 else if (Configuration.doAutoCreate()) 624 this.type = new Enumeration<TestReportParticipantType>(new TestReportParticipantTypeEnumFactory()); // bb 625 return this.type; 626 } 627 628 public boolean hasTypeElement() { 629 return this.type != null && !this.type.isEmpty(); 630 } 631 632 public boolean hasType() { 633 return this.type != null && !this.type.isEmpty(); 634 } 635 636 /** 637 * @param value {@link #type} (The type of participant.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 638 */ 639 public TestReportParticipantComponent setTypeElement(Enumeration<TestReportParticipantType> value) { 640 this.type = value; 641 return this; 642 } 643 644 /** 645 * @return The type of participant. 646 */ 647 public TestReportParticipantType getType() { 648 return this.type == null ? null : this.type.getValue(); 649 } 650 651 /** 652 * @param value The type of participant. 653 */ 654 public TestReportParticipantComponent setType(TestReportParticipantType value) { 655 if (this.type == null) 656 this.type = new Enumeration<TestReportParticipantType>(new TestReportParticipantTypeEnumFactory()); 657 this.type.setValue(value); 658 return this; 659 } 660 661 /** 662 * @return {@link #uri} (The uri of the participant. An absolute URL is preferred.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 663 */ 664 public UriType getUriElement() { 665 if (this.uri == null) 666 if (Configuration.errorOnAutoCreate()) 667 throw new Error("Attempt to auto-create TestReportParticipantComponent.uri"); 668 else if (Configuration.doAutoCreate()) 669 this.uri = new UriType(); // bb 670 return this.uri; 671 } 672 673 public boolean hasUriElement() { 674 return this.uri != null && !this.uri.isEmpty(); 675 } 676 677 public boolean hasUri() { 678 return this.uri != null && !this.uri.isEmpty(); 679 } 680 681 /** 682 * @param value {@link #uri} (The uri of the participant. An absolute URL is preferred.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 683 */ 684 public TestReportParticipantComponent setUriElement(UriType value) { 685 this.uri = value; 686 return this; 687 } 688 689 /** 690 * @return The uri of the participant. An absolute URL is preferred. 691 */ 692 public String getUri() { 693 return this.uri == null ? null : this.uri.getValue(); 694 } 695 696 /** 697 * @param value The uri of the participant. An absolute URL is preferred. 698 */ 699 public TestReportParticipantComponent setUri(String value) { 700 if (this.uri == null) 701 this.uri = new UriType(); 702 this.uri.setValue(value); 703 return this; 704 } 705 706 /** 707 * @return {@link #display} (The display name of the participant.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 708 */ 709 public StringType getDisplayElement() { 710 if (this.display == null) 711 if (Configuration.errorOnAutoCreate()) 712 throw new Error("Attempt to auto-create TestReportParticipantComponent.display"); 713 else if (Configuration.doAutoCreate()) 714 this.display = new StringType(); // bb 715 return this.display; 716 } 717 718 public boolean hasDisplayElement() { 719 return this.display != null && !this.display.isEmpty(); 720 } 721 722 public boolean hasDisplay() { 723 return this.display != null && !this.display.isEmpty(); 724 } 725 726 /** 727 * @param value {@link #display} (The display name of the participant.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 728 */ 729 public TestReportParticipantComponent setDisplayElement(StringType value) { 730 this.display = value; 731 return this; 732 } 733 734 /** 735 * @return The display name of the participant. 736 */ 737 public String getDisplay() { 738 return this.display == null ? null : this.display.getValue(); 739 } 740 741 /** 742 * @param value The display name of the participant. 743 */ 744 public TestReportParticipantComponent setDisplay(String value) { 745 if (Utilities.noString(value)) 746 this.display = null; 747 else { 748 if (this.display == null) 749 this.display = new StringType(); 750 this.display.setValue(value); 751 } 752 return this; 753 } 754 755 protected void listChildren(List<Property> children) { 756 super.listChildren(children); 757 children.add(new Property("type", "code", "The type of participant.", 0, 1, type)); 758 children.add(new Property("uri", "uri", "The uri of the participant. An absolute URL is preferred.", 0, 1, uri)); 759 children.add(new Property("display", "string", "The display name of the participant.", 0, 1, display)); 760 } 761 762 @Override 763 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 764 switch (_hash) { 765 case 3575610: /*type*/ return new Property("type", "code", "The type of participant.", 0, 1, type); 766 case 116076: /*uri*/ return new Property("uri", "uri", "The uri of the participant. An absolute URL is preferred.", 0, 1, uri); 767 case 1671764162: /*display*/ return new Property("display", "string", "The display name of the participant.", 0, 1, display); 768 default: return super.getNamedProperty(_hash, _name, _checkValid); 769 } 770 771 } 772 773 @Override 774 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 775 switch (hash) { 776 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<TestReportParticipantType> 777 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 778 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 779 default: return super.getProperty(hash, name, checkValid); 780 } 781 782 } 783 784 @Override 785 public Base setProperty(int hash, String name, Base value) throws FHIRException { 786 switch (hash) { 787 case 3575610: // type 788 value = new TestReportParticipantTypeEnumFactory().fromType(castToCode(value)); 789 this.type = (Enumeration) value; // Enumeration<TestReportParticipantType> 790 return value; 791 case 116076: // uri 792 this.uri = castToUri(value); // UriType 793 return value; 794 case 1671764162: // display 795 this.display = castToString(value); // StringType 796 return value; 797 default: return super.setProperty(hash, name, value); 798 } 799 800 } 801 802 @Override 803 public Base setProperty(String name, Base value) throws FHIRException { 804 if (name.equals("type")) { 805 value = new TestReportParticipantTypeEnumFactory().fromType(castToCode(value)); 806 this.type = (Enumeration) value; // Enumeration<TestReportParticipantType> 807 } else if (name.equals("uri")) { 808 this.uri = castToUri(value); // UriType 809 } else if (name.equals("display")) { 810 this.display = castToString(value); // StringType 811 } else 812 return super.setProperty(name, value); 813 return value; 814 } 815 816 @Override 817 public Base makeProperty(int hash, String name) throws FHIRException { 818 switch (hash) { 819 case 3575610: return getTypeElement(); 820 case 116076: return getUriElement(); 821 case 1671764162: return getDisplayElement(); 822 default: return super.makeProperty(hash, name); 823 } 824 825 } 826 827 @Override 828 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 829 switch (hash) { 830 case 3575610: /*type*/ return new String[] {"code"}; 831 case 116076: /*uri*/ return new String[] {"uri"}; 832 case 1671764162: /*display*/ return new String[] {"string"}; 833 default: return super.getTypesForProperty(hash, name); 834 } 835 836 } 837 838 @Override 839 public Base addChild(String name) throws FHIRException { 840 if (name.equals("type")) { 841 throw new FHIRException("Cannot call addChild on a singleton property TestReport.type"); 842 } 843 else if (name.equals("uri")) { 844 throw new FHIRException("Cannot call addChild on a singleton property TestReport.uri"); 845 } 846 else if (name.equals("display")) { 847 throw new FHIRException("Cannot call addChild on a singleton property TestReport.display"); 848 } 849 else 850 return super.addChild(name); 851 } 852 853 public TestReportParticipantComponent copy() { 854 TestReportParticipantComponent dst = new TestReportParticipantComponent(); 855 copyValues(dst); 856 dst.type = type == null ? null : type.copy(); 857 dst.uri = uri == null ? null : uri.copy(); 858 dst.display = display == null ? null : display.copy(); 859 return dst; 860 } 861 862 @Override 863 public boolean equalsDeep(Base other_) { 864 if (!super.equalsDeep(other_)) 865 return false; 866 if (!(other_ instanceof TestReportParticipantComponent)) 867 return false; 868 TestReportParticipantComponent o = (TestReportParticipantComponent) other_; 869 return compareDeep(type, o.type, true) && compareDeep(uri, o.uri, true) && compareDeep(display, o.display, true) 870 ; 871 } 872 873 @Override 874 public boolean equalsShallow(Base other_) { 875 if (!super.equalsShallow(other_)) 876 return false; 877 if (!(other_ instanceof TestReportParticipantComponent)) 878 return false; 879 TestReportParticipantComponent o = (TestReportParticipantComponent) other_; 880 return compareValues(type, o.type, true) && compareValues(uri, o.uri, true) && compareValues(display, o.display, true) 881 ; 882 } 883 884 public boolean isEmpty() { 885 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, uri, display); 886 } 887 888 public String fhirType() { 889 return "TestReport.participant"; 890 891 } 892 893 } 894 895 @Block() 896 public static class TestReportSetupComponent extends BackboneElement implements IBaseBackboneElement { 897 /** 898 * Action would contain either an operation or an assertion. 899 */ 900 @Child(name = "action", type = {}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 901 @Description(shortDefinition="A setup operation or assert that was executed", formalDefinition="Action would contain either an operation or an assertion." ) 902 protected List<SetupActionComponent> action; 903 904 private static final long serialVersionUID = -123374486L; 905 906 /** 907 * Constructor 908 */ 909 public TestReportSetupComponent() { 910 super(); 911 } 912 913 /** 914 * @return {@link #action} (Action would contain either an operation or an assertion.) 915 */ 916 public List<SetupActionComponent> getAction() { 917 if (this.action == null) 918 this.action = new ArrayList<SetupActionComponent>(); 919 return this.action; 920 } 921 922 /** 923 * @return Returns a reference to <code>this</code> for easy method chaining 924 */ 925 public TestReportSetupComponent setAction(List<SetupActionComponent> theAction) { 926 this.action = theAction; 927 return this; 928 } 929 930 public boolean hasAction() { 931 if (this.action == null) 932 return false; 933 for (SetupActionComponent item : this.action) 934 if (!item.isEmpty()) 935 return true; 936 return false; 937 } 938 939 public SetupActionComponent addAction() { //3 940 SetupActionComponent t = new SetupActionComponent(); 941 if (this.action == null) 942 this.action = new ArrayList<SetupActionComponent>(); 943 this.action.add(t); 944 return t; 945 } 946 947 public TestReportSetupComponent addAction(SetupActionComponent t) { //3 948 if (t == null) 949 return this; 950 if (this.action == null) 951 this.action = new ArrayList<SetupActionComponent>(); 952 this.action.add(t); 953 return this; 954 } 955 956 /** 957 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 958 */ 959 public SetupActionComponent getActionFirstRep() { 960 if (getAction().isEmpty()) { 961 addAction(); 962 } 963 return getAction().get(0); 964 } 965 966 protected void listChildren(List<Property> children) { 967 super.listChildren(children); 968 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action)); 969 } 970 971 @Override 972 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 973 switch (_hash) { 974 case -1422950858: /*action*/ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action); 975 default: return super.getNamedProperty(_hash, _name, _checkValid); 976 } 977 978 } 979 980 @Override 981 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 982 switch (hash) { 983 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // SetupActionComponent 984 default: return super.getProperty(hash, name, checkValid); 985 } 986 987 } 988 989 @Override 990 public Base setProperty(int hash, String name, Base value) throws FHIRException { 991 switch (hash) { 992 case -1422950858: // action 993 this.getAction().add((SetupActionComponent) value); // SetupActionComponent 994 return value; 995 default: return super.setProperty(hash, name, value); 996 } 997 998 } 999 1000 @Override 1001 public Base setProperty(String name, Base value) throws FHIRException { 1002 if (name.equals("action")) { 1003 this.getAction().add((SetupActionComponent) value); 1004 } else 1005 return super.setProperty(name, value); 1006 return value; 1007 } 1008 1009 @Override 1010 public Base makeProperty(int hash, String name) throws FHIRException { 1011 switch (hash) { 1012 case -1422950858: return addAction(); 1013 default: return super.makeProperty(hash, name); 1014 } 1015 1016 } 1017 1018 @Override 1019 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1020 switch (hash) { 1021 case -1422950858: /*action*/ return new String[] {}; 1022 default: return super.getTypesForProperty(hash, name); 1023 } 1024 1025 } 1026 1027 @Override 1028 public Base addChild(String name) throws FHIRException { 1029 if (name.equals("action")) { 1030 return addAction(); 1031 } 1032 else 1033 return super.addChild(name); 1034 } 1035 1036 public TestReportSetupComponent copy() { 1037 TestReportSetupComponent dst = new TestReportSetupComponent(); 1038 copyValues(dst); 1039 if (action != null) { 1040 dst.action = new ArrayList<SetupActionComponent>(); 1041 for (SetupActionComponent i : action) 1042 dst.action.add(i.copy()); 1043 }; 1044 return dst; 1045 } 1046 1047 @Override 1048 public boolean equalsDeep(Base other_) { 1049 if (!super.equalsDeep(other_)) 1050 return false; 1051 if (!(other_ instanceof TestReportSetupComponent)) 1052 return false; 1053 TestReportSetupComponent o = (TestReportSetupComponent) other_; 1054 return compareDeep(action, o.action, true); 1055 } 1056 1057 @Override 1058 public boolean equalsShallow(Base other_) { 1059 if (!super.equalsShallow(other_)) 1060 return false; 1061 if (!(other_ instanceof TestReportSetupComponent)) 1062 return false; 1063 TestReportSetupComponent o = (TestReportSetupComponent) other_; 1064 return true; 1065 } 1066 1067 public boolean isEmpty() { 1068 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 1069 } 1070 1071 public String fhirType() { 1072 return "TestReport.setup"; 1073 1074 } 1075 1076 } 1077 1078 @Block() 1079 public static class SetupActionComponent extends BackboneElement implements IBaseBackboneElement { 1080 /** 1081 * The operation performed. 