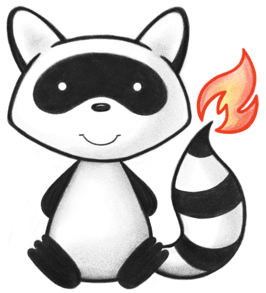
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification. 053 */ 054@ResourceDef(name="TestScript", profile="http://hl7.org/fhir/Profile/TestScript") 055@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "origin", "destination", "metadata", "fixture", "profile", "variable", "rule", "ruleset", "setup", "test", "teardown"}) 056public class TestScript extends MetadataResource { 057 058 public enum ContentType { 059 /** 060 * XML content-type corresponding to the application/fhir+xml mime-type. 061 */ 062 XML, 063 /** 064 * JSON content-type corresponding to the application/fhir+json mime-type. 065 */ 066 JSON, 067 /** 068 * RDF content-type corresponding to the text/turtle mime-type. 069 */ 070 TTL, 071 /** 072 * Prevent the use of the corresponding http header. 073 */ 074 NONE, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 public static ContentType fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("xml".equals(codeString)) 083 return XML; 084 if ("json".equals(codeString)) 085 return JSON; 086 if ("ttl".equals(codeString)) 087 return TTL; 088 if ("none".equals(codeString)) 089 return NONE; 090 if (Configuration.isAcceptInvalidEnums()) 091 return null; 092 else 093 throw new FHIRException("Unknown ContentType code '"+codeString+"'"); 094 } 095 public String toCode() { 096 switch (this) { 097 case XML: return "xml"; 098 case JSON: return "json"; 099 case TTL: return "ttl"; 100 case NONE: return "none"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getSystem() { 106 switch (this) { 107 case XML: return "http://hl7.org/fhir/content-type"; 108 case JSON: return "http://hl7.org/fhir/content-type"; 109 case TTL: return "http://hl7.org/fhir/content-type"; 110 case NONE: return "http://hl7.org/fhir/content-type"; 111 case NULL: return null; 112 default: return "?"; 113 } 114 } 115 public String getDefinition() { 116 switch (this) { 117 case XML: return "XML content-type corresponding to the application/fhir+xml mime-type."; 118 case JSON: return "JSON content-type corresponding to the application/fhir+json mime-type."; 119 case TTL: return "RDF content-type corresponding to the text/turtle mime-type."; 120 case NONE: return "Prevent the use of the corresponding http header."; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 public String getDisplay() { 126 switch (this) { 127 case XML: return "xml"; 128 case JSON: return "json"; 129 case TTL: return "ttl"; 130 case NONE: return "none"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 } 136 137 public static class ContentTypeEnumFactory implements EnumFactory<ContentType> { 138 public ContentType fromCode(String codeString) throws IllegalArgumentException { 139 if (codeString == null || "".equals(codeString)) 140 if (codeString == null || "".equals(codeString)) 141 return null; 142 if ("xml".equals(codeString)) 143 return ContentType.XML; 144 if ("json".equals(codeString)) 145 return ContentType.JSON; 146 if ("ttl".equals(codeString)) 147 return ContentType.TTL; 148 if ("none".equals(codeString)) 149 return ContentType.NONE; 150 throw new IllegalArgumentException("Unknown ContentType code '"+codeString+"'"); 151 } 152 public Enumeration<ContentType> fromType(PrimitiveType<?> code) throws FHIRException { 153 if (code == null) 154 return null; 155 if (code.isEmpty()) 156 return new Enumeration<ContentType>(this); 157 String codeString = code.asStringValue(); 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("xml".equals(codeString)) 161 return new Enumeration<ContentType>(this, ContentType.XML); 162 if ("json".equals(codeString)) 163 return new Enumeration<ContentType>(this, ContentType.JSON); 164 if ("ttl".equals(codeString)) 165 return new Enumeration<ContentType>(this, ContentType.TTL); 166 if ("none".equals(codeString)) 167 return new Enumeration<ContentType>(this, ContentType.NONE); 168 throw new FHIRException("Unknown ContentType code '"+codeString+"'"); 169 } 170 public String toCode(ContentType code) { 171 if (code == ContentType.NULL) 172 return null; 173 if (code == ContentType.XML) 174 return "xml"; 175 if (code == ContentType.JSON) 176 return "json"; 177 if (code == ContentType.TTL) 178 return "ttl"; 179 if (code == ContentType.NONE) 180 return "none"; 181 return "?"; 182 } 183 public String toSystem(ContentType code) { 184 return code.getSystem(); 185 } 186 } 187 188 public enum AssertionDirectionType { 189 /** 190 * The assertion is evaluated on the response. This is the default value. 191 */ 192 RESPONSE, 193 /** 194 * The assertion is evaluated on the request. 195 */ 196 REQUEST, 197 /** 198 * added to help the parsers with the generic types 199 */ 200 NULL; 201 public static AssertionDirectionType fromCode(String codeString) throws FHIRException { 202 if (codeString == null || "".equals(codeString)) 203 return null; 204 if ("response".equals(codeString)) 205 return RESPONSE; 206 if ("request".equals(codeString)) 207 return REQUEST; 208 if (Configuration.isAcceptInvalidEnums()) 209 return null; 210 else 211 throw new FHIRException("Unknown AssertionDirectionType code '"+codeString+"'"); 212 } 213 public String toCode() { 214 switch (this) { 215 case RESPONSE: return "response"; 216 case REQUEST: return "request"; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 public String getSystem() { 222 switch (this) { 223 case RESPONSE: return "http://hl7.org/fhir/assert-direction-codes"; 224 case REQUEST: return "http://hl7.org/fhir/assert-direction-codes"; 225 case NULL: return null; 226 default: return "?"; 227 } 228 } 229 public String getDefinition() { 230 switch (this) { 231 case RESPONSE: return "The assertion is evaluated on the response. This is the default value."; 232 case REQUEST: return "The assertion is evaluated on the request."; 233 case NULL: return null; 234 default: return "?"; 235 } 236 } 237 public String getDisplay() { 238 switch (this) { 239 case RESPONSE: return "response"; 240 case REQUEST: return "request"; 241 case NULL: return null; 242 default: return "?"; 243 } 244 } 245 } 246 247 public static class AssertionDirectionTypeEnumFactory implements EnumFactory<AssertionDirectionType> { 248 public AssertionDirectionType fromCode(String codeString) throws IllegalArgumentException { 249 if (codeString == null || "".equals(codeString)) 250 if (codeString == null || "".equals(codeString)) 251 return null; 252 if ("response".equals(codeString)) 253 return AssertionDirectionType.RESPONSE; 254 if ("request".equals(codeString)) 255 return AssertionDirectionType.REQUEST; 256 throw new IllegalArgumentException("Unknown AssertionDirectionType code '"+codeString+"'"); 257 } 258 public Enumeration<AssertionDirectionType> fromType(PrimitiveType<?> code) throws FHIRException { 259 if (code == null) 260 return null; 261 if (code.isEmpty()) 262 return new Enumeration<AssertionDirectionType>(this); 263 String codeString = code.asStringValue(); 264 if (codeString == null || "".equals(codeString)) 265 return null; 266 if ("response".equals(codeString)) 267 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.RESPONSE); 268 if ("request".equals(codeString)) 269 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.REQUEST); 270 throw new FHIRException("Unknown AssertionDirectionType code '"+codeString+"'"); 271 } 272 public String toCode(AssertionDirectionType code) { 273 if (code == AssertionDirectionType.NULL) 274 return null; 275 if (code == AssertionDirectionType.RESPONSE) 276 return "response"; 277 if (code == AssertionDirectionType.REQUEST) 278 return "request"; 279 return "?"; 280 } 281 public String toSystem(AssertionDirectionType code) { 282 return code.getSystem(); 283 } 284 } 285 286 public enum AssertionOperatorType { 287 /** 288 * Default value. Equals comparison. 289 */ 290 EQUALS, 291 /** 292 * Not equals comparison. 293 */ 294 NOTEQUALS, 295 /** 296 * Compare value within a known set of values. 297 */ 298 IN, 299 /** 300 * Compare value not within a known set of values. 301 */ 302 NOTIN, 303 /** 304 * Compare value to be greater than a known value. 305 */ 306 GREATERTHAN, 307 /** 308 * Compare value to be less than a known value. 309 */ 310 LESSTHAN, 311 /** 312 * Compare value is empty. 313 */ 314 EMPTY, 315 /** 316 * Compare value is not empty. 317 */ 318 NOTEMPTY, 319 /** 320 * Compare value string contains a known value. 321 */ 322 CONTAINS, 323 /** 324 * Compare value string does not contain a known value. 325 */ 326 NOTCONTAINS, 327 /** 328 * Evaluate the fluentpath expression as a boolean condition. 329 */ 330 EVAL, 331 /** 332 * added to help the parsers with the generic types 333 */ 334 NULL; 335 public static AssertionOperatorType fromCode(String codeString) throws FHIRException { 336 if (codeString == null || "".equals(codeString)) 337 return null; 338 if ("equals".equals(codeString)) 339 return EQUALS; 340 if ("notEquals".equals(codeString)) 341 return NOTEQUALS; 342 if ("in".equals(codeString)) 343 return IN; 344 if ("notIn".equals(codeString)) 345 return NOTIN; 346 if ("greaterThan".equals(codeString)) 347 return GREATERTHAN; 348 if ("lessThan".equals(codeString)) 349 return LESSTHAN; 350 if ("empty".equals(codeString)) 351 return EMPTY; 352 if ("notEmpty".equals(codeString)) 353 return NOTEMPTY; 354 if ("contains".equals(codeString)) 355 return CONTAINS; 356 if ("notContains".equals(codeString)) 357 return NOTCONTAINS; 358 if ("eval".equals(codeString)) 359 return EVAL; 360 if (Configuration.isAcceptInvalidEnums()) 361 return null; 362 else 363 throw new FHIRException("Unknown AssertionOperatorType code '"+codeString+"'"); 364 } 365 public String toCode() { 366 switch (this) { 367 case EQUALS: return "equals"; 368 case NOTEQUALS: return "notEquals"; 369 case IN: return "in"; 370 case NOTIN: return "notIn"; 371 case GREATERTHAN: return "greaterThan"; 372 case LESSTHAN: return "lessThan"; 373 case EMPTY: return "empty"; 374 case NOTEMPTY: return "notEmpty"; 375 case CONTAINS: return "contains"; 376 case NOTCONTAINS: return "notContains"; 377 case EVAL: return "eval"; 378 case NULL: return null; 379 default: return "?"; 380 } 381 } 382 public String getSystem() { 383 switch (this) { 384 case EQUALS: return "http://hl7.org/fhir/assert-operator-codes"; 385 case NOTEQUALS: return "http://hl7.org/fhir/assert-operator-codes"; 386 case IN: return "http://hl7.org/fhir/assert-operator-codes"; 387 case NOTIN: return "http://hl7.org/fhir/assert-operator-codes"; 388 case GREATERTHAN: return "http://hl7.org/fhir/assert-operator-codes"; 389 case LESSTHAN: return "http://hl7.org/fhir/assert-operator-codes"; 390 case EMPTY: return "http://hl7.org/fhir/assert-operator-codes"; 391 case NOTEMPTY: return "http://hl7.org/fhir/assert-operator-codes"; 392 case CONTAINS: return "http://hl7.org/fhir/assert-operator-codes"; 393 case NOTCONTAINS: return "http://hl7.org/fhir/assert-operator-codes"; 394 case EVAL: return "http://hl7.org/fhir/assert-operator-codes"; 395 case NULL: return null; 396 default: return "?"; 397 } 398 } 399 public String getDefinition() { 400 switch (this) { 401 case EQUALS: return "Default value. Equals comparison."; 402 case NOTEQUALS: return "Not equals comparison."; 403 case IN: return "Compare value within a known set of values."; 404 case NOTIN: return "Compare value not within a known set of values."; 405 case GREATERTHAN: return "Compare value to be greater than a known value."; 406 case LESSTHAN: return "Compare value to be less than a known value."; 407 case EMPTY: return "Compare value is empty."; 408 case NOTEMPTY: return "Compare value is not empty."; 409 case CONTAINS: return "Compare value string contains a known value."; 410 case NOTCONTAINS: return "Compare value string does not contain a known value."; 411 case EVAL: return "Evaluate the fluentpath expression as a boolean condition."; 412 case NULL: return null; 413 default: return "?"; 414 } 415 } 416 public String getDisplay() { 417 switch (this) { 418 case EQUALS: return "equals"; 419 case NOTEQUALS: return "notEquals"; 420 case IN: return "in"; 421 case NOTIN: return "notIn"; 422 case GREATERTHAN: return "greaterThan"; 423 case LESSTHAN: return "lessThan"; 424 case EMPTY: return "empty"; 425 case NOTEMPTY: return "notEmpty"; 426 case CONTAINS: return "contains"; 427 case NOTCONTAINS: return "notContains"; 428 case EVAL: return "evaluate"; 429 case NULL: return null; 430 default: return "?"; 431 } 432 } 433 } 434 435 public static class AssertionOperatorTypeEnumFactory implements EnumFactory<AssertionOperatorType> { 436 public AssertionOperatorType fromCode(String codeString) throws IllegalArgumentException { 437 if (codeString == null || "".equals(codeString)) 438 if (codeString == null || "".equals(codeString)) 439 return null; 440 if ("equals".equals(codeString)) 441 return AssertionOperatorType.EQUALS; 442 if ("notEquals".equals(codeString)) 443 return AssertionOperatorType.NOTEQUALS; 444 if ("in".equals(codeString)) 445 return AssertionOperatorType.IN; 446 if ("notIn".equals(codeString)) 447 return AssertionOperatorType.NOTIN; 448 if ("greaterThan".equals(codeString)) 449 return AssertionOperatorType.GREATERTHAN; 450 if ("lessThan".equals(codeString)) 451 return AssertionOperatorType.LESSTHAN; 452 if ("empty".equals(codeString)) 453 return AssertionOperatorType.EMPTY; 454 if ("notEmpty".equals(codeString)) 455 return AssertionOperatorType.NOTEMPTY; 456 if ("contains".equals(codeString)) 457 return AssertionOperatorType.CONTAINS; 458 if ("notContains".equals(codeString)) 459 return AssertionOperatorType.NOTCONTAINS; 460 if ("eval".equals(codeString)) 461 return AssertionOperatorType.EVAL; 462 throw new IllegalArgumentException("Unknown AssertionOperatorType code '"+codeString+"'"); 463 } 464 public Enumeration<AssertionOperatorType> fromType(PrimitiveType<?> code) throws FHIRException { 465 if (code == null) 466 return null; 467 if (code.isEmpty()) 468 return new Enumeration<AssertionOperatorType>(this); 469 String codeString = code.asStringValue(); 470 if (codeString == null || "".equals(codeString)) 471 return null; 472 if ("equals".equals(codeString)) 473 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EQUALS); 474 if ("notEquals".equals(codeString)) 475 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTEQUALS); 476 if ("in".equals(codeString)) 477 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.IN); 478 if ("notIn".equals(codeString)) 479 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTIN); 480 if ("greaterThan".equals(codeString)) 481 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.GREATERTHAN); 482 if ("lessThan".equals(codeString)) 483 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.LESSTHAN); 484 if ("empty".equals(codeString)) 485 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EMPTY); 486 if ("notEmpty".equals(codeString)) 487 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTEMPTY); 488 if ("contains".equals(codeString)) 489 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.CONTAINS); 490 if ("notContains".equals(codeString)) 491 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTCONTAINS); 492 if ("eval".equals(codeString)) 493 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EVAL); 494 throw new FHIRException("Unknown AssertionOperatorType code '"+codeString+"'"); 495 } 496 public String toCode(AssertionOperatorType code) { 497 if (code == AssertionOperatorType.NULL) 498 return null; 499 if (code == AssertionOperatorType.EQUALS) 500 return "equals"; 501 if (code == AssertionOperatorType.NOTEQUALS) 502 return "notEquals"; 503 if (code == AssertionOperatorType.IN) 504 return "in"; 505 if (code == AssertionOperatorType.NOTIN) 506 return "notIn"; 507 if (code == AssertionOperatorType.GREATERTHAN) 508 return "greaterThan"; 509 if (code == AssertionOperatorType.LESSTHAN) 510 return "lessThan"; 511 if (code == AssertionOperatorType.EMPTY) 512 return "empty"; 513 if (code == AssertionOperatorType.NOTEMPTY) 514 return "notEmpty"; 515 if (code == AssertionOperatorType.CONTAINS) 516 return "contains"; 517 if (code == AssertionOperatorType.NOTCONTAINS) 518 return "notContains"; 519 if (code == AssertionOperatorType.EVAL) 520 return "eval"; 521 return "?"; 522 } 523 public String toSystem(AssertionOperatorType code) { 524 return code.getSystem(); 525 } 526 } 527 528 public enum TestScriptRequestMethodCode { 529 /** 530 * HTTP DELETE operation 531 */ 532 DELETE, 533 /** 534 * HTTP GET operation 535 */ 536 GET, 537 /** 538 * HTTP OPTIONS operation 539 */ 540 OPTIONS, 541 /** 542 * HTTP PATCH operation 543 */ 544 PATCH, 545 /** 546 * HTTP POST operation 547 */ 548 POST, 549 /** 550 * HTTP PUT operation 551 */ 552 PUT, 553 /** 554 * added to help the parsers with the generic types 555 */ 556 NULL; 557 public static TestScriptRequestMethodCode fromCode(String codeString) throws FHIRException { 558 if (codeString == null || "".equals(codeString)) 559 return null; 560 if ("delete".equals(codeString)) 561 return DELETE; 562 if ("get".equals(codeString)) 563 return GET; 564 if ("options".equals(codeString)) 565 return OPTIONS; 566 if ("patch".equals(codeString)) 567 return PATCH; 568 if ("post".equals(codeString)) 569 return POST; 570 if ("put".equals(codeString)) 571 return PUT; 572 if (Configuration.isAcceptInvalidEnums()) 573 return null; 574 else 575 throw new FHIRException("Unknown TestScriptRequestMethodCode code '"+codeString+"'"); 576 } 577 public String toCode() { 578 switch (this) { 579 case DELETE: return "delete"; 580 case GET: return "get"; 581 case OPTIONS: return "options"; 582 case PATCH: return "patch"; 583 case POST: return "post"; 584 case PUT: return "put"; 585 case NULL: return null; 586 default: return "?"; 587 } 588 } 589 public String getSystem() { 590 switch (this) { 591 case DELETE: return "http://hl7.org/fhir/http-operations"; 592 case GET: return "http://hl7.org/fhir/http-operations"; 593 case OPTIONS: return "http://hl7.org/fhir/http-operations"; 594 case PATCH: return "http://hl7.org/fhir/http-operations"; 595 case POST: return "http://hl7.org/fhir/http-operations"; 596 case PUT: return "http://hl7.org/fhir/http-operations"; 597 case NULL: return null; 598 default: return "?"; 599 } 600 } 601 public String getDefinition() { 602 switch (this) { 603 case DELETE: return "HTTP DELETE operation"; 604 case GET: return "HTTP GET operation"; 605 case OPTIONS: return "HTTP OPTIONS operation"; 606 case PATCH: return "HTTP PATCH operation"; 607 case POST: return "HTTP POST operation"; 608 case PUT: return "HTTP PUT operation"; 609 case NULL: return null; 610 default: return "?"; 611 } 612 } 613 public String getDisplay() { 614 switch (this) { 615 case DELETE: return "DELETE"; 616 case GET: return "GET"; 617 case OPTIONS: return "OPTIONS"; 618 case PATCH: return "PATCH"; 619 case POST: return "POST"; 620 case PUT: return "PUT"; 621 case NULL: return null; 622 default: return "?"; 623 } 624 } 625 } 626 627 public static class TestScriptRequestMethodCodeEnumFactory implements EnumFactory<TestScriptRequestMethodCode> { 628 public TestScriptRequestMethodCode fromCode(String codeString) throws IllegalArgumentException { 629 if (codeString == null || "".equals(codeString)) 630 if (codeString == null || "".equals(codeString)) 631 return null; 632 if ("delete".equals(codeString)) 633 return TestScriptRequestMethodCode.DELETE; 634 if ("get".equals(codeString)) 635 return TestScriptRequestMethodCode.GET; 636 if ("options".equals(codeString)) 637 return TestScriptRequestMethodCode.OPTIONS; 638 if ("patch".equals(codeString)) 639 return TestScriptRequestMethodCode.PATCH; 640 if ("post".equals(codeString)) 641 return TestScriptRequestMethodCode.POST; 642 if ("put".equals(codeString)) 643 return TestScriptRequestMethodCode.PUT; 644 throw new IllegalArgumentException("Unknown TestScriptRequestMethodCode code '"+codeString+"'"); 645 } 646 public Enumeration<TestScriptRequestMethodCode> fromType(PrimitiveType<?> code) throws FHIRException { 647 if (code == null) 648 return null; 649 if (code.isEmpty()) 650 return new Enumeration<TestScriptRequestMethodCode>(this); 651 String codeString = code.asStringValue(); 652 if (codeString == null || "".equals(codeString)) 653 return null; 654 if ("delete".equals(codeString)) 655 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.DELETE); 656 if ("get".equals(codeString)) 657 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.GET); 658 if ("options".equals(codeString)) 659 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.OPTIONS); 660 if ("patch".equals(codeString)) 661 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.PATCH); 662 if ("post".equals(codeString)) 663 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.POST); 664 if ("put".equals(codeString)) 665 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.PUT); 666 throw new FHIRException("Unknown TestScriptRequestMethodCode code '"+codeString+"'"); 667 } 668 public String toCode(TestScriptRequestMethodCode code) { 669 if (code == TestScriptRequestMethodCode.NULL) 670 return null; 671 if (code == TestScriptRequestMethodCode.DELETE) 672 return "delete"; 673 if (code == TestScriptRequestMethodCode.GET) 674 return "get"; 675 if (code == TestScriptRequestMethodCode.OPTIONS) 676 return "options"; 677 if (code == TestScriptRequestMethodCode.PATCH) 678 return "patch"; 679 if (code == TestScriptRequestMethodCode.POST) 680 return "post"; 681 if (code == TestScriptRequestMethodCode.PUT) 682 return "put"; 683 return "?"; 684 } 685 public String toSystem(TestScriptRequestMethodCode code) { 686 return code.getSystem(); 687 } 688 } 689 690 public enum AssertionResponseTypes { 691 /** 692 * Response code is 200. 693 */ 694 OKAY, 695 /** 696 * Response code is 201. 697 */ 698 CREATED, 699 /** 700 * Response code is 204. 701 */ 702 NOCONTENT, 703 /** 704 * Response code is 304. 705 */ 706 NOTMODIFIED, 707 /** 708 * Response code is 400. 709 */ 710 BAD, 711 /** 712 * Response code is 403. 713 */ 714 FORBIDDEN, 715 /** 716 * Response code is 404. 717 */ 718 NOTFOUND, 719 /** 720 * Response code is 405. 721 */ 722 METHODNOTALLOWED, 723 /** 724 * Response code is 409. 725 */ 726 CONFLICT, 727 /** 728 * Response code is 410. 729 */ 730 GONE, 731 /** 732 * Response code is 412. 733 */ 734 PRECONDITIONFAILED, 735 /** 736 * Response code is 422. 737 */ 738 UNPROCESSABLE, 739 /** 740 * added to help the parsers with the generic types 741 */ 742 NULL; 743 public static AssertionResponseTypes fromCode(String codeString) throws FHIRException { 744 if (codeString == null || "".equals(codeString)) 745 return null; 746 if ("okay".equals(codeString)) 747 return OKAY; 748 if ("created".equals(codeString)) 749 return CREATED; 750 if ("noContent".equals(codeString)) 751 return NOCONTENT; 752 if ("notModified".equals(codeString)) 753 return NOTMODIFIED; 754 if ("bad".equals(codeString)) 755 return BAD; 756 if ("forbidden".equals(codeString)) 757 return FORBIDDEN; 758 if ("notFound".equals(codeString)) 759 return NOTFOUND; 760 if ("methodNotAllowed".equals(codeString)) 761 return METHODNOTALLOWED; 762 if ("conflict".equals(codeString)) 763 return CONFLICT; 764 if ("gone".equals(codeString)) 765 return GONE; 766 if ("preconditionFailed".equals(codeString)) 767 return PRECONDITIONFAILED; 768 if ("unprocessable".equals(codeString)) 769 return UNPROCESSABLE; 770 if (Configuration.isAcceptInvalidEnums()) 771 return null; 772 else 773 throw new FHIRException("Unknown AssertionResponseTypes code '"+codeString+"'"); 774 } 775 public String toCode() { 776 switch (this) { 777 case OKAY: return "okay"; 778 case CREATED: return "created"; 779 case NOCONTENT: return "noContent"; 780 case NOTMODIFIED: return "notModified"; 781 case BAD: return "bad"; 782 case FORBIDDEN: return "forbidden"; 783 case NOTFOUND: return "notFound"; 784 case METHODNOTALLOWED: return "methodNotAllowed"; 785 case CONFLICT: return "conflict"; 786 case GONE: return "gone"; 787 case PRECONDITIONFAILED: return "preconditionFailed"; 788 case UNPROCESSABLE: return "unprocessable"; 789 case NULL: return null; 790 default: return "?"; 791 } 792 } 793 public String getSystem() { 794 switch (this) { 795 case OKAY: return "http://hl7.org/fhir/assert-response-code-types"; 796 case CREATED: return "http://hl7.org/fhir/assert-response-code-types"; 797 case NOCONTENT: return "http://hl7.org/fhir/assert-response-code-types"; 798 case NOTMODIFIED: return "http://hl7.org/fhir/assert-response-code-types"; 799 case BAD: return "http://hl7.org/fhir/assert-response-code-types"; 800 case FORBIDDEN: return "http://hl7.org/fhir/assert-response-code-types"; 801 case NOTFOUND: return "http://hl7.org/fhir/assert-response-code-types"; 802 case METHODNOTALLOWED: return "http://hl7.org/fhir/assert-response-code-types"; 803 case CONFLICT: return "http://hl7.org/fhir/assert-response-code-types"; 804 case GONE: return "http://hl7.org/fhir/assert-response-code-types"; 805 case PRECONDITIONFAILED: return "http://hl7.org/fhir/assert-response-code-types"; 806 case UNPROCESSABLE: return "http://hl7.org/fhir/assert-response-code-types"; 807 case NULL: return null; 808 default: return "?"; 809 } 810 } 811 public String getDefinition() { 812 switch (this) { 813 case OKAY: return "Response code is 200."; 814 case CREATED: return "Response code is 201."; 815 case NOCONTENT: return "Response code is 204."; 816 case NOTMODIFIED: return "Response code is 304."; 817 case BAD: return "Response code is 400."; 818 case FORBIDDEN: return "Response code is 403."; 819 case NOTFOUND: return "Response code is 404."; 820 case METHODNOTALLOWED: return "Response code is 405."; 821 case CONFLICT: return "Response code is 409."; 822 case GONE: return "Response code is 410."; 823 case PRECONDITIONFAILED: return "Response code is 412."; 824 case UNPROCESSABLE: return "Response code is 422."; 825 case NULL: return null; 826 default: return "?"; 827 } 828 } 829 public String getDisplay() { 830 switch (this) { 831 case OKAY: return "okay"; 832 case CREATED: return "created"; 833 case NOCONTENT: return "noContent"; 834 case NOTMODIFIED: return "notModified"; 835 case BAD: return "bad"; 836 case FORBIDDEN: return "forbidden"; 837 case NOTFOUND: return "notFound"; 838 case METHODNOTALLOWED: return "methodNotAllowed"; 839 case CONFLICT: return "conflict"; 840 case GONE: return "gone"; 841 case PRECONDITIONFAILED: return "preconditionFailed"; 842 case UNPROCESSABLE: return "unprocessable"; 843 case NULL: return null; 844 default: return "?"; 845 } 846 } 847 } 848 849 public static class AssertionResponseTypesEnumFactory implements EnumFactory<AssertionResponseTypes> { 850 public AssertionResponseTypes fromCode(String codeString) throws IllegalArgumentException { 851 if (codeString == null || "".equals(codeString)) 852 if (codeString == null || "".equals(codeString)) 853 return null; 854 if ("okay".equals(codeString)) 855 return AssertionResponseTypes.OKAY; 856 if ("created".equals(codeString)) 857 return AssertionResponseTypes.CREATED; 858 if ("noContent".equals(codeString)) 859 return AssertionResponseTypes.NOCONTENT; 860 if ("notModified".equals(codeString)) 861 return AssertionResponseTypes.NOTMODIFIED; 862 if ("bad".equals(codeString)) 863 return AssertionResponseTypes.BAD; 864 if ("forbidden".equals(codeString)) 865 return AssertionResponseTypes.FORBIDDEN; 866 if ("notFound".equals(codeString)) 867 return AssertionResponseTypes.NOTFOUND; 868 if ("methodNotAllowed".equals(codeString)) 869 return AssertionResponseTypes.METHODNOTALLOWED; 870 if ("conflict".equals(codeString)) 871 return AssertionResponseTypes.CONFLICT; 872 if ("gone".equals(codeString)) 873 return AssertionResponseTypes.GONE; 874 if ("preconditionFailed".equals(codeString)) 875 return AssertionResponseTypes.PRECONDITIONFAILED; 876 if ("unprocessable".equals(codeString)) 877 return AssertionResponseTypes.UNPROCESSABLE; 878 throw new IllegalArgumentException("Unknown AssertionResponseTypes code '"+codeString+"'"); 879 } 880 public Enumeration<AssertionResponseTypes> fromType(PrimitiveType<?> code) throws FHIRException { 881 if (code == null) 882 return null; 883 if (code.isEmpty()) 884 return new Enumeration<AssertionResponseTypes>(this); 885 String codeString = code.asStringValue(); 886 if (codeString == null || "".equals(codeString)) 887 return null; 888 if ("okay".equals(codeString)) 889 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.OKAY); 890 if ("created".equals(codeString)) 891 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.CREATED); 892 if ("noContent".equals(codeString)) 893 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOCONTENT); 894 if ("notModified".equals(codeString)) 895 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOTMODIFIED); 896 if ("bad".equals(codeString)) 897 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.BAD); 898 if ("forbidden".equals(codeString)) 899 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.FORBIDDEN); 900 if ("notFound".equals(codeString)) 901 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOTFOUND); 902 if ("methodNotAllowed".equals(codeString)) 903 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.METHODNOTALLOWED); 904 if ("conflict".equals(codeString)) 905 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.CONFLICT); 906 if ("gone".equals(codeString)) 907 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.GONE); 908 if ("preconditionFailed".equals(codeString)) 909 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.PRECONDITIONFAILED); 910 if ("unprocessable".equals(codeString)) 911 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.UNPROCESSABLE); 912 throw new FHIRException("Unknown AssertionResponseTypes code '"+codeString+"'"); 913 } 914 public String toCode(AssertionResponseTypes code) { 915 if (code == AssertionResponseTypes.NULL) 916 return null; 917 if (code == AssertionResponseTypes.OKAY) 918 return "okay"; 919 if (code == AssertionResponseTypes.CREATED) 920 return "created"; 921 if (code == AssertionResponseTypes.NOCONTENT) 922 return "noContent"; 923 if (code == AssertionResponseTypes.NOTMODIFIED) 924 return "notModified"; 925 if (code == AssertionResponseTypes.BAD) 926 return "bad"; 927 if (code == AssertionResponseTypes.FORBIDDEN) 928 return "forbidden"; 929 if (code == AssertionResponseTypes.NOTFOUND) 930 return "notFound"; 931 if (code == AssertionResponseTypes.METHODNOTALLOWED) 932 return "methodNotAllowed"; 933 if (code == AssertionResponseTypes.CONFLICT) 934 return "conflict"; 935 if (code == AssertionResponseTypes.GONE) 936 return "gone"; 937 if (code == AssertionResponseTypes.PRECONDITIONFAILED) 938 return "preconditionFailed"; 939 if (code == AssertionResponseTypes.UNPROCESSABLE) 940 return "unprocessable"; 941 return "?"; 942 } 943 public String toSystem(AssertionResponseTypes code) { 944 return code.getSystem(); 945 } 946 } 947 948 @Block() 949 public static class TestScriptOriginComponent extends BackboneElement implements IBaseBackboneElement { 950 /** 951 * Abstract name given to an origin server in this test script. The name is provided as a number starting at 1. 952 */ 953 @Child(name = "index", type = {IntegerType.class}, order=1, min=1, max=1, modifier=false, summary=false) 954 @Description(shortDefinition="The index of the abstract origin server starting at 1", formalDefinition="Abstract name given to an origin server in this test script. The name is provided as a number starting at 1." ) 955 protected IntegerType index; 956 957 /** 958 * The type of origin profile the test system supports. 959 */ 960 @Child(name = "profile", type = {Coding.class}, order=2, min=1, max=1, modifier=false, summary=false) 961 @Description(shortDefinition="FHIR-Client | FHIR-SDC-FormFiller", formalDefinition="The type of origin profile the test system supports." ) 962 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/testscript-profile-origin-types") 963 protected Coding profile; 964 965 private static final long serialVersionUID = -1239935149L; 966 967 /** 968 * Constructor 969 */ 970 public TestScriptOriginComponent() { 971 super(); 972 } 973 974 /** 975 * Constructor 976 */ 977 public TestScriptOriginComponent(IntegerType index, Coding profile) { 978 super(); 979 this.index = index; 980 this.profile = profile; 981 } 982 983 /** 984 * @return {@link #index} (Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.). This is the underlying object with id, value and extensions. The accessor "getIndex" gives direct access to the value 985 */ 986 public IntegerType getIndexElement() { 987 if (this.index == null) 988 if (Configuration.errorOnAutoCreate()) 989 throw new Error("Attempt to auto-create TestScriptOriginComponent.index"); 990 else if (Configuration.doAutoCreate()) 991 this.index = new IntegerType(); // bb 992 return this.index; 993 } 994 995 public boolean hasIndexElement() { 996 return this.index != null && !this.index.isEmpty(); 997 } 998 999 public boolean hasIndex() { 1000 return this.index != null && !this.index.isEmpty(); 1001 } 1002 1003 /** 1004 * @param value {@link #index} (Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.). This is the underlying object with id, value and extensions. The accessor "getIndex" gives direct access to the value 1005 */ 1006 public TestScriptOriginComponent setIndexElement(IntegerType value) { 1007 this.index = value; 1008 return this; 1009 } 1010 1011 /** 1012 * @return Abstract name given to an origin server in this test script. The name is provided as a number starting at 1. 1013 */ 1014 public int getIndex() { 1015 return this.index == null || this.index.isEmpty() ? 0 : this.index.getValue(); 1016 } 1017 1018 /** 1019 * @param value Abstract name given to an origin server in this test script. The name is provided as a number starting at 1. 1020 */ 1021 public TestScriptOriginComponent setIndex(int value) { 1022 if (this.index == null) 1023 this.index = new IntegerType(); 1024 this.index.setValue(value); 1025 return this; 1026 } 1027 1028 /** 1029 * @return {@link #profile} (The type of origin profile the test system supports.) 1030 */ 1031 public Coding getProfile() { 1032 if (this.profile == null) 1033 if (Configuration.errorOnAutoCreate()) 1034 throw new Error("Attempt to auto-create TestScriptOriginComponent.profile"); 1035 else if (Configuration.doAutoCreate()) 1036 this.profile = new Coding(); // cc 1037 return this.profile; 1038 } 1039 1040 public boolean hasProfile() { 1041 return this.profile != null && !this.profile.isEmpty(); 1042 } 1043 1044 /** 1045 * @param value {@link #profile} (The type of origin profile the test system supports.) 1046 */ 1047 public TestScriptOriginComponent setProfile(Coding value) { 1048 this.profile = value; 1049 return this; 1050 } 1051 1052 protected void listChildren(List<Property> children) { 1053 super.listChildren(children); 1054 children.add(new Property("index", "integer", "Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.", 0, 1, index)); 1055 children.add(new Property("profile", "Coding", "The type of origin profile the test system supports.", 0, 1, profile)); 1056 } 1057 1058 @Override 1059 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1060 switch (_hash) { 1061 case 100346066: /*index*/ return new Property("index", "integer", "Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.", 0, 1, index); 1062 case -309425751: /*profile*/ return new Property("profile", "Coding", "The type of origin profile the test system supports.", 0, 1, profile); 1063 default: return super.getNamedProperty(_hash, _name, _checkValid); 1064 } 1065 1066 } 1067 1068 @Override 1069 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1070 switch (hash) { 1071 case 100346066: /*index*/ return this.index == null ? new Base[0] : new Base[] {this.index}; // IntegerType 1072 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // Coding 1073 default: return super.getProperty(hash, name, checkValid); 1074 } 1075 1076 } 1077 1078 @Override 1079 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1080 switch (hash) { 1081 case 100346066: // index 1082 this.index = castToInteger(value); // IntegerType 1083 return value; 1084 case -309425751: // profile 1085 this.profile = castToCoding(value); // Coding 1086 return value; 1087 default: return super.setProperty(hash, name, value); 1088 } 1089 1090 } 1091 1092 @Override 1093 public Base setProperty(String name, Base value) throws FHIRException { 1094 if (name.equals("index")) { 1095 this.index = castToInteger(value); // IntegerType 1096 } else if (name.equals("profile")) { 1097 this.profile = castToCoding(value); // Coding 1098 } else 1099 return super.setProperty(name, value); 1100 return value; 1101 } 1102 1103 @Override 1104 public Base makeProperty(int hash, String name) throws FHIRException { 1105 switch (hash) { 1106 case 100346066: return getIndexElement(); 1107 case -309425751: return getProfile(); 1108 default: return super.makeProperty(hash, name); 1109 } 1110 1111 } 1112 1113 @Override 1114 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1115 switch (hash) { 1116 case 100346066: /*index*/ return new String[] {"integer"}; 1117 case -309425751: /*profile*/ return new String[] {"Coding"}; 1118 default: return super.getTypesForProperty(hash, name); 1119 } 1120 1121 } 1122 1123 @Override 1124 public Base addChild(String name) throws FHIRException { 1125 if (name.equals("index")) { 1126 throw new FHIRException("Cannot call addChild on a singleton property TestScript.index"); 1127 } 1128 else if (name.equals("profile")) { 1129 this.profile = new Coding(); 1130 return this.profile; 1131 } 1132 else 1133 return super.addChild(name); 1134 } 1135 1136 public TestScriptOriginComponent copy() { 1137 TestScriptOriginComponent dst = new TestScriptOriginComponent(); 1138 copyValues(dst); 1139 dst.index = index == null ? null : index.copy(); 1140 dst.profile = profile == null ? null : profile.copy(); 1141 return dst; 1142 } 1143 1144 @Override 1145 public boolean equalsDeep(Base other_) { 1146 if (!super.equalsDeep(other_)) 1147 return false; 1148 if (!(other_ instanceof TestScriptOriginComponent)) 1149 return false; 1150 TestScriptOriginComponent o = (TestScriptOriginComponent) other_; 1151 return compareDeep(index, o.index, true) && compareDeep(profile, o.profile, true); 1152 } 1153 1154 @Override 1155 public boolean equalsShallow(Base other_) { 1156 if (!super.equalsShallow(other_)) 1157 return false; 1158 if (!(other_ instanceof TestScriptOriginComponent)) 1159 return false; 1160 TestScriptOriginComponent o = (TestScriptOriginComponent) other_; 1161 return compareValues(index, o.index, true); 1162 } 1163 1164 public boolean isEmpty() { 1165 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(index, profile); 1166 } 1167 1168 public String fhirType() { 1169 return "TestScript.origin"; 1170 1171 } 1172 1173 } 1174 1175 @Block() 1176 public static class TestScriptDestinationComponent extends BackboneElement implements IBaseBackboneElement { 1177 /** 1178 * Abstract name given to a destination server in this test script. The name is provided as a number starting at 1. 1179 */ 1180 @Child(name = "index", type = {IntegerType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1181 @Description(shortDefinition="The index of the abstract destination server starting at 1", formalDefinition="Abstract name given to a destination server in this test script. The name is provided as a number starting at 1." ) 1182 protected IntegerType index; 1183 1184 /** 1185 * The type of destination profile the test system supports. 1186 */ 1187 @Child(name = "profile", type = {Coding.class}, order=2, min=1, max=1, modifier=false, summary=false) 1188 @Description(shortDefinition="FHIR-Server | FHIR-SDC-FormManager | FHIR-SDC-FormReceiver | FHIR-SDC-FormProcessor", formalDefinition="The type of destination profile the test system supports." ) 1189 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/testscript-profile-destination-types") 1190 protected Coding profile; 1191 1192 private static final long serialVersionUID = -1239935149L; 1193 1194 /** 1195 * Constructor 1196 */ 1197 public TestScriptDestinationComponent() { 1198 super(); 1199 } 1200 1201 /** 1202 * Constructor 1203 */ 1204 public TestScriptDestinationComponent(IntegerType index, Coding profile) { 1205 super(); 1206 this.index = index; 1207 this.profile = profile; 1208 } 1209 1210 /** 1211 * @return {@link #index} (Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.). This is the underlying object with id, value and extensions. The accessor "getIndex" gives direct access to the value 1212 */ 1213 public IntegerType getIndexElement() { 1214 if (this.index == null) 1215 if (Configuration.errorOnAutoCreate()) 1216 throw new Error("Attempt to auto-create TestScriptDestinationComponent.index"); 1217 else if (Configuration.doAutoCreate()) 1218 this.index = new IntegerType(); // bb 1219 return this.index; 1220 } 1221 1222 public boolean hasIndexElement() { 1223 return this.index != null && !this.index.isEmpty(); 1224 } 1225 1226 public boolean hasIndex() { 1227 return this.index != null && !this.index.isEmpty(); 1228 } 1229 1230 /** 1231 * @param value {@link #index} (Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.). This is the underlying object with id, value and extensions. The accessor "getIndex" gives direct access to the value 1232 */ 1233 public TestScriptDestinationComponent setIndexElement(IntegerType value) { 1234 this.index = value; 1235 return this; 1236 } 1237 1238 /** 1239 * @return Abstract name given to a destination server in this test script. The name is provided as a number starting at 1. 1240 */ 1241 public int getIndex() { 1242 return this.index == null || this.index.isEmpty() ? 0 : this.index.getValue(); 1243 } 1244 1245 /** 1246 * @param value Abstract name given to a destination server in this test script. The name is provided as a number starting at 1. 1247 */ 1248 public TestScriptDestinationComponent setIndex(int value) { 1249 if (this.index == null) 1250 this.index = new IntegerType(); 1251 this.index.setValue(value); 1252 return this; 1253 } 1254 1255 /** 1256 * @return {@link #profile} (The type of destination profile the test system supports.) 1257 */ 1258 public Coding getProfile() { 1259 if (this.profile == null) 1260 if (Configuration.errorOnAutoCreate()) 1261 throw new Error("Attempt to auto-create TestScriptDestinationComponent.profile"); 1262 else if (Configuration.doAutoCreate()) 1263 this.profile = new Coding(); // cc 1264 return this.profile; 1265 } 1266 1267 public boolean hasProfile() { 1268 return this.profile != null && !this.profile.isEmpty(); 1269 } 1270 1271 /** 1272 * @param value {@link #profile} (The type of destination profile the test system supports.) 1273 */ 1274 public TestScriptDestinationComponent setProfile(Coding value) { 1275 this.profile = value; 1276 return this; 1277 } 1278 1279 protected void listChildren(List<Property> children) { 1280 super.listChildren(children); 1281 children.add(new Property("index", "integer", "Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.", 0, 1, index)); 1282 children.add(new Property("profile", "Coding", "The type of destination profile the test system supports.", 0, 1, profile)); 1283 } 1284 1285 @Override 1286 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1287 switch (_hash) { 1288 case 100346066: /*index*/ return new Property("index", "integer", "Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.", 0, 1, index); 1289 case -309425751: /*profile*/ return new Property("profile", "Coding", "The type of destination profile the test system supports.", 0, 1, profile); 1290 default: return super.getNamedProperty(_hash, _name, _checkValid); 1291 } 1292 1293 } 1294 1295 @Override 1296 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1297 switch (hash) { 1298 case 100346066: /*index*/ return this.index == null ? new Base[0] : new Base[] {this.index}; // IntegerType 1299 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // Coding 1300 default: return super.getProperty(hash, name, checkValid); 1301 } 1302 1303 } 1304 1305 @Override 1306 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1307 switch (hash) { 1308 case 100346066: // index 1309 this.index = castToInteger(value); // IntegerType 1310 return value; 1311 case -309425751: // profile 1312 this.profile = castToCoding(value); // Coding 1313 return value; 1314 default: return super.setProperty(hash, name, value); 1315 } 1316 1317 } 1318 1319 @Override 1320 public Base setProperty(String name, Base value) throws FHIRException { 1321 if (name.equals("index")) { 1322 this.index = castToInteger(value); // IntegerType 1323 } else if (name.equals("profile")) { 1324 this.profile = castToCoding(value); // Coding 1325 } else 1326 return super.setProperty(name, value); 1327 return value; 1328 } 1329 1330 @Override 1331 public Base makeProperty(int hash, String name) throws FHIRException { 1332 switch (hash) { 1333 case 100346066: return getIndexElement(); 1334 case -309425751: return getProfile(); 1335 default: return super.makeProperty(hash, name); 1336 } 1337 1338 } 1339 1340 @Override 1341 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1342 switch (hash) { 1343 case 100346066: /*index*/ return new String[] {"integer"}; 1344 case -309425751: /*profile*/ return new String[] {"Coding"}; 1345 default: return super.getTypesForProperty(hash, name); 1346 } 1347 1348 } 1349 1350 @Override 1351 public Base addChild(String name) throws FHIRException { 1352 if (name.equals("index")) { 1353 throw new FHIRException("Cannot call addChild on a singleton property TestScript.index"); 1354 } 1355 else if (name.equals("profile")) { 1356 this.profile = new Coding(); 1357 return this.profile; 1358 } 1359 else 1360 return super.addChild(name); 1361 } 1362 1363 public TestScriptDestinationComponent copy() { 1364 TestScriptDestinationComponent dst = new TestScriptDestinationComponent(); 1365 copyValues(dst); 1366 dst.index = index == null ? null : index.copy(); 1367 dst.profile = profile == null ? null : profile.copy(); 1368 return dst; 1369 } 1370 1371 @Override 1372 public boolean equalsDeep(Base other_) { 1373 if (!super.equalsDeep(other_)) 1374 return false; 1375 if (!(other_ instanceof TestScriptDestinationComponent)) 1376 return false; 1377 TestScriptDestinationComponent o = (TestScriptDestinationComponent) other_; 1378 return compareDeep(index, o.index, true) && compareDeep(profile, o.profile, true); 1379 } 1380 1381 @Override 1382 public boolean equalsShallow(Base other_) { 1383 if (!super.equalsShallow(other_)) 1384 return false; 1385 if (!(other_ instanceof TestScriptDestinationComponent)) 1386 return false; 1387 TestScriptDestinationComponent o = (TestScriptDestinationComponent) other_; 1388 return compareValues(index, o.index, true); 1389 } 1390 1391 public boolean isEmpty() { 1392 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(index, profile); 1393 } 1394 1395 public String fhirType() { 1396 return "TestScript.destination"; 1397 1398 } 1399 1400 } 1401 1402 @Block() 1403 public static class TestScriptMetadataComponent extends BackboneElement implements IBaseBackboneElement { 1404 /** 1405 * A link to the FHIR specification that this test is covering. 1406 */ 1407 @Child(name = "link", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1408 @Description(shortDefinition="Links to the FHIR specification", formalDefinition="A link to the FHIR specification that this test is covering." ) 1409 protected List<TestScriptMetadataLinkComponent> link; 1410 1411 /** 1412 * Capabilities that must exist and are assumed to function correctly on the FHIR server being tested. 1413 */ 1414 @Child(name = "capability", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1415 @Description(shortDefinition="Capabilities that are assumed to function correctly on the FHIR server being tested", formalDefinition="Capabilities that must exist and are assumed to function correctly on the FHIR server being tested." ) 1416 protected List<TestScriptMetadataCapabilityComponent> capability; 1417 1418 private static final long serialVersionUID = 745183328L; 1419 1420 /** 1421 * Constructor 1422 */ 1423 public TestScriptMetadataComponent() { 1424 super(); 1425 } 1426 1427 /** 1428 * @return {@link #link} (A link to the FHIR specification that this test is covering.) 1429 */ 1430 public List<TestScriptMetadataLinkComponent> getLink() { 1431 if (this.link == null) 1432 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1433 return this.link; 1434 } 1435 1436 /** 1437 * @return Returns a reference to <code>this</code> for easy method chaining 1438 */ 1439 public TestScriptMetadataComponent setLink(List<TestScriptMetadataLinkComponent> theLink) { 1440 this.link = theLink; 1441 return this; 1442 } 1443 1444 public boolean hasLink() { 1445 if (this.link == null) 1446 return false; 1447 for (TestScriptMetadataLinkComponent item : this.link) 1448 if (!item.isEmpty()) 1449 return true; 1450 return false; 1451 } 1452 1453 public TestScriptMetadataLinkComponent addLink() { //3 1454 TestScriptMetadataLinkComponent t = new TestScriptMetadataLinkComponent(); 1455 if (this.link == null) 1456 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1457 this.link.add(t); 1458 return t; 1459 } 1460 1461 public TestScriptMetadataComponent addLink(TestScriptMetadataLinkComponent t) { //3 1462 if (t == null) 1463 return this; 1464 if (this.link == null) 1465 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1466 this.link.add(t); 1467 return this; 1468 } 1469 1470 /** 1471 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist 1472 */ 1473 public TestScriptMetadataLinkComponent getLinkFirstRep() { 1474 if (getLink().isEmpty()) { 1475 addLink(); 1476 } 1477 return getLink().get(0); 1478 } 1479 1480 /** 1481 * @return {@link #capability} (Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.) 