1082 */ 1083 @Child(name = "operation", type = {}, order=1, min=0, max=1, modifier=false, summary=false) 1084 @Description(shortDefinition="The operation to perform", formalDefinition="The operation performed." ) 1085 protected SetupActionOperationComponent operation; 1086 1087 /** 1088 * The results of the assertion performed on the previous operations. 1089 */ 1090 @Child(name = "assert", type = {}, order=2, min=0, max=1, modifier=false, summary=false) 1091 @Description(shortDefinition="The assertion to perform", formalDefinition="The results of the assertion performed on the previous operations." ) 1092 protected SetupActionAssertComponent assert_; 1093 1094 private static final long serialVersionUID = -252088305L; 1095 1096 /** 1097 * Constructor 1098 */ 1099 public SetupActionComponent() { 1100 super(); 1101 } 1102 1103 /** 1104 * @return {@link #operation} (The operation performed.) 1105 */ 1106 public SetupActionOperationComponent getOperation() { 1107 if (this.operation == null) 1108 if (Configuration.errorOnAutoCreate()) 1109 throw new Error("Attempt to auto-create SetupActionComponent.operation"); 1110 else if (Configuration.doAutoCreate()) 1111 this.operation = new SetupActionOperationComponent(); // cc 1112 return this.operation; 1113 } 1114 1115 public boolean hasOperation() { 1116 return this.operation != null && !this.operation.isEmpty(); 1117 } 1118 1119 /** 1120 * @param value {@link #operation} (The operation performed.) 1121 */ 1122 public SetupActionComponent setOperation(SetupActionOperationComponent value) { 1123 this.operation = value; 1124 return this; 1125 } 1126 1127 /** 1128 * @return {@link #assert_} (The results of the assertion performed on the previous operations.) 1129 */ 1130 public SetupActionAssertComponent getAssert() { 1131 if (this.assert_ == null) 1132 if (Configuration.errorOnAutoCreate()) 1133 throw new Error("Attempt to auto-create SetupActionComponent.assert_"); 1134 else if (Configuration.doAutoCreate()) 1135 this.assert_ = new SetupActionAssertComponent(); // cc 1136 return this.assert_; 1137 } 1138 1139 public boolean hasAssert() { 1140 return this.assert_ != null && !this.assert_.isEmpty(); 1141 } 1142 1143 /** 1144 * @param value {@link #assert_} (The results of the assertion performed on the previous operations.) 1145 */ 1146 public SetupActionComponent setAssert(SetupActionAssertComponent value) { 1147 this.assert_ = value; 1148 return this; 1149 } 1150 1151 protected void listChildren(List<Property> children) { 1152 super.listChildren(children); 1153 children.add(new Property("operation", "", "The operation performed.", 0, 1, operation)); 1154 children.add(new Property("assert", "", "The results of the assertion performed on the previous operations.", 0, 1, assert_)); 1155 } 1156 1157 @Override 1158 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1159 switch (_hash) { 1160 case 1662702951: /*operation*/ return new Property("operation", "", "The operation performed.", 0, 1, operation); 1161 case -1408208058: /*assert*/ return new Property("assert", "", "The results of the assertion performed on the previous operations.", 0, 1, assert_); 1162 default: return super.getNamedProperty(_hash, _name, _checkValid); 1163 } 1164 1165 } 1166 1167 @Override 1168 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1169 switch (hash) { 1170 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 1171 case -1408208058: /*assert*/ return this.assert_ == null ? new Base[0] : new Base[] {this.assert_}; // SetupActionAssertComponent 1172 default: return super.getProperty(hash, name, checkValid); 1173 } 1174 1175 } 1176 1177 @Override 1178 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1179 switch (hash) { 1180 case 1662702951: // operation 1181 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1182 return value; 1183 case -1408208058: // assert 1184 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1185 return value; 1186 default: return super.setProperty(hash, name, value); 1187 } 1188 1189 } 1190 1191 @Override 1192 public Base setProperty(String name, Base value) throws FHIRException { 1193 if (name.equals("operation")) { 1194 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1195 } else if (name.equals("assert")) { 1196 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1197 } else 1198 return super.setProperty(name, value); 1199 return value; 1200 } 1201 1202 @Override 1203 public Base makeProperty(int hash, String name) throws FHIRException { 1204 switch (hash) { 1205 case 1662702951: return getOperation(); 1206 case -1408208058: return getAssert(); 1207 default: return super.makeProperty(hash, name); 1208 } 1209 1210 } 1211 1212 @Override 1213 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1214 switch (hash) { 1215 case 1662702951: /*operation*/ return new String[] {}; 1216 case -1408208058: /*assert*/ return new String[] {}; 1217 default: return super.getTypesForProperty(hash, name); 1218 } 1219 1220 } 1221 1222 @Override 1223 public Base addChild(String name) throws FHIRException { 1224 if (name.equals("operation")) { 1225 this.operation = new SetupActionOperationComponent(); 1226 return this.operation; 1227 } 1228 else if (name.equals("assert")) { 1229 this.assert_ = new SetupActionAssertComponent(); 1230 return this.assert_; 1231 } 1232 else 1233 return super.addChild(name); 1234 } 1235 1236 public SetupActionComponent copy() { 1237 SetupActionComponent dst = new SetupActionComponent(); 1238 copyValues(dst); 1239 dst.operation = operation == null ? null : operation.copy(); 1240 dst.assert_ = assert_ == null ? null : assert_.copy(); 1241 return dst; 1242 } 1243 1244 @Override 1245 public boolean equalsDeep(Base other_) { 1246 if (!super.equalsDeep(other_)) 1247 return false; 1248 if (!(other_ instanceof SetupActionComponent)) 1249 return false; 1250 SetupActionComponent o = (SetupActionComponent) other_; 1251 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 1252 } 1253 1254 @Override 1255 public boolean equalsShallow(Base other_) { 1256 if (!super.equalsShallow(other_)) 1257 return false; 1258 if (!(other_ instanceof SetupActionComponent)) 1259 return false; 1260 SetupActionComponent o = (SetupActionComponent) other_; 1261 return true; 1262 } 1263 1264 public boolean isEmpty() { 1265 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 1266 } 1267 1268 public String fhirType() { 1269 return "TestReport.setup.action"; 1270 1271 } 1272 1273 } 1274 1275 @Block() 1276 public static class SetupActionOperationComponent extends BackboneElement implements IBaseBackboneElement { 1277 /** 1278 * The result of this operation. 1279 */ 1280 @Child(name = "result", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1281 @Description(shortDefinition="pass | skip | fail | warning | error", formalDefinition="The result of this operation." ) 1282 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-action-result-codes") 1283 protected Enumeration<TestReportActionResult> result; 1284 1285 /** 1286 * An explanatory message associated with the result. 1287 */ 1288 @Child(name = "message", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1289 @Description(shortDefinition="A message associated with the result", formalDefinition="An explanatory message associated with the result." ) 1290 protected MarkdownType message; 1291 1292 /** 1293 * A link to further details on the result. 1294 */ 1295 @Child(name = "detail", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1296 @Description(shortDefinition="A link to further details on the result", formalDefinition="A link to further details on the result." ) 1297 protected UriType detail; 1298 1299 private static final long serialVersionUID = 269088798L; 1300 1301 /** 1302 * Constructor 1303 */ 1304 public SetupActionOperationComponent() { 1305 super(); 1306 } 1307 1308 /** 1309 * Constructor 1310 */ 1311 public SetupActionOperationComponent(Enumeration<TestReportActionResult> result) { 1312 super(); 1313 this.result = result; 1314 } 1315 1316 /** 1317 * @return {@link #result} (The result of this operation.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1318 */ 1319 public Enumeration<TestReportActionResult> getResultElement() { 1320 if (this.result == null) 1321 if (Configuration.errorOnAutoCreate()) 1322 throw new Error("Attempt to auto-create SetupActionOperationComponent.result"); 1323 else if (Configuration.doAutoCreate()) 1324 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); // bb 1325 return this.result; 1326 } 1327 1328 public boolean hasResultElement() { 1329 return this.result != null && !this.result.isEmpty(); 1330 } 1331 1332 public boolean hasResult() { 1333 return this.result != null && !this.result.isEmpty(); 1334 } 1335 1336 /** 1337 * @param value {@link #result} (The result of this operation.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1338 */ 1339 public SetupActionOperationComponent setResultElement(Enumeration<TestReportActionResult> value) { 1340 this.result = value; 1341 return this; 1342 } 1343 1344 /** 1345 * @return The result of this operation. 1346 */ 1347 public TestReportActionResult getResult() { 1348 return this.result == null ? null : this.result.getValue(); 1349 } 1350 1351 /** 1352 * @param value The result of this operation. 1353 */ 1354 public SetupActionOperationComponent setResult(TestReportActionResult value) { 1355 if (this.result == null) 1356 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); 1357 this.result.setValue(value); 1358 return this; 1359 } 1360 1361 /** 1362 * @return {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1363 */ 1364 public MarkdownType getMessageElement() { 1365 if (this.message == null) 1366 if (Configuration.errorOnAutoCreate()) 1367 throw new Error("Attempt to auto-create SetupActionOperationComponent.message"); 1368 else if (Configuration.doAutoCreate()) 1369 this.message = new MarkdownType(); // bb 1370 return this.message; 1371 } 1372 1373 public boolean hasMessageElement() { 1374 return this.message != null && !this.message.isEmpty(); 1375 } 1376 1377 public boolean hasMessage() { 1378 return this.message != null && !this.message.isEmpty(); 1379 } 1380 1381 /** 1382 * @param value {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1383 */ 1384 public SetupActionOperationComponent setMessageElement(MarkdownType value) { 1385 this.message = value; 1386 return this; 1387 } 1388 1389 /** 1390 * @return An explanatory message associated with the result. 1391 */ 1392 public String getMessage() { 1393 return this.message == null ? null : this.message.getValue(); 1394 } 1395 1396 /** 1397 * @param value An explanatory message associated with the result. 1398 */ 1399 public SetupActionOperationComponent setMessage(String value) { 1400 if (value == null) 1401 this.message = null; 1402 else { 1403 if (this.message == null) 1404 this.message = new MarkdownType(); 1405 this.message.setValue(value); 1406 } 1407 return this; 1408 } 1409 1410 /** 1411 * @return {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1412 */ 1413 public UriType getDetailElement() { 1414 if (this.detail == null) 1415 if (Configuration.errorOnAutoCreate()) 1416 throw new Error("Attempt to auto-create SetupActionOperationComponent.detail"); 1417 else if (Configuration.doAutoCreate()) 1418 this.detail = new UriType(); // bb 1419 return this.detail; 1420 } 1421 1422 public boolean hasDetailElement() { 1423 return this.detail != null && !this.detail.isEmpty(); 1424 } 1425 1426 public boolean hasDetail() { 1427 return this.detail != null && !this.detail.isEmpty(); 1428 } 1429 1430 /** 1431 * @param value {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1432 */ 1433 public SetupActionOperationComponent setDetailElement(UriType value) { 1434 this.detail = value; 1435 return this; 1436 } 1437 1438 /** 1439 * @return A link to further details on the result. 1440 */ 1441 public String getDetail() { 1442 return this.detail == null ? null : this.detail.getValue(); 1443 } 1444 1445 /** 1446 * @param value A link to further details on the result. 1447 */ 1448 public SetupActionOperationComponent setDetail(String value) { 1449 if (Utilities.noString(value)) 1450 this.detail = null; 1451 else { 1452 if (this.detail == null) 1453 this.detail = new UriType(); 1454 this.detail.setValue(value); 1455 } 1456 return this; 1457 } 1458 1459 protected void listChildren(List<Property> children) { 1460 super.listChildren(children); 1461 children.add(new Property("result", "code", "The result of this operation.", 0, 1, result)); 1462 children.add(new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message)); 1463 children.add(new Property("detail", "uri", "A link to further details on the result.", 0, 1, detail)); 1464 } 1465 1466 @Override 1467 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1468 switch (_hash) { 1469 case -934426595: /*result*/ return new Property("result", "code", "The result of this operation.", 0, 1, result); 1470 case 954925063: /*message*/ return new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message); 1471 case -1335224239: /*detail*/ return new Property("detail", "uri", "A link to further details on the result.", 0, 1, detail); 1472 default: return super.getNamedProperty(_hash, _name, _checkValid); 1473 } 1474 1475 } 1476 1477 @Override 1478 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1479 switch (hash) { 1480 case -934426595: /*result*/ return this.result == null ? new Base[0] : new Base[] {this.result}; // Enumeration<TestReportActionResult> 1481 case 954925063: /*message*/ return this.message == null ? new Base[0] : new Base[] {this.message}; // MarkdownType 1482 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // UriType 1483 default: return super.getProperty(hash, name, checkValid); 1484 } 1485 1486 } 1487 1488 @Override 1489 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1490 switch (hash) { 1491 case -934426595: // result 1492 value = new TestReportActionResultEnumFactory().fromType(castToCode(value)); 1493 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1494 return value; 1495 case 954925063: // message 1496 this.message = castToMarkdown(value); // MarkdownType 1497 return value; 1498 case -1335224239: // detail 1499 this.detail = castToUri(value); // UriType 1500 return value; 1501 default: return super.setProperty(hash, name, value); 1502 } 1503 1504 } 1505 1506 @Override 1507 public Base setProperty(String name, Base value) throws FHIRException { 1508 if (name.equals("result")) { 1509 value = new TestReportActionResultEnumFactory().fromType(castToCode(value)); 1510 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1511 } else if (name.equals("message")) { 1512 this.message = castToMarkdown(value); // MarkdownType 1513 } else if (name.equals("detail")) { 1514 this.detail = castToUri(value); // UriType 1515 } else 1516 return super.setProperty(name, value); 1517 return value; 1518 } 1519 1520 @Override 1521 public Base makeProperty(int hash, String name) throws FHIRException { 1522 switch (hash) { 1523 case -934426595: return getResultElement(); 1524 case 954925063: return getMessageElement(); 1525 case -1335224239: return getDetailElement(); 1526 default: return super.makeProperty(hash, name); 1527 } 1528 1529 } 1530 1531 @Override 1532 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1533 switch (hash) { 1534 case -934426595: /*result*/ return new String[] {"code"}; 1535 case 954925063: /*message*/ return new String[] {"markdown"}; 1536 case -1335224239: /*detail*/ return new String[] {"uri"}; 1537 default: return super.getTypesForProperty(hash, name); 1538 } 1539 1540 } 1541 1542 @Override 1543 public Base addChild(String name) throws FHIRException { 1544 if (name.equals("result")) { 1545 throw new FHIRException("Cannot call addChild on a singleton property TestReport.result"); 1546 } 1547 else if (name.equals("message")) { 1548 throw new FHIRException("Cannot call addChild on a singleton property TestReport.message"); 1549 } 1550 else if (name.equals("detail")) { 1551 throw new FHIRException("Cannot call addChild on a singleton property TestReport.detail"); 1552 } 1553 else 1554 return super.addChild(name); 1555 } 1556 1557 public SetupActionOperationComponent copy() { 1558 SetupActionOperationComponent dst = new SetupActionOperationComponent(); 1559 copyValues(dst); 1560 dst.result = result == null ? null : result.copy(); 1561 dst.message = message == null ? null : message.copy(); 1562 dst.detail = detail == null ? null : detail.copy(); 1563 return dst; 1564 } 1565 1566 @Override 1567 public boolean equalsDeep(Base other_) { 1568 if (!super.equalsDeep(other_)) 1569 return false; 1570 if (!(other_ instanceof SetupActionOperationComponent)) 1571 return false; 1572 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 1573 return compareDeep(result, o.result, true) && compareDeep(message, o.message, true) && compareDeep(detail, o.detail, true) 1574 ; 1575 } 1576 1577 @Override 1578 public boolean equalsShallow(Base other_) { 1579 if (!super.equalsShallow(other_)) 1580 return false; 1581 if (!(other_ instanceof SetupActionOperationComponent)) 1582 return false; 1583 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 1584 return compareValues(result, o.result, true) && compareValues(message, o.message, true) && compareValues(detail, o.detail, true) 1585 ; 1586 } 1587 1588 public boolean isEmpty() { 1589 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(result, message, detail 1590 ); 1591 } 1592 1593 public String fhirType() { 1594 return "TestReport.setup.action.operation"; 1595 1596 } 1597 1598 } 1599 1600 @Block() 1601 public static class SetupActionAssertComponent extends BackboneElement implements IBaseBackboneElement { 1602 /** 1603 * The result of this assertion. 1604 */ 1605 @Child(name = "result", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1606 @Description(shortDefinition="pass | skip | fail | warning | error", formalDefinition="The result of this assertion." ) 1607 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-action-result-codes") 1608 protected Enumeration<TestReportActionResult> result; 1609 1610 /** 1611 * An explanatory message associated with the result. 1612 */ 1613 @Child(name = "message", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1614 @Description(shortDefinition="A message associated with the result", formalDefinition="An explanatory message associated with the result." ) 1615 protected MarkdownType message; 1616 1617 /** 1618 * A link to further details on the result. 1619 */ 1620 @Child(name = "detail", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1621 @Description(shortDefinition="A link to further details on the result", formalDefinition="A link to further details on the result." ) 1622 protected StringType detail; 1623 1624 private static final long serialVersionUID = 467968193L; 1625 1626 /** 1627 * Constructor 1628 */ 1629 public SetupActionAssertComponent() { 1630 super(); 1631 } 1632 1633 /** 1634 * Constructor 1635 */ 1636 public SetupActionAssertComponent(Enumeration<TestReportActionResult> result) { 1637 super(); 1638 this.result = result; 1639 } 1640 1641 /** 1642 * @return {@link #result} (The result of this assertion.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1643 */ 1644 public Enumeration<TestReportActionResult> getResultElement() { 1645 if (this.result == null) 1646 if (Configuration.errorOnAutoCreate()) 1647 throw new Error("Attempt to auto-create SetupActionAssertComponent.result"); 1648 else if (Configuration.doAutoCreate()) 1649 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); // bb 1650 return this.result; 1651 } 1652 1653 public boolean hasResultElement() { 1654 return this.result != null && !this.result.isEmpty(); 1655 } 1656 1657 public boolean hasResult() { 1658 return this.result != null && !this.result.isEmpty(); 1659 } 1660 1661 /** 1662 * @param value {@link #result} (The result of this assertion.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 1663 */ 1664 public SetupActionAssertComponent setResultElement(Enumeration<TestReportActionResult> value) { 1665 this.result = value; 1666 return this; 1667 } 1668 1669 /** 1670 * @return The result of this assertion. 1671 */ 1672 public TestReportActionResult getResult() { 1673 return this.result == null ? null : this.result.getValue(); 1674 } 1675 1676 /** 1677 * @param value The result of this assertion. 1678 */ 1679 public SetupActionAssertComponent setResult(TestReportActionResult value) { 1680 if (this.result == null) 1681 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); 1682 this.result.setValue(value); 1683 return this; 1684 } 1685 1686 /** 1687 * @return {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1688 */ 1689 public MarkdownType getMessageElement() { 1690 if (this.message == null) 1691 if (Configuration.errorOnAutoCreate()) 1692 throw new Error("Attempt to auto-create SetupActionAssertComponent.message"); 1693 else if (Configuration.doAutoCreate()) 1694 this.message = new MarkdownType(); // bb 1695 return this.message; 1696 } 1697 1698 public boolean hasMessageElement() { 1699 return this.message != null && !this.message.isEmpty(); 1700 } 1701 1702 public boolean hasMessage() { 1703 return this.message != null && !this.message.isEmpty(); 1704 } 1705 1706 /** 1707 * @param value {@link #message} (An explanatory message associated with the result.). This is the underlying object with id, value and extensions. The accessor "getMessage" gives direct access to the value 1708 */ 1709 public SetupActionAssertComponent setMessageElement(MarkdownType value) { 1710 this.message = value; 1711 return this; 1712 } 1713 1714 /** 1715 * @return An explanatory message associated with the result. 1716 */ 1717 public String getMessage() { 1718 return this.message == null ? null : this.message.getValue(); 1719 } 1720 1721 /** 1722 * @param value An explanatory message associated with the result. 1723 */ 1724 public SetupActionAssertComponent setMessage(String value) { 1725 if (value == null) 1726 this.message = null; 1727 else { 1728 if (this.message == null) 1729 this.message = new MarkdownType(); 1730 this.message.setValue(value); 1731 } 1732 return this; 1733 } 1734 1735 /** 1736 * @return {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1737 */ 1738 public StringType getDetailElement() { 1739 if (this.detail == null) 1740 if (Configuration.errorOnAutoCreate()) 1741 throw new Error("Attempt to auto-create SetupActionAssertComponent.detail"); 1742 else if (Configuration.doAutoCreate()) 1743 this.detail = new StringType(); // bb 1744 return this.detail; 1745 } 1746 1747 public boolean hasDetailElement() { 1748 return this.detail != null && !this.detail.isEmpty(); 1749 } 1750 1751 public boolean hasDetail() { 1752 return this.detail != null && !this.detail.isEmpty(); 1753 } 1754 1755 /** 1756 * @param value {@link #detail} (A link to further details on the result.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value 1757 */ 1758 public SetupActionAssertComponent setDetailElement(StringType value) { 1759 this.detail = value; 1760 return this; 1761 } 1762 1763 /** 1764 * @return A link to further details on the result. 1765 */ 1766 public String getDetail() { 1767 return this.detail == null ? null : this.detail.getValue(); 1768 } 1769 1770 /** 1771 * @param value A link to further details on the result. 1772 */ 1773 public SetupActionAssertComponent setDetail(String value) { 1774 if (Utilities.noString(value)) 1775 this.detail = null; 1776 else { 1777 if (this.detail == null) 1778 this.detail = new StringType(); 1779 this.detail.setValue(value); 1780 } 1781 return this; 1782 } 1783 1784 protected void listChildren(List<Property> children) { 1785 super.listChildren(children); 1786 children.add(new Property("result", "code", "The result of this assertion.", 0, 1, result)); 1787 children.add(new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message)); 1788 children.add(new Property("detail", "string", "A link to further details on the result.", 0, 1, detail)); 1789 } 1790 1791 @Override 1792 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1793 switch (_hash) { 1794 case -934426595: /*result*/ return new Property("result", "code", "The result of this assertion.", 0, 1, result); 1795 case 954925063: /*message*/ return new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message); 1796 case -1335224239: /*detail*/ return new Property("detail", "string", "A link to further details on the result.", 0, 1, detail); 1797 default: return super.getNamedProperty(_hash, _name, _checkValid); 1798 } 1799 1800 } 1801 1802 @Override 1803 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1804 switch (hash) { 1805 case -934426595: /*result*/ return this.result == null ? new Base[0] : new Base[] {this.result}; // Enumeration<TestReportActionResult> 1806 case 954925063: /*message*/ return this.message == null ? new Base[0] : new Base[] {this.message}; // MarkdownType 1807 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // StringType 1808 default: return super.getProperty(hash, name, checkValid); 1809 } 1810 1811 } 1812 1813 @Override 1814 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1815 switch (hash) { 1816 case -934426595: // result 1817 value = new TestReportActionResultEnumFactory().fromType(castToCode(value)); 1818 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1819 return value; 1820 case 954925063: // message 1821 this.message = castToMarkdown(value); // MarkdownType 1822 return value; 1823 case -1335224239: // detail 1824 this.detail = castToString(value); // StringType 1825 return value; 1826 default: return super.setProperty(hash, name, value); 1827 } 1828 1829 } 1830 1831 @Override 1832 public Base setProperty(String name, Base value) throws FHIRException { 1833 if (name.equals("result")) { 1834 value = new TestReportActionResultEnumFactory().fromType(castToCode(value)); 1835 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1836 } else if (name.equals("message")) { 1837 this.message = castToMarkdown(value); // MarkdownType 1838 } else if (name.equals("detail")) { 1839 this.detail = castToString(value); // StringType 1840 } else 1841 return super.setProperty(name, value); 1842 return value; 1843 } 1844 1845 @Override 1846 public Base makeProperty(int hash, String name) throws FHIRException { 1847 switch (hash) { 1848 case -934426595: return getResultElement(); 1849 case 954925063: return getMessageElement(); 1850 case -1335224239: return getDetailElement(); 1851 default: return super.makeProperty(hash, name); 1852 } 1853 1854 } 1855 1856 @Override 1857 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1858 switch (hash) { 1859 case -934426595: /*result*/ return new String[] {"code"}; 1860 case 954925063: /*message*/ return new String[] {"markdown"}; 1861 case -1335224239: /*detail*/ return new String[] {"string"}; 1862 default: return super.getTypesForProperty(hash, name); 1863 } 1864 1865 } 1866 1867 @Override 1868 public Base addChild(String name) throws FHIRException { 1869 if (name.equals("result")) { 1870 throw new FHIRException("Cannot call addChild on a singleton property TestReport.result"); 1871 } 1872 else if (name.equals("message")) { 1873 throw new FHIRException("Cannot call addChild on a singleton property TestReport.message"); 1874 } 1875 else if (name.equals("detail")) { 1876 throw new FHIRException("Cannot call addChild on a singleton property TestReport.detail"); 1877 } 1878 else 1879 return super.addChild(name); 1880 } 1881 1882 public SetupActionAssertComponent copy() { 1883 SetupActionAssertComponent dst = new SetupActionAssertComponent(); 1884 copyValues(dst); 1885 dst.result = result == null ? null : result.copy(); 1886 dst.message = message == null ? null : message.copy(); 1887 dst.detail = detail == null ? null : detail.copy(); 1888 return dst; 1889 } 1890 1891 @Override 1892 public boolean equalsDeep(Base other_) { 1893 if (!super.equalsDeep(other_)) 1894 return false; 1895 if (!(other_ instanceof SetupActionAssertComponent)) 1896 return false; 1897 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 1898 return compareDeep(result, o.result, true) && compareDeep(message, o.message, true) && compareDeep(detail, o.detail, true) 1899 ; 1900 } 1901 1902 @Override 1903 public boolean equalsShallow(Base other_) { 1904 if (!super.equalsShallow(other_)) 1905 return false; 1906 if (!