1482 */ 1483 public List<TestScriptMetadataCapabilityComponent> getCapability() { 1484 if (this.capability == null) 1485 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1486 return this.capability; 1487 } 1488 1489 /** 1490 * @return Returns a reference to <code>this</code> for easy method chaining 1491 */ 1492 public TestScriptMetadataComponent setCapability(List<TestScriptMetadataCapabilityComponent> theCapability) { 1493 this.capability = theCapability; 1494 return this; 1495 } 1496 1497 public boolean hasCapability() { 1498 if (this.capability == null) 1499 return false; 1500 for (TestScriptMetadataCapabilityComponent item : this.capability) 1501 if (!item.isEmpty()) 1502 return true; 1503 return false; 1504 } 1505 1506 public TestScriptMetadataCapabilityComponent addCapability() { //3 1507 TestScriptMetadataCapabilityComponent t = new TestScriptMetadataCapabilityComponent(); 1508 if (this.capability == null) 1509 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1510 this.capability.add(t); 1511 return t; 1512 } 1513 1514 public TestScriptMetadataComponent addCapability(TestScriptMetadataCapabilityComponent t) { //3 1515 if (t == null) 1516 return this; 1517 if (this.capability == null) 1518 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1519 this.capability.add(t); 1520 return this; 1521 } 1522 1523 /** 1524 * @return The first repetition of repeating field {@link #capability}, creating it if it does not already exist 1525 */ 1526 public TestScriptMetadataCapabilityComponent getCapabilityFirstRep() { 1527 if (getCapability().isEmpty()) { 1528 addCapability(); 1529 } 1530 return getCapability().get(0); 1531 } 1532 1533 protected void listChildren(List<Property> children) { 1534 super.listChildren(children); 1535 children.add(new Property("link", "", "A link to the FHIR specification that this test is covering.", 0, java.lang.Integer.MAX_VALUE, link)); 1536 children.add(new Property("capability", "", "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.", 0, java.lang.Integer.MAX_VALUE, capability)); 1537 } 1538 1539 @Override 1540 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1541 switch (_hash) { 1542 case 3321850: /*link*/ return new Property("link", "", "A link to the FHIR specification that this test is covering.", 0, java.lang.Integer.MAX_VALUE, link); 1543 case -783669992: /*capability*/ return new Property("capability", "", "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.", 0, java.lang.Integer.MAX_VALUE, capability); 1544 default: return super.getNamedProperty(_hash, _name, _checkValid); 1545 } 1546 1547 } 1548 1549 @Override 1550 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1551 switch (hash) { 1552 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // TestScriptMetadataLinkComponent 1553 case -783669992: /*capability*/ return this.capability == null ? new Base[0] : this.capability.toArray(new Base[this.capability.size()]); // TestScriptMetadataCapabilityComponent 1554 default: return super.getProperty(hash, name, checkValid); 1555 } 1556 1557 } 1558 1559 @Override 1560 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1561 switch (hash) { 1562 case 3321850: // link 1563 this.getLink().add((TestScriptMetadataLinkComponent) value); // TestScriptMetadataLinkComponent 1564 return value; 1565 case -783669992: // capability 1566 this.getCapability().add((TestScriptMetadataCapabilityComponent) value); // TestScriptMetadataCapabilityComponent 1567 return value; 1568 default: return super.setProperty(hash, name, value); 1569 } 1570 1571 } 1572 1573 @Override 1574 public Base setProperty(String name, Base value) throws FHIRException { 1575 if (name.equals("link")) { 1576 this.getLink().add((TestScriptMetadataLinkComponent) value); 1577 } else if (name.equals("capability")) { 1578 this.getCapability().add((TestScriptMetadataCapabilityComponent) value); 1579 } else 1580 return super.setProperty(name, value); 1581 return value; 1582 } 1583 1584 @Override 1585 public Base makeProperty(int hash, String name) throws FHIRException { 1586 switch (hash) { 1587 case 3321850: return addLink(); 1588 case -783669992: return addCapability(); 1589 default: return super.makeProperty(hash, name); 1590 } 1591 1592 } 1593 1594 @Override 1595 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1596 switch (hash) { 1597 case 3321850: /*link*/ return new String[] {}; 1598 case -783669992: /*capability*/ return new String[] {}; 1599 default: return super.getTypesForProperty(hash, name); 1600 } 1601 1602 } 1603 1604 @Override 1605 public Base addChild(String name) throws FHIRException { 1606 if (name.equals("link")) { 1607 return addLink(); 1608 } 1609 else if (name.equals("capability")) { 1610 return addCapability(); 1611 } 1612 else 1613 return super.addChild(name); 1614 } 1615 1616 public TestScriptMetadataComponent copy() { 1617 TestScriptMetadataComponent dst = new TestScriptMetadataComponent(); 1618 copyValues(dst); 1619 if (link != null) { 1620 dst.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1621 for (TestScriptMetadataLinkComponent i : link) 1622 dst.link.add(i.copy()); 1623 }; 1624 if (capability != null) { 1625 dst.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1626 for (TestScriptMetadataCapabilityComponent i : capability) 1627 dst.capability.add(i.copy()); 1628 }; 1629 return dst; 1630 } 1631 1632 @Override 1633 public boolean equalsDeep(Base other_) { 1634 if (!super.equalsDeep(other_)) 1635 return false; 1636 if (!(other_ instanceof TestScriptMetadataComponent)) 1637 return false; 1638 TestScriptMetadataComponent o = (TestScriptMetadataComponent) other_; 1639 return compareDeep(link, o.link, true) && compareDeep(capability, o.capability, true); 1640 } 1641 1642 @Override 1643 public boolean equalsShallow(Base other_) { 1644 if (!super.equalsShallow(other_)) 1645 return false; 1646 if (!(other_ instanceof TestScriptMetadataComponent)) 1647 return false; 1648 TestScriptMetadataComponent o = (TestScriptMetadataComponent) other_; 1649 return true; 1650 } 1651 1652 public boolean isEmpty() { 1653 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(link, capability); 1654 } 1655 1656 public String fhirType() { 1657 return "TestScript.metadata"; 1658 1659 } 1660 1661 } 1662 1663 @Block() 1664 public static class TestScriptMetadataLinkComponent extends BackboneElement implements IBaseBackboneElement { 1665 /** 1666 * URL to a particular requirement or feature within the FHIR specification. 1667 */ 1668 @Child(name = "url", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1669 @Description(shortDefinition="URL to the specification", formalDefinition="URL to a particular requirement or feature within the FHIR specification." ) 1670 protected UriType url; 1671 1672 /** 1673 * Short description of the link. 1674 */ 1675 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1676 @Description(shortDefinition="Short description", formalDefinition="Short description of the link." ) 1677 protected StringType description; 1678 1679 private static final long serialVersionUID = 213372298L; 1680 1681 /** 1682 * Constructor 1683 */ 1684 public TestScriptMetadataLinkComponent() { 1685 super(); 1686 } 1687 1688 /** 1689 * Constructor 1690 */ 1691 public TestScriptMetadataLinkComponent(UriType url) { 1692 super(); 1693 this.url = url; 1694 } 1695 1696 /** 1697 * @return {@link #url} (URL to a particular requirement or feature within the FHIR specification.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1698 */ 1699 public UriType getUrlElement() { 1700 if (this.url == null) 1701 if (Configuration.errorOnAutoCreate()) 1702 throw new Error("Attempt to auto-create TestScriptMetadataLinkComponent.url"); 1703 else if (Configuration.doAutoCreate()) 1704 this.url = new UriType(); // bb 1705 return this.url; 1706 } 1707 1708 public boolean hasUrlElement() { 1709 return this.url != null && !this.url.isEmpty(); 1710 } 1711 1712 public boolean hasUrl() { 1713 return this.url != null && !this.url.isEmpty(); 1714 } 1715 1716 /** 1717 * @param value {@link #url} (URL to a particular requirement or feature within the FHIR specification.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1718 */ 1719 public TestScriptMetadataLinkComponent setUrlElement(UriType value) { 1720 this.url = value; 1721 return this; 1722 } 1723 1724 /** 1725 * @return URL to a particular requirement or feature within the FHIR specification. 1726 */ 1727 public String getUrl() { 1728 return this.url == null ? null : this.url.getValue(); 1729 } 1730 1731 /** 1732 * @param value URL to a particular requirement or feature within the FHIR specification. 1733 */ 1734 public TestScriptMetadataLinkComponent setUrl(String value) { 1735 if (this.url == null) 1736 this.url = new UriType(); 1737 this.url.setValue(value); 1738 return this; 1739 } 1740 1741 /** 1742 * @return {@link #description} (Short description of the link.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1743 */ 1744 public StringType getDescriptionElement() { 1745 if (this.description == null) 1746 if (Configuration.errorOnAutoCreate()) 1747 throw new Error("Attempt to auto-create TestScriptMetadataLinkComponent.description"); 1748 else if (Configuration.doAutoCreate()) 1749 this.description = new StringType(); // bb 1750 return this.description; 1751 } 1752 1753 public boolean hasDescriptionElement() { 1754 return this.description != null && !this.description.isEmpty(); 1755 } 1756 1757 public boolean hasDescription() { 1758 return this.description != null && !this.description.isEmpty(); 1759 } 1760 1761 /** 1762 * @param value {@link #description} (Short description of the link.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1763 */ 1764 public TestScriptMetadataLinkComponent setDescriptionElement(StringType value) { 1765 this.description = value; 1766 return this; 1767 } 1768 1769 /** 1770 * @return Short description of the link. 1771 */ 1772 public String getDescription() { 1773 return this.description == null ? null : this.description.getValue(); 1774 } 1775 1776 /** 1777 * @param value Short description of the link. 1778 */ 1779 public TestScriptMetadataLinkComponent setDescription(String value) { 1780 if (Utilities.noString(value)) 1781 this.description = null; 1782 else { 1783 if (this.description == null) 1784 this.description = new StringType(); 1785 this.description.setValue(value); 1786 } 1787 return this; 1788 } 1789 1790 protected void listChildren(List<Property> children) { 1791 super.listChildren(children); 1792 children.add(new Property("url", "uri", "URL to a particular requirement or feature within the FHIR specification.", 0, 1, url)); 1793 children.add(new Property("description", "string", "Short description of the link.", 0, 1, description)); 1794 } 1795 1796 @Override 1797 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1798 switch (_hash) { 1799 case 116079: /*url*/ return new Property("url", "uri", "URL to a particular requirement or feature within the FHIR specification.", 0, 1, url); 1800 case -1724546052: /*description*/ return new Property("description", "string", "Short description of the link.", 0, 1, description); 1801 default: return super.getNamedProperty(_hash, _name, _checkValid); 1802 } 1803 1804 } 1805 1806 @Override 1807 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1808 switch (hash) { 1809 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1810 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1811 default: return super.getProperty(hash, name, checkValid); 1812 } 1813 1814 } 1815 1816 @Override 1817 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1818 switch (hash) { 1819 case 116079: // url 1820 this.url = castToUri(value); // UriType 1821 return value; 1822 case -1724546052: // description 1823 this.description = castToString(value); // StringType 1824 return value; 1825 default: return super.setProperty(hash, name, value); 1826 } 1827 1828 } 1829 1830 @Override 1831 public Base setProperty(String name, Base value) throws FHIRException { 1832 if (name.equals("url")) { 1833 this.url = castToUri(value); // UriType 1834 } else if (name.equals("description")) { 1835 this.description = castToString(value); // StringType 1836 } else 1837 return super.setProperty(name, value); 1838 return value; 1839 } 1840 1841 @Override 1842 public Base makeProperty(int hash, String name) throws FHIRException { 1843 switch (hash) { 1844 case 116079: return getUrlElement(); 1845 case -1724546052: return getDescriptionElement(); 1846 default: return super.makeProperty(hash, name); 1847 } 1848 1849 } 1850 1851 @Override 1852 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1853 switch (hash) { 1854 case 116079: /*url*/ return new String[] {"uri"}; 1855 case -1724546052: /*description*/ return new String[] {"string"}; 1856 default: return super.getTypesForProperty(hash, name); 1857 } 1858 1859 } 1860 1861 @Override 1862 public Base addChild(String name) throws FHIRException { 1863 if (name.equals("url")) { 1864 throw new FHIRException("Cannot call addChild on a singleton property TestScript.url"); 1865 } 1866 else if (name.equals("description")) { 1867 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 1868 } 1869 else 1870 return super.addChild(name); 1871 } 1872 1873 public TestScriptMetadataLinkComponent copy() { 1874 TestScriptMetadataLinkComponent dst = new TestScriptMetadataLinkComponent(); 1875 copyValues(dst); 1876 dst.url = url == null ? null : url.copy(); 1877 dst.description = description == null ? null : description.copy(); 1878 return dst; 1879 } 1880 1881 @Override 1882 public boolean equalsDeep(Base other_) { 1883 if (!super.equalsDeep(other_)) 1884 return false; 1885 if (!(other_ instanceof TestScriptMetadataLinkComponent)) 1886 return false; 1887 TestScriptMetadataLinkComponent o = (TestScriptMetadataLinkComponent) other_; 1888 return compareDeep(url, o.url, true) && compareDeep(description, o.description, true); 1889 } 1890 1891 @Override 1892 public boolean equalsShallow(Base other_) { 1893 if (!super.equalsShallow(other_)) 1894 return false; 1895 if (!(other_ instanceof TestScriptMetadataLinkComponent)) 1896 return false; 1897 TestScriptMetadataLinkComponent o = (TestScriptMetadataLinkComponent) other_; 1898 return compareValues(url, o.url, true) && compareValues(description, o.description, true); 1899 } 1900 1901 public boolean isEmpty() { 1902 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, description); 1903 } 1904 1905 public String fhirType() { 1906 return "TestScript.metadata.link"; 1907 1908 } 1909 1910 } 1911 1912 @Block() 1913 public static class TestScriptMetadataCapabilityComponent extends BackboneElement implements IBaseBackboneElement { 1914 /** 1915 * Whether or not the test execution will require the given capabilities of the server in order for this test script to execute. 1916 */ 1917 @Child(name = "required", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1918 @Description(shortDefinition="Are the capabilities required?", formalDefinition="Whether or not the test execution will require the given capabilities of the server in order for this test script to execute." ) 1919 protected BooleanType required; 1920 1921 /** 1922 * Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute. 1923 */ 1924 @Child(name = "validated", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1925 @Description(shortDefinition="Are the capabilities validated?", formalDefinition="Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute." ) 1926 protected BooleanType validated; 1927 1928 /** 1929 * Description of the capabilities that this test script is requiring the server to support. 1930 */ 1931 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1932 @Description(shortDefinition="The expected capabilities of the server", formalDefinition="Description of the capabilities that this test script is requiring the server to support." ) 1933 protected StringType description; 1934 1935 /** 1936 * Which origin server these requirements apply to. 1937 */ 1938 @Child(name = "origin", type = {IntegerType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1939 @Description(shortDefinition="Which origin server these requirements apply to", formalDefinition="Which origin server these requirements apply to." ) 1940 protected List<IntegerType> origin; 1941 1942 /** 1943 * Which server these requirements apply to. 1944 */ 1945 @Child(name = "destination", type = {IntegerType.class}, order=5, min=0, max=1, modifier=false, summary=false) 1946 @Description(shortDefinition="Which server these requirements apply to", formalDefinition="Which server these requirements apply to." ) 1947 protected IntegerType destination; 1948 1949 /** 1950 * Links to the FHIR specification that describes this interaction and the resources involved in more detail. 1951 */ 1952 @Child(name = "link", type = {UriType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1953 @Description(shortDefinition="Links to the FHIR specification", formalDefinition="Links to the FHIR specification that describes this interaction and the resources involved in more detail." ) 1954 protected List<UriType> link; 1955 1956 /** 1957 * Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped. 1958 */ 1959 @Child(name = "capabilities", type = {CapabilityStatement.class}, order=7, min=1, max=1, modifier=false, summary=false) 1960 @Description(shortDefinition="Required Capability Statement", formalDefinition="Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped." ) 1961 protected Reference capabilities; 1962 1963 /** 1964 * The actual object that is the target of the reference (Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.) 1965 */ 1966 protected CapabilityStatement capabilitiesTarget; 1967 1968 private static final long serialVersionUID = -106110735L; 1969 1970 /** 1971 * Constructor 1972 */ 1973 public TestScriptMetadataCapabilityComponent() { 1974 super(); 1975 } 1976 1977 /** 1978 * Constructor 1979 */ 1980 public TestScriptMetadataCapabilityComponent(Reference capabilities) { 1981 super(); 1982 this.capabilities = capabilities; 1983 } 1984 1985 /** 1986 * @return {@link #required} (Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 1987 */ 1988 public BooleanType getRequiredElement() { 1989 if (this.required == null) 1990 if (Configuration.errorOnAutoCreate()) 1991 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.required"); 1992 else if (Configuration.doAutoCreate()) 1993 this.required = new BooleanType(); // bb 1994 return this.required; 1995 } 1996 1997 public boolean hasRequiredElement() { 1998 return this.required != null && !this.required.isEmpty(); 1999 } 2000 2001 public boolean hasRequired() { 2002 return this.required != null && !this.required.isEmpty(); 2003 } 2004 2005 /** 2006 * @param value {@link #required} (Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.). This is the underlying object with id, value and extensions. The accessor "getRequired" gives direct access to the value 2007 */ 2008 public TestScriptMetadataCapabilityComponent setRequiredElement(BooleanType value) { 2009 this.required = value; 2010 return this; 2011 } 2012 2013 /** 2014 * @return Whether or not the test execution will require the given capabilities of the server in order for this test script to execute. 2015 */ 2016 public boolean getRequired() { 2017 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 2018 } 2019 2020 /** 2021 * @param value Whether or not the test execution will require the given capabilities of the server in order for this test script to execute. 2022 */ 2023 public TestScriptMetadataCapabilityComponent setRequired(boolean value) { 2024 if (this.required == null) 2025 this.required = new BooleanType(); 2026 this.required.setValue(value); 2027 return this; 2028 } 2029 2030 /** 2031 * @return {@link #validated} (Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.). This is the underlying object with id, value and extensions. The accessor "getValidated" gives direct access to the value 2032 */ 2033 public BooleanType getValidatedElement() { 2034 if (this.validated == null) 2035 if (Configuration.errorOnAutoCreate()) 2036 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.validated"); 2037 else if (Configuration.doAutoCreate()) 2038 this.validated = new BooleanType(); // bb 2039 return this.validated; 2040 } 2041 2042 public boolean hasValidatedElement() { 2043 return this.validated != null && !this.validated.isEmpty(); 2044 } 2045 2046 public boolean hasValidated() { 2047 return this.validated != null && !this.validated.isEmpty(); 2048 } 2049 2050 /** 2051 * @param value {@link #validated} (Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.). This is the underlying object with id, value and extensions. The accessor "getValidated" gives direct access to the value 2052 */ 2053 public TestScriptMetadataCapabilityComponent setValidatedElement(BooleanType value) { 2054 this.validated = value; 2055 return this; 2056 } 2057 2058 /** 2059 * @return Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute. 2060 */ 2061 public boolean getValidated() { 2062 return this.validated == null || this.validated.isEmpty() ? false : this.validated.getValue(); 2063 } 2064 2065 /** 2066 * @param value Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute. 2067 */ 2068 public TestScriptMetadataCapabilityComponent setValidated(boolean value) { 2069 if (this.validated == null) 2070 this.validated = new BooleanType(); 2071 this.validated.setValue(value); 2072 return this; 2073 } 2074 2075 /** 2076 * @return {@link #description} (Description of the capabilities that this test script is requiring the server to support.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2077 */ 2078 public StringType getDescriptionElement() { 2079 if (this.description == null) 2080 if (Configuration.errorOnAutoCreate()) 2081 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.description"); 2082 else if (Configuration.doAutoCreate()) 2083 this.description = new StringType(); // bb 2084 return this.description; 2085 } 2086 2087 public boolean hasDescriptionElement() { 2088 return this.description != null && !this.description.isEmpty(); 2089 } 2090 2091 public boolean hasDescription() { 2092 return this.description != null && !this.description.isEmpty(); 2093 } 2094 2095 /** 2096 * @param value {@link #description} (Description of the capabilities that this test script is requiring the server to support.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2097 */ 2098 public TestScriptMetadataCapabilityComponent setDescriptionElement(StringType value) { 2099 this.description = value; 2100 return this; 2101 } 2102 2103 /** 2104 * @return Description of the capabilities that this test script is requiring the server to support. 2105 */ 2106 public String getDescription() { 2107 return this.description == null ? null : this.description.getValue(); 2108 } 2109 2110 /** 2111 * @param value Description of the capabilities that this test script is requiring the server to support. 2112 */ 2113 public TestScriptMetadataCapabilityComponent setDescription(String value) { 2114 if (Utilities.noString(value)) 2115 this.description = null; 2116 else { 2117 if (this.description == null) 2118 this.description = new StringType(); 2119 this.description.setValue(value); 2120 } 2121 return this; 2122 } 2123 2124 /** 2125 * @return {@link #origin} (Which origin server these requirements apply to.) 2126 */ 2127 public List<IntegerType> getOrigin() { 2128 if (this.origin == null) 2129 this.origin = new ArrayList<IntegerType>(); 2130 return this.origin; 2131 } 2132 2133 /** 2134 * @return Returns a reference to <code>this</code> for easy method chaining 2135 */ 2136 public TestScriptMetadataCapabilityComponent setOrigin(List<IntegerType> theOrigin) { 2137 this.origin = theOrigin; 2138 return this; 2139 } 2140 2141 public boolean hasOrigin() { 2142 if (this.origin == null) 2143 return false; 2144 for (IntegerType item : this.origin) 2145 if (!item.isEmpty()) 2146 return true; 2147 return false; 2148 } 2149 2150 /** 2151 * @return {@link #origin} (Which origin server these requirements apply to.) 2152 */ 2153 public IntegerType addOriginElement() {//2 2154 IntegerType t = new IntegerType(); 2155 if (this.origin == null) 2156 this.origin = new ArrayList<IntegerType>(); 2157 this.origin.add(t); 2158 return t; 2159 } 2160 2161 /** 2162 * @param value {@link #origin} (Which origin server these requirements apply to.) 2163 */ 2164 public TestScriptMetadataCapabilityComponent addOrigin(int value) { //1 2165 IntegerType t = new IntegerType(); 2166 t.setValue(value); 2167 if (this.origin == null) 2168 this.origin = new ArrayList<IntegerType>(); 2169 this.origin.add(t); 2170 return this; 2171 } 2172 2173 /** 2174 * @param value {@link #origin} (Which origin server these requirements apply to.) 2175 */ 2176 public boolean hasOrigin(int value) { 2177 if (this.origin == null) 2178 return false; 2179 for (IntegerType v : this.origin) 2180 if (v.getValue().equals(value)) // integer 2181 return true; 2182 return false; 2183 } 2184 2185 /** 2186 * @return {@link #destination} (Which server these requirements apply to.). This is the underlying object with id, value and extensions. The accessor "getDestination" gives direct access to the value 2187 */ 2188 public IntegerType getDestinationElement() { 2189 if (this.destination == null) 2190 if (Configuration.errorOnAutoCreate()) 2191 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.destination"); 2192 else if (Configuration.doAutoCreate()) 2193 this.destination = new IntegerType(); // bb 2194 return this.destination; 2195 } 2196 2197 public boolean hasDestinationElement() { 2198 return this.destination != null && !this.destination.isEmpty(); 2199 } 2200 2201 public boolean hasDestination() { 2202 return this.destination != null && !this.destination.isEmpty(); 2203 } 2204 2205 /** 2206 * @param value {@link #destination} (Which server these requirements apply to.). This is the underlying object with id, value and extensions. The accessor "getDestination" gives direct access to the value 2207 */ 2208 public TestScriptMetadataCapabilityComponent setDestinationElement(IntegerType value) { 2209 this.destination = value; 2210 return this; 2211 } 2212 2213 /** 2214 * @return Which server these requirements apply to. 2215 */ 2216 public int getDestination() { 2217 return this.destination == null || this.destination.isEmpty() ? 0 : this.destination.getValue(); 2218 } 2219 2220 /** 2221 * @param value Which server these requirements apply to. 2222 */ 2223 public TestScriptMetadataCapabilityComponent setDestination(int value) { 2224 if (this.destination == null) 2225 this.destination = new IntegerType(); 2226 this.destination.setValue(value); 2227 return this; 2228 } 2229 2230 /** 2231 * @return {@link #link} (Links to the FHIR specification that describes this interaction and the resources involved in more detail.) 2232 */ 2233 public List<UriType> getLink() { 2234 if (this.link == null) 2235 this.link = new ArrayList<UriType>(); 2236 return this.link; 2237 } 2238 2239 /** 2240 * @return Returns a reference to <code>this</code> for easy method chaining 2241 */ 2242 public TestScriptMetadataCapabilityComponent setLink(List<UriType> theLink) { 2243 this.link = theLink; 2244 return this; 2245 } 2246 2247 public boolean hasLink() { 2248 if (this.link == null) 2249 return false; 2250 for (UriType item : this.link) 2251 if (!item.isEmpty()) 2252 return true; 2253 return false; 2254 } 2255 2256 /** 2257 * @return {@link #link} (Links to the FHIR specification that describes this interaction and the resources involved in more detail.) 2258 */ 2259 public UriType addLinkElement() {//2 2260 UriType t = new UriType(); 2261 if (this.link == null) 2262 this.link = new ArrayList<UriType>(); 2263 this.link.add(t); 2264 return t; 2265 } 2266 2267 /** 2268 * @param value {@link #link} (Links to the FHIR specification that describes this interaction and the resources involved in more detail.) 2269 */ 2270 public TestScriptMetadataCapabilityComponent addLink(String value) { //1 2271 UriType t = new UriType(); 2272 t.setValue(value); 2273 if (this.link == null) 2274 this.link = new ArrayList<UriType>(); 2275 this.link.add(t); 2276 return this; 2277 } 2278 2279 /** 2280 * @param value {@link #link} (Links to the FHIR specification that describes this interaction and the resources involved in more detail.) 2281 */ 2282 public boolean hasLink(String value) { 2283 if (this.link == null) 2284 return false; 2285 for (UriType v : this.link) 2286 if (v.getValue().equals(value)) // uri 2287 return true; 2288 return false; 2289 } 2290 2291 /** 2292 * @return {@link #capabilities} (Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.) 2293 */ 2294 public Reference getCapabilities() { 2295 if (this.capabilities == null) 2296 if (Configuration.errorOnAutoCreate()) 2297 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.capabilities"); 2298 else if (Configuration.doAutoCreate()) 2299 this.capabilities = new Reference(); // cc 2300 return this.capabilities; 2301 } 2302 2303 public boolean hasCapabilities() { 2304 return this.capabilities != null && !this.capabilities.isEmpty(); 2305 } 2306 2307 /** 2308 * @param value {@link #capabilities} (Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.) 2309 */ 2310 public TestScriptMetadataCapabilityComponent setCapabilities(Reference value) { 2311 this.capabilities = value; 2312 return this; 2313 } 2314 2315 /** 2316 * @return {@link #capabilities} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.) 2317 */ 2318 public CapabilityStatement getCapabilitiesTarget() { 2319 if (this.capabilitiesTarget == null) 2320 if (Configuration.errorOnAutoCreate()) 2321 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.capabilities"); 2322 else if (Configuration.doAutoCreate()) 2323 this.capabilitiesTarget = new CapabilityStatement(); // aa 2324 return this.capabilitiesTarget; 2325 } 2326 2327 /** 2328 * @param value {@link #capabilities} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.) 2329 */ 2330 public TestScriptMetadataCapabilityComponent setCapabilitiesTarget(CapabilityStatement value) { 2331 this.capabilitiesTarget = value; 2332 return this; 2333 } 2334 2335 protected void listChildren(List<Property> children) { 2336 super.listChildren(children); 2337 children.add(new Property("required", "boolean", "Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.", 0, 1, required)); 2338 children.add(new Property("validated", "boolean", "Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.", 0, 1, validated)); 2339 children.add(new Property("description", "string", "Description of the capabilities that this test script is requiring the server to support.", 0, 1, description)); 2340 children.add(new Property("origin", "integer", "Which origin server these requirements apply to.", 0, java.lang.Integer.MAX_VALUE, origin)); 2341 children.add(new Property("destination", "integer", "Which server these requirements apply to.", 0, 1, destination)); 2342 children.add(new Property("link", "uri", "Links to the FHIR specification that describes this interaction and the resources involved in more detail.", 0, java.lang.Integer.MAX_VALUE, link)); 2343 children.add(new Property("capabilities", "Reference(CapabilityStatement)", "Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.", 0, 1, capabilities)); 2344 } 2345 2346 @Override 2347 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2348 switch (_hash) { 2349 case -393139297: /*required*/ return new Property("required", "boolean", "Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.", 0, 1, required); 2350 case -1109784050: /*validated*/ return new Property("validated", "boolean", "Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.", 0, 1, validated); 2351 case -1724546052: /*description*/ return new Property("description", "string", "Description of the capabilities that this test script is requiring the server to support.", 0, 1, description); 2352 case -1008619738: /*origin*/ return new Property("origin", "integer", "Which origin server these requirements apply to.", 0, java.lang.Integer.MAX_VALUE, origin); 2353 case -1429847026: /*destination*/ return new Property("destination", "integer", "Which server these requirements apply to.", 0, 1, destination); 2354 case 3321850: /*link*/ return new Property("link", "uri", "Links to the FHIR specification that describes this interaction and the resources involved in more detail.", 0, java.lang.Integer.MAX_VALUE, link); 2355 case -1487597642: /*capabilities*/ return new Property("capabilities", "Reference(CapabilityStatement)", "Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.", 0, 1, capabilities); 2356 default: return super.getNamedProperty(_hash, _name, _checkValid); 2357 } 2358 2359 } 2360 2361 @Override 2362 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2363 switch (hash) { 2364 case -393139297: /*required*/ return this.required == null ? new Base[0] : new Base[] {this.required}; // BooleanType 2365 case -1109784050: /*validated*/ return this.validated == null ? new Base[0] : new Base[] {this.validated}; // BooleanType 2366 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2367 case -1008619738: /*origin*/ return this.origin == null ? new Base[0] : this.origin.toArray(new Base[this.origin.size()]); // IntegerType 2368 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // IntegerType 2369 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // UriType 2370 case -1487597642: /*capabilities*/ return this.capabilities == null ? new Base[0] : new Base[] {this.capabilities}; // Reference 2371 default: return super.getProperty(hash, name, checkValid); 2372 } 2373 2374 } 2375 2376 @Override 2377 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2378 switch (hash) { 2379 case -393139297: // required 2380 this.required = castToBoolean(value); // BooleanType 2381 return value; 2382 case -1109784050: // validated 2383 this.validated = castToBoolean(value); // BooleanType 2384 return value; 2385 case -1724546052: // description 2386 this.description = castToString(value); // StringType 2387 return value; 2388 case -1008619738: // origin 2389 this.getOrigin().add(castToInteger(value)); // IntegerType 2390 return value; 2391 case -1429847026: // destination 2392 this.destination = castToInteger(value); // IntegerType 2393 return value; 2394 case 3321850: // link 2395 this.getLink().add(castToUri(value)); // UriType 2396 return value; 2397 case -1487597642: // capabilities 2398 this.capabilities = castToReference(value); // Reference 2399 return value; 2400 default: return super.setProperty(hash, name, value); 2401 } 2402 2403 } 2404 2405 @Override 2406 public Base setProperty(String name, Base value) throws FHIRException { 2407 if (name.equals("required")) { 2408 this.required = castToBoolean(value); // BooleanType 2409 } else if (name.equals("validated")) { 2410 this.validated = castToBoolean(value); // BooleanType 2411 } else if (name.equals("description")) { 2412 this.description = castToString(value); // StringType 2413 } else if (name.equals("origin")) { 2414 this.getOrigin().add(castToInteger(value)); 2415 } else if (name.equals("destination")) { 2416 this.destination = castToInteger(value); // IntegerType 2417 } else if (name.equals("link")) { 2418 this.getLink().add(castToUri(value)); 2419 } else if (name.equals("capabilities")) { 2420 this.capabilities = castToReference(value); // Reference 2421 } else 2422 return super.setProperty(name, value); 2423 return value; 2424 } 2425 2426 @Override 2427 public Base makeProperty(int hash, String name) throws FHIRException { 2428 switch (hash) { 2429 case -393139297: return getRequiredElement(); 2430 case -1109784050: return getValidatedElement(); 2431 case -1724546052: return getDescriptionElement(); 2432 case -1008619738: return addOriginElement(); 2433 case -1429847026: return getDestinationElement(); 2434 case 3321850: return addLinkElement(); 2435 case -1487597642: return getCapabilities(); 2436 default: return super.makeProperty(hash, name); 2437 } 2438 2439 } 2440 2441 @Override 2442 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2443 switch (hash) { 2444 case -393139297: /*required*/ return new String[] {"boolean"}; 2445 case -1109784050: /*validated*/ return new String[] {"boolean"}; 2446 case -1724546052: /*description*/ return new String[] {"string"}; 2447 case -1008619738: /*origin*/ return new String[] {"integer"}; 2448 case -1429847026: /*destination*/ return new String[] {"integer"}; 2449 case 3321850: /*link*/ return new String[] {"uri"}; 2450 case -1487597642: /*capabilities*/ return new String[] {"Reference"}; 2451 default: return super.getTypesForProperty(hash, name); 2452 } 2453 2454 } 2455 2456 @Override 2457 public Base addChild(String name) throws FHIRException { 2458 if (name.equals("required")) { 2459 throw new FHIRException("Cannot call addChild on a singleton property TestScript.required"); 2460 } 2461 else if (name.equals("validated")) { 2462 throw new FHIRException("Cannot call addChild on a singleton property TestScript.validated"); 2463 } 2464 else if (name.equals("description")) { 2465 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 2466 } 2467 else if (name.equals("origin")) { 2468 throw new FHIRException("Cannot call addChild on a singleton property TestScript.origin"); 2469 } 2470 else if (name.equals("destination")) { 2471 throw new FHIRException("Cannot call addChild on a singleton property TestScript.destination"); 2472 } 2473 else if (name.equals("link")) { 2474 throw new FHIRException("Cannot call addChild on a singleton property TestScript.link"); 2475 } 2476 else if (name.equals("capabilities")) { 2477 this.capabilities = new Reference(); 2478 return this.capabilities; 2479 } 2480 else 2481 return super.addChild(name); 2482 } 2483 2484 public TestScriptMetadataCapabilityComponent copy() { 2485 TestScriptMetadataCapabilityComponent dst = new TestScriptMetadataCapabilityComponent(); 2486 copyValues(dst); 2487 dst.required = required == null ? null : required.copy(); 2488 dst.validated = validated == null ? null : validated.copy(); 2489 dst.description = description == null ? null : description.copy(); 2490 if (origin != null) { 2491 dst.origin = new ArrayList<IntegerType>(); 2492 for (IntegerType i : origin) 2493 dst.origin.add(i.copy()); 2494 }; 2495 dst.destination = destination == null ? null : destination.copy(); 2496 if (link != null) { 2497 dst.link = new ArrayList<UriType>(); 2498 for (UriType i : link) 2499 dst.link.add(i.copy()); 2500 }; 2501 dst.capabilities = capabilities == null ? null : capabilities.copy(); 2502 return dst; 2503 } 2504 2505 @Override 2506 public boolean equalsDeep(Base other_) { 2507 if (!super.equalsDeep(other_)) 2508 return false; 2509 if (!(other_ instanceof TestScriptMetadataCapabilityComponent)) 2510 return false; 2511 TestScriptMetadataCapabilityComponent o = (TestScriptMetadataCapabilityComponent) other_; 2512 return compareDeep(required, o.required, true) && compareDeep(validated, o.validated, true) && compareDeep(description, o.description, true) 2513 && compareDeep(origin, o.origin, true) && compareDeep(destination, o.destination, true) && compareDeep(link, o.link, true) 2514 && compareDeep(capabilities, o.capabilities, true); 2515 } 2516 2517 @Override 2518 public boolean equalsShallow(Base other_) { 2519 if (!super.equalsShallow(other_)) 2520 return false; 2521 if (!(other_ instanceof TestScriptMetadataCapabilityComponent)) 2522 return false; 2523 TestScriptMetadataCapabilityComponent o = (TestScriptMetadataCapabilityComponent) other_; 2524 return compareValues(required, o.required, true) && compareValues(validated, o.validated, true) && compareValues(description, o.description, true) 2525 && compareValues(origin, o.origin, true) && compareValues(destination, o.destination, true) && compareValues(link, o.link, true) 2526 ; 2527 } 2528 2529 public boolean isEmpty() { 2530 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(required, validated, description 2531 , origin, destination, link, capabilities); 2532 } 2533 2534 public String fhirType() { 2535 return "TestScript.metadata.capability"; 2536 2537 } 2538 2539 } 2540 2541 @Block() 2542 public static class TestScriptFixtureComponent extends BackboneElement implements IBaseBackboneElement { 2543 /** 2544 * Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section. 2545 */ 2546 @Child(name = "autocreate", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2547 @Description(shortDefinition="Whether or not to implicitly create the fixture during setup", formalDefinition="Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section." ) 2548 protected BooleanType autocreate; 2549 2550 /** 2551 * Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section. 2552 */ 2553 @Child(name = "autodelete", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2554 @Description(shortDefinition="Whether or not to implicitly delete the fixture during teardown", formalDefinition="Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section." ) 2555 protected BooleanType autodelete; 2556 2557 /** 2558 * Reference to the resource (containing the contents of the resource needed for operations). 2559 */ 2560 @Child(name = "resource", type = {Reference.class}, order=3, min=0, max=1, modifier=false, summary=false) 2561 @Description(shortDefinition="Reference of the resource", formalDefinition="Reference to the resource (containing the contents of the resource needed for operations)." ) 2562 protected Reference resource; 2563 2564 /** 2565 * The actual object that is the target of the reference (Reference to the resource (containing the contents of the resource needed for operations).) 2566 */ 2567 protected Resource resourceTarget; 2568 2569 private static final long serialVersionUID = 1110683307L; 2570 2571 /** 2572 * Constructor 2573 */ 2574 public TestScriptFixtureComponent() { 2575 super(); 2576 } 2577 2578 /** 2579 * @return {@link #autocreate} (Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.). This is the underlying object with id, value and extensions. The accessor "getAutocreate" gives direct access to the value 2580 */ 2581 public BooleanType getAutocreateElement() { 2582 if (this.autocreate == null) 2583 if (Configuration.errorOnAutoCreate()) 2584 throw new Error("Attempt to auto-create TestScriptFixtureComponent.autocreate"); 2585 else if (Configuration.doAutoCreate()) 2586 this.autocreate = new BooleanType(); // bb 2587 return this.autocreate; 2588 } 2589 2590 public boolean hasAutocreateElement() { 2591 return this.autocreate != null && !this.autocreate.isEmpty(); 2592 } 2593 2594 public boolean hasAutocreate() { 2595 return this.autocreate != null && !this.autocreate.isEmpty(); 2596 } 2597 2598 /** 2599 * @param value {@link #autocreate} (Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.). This is the underlying object with id, value and extensions. The accessor "getAutocreate" gives direct access to the value 2600 */ 2601 public TestScriptFixtureComponent setAutocreateElement(BooleanType value) { 2602 this.autocreate = value; 2603 return this; 2604 } 2605 2606 /** 2607 * @return Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section. 2608 */ 2609 public boolean getAutocreate() { 2610 return this.autocreate == null || this.autocreate.isEmpty() ? false : this.autocreate.getValue(); 2611 } 2612 2613 /** 2614 * @param value Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section. 2615 */ 2616 public TestScriptFixtureComponent setAutocreate(boolean value) { 2617 if (this.autocreate == null) 2618 this.autocreate = new BooleanType(); 2619 this.autocreate.setValue(value); 2620 return this; 2621 } 2622 2623 /** 2624 * @return {@link #autodelete} (Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.). This is the underlying object with id, value and extensions. The accessor "getAutodelete" gives direct access to the value 2625 */ 2626 public BooleanType getAutodeleteElement() { 2627 if (this.autodelete == null) 2628 if (Configuration.errorOnAutoCreate()) 2629 throw new Error("Attempt to auto-create TestScriptFixtureComponent.autodelete"); 2630 else if (Configuration.doAutoCreate()) 2631 this.autodelete = new BooleanType(); // bb 2632 return this.autodelete; 2633 } 2634 2635 public boolean hasAutodeleteElement() { 2636 return this.autodelete != null && !this.autodelete.isEmpty(); 2637 } 2638 2639 public boolean hasAutodelete() { 2640 return this.autodelete != null && !this.autodelete.isEmpty(); 2641 } 2642 2643 /** 2644 * @param value {@link #autodelete} (Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.). This is the underlying object with id, value and extensions. The accessor "getAutodelete" gives direct access to the value 2645 */ 2646 public TestScriptFixtureComponent setAutodeleteElement(BooleanType value) { 2647 this.autodelete = value; 2648 return this; 2649 } 2650 2651 /** 2652 * @return Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section. 2653 */ 2654 public boolean getAutodelete() { 2655 return this.autodelete == null || this.autodelete.isEmpty() ? false : this.autodelete.getValue(); 2656 } 2657 2658 /** 2659 * @param value Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section. 2660 */ 2661 public TestScriptFixtureComponent setAutodelete(boolean value) { 2662 if (this.autodelete == null) 2663 this.autodelete = new BooleanType(); 2664 this.autodelete.setValue(value); 2665 return this; 2666 } 2667 2668 /** 2669 * @return {@link #resource} (Reference to the resource (containing the contents of the resource needed for operations).) 2670 */ 2671 public Reference getResource() { 2672 if (this.resource == null) 2673 if (Configuration.errorOnAutoCreate()) 2674 throw new Error("Attempt to auto-create TestScriptFixtureComponent.resource"); 2675 else if (Configuration.doAutoCreate()) 2676 this.resource = new Reference(); // cc 2677 return this.resource; 2678 } 2679 2680 public boolean hasResource() { 2681 return this.resource != null && !this.resource.isEmpty(); 2682 } 2683 2684 /** 2685 * @param value {@link #resource} (Reference to the resource (containing the contents of the resource needed for operations).) 2686 */ 2687 public TestScriptFixtureComponent setResource(Reference value) { 2688 this.resource = value; 2689 return this; 2690 } 2691 2692 /** 2693 * @return {@link #resource} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the resource (containing the contents of the resource needed for operations).) 2694 */ 2695 public Resource getResourceTarget() { 2696 return this.resourceTarget; 2697 } 2698 2699 /** 2700 * @param value {@link #resource} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the resource (containing the contents of the resource needed for operations).) 2701 */ 2702 public TestScriptFixtureComponent setResourceTarget(Resource value) { 2703 this.resourceTarget = value; 2704 return this; 2705 } 2706 2707 protected void listChildren(List<Property> children) { 2708 super.listChildren(children); 2709 children.add(new Property("autocreate", "boolean", "Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.", 0, 1, autocreate)); 2710 children.add(new Property("autodelete", "boolean", "Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.", 0, 1, autodelete)); 2711 children.add(new Property("resource", "Reference(Any)", "Reference to the resource (containing the contents of the resource needed for operations).", 0, 1, resource)); 2712 } 2713 2714 @Override 2715 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2716 switch (_hash) { 2717 case 73154411: /*autocreate*/ return new Property("autocreate", "boolean", "Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.", 0, 1, autocreate); 2718 case 89990170: /*autodelete*/ return new Property("autodelete", "boolean", "Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.", 0, 1, autodelete); 2719 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "Reference to the resource (containing the contents of the resource needed for operations).", 0, 1, resource); 2720 default: return super.getNamedProperty(_hash, _name, _checkValid); 2721 } 2722 2723 } 2724 2725 @Override 2726 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2727 switch (hash) { 2728 case 73154411: /*autocreate*/ return this.autocreate == null ? new Base[0] : new Base[] {this.autocreate}; // BooleanType 2729 case 89990170: /*autodelete*/ return this.autodelete == null ? new Base[0] : new Base[] {this.autodelete}; // BooleanType 2730 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 2731 default: return super.getProperty(hash, name, checkValid); 2732 } 2733 2734 } 2735 2736 @Override 2737 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2738 switch (hash) { 2739 case 73154411: // autocreate 2740 this.autocreate = castToBoolean(value); // BooleanType 2741 return value; 2742 case 89990170: // autodelete 2743 this.autodelete = castToBoolean(value); // BooleanType 2744 return value; 2745 case -341064690: // resource 2746 this.resource = castToReference(value); // Reference 2747 return value; 2748 default: return super.setProperty(hash, name, value); 2749 } 2750 2751 } 2752 2753 @Override 2754 public Base setProperty(String name, Base value) throws FHIRException { 2755 if (name.equals("autocreate")) { 2756 this.autocreate = castToBoolean(value); // BooleanType 2757 } else if (name.equals("autodelete")) { 2758 this.autodelete = castToBoolean(value); // BooleanType 2759 } else if (name.equals("resource")) { 2760 this.resource = castToReference(value); // Reference 2761 } else 2762 return super.setProperty(name, value); 2763 return value; 2764 } 2765 2766 @Override 2767 public Base makeProperty(int hash, String name) throws FHIRException { 2768 switch (hash) { 2769 case 73154411: return getAutocreateElement(); 2770 case 89990170: return getAutodeleteElement(); 2771 case -341064690: return getResource(); 2772 default: return super.makeProperty(hash, name); 2773 } 2774 2775 } 2776 2777 @Override 2778 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2779 switch (hash) { 2780 case 73154411: /*autocreate*/ return new String[] {"boolean"}; 2781 case 89990170: /*autodelete*/ return new String[] {"boolean"}; 2782 case -341064690: /*resource*/ return new String[] {"Reference"}; 2783 default: return super.getTypesForProperty(hash, name); 2784 } 2785 2786 } 2787 2788 @Override 2789 public Base addChild(String name) throws FHIRException { 2790 if (name.equals("autocreate")) { 2791 throw new FHIRException("Cannot call addChild on a singleton property TestScript.autocreate"); 2792 } 2793 else if (name.equals("autodelete")) { 2794 throw new FHIRException("Cannot call addChild on a singleton property TestScript.autodelete"); 2795 } 2796 else if (name.equals("resource")) { 2797 this.resource = new Reference(); 2798 return this.resource; 2799 } 2800 else 2801 return super.addChild(name); 2802 } 2803 2804 public TestScriptFixtureComponent copy() { 2805 TestScriptFixtureComponent dst = new TestScriptFixtureComponent(); 2806 copyValues(dst); 2807 dst.autocreate = autocreate == null ? null : autocreate.copy(); 2808 dst.autodelete = autodelete == null ? null : autodelete.copy(); 2809 dst.resource = resource == null ? null : resource.copy(); 2810 return dst; 2811 } 2812 2813 @Override 2814 public boolean equalsDeep(Base other_) { 2815 if (!super.equalsDeep(other_)) 2816 return false; 2817 if (!(other_ instanceof TestScriptFixtureComponent)) 2818 return false; 2819 TestScriptFixtureComponent o = (TestScriptFixtureComponent) other_; 2820 return compareDeep(autocreate, o.autocreate, true) && compareDeep(autodelete, o.autodelete, true) 2821 && compareDeep(resource, o.resource, true); 2822 } 2823 2824 @Override 2825 public boolean equalsShallow(Base other_) { 2826 if (!super.equalsShallow(other_)) 2827 return false; 2828 if (!(other_ instanceof TestScriptFixtureComponent)) 2829 return false; 2830 TestScriptFixtureComponent o = (TestScriptFixtureComponent) other_; 2831 return compareValues(autocreate, o.autocreate, true) && compareValues(autodelete, o.autodelete, true) 2832 ; 2833 } 2834 2835 public boolean isEmpty() { 2836 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(autocreate, autodelete, resource 2837 ); 2838 } 2839 2840 public String fhirType() { 2841 return "TestScript.fixture"; 2842 2843 } 2844 2845 } 2846 2847 @Block() 2848 public static class TestScriptVariableComponent extends BackboneElement implements IBaseBackboneElement { 2849 /** 2850 * Descriptive name for this variable. 2851 */ 2852 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2853 @Description(shortDefinition="Descriptive name for this variable", formalDefinition="Descriptive name for this variable." ) 2854 protected StringType name; 2855 2856 /** 2857 * A default, hard-coded, or user-defined value for this variable. 2858 */ 2859 @Child(name = "defaultValue", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2860 @Description(shortDefinition="Default, hard-coded, or user-defined value for this variable", formalDefinition="A default, hard-coded, or user-defined value for this variable." ) 2861 protected StringType defaultValue; 2862 2863 /** 2864 * A free text natural language description of the variable and its purpose. 2865 */ 2866 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2867 @Description(shortDefinition="Natural language description of the variable", formalDefinition="A free text natural language description of the variable and its purpose." ) 2868 protected StringType description; 2869 2870 /** 2871 * The fluentpath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 2872 */ 2873 @Child(name = "expression", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2874 @Description(shortDefinition="The fluentpath expression against the fixture body", formalDefinition="The fluentpath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified." ) 2875 protected StringType expression; 2876 2877 /** 2878 * Will be used to grab the HTTP header field value from the headers that sourceId is pointing to. 2879 */ 2880 @Child(name = "headerField", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2881 @Description(shortDefinition="HTTP header field name for source", formalDefinition="Will be used to grab the HTTP header field value from the headers that sourceId is pointing to." ) 2882 protected StringType headerField; 2883 2884 /** 2885 * Displayable text string with hint help information to the user when entering a default value. 2886 */ 2887 @Child(name = "hint", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 2888 @Description(shortDefinition="Hint help text for default value to enter", formalDefinition="Displayable text string with hint help information to the user when entering a default value." ) 2889 protected StringType hint; 2890 2891 /** 2892 * XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 2893 */ 2894 @Child(name = "path", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 2895 @Description(shortDefinition="XPath or JSONPath against the fixture body", formalDefinition="XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified." ) 2896 protected StringType path; 2897 2898 /** 2899 * Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable. 2900 */ 2901 @Child(name = "sourceId", type = {IdType.class}, order=8, min=0, max=1, modifier=false, summary=false) 2902 @Description(shortDefinition="Fixture Id of source expression or headerField within this variable", formalDefinition="Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable." ) 2903 protected IdType sourceId; 2904 2905 private static final long serialVersionUID = -1592325432L; 2906 2907 /** 2908 * Constructor 2909 */ 2910 public TestScriptVariableComponent() { 2911 super(); 2912 } 2913 2914 /** 2915 * Constructor 2916 */ 2917 public TestScriptVariableComponent(StringType name) { 2918 super(); 2919 this.name = name; 2920 } 2921 2922 /** 2923 * @return {@link #name} (Descriptive name for this variable.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2924 */ 2925 public StringType getNameElement() { 2926 if (this.name == null) 2927 if (Configuration.errorOnAutoCreate()) 2928 throw new Error("Attempt to auto-create TestScriptVariableComponent.name"); 2929 else if (Configuration.doAutoCreate()) 2930 this.name = new StringType(); // bb 2931 return this.name; 2932 } 2933 2934 public boolean hasNameElement() { 2935 return this.name != null && !this.name.isEmpty(); 2936 } 2937 2938 public boolean hasName() { 2939 return this.name != null && !this.name.isEmpty(); 2940 } 2941 2942 /** 2943 * @param value {@link #name} (Descriptive name for this variable.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2944 */ 2945 public TestScriptVariableComponent setNameElement(StringType value) { 2946 this.name = value; 2947 return this; 2948 } 2949 2950 /** 2951 * @return Descriptive name for this variable. 2952 */ 2953 public String getName() { 2954 return this.name == null ? null : this.name.getValue(); 2955 } 2956 2957 /** 2958 * @param value Descriptive name for this variable. 2959 */ 2960 public TestScriptVariableComponent setName(String value) { 2961 if (this.name == null) 2962 this.name = new StringType(); 2963 this.name.setValue(value); 2964 return this; 2965 } 2966 2967 /** 2968 * @return {@link #defaultValue} (A default, hard-coded, or user-defined value for this variable.). This is the underlying object with id, value and extensions. The accessor "getDefaultValue" gives direct access to the value 2969 */ 2970 public StringType getDefaultValueElement() { 2971 if (this.defaultValue == null) 2972 if (Configuration.errorOnAutoCreate()) 2973 throw new Error("Attempt to auto-create TestScriptVariableComponent.defaultValue"); 2974 else if (Configuration.doAutoCreate()) 2975 this.defaultValue = new StringType(); // bb 2976 return this.defaultValue; 2977 } 2978 2979 public boolean hasDefaultValueElement() { 2980 return this.defaultValue != null && !this.defaultValue.isEmpty(); 2981 } 2982 2983 public boolean hasDefaultValue() { 2984 return this.defaultValue != null && !this.defaultValue.isEmpty(); 2985 } 2986 2987 /** 2988 * @param value {@link #defaultValue} (A default, hard-coded, or user-defined value for this variable.). This is the underlying object with id, value and extensions. The accessor "getDefaultValue" gives direct access to the value 2989 */ 2990 public TestScriptVariableComponent setDefaultValueElement(StringType value) { 2991 this.defaultValue = value; 2992 return this; 2993 } 2994 2995 /** 2996 * @return A default, hard-coded, or user-defined value for this variable. 2997 */ 2998 public String getDefaultValue() { 2999 return this.defaultValue == null ? null : this.defaultValue.getValue(); 3000 } 3001 3002 /** 3003 * @param value A default, hard-coded, or user-defined value for this variable. 3004 */ 3005 public TestScriptVariableComponent setDefaultValue(String value) { 3006 if (Utilities.noString(value)) 3007 this.defaultValue = null; 3008 else { 3009 if (this.defaultValue == null) 3010 this.defaultValue = new StringType(); 3011 this.defaultValue.setValue(value); 3012 } 3013 return this; 3014 } 3015 3016 /** 3017 * @return {@link #description} (A free text natural language description of the variable and its purpose.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3018 */ 3019 public StringType getDescriptionElement() { 3020 if (this.description == null) 3021 if (Configuration.errorOnAutoCreate()) 3022 throw new Error("Attempt to auto-create TestScriptVariableComponent.description"); 3023 else if (Configuration.doAutoCreate()) 3024 this.description = new StringType(); // bb 3025 return this.description; 3026 } 3027 3028 public boolean hasDescriptionElement() { 3029 return this.description != null && !this.description.isEmpty(); 3030 } 3031 3032 public boolean hasDescription() { 3033 return this.description != null && !this.description.isEmpty(); 3034 } 3035 3036 /** 3037 * @param value {@link #description} (A free text natural language description of the variable and its purpose.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3038 */ 3039 public TestScriptVariableComponent setDescriptionElement(StringType value) { 3040 this.description = value; 3041 return this; 3042 } 3043 3044 /** 3045 * @return A free text natural language description of the variable and its purpose. 3046 */ 3047 public String getDescription() { 3048 return this.description == null ? null : this.description.getValue(); 3049 } 3050 3051 /** 3052 * @param value A free text natural language description of the variable and its purpose. 3053 */ 3054 public TestScriptVariableComponent setDescription(String value) { 3055 if (Utilities.noString(value)) 3056 this.description = null; 3057 else { 3058 if (this.description == null) 3059 this.description = new StringType(); 3060 this.description.setValue(value); 3061 } 3062 return this; 3063 } 3064 3065 /** 3066 * @return {@link #expression} (The fluentpath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 3067 */ 3068 public StringType getExpressionElement() { 3069 if (this.expression == null) 3070 if (Configuration.errorOnAutoCreate()) 3071 throw new Error("Attempt to auto-create TestScriptVariableComponent.expression"); 3072 else if (Configuration.doAutoCreate()) 3073 this.expression = new StringType(); // bb 3074 return this.expression; 3075 } 3076 3077 public boolean hasExpressionElement() { 3078 return this.expression != null && !this.expression.isEmpty(); 3079 } 3080 3081 public boolean hasExpression() { 3082 return this.expression != null && !this.expression.isEmpty(); 3083 } 3084 3085 /** 3086 * @param value {@link #expression} (The fluentpath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 3087 */ 3088 public TestScriptVariableComponent setExpressionElement(StringType value) { 3089 this.expression = value; 3090 return this; 3091 } 3092 3093 /** 3094 * @return The fluentpath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 3095 */ 3096 public String getExpression() { 3097 return this.expression == null ? null : this.expression.getValue(); 3098 } 3099 3100 /** 3101 * @param value The fluentpath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 3102 */ 3103 public TestScriptVariableComponent setExpression(String value) { 3104 if (Utilities.noString(value)) 3105 this.expression = null; 3106 else { 3107 if (this.expression == null) 3108 this.expression = new StringType(); 3109 this.expression.setValue(value); 3110 } 3111 return this; 3112 } 3113 3114 /** 3115 * @return {@link #headerField} (Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.). This is the underlying object with id, value and extensions. The accessor "getHeaderField" gives direct access to the value 3116 */ 3117 public StringType getHeaderFieldElement() { 3118 if (this.headerField == null) 3119 if (Configuration.errorOnAutoCreate()) 3120 throw new Error("Attempt to auto-create TestScriptVariableComponent.headerField"); 3121 else if (Configuration.doAutoCreate()) 3122 this.headerField = new StringType(); // bb 3123 return this.headerField; 3124 } 3125 3126 public boolean hasHeaderFieldElement() { 3127 return this.headerField != null && !this.headerField.isEmpty(); 3128 } 3129 3130 public boolean hasHeaderField() { 3131 return this.headerField != null && !this.headerField.isEmpty(); 3132 } 3133 3134 /** 3135 * @param value {@link #headerField} (Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.). This is the underlying object with id, value and extensions. The accessor "getHeaderField" gives direct access to the value 3136 */ 3137 public TestScriptVariableComponent setHeaderFieldElement(StringType value) { 3138 this.headerField = value; 3139 return this; 3140 } 3141 3142 /** 3143 * @return Will be used to grab the HTTP header field value from the headers that sourceId is pointing to. 3144 */ 3145 public String getHeaderField() { 3146 return this.headerField == null ? null : this.headerField.getValue(); 3147 } 3148 3149 /** 3150 * @param value Will be used to grab the HTTP header field value from the headers that sourceId is pointing to. 3151 */ 3152 public TestScriptVariableComponent setHeaderField(String value) { 3153 if (Utilities.noString(value)) 3154 this.headerField = null; 3155 else { 3156 if (this.headerField == null) 3157 this.headerField = new StringType(); 3158 this.headerField.setValue(value); 3159 } 3160 return this; 3161 } 3162 3163 /** 3164 * @return {@link #hint} (Displayable text string with hint help information to the user when entering a default value.). This is the underlying object with id, value and extensions. The accessor "getHint" gives direct access to the value 3165 */ 3166 public StringType getHintElement() { 3167 if (this.hint == null) 3168 if (Configuration.errorOnAutoCreate()) 3169 throw new Error("Attempt to auto-create TestScriptVariableComponent.hint"); 3170 else if (Configuration.doAutoCreate()) 3171 this.hint = new StringType(); // bb 3172 return this.hint; 3173 } 3174 3175 public boolean hasHintElement() { 3176 return this.hint != null && !this.hint.isEmpty(); 3177 } 3178 3179 public boolean hasHint() { 3180 return this.hint != null && !this.hint.isEmpty(); 3181 } 3182 3183 /** 3184 * @param value {@link #hint} (Displayable text string with hint help information to the user when entering a default value.). This is the underlying object with id, value and extensions. The accessor "getHint" gives direct access to the value 3185 */ 3186 public TestScriptVariableComponent setHintElement(StringType value) { 3187 this.hint = value; 3188 return this; 3189 } 3190 3191 /** 3192 * @return Displayable text string with hint help information to the user when entering a default value. 3193 */ 3194 public String getHint() { 3195 return this.hint == null ? null : this.hint.getValue(); 3196 } 3197 3198 /** 3199 * @param value Displayable text string with hint help information to the user when entering a default value. 3200 */ 3201 public TestScriptVariableComponent setHint(String value) { 3202 if (Utilities.noString(value)) 3203 this.hint = null; 3204 else { 3205 if (this.hint == null) 3206 this.hint = new StringType(); 3207 this.hint.setValue(value); 3208 } 3209 return this; 3210 } 3211 3212 /** 3213 * @return {@link #path} (XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 3214 */ 3215 public StringType getPathElement() { 3216 if (this.path == null) 3217 if (Configuration.errorOnAutoCreate()) 3218 throw new Error("Attempt to auto-create TestScriptVariableComponent.path"); 3219 else if (Configuration.doAutoCreate()) 3220 this.path = new StringType(); // bb 3221 return this.path; 3222 } 3223 3224 public boolean hasPathElement() { 3225 return this.path != null && !this.path.isEmpty(); 3226 } 3227 3228 public boolean hasPath() { 3229 return this.path != null && !this.path.isEmpty(); 3230 } 3231 3232 /** 3233 * @param value {@link #path} (XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 3234 */ 3235 public TestScriptVariableComponent setPathElement(StringType value) { 3236 this.path = value; 3237 return this; 3238 } 3239 3240 /** 3241 * @return XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 3242 */ 3243 public String getPath() { 3244 return this.path == null ? null : this.path.getValue(); 3245 } 3246 3247 /** 3248 * @param value XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified. 3249 */ 3250 public TestScriptVariableComponent setPath(String value) { 3251 if (Utilities.noString(value)) 3252 this.path = null; 3253 else { 3254 if (this.path == null) 3255 this.path = new StringType(); 3256 this.path.setValue(value); 3257 } 3258 return this; 3259 } 3260 3261 /** 3262 * @return {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 3263 */ 3264 public IdType getSourceIdElement() { 3265 if (this.sourceId == null) 3266 if (Configuration.errorOnAutoCreate()) 3267 throw new Error("Attempt to auto-create TestScriptVariableComponent.sourceId"); 3268 else if (Configuration.doAutoCreate()) 3269 this.sourceId = new IdType(); // bb 3270 return this.sourceId; 3271 } 3272 3273 public boolean hasSourceIdElement() { 3274 return this.sourceId != null && !this.sourceId.isEmpty(); 3275 } 3276 3277 public boolean hasSourceId() { 3278 return this.sourceId != null && !this.sourceId.isEmpty(); 3279 } 3280 3281 /** 3282 * @param value {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 3283 */ 3284 public TestScriptVariableComponent setSourceIdElement(IdType value) { 3285 this.sourceId = value; 3286 return this; 3287 } 3288 3289 /** 3290 * @return Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable. 3291 */ 3292 public String getSourceId() { 3293 return this.sourceId == null ? null : this.sourceId.getValue(); 3294 } 3295 3296 /** 3297 * @param value Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable. 3298 */ 3299 public TestScriptVariableComponent setSourceId(String value) { 3300 if (Utilities.noString(value)) 3301 this.sourceId = null; 3302 else { 3303 if (this.sourceId == null) 3304 this.sourceId = new IdType(); 3305 this.sourceId.setValue(value); 3306 } 3307 return this; 3308 } 3309 3310 protected void listChildren(List<Property> children) { 3311 super.listChildren(children); 3312 children.add(new Property("name", "string", "Descriptive name for this variable.", 0, 1, name)); 3313 children.add(new Property("defaultValue", "string", "A default, hard-coded, or user-defined value for this variable.", 0, 1, defaultValue)); 3314 children.add(new Property("description", "string", "A free text natural language description of the variable and its purpose.", 0, 1, description)); 3315 children.add(new Property("expression", "string", "The fluentpath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 0, 1, expression)); 3316 children.add(new Property("headerField", "string", "Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.", 0, 1, headerField)); 3317 children.add(new Property("hint", "string", "Displayable text string with hint help information to the user when entering a default value.", 0, 1, hint)); 3318 children.add(new Property("path", "string", "XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 0, 1, path)); 3319 children.add(new Property("sourceId", "id", "Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.", 0, 1, sourceId)); 3320 } 3321 3322 @Override 3323 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3324 switch (_hash) { 3325 case 3373707: /*name*/ return new Property("name", "string", "Descriptive name for this variable.", 0, 1, name); 3326 case -659125328: /*defaultValue*/ return new Property("defaultValue", "string", "A default, hard-coded, or user-defined value for this variable.", 0, 1, defaultValue); 3327 case -1724546052: /*description*/ return new Property("description", "string", "A free text natural language description of the variable and its purpose.", 0, 1, description); 3328 case -1795452264: /*expression*/ return new Property("expression", "string", "The fluentpath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 0, 1, expression); 3329 case 1160732269: /*headerField*/ return new Property("headerField", "string", "Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.", 0, 1, headerField); 3330 case 3202695: /*hint*/ return new Property("hint", "string", "Displayable text string with hint help information to the user when entering a default value.", 0, 1, hint); 3331 case 3433509: /*path*/ return new Property("path", "string", "XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 0, 1, path); 3332 case 1746327190: /*sourceId*/ return new Property("sourceId", "id", "Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.", 0, 1, sourceId); 3333 default: return super.getNamedProperty(_hash, _name, _checkValid); 3334 } 3335 3336 } 3337 3338 @Override 3339 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3340 switch (hash) { 3341 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3342 case -659125328: /*defaultValue*/ return this.defaultValue == null ? new Base[0] : new Base[] {this.defaultValue}; // StringType 3343 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 3344 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 3345 case 1160732269: /*headerField*/ return this.headerField == null ? new Base[0] : new Base[] {this.headerField}; // StringType 3346 case 3202695: /*hint*/ return this.hint == null ? new Base[0] : new Base[] {this.hint}; // StringType 3347 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 3348 case 1746327190: /*sourceId*/ return this.sourceId == null ? new Base[0] : new Base[] {this.sourceId}; // IdType 3349 default: return super.getProperty(hash, name, checkValid); 3350 } 3351 3352 } 3353 3354 @Override 3355 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3356 switch (hash) { 3357 case 3373707: // name 3358 this.name = castToString(value); // StringType 3359 return value; 3360 case -659125328: // defaultValue 3361 this.defaultValue = castToString(value); // StringType 3362 return value; 3363 case -1724546052: // description 3364 this.description = castToString(value); // StringType 3365 return value; 3366 case -1795452264: // expression 3367 this.expression = castToString(value); // StringType 3368 return value; 3369 case 1160732269: // headerField 3370 this.headerField = castToString(value); // StringType 3371 return value; 3372 case 3202695: // hint 3373 this.hint = castToString(value); // StringType 3374 return value; 3375 case 3433509: // path 3376 this.path = castToString(value); // StringType 3377 return value; 3378 case 1746327190: // sourceId 3379 this.sourceId = castToId(value); // IdType 3380 return value; 3381 default: return super.setProperty(hash, name, value); 3382 } 3383 3384 } 3385 3386 @Override 3387 public Base setProperty(String name, Base value) throws FHIRException { 3388 if (name.equals("name")) { 3389 this.name = castToString(value); // StringType 3390 } else if (name.equals("defaultValue")) { 3391 this.defaultValue = castToString(value); // StringType 3392 } else if (name.equals("description")) { 3393 this.description = castToString(value); // StringType 3394 } else if (name.equals("expression")) { 3395 this.expression = castToString(value); // StringType 3396 } else if (name.equals("headerField")) { 3397 this.headerField = castToString(value); // StringType 3398 } else if (name.equals("hint")) { 3399 this.hint = castToString(value); // StringType 3400 } else if (name.equals("path")) { 3401 this.path = castToString(value); // StringType 3402 } else if (name.equals("sourceId")) { 3403 this.sourceId = castToId(value); // IdType 3404 } else 3405 return super.setProperty(name, value); 3406 return value; 3407 } 3408 3409 @Override 3410 public Base makeProperty(int hash, String name) throws FHIRException { 3411 switch (hash) { 3412 case 3373707: return getNameElement(); 3413 case -659125328: return getDefaultValueElement(); 3414 case -1724546052: return getDescriptionElement(); 3415 case -1795452264: return getExpressionElement(); 3416 case 1160732269: return getHeaderFieldElement(); 3417 case 3202695: return getHintElement(); 3418 case 3433509: return getPathElement(); 3419 case 1746327190: return getSourceIdElement(); 3420 default: return super.makeProperty(hash, name); 3421 } 3422 3423 } 3424 3425 @Override 3426 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3427 switch (hash) { 3428 case 3373707: /*name*/ return new String[] {"string"}; 3429 case -659125328: /*defaultValue*/ return new String[] {"string"}; 3430 case -1724546052: /*description*/ return new String[] {"string"}; 3431 case -1795452264: /*expression*/ return new String[] {"string"}; 3432 case 1160732269: /*headerField*/ return new String[] {"string"}; 3433 case 3202695: /*hint*/ return new String[] {"string"}; 3434 case 3433509: /*path*/ return new String[] {"string"}; 3435 case 1746327190: /*sourceId*/ return new String[] {"id"}; 3436 default: return super.getTypesForProperty(hash, name); 3437 } 3438 3439 } 3440 3441 @Override 3442 public Base addChild(String name) throws FHIRException { 3443 if (name.equals("name")) { 3444 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 3445 } 3446 else if (name.equals("defaultValue")) { 3447 throw new FHIRException("Cannot call addChild on a singleton property TestScript.defaultValue"); 3448 } 3449 else if (name.equals("description")) { 3450 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 3451 } 3452 else if (name.equals("expression")) { 3453 throw new FHIRException("Cannot call addChild on a singleton property TestScript.expression"); 3454 } 3455 else if (name.equals("headerField")) { 3456 throw new FHIRException("Cannot call addChild on a singleton property TestScript.headerField"); 3457 } 3458 else if (name.equals("hint")) { 3459 throw new FHIRException("Cannot call addChild on a singleton property TestScript.hint"); 3460 } 3461 else if (name.equals("path")) { 3462 throw new FHIRException("Cannot call addChild on a singleton property TestScript.path"); 3463 } 3464 else if (name.equals("sourceId")) { 3465 throw new FHIRException("Cannot call addChild on a singleton property TestScript.sourceId"); 3466 } 3467 else 3468 return super.addChild(name); 3469 } 3470 3471 public TestScriptVariableComponent copy() { 3472 TestScriptVariableComponent dst = new TestScriptVariableComponent(); 3473 copyValues(dst); 3474 dst.name = name == null ? null : name.copy(); 3475 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 3476 dst.description = description == null ? null : description.copy(); 3477 dst.expression = expression == null ? null : expression.copy(); 3478 dst.headerField = headerField == null ? null : headerField.copy(); 3479 dst.hint = hint == null ? null : hint.copy(); 3480 dst.path = path == null ? null : path.copy(); 3481 dst.sourceId = sourceId == null ? null : sourceId.copy(); 3482 return dst; 3483 } 3484 3485 @Override 3486 public boolean equalsDeep(Base other_) { 3487 if (!super.equalsDeep(other_)) 3488 return false; 3489 if (!(other_ instanceof TestScriptVariableComponent)) 3490 return false; 3491 TestScriptVariableComponent o = (TestScriptVariableComponent) other_; 3492 return compareDeep(name, o.name, true) && compareDeep(defaultValue, o.defaultValue, true) && compareDeep(description, o.description, true) 3493 && compareDeep(expression, o.expression, true) && compareDeep(headerField, o.headerField, true) 3494 && compareDeep(hint, o.hint, true) && compareDeep(path, o.path, true) && compareDeep(sourceId, o.sourceId, true) 3495 ; 3496 } 3497 3498 @Override 3499 public boolean equalsShallow(Base other_) { 3500 if (!super.equalsShallow(other_)) 3501 return false; 3502 if (!(other_ instanceof TestScriptVariableComponent)) 3503 return false; 3504 TestScriptVariableComponent o = (TestScriptVariableComponent) other_; 3505 return compareValues(name, o.name, true) && compareValues(defaultValue, o.defaultValue, true) && compareValues(description, o.description, true) 3506 && compareValues(expression, o.expression, true) && compareValues(headerField, o.headerField, true) 3507 && compareValues(hint, o.hint, true) && compareValues(path, o.path, true) && compareValues(sourceId, o.sourceId, true) 3508 ; 3509 } 3510 3511 public boolean isEmpty() { 3512 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, defaultValue, description 3513 , expression, headerField, hint, path, sourceId); 3514 } 3515 3516 public String fhirType() { 3517 return "TestScript.variable"; 3518 3519 } 3520 3521 } 3522 3523 @Block() 3524 public static class TestScriptRuleComponent extends BackboneElement implements IBaseBackboneElement { 3525 /** 3526 * Reference to the resource (containing the contents of the rule needed for assertions). 3527 */ 3528 @Child(name = "resource", type = {Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 3529 @Description(shortDefinition="Assert rule resource reference", formalDefinition="Reference to the resource (containing the contents of the rule needed for assertions)." ) 3530 protected Reference resource; 3531 3532 /** 3533 * The actual object that is the target of the reference (Reference to the resource (containing the contents of the rule needed for assertions).) 3534 */ 3535 protected Resource resourceTarget; 3536 3537 /** 3538 * Each rule template can take one or more parameters for rule evaluation. 3539 */ 3540 @Child(name = "param", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3541 @Description(shortDefinition="Rule parameter template", formalDefinition="Each rule template can take one or more parameters for rule evaluation." ) 3542 protected List<RuleParamComponent> param; 3543 3544 private static final long serialVersionUID = -1869267735L; 3545 3546 /** 3547 * Constructor 3548 */ 3549 public TestScriptRuleComponent() { 3550 super(); 3551 } 3552 3553 /** 3554 * Constructor 3555 */ 3556 public TestScriptRuleComponent(Reference resource) { 3557 super(); 3558 this.resource = resource; 3559 } 3560 3561 /** 3562 * @return {@link #resource} (Reference to the resource (containing the contents of the rule needed for assertions).) 3563 */ 3564 public Reference getResource() { 3565 if (this.resource == null) 3566 if (Configuration.errorOnAutoCreate()) 3567 throw new Error("Attempt to auto-create TestScriptRuleComponent.resource"); 3568 else if (Configuration.doAutoCreate()) 3569 this.resource = new Reference(); // cc 3570 return this.resource; 3571 } 3572 3573 public boolean hasResource() { 3574 return this.resource != null && !this.resource.isEmpty(); 3575 } 3576 3577 /** 3578 * @param value {@link #resource} (Reference to the resource (containing the contents of the rule needed for assertions).) 3579 */ 3580 public TestScriptRuleComponent setResource(Reference value) { 3581 this.resource = value; 3582 return this; 3583 } 3584 3585 /** 3586 * @return {@link #resource} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the resource (containing the contents of the rule needed for assertions).) 3587 */ 3588 public Resource getResourceTarget() { 3589 return this.resourceTarget; 3590 } 3591 3592 /** 3593 * @param value {@link #resource} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the resource (containing the contents of the rule needed for assertions).) 3594 */ 3595 public TestScriptRuleComponent setResourceTarget(Resource value) { 3596 this.resourceTarget = value; 3597 return this; 3598 } 3599 3600 /** 3601 * @return {@link #param} (Each rule template can take one or more parameters for rule evaluation.) 3602 */ 3603 public List<RuleParamComponent> getParam() { 3604 if (this.param == null) 3605 this.param = new ArrayList<RuleParamComponent>(); 3606 return this.param; 3607 } 3608 3609 /** 3610 * @return Returns a reference to <code>this</code> for easy method chaining 3611 */ 3612 public TestScriptRuleComponent setParam(List<RuleParamComponent> theParam) { 3613 this.param = theParam; 3614 return this; 3615 } 3616 3617 public boolean hasParam() { 3618 if (this.param == null) 3619 return false; 3620 for (RuleParamComponent item : this.param) 3621 if (!item.isEmpty()) 3622 return true; 3623 return false; 3624 } 3625 3626 public RuleParamComponent addParam() { //3 3627 RuleParamComponent t = new RuleParamComponent(); 3628 if (this.param == null) 3629 this.param = new ArrayList<RuleParamComponent>(); 3630 this.param.add(t); 3631 return t; 3632 } 3633 3634 public TestScriptRuleComponent addParam(RuleParamComponent t) { //3 3635 if (t == null) 3636 return this; 3637 if (this.param == null) 3638 this.param = new ArrayList<RuleParamComponent>(); 3639 this.param.add(t); 3640 return this; 3641 } 3642 3643 /** 3644 * @return The first repetition of repeating field {@link #param}, creating it if it does not already exist 3645 */ 3646 public RuleParamComponent getParamFirstRep() { 3647 if (getParam().isEmpty()) { 3648 addParam(); 3649 } 3650 return getParam().get(0); 3651 } 3652 3653 protected void listChildren(List<Property> children) { 3654 super.listChildren(children); 3655 children.add(new Property("resource", "Reference(Any)", "Reference to the resource (containing the contents of the rule needed for assertions).", 0, 1, resource)); 3656 children.add(new Property("param", "", "Each rule template can take one or more parameters for rule evaluation.", 0, java.lang.Integer.MAX_VALUE, param)); 3657 } 3658 3659 @Override 3660 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3661 switch (_hash) { 3662 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "Reference to the resource (containing the contents of the rule needed for assertions).", 0, 1, resource); 3663 case 106436749: /*param*/ return new Property("param", "", "Each rule template can take one or more parameters for rule evaluation.", 0, java.lang.Integer.MAX_VALUE, param); 3664 default: return super.getNamedProperty(_hash, _name, _checkValid); 3665 } 3666 3667 } 3668 3669 @Override 3670 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3671 switch (hash) { 3672 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 3673 case 106436749: /*param*/ return this.param == null ? new Base[0] : this.param.toArray(new Base[this.param.size()]); // RuleParamComponent 3674 default: return super.getProperty(hash, name, checkValid); 3675 } 3676 3677 } 3678 3679 @Override 3680 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3681 switch (hash) { 3682 case -341064690: // resource 3683 this.resource = castToReference(value); // Reference 3684 return value; 3685 case 106436749: // param 3686 this.getParam().add((RuleParamComponent) value); // RuleParamComponent 3687 return value; 3688 default: return super.setProperty(hash, name, value); 3689 } 3690 3691 } 3692 3693 @Override 3694 public Base setProperty(String name, Base value) throws FHIRException { 3695 if (name.equals("resource")) { 3696 this.resource = castToReference(value); // Reference 3697 } else if (name.equals("param")) { 3698 this.getParam().add((RuleParamComponent) value); 3699 } else 3700 return super.setProperty(name, value); 3701 return value; 3702 } 3703 3704 @Override 3705 public Base makeProperty(int hash, String name) throws FHIRException { 3706 switch (hash) { 3707 case -341064690: return getResource(); 3708 case 106436749: return addParam(); 3709 default: return super.makeProperty(hash, name); 3710 } 3711 3712 } 3713 3714 @Override 3715 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3716 switch (hash) { 3717 case -341064690: /*resource*/ return new String[] {"Reference"}; 3718 case 106436749: /*param*/ return new String[] {}; 3719 default: return super.getTypesForProperty(hash, name); 3720 } 3721 3722 } 3723 3724 @Override 3725 public Base addChild(String name) throws FHIRException { 3726 if (name.equals("resource")) { 3727 this.resource = new Reference(); 3728 return this.resource; 3729 } 3730 else if (name.equals("param")) { 3731 return addParam(); 3732 } 3733 else 3734 return super.addChild(name); 3735 } 3736 3737 public TestScriptRuleComponent copy() { 3738 TestScriptRuleComponent dst = new TestScriptRuleComponent(); 3739 copyValues(dst); 3740 dst.resource = resource == null ? null : resource.copy(); 3741 if (param != null) { 3742 dst.param = new ArrayList<RuleParamComponent>(); 3743 for (RuleParamComponent i : param) 3744 dst.param.add(i.copy()); 3745 }; 3746 return dst; 3747 } 3748 3749 @Override 3750 public boolean equalsDeep(Base other_) { 3751 if (!super.equalsDeep(other_)) 3752 return false; 3753 if (!(other_ instanceof TestScriptRuleComponent)) 3754 return false; 3755 TestScriptRuleComponent o = (TestScriptRuleComponent) other_; 3756 return compareDeep(resource, o.resource, true) && compareDeep(param, o.param, true); 3757 } 3758 3759 @Override 3760 public boolean equalsShallow(Base other_) { 3761 if (!super.equalsShallow(other_)) 3762 return false; 3763 if (!(other_ instanceof TestScriptRuleComponent)) 3764 return false; 3765 TestScriptRuleComponent o = (TestScriptRuleComponent) other_; 3766 return true; 3767 } 3768 3769 public boolean isEmpty() { 3770 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(resource, param); 3771 } 3772 3773 public String fhirType() { 3774 return "TestScript.rule"; 3775 3776 } 3777 3778 } 3779 3780 @Block() 3781 public static class RuleParamComponent extends BackboneElement implements IBaseBackboneElement { 3782 /** 3783 * Descriptive name for this parameter that matches the external assert rule parameter name. 3784 */ 3785 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3786 @Description(shortDefinition="Parameter name matching external assert rule parameter", formalDefinition="Descriptive name for this parameter that matches the external assert rule parameter name." ) 3787 protected StringType name; 3788 3789 /** 3790 * The explicit or dynamic value for the parameter that will be passed on to the external rule template. 3791 */ 3792 @Child(name = "value", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3793 @Description(shortDefinition="Parameter value defined either explicitly or dynamically", formalDefinition="The explicit or dynamic value for the parameter that will be passed on to the external rule template." ) 3794 protected StringType value; 3795 3796 private static final long serialVersionUID = 395259392L; 3797 3798 /** 3799 * Constructor 3800 */ 3801 public RuleParamComponent() { 3802 super(); 3803 } 3804 3805 /** 3806 * Constructor 3807 */ 3808 public RuleParamComponent(StringType name) { 3809 super(); 3810 this.name = name; 3811 } 3812 3813 /** 3814 * @return {@link #name} (Descriptive name for this parameter that matches the external assert rule parameter name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3815 */ 3816 public StringType getNameElement() { 3817 if (this.name == null) 3818 if (Configuration.errorOnAutoCreate()) 3819 throw new Error("Attempt to auto-create RuleParamComponent.name"); 3820 else if (Configuration.doAutoCreate()) 3821 this.name = new StringType(); // bb 3822 return this.name; 3823 } 3824 3825 public boolean hasNameElement() { 3826 return this.name != null && !this.name.isEmpty(); 3827 } 3828 3829 public boolean hasName() { 3830 return this.name != null && !this.name.isEmpty(); 3831 } 3832 3833 /** 3834 * @param value {@link #name} (Descriptive name for this parameter that matches the external assert rule parameter name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3835 */ 3836 public RuleParamComponent setNameElement(StringType value) { 3837 this.name = value; 3838 return this; 3839 } 3840 3841 /** 3842 * @return Descriptive name for this parameter that matches the external assert rule parameter name. 3843 */ 3844 public String getName() { 3845 return this.name == null ? null : this.name.getValue(); 3846 } 3847 3848 /** 3849 * @param value Descriptive name for this parameter that matches the external assert rule parameter name. 3850 */ 3851 public RuleParamComponent setName(String value) { 3852 if (this.name == null) 3853 this.name = new StringType(); 3854 this.name.setValue(value); 3855 return this; 3856 } 3857 3858 /** 3859 * @return {@link #value} (The explicit or dynamic value for the parameter that will be passed on to the external rule template.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 3860 */ 3861 public StringType getValueElement() { 3862 if (this.value == null) 3863 if (Configuration.errorOnAutoCreate()) 3864 throw new Error("Attempt to auto-create RuleParamComponent.value"); 3865 else if (Configuration.doAutoCreate()) 3866 this.value = new StringType(); // bb 3867 return this.value; 3868 } 3869 3870 public boolean hasValueElement() { 3871 return this.value != null && !this.value.isEmpty(); 3872 } 3873 3874 public boolean hasValue() { 3875 return this.value != null && !this.value.isEmpty(); 3876 } 3877 3878 /** 3879 * @param value {@link #value} (The explicit or dynamic value for the parameter that will be passed on to the external rule template.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 3880 */ 3881 public RuleParamComponent setValueElement(StringType value) { 3882 this.value = value; 3883 return this; 3884 } 3885 3886 /** 3887 * @return The explicit or dynamic value for the parameter that will be passed on to the external rule template. 3888 */ 3889 public String getValue() { 3890 return this.value == null ? null : this.value.getValue(); 3891 } 3892 3893 /** 3894 * @param value The explicit or dynamic value for the parameter that will be passed on to the external rule template. 3895 */ 3896 public RuleParamComponent setValue(String value) { 3897 if (Utilities.noString(value)) 3898 this.value = null; 3899 else { 3900 if (this.value == null) 3901 this.value = new StringType(); 3902 this.value.setValue(value); 3903 } 3904 return this; 3905 } 3906 3907 protected void listChildren(List<Property> children) { 3908 super.listChildren(children); 3909 children.add(new Property("name", "string", "Descriptive name for this parameter that matches the external assert rule parameter name.", 0, 1, name)); 3910 children.add(new Property("value", "string", "The explicit or dynamic value for the parameter that will be passed on to the external rule template.", 0, 1, value)); 3911 } 3912 3913 @Override 3914 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3915 switch (_hash) { 3916 case 3373707: /*name*/ return new Property("name", "string", "Descriptive name for this parameter that matches the external assert rule parameter name.", 0, 1, name); 3917 case 111972721: /*value*/ return new Property("value", "string", "The explicit or dynamic value for the parameter that will be passed on to the external rule template.", 0, 1, value); 3918 default: return super.getNamedProperty(_hash, _name, _checkValid); 3919 } 3920 3921 } 3922 3923 @Override 3924 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3925 switch (hash) { 3926 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3927 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 3928 default: return super.getProperty(hash, name, checkValid); 3929 } 3930 3931 } 3932 3933 @Override 3934 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3935 switch (hash) { 3936 case 3373707: // name 3937 this.name = castToString(value); // StringType 3938 return value; 3939 case 111972721: // value 3940 this.value = castToString(value); // StringType 3941 return value; 3942 default: return super.setProperty(hash, name, value); 3943 } 3944 3945 } 3946 3947 @Override 3948 public Base setProperty(String name, Base value) throws FHIRException { 3949 if (name.equals("name")) { 3950 this.name = castToString(value); // StringType 3951 } else if (name.equals("value")) { 3952 this.value = castToString(value); // StringType 3953 } else 3954 return super.setProperty(name, value); 3955 return value; 3956 } 3957 3958 @Override 3959 public Base makeProperty(int hash, String name) throws FHIRException { 3960 switch (hash) { 3961 case 3373707: return getNameElement(); 3962 case 111972721: return getValueElement(); 3963 default: return super.makeProperty(hash, name); 3964 } 3965 3966 } 3967 3968 @Override 3969 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3970 switch (hash) { 3971 case 3373707: /*name*/ return new String[] {"string"}; 3972 case 111972721: /*value*/ return new String[] {"string"}; 3973 default: return super.getTypesForProperty(hash, name); 3974 } 3975 3976 } 3977 3978 @Override 3979 public Base addChild(String name) throws FHIRException { 3980 if (name.equals("name")) { 3981 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 3982 } 3983 else if (name.equals("value")) { 3984 throw new FHIRException("Cannot call addChild on a singleton property TestScript.value"); 3985 } 3986 else 3987 return super.addChild(name); 3988 } 3989 3990 public RuleParamComponent copy() { 3991 RuleParamComponent dst = new RuleParamComponent(); 3992 copyValues(dst); 3993 dst.name = name == null ? null : name.copy(); 3994 dst.value = value == null ? null : value.copy(); 3995 return dst; 3996 } 3997 3998 @Override 3999 public boolean equalsDeep(Base other_) { 4000 if (!super.equalsDeep(other_)) 4001 return false; 4002 if (!(other_ instanceof RuleParamComponent)) 4003 return false; 4004 RuleParamComponent o = (RuleParamComponent) other_; 4005 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 4006 } 4007 4008 @Override 4009 public boolean equalsShallow(Base other_) { 4010 if (!super.equalsShallow(other_)) 4011 return false; 4012 if (!(other_ instanceof RuleParamComponent)) 4013 return false; 4014 RuleParamComponent o = (RuleParamComponent) other_; 4015 return compareValues(name, o.name, true) && compareValues(value, o.value, true); 4016 } 4017 4018 public boolean isEmpty() { 4019 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 4020 } 4021 4022 public String fhirType() { 4023 return "TestScript.rule.param"; 4024 4025 } 4026 4027 } 4028 4029 @Block() 4030 public static class TestScriptRulesetComponent extends BackboneElement implements IBaseBackboneElement { 4031 /** 4032 * Reference to the resource (containing the contents of the ruleset needed for assertions). 4033 */ 4034 @Child(name = "resource", type = {Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 4035 @Description(shortDefinition="Assert ruleset resource reference", formalDefinition="Reference to the resource (containing the contents of the ruleset needed for assertions)." ) 4036 protected Reference resource; 4037 4038 /** 4039 * The actual object that is the target of the reference (Reference to the resource (containing the contents of the ruleset needed for assertions).) 4040 */ 4041 protected Resource resourceTarget; 4042 4043 /** 4044 * The referenced rule within the external ruleset template. 4045 */ 4046 @Child(name = "rule", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4047 @Description(shortDefinition="The referenced rule within the ruleset", formalDefinition="The referenced rule within the external ruleset template." ) 4048 protected List<RulesetRuleComponent> rule; 4049 4050 private static final long serialVersionUID = 1260261423L; 4051 4052 /** 4053 * Constructor 4054 */ 4055 public TestScriptRulesetComponent() { 4056 super(); 4057 } 4058 4059 /** 4060 * Constructor 4061 */ 4062 public TestScriptRulesetComponent(Reference resource) { 4063 super(); 4064 this.resource = resource; 4065 } 4066 4067 /** 4068 * @return {@link #resource} (Reference to the resource (containing the contents of the ruleset needed for assertions).) 4069 */ 4070 public Reference getResource() { 4071 if (this.resource == null) 4072 if (Configuration.errorOnAutoCreate()) 4073 throw new Error("Attempt to auto-create TestScriptRulesetComponent.resource"); 4074 else if (Configuration.doAutoCreate()) 4075 this.resource = new Reference(); // cc 4076 return this.resource; 4077 } 4078 4079 public boolean hasResource() { 4080 return this.resource != null && !this.resource.isEmpty(); 4081 } 4082 4083 /** 4084 * @param value {@link #resource} (Reference to the resource (containing the contents of the ruleset needed for assertions).) 4085 */ 4086 public TestScriptRulesetComponent setResource(Reference value) { 4087 this.resource = value; 4088 return this; 4089 } 4090 4091 /** 4092 * @return {@link #resource} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the resource (containing the contents of the ruleset needed for assertions).) 4093 */ 4094 public Resource getResourceTarget() { 4095 return this.resourceTarget; 4096 } 4097 4098 /** 4099 * @param value {@link #resource} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the resource (containing the contents of the ruleset needed for assertions).) 4100 */ 4101 public TestScriptRulesetComponent setResourceTarget(Resource value) { 4102 this.resourceTarget = value; 4103 return this; 4104 } 4105 4106 /** 4107 * @return {@link #rule} (The referenced rule within the external ruleset template.) 