(other_ instanceof SetupActionAssertComponent)) 1907 return false; 1908 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 1909 return compareValues(result, o.result, true) && compareValues(message, o.message, true) && compareValues(detail, o.detail, true) 1910 ; 1911 } 1912 1913 public boolean isEmpty() { 1914 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(result, message, detail 1915 ); 1916 } 1917 1918 public String fhirType() { 1919 return "TestReport.setup.action.assert"; 1920 1921 } 1922 1923 } 1924 1925 @Block() 1926 public static class TestReportTestComponent extends BackboneElement implements IBaseBackboneElement { 1927 /** 1928 * The name of this test used for tracking/logging purposes by test engines. 1929 */ 1930 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1931 @Description(shortDefinition="Tracking/logging name of this test", formalDefinition="The name of this test used for tracking/logging purposes by test engines." ) 1932 protected StringType name; 1933 1934 /** 1935 * A short description of the test used by test engines for tracking and reporting purposes. 1936 */ 1937 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1938 @Description(shortDefinition="Tracking/reporting short description of the test", formalDefinition="A short description of the test used by test engines for tracking and reporting purposes." ) 1939 protected StringType description; 1940 1941 /** 1942 * Action would contain either an operation or an assertion. 1943 */ 1944 @Child(name = "action", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1945 @Description(shortDefinition="A test operation or assert that was performed", formalDefinition="Action would contain either an operation or an assertion." ) 1946 protected List<TestActionComponent> action; 1947 1948 private static final long serialVersionUID = -865006110L; 1949 1950 /** 1951 * Constructor 1952 */ 1953 public TestReportTestComponent() { 1954 super(); 1955 } 1956 1957 /** 1958 * @return {@link #name} (The name of this test used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1959 */ 1960 public StringType getNameElement() { 1961 if (this.name == null) 1962 if (Configuration.errorOnAutoCreate()) 1963 throw new Error("Attempt to auto-create TestReportTestComponent.name"); 1964 else if (Configuration.doAutoCreate()) 1965 this.name = new StringType(); // bb 1966 return this.name; 1967 } 1968 1969 public boolean hasNameElement() { 1970 return this.name != null && !this.name.isEmpty(); 1971 } 1972 1973 public boolean hasName() { 1974 return this.name != null && !this.name.isEmpty(); 1975 } 1976 1977 /** 1978 * @param value {@link #name} (The name of this test used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1979 */ 1980 public TestReportTestComponent setNameElement(StringType value) { 1981 this.name = value; 1982 return this; 1983 } 1984 1985 /** 1986 * @return The name of this test used for tracking/logging purposes by test engines. 1987 */ 1988 public String getName() { 1989 return this.name == null ? null : this.name.getValue(); 1990 } 1991 1992 /** 1993 * @param value The name of this test used for tracking/logging purposes by test engines. 1994 */ 1995 public TestReportTestComponent setName(String value) { 1996 if (Utilities.noString(value)) 1997 this.name = null; 1998 else { 1999 if (this.name == null) 2000 this.name = new StringType(); 2001 this.name.setValue(value); 2002 } 2003 return this; 2004 } 2005 2006 /** 2007 * @return {@link #description} (A short description of the test used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2008 */ 2009 public StringType getDescriptionElement() { 2010 if (this.description == null) 2011 if (Configuration.errorOnAutoCreate()) 2012 throw new Error("Attempt to auto-create TestReportTestComponent.description"); 2013 else if (Configuration.doAutoCreate()) 2014 this.description = new StringType(); // bb 2015 return this.description; 2016 } 2017 2018 public boolean hasDescriptionElement() { 2019 return this.description != null && !this.description.isEmpty(); 2020 } 2021 2022 public boolean hasDescription() { 2023 return this.description != null && !this.description.isEmpty(); 2024 } 2025 2026 /** 2027 * @param value {@link #description} (A short description of the test used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2028 */ 2029 public TestReportTestComponent setDescriptionElement(StringType value) { 2030 this.description = value; 2031 return this; 2032 } 2033 2034 /** 2035 * @return A short description of the test used by test engines for tracking and reporting purposes. 2036 */ 2037 public String getDescription() { 2038 return this.description == null ? null : this.description.getValue(); 2039 } 2040 2041 /** 2042 * @param value A short description of the test used by test engines for tracking and reporting purposes. 2043 */ 2044 public TestReportTestComponent setDescription(String value) { 2045 if (Utilities.noString(value)) 2046 this.description = null; 2047 else { 2048 if (this.description == null) 2049 this.description = new StringType(); 2050 this.description.setValue(value); 2051 } 2052 return this; 2053 } 2054 2055 /** 2056 * @return {@link #action} (Action would contain either an operation or an assertion.) 2057 */ 2058 public List<TestActionComponent> getAction() { 2059 if (this.action == null) 2060 this.action = new ArrayList<TestActionComponent>(); 2061 return this.action; 2062 } 2063 2064 /** 2065 * @return Returns a reference to <code>this</code> for easy method chaining 2066 */ 2067 public TestReportTestComponent setAction(List<TestActionComponent> theAction) { 2068 this.action = theAction; 2069 return this; 2070 } 2071 2072 public boolean hasAction() { 2073 if (this.action == null) 2074 return false; 2075 for (TestActionComponent item : this.action) 2076 if (!item.isEmpty()) 2077 return true; 2078 return false; 2079 } 2080 2081 public TestActionComponent addAction() { //3 2082 TestActionComponent t = new TestActionComponent(); 2083 if (this.action == null) 2084 this.action = new ArrayList<TestActionComponent>(); 2085 this.action.add(t); 2086 return t; 2087 } 2088 2089 public TestReportTestComponent addAction(TestActionComponent t) { //3 2090 if (t == null) 2091 return this; 2092 if (this.action == null) 2093 this.action = new ArrayList<TestActionComponent>(); 2094 this.action.add(t); 2095 return this; 2096 } 2097 2098 /** 2099 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 2100 */ 2101 public TestActionComponent getActionFirstRep() { 2102 if (getAction().isEmpty()) { 2103 addAction(); 2104 } 2105 return getAction().get(0); 2106 } 2107 2108 protected void listChildren(List<Property> children) { 2109 super.listChildren(children); 2110 children.add(new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name)); 2111 children.add(new Property("description", "string", "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, description)); 2112 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action)); 2113 } 2114 2115 @Override 2116 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2117 switch (_hash) { 2118 case 3373707: /*name*/ return new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name); 2119 case -1724546052: /*description*/ return new Property("description", "string", "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, description); 2120 case -1422950858: /*action*/ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action); 2121 default: return super.getNamedProperty(_hash, _name, _checkValid); 2122 } 2123 2124 } 2125 2126 @Override 2127 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2128 switch (hash) { 2129 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2130 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2131 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TestActionComponent 2132 default: return super.getProperty(hash, name, checkValid); 2133 } 2134 2135 } 2136 2137 @Override 2138 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2139 switch (hash) { 2140 case 3373707: // name 2141 this.name = castToString(value); // StringType 2142 return value; 2143 case -1724546052: // description 2144 this.description = castToString(value); // StringType 2145 return value; 2146 case -1422950858: // action 2147 this.getAction().add((TestActionComponent) value); // TestActionComponent 2148 return value; 2149 default: return super.setProperty(hash, name, value); 2150 } 2151 2152 } 2153 2154 @Override 2155 public Base setProperty(String name, Base value) throws FHIRException { 2156 if (name.equals("name")) { 2157 this.name = castToString(value); // StringType 2158 } else if (name.equals("description")) { 2159 this.description = castToString(value); // StringType 2160 } else if (name.equals("action")) { 2161 this.getAction().add((TestActionComponent) value); 2162 } else 2163 return super.setProperty(name, value); 2164 return value; 2165 } 2166 2167 @Override 2168 public Base makeProperty(int hash, String name) throws FHIRException { 2169 switch (hash) { 2170 case 3373707: return getNameElement(); 2171 case -1724546052: return getDescriptionElement(); 2172 case -1422950858: return addAction(); 2173 default: return super.makeProperty(hash, name); 2174 } 2175 2176 } 2177 2178 @Override 2179 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2180 switch (hash) { 2181 case 3373707: /*name*/ return new String[] {"string"}; 2182 case -1724546052: /*description*/ return new String[] {"string"}; 2183 case -1422950858: /*action*/ return new String[] {}; 2184 default: return super.getTypesForProperty(hash, name); 2185 } 2186 2187 } 2188 2189 @Override 2190 public Base addChild(String name) throws FHIRException { 2191 if (name.equals("name")) { 2192 throw new FHIRException("Cannot call addChild on a singleton property TestReport.name"); 2193 } 2194 else if (name.equals("description")) { 2195 throw new FHIRException("Cannot call addChild on a singleton property TestReport.description"); 2196 } 2197 else if (name.equals("action")) { 2198 return addAction(); 2199 } 2200 else 2201 return super.addChild(name); 2202 } 2203 2204 public TestReportTestComponent copy() { 2205 TestReportTestComponent dst = new TestReportTestComponent(); 2206 copyValues(dst); 2207 dst.name = name == null ? null : name.copy(); 2208 dst.description = description == null ? null : description.copy(); 2209 if (action != null) { 2210 dst.action = new ArrayList<TestActionComponent>(); 2211 for (TestActionComponent i : action) 2212 dst.action.add(i.copy()); 2213 }; 2214 return dst; 2215 } 2216 2217 @Override 2218 public boolean equalsDeep(Base other_) { 2219 if (!super.equalsDeep(other_)) 2220 return false; 2221 if (!(other_ instanceof TestReportTestComponent)) 2222 return false; 2223 TestReportTestComponent o = (TestReportTestComponent) other_; 2224 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(action, o.action, true) 2225 ; 2226 } 2227 2228 @Override 2229 public boolean equalsShallow(Base other_) { 2230 if (!super.equalsShallow(other_)) 2231 return false; 2232 if (!(other_ instanceof TestReportTestComponent)) 2233 return false; 2234 TestReportTestComponent o = (TestReportTestComponent) other_; 2235 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 2236 } 2237 2238 public boolean isEmpty() { 2239 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description, action 2240 ); 2241 } 2242 2243 public String fhirType() { 2244 return "TestReport.test"; 2245 2246 } 2247 2248 } 2249 2250 @Block() 2251 public static class TestActionComponent extends BackboneElement implements IBaseBackboneElement { 2252 /** 2253 * An operation would involve a REST request to a server. 2254 */ 2255 @Child(name = "operation", type = {SetupActionOperationComponent.class}, order=1, min=0, max=1, modifier=false, summary=false) 2256 @Description(shortDefinition="The operation performed", formalDefinition="An operation would involve a REST request to a server." ) 2257 protected SetupActionOperationComponent operation; 2258 2259 /** 2260 * The results of the assertion performed on the previous operations. 2261 */ 2262 @Child(name = "assert", type = {SetupActionAssertComponent.class}, order=2, min=0, max=1, modifier=false, summary=false) 2263 @Description(shortDefinition="The assertion performed", formalDefinition="The results of the assertion performed on the previous operations." ) 2264 protected SetupActionAssertComponent assert_; 2265 2266 private static final long serialVersionUID = -252088305L; 2267 2268 /** 2269 * Constructor 2270 */ 2271 public TestActionComponent() { 2272 super(); 2273 } 2274 2275 /** 2276 * @return {@link #operation} (An operation would involve a REST request to a server.) 2277 */ 2278 public SetupActionOperationComponent getOperation() { 2279 if (this.operation == null) 2280 if (Configuration.errorOnAutoCreate()) 2281 throw new Error("Attempt to auto-create TestActionComponent.operation"); 2282 else if (Configuration.doAutoCreate()) 2283 this.operation = new SetupActionOperationComponent(); // cc 2284 return this.operation; 2285 } 2286 2287 public boolean hasOperation() { 2288 return this.operation != null && !this.operation.isEmpty(); 2289 } 2290 2291 /** 2292 * @param value {@link #operation} (An operation would involve a REST request to a server.) 2293 */ 2294 public TestActionComponent setOperation(SetupActionOperationComponent value) { 2295 this.operation = value; 2296 return this; 2297 } 2298 2299 /** 2300 * @return {@link #assert_} (The results of the assertion performed on the previous operations.) 2301 */ 2302 public SetupActionAssertComponent getAssert() { 2303 if (this.assert_ == null) 2304 if (Configuration.errorOnAutoCreate()) 2305 throw new Error("Attempt to auto-create TestActionComponent.assert_"); 2306 else if (Configuration.doAutoCreate()) 2307 this.assert_ = new SetupActionAssertComponent(); // cc 2308 return this.assert_; 2309 } 2310 2311 public boolean hasAssert() { 2312 return this.assert_ != null && !this.assert_.isEmpty(); 2313 } 2314 2315 /** 2316 * @param value {@link #assert_} (The results of the assertion performed on the previous operations.) 