4108 */ 4109 public List<RulesetRuleComponent> getRule() { 4110 if (this.rule == null) 4111 this.rule = new ArrayList<RulesetRuleComponent>(); 4112 return this.rule; 4113 } 4114 4115 /** 4116 * @return Returns a reference to <code>this</code> for easy method chaining 4117 */ 4118 public TestScriptRulesetComponent setRule(List<RulesetRuleComponent> theRule) { 4119 this.rule = theRule; 4120 return this; 4121 } 4122 4123 public boolean hasRule() { 4124 if (this.rule == null) 4125 return false; 4126 for (RulesetRuleComponent item : this.rule) 4127 if (!item.isEmpty()) 4128 return true; 4129 return false; 4130 } 4131 4132 public RulesetRuleComponent addRule() { //3 4133 RulesetRuleComponent t = new RulesetRuleComponent(); 4134 if (this.rule == null) 4135 this.rule = new ArrayList<RulesetRuleComponent>(); 4136 this.rule.add(t); 4137 return t; 4138 } 4139 4140 public TestScriptRulesetComponent addRule(RulesetRuleComponent t) { //3 4141 if (t == null) 4142 return this; 4143 if (this.rule == null) 4144 this.rule = new ArrayList<RulesetRuleComponent>(); 4145 this.rule.add(t); 4146 return this; 4147 } 4148 4149 /** 4150 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist 4151 */ 4152 public RulesetRuleComponent getRuleFirstRep() { 4153 if (getRule().isEmpty()) { 4154 addRule(); 4155 } 4156 return getRule().get(0); 4157 } 4158 4159 protected void listChildren(List<Property> children) { 4160 super.listChildren(children); 4161 children.add(new Property("resource", "Reference(Any)", "Reference to the resource (containing the contents of the ruleset needed for assertions).", 0, 1, resource)); 4162 children.add(new Property("rule", "", "The referenced rule within the external ruleset template.", 0, java.lang.Integer.MAX_VALUE, rule)); 4163 } 4164 4165 @Override 4166 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4167 switch (_hash) { 4168 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "Reference to the resource (containing the contents of the ruleset needed for assertions).", 0, 1, resource); 4169 case 3512060: /*rule*/ return new Property("rule", "", "The referenced rule within the external ruleset template.", 0, java.lang.Integer.MAX_VALUE, rule); 4170 default: return super.getNamedProperty(_hash, _name, _checkValid); 4171 } 4172 4173 } 4174 4175 @Override 4176 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4177 switch (hash) { 4178 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 4179 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // RulesetRuleComponent 4180 default: return super.getProperty(hash, name, checkValid); 4181 } 4182 4183 } 4184 4185 @Override 4186 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4187 switch (hash) { 4188 case -341064690: // resource 4189 this.resource = castToReference(value); // Reference 4190 return value; 4191 case 3512060: // rule 4192 this.getRule().add((RulesetRuleComponent) value); // RulesetRuleComponent 4193 return value; 4194 default: return super.setProperty(hash, name, value); 4195 } 4196 4197 } 4198 4199 @Override 4200 public Base setProperty(String name, Base value) throws FHIRException { 4201 if (name.equals("resource")) { 4202 this.resource = castToReference(value); // Reference 4203 } else if (name.equals("rule")) { 4204 this.getRule().add((RulesetRuleComponent) value); 4205 } else 4206 return super.setProperty(name, value); 4207 return value; 4208 } 4209 4210 @Override 4211 public Base makeProperty(int hash, String name) throws FHIRException { 4212 switch (hash) { 4213 case -341064690: return getResource(); 4214 case 3512060: return addRule(); 4215 default: return super.makeProperty(hash, name); 4216 } 4217 4218 } 4219 4220 @Override 4221 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4222 switch (hash) { 4223 case -341064690: /*resource*/ return new String[] {"Reference"}; 4224 case 3512060: /*rule*/ return new String[] {}; 4225 default: return super.getTypesForProperty(hash, name); 4226 } 4227 4228 } 4229 4230 @Override 4231 public Base addChild(String name) throws FHIRException { 4232 if (name.equals("resource")) { 4233 this.resource = new Reference(); 4234 return this.resource; 4235 } 4236 else if (name.equals("rule")) { 4237 return addRule(); 4238 } 4239 else 4240 return super.addChild(name); 4241 } 4242 4243 public TestScriptRulesetComponent copy() { 4244 TestScriptRulesetComponent dst = new TestScriptRulesetComponent(); 4245 copyValues(dst); 4246 dst.resource = resource == null ? null : resource.copy(); 4247 if (rule != null) { 4248 dst.rule = new ArrayList<RulesetRuleComponent>(); 4249 for (RulesetRuleComponent i : rule) 4250 dst.rule.add(i.copy()); 4251 }; 4252 return dst; 4253 } 4254 4255 @Override 4256 public boolean equalsDeep(Base other_) { 4257 if (!super.equalsDeep(other_)) 4258 return false; 4259 if (!(other_ instanceof TestScriptRulesetComponent)) 4260 return false; 4261 TestScriptRulesetComponent o = (TestScriptRulesetComponent) other_; 4262 return compareDeep(resource, o.resource, true) && compareDeep(rule, o.rule, true); 4263 } 4264 4265 @Override 4266 public boolean equalsShallow(Base other_) { 4267 if (!super.equalsShallow(other_)) 4268 return false; 4269 if (!(other_ instanceof TestScriptRulesetComponent)) 4270 return false; 4271 TestScriptRulesetComponent o = (TestScriptRulesetComponent) other_; 4272 return true; 4273 } 4274 4275 public boolean isEmpty() { 4276 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(resource, rule); 4277 } 4278 4279 public String fhirType() { 4280 return "TestScript.ruleset"; 4281 4282 } 4283 4284 } 4285 4286 @Block() 4287 public static class RulesetRuleComponent extends BackboneElement implements IBaseBackboneElement { 4288 /** 4289 * Id of the referenced rule within the external ruleset template. 4290 */ 4291 @Child(name = "ruleId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4292 @Description(shortDefinition="Id of referenced rule within the ruleset", formalDefinition="Id of the referenced rule within the external ruleset template." ) 4293 protected IdType ruleId; 4294 4295 /** 4296 * Each rule template can take one or more parameters for rule evaluation. 4297 */ 4298 @Child(name = "param", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4299 @Description(shortDefinition="Ruleset rule parameter template", formalDefinition="Each rule template can take one or more parameters for rule evaluation." ) 4300 protected List<RulesetRuleParamComponent> param; 4301 4302 private static final long serialVersionUID = -1399866981L; 4303 4304 /** 4305 * Constructor 4306 */ 4307 public RulesetRuleComponent() { 4308 super(); 4309 } 4310 4311 /** 4312 * Constructor 4313 */ 4314 public RulesetRuleComponent(IdType ruleId) { 4315 super(); 4316 this.ruleId = ruleId; 4317 } 4318 4319 /** 4320 * @return {@link #ruleId} (Id of the referenced rule within the external ruleset template.). This is the underlying object with id, value and extensions. The accessor "getRuleId" gives direct access to the value 4321 */ 4322 public IdType getRuleIdElement() { 4323 if (this.ruleId == null) 4324 if (Configuration.errorOnAutoCreate()) 4325 throw new Error("Attempt to auto-create RulesetRuleComponent.ruleId"); 4326 else if (Configuration.doAutoCreate()) 4327 this.ruleId = new IdType(); // bb 4328 return this.ruleId; 4329 } 4330 4331 public boolean hasRuleIdElement() { 4332 return this.ruleId != null && !this.ruleId.isEmpty(); 4333 } 4334 4335 public boolean hasRuleId() { 4336 return this.ruleId != null && !this.ruleId.isEmpty(); 4337 } 4338 4339 /** 4340 * @param value {@link #ruleId} (Id of the referenced rule within the external ruleset template.). This is the underlying object with id, value and extensions. The accessor "getRuleId" gives direct access to the value 4341 */ 4342 public RulesetRuleComponent setRuleIdElement(IdType value) { 4343 this.ruleId = value; 4344 return this; 4345 } 4346 4347 /** 4348 * @return Id of the referenced rule within the external ruleset template. 4349 */ 4350 public String getRuleId() { 4351 return this.ruleId == null ? null : this.ruleId.getValue(); 4352 } 4353 4354 /** 4355 * @param value Id of the referenced rule within the external ruleset template. 4356 */ 4357 public RulesetRuleComponent setRuleId(String value) { 4358 if (this.ruleId == null) 4359 this.ruleId = new IdType(); 4360 this.ruleId.setValue(value); 4361 return this; 4362 } 4363 4364 /** 4365 * @return {@link #param} (Each rule template can take one or more parameters for rule evaluation.) 4366 */ 4367 public List<RulesetRuleParamComponent> getParam() { 4368 if (this.param == null) 4369 this.param = new ArrayList<RulesetRuleParamComponent>(); 4370 return this.param; 4371 } 4372 4373 /** 4374 * @return Returns a reference to <code>this</code> for easy method chaining 4375 */ 4376 public RulesetRuleComponent setParam(List<RulesetRuleParamComponent> theParam) { 4377 this.param = theParam; 4378 return this; 4379 } 4380 4381 public boolean hasParam() { 4382 if (this.param == null) 4383 return false; 4384 for (RulesetRuleParamComponent item : this.param) 4385 if (!item.isEmpty()) 4386 return true; 4387 return false; 4388 } 4389 4390 public RulesetRuleParamComponent addParam() { //3 4391 RulesetRuleParamComponent t = new RulesetRuleParamComponent(); 4392 if (this.param == null) 4393 this.param = new ArrayList<RulesetRuleParamComponent>(); 4394 this.param.add(t); 4395 return t; 4396 } 4397 4398 public RulesetRuleComponent addParam(RulesetRuleParamComponent t) { //3 4399 if (t == null) 4400 return this; 4401 if (this.param == null) 4402 this.param = new ArrayList<RulesetRuleParamComponent>(); 4403 this.param.add(t); 4404 return this; 4405 } 4406 4407 /** 4408 * @return The first repetition of repeating field {@link #param}, creating it if it does not already exist 4409 */ 4410 public RulesetRuleParamComponent getParamFirstRep() { 4411 if (getParam().isEmpty()) { 4412 addParam(); 4413 } 4414 return getParam().get(0); 4415 } 4416 4417 protected void listChildren(List<Property> children) { 4418 super.listChildren(children); 4419 children.add(new Property("ruleId", "id", "Id of the referenced rule within the external ruleset template.", 0, 1, ruleId)); 4420 children.add(new Property("param", "", "Each rule template can take one or more parameters for rule evaluation.", 0, java.lang.Integer.MAX_VALUE, param)); 4421 } 4422 4423 @Override 4424 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4425 switch (_hash) { 4426 case -919875273: /*ruleId*/ return new Property("ruleId", "id", "Id of the referenced rule within the external ruleset template.", 0, 1, ruleId); 4427 case 106436749: /*param*/ return new Property("param", "", "Each rule template can take one or more parameters for rule evaluation.", 0, java.lang.Integer.MAX_VALUE, param); 4428 default: return super.getNamedProperty(_hash, _name, _checkValid); 4429 } 4430 4431 } 4432 4433 @Override 4434 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4435 switch (hash) { 4436 case -919875273: /*ruleId*/ return this.ruleId == null ? new Base[0] : new Base[] {this.ruleId}; // IdType 4437 case 106436749: /*param*/ return this.param == null ? new Base[0] : this.param.toArray(new Base[this.param.size()]); // RulesetRuleParamComponent 4438 default: return super.getProperty(hash, name, checkValid); 4439 } 4440 4441 } 4442 4443 @Override 4444 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4445 switch (hash) { 4446 case -919875273: // ruleId 4447 this.ruleId = castToId(value); // IdType 4448 return value; 4449 case 106436749: // param 4450 this.getParam().add((RulesetRuleParamComponent) value); // RulesetRuleParamComponent 4451 return value; 4452 default: return super.setProperty(hash, name, value); 4453 } 4454 4455 } 4456 4457 @Override 4458 public Base setProperty(String name, Base value) throws FHIRException { 4459 if (name.equals("ruleId")) { 4460 this.ruleId = castToId(value); // IdType 4461 } else if (name.equals("param")) { 4462 this.getParam().add((RulesetRuleParamComponent) value); 4463 } else 4464 return super.setProperty(name, value); 4465 return value; 4466 } 4467 4468 @Override 4469 public Base makeProperty(int hash, String name) throws FHIRException { 4470 switch (hash) { 4471 case -919875273: return getRuleIdElement(); 4472 case 106436749: return addParam(); 4473 default: return super.makeProperty(hash, name); 4474 } 4475 4476 } 4477 4478 @Override 4479 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4480 switch (hash) { 4481 case -919875273: /*ruleId*/ return new String[] {"id"}; 4482 case 106436749: /*param*/ return new String[] {}; 4483 default: return super.getTypesForProperty(hash, name); 4484 } 4485 4486 } 4487 4488 @Override 4489 public Base addChild(String name) throws FHIRException { 4490 if (name.equals("ruleId")) { 4491 throw new FHIRException("Cannot call addChild on a singleton property TestScript.ruleId"); 4492 } 4493 else if (name.equals("param")) { 4494 return addParam(); 4495 } 4496 else 4497 return super.addChild(name); 4498 } 4499 4500 public RulesetRuleComponent copy() { 4501 RulesetRuleComponent dst = new RulesetRuleComponent(); 4502 copyValues(dst); 4503 dst.ruleId = ruleId == null ? null : ruleId.copy(); 4504 if (param != null) { 4505 dst.param = new ArrayList<RulesetRuleParamComponent>(); 4506 for (RulesetRuleParamComponent i : param) 4507 dst.param.add(i.copy()); 4508 }; 4509 return dst; 4510 } 4511 4512 @Override 4513 public boolean equalsDeep(Base other_) { 4514 if (!super.equalsDeep(other_)) 4515 return false; 4516 if (!(other_ instanceof RulesetRuleComponent)) 4517 return false; 4518 RulesetRuleComponent o = (RulesetRuleComponent) other_; 4519 return compareDeep(ruleId, o.ruleId, true) && compareDeep(param, o.param, true); 4520 } 4521 4522 @Override 4523 public boolean equalsShallow(Base other_) { 4524 if (!super.equalsShallow(other_)) 4525 return false; 4526 if (!(other_ instanceof RulesetRuleComponent)) 4527 return false; 4528 RulesetRuleComponent o = (RulesetRuleComponent) other_; 4529 return compareValues(ruleId, o.ruleId, true); 4530 } 4531 4532 public boolean isEmpty() { 4533 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(ruleId, param); 4534 } 4535 4536 public String fhirType() { 4537 return "TestScript.ruleset.rule"; 4538 4539 } 4540 4541 } 4542 4543 @Block() 4544 public static class RulesetRuleParamComponent extends BackboneElement implements IBaseBackboneElement { 4545 /** 4546 * Descriptive name for this parameter that matches the external assert ruleset rule parameter name. 4547 */ 4548 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 4549 @Description(shortDefinition="Parameter name matching external assert ruleset rule parameter", formalDefinition="Descriptive name for this parameter that matches the external assert ruleset rule parameter name." ) 4550 protected StringType name; 4551 4552 /** 4553 * The value for the parameter that will be passed on to the external ruleset rule template. 4554 */ 4555 @Child(name = "value", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 4556 @Description(shortDefinition="Parameter value defined either explicitly or dynamically", formalDefinition="The value for the parameter that will be passed on to the external ruleset rule template." ) 4557 protected StringType value; 4558 4559 private static final long serialVersionUID = 395259392L; 4560 4561 /** 4562 * Constructor 4563 */ 4564 public RulesetRuleParamComponent() { 4565 super(); 4566 } 4567 4568 /** 4569 * Constructor 4570 */ 4571 public RulesetRuleParamComponent(StringType name) { 4572 super(); 4573 this.name = name; 4574 } 4575 4576 /** 4577 * @return {@link #name} (Descriptive name for this parameter that matches the external assert ruleset rule parameter name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4578 */ 4579 public StringType getNameElement() { 4580 if (this.name == null) 4581 if (Configuration.errorOnAutoCreate()) 4582 throw new Error("Attempt to auto-create RulesetRuleParamComponent.name"); 4583 else if (Configuration.doAutoCreate()) 4584 this.name = new StringType(); // bb 4585 return this.name; 4586 } 4587 4588 public boolean hasNameElement() { 4589 return this.name != null && !this.name.isEmpty(); 4590 } 4591 4592 public boolean hasName() { 4593 return this.name != null && !this.name.isEmpty(); 4594 } 4595 4596 /** 4597 * @param value {@link #name} (Descriptive name for this parameter that matches the external assert ruleset rule parameter name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4598 */ 4599 public RulesetRuleParamComponent setNameElement(StringType value) { 4600 this.name = value; 4601 return this; 4602 } 4603 4604 /** 4605 * @return Descriptive name for this parameter that matches the external assert ruleset rule parameter name. 4606 */ 4607 public String getName() { 4608 return this.name == null ? null : this.name.getValue(); 4609 } 4610 4611 /** 4612 * @param value Descriptive name for this parameter that matches the external assert ruleset rule parameter name. 4613 */ 4614 public RulesetRuleParamComponent setName(String value) { 4615 if (this.name == null) 4616 this.name = new StringType(); 4617 this.name.setValue(value); 4618 return this; 4619 } 4620 4621 /** 4622 * @return {@link #value} (The value for the parameter that will be passed on to the external ruleset rule template.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 4623 */ 4624 public StringType getValueElement() { 4625 if (this.value == null) 4626 if (Configuration.errorOnAutoCreate()) 4627 throw new Error("Attempt to auto-create RulesetRuleParamComponent.value"); 4628 else if (Configuration.doAutoCreate()) 4629 this.value = new StringType(); // bb 4630 return this.value; 4631 } 4632 4633 public boolean hasValueElement() { 4634 return this.value != null && !this.value.isEmpty(); 4635 } 4636 4637 public boolean hasValue() { 4638 return this.value != null && !this.value.isEmpty(); 4639 } 4640 4641 /** 4642 * @param value {@link #value} (The value for the parameter that will be passed on to the external ruleset rule template.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 4643 */ 4644 public RulesetRuleParamComponent setValueElement(StringType value) { 4645 this.value = value; 4646 return this; 4647 } 4648 4649 /** 4650 * @return The value for the parameter that will be passed on to the external ruleset rule template. 4651 */ 4652 public String getValue() { 4653 return this.value == null ? null : this.value.getValue(); 4654 } 4655 4656 /** 4657 * @param value The value for the parameter that will be passed on to the external ruleset rule template. 4658 */ 4659 public RulesetRuleParamComponent setValue(String value) { 4660 if (Utilities.noString(value)) 4661 this.value = null; 4662 else { 4663 if (this.value == null) 4664 this.value = new StringType(); 4665 this.value.setValue(value); 4666 } 4667 return this; 4668 } 4669 4670 protected void listChildren(List<Property> children) { 4671 super.listChildren(children); 4672 children.add(new Property("name", "string", "Descriptive name for this parameter that matches the external assert ruleset rule parameter name.", 0, 1, name)); 4673 children.add(new Property("value", "string", "The value for the parameter that will be passed on to the external ruleset rule template.", 0, 1, value)); 4674 } 4675 4676 @Override 4677 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4678 switch (_hash) { 4679 case 3373707: /*name*/ return new Property("name", "string", "Descriptive name for this parameter that matches the external assert ruleset rule parameter name.", 0, 1, name); 4680 case 111972721: /*value*/ return new Property("value", "string", "The value for the parameter that will be passed on to the external ruleset rule template.", 0, 1, value); 4681 default: return super.getNamedProperty(_hash, _name, _checkValid); 4682 } 4683 4684 } 4685 4686 @Override 4687 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4688 switch (hash) { 4689 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4690 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 4691 default: return super.getProperty(hash, name, checkValid); 4692 } 4693 4694 } 4695 4696 @Override 4697 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4698 switch (hash) { 4699 case 3373707: // name 4700 this.name = castToString(value); // StringType 4701 return value; 4702 case 111972721: // value 4703 this.value = castToString(value); // StringType 4704 return value; 4705 default: return super.setProperty(hash, name, value); 4706 } 4707 4708 } 4709 4710 @Override 4711 public Base setProperty(String name, Base value) throws FHIRException { 4712 if (name.equals("name")) { 4713 this.name = castToString(value); // StringType 4714 } else if (name.equals("value")) { 4715 this.value = castToString(value); // StringType 4716 } else 4717 return super.setProperty(name, value); 4718 return value; 4719 } 4720 4721 @Override 4722 public Base makeProperty(int hash, String name) throws FHIRException { 4723 switch (hash) { 4724 case 3373707: return getNameElement(); 4725 case 111972721: return getValueElement(); 4726 default: return super.makeProperty(hash, name); 4727 } 4728 4729 } 4730 4731 @Override 4732 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4733 switch (hash) { 4734 case 3373707: /*name*/ return new String[] {"string"}; 4735 case 111972721: /*value*/ return new String[] {"string"}; 4736 default: return super.getTypesForProperty(hash, name); 4737 } 4738 4739 } 4740 4741 @Override 4742 public Base addChild(String name) throws FHIRException { 4743 if (name.equals("name")) { 4744 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 4745 } 4746 else if (name.equals("value")) { 4747 throw new FHIRException("Cannot call addChild on a singleton property TestScript.value"); 4748 } 4749 else 4750 return super.addChild(name); 4751 } 4752 4753 public RulesetRuleParamComponent copy() { 4754 RulesetRuleParamComponent dst = new RulesetRuleParamComponent(); 4755 copyValues(dst); 4756 dst.name = name == null ? null : name.copy(); 4757 dst.value = value == null ? null : value.copy(); 4758 return dst; 4759 } 4760 4761 @Override 4762 public boolean equalsDeep(Base other_) { 4763 if (!super.equalsDeep(other_)) 4764 return false; 4765 if (!(other_ instanceof RulesetRuleParamComponent)) 4766 return false; 4767 RulesetRuleParamComponent o = (RulesetRuleParamComponent) other_; 4768 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 4769 } 4770 4771 @Override 4772 public boolean equalsShallow(Base other_) { 4773 if (!super.equalsShallow(other_)) 4774 return false; 4775 if (!(other_ instanceof RulesetRuleParamComponent)) 4776 return false; 4777 RulesetRuleParamComponent o = (RulesetRuleParamComponent) other_; 4778 return compareValues(name, o.name, true) && compareValues(value, o.value, true); 4779 } 4780 4781 public boolean isEmpty() { 4782 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 4783 } 4784 4785 public String fhirType() { 4786 return "TestScript.ruleset.rule.param"; 4787 4788 } 4789 4790 } 4791 4792 @Block() 4793 public static class TestScriptSetupComponent extends BackboneElement implements IBaseBackboneElement { 4794 /** 4795 * Action would contain either an operation or an assertion. 4796 */ 4797 @Child(name = "action", type = {}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4798 @Description(shortDefinition="A setup operation or assert to perform", formalDefinition="Action would contain either an operation or an assertion." ) 4799 protected List<SetupActionComponent> action; 4800 4801 private static final long serialVersionUID = -123374486L; 4802 4803 /** 4804 * Constructor 4805 */ 4806 public TestScriptSetupComponent() { 4807 super(); 4808 } 4809 4810 /** 4811 * @return {@link #action} (Action would contain either an operation or an assertion.) 4812 */ 4813 public List<SetupActionComponent> getAction() { 4814 if (this.action == null) 4815 this.action = new ArrayList<SetupActionComponent>(); 4816 return this.action; 4817 } 4818 4819 /** 4820 * @return Returns a reference to <code>this</code> for easy method chaining 4821 */ 4822 public TestScriptSetupComponent setAction(List<SetupActionComponent> theAction) { 4823 this.action = theAction; 4824 return this; 4825 } 4826 4827 public boolean hasAction() { 4828 if (this.action == null) 4829 return false; 4830 for (SetupActionComponent item : this.action) 4831 if (!item.isEmpty()) 4832 return true; 4833 return false; 4834 } 4835 4836 public SetupActionComponent addAction() { //3 4837 SetupActionComponent t = new SetupActionComponent(); 4838 if (this.action == null) 4839 this.action = new ArrayList<SetupActionComponent>(); 4840 this.action.add(t); 4841 return t; 4842 } 4843 4844 public TestScriptSetupComponent addAction(SetupActionComponent t) { //3 4845 if (t == null) 4846 return this; 4847 if (this.action == null) 4848 this.action = new ArrayList<SetupActionComponent>(); 4849 this.action.add(t); 4850 return this; 4851 } 4852 4853 /** 4854 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 4855 */ 4856 public SetupActionComponent getActionFirstRep() { 4857 if (getAction().isEmpty()) { 4858 addAction(); 4859 } 4860 return getAction().get(0); 4861 } 4862 4863 protected void listChildren(List<Property> children) { 4864 super.listChildren(children); 4865 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action)); 4866 } 4867 4868 @Override 4869 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4870 switch (_hash) { 4871 case -1422950858: /*action*/ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action); 4872 default: return super.getNamedProperty(_hash, _name, _checkValid); 4873 } 4874 4875 } 4876 4877 @Override 4878 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4879 switch (hash) { 4880 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // SetupActionComponent 4881 default: return super.getProperty(hash, name, checkValid); 4882 } 4883 4884 } 4885 4886 @Override 4887 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4888 switch (hash) { 4889 case -1422950858: // action 4890 this.getAction().add((SetupActionComponent) value); // SetupActionComponent 4891 return value; 4892 default: return super.setProperty(hash, name, value); 4893 } 4894 4895 } 4896 4897 @Override 4898 public Base setProperty(String name, Base value) throws FHIRException { 4899 if (name.equals("action")) { 4900 this.getAction().add((SetupActionComponent) value); 4901 } else 4902 return super.setProperty(name, value); 4903 return value; 4904 } 4905 4906 @Override 4907 public Base makeProperty(int hash, String name) throws FHIRException { 4908 switch (hash) { 4909 case -1422950858: return addAction(); 4910 default: return super.makeProperty(hash, name); 4911 } 4912 4913 } 4914 4915 @Override 4916 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4917 switch (hash) { 4918 case -1422950858: /*action*/ return new String[] {}; 4919 default: return super.getTypesForProperty(hash, name); 4920 } 4921 4922 } 4923 4924 @Override 4925 public Base addChild(String name) throws FHIRException { 4926 if (name.equals("action")) { 4927 return addAction(); 4928 } 4929 else 4930 return super.addChild(name); 4931 } 4932 4933 public TestScriptSetupComponent copy() { 4934 TestScriptSetupComponent dst = new TestScriptSetupComponent(); 4935 copyValues(dst); 4936 if (action != null) { 4937 dst.action = new ArrayList<SetupActionComponent>(); 4938 for (SetupActionComponent i : action) 4939 dst.action.add(i.copy()); 4940 }; 4941 return dst; 4942 } 4943 4944 @Override 4945 public boolean equalsDeep(Base other_) { 4946 if (!super.equalsDeep(other_)) 4947 return false; 4948 if (!(other_ instanceof TestScriptSetupComponent)) 4949 return false; 4950 TestScriptSetupComponent o = (TestScriptSetupComponent) other_; 4951 return compareDeep(action, o.action, true); 4952 } 4953 4954 @Override 4955 public boolean equalsShallow(Base other_) { 4956 if (!super.equalsShallow(other_)) 4957 return false; 4958 if (!(other_ instanceof TestScriptSetupComponent)) 4959 return false; 4960 TestScriptSetupComponent o = (TestScriptSetupComponent) other_; 4961 return true; 4962 } 4963 4964 public boolean isEmpty() { 4965 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 4966 } 4967 4968 public String fhirType() { 4969 return "TestScript.setup"; 4970 4971 } 4972 4973 } 4974 4975 @Block() 4976 public static class SetupActionComponent extends BackboneElement implements IBaseBackboneElement { 4977 /** 4978 * The operation to perform. 4979 */ 4980 @Child(name = "operation", type = {}, order=1, min=0, max=1, modifier=false, summary=false) 4981 @Description(shortDefinition="The setup operation to perform", formalDefinition="The operation to perform." ) 4982 protected SetupActionOperationComponent operation; 4983 4984 /** 4985 * Evaluates the results of previous operations to determine if the server under test behaves appropriately. 4986 */ 4987 @Child(name = "assert", type = {}, order=2, min=0, max=1, modifier=false, summary=false) 4988 @Description(shortDefinition="The assertion to perform", formalDefinition="Evaluates the results of previous operations to determine if the server under test behaves appropriately." ) 4989 protected SetupActionAssertComponent assert_; 4990 4991 private static final long serialVersionUID = -252088305L; 4992 4993 /** 4994 * Constructor 4995 */ 4996 public SetupActionComponent() { 4997 super(); 4998 } 4999 5000 /** 5001 * @return {@link #operation} (The operation to perform.) 5002 */ 5003 public SetupActionOperationComponent getOperation() { 5004 if (this.operation == null) 5005 if (Configuration.errorOnAutoCreate()) 5006 throw new Error("Attempt to auto-create SetupActionComponent.operation"); 5007 else if (Configuration.doAutoCreate()) 5008 this.operation = new SetupActionOperationComponent(); // cc 5009 return this.operation; 5010 } 5011 5012 public boolean hasOperation() { 5013 return this.operation != null && !this.operation.isEmpty(); 5014 } 5015 5016 /** 5017 * @param value {@link #operation} (The operation to perform.) 5018 */ 5019 public SetupActionComponent setOperation(SetupActionOperationComponent value) { 5020 this.operation = value; 5021 return this; 5022 } 5023 5024 /** 5025 * @return {@link #assert_} (Evaluates the results of previous operations to determine if the server under test behaves appropriately.) 5026 */ 5027 public SetupActionAssertComponent getAssert() { 5028 if (this.assert_ == null) 5029 if (Configuration.errorOnAutoCreate()) 5030 throw new Error("Attempt to auto-create SetupActionComponent.assert_"); 5031 else if (Configuration.doAutoCreate()) 5032 this.assert_ = new SetupActionAssertComponent(); // cc 5033 return this.assert_; 5034 } 5035 5036 public boolean hasAssert() { 5037 return this.assert_ != null && !this.assert_.isEmpty(); 5038 } 5039 5040 /** 5041 * @param value {@link #assert_} (Evaluates the results of previous operations to determine if the server under test behaves appropriately.) 5042 */ 5043 public SetupActionComponent setAssert(SetupActionAssertComponent value) { 5044 this.assert_ = value; 5045 return this; 5046 } 5047 5048 protected void listChildren(List<Property> children) { 5049 super.listChildren(children); 5050 children.add(new Property("operation", "", "The operation to perform.", 0, 1, operation)); 5051 children.add(new Property("assert", "", "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 0, 1, assert_)); 5052 } 5053 5054 @Override 5055 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5056 switch (_hash) { 5057 case 1662702951: /*operation*/ return new Property("operation", "", "The operation to perform.", 0, 1, operation); 5058 case -1408208058: /*assert*/ return new Property("assert", "", "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 0, 1, assert_); 5059 default: return super.getNamedProperty(_hash, _name, _checkValid); 5060 } 5061 5062 } 5063 5064 @Override 5065 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5066 switch (hash) { 5067 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 5068 case -1408208058: /*assert*/ return this.assert_ == null ? new Base[0] : new Base[] {this.assert_}; // SetupActionAssertComponent 5069 default: return super.getProperty(hash, name, checkValid); 5070 } 5071 5072 } 5073 5074 @Override 5075 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5076 switch (hash) { 5077 case 1662702951: // operation 5078 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 5079 return value; 5080 case -1408208058: // assert 5081 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 5082 return value; 5083 default: return super.setProperty(hash, name, value); 5084 } 5085 5086 } 5087 5088 @Override 5089 public Base setProperty(String name, Base value) throws FHIRException { 5090 if (name.equals("operation")) { 5091 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 5092 } else if (name.equals("assert")) { 5093 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 5094 } else 5095 return super.setProperty(name, value); 5096 return value; 5097 } 5098 5099 @Override 5100 public Base makeProperty(int hash, String name) throws FHIRException { 5101 switch (hash) { 5102 case 1662702951: return getOperation(); 5103 case -1408208058: return getAssert(); 5104 default: return super.makeProperty(hash, name); 5105 } 5106 5107 } 5108 5109 @Override 5110 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5111 switch (hash) { 5112 case 1662702951: /*operation*/ return new String[] {}; 5113 case -1408208058: /*assert*/ return new String[] {}; 5114 default: return super.getTypesForProperty(hash, name); 5115 } 5116 5117 } 5118 5119 @Override 5120 public Base addChild(String name) throws FHIRException { 5121 if (name.equals("operation")) { 5122 this.operation = new SetupActionOperationComponent(); 5123 return this.operation; 5124 } 5125 else if (name.equals("assert")) { 5126 this.assert_ = new SetupActionAssertComponent(); 5127 return this.assert_; 5128 } 5129 else 5130 return super.addChild(name); 5131 } 5132 5133 public SetupActionComponent copy() { 5134 SetupActionComponent dst = new SetupActionComponent(); 5135 copyValues(dst); 5136 dst.operation = operation == null ? null : operation.copy(); 5137 dst.assert_ = assert_ == null ? null : assert_.copy(); 5138 return dst; 5139 } 5140 5141 @Override 5142 public boolean equalsDeep(Base other_) { 5143 if (!super.equalsDeep(other_)) 5144 return false; 5145 if (!(other_ instanceof SetupActionComponent)) 5146 return false; 5147 SetupActionComponent o = (SetupActionComponent) other_; 5148 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 5149 } 5150 5151 @Override 5152 public boolean equalsShallow(Base other_) { 5153 if (!super.equalsShallow(other_)) 5154 return false; 5155 if (!(other_ instanceof SetupActionComponent)) 5156 return false; 5157 SetupActionComponent o = (SetupActionComponent) other_; 5158 return true; 5159 } 5160 5161 public boolean isEmpty() { 5162 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 5163 } 5164 5165 public String fhirType() { 5166 return "TestScript.setup.action"; 5167 5168 } 5169 5170 } 5171 5172 @Block() 5173 public static class SetupActionOperationComponent extends BackboneElement implements IBaseBackboneElement { 5174 /** 5175 * Server interaction or operation type. 5176 */ 5177 @Child(name = "type", type = {Coding.class}, order=1, min=0, max=1, modifier=false, summary=false) 5178 @Description(shortDefinition="The operation code type that will be executed", formalDefinition="Server interaction or operation type." ) 5179 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/testscript-operation-codes") 5180 protected Coding type; 5181 5182 /** 5183 * The type of the resource. See http://build.fhir.org/resourcelist.html. 5184 */ 5185 @Child(name = "resource", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 5186 @Description(shortDefinition="Resource type", formalDefinition="The type of the resource. See http://build.fhir.org/resourcelist.html." ) 5187 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/defined-types") 5188 protected CodeType resource; 5189 5190 /** 5191 * The label would be used for tracking/logging purposes by test engines. 5192 */ 5193 @Child(name = "label", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5194 @Description(shortDefinition="Tracking/logging operation label", formalDefinition="The label would be used for tracking/logging purposes by test engines." ) 5195 protected StringType label; 5196 5197 /** 5198 * The description would be used by test engines for tracking and reporting purposes. 5199 */ 5200 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 5201 @Description(shortDefinition="Tracking/reporting operation description", formalDefinition="The description would be used by test engines for tracking and reporting purposes." ) 5202 protected StringType description; 5203 5204 /** 5205 * The content-type or mime-type to use for RESTful operation in the 'Accept' header. 5206 */ 5207 @Child(name = "accept", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 5208 @Description(shortDefinition="xml | json | ttl | none", formalDefinition="The content-type or mime-type to use for RESTful operation in the 'Accept' header." ) 5209 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/content-type") 5210 protected Enumeration<ContentType> accept; 5211 5212 /** 5213 * The content-type or mime-type to use for RESTful operation in the 'Content-Type' header. 5214 */ 5215 @Child(name = "contentType", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 5216 @Description(shortDefinition="xml | json | ttl | none", formalDefinition="The content-type or mime-type to use for RESTful operation in the 'Content-Type' header." ) 5217 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/content-type") 5218 protected Enumeration<ContentType> contentType; 5219 5220 /** 5221 * The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section. 5222 */ 5223 @Child(name = "destination", type = {IntegerType.class}, order=7, min=0, max=1, modifier=false, summary=false) 5224 @Description(shortDefinition="Server responding to the request", formalDefinition="The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section." ) 5225 protected IntegerType destination; 5226 5227 /** 5228 * Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths. 5229 */ 5230 @Child(name = "encodeRequestUrl", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=false) 5231 @Description(shortDefinition="Whether or not to send the request url in encoded format", formalDefinition="Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths." ) 5232 protected BooleanType encodeRequestUrl; 5233 5234 /** 5235 * The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section. 5236 */ 5237 @Child(name = "origin", type = {IntegerType.class}, order=9, min=0, max=1, modifier=false, summary=false) 5238 @Description(shortDefinition="Server initiating the request", formalDefinition="The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section." ) 5239 protected IntegerType origin; 5240 5241 /** 5242 * Path plus parameters after [type]. Used to set parts of the request URL explicitly. 5243 */ 5244 @Child(name = "params", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 5245 @Description(shortDefinition="Explicitly defined path parameters", formalDefinition="Path plus parameters after [type]. Used to set parts of the request URL explicitly." ) 5246 protected StringType params; 5247 5248 /** 5249 * Header elements would be used to set HTTP headers. 5250 */ 5251 @Child(name = "requestHeader", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5252 @Description(shortDefinition="Each operation can have one or more header elements", formalDefinition="Header elements would be used to set HTTP headers." ) 5253 protected List<SetupActionOperationRequestHeaderComponent> requestHeader; 5254 5255 /** 5256 * The fixture id (maybe new) to map to the request. 5257 */ 5258 @Child(name = "requestId", type = {IdType.class}, order=12, min=0, max=1, modifier=false, summary=false) 5259 @Description(shortDefinition="Fixture Id of mapped request", formalDefinition="The fixture id (maybe new) to map to the request." ) 5260 protected IdType requestId; 5261 5262 /** 5263 * The fixture id (maybe new) to map to the response. 5264 */ 5265 @Child(name = "responseId", type = {IdType.class}, order=13, min=0, max=1, modifier=false, summary=false) 5266 @Description(shortDefinition="Fixture Id of mapped response", formalDefinition="The fixture id (maybe new) to map to the response." ) 5267 protected IdType responseId; 5268 5269 /** 5270 * The id of the fixture used as the body of a PUT or POST request. 5271 */ 5272 @Child(name = "sourceId", type = {IdType.class}, order=14, min=0, max=1, modifier=false, summary=false) 5273 @Description(shortDefinition="Fixture Id of body for PUT and POST requests", formalDefinition="The id of the fixture used as the body of a PUT or POST request." ) 5274 protected IdType sourceId; 5275 5276 /** 5277 * Id of fixture used for extracting the [id], [type], and [vid] for GET requests. 5278 */ 5279 @Child(name = "targetId", type = {IdType.class}, order=15, min=0, max=1, modifier=false, summary=false) 5280 @Description(shortDefinition="Id of fixture used for extracting the [id], [type], and [vid] for GET requests", formalDefinition="Id of fixture used for extracting the [id], [type], and [vid] for GET requests." ) 5281 protected IdType targetId; 5282 5283 /** 5284 * Complete request URL. 5285 */ 5286 @Child(name = "url", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 5287 @Description(shortDefinition="Request URL", formalDefinition="Complete request URL." ) 5288 protected StringType url; 5289 5290 private static final long serialVersionUID = -488909648L; 5291 5292 /** 5293 * Constructor 5294 */ 5295 public SetupActionOperationComponent() { 5296 super(); 5297 } 5298 5299 /** 5300 * @return {@link #type} (Server interaction or operation type.) 5301 */ 5302 public Coding getType() { 5303 if (this.type == null) 5304 if (Configuration.errorOnAutoCreate()) 5305 throw new Error("Attempt to auto-create SetupActionOperationComponent.type"); 5306 else if (Configuration.doAutoCreate()) 5307 this.type = new Coding(); // cc 5308 return this.type; 5309 } 5310 5311 public boolean hasType() { 5312 return this.type != null && !this.type.isEmpty(); 5313 } 5314 5315 /** 5316 * @param value {@link #type} (Server interaction or operation type.) 5317 */ 5318 public SetupActionOperationComponent setType(Coding value) { 5319 this.type = value; 5320 return this; 5321 } 5322 5323 /** 5324 * @return {@link #resource} (The type of the resource. See http://build.fhir.org/resourcelist.html.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 5325 */ 5326 public CodeType getResourceElement() { 5327 if (this.resource == null) 5328 if (Configuration.errorOnAutoCreate()) 5329 throw new Error("Attempt to auto-create SetupActionOperationComponent.resource"); 5330 else if (Configuration.doAutoCreate()) 5331 this.resource = new CodeType(); // bb 5332 return this.resource; 5333 } 5334 5335 public boolean hasResourceElement() { 5336 return this.resource != null && !this.resource.isEmpty(); 5337 } 5338 5339 public boolean hasResource() { 5340 return this.resource != null && !this.resource.isEmpty(); 5341 } 5342 5343 /** 5344 * @param value {@link #resource} (The type of the resource. See http://build.fhir.org/resourcelist.html.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 5345 */ 5346 public SetupActionOperationComponent setResourceElement(CodeType value) { 5347 this.resource = value; 5348 return this; 5349 } 5350 5351 /** 5352 * @return The type of the resource. See http://build.fhir.org/resourcelist.html. 5353 */ 5354 public String getResource() { 5355 return this.resource == null ? null : this.resource.getValue(); 5356 } 5357 5358 /** 5359 * @param value The type of the resource. See http://build.fhir.org/resourcelist.html. 5360 */ 5361 public SetupActionOperationComponent setResource(String value) { 5362 if (Utilities.noString(value)) 5363 this.resource = null; 5364 else { 5365 if (this.resource == null) 5366 this.resource = new CodeType(); 5367 this.resource.setValue(value); 5368 } 5369 return this; 5370 } 5371 5372 /** 5373 * @return {@link #label} (The label would be used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 5374 */ 5375 public StringType getLabelElement() { 5376 if (this.label == null) 5377 if (Configuration.errorOnAutoCreate()) 5378 throw new Error("Attempt to auto-create SetupActionOperationComponent.label"); 5379 else if (Configuration.doAutoCreate()) 5380 this.label = new StringType(); // bb 5381 return this.label; 5382 } 5383 5384 public boolean hasLabelElement() { 5385 return this.label != null && !this.label.isEmpty(); 5386 } 5387 5388 public boolean hasLabel() { 5389 return this.label != null && !this.label.isEmpty(); 5390 } 5391 5392 /** 5393 * @param value {@link #label} (The label would be used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 5394 */ 5395 public SetupActionOperationComponent setLabelElement(StringType value) { 5396 this.label = value; 5397 return this; 5398 } 5399 5400 /** 5401 * @return The label would be used for tracking/logging purposes by test engines. 5402 */ 5403 public String getLabel() { 5404 return this.label == null ? null : this.label.getValue(); 5405 } 5406 5407 /** 5408 * @param value The label would be used for tracking/logging purposes by test engines. 5409 */ 5410 public SetupActionOperationComponent setLabel(String value) { 5411 if (Utilities.noString(value)) 5412 this.label = null; 5413 else { 5414 if (this.label == null) 5415 this.label = new StringType(); 5416 this.label.setValue(value); 5417 } 5418 return this; 5419 } 5420 5421 /** 5422 * @return {@link #description} (The description would be used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5423 */ 5424 public StringType getDescriptionElement() { 5425 if (this.description == null) 5426 if (Configuration.errorOnAutoCreate()) 5427 throw new Error("Attempt to auto-create SetupActionOperationComponent.description"); 5428 else if (Configuration.doAutoCreate()) 5429 this.description = new StringType(); // bb 5430 return this.description; 5431 } 5432 5433 public boolean hasDescriptionElement() { 5434 return this.description != null && !this.description.isEmpty(); 5435 } 5436 5437 public boolean hasDescription() { 5438 return this.description != null && !this.description.isEmpty(); 5439 } 5440 5441 /** 5442 * @param value {@link #description} (The description would be used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 5443 */ 5444 public SetupActionOperationComponent setDescriptionElement(StringType value) { 5445 this.description = value; 5446 return this; 5447 } 5448 5449 /** 5450 * @return The description would be used by test engines for tracking and reporting purposes. 5451 */ 5452 public String getDescription() { 5453 return this.description == null ? null : this.description.getValue(); 5454 } 5455 5456 /** 5457 * @param value The description would be used by test engines for tracking and reporting purposes. 5458 */ 5459 public SetupActionOperationComponent setDescription(String value) { 5460 if (Utilities.noString(value)) 5461 this.description = null; 5462 else { 5463 if (this.description == null) 5464 this.description = new StringType(); 5465 this.description.setValue(value); 5466 } 5467 return this; 5468 } 5469 5470 /** 5471 * @return {@link #accept} (The content-type or mime-type to use for RESTful operation in the 'Accept' header.). This is the underlying object with id, value and extensions. The accessor "getAccept" gives direct access to the value 5472 */ 5473 public Enumeration<ContentType> getAcceptElement() { 5474 if (this.accept == null) 5475 if (Configuration.errorOnAutoCreate()) 5476 throw new Error("Attempt to auto-create SetupActionOperationComponent.accept"); 5477 else if (Configuration.doAutoCreate()) 5478 this.accept = new Enumeration<ContentType>(new ContentTypeEnumFactory()); // bb 5479 return this.accept; 5480 } 5481 5482 public boolean hasAcceptElement() { 5483 return this.accept != null && !this.accept.isEmpty(); 5484 } 5485 5486 public boolean hasAccept() { 5487 return this.accept != null && !this.accept.isEmpty(); 5488 } 5489 5490 /** 5491 * @param value {@link #accept} (The content-type or mime-type to use for RESTful operation in the 'Accept' header.). This is the underlying object with id, value and extensions. The accessor "getAccept" gives direct access to the value 5492 */ 5493 public SetupActionOperationComponent setAcceptElement(Enumeration<ContentType> value) { 5494 this.accept = value; 5495 return this; 5496 } 5497 5498 /** 5499 * @return The content-type or mime-type to use for RESTful operation in the 'Accept' header. 5500 */ 5501 public ContentType getAccept() { 5502 return this.accept == null ? null : this.accept.getValue(); 5503 } 5504 5505 /** 5506 * @param value The content-type or mime-type to use for RESTful operation in the 'Accept' header. 5507 */ 5508 public SetupActionOperationComponent setAccept(ContentType value) { 5509 if (value == null) 5510 this.accept = null; 5511 else { 5512 if (this.accept == null) 5513 this.accept = new Enumeration<ContentType>(new ContentTypeEnumFactory()); 5514 this.accept.setValue(value); 5515 } 5516 return this; 5517 } 5518 5519 /** 5520 * @return {@link #contentType} (The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 5521 */ 5522 public Enumeration<ContentType> getContentTypeElement() { 5523 if (this.contentType == null) 5524 if (Configuration.errorOnAutoCreate()) 5525 throw new Error("Attempt to auto-create SetupActionOperationComponent.contentType"); 5526 else if (Configuration.doAutoCreate()) 5527 this.contentType = new Enumeration<ContentType>(new ContentTypeEnumFactory()); // bb 5528 return this.contentType; 5529 } 5530 5531 public boolean hasContentTypeElement() { 5532 return this.contentType != null && !this.contentType.isEmpty(); 5533 } 5534 5535 public boolean hasContentType() { 5536 return this.contentType != null && !this.contentType.isEmpty(); 5537 } 5538 5539 /** 5540 * @param value {@link #contentType} (The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 5541 */ 5542 public SetupActionOperationComponent setContentTypeElement(Enumeration<ContentType> value) { 5543 this.contentType = value; 5544 return this; 5545 } 5546 5547 /** 5548 * @return The content-type or mime-type to use for RESTful operation in the 'Content-Type' header. 5549 */ 5550 public ContentType getContentType() { 5551 return this.contentType == null ? null : this.contentType.getValue(); 5552 } 5553 5554 /** 5555 * @param value The content-type or mime-type to use for RESTful operation in the 'Content-Type' header. 5556 */ 5557 public SetupActionOperationComponent setContentType(ContentType value) { 5558 if (value == null) 5559 this.contentType = null; 5560 else { 5561 if (this.contentType == null) 5562 this.contentType = new Enumeration<ContentType>(new ContentTypeEnumFactory()); 5563 this.contentType.setValue(value); 5564 } 5565 return this; 5566 } 5567 5568 /** 5569 * @return {@link #destination} (The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.). This is the underlying object with id, value and extensions. The accessor "getDestination" gives direct access to the value 5570 */ 5571 public IntegerType getDestinationElement() { 5572 if (this.destination == null) 5573 if (Configuration.errorOnAutoCreate()) 5574 throw new Error("Attempt to auto-create SetupActionOperationComponent.destination"); 5575 else if (Configuration.doAutoCreate()) 5576 this.destination = new IntegerType(); // bb 5577 return this.destination; 5578 } 5579 5580 public boolean hasDestinationElement() { 5581 return this.destination != null && !this.destination.isEmpty(); 5582 } 5583 5584 public boolean hasDestination() { 5585 return this.destination != null && !this.destination.isEmpty(); 5586 } 5587 5588 /** 5589 * @param value {@link #destination} (The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.). This is the underlying object with id, value and extensions. The accessor "getDestination" gives direct access to the value 5590 */ 5591 public SetupActionOperationComponent setDestinationElement(IntegerType value) { 5592 this.destination = value; 5593 return this; 5594 } 5595 5596 /** 5597 * @return The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section. 5598 */ 5599 public int getDestination() { 5600 return this.destination == null || this.destination.isEmpty() ? 0 : this.destination.getValue(); 5601 } 5602 5603 /** 5604 * @param value The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section. 5605 */ 5606 public SetupActionOperationComponent setDestination(int value) { 5607 if (this.destination == null) 5608 this.destination = new IntegerType(); 5609 this.destination.setValue(value); 5610 return this; 5611 } 5612 5613 /** 5614 * @return {@link #encodeRequestUrl} (Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.). This is the underlying object with id, value and extensions. The accessor "getEncodeRequestUrl" gives direct access to the value 5615 */ 5616 public BooleanType getEncodeRequestUrlElement() { 5617 if (this.encodeRequestUrl == null) 5618 if (Configuration.errorOnAutoCreate()) 5619 throw new Error("Attempt to auto-create SetupActionOperationComponent.encodeRequestUrl"); 5620 else if (Configuration.doAutoCreate()) 5621 this.encodeRequestUrl = new BooleanType(); // bb 5622 return this.encodeRequestUrl; 5623 } 5624 5625 public boolean hasEncodeRequestUrlElement() { 5626 return this.encodeRequestUrl != null && !this.encodeRequestUrl.isEmpty(); 5627 } 5628 5629 public boolean hasEncodeRequestUrl() { 5630 return this.encodeRequestUrl != null && !this.encodeRequestUrl.isEmpty(); 5631 } 5632 5633 /** 5634 * @param value {@link #encodeRequestUrl} (Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.). This is the underlying object with id, value and extensions. The accessor "getEncodeRequestUrl" gives direct access to the value 5635 */ 5636 public SetupActionOperationComponent setEncodeRequestUrlElement(BooleanType value) { 5637 this.encodeRequestUrl = value; 5638 return this; 5639 } 5640 5641 /** 5642 * @return Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths. 5643 */ 5644 public boolean getEncodeRequestUrl() { 5645 return this.encodeRequestUrl == null || this.encodeRequestUrl.isEmpty() ? false : this.encodeRequestUrl.getValue(); 5646 } 5647 5648 /** 5649 * @param value Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths. 5650 */ 5651 public SetupActionOperationComponent setEncodeRequestUrl(boolean value) { 5652 if (this.encodeRequestUrl == null) 5653 this.encodeRequestUrl = new BooleanType(); 5654 this.encodeRequestUrl.setValue(value); 5655 return this; 5656 } 5657 5658 /** 5659 * @return {@link #origin} (The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.). This is the underlying object with id, value and extensions. The accessor "getOrigin" gives direct access to the value 5660 */ 5661 public IntegerType getOriginElement() { 5662 if (this.origin == null) 5663 if (Configuration.errorOnAutoCreate()) 5664 throw new Error("Attempt to auto-create SetupActionOperationComponent.origin"); 5665 else if (Configuration.doAutoCreate()) 5666 this.origin = new IntegerType(); // bb 5667 return this.origin; 5668 } 5669 5670 public boolean hasOriginElement() { 5671 return this.origin != null && !this.origin.isEmpty(); 5672 } 5673 5674 public boolean hasOrigin() { 5675 return this.origin != null && !this.origin.isEmpty(); 5676 } 5677 5678 /** 5679 * @param value {@link #origin} (The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.). This is the underlying object with id, value and extensions. The accessor "getOrigin" gives direct access to the value 5680 */ 5681 public SetupActionOperationComponent setOriginElement(IntegerType value) { 5682 this.origin = value; 5683 return this; 5684 } 5685 5686 /** 5687 * @return The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section. 5688 */ 5689 public int getOrigin() { 5690 return this.origin == null || this.origin.isEmpty() ? 0 : this.origin.getValue(); 5691 } 5692 5693 /** 5694 * @param value The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section. 5695 */ 5696 public SetupActionOperationComponent setOrigin(int value) { 5697 if (this.origin == null) 5698 this.origin = new IntegerType(); 5699 this.origin.setValue(value); 5700 return this; 5701 } 5702 5703 /** 5704 * @return {@link #params} (Path plus parameters after [type]. Used to set parts of the request URL explicitly.). This is the underlying object with id, value and extensions. The accessor "getParams" gives direct access to the value 5705 */ 5706 public StringType getParamsElement() { 5707 if (this.params == null) 5708 if (Configuration.errorOnAutoCreate()) 5709 throw new Error("Attempt to auto-create SetupActionOperationComponent.params"); 5710 else if (Configuration.doAutoCreate()) 5711 this.params = new StringType(); // bb 5712 return this.params; 5713 } 5714 5715 public boolean hasParamsElement() { 5716 return this.params != null && !this.params.isEmpty(); 5717 } 5718 5719 public boolean hasParams() { 5720 return this.params != null && !this.params.isEmpty(); 5721 } 5722 5723 /** 5724 * @param value {@link #params} (Path plus parameters after [type]. Used to set parts of the request URL explicitly.). This is the underlying object with id, value and extensions. The accessor "getParams" gives direct access to the value 5725 */ 5726 public SetupActionOperationComponent setParamsElement(StringType value) { 5727 this.params = value; 5728 return this; 5729 } 5730 5731 /** 5732 * @return Path plus parameters after [type]. Used to set parts of the request URL explicitly. 5733 */ 5734 public String getParams() { 5735 return this.params == null ? null : this.params.getValue(); 5736 } 5737 5738 /** 5739 * @param value Path plus parameters after [type]. Used to set parts of the request URL explicitly. 5740 */ 5741 public SetupActionOperationComponent setParams(String value) { 5742 if (Utilities.noString(value)) 5743 this.params = null; 5744 else { 5745 if (this.params == null) 5746 this.params = new StringType(); 5747 this.params.setValue(value); 5748 } 5749 return this; 5750 } 5751 5752 /** 5753 * @return {@link #requestHeader} (Header elements would be used to set HTTP headers.) 5754 */ 5755 public List<SetupActionOperationRequestHeaderComponent> getRequestHeader() { 5756 if (this.requestHeader == null) 5757 this.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 5758 return this.requestHeader; 5759 } 5760 5761 /** 5762 * @return Returns a reference to <code>this</code> for easy method chaining 5763 */ 5764 public SetupActionOperationComponent setRequestHeader(List<SetupActionOperationRequestHeaderComponent> theRequestHeader) { 5765 this.requestHeader = theRequestHeader; 5766 return this; 5767 } 5768 5769 public boolean hasRequestHeader() { 5770 if (this.requestHeader == null) 5771 return false; 5772 for (SetupActionOperationRequestHeaderComponent item : this.requestHeader) 5773 if (!item.isEmpty()) 5774 return true; 5775 return false; 5776 } 5777 5778 public SetupActionOperationRequestHeaderComponent addRequestHeader() { //3 5779 SetupActionOperationRequestHeaderComponent t = new SetupActionOperationRequestHeaderComponent(); 5780 if (this.requestHeader == null) 5781 this.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 5782 this.requestHeader.add(t); 5783 return t; 5784 } 5785 5786 public SetupActionOperationComponent addRequestHeader(SetupActionOperationRequestHeaderComponent t) { //3 5787 if (t == null) 5788 return this; 5789 if (this.requestHeader == null) 5790 this.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 5791 this.requestHeader.add(t); 5792 return this; 5793 } 5794 5795 /** 5796 * @return The first repetition of repeating field {@link #requestHeader}, creating it if it does not already exist 5797 */ 5798 public SetupActionOperationRequestHeaderComponent getRequestHeaderFirstRep() { 5799 if (getRequestHeader().isEmpty()) { 5800 addRequestHeader(); 5801 } 5802 return getRequestHeader().get(0); 5803 } 5804 5805 /** 5806 * @return {@link #requestId} (The fixture id (maybe new) to map to the request.). This is the underlying object with id, value and extensions. The accessor "getRequestId" gives direct access to the value 5807 */ 5808 public IdType getRequestIdElement() { 5809 if (this.requestId == null) 5810 if (Configuration.errorOnAutoCreate()) 5811 throw new Error("Attempt to auto-create SetupActionOperationComponent.requestId"); 5812 else if (Configuration.doAutoCreate()) 5813 this.requestId = new IdType(); // bb 5814 return this.requestId; 5815 } 5816 5817 public boolean hasRequestIdElement() { 5818 return this.requestId != null && !this.requestId.isEmpty(); 5819 } 5820 5821 public boolean hasRequestId() { 5822 return this.requestId != null && !this.requestId.isEmpty(); 5823 } 5824 5825 /** 5826 * @param value {@link #requestId} (The fixture id (maybe new) to map to the request.). This is the underlying object with id, value and extensions. The accessor "getRequestId" gives direct access to the value 5827 */ 5828 public SetupActionOperationComponent setRequestIdElement(IdType value) { 5829 this.requestId = value; 5830 return this; 5831 } 5832 5833 /** 5834 * @return The fixture id (maybe new) to map to the request. 5835 */ 5836 public String getRequestId() { 5837 return this.requestId == null ? null : this.requestId.getValue(); 5838 } 5839 5840 /** 5841 * @param value The fixture id (maybe new) to map to the request. 5842 */ 5843 public SetupActionOperationComponent setRequestId(String value) { 5844 if (Utilities.noString(value)) 5845 this.requestId = null; 5846 else { 5847 if (this.requestId == null) 5848 this.requestId = new IdType(); 5849 this.requestId.setValue(value); 5850 } 5851 return this; 5852 } 5853 5854 /** 5855 * @return {@link #responseId} (The fixture id (maybe new) to map to the response.). This is the underlying object with id, value and extensions. The accessor "getResponseId" gives direct access to the value 5856 */ 5857 public IdType getResponseIdElement() { 5858 if (this.responseId == null) 5859 if (Configuration.errorOnAutoCreate()) 5860 throw new Error("Attempt to auto-create SetupActionOperationComponent.responseId"); 5861 else if (Configuration.doAutoCreate()) 5862 this.responseId = new IdType(); // bb 5863 return this.responseId; 5864 } 5865 5866 public boolean hasResponseIdElement() { 5867 return this.responseId != null && !this.responseId.isEmpty(); 5868 } 5869 5870 public boolean hasResponseId() { 5871 return this.responseId != null && !this.responseId.isEmpty(); 5872 } 5873 5874 /** 5875 * @param value {@link #responseId} (The fixture id (maybe new) to map to the response.). This is the underlying object with id, value and extensions. The accessor "getResponseId" gives direct access to the value 5876 */ 5877 public SetupActionOperationComponent setResponseIdElement(IdType value) { 5878 this.responseId = value; 5879 return this; 5880 } 5881 5882 /** 5883 * @return The fixture id (maybe new) to map to the response. 5884 */ 5885 public String getResponseId() { 5886 return this.responseId == null ? null : this.responseId.getValue(); 5887 } 5888 5889 /** 5890 * @param value The fixture id (maybe new) to map to the response. 5891 */ 5892 public SetupActionOperationComponent setResponseId(String value) { 5893 if (Utilities.noString(value)) 5894 this.responseId = null; 5895 else { 5896 if (this.responseId == null) 5897 this.responseId = new IdType(); 5898 this.responseId.setValue(value); 5899 } 5900 return this; 5901 } 5902 5903 /** 5904 * @return {@link #sourceId} (The id of the fixture used as the body of a PUT or POST request.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 5905 */ 5906 public IdType getSourceIdElement() { 5907 if (this.sourceId == null) 5908 if (Configuration.errorOnAutoCreate()) 5909 throw new Error("Attempt to auto-create SetupActionOperationComponent.sourceId"); 5910 else if (Configuration.doAutoCreate()) 5911 this.sourceId = new IdType(); // bb 5912 return this.sourceId; 5913 } 5914 5915 public boolean hasSourceIdElement() { 5916 return this.sourceId != null && !this.sourceId.isEmpty(); 5917 } 5918 5919 public boolean hasSourceId() { 5920 return this.sourceId != null && !this.sourceId.isEmpty(); 5921 } 5922 5923 /** 5924 * @param value {@link #sourceId} (The id of the fixture used as the body of a PUT or POST request.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 5925 */ 5926 public SetupActionOperationComponent setSourceIdElement(IdType value) { 5927 this.sourceId = value; 5928 return this; 5929 } 5930 5931 /** 5932 * @return The id of the fixture used as the body of a PUT or POST request. 5933 */ 5934 public String getSourceId() { 5935 return this.sourceId == null ? null : this.sourceId.getValue(); 5936 } 5937 5938 /** 5939 * @param value The id of the fixture used as the body of a PUT or POST request. 5940 */ 5941 public SetupActionOperationComponent setSourceId(String value) { 5942 if (Utilities.noString(value)) 5943 this.sourceId = null; 5944 else { 5945 if (this.sourceId == null) 5946 this.sourceId = new IdType(); 5947 this.sourceId.setValue(value); 5948 } 5949 return this; 5950 } 5951 5952 /** 5953 * @return {@link #targetId} (Id of fixture used for extracting the [id], [type], and [vid] for GET requests.). This is the underlying object with id, value and extensions. The accessor "getTargetId" gives direct access to the value 5954 */ 5955 public IdType getTargetIdElement() { 5956 if (this.targetId == null) 5957 if (Configuration.errorOnAutoCreate()) 5958 throw new Error("Attempt to auto-create SetupActionOperationComponent.targetId"); 5959 else if (Configuration.doAutoCreate()) 5960 this.targetId = new IdType(); // bb 5961 return this.targetId; 5962 } 5963 5964 public boolean hasTargetIdElement() { 5965 return this.targetId != null && !this.targetId.isEmpty(); 5966 } 5967 5968 public boolean hasTargetId() { 5969 return this.targetId != null && !this.targetId.isEmpty(); 5970 } 5971 5972 /** 5973 * @param value {@link #targetId} (Id of fixture used for extracting the [id], [type], and [vid] for GET requests.). This is the underlying object with id, value and extensions. The accessor "getTargetId" gives direct access to the value 5974 */ 5975 public SetupActionOperationComponent setTargetIdElement(IdType value) { 5976 this.targetId = value; 5977 return this; 5978 } 5979 5980 /** 5981 * @return Id of fixture used for extracting the [id], [type], and [vid] for GET requests. 5982 */ 5983 public String getTargetId() { 5984 return this.targetId == null ? null : this.targetId.getValue(); 5985 } 5986 5987 /** 5988 * @param value Id of fixture used for extracting the [id], [type], and [vid] for GET requests. 5989 */ 5990 public SetupActionOperationComponent setTargetId(String value) { 5991 if (Utilities.noString(value)) 5992 this.targetId = null; 5993 else { 5994 if (this.targetId == null) 5995 this.targetId = new IdType(); 5996 this.targetId.setValue(value); 5997 } 5998 return this; 5999 } 6000 6001 /** 6002 * @return {@link #url} (Complete request URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 6003 */ 6004 public StringType getUrlElement() { 6005 if (this.url == null) 6006 if (Configuration.errorOnAutoCreate()) 6007 throw new Error("Attempt to auto-create SetupActionOperationComponent.url"); 6008 else if (Configuration.doAutoCreate()) 6009 this.url = new StringType(); // bb 6010 return this.url; 6011 } 6012 6013 public boolean hasUrlElement() { 6014 return this.url != null && !this.url.isEmpty(); 6015 } 6016 6017 public boolean hasUrl() { 6018 return this.url != null && !this.url.isEmpty(); 6019 } 6020 6021 /** 6022 * @param value {@link #url} (Complete request URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 6023 */ 6024 public SetupActionOperationComponent setUrlElement(StringType value) { 6025 this.url = value; 6026 return this; 6027 } 6028 6029 /** 6030 * @return Complete request URL. 6031 */ 6032 public String getUrl() { 6033 return this.url == null ? null : this.url.getValue(); 6034 } 6035 6036 /** 6037 * @param value Complete request URL. 6038 */ 6039 public SetupActionOperationComponent setUrl(String value) { 6040 if (Utilities.noString(value)) 6041 this.url = null; 6042 else { 6043 if (this.url == null) 6044 this.url = new StringType(); 6045 this.url.setValue(value); 6046 } 6047 return this; 6048 } 6049 6050 protected void listChildren(List<Property> children) { 6051 super.listChildren(children); 6052 children.add(new Property("type", "Coding", "Server interaction or operation type.", 0, 1, type)); 6053 children.add(new Property("resource", "code", "The type of the resource. See http://build.fhir.org/resourcelist.html.", 0, 1, resource)); 6054 children.add(new Property("label", "string", "The label would be used for tracking/logging purposes by test engines.", 0, 1, label)); 6055 children.add(new Property("description", "string", "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description)); 6056 children.add(new Property("accept", "code", "The content-type or mime-type to use for RESTful operation in the 'Accept' header.", 0, 1, accept)); 6057 children.add(new Property("contentType", "code", "The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.", 0, 1, contentType)); 6058 children.add(new Property("destination", "integer", "The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.", 0, 1, destination)); 6059 children.add(new Property("encodeRequestUrl", "boolean", "Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.", 0, 1, encodeRequestUrl)); 6060 children.add(new Property("origin", "integer", "The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.", 0, 1, origin)); 6061 children.add(new Property("params", "string", "Path plus parameters after [type]. Used to set parts of the request URL explicitly.", 0, 1, params)); 6062 children.add(new Property("requestHeader", "", "Header elements would be used to set HTTP headers.", 0, java.lang.Integer.MAX_VALUE, requestHeader)); 6063 children.add(new Property("requestId", "id", "The fixture id (maybe new) to map to the request.", 0, 1, requestId)); 6064 children.add(new Property("responseId", "id", "The fixture id (maybe new) to map to the response.", 0, 1, responseId)); 6065 children.add(new Property("sourceId", "id", "The id of the fixture used as the body of a PUT or POST request.", 0, 1, sourceId)); 6066 children.add(new Property("targetId", "id", "Id of fixture used for extracting the [id], [type], and [vid] for GET requests.", 0, 1, targetId)); 6067 children.add(new Property("url", "string", "Complete request URL.", 0, 1, url)); 6068 } 6069 6070 @Override 6071 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6072 switch (_hash) { 6073 case 3575610: /*type*/ return new Property("type", "Coding", "Server interaction or operation type.", 0, 1, type); 6074 case -341064690: /*resource*/ return new Property("resource", "code", "The type of the resource. See http://build.fhir.org/resourcelist.html.", 0, 1, resource); 6075 case 102727412: /*label*/ return new Property("label", "string", "The label would be used for tracking/logging purposes by test engines.", 0, 1, label); 6076 case -1724546052: /*description*/ return new Property("description", "string", "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description); 6077 case -1423461112: /*accept*/ return new Property("accept", "code", "The content-type or mime-type to use for RESTful operation in the 'Accept' header.", 0, 1, accept); 6078 case -389131437: /*contentType*/ return new Property("contentType", "code", "The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.", 0, 1, contentType); 6079 case -1429847026: /*destination*/ return new Property("destination", "integer", "The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.", 0, 1, destination); 6080 case -1760554218: /*encodeRequestUrl*/ return new Property("encodeRequestUrl", "boolean", "Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.", 0, 1, encodeRequestUrl); 6081 case -1008619738: /*origin*/ return new Property("origin", "integer", "The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.", 0, 1, origin); 6082 case -995427962: /*params*/ return new Property("params", "string", "Path plus parameters after [type]. Used to set parts of the request URL explicitly.", 0, 1, params); 6083 case 1074158076: /*requestHeader*/ return new Property("requestHeader", "", "Header elements would be used to set HTTP headers.", 0, java.lang.Integer.MAX_VALUE, requestHeader); 6084 case 693933066: /*requestId*/ return new Property("requestId", "id", "The fixture id (maybe new) to map to the request.", 0, 1, requestId); 6085 case -633138884: /*responseId*/ return new Property("responseId", "id", "The fixture id (maybe new) to map to the response.", 0, 1, responseId); 6086 case 1746327190: /*sourceId*/ return new Property("sourceId", "id", "The id of the fixture used as the body of a PUT or POST request.", 0, 1, sourceId); 6087 case -441951604: /*targetId*/ return new Property("targetId", "id", "Id of fixture used for extracting the [id], [type], and [vid] for GET requests.", 0, 1, targetId); 6088 case 116079: /*url*/ return new Property("url", "string", "Complete request URL.", 0, 1, url); 6089 default: return super.getNamedProperty(_hash, _name, _checkValid); 6090 } 6091 6092 } 6093 6094 @Override 6095 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6096 switch (hash) { 6097 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 6098 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // CodeType 6099 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 6100 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 6101 case -1423461112: /*accept*/ return this.accept == null ? new Base[0] : new Base[] {this.accept}; // Enumeration<ContentType> 6102 case -389131437: /*contentType*/ return this.contentType == null ? new Base[0] : new Base[] {this.contentType}; // Enumeration<ContentType> 6103 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // IntegerType 6104 case -1760554218: /*encodeRequestUrl*/ return this.encodeRequestUrl == null ? new Base[0] : new Base[] {this.encodeRequestUrl}; // BooleanType 6105 case -1008619738: /*origin*/ return this.origin == null ? new Base[0] : new Base[] {this.origin}; // IntegerType 6106 case -995427962: /*params*/ return this.params == null ? new Base[0] : new Base[] {this.params}; // StringType 6107 case 1074158076: /*requestHeader*/ return this.requestHeader == null ? new Base[0] : this.requestHeader.toArray(new Base[this.requestHeader.size()]); // SetupActionOperationRequestHeaderComponent 6108 case 693933066: /*requestId*/ return this.requestId == null ? new Base[0] : new Base[] {this.requestId}; // IdType 6109 case -633138884: /*responseId*/ return this.responseId == null ? new Base[0] : new Base[] {this.responseId}; // IdType 6110 case 1746327190: /*sourceId*/ return this.sourceId == null ? new Base[0] : new Base[] {this.sourceId}; // IdType 6111 case -441951604: /*targetId*/ return this.targetId == null ? new Base[0] : new Base[] {this.targetId}; // IdType 6112 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // StringType 6113 default: return super.getProperty(hash, name, checkValid); 6114 } 6115 6116 } 6117 6118 @Override 6119 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6120 switch (hash) { 6121 case 3575610: // type 6122 this.type = castToCoding(value); // Coding 6123 return value; 6124 case -341064690: // resource 6125 this.resource = castToCode(value); // CodeType 6126 return value; 6127 case 102727412: // label 6128 this.label = castToString(value); // StringType 6129 return value; 6130 case -1724546052: // description 6131 this.description = castToString(value); // StringType 6132 return value; 6133 case -1423461112: // accept 6134 value = new ContentTypeEnumFactory().fromType(castToCode(value)); 6135 this.accept = (Enumeration) value; // Enumeration<ContentType> 6136 return value; 6137 case -389131437: // contentType 6138 value = new ContentTypeEnumFactory().fromType(castToCode(value)); 6139 this.contentType = (Enumeration) value; // Enumeration<ContentType> 6140 return value; 6141 case -1429847026: // destination 6142 this.destination = castToInteger(value); // IntegerType 6143 return value; 6144 case -1760554218: // encodeRequestUrl 6145 this.encodeRequestUrl = castToBoolean(value); // BooleanType 6146 return value; 6147 case -1008619738: // origin 6148 this.origin = castToInteger(value); // IntegerType 6149 return value; 6150 case -995427962: // params 6151 this.params = castToString(value); // StringType 6152 return value; 6153 case 1074158076: // requestHeader 6154 this.getRequestHeader().add((SetupActionOperationRequestHeaderComponent) value); // SetupActionOperationRequestHeaderComponent 6155 return value; 6156 case 693933066: // requestId 6157 this.requestId = castToId(value); // IdType 6158 return value; 6159 case -633138884: // responseId 6160 this.responseId = castToId(value); // IdType 6161 return value; 6162 case 1746327190: // sourceId 6163 this.sourceId = castToId(value); // IdType 6164 return value; 6165 case -441951604: // targetId 6166 this.targetId = castToId(value); // IdType 6167 return value; 6168 case 116079: // url 6169 this.url = castToString(value); // StringType 6170 return value; 6171 default: return super.setProperty(hash, name, value); 6172 } 6173 6174 } 6175 6176 @Override 6177 public Base setProperty(String name, Base value) throws FHIRException { 6178 if (name.equals("type")) { 6179 this.type = castToCoding(value); // Coding 6180 } else if (name.equals("resource")) { 6181 this.resource = castToCode(value); // CodeType 6182 } else if (name.equals("label")) { 6183 this.label = castToString(value); // StringType 6184 } else if (name.equals("description")) { 6185 this.description = castToString(value); // StringType 6186 } else if (name.equals("accept")) { 6187 value = new ContentTypeEnumFactory().fromType(castToCode(value)); 6188 this.accept = (Enumeration) value; // Enumeration<ContentType> 6189 } else if (name.equals("contentType")) { 6190 value = new ContentTypeEnumFactory().fromType(castToCode(value)); 6191 this.contentType = (Enumeration) value; // Enumeration<ContentType> 6192 } else if (name.equals("destination")) { 6193 this.destination = castToInteger(value); // IntegerType 6194 } else if (name.equals("encodeRequestUrl")) { 6195 this.encodeRequestUrl = castToBoolean(value); // BooleanType 6196 } else if (name.equals("origin")) { 6197 this.origin = castToInteger(value); // IntegerType 6198 } else if (name.equals("params")) { 6199 this.params = castToString(value); // StringType 6200 } else if (name.equals("requestHeader")) { 6201 this.getRequestHeader().add((SetupActionOperationRequestHeaderComponent) value); 6202 } else if (name.equals("requestId")) { 6203 this.requestId = castToId(value); // IdType 6204 } else if (name.equals("responseId")) { 6205 this.responseId = castToId(value); // IdType 6206 } else if (name.equals("sourceId")) { 6207 this.sourceId = castToId(value); // IdType 6208 } else if (name.equals("targetId")) { 6209 this.targetId = castToId(value); // IdType 6210 } else if (name.equals("url")) { 6211 this.url = castToString(value); // StringType 6212 } else 6213 return super.setProperty(name, value); 6214 return value; 6215 } 6216 6217 @Override 6218 public Base makeProperty(int hash, String name) throws FHIRException { 6219 switch (hash) { 6220 case 3575610: return getType(); 6221 case -341064690: return getResourceElement(); 6222 case 102727412: return getLabelElement(); 6223 case -1724546052: return getDescriptionElement(); 6224 case -1423461112: return getAcceptElement(); 6225 case -389131437: return getContentTypeElement(); 6226 case -1429847026: return getDestinationElement(); 6227 case -1760554218: return getEncodeRequestUrlElement(); 6228 case -1008619738: return getOriginElement(); 6229 case -995427962: return getParamsElement(); 6230 case 1074158076: return addRequestHeader(); 6231 case 693933066: return getRequestIdElement(); 6232 case -633138884: return getResponseIdElement(); 6233 case 1746327190: return getSourceIdElement(); 6234 case -441951604: return getTargetIdElement(); 6235 case 116079: return getUrlElement(); 6236 default: return super.makeProperty(hash, name); 6237 } 6238 6239 } 6240 6241 @Override 6242 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6243 switch (hash) { 6244 case 3575610: /*type*/ return new String[] {"Coding"}; 6245 case -341064690: /*resource*/ return new String[] {"code"}; 6246 case 102727412: /*label*/ return new String[] {"string"}; 6247 case -1724546052: /*description*/ return new String[] {"string"}; 6248 case -1423461112: /*accept*/ return new String[] {"code"}; 6249 case -389131437: /*contentType*/ return new String[] {"code"}; 6250 case -1429847026: /*destination*/ return new String[] {"integer"}; 6251 case -1760554218: /*encodeRequestUrl*/ return new String[] {"boolean"}; 6252 case -1008619738: /*origin*/ return new String[] {"integer"}; 6253 case -995427962: /*params*/ return new String[] {"string"}; 6254 case 1074158076: /*requestHeader*/ return new String[] {}; 6255 case 693933066: /*requestId*/ return new String[] {"id"}; 6256 case -633138884: /*responseId*/ return new String[] {"id"}; 6257 case 1746327190: /*sourceId*/ return new String[] {"id"}; 6258 case -441951604: /*targetId*/ return new String[] {"id"}; 6259 case 116079: /*url*/ return new String[] {"string"}; 6260 default: return super.getTypesForProperty(hash, name); 6261 } 6262 6263 } 6264 6265 @Override 6266 public Base addChild(String name) throws FHIRException { 6267 if (name.equals("type")) { 6268 this.type = new Coding(); 6269 return this.type; 6270 } 6271 else if (name.equals("resource")) { 6272 throw new FHIRException("Cannot call addChild on a singleton property TestScript.resource"); 6273 } 6274 else if (name.equals("label")) { 6275 throw new FHIRException("Cannot call addChild on a singleton property TestScript.label"); 6276 } 6277 else if (name.equals("description")) { 6278 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 6279 } 6280 else if (name.equals("accept")) { 6281 throw new FHIRException("Cannot call addChild on a singleton property TestScript.accept"); 6282 } 6283 else if (name.equals("contentType")) { 6284 throw new FHIRException("Cannot call addChild on a singleton property TestScript.contentType"); 6285 } 6286 else if (name.equals("destination")) { 6287 throw new FHIRException("Cannot call addChild on a singleton property TestScript.destination"); 6288 } 6289 else if (name.equals("encodeRequestUrl")) { 6290 throw new FHIRException("Cannot call addChild on a singleton property TestScript.encodeRequestUrl"); 6291 } 6292 else if (name.equals("origin")) { 6293 throw new FHIRException("Cannot call addChild on a singleton property TestScript.origin"); 6294 } 6295 else if (name.equals("params")) { 6296 throw new FHIRException("Cannot call addChild on a singleton property TestScript.params"); 6297 } 6298 else if (name.equals("requestHeader")) { 6299 return addRequestHeader(); 6300 } 6301 else if (name.equals("requestId")) { 6302 throw new FHIRException("Cannot call addChild on a singleton property TestScript.requestId"); 6303 } 6304 else if (name.equals("responseId")) { 6305 throw new FHIRException("Cannot call addChild on a singleton property TestScript.responseId"); 6306 } 6307 else if (name.equals("sourceId")) { 6308 throw new FHIRException("Cannot call addChild on a singleton property TestScript.sourceId"); 6309 } 6310 else if (name.equals("targetId")) { 6311 throw new FHIRException("Cannot call addChild on a singleton property TestScript.targetId"); 6312 } 6313 else if (name.equals("url")) { 6314 throw new FHIRException("Cannot call addChild on a singleton property TestScript.url"); 6315 } 6316 else 6317 return super.addChild(name); 6318 } 6319 6320 public SetupActionOperationComponent copy() { 6321 SetupActionOperationComponent dst = new SetupActionOperationComponent(); 6322 copyValues(dst); 6323 dst.type = type == null ? null : type.copy(); 6324 dst.resource = resource == null ? null : resource.copy(); 6325 dst.label = label == null ? null : label.copy(); 6326 dst.description = description == null ? null : description.copy(); 6327 dst.accept = accept == null ? null : accept.copy(); 6328 dst.contentType = contentType == null ? null : contentType.copy(); 6329 dst.destination = destination == null ? null : destination.copy(); 6330 dst.encodeRequestUrl = encodeRequestUrl == null ? null : encodeRequestUrl.copy(); 6331 dst.origin = origin == null ? null : origin.copy(); 6332 dst.params = params == null ? null : params.copy(); 6333 if (requestHeader != null) { 6334 dst.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 6335 for (SetupActionOperationRequestHeaderComponent i : requestHeader) 6336 dst.requestHeader.add(i.copy()); 6337 }; 6338 dst.requestId = requestId == null ? null : requestId.copy(); 6339 dst.responseId = responseId == null ? null : responseId.copy(); 6340 dst.sourceId = sourceId == null ? null : sourceId.copy(); 6341 dst.targetId = targetId == null ? null : targetId.copy(); 6342 dst.url = url == null ? null : url.copy(); 6343 return dst; 6344 } 6345 6346 @Override 6347 public boolean equalsDeep(Base other_) { 6348 if (!super.equalsDeep(other_)) 6349 return false; 6350 if (!(other_ instanceof SetupActionOperationComponent)) 6351 return false; 6352 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 6353 return compareDeep(type, o.type, true) && compareDeep(resource, o.resource, true) && compareDeep(label, o.label, true) 6354 && compareDeep(description, o.description, true) && compareDeep(accept, o.accept, true) && compareDeep(contentType, o.contentType, true) 6355 && compareDeep(destination, o.destination, true) && compareDeep(encodeRequestUrl, o.encodeRequestUrl, true) 6356 && compareDeep(origin, o.origin, true) && compareDeep(params, o.params, true) && compareDeep(requestHeader, o.requestHeader, true) 6357 && compareDeep(requestId, o.requestId, true) && compareDeep(responseId, o.responseId, true) && compareDeep(sourceId, o.sourceId, true) 6358 && compareDeep(targetId, o.targetId, true) && compareDeep(url, o.url, true); 6359 } 6360 6361 @Override 6362 public boolean equalsShallow(Base other_) { 6363 if (!super.equalsShallow(other_)) 6364 return false; 6365 if (!(other_ instanceof SetupActionOperationComponent)) 6366 return false; 6367 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 6368 return compareValues(resource, o.resource, true) && compareValues(label, o.label, true) && compareValues(description, o.description, true) 6369 && compareValues(accept, o.accept, true) && compareValues(contentType, o.contentType, true) && compareValues(destination, o.destination, true) 6370 && compareValues(encodeRequestUrl, o.encodeRequestUrl, true) && compareValues(origin, o.origin, true) 6371 && compareValues(params, o.params, true) && compareValues(requestId, o.requestId, true) && compareValues(responseId, o.responseId, true) 6372 && compareValues(sourceId, o.sourceId, true) && compareValues(targetId, o.targetId, true) && compareValues(url, o.url, true) 6373 ; 6374 } 6375 6376 public boolean isEmpty() { 6377 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resource, label, description 6378 , accept, contentType, destination, encodeRequestUrl, origin, params, requestHeader 6379 , requestId, responseId, sourceId, targetId, url); 6380 } 6381 6382 public String fhirType() { 6383 return "TestScript.setup.action.operation"; 6384 6385 } 6386 6387 } 6388 6389 @Block() 6390 public static class SetupActionOperationRequestHeaderComponent extends BackboneElement implements IBaseBackboneElement { 6391 /** 6392 * The HTTP header field e.g. "Accept". 6393 */ 6394 @Child(name = "field", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 6395 @Description(shortDefinition="HTTP header field name", formalDefinition="The HTTP header field e.g. \"Accept\"." ) 6396 protected StringType field; 6397 6398 /** 6399 * The value of the header e.g. "application/fhir+xml". 6400 */ 6401 @Child(name = "value", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 6402 @Description(shortDefinition="HTTP headerfield value", formalDefinition="The value of the header e.g. \"application/fhir+xml\"." ) 6403 protected StringType value; 6404 6405 private static final long serialVersionUID = 274395337L; 6406 6407 /** 6408 * Constructor 6409 */ 6410 public SetupActionOperationRequestHeaderComponent() { 6411 super(); 6412 } 6413 6414 /** 6415 * Constructor 6416 */ 6417 public SetupActionOperationRequestHeaderComponent(StringType field, StringType value) { 6418 super(); 6419 this.field = field; 6420 this.value = value; 6421 } 6422 6423 /** 6424 * @return {@link #field} (The HTTP header field e.g. "Accept".). This is the underlying object with id, value and extensions. The accessor "getField" gives direct access to the value 6425 */ 6426 public StringType getFieldElement() { 6427 if (this.field == null) 6428 if (Configuration.errorOnAutoCreate()) 6429 throw new Error("Attempt to auto-create SetupActionOperationRequestHeaderComponent.field"); 6430 else if (Configuration.doAutoCreate()) 6431 this.field = new StringType(); // bb 6432 return this.field; 6433 } 6434 6435 public boolean hasFieldElement() { 6436 return this.field != null && !this.field.isEmpty(); 6437 } 6438 6439 public boolean hasField() { 6440 return this.field != null && !this.field.isEmpty(); 6441 } 6442 6443 /** 6444 * @param value {@link #field} (The HTTP header field e.g. "Accept".). This is the underlying object with id, value and extensions. The accessor "getField" gives direct access to the value 6445 */ 6446 public SetupActionOperationRequestHeaderComponent setFieldElement(StringType value) { 6447 this.field = value; 6448 return this; 6449 } 6450 6451 /** 6452 * @return The HTTP header field e.g. "Accept". 6453 */ 6454 public String getField() { 6455 return this.field == null ? null : this.field.getValue(); 6456 } 6457 6458 /** 6459 * @param value The HTTP header field e.g. "Accept". 6460 */ 6461 public SetupActionOperationRequestHeaderComponent setField(String value) { 6462 if (this.field == null) 6463 this.field = new StringType(); 6464 this.field.setValue(value); 6465 return this; 6466 } 6467 6468 /** 6469 * @return {@link #value} (The value of the header e.g. "application/fhir+xml".). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 6470 */ 6471 public StringType getValueElement() { 6472 if (this.value == null) 6473 if (Configuration.errorOnAutoCreate()) 6474 throw new Error("Attempt to auto-create SetupActionOperationRequestHeaderComponent.value"); 6475 else if (Configuration.doAutoCreate()) 6476 this.value = new StringType(); // bb 6477 return this.value; 6478 } 6479 6480 public boolean hasValueElement() { 6481 return this.value != null && !this.value.isEmpty(); 6482 } 6483 6484 public boolean hasValue() { 6485 return this.value != null && !this.value.isEmpty(); 6486 } 6487 6488 /** 6489 * @param value {@link #value} (The value of the header e.g. "application/fhir+xml".). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 6490 */ 6491 public SetupActionOperationRequestHeaderComponent setValueElement(StringType value) { 6492 this.value = value; 6493 return this; 6494 } 6495 6496 /** 6497 * @return The value of the header e.g. "application/fhir+xml". 6498 */ 6499 public String getValue() { 6500 return this.value == null ? null : this.value.getValue(); 6501 } 6502 6503 /** 6504 * @param value The value of the header e.g. "application/fhir+xml". 6505 */ 6506 public SetupActionOperationRequestHeaderComponent setValue(String value) { 6507 if (this.value == null) 6508 this.value = new StringType(); 6509 this.value.setValue(value); 6510 return this; 6511 } 6512 6513 protected void listChildren(List<Property> children) { 6514 super.listChildren(children); 6515 children.add(new Property("field", "string", "The HTTP header field e.g. \"Accept\".", 0, 1, field)); 6516 children.add(new Property("value", "string", "The value of the header e.g. \"application/fhir+xml\".", 0, 1, value)); 6517 } 6518 6519 @Override 6520 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6521 switch (_hash) { 6522 case 97427706: /*field*/ return new Property("field", "string", "The HTTP header field e.g. \"Accept\".", 0, 1, field); 6523 case 111972721: /*value*/ return new Property("value", "string", "The value of the header e.g. \"application/fhir+xml\".", 0, 1, value); 6524 default: return super.getNamedProperty(_hash, _name, _checkValid); 6525 } 6526 6527 } 6528 6529 @Override 6530 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6531 switch (hash) { 6532 case 97427706: /*field*/ return this.field == null ? new Base[0] : new Base[] {this.field}; // StringType 6533 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 6534 default: return super.getProperty(hash, name, checkValid); 6535 } 6536 6537 } 6538 6539 @Override 6540 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6541 switch (hash) { 6542 case 97427706: // field 6543 this.field = castToString(value); // StringType 6544 return value; 6545 case 111972721: // value 6546 this.value = castToString(value); // StringType 6547 return value; 6548 default: return super.setProperty(hash, name, value); 6549 } 6550 6551 } 6552 6553 @Override 6554 public Base setProperty(String name, Base value) throws FHIRException { 6555 if (name.equals("field")) { 6556 this.field = castToString(value); // StringType 6557 } else if (name.equals("value")) { 6558 this.value = castToString(value); // StringType 6559 } else 6560 return super.setProperty(name, value); 6561 return value; 6562 } 6563 6564 @Override 6565 public Base makeProperty(int hash, String name) throws FHIRException { 6566 switch (hash) { 6567 case 97427706: return getFieldElement(); 6568 case 111972721: return getValueElement(); 6569 default: return super.makeProperty(hash, name); 6570 } 6571 6572 } 6573 6574 @Override 6575 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6576 switch (hash) { 6577 case 97427706: /*field*/ return new String[] {"string"}; 6578 case 111972721: /*value*/ return new String[] {"string"}; 6579 default: return super.getTypesForProperty(hash, name); 6580 } 6581 6582 } 6583 6584 @Override 6585 public Base addChild(String name) throws FHIRException { 6586 if (name.equals("field")) { 6587 throw new FHIRException("Cannot call addChild on a singleton property TestScript.field"); 6588 } 6589 else if (name.equals("value")) { 6590 throw new FHIRException("Cannot call addChild on a singleton property TestScript.value"); 6591 } 6592 else 6593 return super.addChild(name); 6594 } 6595 6596 public SetupActionOperationRequestHeaderComponent copy() { 6597 SetupActionOperationRequestHeaderComponent dst = new SetupActionOperationRequestHeaderComponent(); 6598 copyValues(dst); 6599 dst.field = field == null ? null : field.copy(); 6600 dst.value = value == null ? null : value.copy(); 6601 return dst; 6602 } 6603 6604 @Override 6605 public boolean equalsDeep(Base other_) { 6606 if (!super.equalsDeep(other_)) 6607 return false; 6608 if (!(other_ instanceof SetupActionOperationRequestHeaderComponent)) 6609 return false; 6610 SetupActionOperationRequestHeaderComponent o = (SetupActionOperationRequestHeaderComponent) other_; 6611 return compareDeep(field, o.field, true) && compareDeep(value, o.value, true); 6612 } 6613 6614 @Override 6615 public boolean equalsShallow(Base other_) { 6616 if (!super.equalsShallow(other_)) 6617 return false; 6618 if (!(other_ instanceof SetupActionOperationRequestHeaderComponent)) 6619 return false; 6620 SetupActionOperationRequestHeaderComponent o = (SetupActionOperationRequestHeaderComponent) other_; 6621 return compareValues(field, o.field, true) && compareValues(value, o.value, true); 6622 } 6623 6624 public boolean isEmpty() { 6625 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(field, value); 6626 } 6627 6628 public String fhirType() { 6629 return "TestScript.setup.action.operation.requestHeader"; 6630 6631 } 6632 6633 } 6634 6635 @Block() 6636 public static class SetupActionAssertComponent extends BackboneElement implements IBaseBackboneElement { 6637 /** 6638 * The label would be used for tracking/logging purposes by test engines. 6639 */ 6640 @Child(name = "label", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 6641 @Description(shortDefinition="Tracking/logging assertion label", formalDefinition="The label would be used for tracking/logging purposes by test engines." ) 6642 protected StringType label; 6643 6644 /** 6645 * The description would be used by test engines for tracking and reporting purposes. 6646 */ 6647 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 6648 @Description(shortDefinition="Tracking/reporting assertion description", formalDefinition="The description would be used by test engines for tracking and reporting purposes." ) 6649 protected StringType description; 6650 6651 /** 6652 * The direction to use for the assertion. 6653 */ 6654 @Child(name = "direction", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 6655 @Description(shortDefinition="response | request", formalDefinition="The direction to use for the assertion." ) 6656 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/assert-direction-codes") 6657 protected Enumeration<AssertionDirectionType> direction; 6658 6659 /** 6660 * Id of the source fixture used as the contents to be evaluated by either the "source/expression" or "sourceId/path" definition. 6661 */ 6662 @Child(name = "compareToSourceId", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 6663 @Description(shortDefinition="Id of the source fixture to be evaluated", formalDefinition="Id of the source fixture used as the contents to be evaluated by either the \"source/expression\" or \"sourceId/path\" definition." ) 6664 protected StringType compareToSourceId; 6665 6666 /** 6667 * The fluentpath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 6668 */ 6669 @Child(name = "compareToSourceExpression", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 6670 @Description(shortDefinition="The fluentpath expression to evaluate against the source fixture", formalDefinition="The fluentpath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both." ) 6671 protected StringType compareToSourceExpression; 6672 6673 /** 6674 * XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 6675 */ 6676 @Child(name = "compareToSourcePath", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 6677 @Description(shortDefinition="XPath or JSONPath expression to evaluate against the source fixture", formalDefinition="XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both." ) 6678 protected StringType compareToSourcePath; 6679 6680 /** 6681 * The content-type or mime-type to use for RESTful operation in the 'Content-Type' header. 6682 */ 6683 @Child(name = "contentType", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 6684 @Description(shortDefinition="xml | json | ttl | none", formalDefinition="The content-type or mime-type to use for RESTful operation in the 'Content-Type' header." ) 6685 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/content-type") 6686 protected Enumeration<ContentType> contentType; 6687 6688 /** 6689 * The fluentpath expression to be evaluated against the request or response message contents - HTTP headers and payload. 6690 */ 6691 @Child(name = "expression", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 6692 @Description(shortDefinition="The fluentpath expression to be evaluated", formalDefinition="The fluentpath expression to be evaluated against the request or response message contents - HTTP headers and payload." ) 6693 protected StringType expression; 6694 6695 /** 6696 * The HTTP header field name e.g. 'Location'. 6697 */ 6698 @Child(name = "headerField", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=false) 6699 @Description(shortDefinition="HTTP header field name", formalDefinition="The HTTP header field name e.g. 'Location'." ) 6700 protected StringType headerField; 6701 6702 /** 6703 * The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId. 6704 */ 6705 @Child(name = "minimumId", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 6706 @Description(shortDefinition="Fixture Id of minimum content resource", formalDefinition="The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId." ) 6707 protected StringType minimumId; 6708 6709 /** 6710 * Whether or not the test execution performs validation on the bundle navigation links. 6711 */ 6712 @Child(name = "navigationLinks", type = {BooleanType.class}, order=11, min=0, max=1, modifier=false, summary=false) 6713 @Description(shortDefinition="Perform validation on navigation links?", formalDefinition="Whether or not the test execution performs validation on the bundle navigation links." ) 6714 protected BooleanType navigationLinks; 6715 6716 /** 6717 * The operator type defines the conditional behavior of the assert. If not defined, the default is equals. 6718 */ 6719 @Child(name = "operator", type = {CodeType.class}, order=12, min=0, max=1, modifier=false, summary=false) 6720 @Description(shortDefinition="equals | notEquals | in | notIn | greaterThan | lessThan | empty | notEmpty | contains | notContains | eval", formalDefinition="The operator type defines the conditional behavior of the assert. If not defined, the default is equals." ) 6721 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/assert-operator-codes") 6722 protected Enumeration<AssertionOperatorType> operator; 6723 6724 /** 6725 * The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server. 