2317 */ 2318 public TestActionComponent setAssert(SetupActionAssertComponent value) { 2319 this.assert_ = value; 2320 return this; 2321 } 2322 2323 protected void listChildren(List<Property> children) { 2324 super.listChildren(children); 2325 children.add(new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation)); 2326 children.add(new Property("assert", "@TestReport.setup.action.assert", "The results of the assertion performed on the previous operations.", 0, 1, assert_)); 2327 } 2328 2329 @Override 2330 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2331 switch (_hash) { 2332 case 1662702951: /*operation*/ return new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation); 2333 case -1408208058: /*assert*/ return new Property("assert", "@TestReport.setup.action.assert", "The results of the assertion performed on the previous operations.", 0, 1, assert_); 2334 default: return super.getNamedProperty(_hash, _name, _checkValid); 2335 } 2336 2337 } 2338 2339 @Override 2340 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2341 switch (hash) { 2342 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 2343 case -1408208058: /*assert*/ return this.assert_ == null ? new Base[0] : new Base[] {this.assert_}; // SetupActionAssertComponent 2344 default: return super.getProperty(hash, name, checkValid); 2345 } 2346 2347 } 2348 2349 @Override 2350 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2351 switch (hash) { 2352 case 1662702951: // operation 2353 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2354 return value; 2355 case -1408208058: // assert 2356 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2357 return value; 2358 default: return super.setProperty(hash, name, value); 2359 } 2360 2361 } 2362 2363 @Override 2364 public Base setProperty(String name, Base value) throws FHIRException { 2365 if (name.equals("operation")) { 2366 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2367 } else if (name.equals("assert")) { 2368 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2369 } else 2370 return super.setProperty(name, value); 2371 return value; 2372 } 2373 2374 @Override 2375 public Base makeProperty(int hash, String name) throws FHIRException { 2376 switch (hash) { 2377 case 1662702951: return getOperation(); 2378 case -1408208058: return getAssert(); 2379 default: return super.makeProperty(hash, name); 2380 } 2381 2382 } 2383 2384 @Override 2385 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2386 switch (hash) { 2387 case 1662702951: /*operation*/ return new String[] {"@TestReport.setup.action.operation"}; 2388 case -1408208058: /*assert*/ return new String[] {"@TestReport.setup.action.assert"}; 2389 default: return super.getTypesForProperty(hash, name); 2390 } 2391 2392 } 2393 2394 @Override 2395 public Base addChild(String name) throws FHIRException { 2396 if (name.equals("operation")) { 2397 this.operation = new SetupActionOperationComponent(); 2398 return this.operation; 2399 } 2400 else if (name.equals("assert")) { 2401 this.assert_ = new SetupActionAssertComponent(); 2402 return this.assert_; 2403 } 2404 else 2405 return super.addChild(name); 2406 } 2407 2408 public TestActionComponent copy() { 2409 TestActionComponent dst = new TestActionComponent(); 2410 copyValues(dst); 2411 dst.operation = operation == null ? null : operation.copy(); 2412 dst.assert_ = assert_ == null ? null : assert_.copy(); 2413 return dst; 2414 } 2415 2416 @Override 2417 public boolean equalsDeep(Base other_) { 2418 if (!super.equalsDeep(other_)) 2419 return false; 2420 if (!(other_ instanceof TestActionComponent)) 2421 return false; 2422 TestActionComponent o = (TestActionComponent) other_; 2423 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 2424 } 2425 2426 @Override 2427 public boolean equalsShallow(Base other_) { 2428 if (!super.equalsShallow(other_)) 2429 return false; 2430 if (!(other_ instanceof TestActionComponent)) 2431 return false; 2432 TestActionComponent o = (TestActionComponent) other_; 2433 return true; 2434 } 2435 2436 public boolean isEmpty() { 2437 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 2438 } 2439 2440 public String fhirType() { 2441 return "TestReport.test.action"; 2442 2443 } 2444 2445 } 2446 2447 @Block() 2448 public static class TestReportTeardownComponent extends BackboneElement implements IBaseBackboneElement { 2449 /** 2450 * The teardown action will only contain an operation. 2451 */ 2452 @Child(name = "action", type = {}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2453 @Description(shortDefinition="One or more teardown operations performed", formalDefinition="The teardown action will only contain an operation." ) 2454 protected List<TeardownActionComponent> action; 2455 2456 private static final long serialVersionUID = 1168638089L; 2457 2458 /** 2459 * Constructor 2460 */ 2461 public TestReportTeardownComponent() { 2462 super(); 2463 } 2464 2465 /** 2466 * @return {@link #action} (The teardown action will only contain an operation.) 2467 */ 2468 public List<TeardownActionComponent> getAction() { 2469 if (this.action == null) 2470 this.action = new ArrayList<TeardownActionComponent>(); 2471 return this.action; 2472 } 2473 2474 /** 2475 * @return Returns a reference to <code>this</code> for easy method chaining 2476 */ 2477 public TestReportTeardownComponent setAction(List<TeardownActionComponent> theAction) { 2478 this.action = theAction; 2479 return this; 2480 } 2481 2482 public boolean hasAction() { 2483 if (this.action == null) 2484 return false; 2485 for (TeardownActionComponent item : this.action) 2486 if (!item.isEmpty()) 2487 return true; 2488 return false; 2489 } 2490 2491 public TeardownActionComponent addAction() { //3 2492 TeardownActionComponent t = new TeardownActionComponent(); 2493 if (this.action == null) 2494 this.action = new ArrayList<TeardownActionComponent>(); 2495 this.action.add(t); 2496 return t; 2497 } 2498 2499 public TestReportTeardownComponent addAction(TeardownActionComponent t) { //3 2500 if (t == null) 2501 return this; 2502 if (this.action == null) 2503 this.action = new ArrayList<TeardownActionComponent>(); 2504 this.action.add(t); 2505 return this; 2506 } 2507 2508 /** 2509 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 2510 */ 2511 public TeardownActionComponent getActionFirstRep() { 2512 if (getAction().isEmpty()) { 2513 addAction(); 2514 } 2515 return getAction().get(0); 2516 } 2517 2518 protected void listChildren(List<Property> children) { 2519 super.listChildren(children); 2520 children.add(new Property("action", "", "The teardown action will only contain an operation.", 0, java.lang.Integer.MAX_VALUE, action)); 2521 } 2522 2523 @Override 2524 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2525 switch (_hash) { 2526 case -1422950858: /*action*/ return new Property("action", "", "The teardown action will only contain an operation.", 0, java.lang.Integer.MAX_VALUE, action); 2527 default: return super.getNamedProperty(_hash, _name, _checkValid); 2528 } 2529 2530 } 2531 2532 @Override 2533 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2534 switch (hash) { 2535 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TeardownActionComponent 2536 default: return super.getProperty(hash, name, checkValid); 2537 } 2538 2539 } 2540 2541 @Override 2542 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2543 switch (hash) { 2544 case -1422950858: // action 2545 this.getAction().add((TeardownActionComponent) value); // TeardownActionComponent 2546 return value; 2547 default: return super.setProperty(hash, name, value); 2548 } 2549 2550 } 2551 2552 @Override 2553 public Base setProperty(String name, Base value) throws FHIRException { 2554 if (name.equals("action")) { 2555 this.getAction().add((TeardownActionComponent) value); 2556 } else 2557 return super.setProperty(name, value); 2558 return value; 2559 } 2560 2561 @Override 2562 public Base makeProperty(int hash, String name) throws FHIRException { 2563 switch (hash) { 2564 case -1422950858: return addAction(); 2565 default: return super.makeProperty(hash, name); 2566 } 2567 2568 } 2569 2570 @Override 2571 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2572 switch (hash) { 2573 case -1422950858: /*action*/ return new String[] {}; 2574 default: return super.getTypesForProperty(hash, name); 2575 } 2576 2577 } 2578 2579 @Override 2580 public Base addChild(String name) throws FHIRException { 2581 if (name.equals("action")) { 2582 return addAction(); 2583 } 2584 else 2585 return super.addChild(name); 2586 } 2587 2588 public TestReportTeardownComponent copy() { 2589 TestReportTeardownComponent dst = new TestReportTeardownComponent(); 2590 copyValues(dst); 2591 if (action != null) { 2592 dst.action = new ArrayList<TeardownActionComponent>(); 2593 for (TeardownActionComponent i : action) 2594 dst.action.add(i.copy()); 2595 }; 2596 return dst; 2597 } 2598 2599 @Override 2600 public boolean equalsDeep(Base other_) { 2601 if (!super.equalsDeep(other_)) 2602 return false; 2603 if (!(other_ instanceof TestReportTeardownComponent)) 2604 return false; 2605 TestReportTeardownComponent o = (TestReportTeardownComponent) other_; 2606 return compareDeep(action, o.action, true); 2607 } 2608 2609 @Override 2610 public boolean equalsShallow(Base other_) { 2611 if (!super.equalsShallow(other_)) 2612 return false; 2613 if (!(other_ instanceof TestReportTeardownComponent)) 2614 return false; 2615 TestReportTeardownComponent o = (TestReportTeardownComponent) other_; 2616 return true; 2617 } 2618 2619 public boolean isEmpty() { 2620 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 2621 } 2622 2623 public String fhirType() { 2624 return "TestReport.teardown"; 2625 2626 } 2627 2628 } 2629 2630 @Block() 2631 public static class TeardownActionComponent extends BackboneElement implements IBaseBackboneElement { 2632 /** 2633 * An operation would involve a REST request to a server. 2634 */ 2635 @Child(name = "operation", type = {SetupActionOperationComponent.class}, order=1, min=1, max=1, modifier=false, summary=false) 2636 @Description(shortDefinition="The teardown operation performed", formalDefinition="An operation would involve a REST request to a server." ) 2637 protected SetupActionOperationComponent operation; 2638 2639 private static final long serialVersionUID = -1099598054L; 2640 2641 /** 2642 * Constructor 2643 */ 2644 public TeardownActionComponent() { 2645 super(); 2646 } 2647 2648 /** 2649 * Constructor 2650 */ 2651 public TeardownActionComponent(SetupActionOperationComponent operation) { 2652 super(); 2653 this.operation = operation; 2654 } 2655 2656 /** 2657 * @return {@link #operation} (An operation would involve a REST request to a server.) 2658 */ 2659 public SetupActionOperationComponent getOperation() { 2660 if (this.operation == null) 2661 if (Configuration.errorOnAutoCreate()) 2662 throw new Error("Attempt to auto-create TeardownActionComponent.operation"); 2663 else if (Configuration.doAutoCreate()) 2664 this.operation = new SetupActionOperationComponent(); // cc 2665 return this.operation; 2666 } 2667 2668 public boolean hasOperation() { 2669 return this.operation != null && !this.operation.isEmpty(); 2670 } 2671 2672 /** 2673 * @param value {@link #operation} (An operation would involve a REST request to a server.) 2674 */ 2675 public TeardownActionComponent setOperation(SetupActionOperationComponent value) { 2676 this.operation = value; 2677 return this; 2678 } 2679 2680 protected void listChildren(List<Property> children) { 2681 super.listChildren(children); 2682 children.add(new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation)); 2683 } 2684 2685 @Override 2686 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2687 switch (_hash) { 2688 case 1662702951: /*operation*/ return new Property("operation", "@TestReport.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation); 2689 default: return super.getNamedProperty(_hash, _name, _checkValid); 2690 } 2691 2692 } 2693 2694 @Override 2695 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2696 switch (hash) { 2697 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 2698 default: return super.getProperty(hash, name, checkValid); 2699 } 2700 2701 } 2702 2703 @Override 2704 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2705 switch (hash) { 2706 case 1662702951: // operation 2707 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2708 return value; 2709 default: return super.setProperty(hash, name, value); 2710 } 2711 2712 } 2713 2714 @Override 2715 public Base setProperty(String name, Base value) throws FHIRException { 2716 if (name.equals("operation")) { 2717 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2718 } else 2719 return super.setProperty(name, value); 2720 return value; 2721 } 2722 2723 @Override 2724 public Base makeProperty(int hash, String name) throws FHIRException { 2725 switch (hash) { 2726 case 1662702951: return getOperation(); 2727 default: return super.makeProperty(hash, name); 2728 } 2729 2730 } 2731 2732 @Override 2733 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2734 switch (hash) { 2735 case 1662702951: /*operation*/ return new String[] {"@TestReport.setup.action.operation"}; 2736 default: return super.getTypesForProperty(hash, name); 2737 } 2738 2739 } 2740 2741 @Override 2742 public Base addChild(String name) throws FHIRException { 2743 if (name.equals("operation")) { 2744 this.operation = new SetupActionOperationComponent(); 2745 return this.operation; 2746 } 2747 else 2748 return super.addChild(name); 2749 } 2750 2751 public TeardownActionComponent copy() { 2752 TeardownActionComponent dst = new TeardownActionComponent(); 2753 copyValues(dst); 2754 dst.operation = operation == null ? null : operation.copy(); 2755 return dst; 2756 } 2757 2758 @Override 2759 public boolean equalsDeep(Base other_) { 2760 if (!super.equalsDeep(other_)) 2761 return false; 2762 if (!(other_ instanceof TeardownActionComponent)) 2763 return false; 2764 TeardownActionComponent o = (TeardownActionComponent) other_; 2765 return compareDeep(operation, o.operation, true); 2766 } 2767 2768 @Override 2769 public boolean equalsShallow(Base other_) { 2770 if (!super.equalsShallow(other_)) 2771 return false; 2772 if (!(other_ instanceof TeardownActionComponent)) 2773 return false; 2774 TeardownActionComponent o = (TeardownActionComponent) other_; 2775 return true; 2776 } 2777 2778 public boolean isEmpty() { 2779 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation); 2780 } 2781 2782 public String fhirType() { 2783 return "TestReport.teardown.action"; 2784 2785 } 2786 2787 } 2788 2789 /** 2790 * Identifier for the TestScript assigned for external purposes outside the context of FHIR. 2791 */ 2792 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 2793 @Description(shortDefinition="External identifier", formalDefinition="Identifier for the TestScript assigned for external purposes outside the context of FHIR." ) 2794 protected Identifier identifier; 2795 2796 /** 2797 * A free text natural language name identifying the executed TestScript. 