6726 */ 6727 @Child(name = "path", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 6728 @Description(shortDefinition="XPath or JSONPath expression", formalDefinition="The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server." ) 6729 protected StringType path; 6730 6731 /** 6732 * The request method or HTTP operation code to compare against that used by the client system under test. 6733 */ 6734 @Child(name = "requestMethod", type = {CodeType.class}, order=14, min=0, max=1, modifier=false, summary=false) 6735 @Description(shortDefinition="delete | get | options | patch | post | put", formalDefinition="The request method or HTTP operation code to compare against that used by the client system under test." ) 6736 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/http-operations") 6737 protected Enumeration<TestScriptRequestMethodCode> requestMethod; 6738 6739 /** 6740 * The value to use in a comparison against the request URL path string. 6741 */ 6742 @Child(name = "requestURL", type = {StringType.class}, order=15, min=0, max=1, modifier=false, summary=false) 6743 @Description(shortDefinition="Request URL comparison value", formalDefinition="The value to use in a comparison against the request URL path string." ) 6744 protected StringType requestURL; 6745 6746 /** 6747 * The type of the resource. See http://build.fhir.org/resourcelist.html. 6748 */ 6749 @Child(name = "resource", type = {CodeType.class}, order=16, min=0, max=1, modifier=false, summary=false) 6750 @Description(shortDefinition="Resource type", formalDefinition="The type of the resource. See http://build.fhir.org/resourcelist.html." ) 6751 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/defined-types") 6752 protected CodeType resource; 6753 6754 /** 6755 * okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable. 6756 */ 6757 @Child(name = "response", type = {CodeType.class}, order=17, min=0, max=1, modifier=false, summary=false) 6758 @Description(shortDefinition="okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable", formalDefinition="okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable." ) 6759 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/assert-response-code-types") 6760 protected Enumeration<AssertionResponseTypes> response; 6761 6762 /** 6763 * The value of the HTTP response code to be tested. 6764 */ 6765 @Child(name = "responseCode", type = {StringType.class}, order=18, min=0, max=1, modifier=false, summary=false) 6766 @Description(shortDefinition="HTTP response code to test", formalDefinition="The value of the HTTP response code to be tested." ) 6767 protected StringType responseCode; 6768 6769 /** 6770 * The TestScript.rule this assert will evaluate. 6771 */ 6772 @Child(name = "rule", type = {}, order=19, min=0, max=1, modifier=false, summary=false) 6773 @Description(shortDefinition="The reference to a TestScript.rule", formalDefinition="The TestScript.rule this assert will evaluate." ) 6774 protected ActionAssertRuleComponent rule; 6775 6776 /** 6777 * The TestScript.ruleset this assert will evaluate. 6778 */ 6779 @Child(name = "ruleset", type = {}, order=20, min=0, max=1, modifier=false, summary=false) 6780 @Description(shortDefinition="The reference to a TestScript.ruleset", formalDefinition="The TestScript.ruleset this assert will evaluate." ) 6781 protected ActionAssertRulesetComponent ruleset; 6782 6783 /** 6784 * Fixture to evaluate the XPath/JSONPath expression or the headerField against. 6785 */ 6786 @Child(name = "sourceId", type = {IdType.class}, order=21, min=0, max=1, modifier=false, summary=false) 6787 @Description(shortDefinition="Fixture Id of source expression or headerField", formalDefinition="Fixture to evaluate the XPath/JSONPath expression or the headerField against." ) 6788 protected IdType sourceId; 6789 6790 /** 6791 * The ID of the Profile to validate against. 6792 */ 6793 @Child(name = "validateProfileId", type = {IdType.class}, order=22, min=0, max=1, modifier=false, summary=false) 6794 @Description(shortDefinition="Profile Id of validation profile reference", formalDefinition="The ID of the Profile to validate against." ) 6795 protected IdType validateProfileId; 6796 6797 /** 6798 * The value to compare to. 6799 */ 6800 @Child(name = "value", type = {StringType.class}, order=23, min=0, max=1, modifier=false, summary=false) 6801 @Description(shortDefinition="The value to compare to", formalDefinition="The value to compare to." ) 6802 protected StringType value; 6803 6804 /** 6805 * Whether or not the test execution will produce a warning only on error for this assert. 6806 */ 6807 @Child(name = "warningOnly", type = {BooleanType.class}, order=24, min=0, max=1, modifier=false, summary=false) 6808 @Description(shortDefinition="Will this assert produce a warning only on error?", formalDefinition="Whether or not the test execution will produce a warning only on error for this assert." ) 6809 protected BooleanType warningOnly; 6810 6811 private static final long serialVersionUID = 171718507L; 6812 6813 /** 6814 * Constructor 6815 */ 6816 public SetupActionAssertComponent() { 6817 super(); 6818 } 6819 6820 /** 6821 * @return {@link #label} (The label would be used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 6822 */ 6823 public StringType getLabelElement() { 6824 if (this.label == null) 6825 if (Configuration.errorOnAutoCreate()) 6826 throw new Error("Attempt to auto-create SetupActionAssertComponent.label"); 6827 else if (Configuration.doAutoCreate()) 6828 this.label = new StringType(); // bb 6829 return this.label; 6830 } 6831 6832 public boolean hasLabelElement() { 6833 return this.label != null && !this.label.isEmpty(); 6834 } 6835 6836 public boolean hasLabel() { 6837 return this.label != null && !this.label.isEmpty(); 6838 } 6839 6840 /** 6841 * @param value {@link #label} (The label would be used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 6842 */ 6843 public SetupActionAssertComponent setLabelElement(StringType value) { 6844 this.label = value; 6845 return this; 6846 } 6847 6848 /** 6849 * @return The label would be used for tracking/logging purposes by test engines. 6850 */ 6851 public String getLabel() { 6852 return this.label == null ? null : this.label.getValue(); 6853 } 6854 6855 /** 6856 * @param value The label would be used for tracking/logging purposes by test engines. 6857 */ 6858 public SetupActionAssertComponent setLabel(String value) { 6859 if (Utilities.noString(value)) 6860 this.label = null; 6861 else { 6862 if (this.label == null) 6863 this.label = new StringType(); 6864 this.label.setValue(value); 6865 } 6866 return this; 6867 } 6868 6869 /** 6870 * @return {@link #description} (The description would be used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6871 */ 6872 public StringType getDescriptionElement() { 6873 if (this.description == null) 6874 if (Configuration.errorOnAutoCreate()) 6875 throw new Error("Attempt to auto-create SetupActionAssertComponent.description"); 6876 else if (Configuration.doAutoCreate()) 6877 this.description = new StringType(); // bb 6878 return this.description; 6879 } 6880 6881 public boolean hasDescriptionElement() { 6882 return this.description != null && !this.description.isEmpty(); 6883 } 6884 6885 public boolean hasDescription() { 6886 return this.description != null && !this.description.isEmpty(); 6887 } 6888 6889 /** 6890 * @param value {@link #description} (The description would be used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 6891 */ 6892 public SetupActionAssertComponent setDescriptionElement(StringType value) { 6893 this.description = value; 6894 return this; 6895 } 6896 6897 /** 6898 * @return The description would be used by test engines for tracking and reporting purposes. 6899 */ 6900 public String getDescription() { 6901 return this.description == null ? null : this.description.getValue(); 6902 } 6903 6904 /** 6905 * @param value The description would be used by test engines for tracking and reporting purposes. 6906 */ 6907 public SetupActionAssertComponent setDescription(String value) { 6908 if (Utilities.noString(value)) 6909 this.description = null; 6910 else { 6911 if (this.description == null) 6912 this.description = new StringType(); 6913 this.description.setValue(value); 6914 } 6915 return this; 6916 } 6917 6918 /** 6919 * @return {@link #direction} (The direction to use for the assertion.). This is the underlying object with id, value and extensions. The accessor "getDirection" gives direct access to the value 6920 */ 6921 public Enumeration<AssertionDirectionType> getDirectionElement() { 6922 if (this.direction == null) 6923 if (Configuration.errorOnAutoCreate()) 6924 throw new Error("Attempt to auto-create SetupActionAssertComponent.direction"); 6925 else if (Configuration.doAutoCreate()) 6926 this.direction = new Enumeration<AssertionDirectionType>(new AssertionDirectionTypeEnumFactory()); // bb 6927 return this.direction; 6928 } 6929 6930 public boolean hasDirectionElement() { 6931 return this.direction != null && !this.direction.isEmpty(); 6932 } 6933 6934 public boolean hasDirection() { 6935 return this.direction != null && !this.direction.isEmpty(); 6936 } 6937 6938 /** 6939 * @param value {@link #direction} (The direction to use for the assertion.). This is the underlying object with id, value and extensions. The accessor "getDirection" gives direct access to the value 6940 */ 6941 public SetupActionAssertComponent setDirectionElement(Enumeration<AssertionDirectionType> value) { 6942 this.direction = value; 6943 return this; 6944 } 6945 6946 /** 6947 * @return The direction to use for the assertion. 6948 */ 6949 public AssertionDirectionType getDirection() { 6950 return this.direction == null ? null : this.direction.getValue(); 6951 } 6952 6953 /** 6954 * @param value The direction to use for the assertion. 6955 */ 6956 public SetupActionAssertComponent setDirection(AssertionDirectionType value) { 6957 if (value == null) 6958 this.direction = null; 6959 else { 6960 if (this.direction == null) 6961 this.direction = new Enumeration<AssertionDirectionType>(new AssertionDirectionTypeEnumFactory()); 6962 this.direction.setValue(value); 6963 } 6964 return this; 6965 } 6966 6967 /** 6968 * @return {@link #compareToSourceId} (Id of the source fixture used as the contents to be evaluated by either the "source/expression" or "sourceId/path" definition.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourceId" gives direct access to the value 6969 */ 6970 public StringType getCompareToSourceIdElement() { 6971 if (this.compareToSourceId == null) 6972 if (Configuration.errorOnAutoCreate()) 6973 throw new Error("Attempt to auto-create SetupActionAssertComponent.compareToSourceId"); 6974 else if (Configuration.doAutoCreate()) 6975 this.compareToSourceId = new StringType(); // bb 6976 return this.compareToSourceId; 6977 } 6978 6979 public boolean hasCompareToSourceIdElement() { 6980 return this.compareToSourceId != null && !this.compareToSourceId.isEmpty(); 6981 } 6982 6983 public boolean hasCompareToSourceId() { 6984 return this.compareToSourceId != null && !this.compareToSourceId.isEmpty(); 6985 } 6986 6987 /** 6988 * @param value {@link #compareToSourceId} (Id of the source fixture used as the contents to be evaluated by either the "source/expression" or "sourceId/path" definition.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourceId" gives direct access to the value 6989 */ 6990 public SetupActionAssertComponent setCompareToSourceIdElement(StringType value) { 6991 this.compareToSourceId = value; 6992 return this; 6993 } 6994 6995 /** 6996 * @return Id of the source fixture used as the contents to be evaluated by either the "source/expression" or "sourceId/path" definition. 6997 */ 6998 public String getCompareToSourceId() { 6999 return this.compareToSourceId == null ? null : this.compareToSourceId.getValue(); 7000 } 7001 7002 /** 7003 * @param value Id of the source fixture used as the contents to be evaluated by either the "source/expression" or "sourceId/path" definition. 7004 */ 7005 public SetupActionAssertComponent setCompareToSourceId(String value) { 7006 if (Utilities.noString(value)) 7007 this.compareToSourceId = null; 7008 else { 7009 if (this.compareToSourceId == null) 7010 this.compareToSourceId = new StringType(); 7011 this.compareToSourceId.setValue(value); 7012 } 7013 return this; 7014 } 7015 7016 /** 7017 * @return {@link #compareToSourceExpression} (The fluentpath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourceExpression" gives direct access to the value 7018 */ 7019 public StringType getCompareToSourceExpressionElement() { 7020 if (this.compareToSourceExpression == null) 7021 if (Configuration.errorOnAutoCreate()) 7022 throw new Error("Attempt to auto-create SetupActionAssertComponent.compareToSourceExpression"); 7023 else if (Configuration.doAutoCreate()) 7024 this.compareToSourceExpression = new StringType(); // bb 7025 return this.compareToSourceExpression; 7026 } 7027 7028 public boolean hasCompareToSourceExpressionElement() { 7029 return this.compareToSourceExpression != null && !this.compareToSourceExpression.isEmpty(); 7030 } 7031 7032 public boolean hasCompareToSourceExpression() { 7033 return this.compareToSourceExpression != null && !this.compareToSourceExpression.isEmpty(); 7034 } 7035 7036 /** 7037 * @param value {@link #compareToSourceExpression} (The fluentpath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourceExpression" gives direct access to the value 7038 */ 7039 public SetupActionAssertComponent setCompareToSourceExpressionElement(StringType value) { 7040 this.compareToSourceExpression = value; 7041 return this; 7042 } 7043 7044 /** 7045 * @return The fluentpath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 7046 */ 7047 public String getCompareToSourceExpression() { 7048 return this.compareToSourceExpression == null ? null : this.compareToSourceExpression.getValue(); 7049 } 7050 7051 /** 7052 * @param value The fluentpath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 7053 */ 7054 public SetupActionAssertComponent setCompareToSourceExpression(String value) { 7055 if (Utilities.noString(value)) 7056 this.compareToSourceExpression = null; 7057 else { 7058 if (this.compareToSourceExpression == null) 7059 this.compareToSourceExpression = new StringType(); 7060 this.compareToSourceExpression.setValue(value); 7061 } 7062 return this; 7063 } 7064 7065 /** 7066 * @return {@link #compareToSourcePath} (XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourcePath" gives direct access to the value 7067 */ 7068 public StringType getCompareToSourcePathElement() { 7069 if (this.compareToSourcePath == null) 7070 if (Configuration.errorOnAutoCreate()) 7071 throw new Error("Attempt to auto-create SetupActionAssertComponent.compareToSourcePath"); 7072 else if (Configuration.doAutoCreate()) 7073 this.compareToSourcePath = new StringType(); // bb 7074 return this.compareToSourcePath; 7075 } 7076 7077 public boolean hasCompareToSourcePathElement() { 7078 return this.compareToSourcePath != null && !this.compareToSourcePath.isEmpty(); 7079 } 7080 7081 public boolean hasCompareToSourcePath() { 7082 return this.compareToSourcePath != null && !this.compareToSourcePath.isEmpty(); 7083 } 7084 7085 /** 7086 * @param value {@link #compareToSourcePath} (XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.). This is the underlying object with id, value and extensions. The accessor "getCompareToSourcePath" gives direct access to the value 7087 */ 7088 public SetupActionAssertComponent setCompareToSourcePathElement(StringType value) { 7089 this.compareToSourcePath = value; 7090 return this; 7091 } 7092 7093 /** 7094 * @return XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 7095 */ 7096 public String getCompareToSourcePath() { 7097 return this.compareToSourcePath == null ? null : this.compareToSourcePath.getValue(); 7098 } 7099 7100 /** 7101 * @param value XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both. 7102 */ 7103 public SetupActionAssertComponent setCompareToSourcePath(String value) { 7104 if (Utilities.noString(value)) 7105 this.compareToSourcePath = null; 7106 else { 7107 if (this.compareToSourcePath == null) 7108 this.compareToSourcePath = new StringType(); 7109 this.compareToSourcePath.setValue(value); 7110 } 7111 return this; 7112 } 7113 7114 /** 7115 * @return {@link #contentType} (The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 7116 */ 7117 public Enumeration<ContentType> getContentTypeElement() { 7118 if (this.contentType == null) 7119 if (Configuration.errorOnAutoCreate()) 7120 throw new Error("Attempt to auto-create SetupActionAssertComponent.contentType"); 7121 else if (Configuration.doAutoCreate()) 7122 this.contentType = new Enumeration<ContentType>(new ContentTypeEnumFactory()); // bb 7123 return this.contentType; 7124 } 7125 7126 public boolean hasContentTypeElement() { 7127 return this.contentType != null && !this.contentType.isEmpty(); 7128 } 7129 7130 public boolean hasContentType() { 7131 return this.contentType != null && !this.contentType.isEmpty(); 7132 } 7133 7134 /** 7135 * @param value {@link #contentType} (The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.). This is the underlying object with id, value and extensions. The accessor "getContentType" gives direct access to the value 7136 */ 7137 public SetupActionAssertComponent setContentTypeElement(Enumeration<ContentType> value) { 7138 this.contentType = value; 7139 return this; 7140 } 7141 7142 /** 7143 * @return The content-type or mime-type to use for RESTful operation in the 'Content-Type' header. 7144 */ 7145 public ContentType getContentType() { 7146 return this.contentType == null ? null : this.contentType.getValue(); 7147 } 7148 7149 /** 7150 * @param value The content-type or mime-type to use for RESTful operation in the 'Content-Type' header. 7151 */ 7152 public SetupActionAssertComponent setContentType(ContentType value) { 7153 if (value == null) 7154 this.contentType = null; 7155 else { 7156 if (this.contentType == null) 7157 this.contentType = new Enumeration<ContentType>(new ContentTypeEnumFactory()); 7158 this.contentType.setValue(value); 7159 } 7160 return this; 7161 } 7162 7163 /** 7164 * @return {@link #expression} (The fluentpath expression to be evaluated against the request or response message contents - HTTP headers and payload.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 7165 */ 7166 public StringType getExpressionElement() { 7167 if (this.expression == null) 7168 if (Configuration.errorOnAutoCreate()) 7169 throw new Error("Attempt to auto-create SetupActionAssertComponent.expression"); 7170 else if (Configuration.doAutoCreate()) 7171 this.expression = new StringType(); // bb 7172 return this.expression; 7173 } 7174 7175 public boolean hasExpressionElement() { 7176 return this.expression != null && !this.expression.isEmpty(); 7177 } 7178 7179 public boolean hasExpression() { 7180 return this.expression != null && !this.expression.isEmpty(); 7181 } 7182 7183 /** 7184 * @param value {@link #expression} (The fluentpath expression to be evaluated against the request or response message contents - HTTP headers and payload.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 7185 */ 7186 public SetupActionAssertComponent setExpressionElement(StringType value) { 7187 this.expression = value; 7188 return this; 7189 } 7190 7191 /** 7192 * @return The fluentpath expression to be evaluated against the request or response message contents - HTTP headers and payload. 7193 */ 7194 public String getExpression() { 7195 return this.expression == null ? null : this.expression.getValue(); 7196 } 7197 7198 /** 7199 * @param value The fluentpath expression to be evaluated against the request or response message contents - HTTP headers and payload. 7200 */ 7201 public SetupActionAssertComponent setExpression(String value) { 7202 if (Utilities.noString(value)) 7203 this.expression = null; 7204 else { 7205 if (this.expression == null) 7206 this.expression = new StringType(); 7207 this.expression.setValue(value); 7208 } 7209 return this; 7210 } 7211 7212 /** 7213 * @return {@link #headerField} (The HTTP header field name e.g. 'Location'.). This is the underlying object with id, value and extensions. The accessor "getHeaderField" gives direct access to the value 7214 */ 7215 public StringType getHeaderFieldElement() { 7216 if (this.headerField == null) 7217 if (Configuration.errorOnAutoCreate()) 7218 throw new Error("Attempt to auto-create SetupActionAssertComponent.headerField"); 7219 else if (Configuration.doAutoCreate()) 7220 this.headerField = new StringType(); // bb 7221 return this.headerField; 7222 } 7223 7224 public boolean hasHeaderFieldElement() { 7225 return this.headerField != null && !this.headerField.isEmpty(); 7226 } 7227 7228 public boolean hasHeaderField() { 7229 return this.headerField != null && !this.headerField.isEmpty(); 7230 } 7231 7232 /** 7233 * @param value {@link #headerField} (The HTTP header field name e.g. 'Location'.). This is the underlying object with id, value and extensions. The accessor "getHeaderField" gives direct access to the value 7234 */ 7235 public SetupActionAssertComponent setHeaderFieldElement(StringType value) { 7236 this.headerField = value; 7237 return this; 7238 } 7239 7240 /** 7241 * @return The HTTP header field name e.g. 'Location'. 7242 */ 7243 public String getHeaderField() { 7244 return this.headerField == null ? null : this.headerField.getValue(); 7245 } 7246 7247 /** 7248 * @param value The HTTP header field name e.g. 'Location'. 7249 */ 7250 public SetupActionAssertComponent setHeaderField(String value) { 7251 if (Utilities.noString(value)) 7252 this.headerField = null; 7253 else { 7254 if (this.headerField == null) 7255 this.headerField = new StringType(); 7256 this.headerField.setValue(value); 7257 } 7258 return this; 7259 } 7260 7261 /** 7262 * @return {@link #minimumId} (The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.). This is the underlying object with id, value and extensions. The accessor "getMinimumId" gives direct access to the value 7263 */ 7264 public StringType getMinimumIdElement() { 7265 if (this.minimumId == null) 7266 if (Configuration.errorOnAutoCreate()) 7267 throw new Error("Attempt to auto-create SetupActionAssertComponent.minimumId"); 7268 else if (Configuration.doAutoCreate()) 7269 this.minimumId = new StringType(); // bb 7270 return this.minimumId; 7271 } 7272 7273 public boolean hasMinimumIdElement() { 7274 return this.minimumId != null && !this.minimumId.isEmpty(); 7275 } 7276 7277 public boolean hasMinimumId() { 7278 return this.minimumId != null && !this.minimumId.isEmpty(); 7279 } 7280 7281 /** 7282 * @param value {@link #minimumId} (The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.). This is the underlying object with id, value and extensions. The accessor "getMinimumId" gives direct access to the value 7283 */ 7284 public SetupActionAssertComponent setMinimumIdElement(StringType value) { 7285 this.minimumId = value; 7286 return this; 7287 } 7288 7289 /** 7290 * @return The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId. 7291 */ 7292 public String getMinimumId() { 7293 return this.minimumId == null ? null : this.minimumId.getValue(); 7294 } 7295 7296 /** 7297 * @param value The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId. 7298 */ 7299 public SetupActionAssertComponent setMinimumId(String value) { 7300 if (Utilities.noString(value)) 7301 this.minimumId = null; 7302 else { 7303 if (this.minimumId == null) 7304 this.minimumId = new StringType(); 7305 this.minimumId.setValue(value); 7306 } 7307 return this; 7308 } 7309 7310 /** 7311 * @return {@link #navigationLinks} (Whether or not the test execution performs validation on the bundle navigation links.). This is the underlying object with id, value and extensions. The accessor "getNavigationLinks" gives direct access to the value 7312 */ 7313 public BooleanType getNavigationLinksElement() { 7314 if (this.navigationLinks == null) 7315 if (Configuration.errorOnAutoCreate()) 7316 throw new Error("Attempt to auto-create SetupActionAssertComponent.navigationLinks"); 7317 else if (Configuration.doAutoCreate()) 7318 this.navigationLinks = new BooleanType(); // bb 7319 return this.navigationLinks; 7320 } 7321 7322 public boolean hasNavigationLinksElement() { 7323 return this.navigationLinks != null && !this.navigationLinks.isEmpty(); 7324 } 7325 7326 public boolean hasNavigationLinks() { 7327 return this.navigationLinks != null && !this.navigationLinks.isEmpty(); 7328 } 7329 7330 /** 7331 * @param value {@link #navigationLinks} (Whether or not the test execution performs validation on the bundle navigation links.). This is the underlying object with id, value and extensions. The accessor "getNavigationLinks" gives direct access to the value 7332 */ 7333 public SetupActionAssertComponent setNavigationLinksElement(BooleanType value) { 7334 this.navigationLinks = value; 7335 return this; 7336 } 7337 7338 /** 7339 * @return Whether or not the test execution performs validation on the bundle navigation links. 7340 */ 7341 public boolean getNavigationLinks() { 7342 return this.navigationLinks == null || this.navigationLinks.isEmpty() ? false : this.navigationLinks.getValue(); 7343 } 7344 7345 /** 7346 * @param value Whether or not the test execution performs validation on the bundle navigation links. 7347 */ 7348 public SetupActionAssertComponent setNavigationLinks(boolean value) { 7349 if (this.navigationLinks == null) 7350 this.navigationLinks = new BooleanType(); 7351 this.navigationLinks.setValue(value); 7352 return this; 7353 } 7354 7355 /** 7356 * @return {@link #operator} (The operator type defines the conditional behavior of the assert. If not defined, the default is equals.). This is the underlying object with id, value and extensions. The accessor "getOperator" gives direct access to the value 7357 */ 7358 public Enumeration<AssertionOperatorType> getOperatorElement() { 7359 if (this.operator == null) 7360 if (Configuration.errorOnAutoCreate()) 7361 throw new Error("Attempt to auto-create SetupActionAssertComponent.operator"); 7362 else if (Configuration.doAutoCreate()) 7363 this.operator = new Enumeration<AssertionOperatorType>(new AssertionOperatorTypeEnumFactory()); // bb 7364 return this.operator; 7365 } 7366 7367 public boolean hasOperatorElement() { 7368 return this.operator != null && !this.operator.isEmpty(); 7369 } 7370 7371 public boolean hasOperator() { 7372 return this.operator != null && !this.operator.isEmpty(); 7373 } 7374 7375 /** 7376 * @param value {@link #operator} (The operator type defines the conditional behavior of the assert. If not defined, the default is equals.). This is the underlying object with id, value and extensions. The accessor "getOperator" gives direct access to the value 7377 */ 7378 public SetupActionAssertComponent setOperatorElement(Enumeration<AssertionOperatorType> value) { 7379 this.operator = value; 7380 return this; 7381 } 7382 7383 /** 7384 * @return The operator type defines the conditional behavior of the assert. If not defined, the default is equals. 7385 */ 7386 public AssertionOperatorType getOperator() { 7387 return this.operator == null ? null : this.operator.getValue(); 7388 } 7389 7390 /** 7391 * @param value The operator type defines the conditional behavior of the assert. If not defined, the default is equals. 7392 */ 7393 public SetupActionAssertComponent setOperator(AssertionOperatorType value) { 7394 if (value == null) 7395 this.operator = null; 7396 else { 7397 if (this.operator == null) 7398 this.operator = new Enumeration<AssertionOperatorType>(new AssertionOperatorTypeEnumFactory()); 7399 this.operator.setValue(value); 7400 } 7401 return this; 7402 } 7403 7404 /** 7405 * @return {@link #path} (The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 7406 */ 7407 public StringType getPathElement() { 7408 if (this.path == null) 7409 if (Configuration.errorOnAutoCreate()) 7410 throw new Error("Attempt to auto-create SetupActionAssertComponent.path"); 7411 else if (Configuration.doAutoCreate()) 7412 this.path = new StringType(); // bb 7413 return this.path; 7414 } 7415 7416 public boolean hasPathElement() { 7417 return this.path != null && !this.path.isEmpty(); 7418 } 7419 7420 public boolean hasPath() { 7421 return this.path != null && !this.path.isEmpty(); 7422 } 7423 7424 /** 7425 * @param value {@link #path} (The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 7426 */ 7427 public SetupActionAssertComponent setPathElement(StringType value) { 7428 this.path = value; 7429 return this; 7430 } 7431 7432 /** 7433 * @return The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server. 7434 */ 7435 public String getPath() { 7436 return this.path == null ? null : this.path.getValue(); 7437 } 7438 7439 /** 7440 * @param value The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server. 7441 */ 7442 public SetupActionAssertComponent setPath(String value) { 7443 if (Utilities.noString(value)) 7444 this.path = null; 7445 else { 7446 if (this.path == null) 7447 this.path = new StringType(); 7448 this.path.setValue(value); 7449 } 7450 return this; 7451 } 7452 7453 /** 7454 * @return {@link #requestMethod} (The request method or HTTP operation code to compare against that used by the client system under test.). This is the underlying object with id, value and extensions. The accessor "getRequestMethod" gives direct access to the value 7455 */ 7456 public Enumeration<TestScriptRequestMethodCode> getRequestMethodElement() { 7457 if (this.requestMethod == null) 7458 if (Configuration.errorOnAutoCreate()) 7459 throw new Error("Attempt to auto-create SetupActionAssertComponent.requestMethod"); 7460 else if (Configuration.doAutoCreate()) 7461 this.requestMethod = new Enumeration<TestScriptRequestMethodCode>(new TestScriptRequestMethodCodeEnumFactory()); // bb 7462 return this.requestMethod; 7463 } 7464 7465 public boolean hasRequestMethodElement() { 7466 return this.requestMethod != null && !this.requestMethod.isEmpty(); 7467 } 7468 7469 public boolean hasRequestMethod() { 7470 return this.requestMethod != null && !this.requestMethod.isEmpty(); 7471 } 7472 7473 /** 7474 * @param value {@link #requestMethod} (The request method or HTTP operation code to compare against that used by the client system under test.). This is the underlying object with id, value and extensions. The accessor "getRequestMethod" gives direct access to the value 7475 */ 7476 public SetupActionAssertComponent setRequestMethodElement(Enumeration<TestScriptRequestMethodCode> value) { 7477 this.requestMethod = value; 7478 return this; 7479 } 7480 7481 /** 7482 * @return The request method or HTTP operation code to compare against that used by the client system under test. 7483 */ 7484 public TestScriptRequestMethodCode getRequestMethod() { 7485 return this.requestMethod == null ? null : this.requestMethod.getValue(); 7486 } 7487 7488 /** 7489 * @param value The request method or HTTP operation code to compare against that used by the client system under test. 7490 */ 7491 public SetupActionAssertComponent setRequestMethod(TestScriptRequestMethodCode value) { 7492 if (value == null) 7493 this.requestMethod = null; 7494 else { 7495 if (this.requestMethod == null) 7496 this.requestMethod = new Enumeration<TestScriptRequestMethodCode>(new TestScriptRequestMethodCodeEnumFactory()); 7497 this.requestMethod.setValue(value); 7498 } 7499 return this; 7500 } 7501 7502 /** 7503 * @return {@link #requestURL} (The value to use in a comparison against the request URL path string.). This is the underlying object with id, value and extensions. The accessor "getRequestURL" gives direct access to the value 7504 */ 7505 public StringType getRequestURLElement() { 7506 if (this.requestURL == null) 7507 if (Configuration.errorOnAutoCreate()) 7508 throw new Error("Attempt to auto-create SetupActionAssertComponent.requestURL"); 7509 else if (Configuration.doAutoCreate()) 7510 this.requestURL = new StringType(); // bb 7511 return this.requestURL; 7512 } 7513 7514 public boolean hasRequestURLElement() { 7515 return this.requestURL != null && !this.requestURL.isEmpty(); 7516 } 7517 7518 public boolean hasRequestURL() { 7519 return this.requestURL != null && !this.requestURL.isEmpty(); 7520 } 7521 7522 /** 7523 * @param value {@link #requestURL} (The value to use in a comparison against the request URL path string.). This is the underlying object with id, value and extensions. The accessor "getRequestURL" gives direct access to the value 7524 */ 7525 public SetupActionAssertComponent setRequestURLElement(StringType value) { 7526 this.requestURL = value; 7527 return this; 7528 } 7529 7530 /** 7531 * @return The value to use in a comparison against the request URL path string. 7532 */ 7533 public String getRequestURL() { 7534 return this.requestURL == null ? null : this.requestURL.getValue(); 7535 } 7536 7537 /** 7538 * @param value The value to use in a comparison against the request URL path string. 7539 */ 7540 public SetupActionAssertComponent setRequestURL(String value) { 7541 if (Utilities.noString(value)) 7542 this.requestURL = null; 7543 else { 7544 if (this.requestURL == null) 7545 this.requestURL = new StringType(); 7546 this.requestURL.setValue(value); 7547 } 7548 return this; 7549 } 7550 7551 /** 7552 * @return {@link #resource} (The type of the resource. See http://build.fhir.org/resourcelist.html.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 7553 */ 7554 public CodeType getResourceElement() { 7555 if (this.resource == null) 7556 if (Configuration.errorOnAutoCreate()) 7557 throw new Error("Attempt to auto-create SetupActionAssertComponent.resource"); 7558 else if (Configuration.doAutoCreate()) 7559 this.resource = new CodeType(); // bb 7560 return this.resource; 7561 } 7562 7563 public boolean hasResourceElement() { 7564 return this.resource != null && !this.resource.isEmpty(); 7565 } 7566 7567 public boolean hasResource() { 7568 return this.resource != null && !this.resource.isEmpty(); 7569 } 7570 7571 /** 7572 * @param value {@link #resource} (The type of the resource. See http://build.fhir.org/resourcelist.html.). This is the underlying object with id, value and extensions. The accessor "getResource" gives direct access to the value 7573 */ 7574 public SetupActionAssertComponent setResourceElement(CodeType value) { 7575 this.resource = value; 7576 return this; 7577 } 7578 7579 /** 7580 * @return The type of the resource. See http://build.fhir.org/resourcelist.html. 7581 */ 7582 public String getResource() { 7583 return this.resource == null ? null : this.resource.getValue(); 7584 } 7585 7586 /** 7587 * @param value The type of the resource. See http://build.fhir.org/resourcelist.html. 7588 */ 7589 public SetupActionAssertComponent setResource(String value) { 7590 if (Utilities.noString(value)) 7591 this.resource = null; 7592 else { 7593 if (this.resource == null) 7594 this.resource = new CodeType(); 7595 this.resource.setValue(value); 7596 } 7597 return this; 7598 } 7599 7600 /** 7601 * @return {@link #response} (okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable.). This is the underlying object with id, value and extensions. The accessor "getResponse" gives direct access to the value 7602 */ 7603 public Enumeration<AssertionResponseTypes> getResponseElement() { 7604 if (this.response == null) 7605 if (Configuration.errorOnAutoCreate()) 7606 throw new Error("Attempt to auto-create SetupActionAssertComponent.response"); 7607 else if (Configuration.doAutoCreate()) 7608 this.response = new Enumeration<AssertionResponseTypes>(new AssertionResponseTypesEnumFactory()); // bb 7609 return this.response; 7610 } 7611 7612 public boolean hasResponseElement() { 7613 return this.response != null && !this.response.isEmpty(); 7614 } 7615 7616 public boolean hasResponse() { 7617 return this.response != null && !this.response.isEmpty(); 7618 } 7619 7620 /** 7621 * @param value {@link #response} (okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable.). This is the underlying object with id, value and extensions. The accessor "getResponse" gives direct access to the value 7622 */ 7623 public SetupActionAssertComponent setResponseElement(Enumeration<AssertionResponseTypes> value) { 7624 this.response = value; 7625 return this; 7626 } 7627 7628 /** 7629 * @return okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable. 7630 */ 7631 public AssertionResponseTypes getResponse() { 7632 return this.response == null ? null : this.response.getValue(); 7633 } 7634 7635 /** 7636 * @param value okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable. 7637 */ 7638 public SetupActionAssertComponent setResponse(AssertionResponseTypes value) { 7639 if (value == null) 7640 this.response = null; 7641 else { 7642 if (this.response == null) 7643 this.response = new Enumeration<AssertionResponseTypes>(new AssertionResponseTypesEnumFactory()); 7644 this.response.setValue(value); 7645 } 7646 return this; 7647 } 7648 7649 /** 7650 * @return {@link #responseCode} (The value of the HTTP response code to be tested.). This is the underlying object with id, value and extensions. The accessor "getResponseCode" gives direct access to the value 7651 */ 7652 public StringType getResponseCodeElement() { 7653 if (this.responseCode == null) 7654 if (Configuration.errorOnAutoCreate()) 7655 throw new Error("Attempt to auto-create SetupActionAssertComponent.responseCode"); 7656 else if (Configuration.doAutoCreate()) 7657 this.responseCode = new StringType(); // bb 7658 return this.responseCode; 7659 } 7660 7661 public boolean hasResponseCodeElement() { 7662 return this.responseCode != null && !this.responseCode.isEmpty(); 7663 } 7664 7665 public boolean hasResponseCode() { 7666 return this.responseCode != null && !this.responseCode.isEmpty(); 7667 } 7668 7669 /** 7670 * @param value {@link #responseCode} (The value of the HTTP response code to be tested.). This is the underlying object with id, value and extensions. The accessor "getResponseCode" gives direct access to the value 7671 */ 7672 public SetupActionAssertComponent setResponseCodeElement(StringType value) { 7673 this.responseCode = value; 7674 return this; 7675 } 7676 7677 /** 7678 * @return The value of the HTTP response code to be tested. 7679 */ 7680 public String getResponseCode() { 7681 return this.responseCode == null ? null : this.responseCode.getValue(); 7682 } 7683 7684 /** 7685 * @param value The value of the HTTP response code to be tested. 7686 */ 7687 public SetupActionAssertComponent setResponseCode(String value) { 7688 if (Utilities.noString(value)) 7689 this.responseCode = null; 7690 else { 7691 if (this.responseCode == null) 7692 this.responseCode = new StringType(); 7693 this.responseCode.setValue(value); 7694 } 7695 return this; 7696 } 7697 7698 /** 7699 * @return {@link #rule} (The TestScript.rule this assert will evaluate.) 7700 */ 7701 public ActionAssertRuleComponent getRule() { 7702 if (this.rule == null) 7703 if (Configuration.errorOnAutoCreate()) 7704 throw new Error("Attempt to auto-create SetupActionAssertComponent.rule"); 7705 else if (Configuration.doAutoCreate()) 7706 this.rule = new ActionAssertRuleComponent(); // cc 7707 return this.rule; 7708 } 7709 7710 public boolean hasRule() { 7711 return this.rule != null && !this.rule.isEmpty(); 7712 } 7713 7714 /** 7715 * @param value {@link #rule} (The TestScript.rule this assert will evaluate.) 7716 */ 7717 public SetupActionAssertComponent setRule(ActionAssertRuleComponent value) { 7718 this.rule = value; 7719 return this; 7720 } 7721 7722 /** 7723 * @return {@link #ruleset} (The TestScript.ruleset this assert will evaluate.) 7724 */ 7725 public ActionAssertRulesetComponent getRuleset() { 7726 if (this.ruleset == null) 7727 if (Configuration.errorOnAutoCreate()) 7728 throw new Error("Attempt to auto-create SetupActionAssertComponent.ruleset"); 7729 else if (Configuration.doAutoCreate()) 7730 this.ruleset = new ActionAssertRulesetComponent(); // cc 7731 return this.ruleset; 7732 } 7733 7734 public boolean hasRuleset() { 7735 return this.ruleset != null && !this.ruleset.isEmpty(); 7736 } 7737 7738 /** 7739 * @param value {@link #ruleset} (The TestScript.ruleset this assert will evaluate.) 7740 */ 7741 public SetupActionAssertComponent setRuleset(ActionAssertRulesetComponent value) { 7742 this.ruleset = value; 7743 return this; 7744 } 7745 7746 /** 7747 * @return {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression or the headerField against.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 7748 */ 7749 public IdType getSourceIdElement() { 7750 if (this.sourceId == null) 7751 if (Configuration.errorOnAutoCreate()) 7752 throw new Error("Attempt to auto-create SetupActionAssertComponent.sourceId"); 7753 else if (Configuration.doAutoCreate()) 7754 this.sourceId = new IdType(); // bb 7755 return this.sourceId; 7756 } 7757 7758 public boolean hasSourceIdElement() { 7759 return this.sourceId != null && !this.sourceId.isEmpty(); 7760 } 7761 7762 public boolean hasSourceId() { 7763 return this.sourceId != null && !this.sourceId.isEmpty(); 7764 } 7765 7766 /** 7767 * @param value {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression or the headerField against.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 7768 */ 7769 public SetupActionAssertComponent setSourceIdElement(IdType value) { 7770 this.sourceId = value; 7771 return this; 7772 } 7773 7774 /** 7775 * @return Fixture to evaluate the XPath/JSONPath expression or the headerField against. 7776 */ 7777 public String getSourceId() { 7778 return this.sourceId == null ? null : this.sourceId.getValue(); 7779 } 7780 7781 /** 7782 * @param value Fixture to evaluate the XPath/JSONPath expression or the headerField against. 7783 */ 7784 public SetupActionAssertComponent setSourceId(String value) { 7785 if (Utilities.noString(value)) 7786 this.sourceId = null; 7787 else { 7788 if (this.sourceId == null) 7789 this.sourceId = new IdType(); 7790 this.sourceId.setValue(value); 7791 } 7792 return this; 7793 } 7794 7795 /** 7796 * @return {@link #validateProfileId} (The ID of the Profile to validate against.). This is the underlying object with id, value and extensions. The accessor "getValidateProfileId" gives direct access to the value 7797 */ 7798 public IdType getValidateProfileIdElement() { 7799 if (this.validateProfileId == null) 7800 if (Configuration.errorOnAutoCreate()) 7801 throw new Error("Attempt to auto-create SetupActionAssertComponent.validateProfileId"); 7802 else if (Configuration.doAutoCreate()) 7803 this.validateProfileId = new IdType(); // bb 7804 return this.validateProfileId; 7805 } 7806 7807 public boolean hasValidateProfileIdElement() { 7808 return this.validateProfileId != null && !this.validateProfileId.isEmpty(); 7809 } 7810 7811 public boolean hasValidateProfileId() { 7812 return this.validateProfileId != null && !this.validateProfileId.isEmpty(); 7813 } 7814 7815 /** 7816 * @param value {@link #validateProfileId} (The ID of the Profile to validate against.). This is the underlying object with id, value and extensions. The accessor "getValidateProfileId" gives direct access to the value 7817 */ 7818 public SetupActionAssertComponent setValidateProfileIdElement(IdType value) { 7819 this.validateProfileId = value; 7820 return this; 7821 } 7822 7823 /** 7824 * @return The ID of the Profile to validate against. 7825 */ 7826 public String getValidateProfileId() { 7827 return this.validateProfileId == null ? null : this.validateProfileId.getValue(); 7828 } 7829 7830 /** 7831 * @param value The ID of the Profile to validate against. 7832 */ 7833 public SetupActionAssertComponent setValidateProfileId(String value) { 7834 if (Utilities.noString(value)) 7835 this.validateProfileId = null; 7836 else { 7837 if (this.validateProfileId == null) 7838 this.validateProfileId = new IdType(); 7839 this.validateProfileId.setValue(value); 7840 } 7841 return this; 7842 } 7843 7844 /** 7845 * @return {@link #value} (The value to compare to.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 7846 */ 7847 public StringType getValueElement() { 7848 if (this.value == null) 7849 if (Configuration.errorOnAutoCreate()) 7850 throw new Error("Attempt to auto-create SetupActionAssertComponent.value"); 7851 else if (Configuration.doAutoCreate()) 7852 this.value = new StringType(); // bb 7853 return this.value; 7854 } 7855 7856 public boolean hasValueElement() { 7857 return this.value != null && !this.value.isEmpty(); 7858 } 7859 7860 public boolean hasValue() { 7861 return this.value != null && !this.value.isEmpty(); 7862 } 7863 7864 /** 7865 * @param value {@link #value} (The value to compare to.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 7866 */ 7867 public SetupActionAssertComponent setValueElement(StringType value) { 7868 this.value = value; 7869 return this; 7870 } 7871 7872 /** 7873 * @return The value to compare to. 7874 */ 7875 public String getValue() { 7876 return this.value == null ? null : this.value.getValue(); 7877 } 7878 7879 /** 7880 * @param value The value to compare to. 7881 */ 7882 public SetupActionAssertComponent setValue(String value) { 7883 if (Utilities.noString(value)) 7884 this.value = null; 7885 else { 7886 if (this.value == null) 7887 this.value = new StringType(); 7888 this.value.setValue(value); 7889 } 7890 return this; 7891 } 7892 7893 /** 7894 * @return {@link #warningOnly} (Whether or not the test execution will produce a warning only on error for this assert.). This is the underlying object with id, value and extensions. The accessor "getWarningOnly" gives direct access to the value 7895 */ 7896 public BooleanType getWarningOnlyElement() { 7897 if (this.warningOnly == null) 7898 if (Configuration.errorOnAutoCreate()) 7899 throw new Error("Attempt to auto-create SetupActionAssertComponent.warningOnly"); 7900 else if (Configuration.doAutoCreate()) 7901 this.warningOnly = new BooleanType(); // bb 7902 return this.warningOnly; 7903 } 7904 7905 public boolean hasWarningOnlyElement() { 7906 return this.warningOnly != null && !this.warningOnly.isEmpty(); 7907 } 7908 7909 public boolean hasWarningOnly() { 7910 return this.warningOnly != null && !this.warningOnly.isEmpty(); 7911 } 7912 7913 /** 7914 * @param value {@link #warningOnly} (Whether or not the test execution will produce a warning only on error for this assert.). This is the underlying object with id, value and extensions. The accessor "getWarningOnly" gives direct access to the value 7915 */ 7916 public SetupActionAssertComponent setWarningOnlyElement(BooleanType value) { 7917 this.warningOnly = value; 7918 return this; 7919 } 7920 7921 /** 7922 * @return Whether or not the test execution will produce a warning only on error for this assert. 7923 */ 7924 public boolean getWarningOnly() { 7925 return this.warningOnly == null || this.warningOnly.isEmpty() ? false : this.warningOnly.getValue(); 7926 } 7927 7928 /** 7929 * @param value Whether or not the test execution will produce a warning only on error for this assert. 7930 */ 7931 public SetupActionAssertComponent setWarningOnly(boolean value) { 7932 if (this.warningOnly == null) 7933 this.warningOnly = new BooleanType(); 7934 this.warningOnly.setValue(value); 7935 return this; 7936 } 7937 7938 protected void listChildren(List<Property> children) { 7939 super.listChildren(children); 7940 children.add(new Property("label", "string", "The label would be used for tracking/logging purposes by test engines.", 0, 1, label)); 7941 children.add(new Property("description", "string", "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description)); 7942 children.add(new Property("direction", "code", "The direction to use for the assertion.", 0, 1, direction)); 7943 children.add(new Property("compareToSourceId", "string", "Id of the source fixture used as the contents to be evaluated by either the \"source/expression\" or \"sourceId/path\" definition.", 0, 1, compareToSourceId)); 7944 children.add(new Property("compareToSourceExpression", "string", "The fluentpath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 0, 1, compareToSourceExpression)); 7945 children.add(new Property("compareToSourcePath", "string", "XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 0, 1, compareToSourcePath)); 7946 children.add(new Property("contentType", "code", "The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.", 0, 1, contentType)); 7947 children.add(new Property("expression", "string", "The fluentpath expression to be evaluated against the request or response message contents - HTTP headers and payload.", 0, 1, expression)); 7948 children.add(new Property("headerField", "string", "The HTTP header field name e.g. 'Location'.", 0, 1, headerField)); 7949 children.add(new Property("minimumId", "string", "The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.", 0, 1, minimumId)); 7950 children.add(new Property("navigationLinks", "boolean", "Whether or not the test execution performs validation on the bundle navigation links.", 0, 1, navigationLinks)); 7951 children.add(new Property("operator", "code", "The operator type defines the conditional behavior of the assert. If not defined, the default is equals.", 0, 1, operator)); 7952 children.add(new Property("path", "string", "The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.", 0, 1, path)); 7953 children.add(new Property("requestMethod", "code", "The request method or HTTP operation code to compare against that used by the client system under test.", 0, 1, requestMethod)); 7954 children.add(new Property("requestURL", "string", "The value to use in a comparison against the request URL path string.", 0, 1, requestURL)); 7955 children.add(new Property("resource", "code", "The type of the resource. See http://build.fhir.org/resourcelist.html.", 0, 1, resource)); 7956 children.add(new Property("response", "code", "okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable.", 0, 1, response)); 7957 children.add(new Property("responseCode", "string", "The value of the HTTP response code to be tested.", 0, 1, responseCode)); 7958 children.add(new Property("rule", "", "The TestScript.rule this assert will evaluate.", 0, 1, rule)); 7959 children.add(new Property("ruleset", "", "The TestScript.ruleset this assert will evaluate.", 0, 1, ruleset)); 7960 children.add(new Property("sourceId", "id", "Fixture to evaluate the XPath/JSONPath expression or the headerField against.", 0, 1, sourceId)); 7961 children.add(new Property("validateProfileId", "id", "The ID of the Profile to validate against.", 0, 1, validateProfileId)); 7962 children.add(new Property("value", "string", "The value to compare to.", 0, 1, value)); 7963 children.add(new Property("warningOnly", "boolean", "Whether or not the test execution will produce a warning only on error for this assert.", 0, 1, warningOnly)); 7964 } 7965 7966 @Override 7967 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7968 switch (_hash) { 7969 case 102727412: /*label*/ return new Property("label", "string", "The label would be used for tracking/logging purposes by test engines.", 0, 1, label); 7970 case -1724546052: /*description*/ return new Property("description", "string", "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description); 7971 case -962590849: /*direction*/ return new Property("direction", "code", "The direction to use for the assertion.", 0, 1, direction); 7972 case 2081856758: /*compareToSourceId*/ return new Property("compareToSourceId", "string", "Id of the source fixture used as the contents to be evaluated by either the \"source/expression\" or \"sourceId/path\" definition.", 0, 1, compareToSourceId); 7973 case -1415702669: /*compareToSourceExpression*/ return new Property("compareToSourceExpression", "string", "The fluentpath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 0, 1, compareToSourceExpression); 7974 case -790206144: /*compareToSourcePath*/ return new Property("compareToSourcePath", "string", "XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 0, 1, compareToSourcePath); 7975 case -389131437: /*contentType*/ return new Property("contentType", "code", "The content-type or mime-type to use for RESTful operation in the 'Content-Type' header.", 0, 1, contentType); 7976 case -1795452264: /*expression*/ return new Property("expression", "string", "The fluentpath expression to be evaluated against the request or response message contents - HTTP headers and payload.", 0, 1, expression); 7977 case 1160732269: /*headerField*/ return new Property("headerField", "string", "The HTTP header field name e.g. 'Location'.", 0, 1, headerField); 7978 case 818925001: /*minimumId*/ return new Property("minimumId", "string", "The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.", 0, 1, minimumId); 7979 case 1001488901: /*navigationLinks*/ return new Property("navigationLinks", "boolean", "Whether or not the test execution performs validation on the bundle navigation links.", 0, 1, navigationLinks); 7980 case -500553564: /*operator*/ return new Property("operator", "code", "The operator type defines the conditional behavior of the assert. If not defined, the default is equals.", 0, 1, operator); 7981 case 3433509: /*path*/ return new Property("path", "string", "The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.", 0, 1, path); 7982 case 1217874000: /*requestMethod*/ return new Property("requestMethod", "code", "The request method or HTTP operation code to compare against that used by the client system under test.", 0, 1, requestMethod); 7983 case 37099616: /*requestURL*/ return new Property("requestURL", "string", "The value to use in a comparison against the request URL path string.", 0, 1, requestURL); 7984 case -341064690: /*resource*/ return new Property("resource", "code", "The type of the resource. See http://build.fhir.org/resourcelist.html.", 0, 1, resource); 7985 case -340323263: /*response*/ return new Property("response", "code", "okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable.", 0, 1, response); 7986 case 1438723534: /*responseCode*/ return new Property("responseCode", "string", "The value of the HTTP response code to be tested.", 0, 1, responseCode); 7987 case 3512060: /*rule*/ return new Property("rule", "", "The TestScript.rule this assert will evaluate.", 0, 1, rule); 7988 case 1548678118: /*ruleset*/ return new Property("ruleset", "", "The TestScript.ruleset this assert will evaluate.", 0, 1, ruleset); 7989 case 1746327190: /*sourceId*/ return new Property("sourceId", "id", "Fixture to evaluate the XPath/JSONPath expression or the headerField against.", 0, 1, sourceId); 7990 case 1555541038: /*validateProfileId*/ return new Property("validateProfileId", "id", "The ID of the Profile to validate against.", 0, 1, validateProfileId); 7991 case 111972721: /*value*/ return new Property("value", "string", "The value to compare to.", 0, 1, value); 7992 case -481159832: /*warningOnly*/ return new Property("warningOnly", "boolean", "Whether or not the test execution will produce a warning only on error for this assert.", 0, 1, warningOnly); 7993 default: return super.getNamedProperty(_hash, _name, _checkValid); 7994 } 7995 7996 } 7997 7998 @Override 7999 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8000 switch (hash) { 8001 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 8002 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 8003 case -962590849: /*direction*/ return this.direction == null ? new Base[0] : new Base[] {this.direction}; // Enumeration<AssertionDirectionType> 8004 case 2081856758: /*compareToSourceId*/ return this.compareToSourceId == null ? new Base[0] : new Base[] {this.compareToSourceId}; // StringType 8005 case -1415702669: /*compareToSourceExpression*/ return this.compareToSourceExpression == null ? new Base[0] : new Base[] {this.compareToSourceExpression}; // StringType 8006 case -790206144: /*compareToSourcePath*/ return this.compareToSourcePath == null ? new Base[0] : new Base[] {this.compareToSourcePath}; // StringType 8007 case -389131437: /*contentType*/ return this.contentType == null ? new Base[0] : new Base[] {this.contentType}; // Enumeration<ContentType> 8008 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 8009 case 1160732269: /*headerField*/ return this.headerField == null ? new Base[0] : new Base[] {this.headerField}; // StringType 8010 case 818925001: /*minimumId*/ return this.minimumId == null ? new Base[0] : new Base[] {this.minimumId}; // StringType 8011 case 1001488901: /*navigationLinks*/ return this.navigationLinks == null ? new Base[0] : new Base[] {this.navigationLinks}; // BooleanType 8012 case -500553564: /*operator*/ return this.operator == null ? new Base[0] : new Base[] {this.operator}; // Enumeration<AssertionOperatorType> 8013 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 8014 case 1217874000: /*requestMethod*/ return this.requestMethod == null ? new Base[0] : new Base[] {this.requestMethod}; // Enumeration<TestScriptRequestMethodCode> 8015 case 37099616: /*requestURL*/ return this.requestURL == null ? new Base[0] : new Base[] {this.requestURL}; // StringType 8016 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // CodeType 8017 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // Enumeration<AssertionResponseTypes> 8018 case 1438723534: /*responseCode*/ return this.responseCode == null ? new Base[0] : new Base[] {this.responseCode}; // StringType 8019 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : new Base[] {this.rule}; // ActionAssertRuleComponent 8020 case 1548678118: /*ruleset*/ return this.ruleset == null ? new Base[0] : new Base[] {this.ruleset}; // ActionAssertRulesetComponent 8021 case 1746327190: /*sourceId*/ return this.sourceId == null ? new Base[0] : new Base[] {this.sourceId}; // IdType 8022 case 1555541038: /*validateProfileId*/ return this.validateProfileId == null ? new Base[0] : new Base[] {this.validateProfileId}; // IdType 8023 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 8024 case -481159832: /*warningOnly*/ return this.warningOnly == null ? new Base[0] : new Base[] {this.warningOnly}; // BooleanType 8025 default: return super.getProperty(hash, name, checkValid); 8026 } 8027 8028 } 8029 8030 @Override 8031 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8032 switch (hash) { 8033 case 102727412: // label 8034 this.label = castToString(value); // StringType 8035 return value; 8036 case -1724546052: // description 8037 this.description = castToString(value); // StringType 8038 return value; 8039 case -962590849: // direction 8040 value = new AssertionDirectionTypeEnumFactory().fromType(castToCode(value)); 8041 this.direction = (Enumeration) value; // Enumeration<AssertionDirectionType> 8042 return value; 8043 case 2081856758: // compareToSourceId 8044 this.compareToSourceId = castToString(value); // StringType 8045 return value; 8046 case -1415702669: // compareToSourceExpression 8047 this.compareToSourceExpression = castToString(value); // StringType 8048 return value; 8049 case -790206144: // compareToSourcePath 8050 this.compareToSourcePath = castToString(value); // StringType 8051 return value; 8052 case -389131437: // contentType 8053 value = new ContentTypeEnumFactory().fromType(castToCode(value)); 8054 this.contentType = (Enumeration) value; // Enumeration<ContentType> 8055 return value; 8056 case -1795452264: // expression 8057 this.expression = castToString(value); // StringType 8058 return value; 8059 case 1160732269: // headerField 8060 this.headerField = castToString(value); // StringType 8061 return value; 8062 case 818925001: // minimumId 8063 this.minimumId = castToString(value); // StringType 8064 return value; 8065 case 1001488901: // navigationLinks 8066 this.navigationLinks = castToBoolean(value); // BooleanType 8067 return value; 8068 case -500553564: // operator 8069 value = new AssertionOperatorTypeEnumFactory().fromType(castToCode(value)); 8070 this.operator = (Enumeration) value; // Enumeration<AssertionOperatorType> 8071 return value; 8072 case 3433509: // path 8073 this.path = castToString(value); // StringType 8074 return value; 8075 case 1217874000: // requestMethod 8076 value = new TestScriptRequestMethodCodeEnumFactory().fromType(castToCode(value)); 8077 this.requestMethod = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 8078 return value; 8079 case 37099616: // requestURL 8080 this.requestURL = castToString(value); // StringType 8081 return value; 8082 case -341064690: // resource 8083 this.resource = castToCode(value); // CodeType 8084 return value; 8085 case -340323263: // response 8086 value = new AssertionResponseTypesEnumFactory().fromType(castToCode(value)); 8087 this.response = (Enumeration) value; // Enumeration<AssertionResponseTypes> 8088 return value; 8089 case 1438723534: // responseCode 8090 this.responseCode = castToString(value); // StringType 8091 return value; 8092 case 3512060: // rule 8093 this.rule = (ActionAssertRuleComponent) value; // ActionAssertRuleComponent 8094 return value; 8095 case 1548678118: // ruleset 8096 this.ruleset = (ActionAssertRulesetComponent) value; // ActionAssertRulesetComponent 8097 return value; 8098 case 1746327190: // sourceId 8099 this.sourceId = castToId(value); // IdType 8100 return value; 8101 case 1555541038: // validateProfileId 8102 this.validateProfileId = castToId(value); // IdType 8103 return value; 8104 case 111972721: // value 8105 this.value = castToString(value); // StringType 8106 return value; 8107 case -481159832: // warningOnly 8108 this.warningOnly = castToBoolean(value); // BooleanType 8109 return value; 8110 default: return super.setProperty(hash, name, value); 8111 } 8112 8113 } 8114 8115 @Override 8116 public Base setProperty(String name, Base value) throws FHIRException { 8117 if (name.equals("label")) { 8118 this.label = castToString(value); // StringType 8119 } else if (name.equals("description")) { 8120 this.description = castToString(value); // StringType 8121 } else if (name.equals("direction")) { 8122 value = new AssertionDirectionTypeEnumFactory().fromType(castToCode(value)); 8123 this.direction = (Enumeration) value; // Enumeration<AssertionDirectionType> 8124 } else if (name.equals("compareToSourceId")) { 8125 this.compareToSourceId = castToString(value); // StringType 8126 } else if (name.equals("compareToSourceExpression")) { 8127 this.compareToSourceExpression = castToString(value); // StringType 8128 } else if (name.equals("compareToSourcePath")) { 8129 this.compareToSourcePath = castToString(value); // StringType 8130 } else if (name.equals("contentType")) { 8131 value = new ContentTypeEnumFactory().fromType(castToCode(value)); 8132 this.contentType = (Enumeration) value; // Enumeration<ContentType> 8133 } else if (name.equals("expression")) { 8134 this.expression = castToString(value); // StringType 8135 } else if (name.equals("headerField")) { 8136 this.headerField = castToString(value); // StringType 8137 } else if (name.equals("minimumId")) { 8138 this.minimumId = castToString(value); // StringType 8139 } else if (name.equals("navigationLinks")) { 8140 this.navigationLinks = castToBoolean(value); // BooleanType 8141 } else if (name.equals("operator")) { 8142 value = new AssertionOperatorTypeEnumFactory().fromType(castToCode(value)); 8143 this.operator = (Enumeration) value; // Enumeration<AssertionOperatorType> 8144 } else if (name.equals("path")) { 8145 this.path = castToString(value); // StringType 8146 } else if (name.equals("requestMethod")) { 8147 value = new TestScriptRequestMethodCodeEnumFactory().fromType(castToCode(value)); 8148 this.requestMethod = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 8149 } else if (name.equals("requestURL")) { 8150 this.requestURL = castToString(value); // StringType 8151 } else if (name.equals("resource")) { 8152 this.resource = castToCode(value); // CodeType 8153 } else if (name.equals("response")) { 8154 value = new AssertionResponseTypesEnumFactory().fromType(castToCode(value)); 8155 this.response = (Enumeration) value; // Enumeration<AssertionResponseTypes> 8156 } else if (name.equals("responseCode")) { 8157 this.responseCode = castToString(value); // StringType 8158 } else if (name.equals("rule")) { 8159 this.rule = (ActionAssertRuleComponent) value; // ActionAssertRuleComponent 8160 } else if (name.equals("ruleset")) { 8161 this.ruleset = (ActionAssertRulesetComponent) value; // ActionAssertRulesetComponent 8162 } else if (name.equals("sourceId")) { 8163 this.sourceId = castToId(value); // IdType 8164 } else if (name.equals("validateProfileId")) { 8165 this.validateProfileId = castToId(value); // IdType 8166 } else if (name.equals("value")) { 8167 this.value = castToString(value); // StringType 8168 } else if (name.equals("warningOnly")) { 8169 this.warningOnly = castToBoolean(value); // BooleanType 8170 } else 8171 return super.setProperty(name, value); 8172 return value; 8173 } 8174 8175 @Override 8176 public Base makeProperty(int hash, String name) throws FHIRException { 8177 switch (hash) { 8178 case 102727412: return getLabelElement(); 8179 case -1724546052: return getDescriptionElement(); 8180 case -962590849: return getDirectionElement(); 8181 case 2081856758: return getCompareToSourceIdElement(); 8182 case -1415702669: return getCompareToSourceExpressionElement(); 8183 case -790206144: return getCompareToSourcePathElement(); 8184 case -389131437: return getContentTypeElement(); 8185 case -1795452264: return getExpressionElement(); 8186 case 1160732269: return getHeaderFieldElement(); 8187 case 818925001: return getMinimumIdElement(); 8188 case 1001488901: return getNavigationLinksElement(); 8189 case -500553564: return getOperatorElement(); 8190 case 3433509: return getPathElement(); 8191 case 1217874000: return getRequestMethodElement(); 8192 case 37099616: return getRequestURLElement(); 8193 case -341064690: return getResourceElement(); 8194 case -340323263: return getResponseElement(); 8195 case 1438723534: return getResponseCodeElement(); 8196 case 3512060: return getRule(); 8197 case 1548678118: return getRuleset(); 8198 case 1746327190: return getSourceIdElement(); 8199 case 1555541038: return getValidateProfileIdElement(); 8200 case 111972721: return getValueElement(); 8201 case -481159832: return getWarningOnlyElement(); 8202 default: return super.makeProperty(hash, name); 8203 } 8204 8205 } 8206 8207 @Override 8208 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8209 switch (hash) { 8210 case 102727412: /*label*/ return new String[] {"string"}; 8211 case -1724546052: /*description*/ return new String[] {"string"}; 8212 case -962590849: /*direction*/ return new String[] {"code"}; 8213 case 2081856758: /*compareToSourceId*/ return new String[] {"string"}; 8214 case -1415702669: /*compareToSourceExpression*/ return new String[] {"string"}; 8215 case -790206144: /*compareToSourcePath*/ return new String[] {"string"}; 8216 case -389131437: /*contentType*/ return new String[] {"code"}; 8217 case -1795452264: /*expression*/ return new String[] {"string"}; 8218 case 1160732269: /*headerField*/ return new String[] {"string"}; 8219 case 818925001: /*minimumId*/ return new String[] {"string"}; 8220 case 1001488901: /*navigationLinks*/ return new String[] {"boolean"}; 8221 case -500553564: /*operator*/ return new String[] {"code"}; 8222 case 3433509: /*path*/ return new String[] {"string"}; 8223 case 1217874000: /*requestMethod*/ return new String[] {"code"}; 8224 case 37099616: /*requestURL*/ return new String[] {"string"}; 8225 case -341064690: /*resource*/ return new String[] {"code"}; 8226 case -340323263: /*response*/ return new String[] {"code"}; 8227 case 1438723534: /*responseCode*/ return new String[] {"string"}; 8228 case 3512060: /*rule*/ return new String[] {}; 8229 case 1548678118: /*ruleset*/ return new String[] {}; 8230 case 1746327190: /*sourceId*/ return new String[] {"id"}; 8231 case 1555541038: /*validateProfileId*/ return new String[] {"id"}; 8232 case 111972721: /*value*/ return new String[] {"string"}; 8233 case -481159832: /*warningOnly*/ return new String[] {"boolean"}; 8234 default: return super.getTypesForProperty(hash, name); 8235 } 8236 8237 } 8238 8239 @Override 8240 public Base addChild(String name) throws FHIRException { 8241 if (name.equals("label")) { 8242 throw new FHIRException("Cannot call addChild on a singleton property TestScript.label"); 8243 } 8244 else if (name.equals("description")) { 8245 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 8246 } 8247 else if (name.equals("direction")) { 8248 throw new FHIRException("Cannot call addChild on a singleton property TestScript.direction"); 8249 } 8250 else if (name.equals("compareToSourceId")) { 8251 throw new FHIRException("Cannot call addChild on a singleton property TestScript.compareToSourceId"); 8252 } 8253 else if (name.equals("compareToSourceExpression")) { 8254 throw new FHIRException("Cannot call addChild on a singleton property TestScript.compareToSourceExpression"); 8255 } 8256 else if (name.equals("compareToSourcePath")) { 8257 throw new FHIRException("Cannot call addChild on a singleton property TestScript.compareToSourcePath"); 8258 } 8259 else if (name.equals("contentType")) { 8260 throw new FHIRException("Cannot call addChild on a singleton property TestScript.contentType"); 8261 } 8262 else if (name.equals("expression")) { 8263 throw new FHIRException("Cannot call addChild on a singleton property TestScript.expression"); 8264 } 8265 else if (name.equals("headerField")) { 8266 throw new FHIRException("Cannot call addChild on a singleton property TestScript.headerField"); 8267 } 8268 else if (name.equals("minimumId")) { 8269 throw new FHIRException("Cannot call addChild on a singleton property TestScript.minimumId"); 8270 } 8271 else if (name.equals("navigationLinks")) { 8272 throw new FHIRException("Cannot call addChild on a singleton property TestScript.navigationLinks"); 8273 } 8274 else if (name.equals("operator")) { 8275 throw new FHIRException("Cannot call addChild on a singleton property TestScript.operator"); 8276 } 8277 else if (name.equals("path")) { 8278 throw new FHIRException("Cannot call addChild on a singleton property TestScript.path"); 8279 } 8280 else if (name.equals("requestMethod")) { 8281 throw new FHIRException("Cannot call addChild on a singleton property TestScript.requestMethod"); 8282 } 8283 else if (name.equals("requestURL")) { 8284 throw new FHIRException("Cannot call addChild on a singleton property TestScript.requestURL"); 8285 } 8286 else if (name.equals("resource")) { 8287 throw new FHIRException("Cannot call addChild on a singleton property TestScript.resource"); 8288 } 8289 else if (name.equals("response")) { 8290 throw new FHIRException("Cannot call addChild on a singleton property TestScript.response"); 8291 } 8292 else if (name.equals("responseCode")) { 8293 throw new FHIRException("Cannot call addChild on a singleton property TestScript.responseCode"); 8294 } 8295 else if (name.equals("rule")) { 8296 this.rule = new ActionAssertRuleComponent(); 8297 return this.rule; 8298 } 8299 else if (name.equals("ruleset")) { 8300 this.ruleset = new ActionAssertRulesetComponent(); 8301 return this.ruleset; 8302 } 8303 else if (name.equals("sourceId")) { 8304 throw new FHIRException("Cannot call addChild on a singleton property TestScript.sourceId"); 8305 } 8306 else if (name.equals("validateProfileId")) { 8307 throw new FHIRException("Cannot call addChild on a singleton property TestScript.validateProfileId"); 8308 } 8309 else if (name.equals("value")) { 8310 throw new FHIRException("Cannot call addChild on a singleton property TestScript.value"); 8311 } 8312 else if (name.equals("warningOnly")) { 8313 throw new FHIRException("Cannot call addChild on a singleton property TestScript.warningOnly"); 8314 } 8315 else 8316 return super.addChild(name); 8317 } 8318 8319 public SetupActionAssertComponent copy() { 8320 SetupActionAssertComponent dst = new SetupActionAssertComponent(); 8321 copyValues(dst); 8322 dst.label = label == null ? null : label.copy(); 8323 dst.description = description == null ? null : description.copy(); 8324 dst.direction = direction == null ? null : direction.copy(); 8325 dst.compareToSourceId = compareToSourceId == null ? null : compareToSourceId.copy(); 8326 dst.compareToSourceExpression = compareToSourceExpression == null ? null : compareToSourceExpression.copy(); 8327 dst.compareToSourcePath = compareToSourcePath == null ? null : compareToSourcePath.copy(); 8328 dst.contentType = contentType == null ? null : contentType.copy(); 8329 dst.expression = expression == null ? null : expression.copy(); 8330 dst.headerField = headerField == null ? null : headerField.copy(); 8331 dst.minimumId = minimumId == null ? null : minimumId.copy(); 8332 dst.navigationLinks = navigationLinks == null ? null : navigationLinks.copy(); 8333 dst.operator = operator == null ? null : operator.copy(); 8334 dst.path = path == null ? null : path.copy(); 8335 dst.requestMethod = requestMethod == null ? null : requestMethod.copy(); 8336 dst.requestURL = requestURL == null ? null : requestURL.copy(); 8337 dst.resource = resource == null ? null : resource.copy(); 8338 dst.response = response == null ? null : response.copy(); 8339 dst.responseCode = responseCode == null ? null : responseCode.copy(); 8340 dst.rule = rule == null ? null : rule.copy(); 8341 dst.ruleset = ruleset == null ? null : ruleset.copy(); 8342 dst.sourceId = sourceId == null ? null : sourceId.copy(); 8343 dst.validateProfileId = validateProfileId == null ? null : validateProfileId.copy(); 8344 dst.value = value == null ? null : value.copy(); 8345 dst.warningOnly = warningOnly == null ? null : warningOnly.copy(); 8346 return dst; 8347 } 8348 8349 @Override 8350 public boolean equalsDeep(Base other_) { 8351 if (!super.equalsDeep(other_)) 8352 return false; 8353 if (!(other_ instanceof SetupActionAssertComponent)) 8354 return false; 8355 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 8356 return compareDeep(label, o.label, true) && compareDeep(description, o.description, true) && compareDeep(direction, o.direction, true) 8357 && compareDeep(compareToSourceId, o.compareToSourceId, true) && compareDeep(compareToSourceExpression, o.compareToSourceExpression, true) 8358 && compareDeep(compareToSourcePath, o.compareToSourcePath, true) && compareDeep(contentType, o.contentType, true) 8359 && compareDeep(expression, o.expression, true) && compareDeep(headerField, o.headerField, true) 8360 && compareDeep(minimumId, o.minimumId, true) && compareDeep(navigationLinks, o.navigationLinks, true) 8361 && compareDeep(operator, o.operator, true) && compareDeep(path, o.path, true) && compareDeep(requestMethod, o.requestMethod, true) 8362 && compareDeep(requestURL, o.requestURL, true) && compareDeep(resource, o.resource, true) && compareDeep(response, o.response, true) 8363 && compareDeep(responseCode, o.responseCode, true) && compareDeep(rule, o.rule, true) && compareDeep(ruleset, o.ruleset, true) 8364 && compareDeep(sourceId, o.sourceId, true) && compareDeep(validateProfileId, o.validateProfileId, true) 8365 && compareDeep(value, o.value, true) && compareDeep(warningOnly, o.warningOnly, true); 8366 } 8367 8368 @Override 8369 public boolean equalsShallow(Base other_) { 8370 if (!super.equalsShallow(other_)) 8371 return false; 8372 if (!(other_ instanceof SetupActionAssertComponent)) 8373 return false; 8374 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 8375 return compareValues(label, o.label, true) && compareValues(description, o.description, true) && compareValues(direction, o.direction, true) 8376 && compareValues(compareToSourceId, o.compareToSourceId, true) && compareValues(compareToSourceExpression, o.compareToSourceExpression, true) 8377 && compareValues(compareToSourcePath, o.compareToSourcePath, true) && compareValues(contentType, o.contentType, true) 8378 && compareValues(expression, o.expression, true) && compareValues(headerField, o.headerField, true) 8379 && compareValues(minimumId, o.minimumId, true) && compareValues(navigationLinks, o.navigationLinks, true) 8380 && compareValues(operator, o.operator, true) && compareValues(path, o.path, true) && compareValues(requestMethod, o.requestMethod, true) 8381 && compareValues(requestURL, o.requestURL, true) && compareValues(resource, o.resource, true) && compareValues(response, o.response, true) 8382 && compareValues(responseCode, o.responseCode, true) && compareValues(sourceId, o.sourceId, true) && compareValues(validateProfileId, o.validateProfileId, true) 8383 && compareValues(value, o.value, true) && compareValues(warningOnly, o.warningOnly, true); 8384 } 8385 8386 public boolean isEmpty() { 8387 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, description, direction 8388 , compareToSourceId, compareToSourceExpression, compareToSourcePath, contentType, expression 8389 , headerField, minimumId, navigationLinks, operator, path, requestMethod, requestURL 8390 , resource, response, responseCode, rule, ruleset, sourceId, validateProfileId 8391 , value, warningOnly); 8392 } 8393 8394 public String fhirType() { 8395 return "TestScript.setup.action.assert"; 8396 8397 } 8398 8399 } 8400 8401 @Block() 8402 public static class ActionAssertRuleComponent extends BackboneElement implements IBaseBackboneElement { 8403 /** 8404 * The TestScript.rule id value this assert will evaluate. 8405 */ 8406 @Child(name = "ruleId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 8407 @Description(shortDefinition="Id of the TestScript.rule", formalDefinition="The TestScript.rule id value this assert will evaluate." ) 8408 protected IdType ruleId; 8409 8410 /** 8411 * Each rule template can take one or more parameters for rule evaluation. 8412 */ 8413 @Child(name = "param", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8414 @Description(shortDefinition="Rule parameter template", formalDefinition="Each rule template can take one or more parameters for rule evaluation." ) 8415 protected List<ActionAssertRuleParamComponent> param; 8416 8417 private static final long serialVersionUID = -1860715431L; 8418 8419 /** 8420 * Constructor 8421 */ 8422 public ActionAssertRuleComponent() { 8423 super(); 8424 } 8425 8426 /** 8427 * Constructor 8428 */ 8429 public ActionAssertRuleComponent(IdType ruleId) { 8430 super(); 8431 this.ruleId = ruleId; 8432 } 8433 8434 /** 8435 * @return {@link #ruleId} (The TestScript.rule id value this assert will evaluate.). This is the underlying object with id, value and extensions. The accessor "getRuleId" gives direct access to the value 8436 */ 8437 public IdType getRuleIdElement() { 8438 if (this.ruleId == null) 8439 if (Configuration.errorOnAutoCreate()) 8440 throw new Error("Attempt to auto-create ActionAssertRuleComponent.ruleId"); 8441 else if (Configuration.doAutoCreate()) 8442 this.ruleId = new IdType(); // bb 8443 return this.ruleId; 8444 } 8445 8446 public boolean hasRuleIdElement() { 8447 return this.ruleId != null && !this.ruleId.isEmpty(); 8448 } 8449 8450 public boolean hasRuleId() { 8451 return this.ruleId != null && !this.ruleId.isEmpty(); 8452 } 8453 8454 /** 8455 * @param value {@link #ruleId} (The TestScript.rule id value this assert will evaluate.). This is the underlying object with id, value and extensions. The accessor "getRuleId" gives direct access to the value 8456 */ 8457 public ActionAssertRuleComponent setRuleIdElement(IdType value) { 8458 this.ruleId = value; 8459 return this; 8460 } 8461 8462 /** 8463 * @return The TestScript.rule id value this assert will evaluate. 8464 */ 8465 public String getRuleId() { 8466 return this.ruleId == null ? null : this.ruleId.getValue(); 8467 } 8468 8469 /** 8470 * @param value The TestScript.rule id value this assert will evaluate. 8471 */ 8472 public ActionAssertRuleComponent setRuleId(String value) { 8473 if (this.ruleId == null) 8474 this.ruleId = new IdType(); 8475 this.ruleId.setValue(value); 8476 return this; 8477 } 8478 8479 /** 8480 * @return {@link #param} (Each rule template can take one or more parameters for rule evaluation.) 8481 */ 8482 public List<ActionAssertRuleParamComponent> getParam() { 8483 if (this.param == null) 8484 this.param = new ArrayList<ActionAssertRuleParamComponent>(); 8485 return this.param; 8486 } 8487 8488 /** 8489 * @return Returns a reference to <code>this</code> for easy method chaining 8490 */ 8491 public ActionAssertRuleComponent setParam(List<ActionAssertRuleParamComponent> theParam) { 8492 this.param = theParam; 8493 return this; 8494 } 8495 8496 public boolean hasParam() { 8497 if (this.param == null) 8498 return false; 8499 for (ActionAssertRuleParamComponent item : this.param) 8500 if (!item.isEmpty()) 8501 return true; 8502 return false; 8503 } 8504 8505 public ActionAssertRuleParamComponent addParam() { //3 8506 ActionAssertRuleParamComponent t = new ActionAssertRuleParamComponent(); 8507 if (this.param == null) 8508 this.param = new ArrayList<ActionAssertRuleParamComponent>(); 8509 this.param.add(t); 8510 return t; 8511 } 8512 8513 public ActionAssertRuleComponent addParam(ActionAssertRuleParamComponent t) { //3 8514 if (t == null) 8515 return this; 8516 if (this.param == null) 8517 this.param = new ArrayList<ActionAssertRuleParamComponent>(); 8518 this.param.add(t); 8519 return this; 8520 } 8521 8522 /** 8523 * @return The first repetition of repeating field {@link #param}, creating it if it does not already exist 8524 */ 8525 public ActionAssertRuleParamComponent getParamFirstRep() { 8526 if (getParam().isEmpty()) { 8527 addParam(); 8528 } 8529 return getParam().get(0); 8530 } 8531 8532 protected void listChildren(List<Property> children) { 8533 super.listChildren(children); 8534 children.add(new Property("ruleId", "id", "The TestScript.rule id value this assert will evaluate.", 0, 1, ruleId)); 8535 children.add(new Property("param", "", "Each rule template can take one or more parameters for rule evaluation.", 0, java.lang.Integer.MAX_VALUE, param)); 8536 } 8537 8538 @Override 8539 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8540 switch (_hash) { 8541 case -919875273: /*ruleId*/ return new Property("ruleId", "id", "The TestScript.rule id value this assert will evaluate.", 0, 1, ruleId); 8542 case 106436749: /*param*/ return new Property("param", "", "Each rule template can take one or more parameters for rule evaluation.", 0, java.lang.Integer.MAX_VALUE, param); 8543 default: return super.getNamedProperty(_hash, _name, _checkValid); 8544 } 8545 8546 } 8547 8548 @Override 8549 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8550 switch (hash) { 8551 case -919875273: /*ruleId*/ return this.ruleId == null ? new Base[0] : new Base[] {this.ruleId}; // IdType 8552 case 106436749: /*param*/ return this.param == null ? new Base[0] : this.param.toArray(new Base[this.param.size()]); // ActionAssertRuleParamComponent 8553 default: return super.getProperty(hash, name, checkValid); 8554 } 8555 8556 } 8557 8558 @Override 8559 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8560 switch (hash) { 8561 case -919875273: // ruleId 8562 this.ruleId = castToId(value); // IdType 8563 return value; 8564 case 106436749: // param 8565 this.getParam().add((ActionAssertRuleParamComponent) value); // ActionAssertRuleParamComponent 8566 return value; 8567 default: return super.setProperty(hash, name, value); 8568 } 8569 8570 } 8571 8572 @Override 8573 public Base setProperty(String name, Base value) throws FHIRException { 8574 if (name.equals("ruleId")) { 8575 this.ruleId = castToId(value); // IdType 8576 } else if (name.equals("param")) { 8577 this.getParam().add((ActionAssertRuleParamComponent) value); 8578 } else 8579 return super.setProperty(name, value); 8580 return value; 8581 } 8582 8583 @Override 8584 public Base makeProperty(int hash, String name) throws FHIRException { 8585 switch (hash) { 8586 case -919875273: return getRuleIdElement(); 8587 case 106436749: return addParam(); 8588 default: return super.makeProperty(hash, name); 8589 } 8590 8591 } 8592 8593 @Override 8594 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8595 switch (hash) { 8596 case -919875273: /*ruleId*/ return new String[] {"id"}; 8597 case 106436749: /*param*/ return new String[] {}; 8598 default: return super.getTypesForProperty(hash, name); 8599 } 8600 8601 } 8602 8603 @Override 8604 public Base addChild(String name) throws FHIRException { 8605 if (name.equals("ruleId")) { 8606 throw new FHIRException("Cannot call addChild on a singleton property TestScript.ruleId"); 8607 } 8608 else if (name.equals("param")) { 8609 return addParam(); 8610 } 8611 else 8612 return super.addChild(name); 8613 } 8614 8615 public ActionAssertRuleComponent copy() { 8616 ActionAssertRuleComponent dst = new ActionAssertRuleComponent(); 8617 copyValues(dst); 8618 dst.ruleId = ruleId == null ? null : ruleId.copy(); 8619 if (param != null) { 8620 dst.param = new ArrayList<ActionAssertRuleParamComponent>(); 8621 for (ActionAssertRuleParamComponent i : param) 8622 dst.param.add(i.copy()); 8623 }; 8624 return dst; 8625 } 8626 8627 @Override 8628 public boolean equalsDeep(Base other_) { 8629 if (!super.equalsDeep(other_)) 8630 return false; 8631 if (!(other_ instanceof ActionAssertRuleComponent)) 8632 return false; 8633 ActionAssertRuleComponent o = (ActionAssertRuleComponent) other_; 8634 return compareDeep(ruleId, o.ruleId, true) && compareDeep(param, o.param, true); 8635 } 8636 8637 @Override 8638 public boolean equalsShallow(Base other_) { 8639 if (!super.equalsShallow(other_)) 8640 return false; 8641 if (!(other_ instanceof ActionAssertRuleComponent)) 8642 return false; 8643 ActionAssertRuleComponent o = (ActionAssertRuleComponent) other_; 8644 return compareValues(ruleId, o.ruleId, true); 8645 } 8646 8647 public boolean isEmpty() { 8648 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(ruleId, param); 8649 } 8650 8651 public String fhirType() { 8652 return "TestScript.setup.action.assert.rule"; 8653 8654 } 8655 8656 } 8657 8658 @Block() 8659 public static class ActionAssertRuleParamComponent extends BackboneElement implements IBaseBackboneElement { 8660 /** 8661 * Descriptive name for this parameter that matches the external assert rule parameter name. 8662 */ 8663 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 8664 @Description(shortDefinition="Parameter name matching external assert rule parameter", formalDefinition="Descriptive name for this parameter that matches the external assert rule parameter name." ) 8665 protected StringType name; 8666 8667 /** 8668 * The value for the parameter that will be passed on to the external rule template. 8669 */ 8670 @Child(name = "value", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 8671 @Description(shortDefinition="Parameter value defined either explicitly or dynamically", formalDefinition="The value for the parameter that will be passed on to the external rule template." ) 8672 protected StringType value; 8673 8674 private static final long serialVersionUID = 395259392L; 8675 8676 /** 8677 * Constructor 8678 */ 8679 public ActionAssertRuleParamComponent() { 8680 super(); 8681 } 8682 8683 /** 8684 * Constructor 8685 */ 8686 public ActionAssertRuleParamComponent(StringType name, StringType value) { 8687 super(); 8688 this.name = name; 8689 this.value = value; 8690 } 8691 8692 /** 8693 * @return {@link #name} (Descriptive name for this parameter that matches the external assert rule parameter name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 8694 */ 8695 public StringType getNameElement() { 8696 if (this.name == null) 8697 if (Configuration.errorOnAutoCreate()) 8698 throw new Error("Attempt to auto-create ActionAssertRuleParamComponent.name"); 8699 else if (Configuration.doAutoCreate()) 8700 this.name = new StringType(); // bb 8701 return this.name; 8702 } 8703 8704 public boolean hasNameElement() { 8705 return this.name != null && !this.name.isEmpty(); 8706 } 8707 8708 public boolean hasName() { 8709 return this.name != null && !this.name.isEmpty(); 8710 } 8711 8712 /** 8713 * @param value {@link #name} (Descriptive name for this parameter that matches the external assert rule parameter name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 8714 */ 8715 public ActionAssertRuleParamComponent setNameElement(StringType value) { 8716 this.name = value; 8717 return this; 8718 } 8719 8720 /** 8721 * @return Descriptive name for this parameter that matches the external assert rule parameter name. 8722 */ 8723 public String getName() { 8724 return this.name == null ? null : this.name.getValue(); 8725 } 8726 8727 /** 8728 * @param value Descriptive name for this parameter that matches the external assert rule parameter name. 8729 */ 8730 public ActionAssertRuleParamComponent setName(String value) { 8731 if (this.name == null) 8732 this.name = new StringType(); 8733 this.name.setValue(value); 8734 return this; 8735 } 8736 8737 /** 8738 * @return {@link #value} (The value for the parameter that will be passed on to the external rule template.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 8739 */ 8740 public StringType getValueElement() { 8741 if (this.value == null) 8742 if (Configuration.errorOnAutoCreate()) 8743 throw new Error("Attempt to auto-create ActionAssertRuleParamComponent.value"); 8744 else if (Configuration.doAutoCreate()) 8745 this.value = new StringType(); // bb 8746 return this.value; 8747 } 8748 8749 public boolean hasValueElement() { 8750 return this.value != null && !this.value.isEmpty(); 8751 } 8752 8753 public boolean hasValue() { 8754 return this.value != null && !this.value.isEmpty(); 8755 } 8756 8757 /** 8758 * @param value {@link #value} (The value for the parameter that will be passed on to the external rule template.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 8759 */ 8760 public ActionAssertRuleParamComponent setValueElement(StringType value) { 8761 this.value = value; 8762 return this; 8763 } 8764 8765 /** 8766 * @return The value for the parameter that will be passed on to the external rule template. 8767 */ 8768 public String getValue() { 8769 return this.value == null ? null : this.value.getValue(); 8770 } 8771 8772 /** 8773 * @param value The value for the parameter that will be passed on to the external rule template. 8774 */ 8775 public ActionAssertRuleParamComponent setValue(String value) { 8776 if (this.value == null) 8777 this.value = new StringType(); 8778 this.value.setValue(value); 8779 return this; 8780 } 8781 8782 protected void listChildren(List<Property> children) { 8783 super.listChildren(children); 8784 children.add(new Property("name", "string", "Descriptive name for this parameter that matches the external assert rule parameter name.", 0, 1, name)); 8785 children.add(new Property("value", "string", "The value for the parameter that will be passed on to the external rule template.", 0, 1, value)); 8786 } 8787 8788 @Override 8789 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8790 switch (_hash) { 8791 case 3373707: /*name*/ return new Property("name", "string", "Descriptive name for this parameter that matches the external assert rule parameter name.", 0, 1, name); 8792 case 111972721: /*value*/ return new Property("value", "string", "The value for the parameter that will be passed on to the external rule template.", 0, 1, value); 8793 default: return super.getNamedProperty(_hash, _name, _checkValid); 8794 } 8795 8796 } 8797 8798 @Override 8799 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8800 switch (hash) { 8801 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 8802 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 8803 default: return super.getProperty(hash, name, checkValid); 8804 } 8805 8806 } 8807 8808 @Override 8809 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8810 switch (hash) { 8811 case 3373707: // name 8812 this.name = castToString(value); // StringType 8813 return value; 8814 case 111972721: // value 8815 this.value = castToString(value); // StringType 8816 return value; 8817 default: return super.setProperty(hash, name, value); 8818 } 8819 8820 } 8821 8822 @Override 8823 public Base setProperty(String name, Base value) throws FHIRException { 8824 if (name.equals("name")) { 8825 this.name = castToString(value); // StringType 8826 } else if (name.equals("value")) { 8827 this.value = castToString(value); // StringType 8828 } else 8829 return super.setProperty(name, value); 8830 return value; 8831 } 8832 8833 @Override 8834 public Base makeProperty(int hash, String name) throws FHIRException { 8835 switch (hash) { 8836 case 3373707: return getNameElement(); 8837 case 111972721: return getValueElement(); 8838 default: return super.makeProperty(hash, name); 8839 } 8840 8841 } 8842 8843 @Override 8844 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8845 switch (hash) { 8846 case 3373707: /*name*/ return new String[] {"string"}; 8847 case 111972721: /*value*/ return new String[] {"string"}; 8848 default: return super.getTypesForProperty(hash, name); 8849 } 8850 8851 } 8852 8853 @Override 8854 public Base addChild(String name) throws FHIRException { 8855 if (name.equals("name")) { 8856 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 8857 } 8858 else if (name.equals("value")) { 8859 throw new FHIRException("Cannot call addChild on a singleton property TestScript.value"); 8860 } 8861 else 8862 return super.addChild(name); 8863 } 8864 8865 public ActionAssertRuleParamComponent copy() { 8866 ActionAssertRuleParamComponent dst = new ActionAssertRuleParamComponent(); 8867 copyValues(dst); 8868 dst.name = name == null ? null : name.copy(); 8869 dst.value = value == null ? null : value.copy(); 8870 return dst; 8871 } 8872 8873 @Override 8874 public boolean equalsDeep(Base other_) { 8875 if (!super.equalsDeep(other_)) 8876 return false; 8877 if (!(other_ instanceof ActionAssertRuleParamComponent)) 8878 return false; 8879 ActionAssertRuleParamComponent o = (ActionAssertRuleParamComponent) other_; 8880 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 8881 } 8882 8883 @Override 8884 public boolean equalsShallow(Base other_) { 8885 if (!super.equalsShallow(other_)) 8886 return false; 8887 if (!(other_ instanceof ActionAssertRuleParamComponent)) 8888 return false; 8889 ActionAssertRuleParamComponent o = (ActionAssertRuleParamComponent) other_; 8890 return compareValues(name, o.name, true) && compareValues(value, o.value, true); 8891 } 8892 8893 public boolean isEmpty() { 8894 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 8895 } 8896 8897 public String fhirType() { 8898 return "TestScript.setup.action.assert.rule.param"; 8899 8900 } 8901 8902 } 8903 8904 @Block() 8905 public static class ActionAssertRulesetComponent extends BackboneElement implements IBaseBackboneElement { 8906 /** 8907 * The TestScript.ruleset id value this assert will evaluate. 8908 */ 8909 @Child(name = "rulesetId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 8910 @Description(shortDefinition="Id of the TestScript.ruleset", formalDefinition="The TestScript.ruleset id value this assert will evaluate." ) 8911 protected IdType rulesetId; 8912 8913 /** 8914 * The referenced rule within the external ruleset template. 8915 */ 8916 @Child(name = "rule", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8917 @Description(shortDefinition="The referenced rule within the ruleset", formalDefinition="The referenced rule within the external ruleset template." ) 8918 protected List<ActionAssertRulesetRuleComponent> rule; 8919 8920 private static final long serialVersionUID = -976736025L; 8921 8922 /** 8923 * Constructor 8924 */ 8925 public ActionAssertRulesetComponent() { 8926 super(); 8927 } 8928 8929 /** 8930 * Constructor 8931 */ 8932 public ActionAssertRulesetComponent(IdType rulesetId) { 8933 super(); 8934 this.rulesetId = rulesetId; 8935 } 8936 8937 /** 8938 * @return {@link #rulesetId} (The TestScript.ruleset id value this assert will evaluate.). This is the underlying object with id, value and extensions. The accessor "getRulesetId" gives direct access to the value 8939 */ 8940 public IdType getRulesetIdElement() { 8941 if (this.rulesetId == null) 8942 if (Configuration.errorOnAutoCreate()) 8943 throw new Error("Attempt to auto-create ActionAssertRulesetComponent.rulesetId"); 8944 else if (Configuration.doAutoCreate()) 8945 this.rulesetId = new IdType(); // bb 8946 return this.rulesetId; 8947 } 8948 8949 public boolean hasRulesetIdElement() { 8950 return this.rulesetId != null && !this.rulesetId.isEmpty(); 8951 } 8952 8953 public boolean hasRulesetId() { 8954 return this.rulesetId != null && !this.rulesetId.isEmpty(); 8955 } 8956 8957 /** 8958 * @param value {@link #rulesetId} (The TestScript.ruleset id value this assert will evaluate.). This is the underlying object with id, value and extensions. The accessor "getRulesetId" gives direct access to the value 8959 */ 8960 public ActionAssertRulesetComponent setRulesetIdElement(IdType value) { 8961 this.rulesetId = value; 8962 return this; 8963 } 8964 8965 /** 8966 * @return The TestScript.ruleset id value this assert will evaluate. 8967 */ 8968 public String getRulesetId() { 8969 return this.rulesetId == null ? null : this.rulesetId.getValue(); 8970 } 8971 8972 /** 8973 * @param value The TestScript.ruleset id value this assert will evaluate. 8974 */ 8975 public ActionAssertRulesetComponent setRulesetId(String value) { 8976 if (this.rulesetId == null) 8977 this.rulesetId = new IdType(); 8978 this.rulesetId.setValue(value); 8979 return this; 8980 } 8981 8982 /** 8983 * @return {@link #rule} (The referenced rule within the external ruleset template.) 