2798 */ 2799 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 2800 @Description(shortDefinition="Informal name of the executed TestScript", formalDefinition="A free text natural language name identifying the executed TestScript." ) 2801 protected StringType name; 2802 2803 /** 2804 * The current state of this test report. 2805 */ 2806 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 2807 @Description(shortDefinition="completed | in-progress | waiting | stopped | entered-in-error", formalDefinition="The current state of this test report." ) 2808 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-status-codes") 2809 protected Enumeration<TestReportStatus> status; 2810 2811 /** 2812 * Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`. 2813 */ 2814 @Child(name = "testScript", type = {TestScript.class}, order=3, min=1, max=1, modifier=false, summary=true) 2815 @Description(shortDefinition="Reference to the version-specific TestScript that was executed to produce this TestReport", formalDefinition="Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`." ) 2816 protected Reference testScript; 2817 2818 /** 2819 * The actual object that is the target of the reference (Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.) 2820 */ 2821 protected TestScript testScriptTarget; 2822 2823 /** 2824 * The overall result from the execution of the TestScript. 2825 */ 2826 @Child(name = "result", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 2827 @Description(shortDefinition="pass | fail | pending", formalDefinition="The overall result from the execution of the TestScript." ) 2828 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/report-result-codes") 2829 protected Enumeration<TestReportResult> result; 2830 2831 /** 2832 * The final score (percentage of tests passed) resulting from the execution of the TestScript. 2833 */ 2834 @Child(name = "score", type = {DecimalType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2835 @Description(shortDefinition="The final score (percentage of tests passed) resulting from the execution of the TestScript", formalDefinition="The final score (percentage of tests passed) resulting from the execution of the TestScript." ) 2836 protected DecimalType score; 2837 2838 /** 2839 * Name of the tester producing this report (Organization or individual). 2840 */ 2841 @Child(name = "tester", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 2842 @Description(shortDefinition="Name of the tester producing this report (Organization or individual)", formalDefinition="Name of the tester producing this report (Organization or individual)." ) 2843 protected StringType tester; 2844 2845 /** 2846 * When the TestScript was executed and this TestReport was generated. 2847 */ 2848 @Child(name = "issued", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 2849 @Description(shortDefinition="When the TestScript was executed and this TestReport was generated", formalDefinition="When the TestScript was executed and this TestReport was generated." ) 2850 protected DateTimeType issued; 2851 2852 /** 2853 * A participant in the test execution, either the execution engine, a client, or a server. 2854 */ 2855 @Child(name = "participant", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2856 @Description(shortDefinition="A participant in the test execution, either the execution engine, a client, or a server", formalDefinition="A participant in the test execution, either the execution engine, a client, or a server." ) 2857 protected List<TestReportParticipantComponent> participant; 2858 2859 /** 2860 * The results of the series of required setup operations before the tests were executed. 2861 */ 2862 @Child(name = "setup", type = {}, order=9, min=0, max=1, modifier=false, summary=false) 2863 @Description(shortDefinition="The results of the series of required setup operations before the tests were executed", formalDefinition="The results of the series of required setup operations before the tests were executed." ) 2864 protected TestReportSetupComponent setup; 2865 2866 /** 2867 * A test executed from the test script. 2868 */ 2869 @Child(name = "test", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2870 @Description(shortDefinition="A test executed from the test script", formalDefinition="A test executed from the test script." ) 2871 protected List<TestReportTestComponent> test; 2872 2873 /** 2874 * The results of the series of operations required to clean up after the all the tests were executed (successfully or otherwise). 2875 */ 2876 @Child(name = "teardown", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 2877 @Description(shortDefinition="The results of running the series of required clean up steps", formalDefinition="The results of the series of operations required to clean up after the all the tests were executed (successfully or otherwise)." ) 2878 protected TestReportTeardownComponent teardown; 2879 2880 private static final long serialVersionUID = 79474516L; 2881 2882 /** 2883 * Constructor 2884 */ 2885 public TestReport() { 2886 super(); 2887 } 2888 2889 /** 2890 * Constructor 2891 */ 2892 public TestReport(Enumeration<TestReportStatus> status, Reference testScript, Enumeration<TestReportResult> result) { 2893 super(); 2894 this.status = status; 2895 this.testScript = testScript; 2896 this.result = result; 2897 } 2898 2899 /** 2900 * @return {@link #identifier} (Identifier for the TestScript assigned for external purposes outside the context of FHIR.) 2901 */ 2902 public Identifier getIdentifier() { 2903 if (this.identifier == null) 2904 if (Configuration.errorOnAutoCreate()) 2905 throw new Error("Attempt to auto-create TestReport.identifier"); 2906 else if (Configuration.doAutoCreate()) 2907 this.identifier = new Identifier(); // cc 2908 return this.identifier; 2909 } 2910 2911 public boolean hasIdentifier() { 2912 return this.identifier != null && !this.identifier.isEmpty(); 2913 } 2914 2915 /** 2916 * @param value {@link #identifier} (Identifier for the TestScript assigned for external purposes outside the context of FHIR.) 2917 */ 2918 public TestReport setIdentifier(Identifier value) { 2919 this.identifier = value; 2920 return this; 2921 } 2922 2923 /** 2924 * @return {@link #name} (A free text natural language name identifying the executed TestScript.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2925 */ 2926 public StringType getNameElement() { 2927 if (this.name == null) 2928 if (Configuration.errorOnAutoCreate()) 2929 throw new Error("Attempt to auto-create TestReport.name"); 2930 else if (Configuration.doAutoCreate()) 2931 this.name = new StringType(); // bb 2932 return this.name; 2933 } 2934 2935 public boolean hasNameElement() { 2936 return this.name != null && !this.name.isEmpty(); 2937 } 2938 2939 public boolean hasName() { 2940 return this.name != null && !this.name.isEmpty(); 2941 } 2942 2943 /** 2944 * @param value {@link #name} (A free text natural language name identifying the executed TestScript.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2945 */ 2946 public TestReport setNameElement(StringType value) { 2947 this.name = value; 2948 return this; 2949 } 2950 2951 /** 2952 * @return A free text natural language name identifying the executed TestScript. 2953 */ 2954 public String getName() { 2955 return this.name == null ? null : this.name.getValue(); 2956 } 2957 2958 /** 2959 * @param value A free text natural language name identifying the executed TestScript. 2960 */ 2961 public TestReport setName(String value) { 2962 if (Utilities.noString(value)) 2963 this.name = null; 2964 else { 2965 if (this.name == null) 2966 this.name = new StringType(); 2967 this.name.setValue(value); 2968 } 2969 return this; 2970 } 2971 2972 /** 2973 * @return {@link #status} (The current state of this test report.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2974 */ 2975 public Enumeration<TestReportStatus> getStatusElement() { 2976 if (this.status == null) 2977 if (Configuration.errorOnAutoCreate()) 2978 throw new Error("Attempt to auto-create TestReport.status"); 2979 else if (Configuration.doAutoCreate()) 2980 this.status = new Enumeration<TestReportStatus>(new TestReportStatusEnumFactory()); // bb 2981 return this.status; 2982 } 2983 2984 public boolean hasStatusElement() { 2985 return this.status != null && !this.status.isEmpty(); 2986 } 2987 2988 public boolean hasStatus() { 2989 return this.status != null && !this.status.isEmpty(); 2990 } 2991 2992 /** 2993 * @param value {@link #status} (The current state of this test report.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2994 */ 2995 public TestReport setStatusElement(Enumeration<TestReportStatus> value) { 2996 this.status = value; 2997 return this; 2998 } 2999 3000 /** 3001 * @return The current state of this test report. 3002 */ 3003 public TestReportStatus getStatus() { 3004 return this.status == null ? null : this.status.getValue(); 3005 } 3006 3007 /** 3008 * @param value The current state of this test report. 3009 */ 3010 public TestReport setStatus(TestReportStatus value) { 3011 if (this.status == null) 3012 this.status = new Enumeration<TestReportStatus>(new TestReportStatusEnumFactory()); 3013 this.status.setValue(value); 3014 return this; 3015 } 3016 3017 /** 3018 * @return {@link #testScript} (Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.) 3019 */ 3020 public Reference getTestScript() { 3021 if (this.testScript == null) 3022 if (Configuration.errorOnAutoCreate()) 3023 throw new Error("Attempt to auto-create TestReport.testScript"); 3024 else if (Configuration.doAutoCreate()) 3025 this.testScript = new Reference(); // cc 3026 return this.testScript; 3027 } 3028 3029 public boolean hasTestScript() { 3030 return this.testScript != null && !this.testScript.isEmpty(); 3031 } 3032 3033 /** 3034 * @param value {@link #testScript} (Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.) 3035 */ 3036 public TestReport setTestScript(Reference value) { 3037 this.testScript = value; 3038 return this; 3039 } 3040 3041 /** 3042 * @return {@link #testScript} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.) 3043 */ 3044 public TestScript getTestScriptTarget() { 3045 if (this.testScriptTarget == null) 3046 if (Configuration.errorOnAutoCreate()) 3047 throw new Error("Attempt to auto-create TestReport.testScript"); 3048 else if (Configuration.doAutoCreate()) 3049 this.testScriptTarget = new TestScript(); // aa 3050 return this.testScriptTarget; 3051 } 3052 3053 /** 3054 * @param value {@link #testScript} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.) 3055 */ 3056 public TestReport setTestScriptTarget(TestScript value) { 3057 this.testScriptTarget = value; 3058 return this; 3059 } 3060 3061 /** 3062 * @return {@link #result} (The overall result from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 3063 */ 3064 public Enumeration<TestReportResult> getResultElement() { 3065 if (this.result == null) 3066 if (Configuration.errorOnAutoCreate()) 3067 throw new Error("Attempt to auto-create TestReport.result"); 3068 else if (Configuration.doAutoCreate()) 3069 this.result = new Enumeration<TestReportResult>(new TestReportResultEnumFactory()); // bb 3070 return this.result; 3071 } 3072 3073 public boolean hasResultElement() { 3074 return this.result != null && !this.result.isEmpty(); 3075 } 3076 3077 public boolean hasResult() { 3078 return this.result != null && !this.result.isEmpty(); 3079 } 3080 3081 /** 3082 * @param value {@link #result} (The overall result from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getResult" gives direct access to the value 3083 */ 3084 public TestReport setResultElement(Enumeration<TestReportResult> value) { 3085 this.result = value; 3086 return this; 3087 } 3088 3089 /** 3090 * @return The overall result from the execution of the TestScript. 3091 */ 3092 public TestReportResult getResult() { 3093 return this.result == null ? null : this.result.getValue(); 3094 } 3095 3096 /** 3097 * @param value The overall result from the execution of the TestScript. 3098 */ 3099 public TestReport setResult(TestReportResult value) { 3100 if (this.result == null) 3101 this.result = new Enumeration<TestReportResult>(new TestReportResultEnumFactory()); 3102 this.result.setValue(value); 3103 return this; 3104 } 3105 3106 /** 3107 * @return {@link #score} (The final score (percentage of tests passed) resulting from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getScore" gives direct access to the value 3108 */ 3109 public DecimalType getScoreElement() { 3110 if (this.score == null) 3111 if (Configuration.errorOnAutoCreate()) 3112 throw new Error("Attempt to auto-create TestReport.score"); 3113 else if (Configuration.doAutoCreate()) 3114 this.score = new DecimalType(); // bb 3115 return this.score; 3116 } 3117 3118 public boolean hasScoreElement() { 3119 return this.score != null && !this.score.isEmpty(); 3120 } 3121 3122 public boolean hasScore() { 3123 return this.score != null && !this.score.isEmpty(); 3124 } 3125 3126 /** 3127 * @param value {@link #score} (The final score (percentage of tests passed) resulting from the execution of the TestScript.). This is the underlying object with id, value and extensions. The accessor "getScore" gives direct access to the value 3128 */ 3129 public TestReport setScoreElement(DecimalType value) { 3130 this.score = value; 3131 return this; 3132 } 3133 3134 /** 3135 * @return The final score (percentage of tests passed) resulting from the execution of the TestScript. 3136 */ 3137 public BigDecimal getScore() { 3138 return this.score == null ? null : this.score.getValue(); 3139 } 3140 3141 /** 3142 * @param value The final score (percentage of tests passed) resulting from the execution of the TestScript. 3143 */ 3144 public TestReport setScore(BigDecimal value) { 3145 if (value == null) 3146 this.score = null; 3147 else { 3148 if (this.score == null) 3149 this.score = new DecimalType(); 3150 this.score.setValue(value); 3151 } 3152 return this; 3153 } 3154 3155 /** 3156 * @param value The final score (percentage of tests passed) resulting from the execution of the TestScript. 3157 */ 3158 public TestReport setScore(long value) { 3159 this.score = new DecimalType(); 3160 this.score.setValue(value); 3161 return this; 3162 } 3163 3164 /** 3165 * @param value The final score (percentage of tests passed) resulting from the execution of the TestScript. 3166 */ 3167 public TestReport setScore(double value) { 3168 this.score = new DecimalType(); 3169 this.score.setValue(value); 3170 return this; 3171 } 3172 3173 /** 3174 * @return {@link #tester} (Name of the tester producing this report (Organization or individual).). This is the underlying object with id, value and extensions. The accessor "getTester" gives direct access to the value 3175 */ 3176 public StringType getTesterElement() { 3177 if (this.tester == null) 3178 if (Configuration.errorOnAutoCreate()) 3179 throw new Error("Attempt to auto-create TestReport.tester"); 3180 else if (Configuration.doAutoCreate()) 3181 this.tester = new StringType(); // bb 3182 return this.tester; 3183 } 3184 3185 public boolean hasTesterElement() { 3186 return this.tester != null && !this.tester.isEmpty(); 3187 } 3188 3189 public boolean hasTester() { 3190 return this.tester != null && !this.tester.isEmpty(); 3191 } 3192 3193 /** 3194 * @param value {@link #tester} (Name of the tester producing this report (Organization or individual).). This is the underlying object with id, value and extensions. The accessor "getTester" gives direct access to the value 3195 */ 3196 public TestReport setTesterElement(StringType value) { 3197 this.tester = value; 3198 return this; 3199 } 3200 3201 /** 3202 * @return Name of the tester producing this report (Organization or individual). 3203 */ 3204 public String getTester() { 3205 return this.tester == null ? null : this.tester.getValue(); 3206 } 3207 3208 /** 3209 * @param value Name of the tester producing this report (Organization or individual). 3210 */ 3211 public TestReport setTester(String value) { 3212 if (Utilities.noString(value)) 3213 this.tester = null; 3214 else { 3215 if (this.tester == null) 3216 this.tester = new StringType(); 3217 this.tester.setValue(value); 3218 } 3219 return this; 3220 } 3221 3222 /** 3223 * @return {@link #issued} (When the TestScript was executed and this TestReport was generated.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 3224 */ 3225 public DateTimeType getIssuedElement() { 3226 if (this.issued == null) 3227 if (Configuration.errorOnAutoCreate()) 3228 throw new Error("Attempt to auto-create TestReport.issued"); 3229 else if (Configuration.doAutoCreate()) 3230 this.issued = new DateTimeType(); // bb 3231 return this.issued; 3232 } 3233 3234 public boolean hasIssuedElement() { 3235 return this.issued != null && !this.issued.isEmpty(); 3236 } 3237 3238 public boolean hasIssued() { 3239 return this.issued != null && !this.issued.isEmpty(); 3240 } 3241 3242 /** 3243 * @param value {@link #issued} (When the TestScript was executed and this TestReport was generated.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 3244 */ 3245 public TestReport setIssuedElement(DateTimeType value) { 3246 this.issued = value; 3247 return this; 3248 } 3249 3250 /** 3251 * @return When the TestScript was executed and this TestReport was generated. 3252 */ 3253 public Date getIssued() { 3254 return this.issued == null ? null : this.issued.getValue(); 3255 } 3256 3257 /** 3258 * @param value When the TestScript was executed and this TestReport was generated. 3259 */ 3260 public TestReport setIssued(Date value) { 3261 if (value == null) 3262 this.issued = null; 3263 else { 3264 if (this.issued == null) 3265 this.issued = new DateTimeType(); 3266 this.issued.setValue(value); 3267 } 3268 return this; 3269 } 3270 3271 /** 3272 * @return {@link #participant} (A participant in the test execution, either the execution engine, a client, or a server.) 3273 */ 3274 public List<TestReportParticipantComponent> getParticipant() { 3275 if (this.participant == null) 3276 this.participant = new ArrayList<TestReportParticipantComponent>(); 3277 return this.participant; 3278 } 3279 3280 /** 3281 * @return Returns a reference to <code>this</code> for easy method chaining 3282 */ 3283 public TestReport setParticipant(List<TestReportParticipantComponent> theParticipant) { 3284 this.participant = theParticipant; 3285 return this; 3286 } 3287 3288 public boolean hasParticipant() { 3289 if (this.participant == null) 3290 return false; 3291 for (TestReportParticipantComponent item : this.participant) 3292 if (!item.isEmpty()) 3293 return true; 3294 return false; 3295 } 3296 3297 public TestReportParticipantComponent addParticipant() { //3 3298 TestReportParticipantComponent t = new TestReportParticipantComponent(); 3299 if (this.participant == null) 3300 this.participant = new ArrayList<TestReportParticipantComponent>(); 3301 this.participant.add(t); 3302 return t; 3303 } 3304 3305 public TestReport addParticipant(TestReportParticipantComponent t) { //3 3306 if (t == null) 3307 return this; 3308 if (this.participant == null) 3309 this.participant = new ArrayList<TestReportParticipantComponent>(); 3310 this.participant.add(t); 3311 return this; 3312 } 3313 3314 /** 3315 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist 3316 */ 3317 public TestReportParticipantComponent getParticipantFirstRep() { 3318 if (getParticipant().isEmpty()) { 3319 addParticipant(); 3320 } 3321 return getParticipant().get(0); 3322 } 3323 3324 /** 3325 * @return {@link #setup} (The results of the series of required setup operations before the tests were executed.) 3326 */ 3327 public TestReportSetupComponent getSetup() { 3328 if (this.setup == null) 3329 if (Configuration.errorOnAutoCreate()) 3330 throw new Error("Attempt to auto-create TestReport.setup"); 3331 else if (Configuration.doAutoCreate()) 3332 this.setup = new TestReportSetupComponent(); // cc 3333 return this.setup; 3334 } 3335 3336 public boolean hasSetup() { 3337 return this.setup != null && !this.setup.isEmpty(); 3338 } 3339 3340 /** 3341 * @param value {@link #setup} (The results of the series of required setup operations before the tests were executed.) 3342 */ 3343 public TestReport setSetup(TestReportSetupComponent value) { 3344 this.setup = value; 3345 return this; 3346 } 3347 3348 /** 3349 * @return {@link #test} (A test executed from the test script.) 3350 */ 3351 public List<TestReportTestComponent> getTest() { 3352 if (this.test == null) 3353 this.test = new ArrayList<TestReportTestComponent>(); 3354 return this.test; 3355 } 3356 3357 /** 3358 * @return Returns a reference to <code>this</code> for easy method chaining 3359 */ 3360 public TestReport setTest(List<TestReportTestComponent> theTest) { 3361 this.test = theTest; 3362 return this; 3363 } 3364 3365 public boolean hasTest() { 3366 if (this.test == null) 3367 return false; 3368 for (TestReportTestComponent item : this.test) 3369 if (!item.isEmpty()) 3370 return true; 3371 return false; 3372 } 3373 3374 public TestReportTestComponent addTest() { //3 3375 TestReportTestComponent t = new TestReportTestComponent(); 3376 if (this.test == null) 3377 this.test = new ArrayList<TestReportTestComponent>(); 3378 this.test.add(t); 3379 return t; 3380 } 3381 3382 public TestReport addTest(TestReportTestComponent t) { //3 3383 if (t == null) 3384 return this; 3385 if (this.test == null) 3386 this.test = new ArrayList<TestReportTestComponent>(); 3387 this.test.add(t); 3388 return this; 3389 } 3390 3391 /** 3392 * @return The first repetition of repeating field {@link #test}, creating it if it does not already exist 3393 */ 3394 public TestReportTestComponent getTestFirstRep() { 3395 if (getTest().isEmpty()) { 3396 addTest(); 3397 } 3398 return getTest().get(0); 3399 } 3400 3401 /** 3402 * @return {@link #teardown} (The results of the series of operations required to clean up after the all the tests were executed (successfully or otherwise).) 3403 */ 3404 public TestReportTeardownComponent getTeardown() { 3405 if (this.teardown == null) 3406 if (Configuration.errorOnAutoCreate()) 3407 throw new Error("Attempt to auto-create TestReport.teardown"); 3408 else if (Configuration.doAutoCreate()) 3409 this.teardown = new TestReportTeardownComponent(); // cc 3410 return this.teardown; 3411 } 3412 3413 public boolean hasTeardown() { 3414 return this.teardown != null && !this.teardown.isEmpty(); 3415 } 3416 3417 /** 3418 * @param value {@link #teardown} (The results of the series of operations required to clean up after the all the tests were executed (successfully or otherwise).) 3419 */ 3420 public TestReport setTeardown(TestReportTeardownComponent value) { 3421 this.teardown = value; 3422 return this; 3423 } 3424 3425 protected void listChildren(List<Property> children) { 3426 super.listChildren(children); 3427 children.add(new Property("identifier", "Identifier", "Identifier for the TestScript assigned for external purposes outside the context of FHIR.", 0, 1, identifier)); 3428 children.add(new Property("name", "string", "A free text natural language name identifying the executed TestScript.", 0, 1, name)); 3429 children.add(new Property("status", "code", "The current state of this test report.", 0, 1, status)); 3430 children.add(new Property("testScript", "Reference(TestScript)", "Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.", 0, 1, testScript)); 3431 children.add(new Property("result", "code", "The overall result from the execution of the TestScript.", 0, 1, result)); 3432 children.add(new Property("score", "decimal", "The final score (percentage of tests passed) resulting from the execution of the TestScript.", 0, 1, score)); 3433 children.add(new Property("tester", "string", "Name of the tester producing this report (Organization or individual).", 0, 1, tester)); 3434 children.add(new Property("issued", "dateTime", "When the TestScript was executed and this TestReport was generated.", 0, 1, issued)); 3435 children.add(new Property("participant", "", "A participant in the test execution, either the execution engine, a client, or a server.", 0, java.lang.Integer.MAX_VALUE, participant)); 3436 children.add(new Property("setup", "", "The results of the series of required setup operations before the tests were executed.", 0, 1, setup)); 3437 children.add(new Property("test", "", "A test executed from the test script.", 0, java.lang.Integer.MAX_VALUE, test)); 3438 children.add(new Property("teardown", "", "The results of the series of operations required to clean up after the all the tests were executed (successfully or otherwise).", 0, 1, teardown)); 3439 } 3440 3441 @Override 3442 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3443 switch (_hash) { 3444 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the TestScript assigned for external purposes outside the context of FHIR.", 0, 1, identifier); 3445 case 3373707: /*name*/ return new Property("name", "string", "A free text natural language name identifying the executed TestScript.", 0, 1, name); 3446 case -892481550: /*status*/ return new Property("status", "code", "The current state of this test report.", 0, 1, status); 3447 case 1712049149: /*testScript*/ return new Property("testScript", "Reference(TestScript)", "Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.", 0, 1, testScript); 3448 case -934426595: /*result*/ return new Property("result", "code", "The overall result from the execution of the TestScript.", 0, 1, result); 3449 case 109264530: /*score*/ return new Property("score", "decimal", "The final score (percentage of tests passed) resulting from the execution of the TestScript.", 0, 1, score); 3450 case -877169473: /*tester*/ return new Property("tester", "string", "Name of the tester producing this report (Organization or individual).", 0, 1, tester); 3451 case -1179159893: /*issued*/ return new Property("issued", "dateTime", "When the TestScript was executed and this TestReport was generated.", 0, 1, issued); 3452 case 767422259: /*participant*/ return new Property("participant", "", "A participant in the test execution, either the execution engine, a client, or a server.", 0, java.lang.Integer.MAX_VALUE, participant); 3453 case 109329021: /*setup*/ return new Property("setup", "", "The results of the series of required setup operations before the tests were executed.", 0, 1, setup); 3454 case 3556498: /*test*/ return new Property("test", "", "A test executed from the test script.", 0, java.lang.Integer.MAX_VALUE, test); 3455 case -1663474172: /*teardown*/ return new Property("teardown", "", "The results of the series of operations required to clean up after the all the tests were executed (successfully or otherwise).", 0, 1, teardown); 3456 default: return super.getNamedProperty(_hash, _name, _checkValid); 3457 } 3458 3459 } 3460 3461 @Override 3462 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3463 switch (hash) { 3464 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 3465 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3466 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<TestReportStatus> 3467 case 1712049149: /*testScript*/ return this.testScript == null ? new Base[0] : new Base[] {this.testScript}; // Reference 3468 case -934426595: /*result*/ return this.result == null ? new Base[0] : new Base[] {this.result}; // Enumeration<TestReportResult> 3469 case 109264530: /*score*/ return this.score == null ? new Base[0] : new Base[] {this.score}; // DecimalType 3470 case -877169473: /*tester*/ return this.tester == null ? new Base[0] : new Base[] {this.tester}; // StringType 3471 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // DateTimeType 3472 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // TestReportParticipantComponent 3473 case 109329021: /*setup*/ return this.setup == null ? new Base[0] : new Base[] {this.setup}; // TestReportSetupComponent 3474 case 3556498: /*test*/ return this.test == null ? new Base[0] : this.test.toArray(new Base[this.test.size()]); // TestReportTestComponent 3475 case -1663474172: /*teardown*/ return this.teardown == null ? new Base[0] : new Base[] {this.teardown}; // TestReportTeardownComponent 3476 default: return super.getProperty(hash, name, checkValid); 3477 } 3478 3479 } 3480 3481 @Override 3482 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3483 switch (hash) { 3484 case -1618432855: // identifier 3485 this.identifier = castToIdentifier(value); // Identifier 3486 return value; 3487 case 3373707: // name 3488 this.name = castToString(value); // StringType 3489 return value; 3490 case -892481550: // status 3491 value = new TestReportStatusEnumFactory().fromType(castToCode(value)); 3492 this.status = (Enumeration) value; // Enumeration<TestReportStatus> 3493 return value; 3494 case 1712049149: // testScript 3495 this.testScript = castToReference(value); // Reference 3496 return value; 3497 case -934426595: // result 3498 value = new TestReportResultEnumFactory().fromType(castToCode(value)); 3499 this.result = (Enumeration) value; // Enumeration<TestReportResult> 3500 return value; 3501 case 109264530: // score 3502 this.score = castToDecimal(value); // DecimalType 3503 return value; 3504 case -877169473: // tester 3505 this.tester = castToString(value); // StringType 3506 return value; 3507 case -1179159893: // issued 3508 this.issued = castToDateTime(value); // DateTimeType 3509 return value; 3510 case 767422259: // participant 3511 this.getParticipant().add((TestReportParticipantComponent) value); // TestReportParticipantComponent 3512 return value; 3513 case 109329021: // setup 3514 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 3515 return value; 3516 case 3556498: // test 3517 this.getTest().add((TestReportTestComponent) value); // TestReportTestComponent 3518 return value; 3519 case -1663474172: // teardown 3520 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 3521 return value; 3522 default: return super.setProperty(hash, name, value); 3523 } 3524 3525 } 3526 3527 @Override 3528 public Base setProperty(String name, Base value) throws FHIRException { 3529 if (name.equals("identifier")) { 3530 this.identifier = castToIdentifier(value); // Identifier 3531 } else if (name.equals("name")) { 3532 this.name = castToString(value); // StringType 3533 } else if (name.equals("status")) { 3534 value = new TestReportStatusEnumFactory().fromType(castToCode(value)); 3535 this.status = (Enumeration) value; // Enumeration<TestReportStatus> 3536 } else if (name.equals("testScript")) { 3537 this.testScript = castToReference(value); // Reference 3538 } else if (name.equals("result")) { 3539 value = new TestReportResultEnumFactory().fromType(castToCode(value)); 3540 this.result = (Enumeration) value; // Enumeration<TestReportResult> 3541 } else if (name.equals("score")) { 3542 this.score = castToDecimal(value); // DecimalType 3543 } else if (name.equals("tester")) { 3544 this.tester = castToString(value); // StringType 3545 } else if (name.equals("issued")) { 3546 this.issued = castToDateTime(value); // DateTimeType 3547 } else if (name.equals("participant")) { 3548 this.getParticipant().add((TestReportParticipantComponent) value); 3549 } else if (name.equals("setup")) { 3550 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 3551 } else if (name.equals("test")) { 3552 this.getTest().add((TestReportTestComponent) value); 3553 } else if (name.equals("teardown")) { 3554 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 3555 } else 3556 return super.setProperty(name, value); 3557 return value; 3558 } 3559 3560 @Override 3561 public Base makeProperty(int hash, String name) throws FHIRException { 3562 switch (hash) { 3563 case -1618432855: return getIdentifier(); 3564 case 3373707: return getNameElement(); 3565 case -892481550: return getStatusElement(); 3566 case 1712049149: return getTestScript(); 3567 case -934426595: return getResultElement(); 3568 case 109264530: return getScoreElement(); 3569 case -877169473: return getTesterElement(); 3570 case -1179159893: return getIssuedElement(); 3571 case 767422259: return addParticipant(); 3572 case 109329021: return getSetup(); 3573 case 3556498: return addTest(); 3574 case -1663474172: return getTeardown(); 3575 default: return super.makeProperty(hash, name); 3576 } 3577 3578 } 3579 3580 @Override 3581 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3582 switch (hash) { 3583 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3584 case 3373707: /*name*/ return new String[] {"string"}; 3585 case -892481550: /*status*/ return new String[] {"code"}; 3586 case 1712049149: /*testScript*/ return new String[] {"Reference"}; 3587 case -934426595: /*result*/ return new String[] {"code"}; 3588 case 109264530: /*score*/ return new String[] {"decimal"}; 3589 case -877169473: /*tester*/ return new String[] {"string"}; 3590 case -1179159893: /*issued*/ return new String[] {"dateTime"}; 3591 case 767422259: /*participant*/ return new String[] {}; 3592 case 109329021: /*setup*/ return new String[] {}; 3593 case 3556498: /*test*/ return new String[] {}; 3594 case -1663474172: /*teardown*/ return new String[] {}; 3595 default: return super.getTypesForProperty(hash, name); 3596 } 3597 3598 } 3599 3600 @Override 3601 public Base addChild(String name) throws FHIRException { 3602 if (name.equals("identifier")) { 3603 this.identifier = new Identifier(); 3604 return this.identifier; 3605 } 3606 else if (name.equals("name")) { 3607 throw new FHIRException("Cannot call addChild on a singleton property TestReport.name"); 3608 } 3609 else if (name.equals("status")) { 3610 throw new FHIRException("Cannot call addChild on a singleton property TestReport.status"); 3611 } 3612 else if (name.equals("testScript")) { 3613 this.testScript = new Reference(); 3614 return this.testScript; 3615 } 3616 else if (name.equals("result")) { 3617 throw new FHIRException("Cannot call addChild on a singleton property TestReport.result"); 3618 } 3619 else if (name.equals("score")) { 3620 throw new FHIRException("Cannot call addChild on a singleton property TestReport.score"); 3621 } 3622 else if (name.equals("tester")) { 3623 throw new FHIRException("Cannot call addChild on a singleton property TestReport.tester"); 3624 } 3625 else if (name.equals("issued")) { 3626 throw new FHIRException("Cannot call addChild on a singleton property TestReport.issued"); 3627 } 3628 else if (name.equals("participant")) { 3629 return addParticipant(); 3630 } 3631 else if (name.equals("setup")) { 3632 this.setup = new TestReportSetupComponent(); 3633 return this.setup; 3634 } 3635 else if (name.equals("test")) { 3636 return addTest(); 3637 } 3638 else if (name.equals("teardown")) { 3639 this.teardown = new TestReportTeardownComponent(); 3640 return this.teardown; 3641 } 3642 else 3643 return super.addChild(name); 3644 } 3645 3646 public String fhirType() { 3647 return "TestReport"; 3648 3649 } 3650 3651 public TestReport copy() { 3652 TestReport dst = new TestReport(); 3653 copyValues(dst); 3654 dst.identifier = identifier == null ? null : identifier.copy(); 3655 dst.name = name == null ? null : name.copy(); 3656 dst.status = status == null ? null : status.copy(); 3657 dst.testScript = testScript == null ? null : testScript.copy(); 3658 dst.result = result == null ? null : result.copy(); 3659 dst.score = score == null ? null : score.copy(); 3660 dst.tester = tester == null ? null : tester.copy(); 3661 dst.issued = issued == null ? null : issued.copy(); 3662 if (participant != null) { 3663 dst.participant = new ArrayList<TestReportParticipantComponent>(); 3664 for (TestReportParticipantComponent i : participant) 3665 dst.participant.add(i.copy()); 3666 }; 3667 dst.setup = setup == null ? null : setup.copy(); 3668 if (test != null) { 3669 dst.test = new ArrayList<TestReportTestComponent>(); 3670 for (TestReportTestComponent i : test) 3671 dst.test.add(i.copy()); 3672 }; 3673 dst.teardown = teardown == null ? null : teardown.copy(); 3674 return dst; 3675 } 3676 3677 protected TestReport typedCopy() { 3678 return copy(); 3679 } 3680 3681 @Override 3682 public boolean equalsDeep(Base other_) { 3683 if (!super.equalsDeep(other_)) 3684 return false; 3685 if (!(other_ instanceof TestReport)) 3686 return false; 3687 TestReport o = (TestReport) other_; 3688 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) && compareDeep(status, o.status, true) 3689 && compareDeep(testScript, o.testScript, true) && compareDeep(result, o.result, true) && compareDeep(score, o.score, true) 3690 && compareDeep(tester, o.tester, true) && compareDeep(issued, o.issued, true) && compareDeep(participant, o.participant, true) 3691 && compareDeep(setup, o.setup, true) && compareDeep(test, o.test, true) && compareDeep(teardown, o.teardown, true) 3692 ; 3693 } 3694 3695 @Override 3696 public boolean equalsShallow(Base other_) { 3697 if (!super.equalsShallow(other_)) 3698 return false; 3699 if (!(other_ instanceof TestReport)) 3700 return false; 3701 TestReport o = (TestReport) other_; 3702 return compareValues(name, o.name, true) && compareValues(status, o.status, true) && compareValues(result, o.result, true) 3703 && compareValues(score, o.score, true) && compareValues(tester, o.tester, true) && compareValues(issued, o.issued, true) 3704 ; 3705 } 3706 3707 public boolean isEmpty() { 3708 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, status 3709 , testScript, result, score, tester, issued, participant, setup, test, teardown 3710 ); 3711 } 3712 3713 @Override 3714 public ResourceType getResourceType() { 3715 return ResourceType.TestReport; 3716 } 3717 3718 /** 3719 * Search parameter: <b>result</b> 3720 * <p> 3721 * Description: <b>The result disposition of the test execution</b><br> 3722 * Type: <b>token</b><br> 3723 * Path: <b>TestReport.result</b><br> 3724 * </p> 3725 */ 3726 @SearchParamDefinition(name="result", path="TestReport.result", description="The result disposition of the test execution", type="token" ) 3727 public static final String SP_RESULT = "result"; 3728 /** 3729 * <b>Fluent Client</b> search parameter constant for <b>result</b> 3730 * <p> 3731 * Description: <b>The result disposition of the test execution</b><br> 3732 * Type: <b>token</b><br> 3733 * Path: <b>TestReport.result</b><br> 3734 * </p> 3735 */ 3736 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESULT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RESULT); 3737 3738 /** 3739 * Search parameter: <b>identifier</b> 3740 * <p> 3741 * Description: <b>An external identifier for the test report</b><br> 3742 * Type: <b>token</b><br> 3743 * Path: <b>TestReport.identifier</b><br> 3744 * </p> 3745 */ 3746 @SearchParamDefinition(name="identifier", path="TestReport.identifier", description="An external identifier for the test report", type="token" ) 3747 public static final String SP_IDENTIFIER = "identifier"; 3748 /** 3749 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3750 * <p> 3751 * Description: <b>An external identifier for the test report</b><br> 3752 * Type: <b>token</b><br> 3753 * Path: <b>TestReport.identifier</b><br> 3754 * </p> 3755 */ 3756 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3757 3758 /** 3759 * Search parameter: <b>tester</b> 3760 * <p> 3761 * Description: <b>The name of the testing organization</b><br> 3762 * Type: <b>string</b><br> 3763 * Path: <b>TestReport.tester</b><br> 3764 * </p> 3765 */ 3766 @SearchParamDefinition(name="tester", path="TestReport.tester", description="The name of the testing organization", type="string" ) 3767 public static final String SP_TESTER = "tester"; 3768 /** 3769 * <b>Fluent Client</b> search parameter constant for <b>tester</b> 3770 * <p> 3771 * Description: <b>The name of the testing organization</b><br> 3772 * Type: <b>string</b><br> 3773 * Path: <b>TestReport.tester</b><br> 3774 * </p> 3775 */ 3776 public static final ca.uhn.fhir.rest.gclient.StringClientParam TESTER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TESTER); 3777 3778 /** 3779 * Search parameter: <b>testscript</b> 3780 * <p> 3781 * Description: <b>The test script executed to produce this report</b><br> 3782 * Type: <b>reference</b><br> 3783 * Path: <b>TestReport.testScript</b><br> 3784 * </p> 3785 */ 3786 @SearchParamDefinition(name="testscript", path="TestReport.testScript", description="The test script executed to produce this report", type="reference", target={TestScript.class } ) 3787 public static final String SP_TESTSCRIPT = "testscript"; 3788 /** 3789 * <b>Fluent Client</b> search parameter constant for <b>testscript</b> 3790 * <p> 3791 * Description: <b>The test script executed to produce this report</b><br> 3792 * Type: <b>reference</b><br> 3793 * Path: <b>TestReport.testScript</b><br> 3794 * </p> 3795 */ 3796 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TESTSCRIPT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_TESTSCRIPT); 3797 3798/** 3799 * Constant for fluent queries to be used to add include statements. Specifies 3800 * the path value of "<b>TestReport:testscript</b>". 3801 */ 3802 public static final ca.uhn.fhir.model.api.Include INCLUDE_TESTSCRIPT = new ca.uhn.fhir.model.api.Include("TestReport:testscript").toLocked(); 3803 3804 /** 3805 * Search parameter: <b>issued</b> 3806 * <p> 3807 * Description: <b>The test report generation date</b><br> 3808 * Type: <b>date</b><br> 3809 * Path: <b>TestReport.issued</b><br> 3810 * </p> 3811 */ 3812 @SearchParamDefinition(name="issued", path="TestReport.issued", description="The test report generation date", type="date" ) 3813 public static final String SP_ISSUED = "issued"; 3814 /** 3815 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 3816 * <p> 3817 * Description: <b>The test report generation date</b><br> 3818 * Type: <b>date</b><br> 3819 * Path: <b>TestReport.issued</b><br> 3820 * </p> 3821 */ 3822 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ISSUED); 3823 3824 /** 3825 * Search parameter: <b>participant</b> 3826 * <p> 3827 * Description: <b>The reference to a participant in the test execution</b><br> 3828 * Type: <b>uri</b><br> 3829 * Path: <b>TestReport.participant.uri</b><br> 3830 * </p> 3831 */ 3832 @SearchParamDefinition(name="participant", path="TestReport.participant.uri", description="The reference to a participant in the test execution", type="uri" ) 3833 public static final String SP_PARTICIPANT = "participant"; 3834 /** 3835 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 3836 * <p> 3837 * Description: <b>The reference to a participant in the test execution</b><br> 3838 * Type: <b>uri</b><br> 3839 * Path: <b>TestReport.participant.uri</b><br> 3840 * </p> 3841 */ 3842 public static final ca.uhn.fhir.rest.gclient.UriClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_PARTICIPANT); 3843 3844 3845}