8984 */ 8985 public List<ActionAssertRulesetRuleComponent> getRule() { 8986 if (this.rule == null) 8987 this.rule = new ArrayList<ActionAssertRulesetRuleComponent>(); 8988 return this.rule; 8989 } 8990 8991 /** 8992 * @return Returns a reference to <code>this</code> for easy method chaining 8993 */ 8994 public ActionAssertRulesetComponent setRule(List<ActionAssertRulesetRuleComponent> theRule) { 8995 this.rule = theRule; 8996 return this; 8997 } 8998 8999 public boolean hasRule() { 9000 if (this.rule == null) 9001 return false; 9002 for (ActionAssertRulesetRuleComponent item : this.rule) 9003 if (!item.isEmpty()) 9004 return true; 9005 return false; 9006 } 9007 9008 public ActionAssertRulesetRuleComponent addRule() { //3 9009 ActionAssertRulesetRuleComponent t = new ActionAssertRulesetRuleComponent(); 9010 if (this.rule == null) 9011 this.rule = new ArrayList<ActionAssertRulesetRuleComponent>(); 9012 this.rule.add(t); 9013 return t; 9014 } 9015 9016 public ActionAssertRulesetComponent addRule(ActionAssertRulesetRuleComponent t) { //3 9017 if (t == null) 9018 return this; 9019 if (this.rule == null) 9020 this.rule = new ArrayList<ActionAssertRulesetRuleComponent>(); 9021 this.rule.add(t); 9022 return this; 9023 } 9024 9025 /** 9026 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist 9027 */ 9028 public ActionAssertRulesetRuleComponent getRuleFirstRep() { 9029 if (getRule().isEmpty()) { 9030 addRule(); 9031 } 9032 return getRule().get(0); 9033 } 9034 9035 protected void listChildren(List<Property> children) { 9036 super.listChildren(children); 9037 children.add(new Property("rulesetId", "id", "The TestScript.ruleset id value this assert will evaluate.", 0, 1, rulesetId)); 9038 children.add(new Property("rule", "", "The referenced rule within the external ruleset template.", 0, java.lang.Integer.MAX_VALUE, rule)); 9039 } 9040 9041 @Override 9042 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9043 switch (_hash) { 9044 case -2073977951: /*rulesetId*/ return new Property("rulesetId", "id", "The TestScript.ruleset id value this assert will evaluate.", 0, 1, rulesetId); 9045 case 3512060: /*rule*/ return new Property("rule", "", "The referenced rule within the external ruleset template.", 0, java.lang.Integer.MAX_VALUE, rule); 9046 default: return super.getNamedProperty(_hash, _name, _checkValid); 9047 } 9048 9049 } 9050 9051 @Override 9052 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9053 switch (hash) { 9054 case -2073977951: /*rulesetId*/ return this.rulesetId == null ? new Base[0] : new Base[] {this.rulesetId}; // IdType 9055 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // ActionAssertRulesetRuleComponent 9056 default: return super.getProperty(hash, name, checkValid); 9057 } 9058 9059 } 9060 9061 @Override 9062 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9063 switch (hash) { 9064 case -2073977951: // rulesetId 9065 this.rulesetId = castToId(value); // IdType 9066 return value; 9067 case 3512060: // rule 9068 this.getRule().add((ActionAssertRulesetRuleComponent) value); // ActionAssertRulesetRuleComponent 9069 return value; 9070 default: return super.setProperty(hash, name, value); 9071 } 9072 9073 } 9074 9075 @Override 9076 public Base setProperty(String name, Base value) throws FHIRException { 9077 if (name.equals("rulesetId")) { 9078 this.rulesetId = castToId(value); // IdType 9079 } else if (name.equals("rule")) { 9080 this.getRule().add((ActionAssertRulesetRuleComponent) value); 9081 } else 9082 return super.setProperty(name, value); 9083 return value; 9084 } 9085 9086 @Override 9087 public Base makeProperty(int hash, String name) throws FHIRException { 9088 switch (hash) { 9089 case -2073977951: return getRulesetIdElement(); 9090 case 3512060: return addRule(); 9091 default: return super.makeProperty(hash, name); 9092 } 9093 9094 } 9095 9096 @Override 9097 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9098 switch (hash) { 9099 case -2073977951: /*rulesetId*/ return new String[] {"id"}; 9100 case 3512060: /*rule*/ return new String[] {}; 9101 default: return super.getTypesForProperty(hash, name); 9102 } 9103 9104 } 9105 9106 @Override 9107 public Base addChild(String name) throws FHIRException { 9108 if (name.equals("rulesetId")) { 9109 throw new FHIRException("Cannot call addChild on a singleton property TestScript.rulesetId"); 9110 } 9111 else if (name.equals("rule")) { 9112 return addRule(); 9113 } 9114 else 9115 return super.addChild(name); 9116 } 9117 9118 public ActionAssertRulesetComponent copy() { 9119 ActionAssertRulesetComponent dst = new ActionAssertRulesetComponent(); 9120 copyValues(dst); 9121 dst.rulesetId = rulesetId == null ? null : rulesetId.copy(); 9122 if (rule != null) { 9123 dst.rule = new ArrayList<ActionAssertRulesetRuleComponent>(); 9124 for (ActionAssertRulesetRuleComponent i : rule) 9125 dst.rule.add(i.copy()); 9126 }; 9127 return dst; 9128 } 9129 9130 @Override 9131 public boolean equalsDeep(Base other_) { 9132 if (!super.equalsDeep(other_)) 9133 return false; 9134 if (!(other_ instanceof ActionAssertRulesetComponent)) 9135 return false; 9136 ActionAssertRulesetComponent o = (ActionAssertRulesetComponent) other_; 9137 return compareDeep(rulesetId, o.rulesetId, true) && compareDeep(rule, o.rule, true); 9138 } 9139 9140 @Override 9141 public boolean equalsShallow(Base other_) { 9142 if (!super.equalsShallow(other_)) 9143 return false; 9144 if (!(other_ instanceof ActionAssertRulesetComponent)) 9145 return false; 9146 ActionAssertRulesetComponent o = (ActionAssertRulesetComponent) other_; 9147 return compareValues(rulesetId, o.rulesetId, true); 9148 } 9149 9150 public boolean isEmpty() { 9151 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(rulesetId, rule); 9152 } 9153 9154 public String fhirType() { 9155 return "TestScript.setup.action.assert.ruleset"; 9156 9157 } 9158 9159 } 9160 9161 @Block() 9162 public static class ActionAssertRulesetRuleComponent extends BackboneElement implements IBaseBackboneElement { 9163 /** 9164 * Id of the referenced rule within the external ruleset template. 9165 */ 9166 @Child(name = "ruleId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 9167 @Description(shortDefinition="Id of referenced rule within the ruleset", formalDefinition="Id of the referenced rule within the external ruleset template." ) 9168 protected IdType ruleId; 9169 9170 /** 9171 * Each rule template can take one or more parameters for rule evaluation. 9172 */ 9173 @Child(name = "param", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9174 @Description(shortDefinition="Rule parameter template", formalDefinition="Each rule template can take one or more parameters for rule evaluation." ) 9175 protected List<ActionAssertRulesetRuleParamComponent> param; 9176 9177 private static final long serialVersionUID = -1850698529L; 9178 9179 /** 9180 * Constructor 9181 */ 9182 public ActionAssertRulesetRuleComponent() { 9183 super(); 9184 } 9185 9186 /** 9187 * Constructor 9188 */ 9189 public ActionAssertRulesetRuleComponent(IdType ruleId) { 9190 super(); 9191 this.ruleId = ruleId; 9192 } 9193 9194 /** 9195 * @return {@link #ruleId} (Id of the referenced rule within the external ruleset template.). This is the underlying object with id, value and extensions. The accessor "getRuleId" gives direct access to the value 9196 */ 9197 public IdType getRuleIdElement() { 9198 if (this.ruleId == null) 9199 if (Configuration.errorOnAutoCreate()) 9200 throw new Error("Attempt to auto-create ActionAssertRulesetRuleComponent.ruleId"); 9201 else if (Configuration.doAutoCreate()) 9202 this.ruleId = new IdType(); // bb 9203 return this.ruleId; 9204 } 9205 9206 public boolean hasRuleIdElement() { 9207 return this.ruleId != null && !this.ruleId.isEmpty(); 9208 } 9209 9210 public boolean hasRuleId() { 9211 return this.ruleId != null && !this.ruleId.isEmpty(); 9212 } 9213 9214 /** 9215 * @param value {@link #ruleId} (Id of the referenced rule within the external ruleset template.). This is the underlying object with id, value and extensions. The accessor "getRuleId" gives direct access to the value 9216 */ 9217 public ActionAssertRulesetRuleComponent setRuleIdElement(IdType value) { 9218 this.ruleId = value; 9219 return this; 9220 } 9221 9222 /** 9223 * @return Id of the referenced rule within the external ruleset template. 9224 */ 9225 public String getRuleId() { 9226 return this.ruleId == null ? null : this.ruleId.getValue(); 9227 } 9228 9229 /** 9230 * @param value Id of the referenced rule within the external ruleset template. 9231 */ 9232 public ActionAssertRulesetRuleComponent setRuleId(String value) { 9233 if (this.ruleId == null) 9234 this.ruleId = new IdType(); 9235 this.ruleId.setValue(value); 9236 return this; 9237 } 9238 9239 /** 9240 * @return {@link #param} (Each rule template can take one or more parameters for rule evaluation.) 9241 */ 9242 public List<ActionAssertRulesetRuleParamComponent> getParam() { 9243 if (this.param == null) 9244 this.param = new ArrayList<ActionAssertRulesetRuleParamComponent>(); 9245 return this.param; 9246 } 9247 9248 /** 9249 * @return Returns a reference to <code>this</code> for easy method chaining 9250 */ 9251 public ActionAssertRulesetRuleComponent setParam(List<ActionAssertRulesetRuleParamComponent> theParam) { 9252 this.param = theParam; 9253 return this; 9254 } 9255 9256 public boolean hasParam() { 9257 if (this.param == null) 9258 return false; 9259 for (ActionAssertRulesetRuleParamComponent item : this.param) 9260 if (!item.isEmpty()) 9261 return true; 9262 return false; 9263 } 9264 9265 public ActionAssertRulesetRuleParamComponent addParam() { //3 9266 ActionAssertRulesetRuleParamComponent t = new ActionAssertRulesetRuleParamComponent(); 9267 if (this.param == null) 9268 this.param = new ArrayList<ActionAssertRulesetRuleParamComponent>(); 9269 this.param.add(t); 9270 return t; 9271 } 9272 9273 public ActionAssertRulesetRuleComponent addParam(ActionAssertRulesetRuleParamComponent t) { //3 9274 if (t == null) 9275 return this; 9276 if (this.param == null) 9277 this.param = new ArrayList<ActionAssertRulesetRuleParamComponent>(); 9278 this.param.add(t); 9279 return this; 9280 } 9281 9282 /** 9283 * @return The first repetition of repeating field {@link #param}, creating it if it does not already exist 9284 */ 9285 public ActionAssertRulesetRuleParamComponent getParamFirstRep() { 9286 if (getParam().isEmpty()) { 9287 addParam(); 9288 } 9289 return getParam().get(0); 9290 } 9291 9292 protected void listChildren(List<Property> children) { 9293 super.listChildren(children); 9294 children.add(new Property("ruleId", "id", "Id of the referenced rule within the external ruleset template.", 0, 1, ruleId)); 9295 children.add(new Property("param", "", "Each rule template can take one or more parameters for rule evaluation.", 0, java.lang.Integer.MAX_VALUE, param)); 9296 } 9297 9298 @Override 9299 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9300 switch (_hash) { 9301 case -919875273: /*ruleId*/ return new Property("ruleId", "id", "Id of the referenced rule within the external ruleset template.", 0, 1, ruleId); 9302 case 106436749: /*param*/ return new Property("param", "", "Each rule template can take one or more parameters for rule evaluation.", 0, java.lang.Integer.MAX_VALUE, param); 9303 default: return super.getNamedProperty(_hash, _name, _checkValid); 9304 } 9305 9306 } 9307 9308 @Override 9309 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9310 switch (hash) { 9311 case -919875273: /*ruleId*/ return this.ruleId == null ? new Base[0] : new Base[] {this.ruleId}; // IdType 9312 case 106436749: /*param*/ return this.param == null ? new Base[0] : this.param.toArray(new Base[this.param.size()]); // ActionAssertRulesetRuleParamComponent 9313 default: return super.getProperty(hash, name, checkValid); 9314 } 9315 9316 } 9317 9318 @Override 9319 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9320 switch (hash) { 9321 case -919875273: // ruleId 9322 this.ruleId = castToId(value); // IdType 9323 return value; 9324 case 106436749: // param 9325 this.getParam().add((ActionAssertRulesetRuleParamComponent) value); // ActionAssertRulesetRuleParamComponent 9326 return value; 9327 default: return super.setProperty(hash, name, value); 9328 } 9329 9330 } 9331 9332 @Override 9333 public Base setProperty(String name, Base value) throws FHIRException { 9334 if (name.equals("ruleId")) { 9335 this.ruleId = castToId(value); // IdType 9336 } else if (name.equals("param")) { 9337 this.getParam().add((ActionAssertRulesetRuleParamComponent) value); 9338 } else 9339 return super.setProperty(name, value); 9340 return value; 9341 } 9342 9343 @Override 9344 public Base makeProperty(int hash, String name) throws FHIRException { 9345 switch (hash) { 9346 case -919875273: return getRuleIdElement(); 9347 case 106436749: return addParam(); 9348 default: return super.makeProperty(hash, name); 9349 } 9350 9351 } 9352 9353 @Override 9354 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9355 switch (hash) { 9356 case -919875273: /*ruleId*/ return new String[] {"id"}; 9357 case 106436749: /*param*/ return new String[] {}; 9358 default: return super.getTypesForProperty(hash, name); 9359 } 9360 9361 } 9362 9363 @Override 9364 public Base addChild(String name) throws FHIRException { 9365 if (name.equals("ruleId")) { 9366 throw new FHIRException("Cannot call addChild on a singleton property TestScript.ruleId"); 9367 } 9368 else if (name.equals("param")) { 9369 return addParam(); 9370 } 9371 else 9372 return super.addChild(name); 9373 } 9374 9375 public ActionAssertRulesetRuleComponent copy() { 9376 ActionAssertRulesetRuleComponent dst = new ActionAssertRulesetRuleComponent(); 9377 copyValues(dst); 9378 dst.ruleId = ruleId == null ? null : ruleId.copy(); 9379 if (param != null) { 9380 dst.param = new ArrayList<ActionAssertRulesetRuleParamComponent>(); 9381 for (ActionAssertRulesetRuleParamComponent i : param) 9382 dst.param.add(i.copy()); 9383 }; 9384 return dst; 9385 } 9386 9387 @Override 9388 public boolean equalsDeep(Base other_) { 9389 if (!super.equalsDeep(other_)) 9390 return false; 9391 if (!(other_ instanceof ActionAssertRulesetRuleComponent)) 9392 return false; 9393 ActionAssertRulesetRuleComponent o = (ActionAssertRulesetRuleComponent) other_; 9394 return compareDeep(ruleId, o.ruleId, true) && compareDeep(param, o.param, true); 9395 } 9396 9397 @Override 9398 public boolean equalsShallow(Base other_) { 9399 if (!super.equalsShallow(other_)) 9400 return false; 9401 if (!(other_ instanceof ActionAssertRulesetRuleComponent)) 9402 return false; 9403 ActionAssertRulesetRuleComponent o = (ActionAssertRulesetRuleComponent) other_; 9404 return compareValues(ruleId, o.ruleId, true); 9405 } 9406 9407 public boolean isEmpty() { 9408 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(ruleId, param); 9409 } 9410 9411 public String fhirType() { 9412 return "TestScript.setup.action.assert.ruleset.rule"; 9413 9414 } 9415 9416 } 9417 9418 @Block() 9419 public static class ActionAssertRulesetRuleParamComponent extends BackboneElement implements IBaseBackboneElement { 9420 /** 9421 * Descriptive name for this parameter that matches the external assert ruleset rule parameter name. 9422 */ 9423 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 9424 @Description(shortDefinition="Parameter name matching external assert ruleset rule parameter", formalDefinition="Descriptive name for this parameter that matches the external assert ruleset rule parameter name." ) 9425 protected StringType name; 9426 9427 /** 9428 * The value for the parameter that will be passed on to the external ruleset rule template. 9429 */ 9430 @Child(name = "value", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 9431 @Description(shortDefinition="Parameter value defined either explicitly or dynamically", formalDefinition="The value for the parameter that will be passed on to the external ruleset rule template." ) 9432 protected StringType value; 9433 9434 private static final long serialVersionUID = 395259392L; 9435 9436 /** 9437 * Constructor 9438 */ 9439 public ActionAssertRulesetRuleParamComponent() { 9440 super(); 9441 } 9442 9443 /** 9444 * Constructor 9445 */ 9446 public ActionAssertRulesetRuleParamComponent(StringType name, StringType value) { 9447 super(); 9448 this.name = name; 9449 this.value = value; 9450 } 9451 9452 /** 9453 * @return {@link #name} (Descriptive name for this parameter that matches the external assert ruleset rule parameter name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 9454 */ 9455 public StringType getNameElement() { 9456 if (this.name == null) 9457 if (Configuration.errorOnAutoCreate()) 9458 throw new Error("Attempt to auto-create ActionAssertRulesetRuleParamComponent.name"); 9459 else if (Configuration.doAutoCreate()) 9460 this.name = new StringType(); // bb 9461 return this.name; 9462 } 9463 9464 public boolean hasNameElement() { 9465 return this.name != null && !this.name.isEmpty(); 9466 } 9467 9468 public boolean hasName() { 9469 return this.name != null && !this.name.isEmpty(); 9470 } 9471 9472 /** 9473 * @param value {@link #name} (Descriptive name for this parameter that matches the external assert ruleset rule parameter name.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 9474 */ 9475 public ActionAssertRulesetRuleParamComponent setNameElement(StringType value) { 9476 this.name = value; 9477 return this; 9478 } 9479 9480 /** 9481 * @return Descriptive name for this parameter that matches the external assert ruleset rule parameter name. 9482 */ 9483 public String getName() { 9484 return this.name == null ? null : this.name.getValue(); 9485 } 9486 9487 /** 9488 * @param value Descriptive name for this parameter that matches the external assert ruleset rule parameter name. 9489 */ 9490 public ActionAssertRulesetRuleParamComponent setName(String value) { 9491 if (this.name == null) 9492 this.name = new StringType(); 9493 this.name.setValue(value); 9494 return this; 9495 } 9496 9497 /** 9498 * @return {@link #value} (The value for the parameter that will be passed on to the external ruleset rule template.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 9499 */ 9500 public StringType getValueElement() { 9501 if (this.value == null) 9502 if (Configuration.errorOnAutoCreate()) 9503 throw new Error("Attempt to auto-create ActionAssertRulesetRuleParamComponent.value"); 9504 else if (Configuration.doAutoCreate()) 9505 this.value = new StringType(); // bb 9506 return this.value; 9507 } 9508 9509 public boolean hasValueElement() { 9510 return this.value != null && !this.value.isEmpty(); 9511 } 9512 9513 public boolean hasValue() { 9514 return this.value != null && !this.value.isEmpty(); 9515 } 9516 9517 /** 9518 * @param value {@link #value} (The value for the parameter that will be passed on to the external ruleset rule template.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 9519 */ 9520 public ActionAssertRulesetRuleParamComponent setValueElement(StringType value) { 9521 this.value = value; 9522 return this; 9523 } 9524 9525 /** 9526 * @return The value for the parameter that will be passed on to the external ruleset rule template. 9527 */ 9528 public String getValue() { 9529 return this.value == null ? null : this.value.getValue(); 9530 } 9531 9532 /** 9533 * @param value The value for the parameter that will be passed on to the external ruleset rule template. 9534 */ 9535 public ActionAssertRulesetRuleParamComponent setValue(String value) { 9536 if (this.value == null) 9537 this.value = new StringType(); 9538 this.value.setValue(value); 9539 return this; 9540 } 9541 9542 protected void listChildren(List<Property> children) { 9543 super.listChildren(children); 9544 children.add(new Property("name", "string", "Descriptive name for this parameter that matches the external assert ruleset rule parameter name.", 0, 1, name)); 9545 children.add(new Property("value", "string", "The value for the parameter that will be passed on to the external ruleset rule template.", 0, 1, value)); 9546 } 9547 9548 @Override 9549 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9550 switch (_hash) { 9551 case 3373707: /*name*/ return new Property("name", "string", "Descriptive name for this parameter that matches the external assert ruleset rule parameter name.", 0, 1, name); 9552 case 111972721: /*value*/ return new Property("value", "string", "The value for the parameter that will be passed on to the external ruleset rule template.", 0, 1, value); 9553 default: return super.getNamedProperty(_hash, _name, _checkValid); 9554 } 9555 9556 } 9557 9558 @Override 9559 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9560 switch (hash) { 9561 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 9562 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 9563 default: return super.getProperty(hash, name, checkValid); 9564 } 9565 9566 } 9567 9568 @Override 9569 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9570 switch (hash) { 9571 case 3373707: // name 9572 this.name = castToString(value); // StringType 9573 return value; 9574 case 111972721: // value 9575 this.value = castToString(value); // StringType 9576 return value; 9577 default: return super.setProperty(hash, name, value); 9578 } 9579 9580 } 9581 9582 @Override 9583 public Base setProperty(String name, Base value) throws FHIRException { 9584 if (name.equals("name")) { 9585 this.name = castToString(value); // StringType 9586 } else if (name.equals("value")) { 9587 this.value = castToString(value); // StringType 9588 } else 9589 return super.setProperty(name, value); 9590 return value; 9591 } 9592 9593 @Override 9594 public Base makeProperty(int hash, String name) throws FHIRException { 9595 switch (hash) { 9596 case 3373707: return getNameElement(); 9597 case 111972721: return getValueElement(); 9598 default: return super.makeProperty(hash, name); 9599 } 9600 9601 } 9602 9603 @Override 9604 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9605 switch (hash) { 9606 case 3373707: /*name*/ return new String[] {"string"}; 9607 case 111972721: /*value*/ return new String[] {"string"}; 9608 default: return super.getTypesForProperty(hash, name); 9609 } 9610 9611 } 9612 9613 @Override 9614 public Base addChild(String name) throws FHIRException { 9615 if (name.equals("name")) { 9616 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 9617 } 9618 else if (name.equals("value")) { 9619 throw new FHIRException("Cannot call addChild on a singleton property TestScript.value"); 9620 } 9621 else 9622 return super.addChild(name); 9623 } 9624 9625 public ActionAssertRulesetRuleParamComponent copy() { 9626 ActionAssertRulesetRuleParamComponent dst = new ActionAssertRulesetRuleParamComponent(); 9627 copyValues(dst); 9628 dst.name = name == null ? null : name.copy(); 9629 dst.value = value == null ? null : value.copy(); 9630 return dst; 9631 } 9632 9633 @Override 9634 public boolean equalsDeep(Base other_) { 9635 if (!super.equalsDeep(other_)) 9636 return false; 9637 if (!(other_ instanceof ActionAssertRulesetRuleParamComponent)) 9638 return false; 9639 ActionAssertRulesetRuleParamComponent o = (ActionAssertRulesetRuleParamComponent) other_; 9640 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 9641 } 9642 9643 @Override 9644 public boolean equalsShallow(Base other_) { 9645 if (!super.equalsShallow(other_)) 9646 return false; 9647 if (!(other_ instanceof ActionAssertRulesetRuleParamComponent)) 9648 return false; 9649 ActionAssertRulesetRuleParamComponent o = (ActionAssertRulesetRuleParamComponent) other_; 9650 return compareValues(name, o.name, true) && compareValues(value, o.value, true); 9651 } 9652 9653 public boolean isEmpty() { 9654 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 9655 } 9656 9657 public String fhirType() { 9658 return "TestScript.setup.action.assert.ruleset.rule.param"; 9659 9660 } 9661 9662 } 9663 9664 @Block() 9665 public static class TestScriptTestComponent extends BackboneElement implements IBaseBackboneElement { 9666 /** 9667 * The name of this test used for tracking/logging purposes by test engines. 9668 */ 9669 @Child(name = "name", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 9670 @Description(shortDefinition="Tracking/logging name of this test", formalDefinition="The name of this test used for tracking/logging purposes by test engines." ) 9671 protected StringType name; 9672 9673 /** 9674 * A short description of the test used by test engines for tracking and reporting purposes. 9675 */ 9676 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 9677 @Description(shortDefinition="Tracking/reporting short description of the test", formalDefinition="A short description of the test used by test engines for tracking and reporting purposes." ) 9678 protected StringType description; 9679 9680 /** 9681 * Action would contain either an operation or an assertion. 9682 */ 9683 @Child(name = "action", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9684 @Description(shortDefinition="A test operation or assert to perform", formalDefinition="Action would contain either an operation or an assertion." ) 9685 protected List<TestActionComponent> action; 9686 9687 private static final long serialVersionUID = -865006110L; 9688 9689 /** 9690 * Constructor 9691 */ 9692 public TestScriptTestComponent() { 9693 super(); 9694 } 9695 9696 /** 9697 * @return {@link #name} (The name of this test used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 9698 */ 9699 public StringType getNameElement() { 9700 if (this.name == null) 9701 if (Configuration.errorOnAutoCreate()) 9702 throw new Error("Attempt to auto-create TestScriptTestComponent.name"); 9703 else if (Configuration.doAutoCreate()) 9704 this.name = new StringType(); // bb 9705 return this.name; 9706 } 9707 9708 public boolean hasNameElement() { 9709 return this.name != null && !this.name.isEmpty(); 9710 } 9711 9712 public boolean hasName() { 9713 return this.name != null && !this.name.isEmpty(); 9714 } 9715 9716 /** 9717 * @param value {@link #name} (The name of this test used for tracking/logging purposes by test engines.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 9718 */ 9719 public TestScriptTestComponent setNameElement(StringType value) { 9720 this.name = value; 9721 return this; 9722 } 9723 9724 /** 9725 * @return The name of this test used for tracking/logging purposes by test engines. 9726 */ 9727 public String getName() { 9728 return this.name == null ? null : this.name.getValue(); 9729 } 9730 9731 /** 9732 * @param value The name of this test used for tracking/logging purposes by test engines. 9733 */ 9734 public TestScriptTestComponent setName(String value) { 9735 if (Utilities.noString(value)) 9736 this.name = null; 9737 else { 9738 if (this.name == null) 9739 this.name = new StringType(); 9740 this.name.setValue(value); 9741 } 9742 return this; 9743 } 9744 9745 /** 9746 * @return {@link #description} (A short description of the test used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 9747 */ 9748 public StringType getDescriptionElement() { 9749 if (this.description == null) 9750 if (Configuration.errorOnAutoCreate()) 9751 throw new Error("Attempt to auto-create TestScriptTestComponent.description"); 9752 else if (Configuration.doAutoCreate()) 9753 this.description = new StringType(); // bb 9754 return this.description; 9755 } 9756 9757 public boolean hasDescriptionElement() { 9758 return this.description != null && !this.description.isEmpty(); 9759 } 9760 9761 public boolean hasDescription() { 9762 return this.description != null && !this.description.isEmpty(); 9763 } 9764 9765 /** 9766 * @param value {@link #description} (A short description of the test used by test engines for tracking and reporting purposes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 9767 */ 9768 public TestScriptTestComponent setDescriptionElement(StringType value) { 9769 this.description = value; 9770 return this; 9771 } 9772 9773 /** 9774 * @return A short description of the test used by test engines for tracking and reporting purposes. 9775 */ 9776 public String getDescription() { 9777 return this.description == null ? null : this.description.getValue(); 9778 } 9779 9780 /** 9781 * @param value A short description of the test used by test engines for tracking and reporting purposes. 9782 */ 9783 public TestScriptTestComponent setDescription(String value) { 9784 if (Utilities.noString(value)) 9785 this.description = null; 9786 else { 9787 if (this.description == null) 9788 this.description = new StringType(); 9789 this.description.setValue(value); 9790 } 9791 return this; 9792 } 9793 9794 /** 9795 * @return {@link #action} (Action would contain either an operation or an assertion.) 9796 */ 9797 public List<TestActionComponent> getAction() { 9798 if (this.action == null) 9799 this.action = new ArrayList<TestActionComponent>(); 9800 return this.action; 9801 } 9802 9803 /** 9804 * @return Returns a reference to <code>this</code> for easy method chaining 9805 */ 9806 public TestScriptTestComponent setAction(List<TestActionComponent> theAction) { 9807 this.action = theAction; 9808 return this; 9809 } 9810 9811 public boolean hasAction() { 9812 if (this.action == null) 9813 return false; 9814 for (TestActionComponent item : this.action) 9815 if (!item.isEmpty()) 9816 return true; 9817 return false; 9818 } 9819 9820 public TestActionComponent addAction() { //3 9821 TestActionComponent t = new TestActionComponent(); 9822 if (this.action == null) 9823 this.action = new ArrayList<TestActionComponent>(); 9824 this.action.add(t); 9825 return t; 9826 } 9827 9828 public TestScriptTestComponent addAction(TestActionComponent t) { //3 9829 if (t == null) 9830 return this; 9831 if (this.action == null) 9832 this.action = new ArrayList<TestActionComponent>(); 9833 this.action.add(t); 9834 return this; 9835 } 9836 9837 /** 9838 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 9839 */ 9840 public TestActionComponent getActionFirstRep() { 9841 if (getAction().isEmpty()) { 9842 addAction(); 9843 } 9844 return getAction().get(0); 9845 } 9846 9847 protected void listChildren(List<Property> children) { 9848 super.listChildren(children); 9849 children.add(new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name)); 9850 children.add(new Property("description", "string", "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, description)); 9851 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action)); 9852 } 9853 9854 @Override 9855 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9856 switch (_hash) { 9857 case 3373707: /*name*/ return new Property("name", "string", "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name); 9858 case -1724546052: /*description*/ return new Property("description", "string", "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, description); 9859 case -1422950858: /*action*/ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, java.lang.Integer.MAX_VALUE, action); 9860 default: return super.getNamedProperty(_hash, _name, _checkValid); 9861 } 9862 9863 } 9864 9865 @Override 9866 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9867 switch (hash) { 9868 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 9869 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 9870 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TestActionComponent 9871 default: return super.getProperty(hash, name, checkValid); 9872 } 9873 9874 } 9875 9876 @Override 9877 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9878 switch (hash) { 9879 case 3373707: // name 9880 this.name = castToString(value); // StringType 9881 return value; 9882 case -1724546052: // description 9883 this.description = castToString(value); // StringType 9884 return value; 9885 case -1422950858: // action 9886 this.getAction().add((TestActionComponent) value); // TestActionComponent 9887 return value; 9888 default: return super.setProperty(hash, name, value); 9889 } 9890 9891 } 9892 9893 @Override 9894 public Base setProperty(String name, Base value) throws FHIRException { 9895 if (name.equals("name")) { 9896 this.name = castToString(value); // StringType 9897 } else if (name.equals("description")) { 9898 this.description = castToString(value); // StringType 9899 } else if (name.equals("action")) { 9900 this.getAction().add((TestActionComponent) value); 9901 } else 9902 return super.setProperty(name, value); 9903 return value; 9904 } 9905 9906 @Override 9907 public Base makeProperty(int hash, String name) throws FHIRException { 9908 switch (hash) { 9909 case 3373707: return getNameElement(); 9910 case -1724546052: return getDescriptionElement(); 9911 case -1422950858: return addAction(); 9912 default: return super.makeProperty(hash, name); 9913 } 9914 9915 } 9916 9917 @Override 9918 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9919 switch (hash) { 9920 case 3373707: /*name*/ return new String[] {"string"}; 9921 case -1724546052: /*description*/ return new String[] {"string"}; 9922 case -1422950858: /*action*/ return new String[] {}; 9923 default: return super.getTypesForProperty(hash, name); 9924 } 9925 9926 } 9927 9928 @Override 9929 public Base addChild(String name) throws FHIRException { 9930 if (name.equals("name")) { 9931 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 9932 } 9933 else if (name.equals("description")) { 9934 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 9935 } 9936 else if (name.equals("action")) { 9937 return addAction(); 9938 } 9939 else 9940 return super.addChild(name); 9941 } 9942 9943 public TestScriptTestComponent copy() { 9944 TestScriptTestComponent dst = new TestScriptTestComponent(); 9945 copyValues(dst); 9946 dst.name = name == null ? null : name.copy(); 9947 dst.description = description == null ? null : description.copy(); 9948 if (action != null) { 9949 dst.action = new ArrayList<TestActionComponent>(); 9950 for (TestActionComponent i : action) 9951 dst.action.add(i.copy()); 9952 }; 9953 return dst; 9954 } 9955 9956 @Override 9957 public boolean equalsDeep(Base other_) { 9958 if (!super.equalsDeep(other_)) 9959 return false; 9960 if (!(other_ instanceof TestScriptTestComponent)) 9961 return false; 9962 TestScriptTestComponent o = (TestScriptTestComponent) other_; 9963 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(action, o.action, true) 9964 ; 9965 } 9966 9967 @Override 9968 public boolean equalsShallow(Base other_) { 9969 if (!super.equalsShallow(other_)) 9970 return false; 9971 if (!(other_ instanceof TestScriptTestComponent)) 9972 return false; 9973 TestScriptTestComponent o = (TestScriptTestComponent) other_; 9974 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 9975 } 9976 9977 public boolean isEmpty() { 9978 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description, action 9979 ); 9980 } 9981 9982 public String fhirType() { 9983 return "TestScript.test"; 9984 9985 } 9986 9987 } 9988 9989 @Block() 9990 public static class TestActionComponent extends BackboneElement implements IBaseBackboneElement { 9991 /** 9992 * An operation would involve a REST request to a server. 9993 */ 9994 @Child(name = "operation", type = {SetupActionOperationComponent.class}, order=1, min=0, max=1, modifier=false, summary=false) 9995 @Description(shortDefinition="The setup operation to perform", formalDefinition="An operation would involve a REST request to a server." ) 9996 protected SetupActionOperationComponent operation; 9997 9998 /** 9999 * Evaluates the results of previous operations to determine if the server under test behaves appropriately. 10000 */ 10001 @Child(name = "assert", type = {SetupActionAssertComponent.class}, order=2, min=0, max=1, modifier=false, summary=false) 10002 @Description(shortDefinition="The setup assertion to perform", formalDefinition="Evaluates the results of previous operations to determine if the server under test behaves appropriately." ) 10003 protected SetupActionAssertComponent assert_; 10004 10005 private static final long serialVersionUID = -252088305L; 10006 10007 /** 10008 * Constructor 10009 */ 10010 public TestActionComponent() { 10011 super(); 10012 } 10013 10014 /** 10015 * @return {@link #operation} (An operation would involve a REST request to a server.) 10016 */ 10017 public SetupActionOperationComponent getOperation() { 10018 if (this.operation == null) 10019 if (Configuration.errorOnAutoCreate()) 10020 throw new Error("Attempt to auto-create TestActionComponent.operation"); 10021 else if (Configuration.doAutoCreate()) 10022 this.operation = new SetupActionOperationComponent(); // cc 10023 return this.operation; 10024 } 10025 10026 public boolean hasOperation() { 10027 return this.operation != null && !this.operation.isEmpty(); 10028 } 10029 10030 /** 10031 * @param value {@link #operation} (An operation would involve a REST request to a server.) 10032 */ 10033 public TestActionComponent setOperation(SetupActionOperationComponent value) { 10034 this.operation = value; 10035 return this; 10036 } 10037 10038 /** 10039 * @return {@link #assert_} (Evaluates the results of previous operations to determine if the server under test behaves appropriately.) 10040 */ 10041 public SetupActionAssertComponent getAssert() { 10042 if (this.assert_ == null) 10043 if (Configuration.errorOnAutoCreate()) 10044 throw new Error("Attempt to auto-create TestActionComponent.assert_"); 10045 else if (Configuration.doAutoCreate()) 10046 this.assert_ = new SetupActionAssertComponent(); // cc 10047 return this.assert_; 10048 } 10049 10050 public boolean hasAssert() { 10051 return this.assert_ != null && !this.assert_.isEmpty(); 10052 } 10053 10054 /** 10055 * @param value {@link #assert_} (Evaluates the results of previous operations to determine if the server under test behaves appropriately.) 10056 */ 10057 public TestActionComponent setAssert(SetupActionAssertComponent value) { 10058 this.assert_ = value; 10059 return this; 10060 } 10061 10062 protected void listChildren(List<Property> children) { 10063 super.listChildren(children); 10064 children.add(new Property("operation", "@TestScript.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation)); 10065 children.add(new Property("assert", "@TestScript.setup.action.assert", "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 0, 1, assert_)); 10066 } 10067 10068 @Override 10069 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10070 switch (_hash) { 10071 case 1662702951: /*operation*/ return new Property("operation", "@TestScript.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation); 10072 case -1408208058: /*assert*/ return new Property("assert", "@TestScript.setup.action.assert", "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 0, 1, assert_); 10073 default: return super.getNamedProperty(_hash, _name, _checkValid); 10074 } 10075 10076 } 10077 10078 @Override 10079 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10080 switch (hash) { 10081 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 10082 case -1408208058: /*assert*/ return this.assert_ == null ? new Base[0] : new Base[] {this.assert_}; // SetupActionAssertComponent 10083 default: return super.getProperty(hash, name, checkValid); 10084 } 10085 10086 } 10087 10088 @Override 10089 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10090 switch (hash) { 10091 case 1662702951: // operation 10092 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 10093 return value; 10094 case -1408208058: // assert 10095 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 10096 return value; 10097 default: return super.setProperty(hash, name, value); 10098 } 10099 10100 } 10101 10102 @Override 10103 public Base setProperty(String name, Base value) throws FHIRException { 10104 if (name.equals("operation")) { 10105 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 10106 } else if (name.equals("assert")) { 10107 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 10108 } else 10109 return super.setProperty(name, value); 10110 return value; 10111 } 10112 10113 @Override 10114 public Base makeProperty(int hash, String name) throws FHIRException { 10115 switch (hash) { 10116 case 1662702951: return getOperation(); 10117 case -1408208058: return getAssert(); 10118 default: return super.makeProperty(hash, name); 10119 } 10120 10121 } 10122 10123 @Override 10124 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10125 switch (hash) { 10126 case 1662702951: /*operation*/ return new String[] {"@TestScript.setup.action.operation"}; 10127 case -1408208058: /*assert*/ return new String[] {"@TestScript.setup.action.assert"}; 10128 default: return super.getTypesForProperty(hash, name); 10129 } 10130 10131 } 10132 10133 @Override 10134 public Base addChild(String name) throws FHIRException { 10135 if (name.equals("operation")) { 10136 this.operation = new SetupActionOperationComponent(); 10137 return this.operation; 10138 } 10139 else if (name.equals("assert")) { 10140 this.assert_ = new SetupActionAssertComponent(); 10141 return this.assert_; 10142 } 10143 else 10144 return super.addChild(name); 10145 } 10146 10147 public TestActionComponent copy() { 10148 TestActionComponent dst = new TestActionComponent(); 10149 copyValues(dst); 10150 dst.operation = operation == null ? null : operation.copy(); 10151 dst.assert_ = assert_ == null ? null : assert_.copy(); 10152 return dst; 10153 } 10154 10155 @Override 10156 public boolean equalsDeep(Base other_) { 10157 if (!super.equalsDeep(other_)) 10158 return false; 10159 if (!(other_ instanceof TestActionComponent)) 10160 return false; 10161 TestActionComponent o = (TestActionComponent) other_; 10162 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 10163 } 10164 10165 @Override 10166 public boolean equalsShallow(Base other_) { 10167 if (!super.equalsShallow(other_)) 10168 return false; 10169 if (!(other_ instanceof TestActionComponent)) 10170 return false; 10171 TestActionComponent o = (TestActionComponent) other_; 10172 return true; 10173 } 10174 10175 public boolean isEmpty() { 10176 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 10177 } 10178 10179 public String fhirType() { 10180 return "TestScript.test.action"; 10181 10182 } 10183 10184 } 10185 10186 @Block() 10187 public static class TestScriptTeardownComponent extends BackboneElement implements IBaseBackboneElement { 10188 /** 10189 * The teardown action will only contain an operation. 10190 */ 10191 @Child(name = "action", type = {}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10192 @Description(shortDefinition="One or more teardown operations to perform", formalDefinition="The teardown action will only contain an operation." ) 10193 protected List<TeardownActionComponent> action; 10194 10195 private static final long serialVersionUID = 1168638089L; 10196 10197 /** 10198 * Constructor 10199 */ 10200 public TestScriptTeardownComponent() { 10201 super(); 10202 } 10203 10204 /** 10205 * @return {@link #action} (The teardown action will only contain an operation.) 10206 */ 10207 public List<TeardownActionComponent> getAction() { 10208 if (this.action == null) 10209 this.action = new ArrayList<TeardownActionComponent>(); 10210 return this.action; 10211 } 10212 10213 /** 10214 * @return Returns a reference to <code>this</code> for easy method chaining 10215 */ 10216 public TestScriptTeardownComponent setAction(List<TeardownActionComponent> theAction) { 10217 this.action = theAction; 10218 return this; 10219 } 10220 10221 public boolean hasAction() { 10222 if (this.action == null) 10223 return false; 10224 for (TeardownActionComponent item : this.action) 10225 if (!item.isEmpty()) 10226 return true; 10227 return false; 10228 } 10229 10230 public TeardownActionComponent addAction() { //3 10231 TeardownActionComponent t = new TeardownActionComponent(); 10232 if (this.action == null) 10233 this.action = new ArrayList<TeardownActionComponent>(); 10234 this.action.add(t); 10235 return t; 10236 } 10237 10238 public TestScriptTeardownComponent addAction(TeardownActionComponent t) { //3 10239 if (t == null) 10240 return this; 10241 if (this.action == null) 10242 this.action = new ArrayList<TeardownActionComponent>(); 10243 this.action.add(t); 10244 return this; 10245 } 10246 10247 /** 10248 * @return The first repetition of repeating field {@link #action}, creating it if it does not already exist 10249 */ 10250 public TeardownActionComponent getActionFirstRep() { 10251 if (getAction().isEmpty()) { 10252 addAction(); 10253 } 10254 return getAction().get(0); 10255 } 10256 10257 protected void listChildren(List<Property> children) { 10258 super.listChildren(children); 10259 children.add(new Property("action", "", "The teardown action will only contain an operation.", 0, java.lang.Integer.MAX_VALUE, action)); 10260 } 10261 10262 @Override 10263 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10264 switch (_hash) { 10265 case -1422950858: /*action*/ return new Property("action", "", "The teardown action will only contain an operation.", 0, java.lang.Integer.MAX_VALUE, action); 10266 default: return super.getNamedProperty(_hash, _name, _checkValid); 10267 } 10268 10269 } 10270 10271 @Override 10272 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10273 switch (hash) { 10274 case -1422950858: /*action*/ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TeardownActionComponent 10275 default: return super.getProperty(hash, name, checkValid); 10276 } 10277 10278 } 10279 10280 @Override 10281 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10282 switch (hash) { 10283 case -1422950858: // action 10284 this.getAction().add((TeardownActionComponent) value); // TeardownActionComponent 10285 return value; 10286 default: return super.setProperty(hash, name, value); 10287 } 10288 10289 } 10290 10291 @Override 10292 public Base setProperty(String name, Base value) throws FHIRException { 10293 if (name.equals("action")) { 10294 this.getAction().add((TeardownActionComponent) value); 10295 } else 10296 return super.setProperty(name, value); 10297 return value; 10298 } 10299 10300 @Override 10301 public Base makeProperty(int hash, String name) throws FHIRException { 10302 switch (hash) { 10303 case -1422950858: return addAction(); 10304 default: return super.makeProperty(hash, name); 10305 } 10306 10307 } 10308 10309 @Override 10310 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10311 switch (hash) { 10312 case -1422950858: /*action*/ return new String[] {}; 10313 default: return super.getTypesForProperty(hash, name); 10314 } 10315 10316 } 10317 10318 @Override 10319 public Base addChild(String name) throws FHIRException { 10320 if (name.equals("action")) { 10321 return addAction(); 10322 } 10323 else 10324 return super.addChild(name); 10325 } 10326 10327 public TestScriptTeardownComponent copy() { 10328 TestScriptTeardownComponent dst = new TestScriptTeardownComponent(); 10329 copyValues(dst); 10330 if (action != null) { 10331 dst.action = new ArrayList<TeardownActionComponent>(); 10332 for (TeardownActionComponent i : action) 10333 dst.action.add(i.copy()); 10334 }; 10335 return dst; 10336 } 10337 10338 @Override 10339 public boolean equalsDeep(Base other_) { 10340 if (!super.equalsDeep(other_)) 10341 return false; 10342 if (!(other_ instanceof TestScriptTeardownComponent)) 10343 return false; 10344 TestScriptTeardownComponent o = (TestScriptTeardownComponent) other_; 10345 return compareDeep(action, o.action, true); 10346 } 10347 10348 @Override 10349 public boolean equalsShallow(Base other_) { 10350 if (!super.equalsShallow(other_)) 10351 return false; 10352 if (!(other_ instanceof TestScriptTeardownComponent)) 10353 return false; 10354 TestScriptTeardownComponent o = (TestScriptTeardownComponent) other_; 10355 return true; 10356 } 10357 10358 public boolean isEmpty() { 10359 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 10360 } 10361 10362 public String fhirType() { 10363 return "TestScript.teardown"; 10364 10365 } 10366 10367 } 10368 10369 @Block() 10370 public static class TeardownActionComponent extends BackboneElement implements IBaseBackboneElement { 10371 /** 10372 * An operation would involve a REST request to a server. 10373 */ 10374 @Child(name = "operation", type = {SetupActionOperationComponent.class}, order=1, min=1, max=1, modifier=false, summary=false) 10375 @Description(shortDefinition="The teardown operation to perform", formalDefinition="An operation would involve a REST request to a server." ) 10376 protected SetupActionOperationComponent operation; 10377 10378 private static final long serialVersionUID = -1099598054L; 10379 10380 /** 10381 * Constructor 10382 */ 10383 public TeardownActionComponent() { 10384 super(); 10385 } 10386 10387 /** 10388 * Constructor 10389 */ 10390 public TeardownActionComponent(SetupActionOperationComponent operation) { 10391 super(); 10392 this.operation = operation; 10393 } 10394 10395 /** 10396 * @return {@link #operation} (An operation would involve a REST request to a server.) 10397 */ 10398 public SetupActionOperationComponent getOperation() { 10399 if (this.operation == null) 10400 if (Configuration.errorOnAutoCreate()) 10401 throw new Error("Attempt to auto-create TeardownActionComponent.operation"); 10402 else if (Configuration.doAutoCreate()) 10403 this.operation = new SetupActionOperationComponent(); // cc 10404 return this.operation; 10405 } 10406 10407 public boolean hasOperation() { 10408 return this.operation != null && !this.operation.isEmpty(); 10409 } 10410 10411 /** 10412 * @param value {@link #operation} (An operation would involve a REST request to a server.) 10413 */ 10414 public TeardownActionComponent setOperation(SetupActionOperationComponent value) { 10415 this.operation = value; 10416 return this; 10417 } 10418 10419 protected void listChildren(List<Property> children) { 10420 super.listChildren(children); 10421 children.add(new Property("operation", "@TestScript.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation)); 10422 } 10423 10424 @Override 10425 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10426 switch (_hash) { 10427 case 1662702951: /*operation*/ return new Property("operation", "@TestScript.setup.action.operation", "An operation would involve a REST request to a server.", 0, 1, operation); 10428 default: return super.getNamedProperty(_hash, _name, _checkValid); 10429 } 10430 10431 } 10432 10433 @Override 10434 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10435 switch (hash) { 10436 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // SetupActionOperationComponent 10437 default: return super.getProperty(hash, name, checkValid); 10438 } 10439 10440 } 10441 10442 @Override 10443 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10444 switch (hash) { 10445 case 1662702951: // operation 10446 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 10447 return value; 10448 default: return super.setProperty(hash, name, value); 10449 } 10450 10451 } 10452 10453 @Override 10454 public Base setProperty(String name, Base value) throws FHIRException { 10455 if (name.equals("operation")) { 10456 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 10457 } else 10458 return super.setProperty(name, value); 10459 return value; 10460 } 10461 10462 @Override 10463 public Base makeProperty(int hash, String name) throws FHIRException { 10464 switch (hash) { 10465 case 1662702951: return getOperation(); 10466 default: return super.makeProperty(hash, name); 10467 } 10468 10469 } 10470 10471 @Override 10472 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10473 switch (hash) { 10474 case 1662702951: /*operation*/ return new String[] {"@TestScript.setup.action.operation"}; 10475 default: return super.getTypesForProperty(hash, name); 10476 } 10477 10478 } 10479 10480 @Override 10481 public Base addChild(String name) throws FHIRException { 10482 if (name.equals("operation")) { 10483 this.operation = new SetupActionOperationComponent(); 10484 return this.operation; 10485 } 10486 else 10487 return super.addChild(name); 10488 } 10489 10490 public TeardownActionComponent copy() { 10491 TeardownActionComponent dst = new TeardownActionComponent(); 10492 copyValues(dst); 10493 dst.operation = operation == null ? null : operation.copy(); 10494 return dst; 10495 } 10496 10497 @Override 10498 public boolean equalsDeep(Base other_) { 10499 if (!super.equalsDeep(other_)) 10500 return false; 10501 if (!(other_ instanceof TeardownActionComponent)) 10502 return false; 10503 TeardownActionComponent o = (TeardownActionComponent) other_; 10504 return compareDeep(operation, o.operation, true); 10505 } 10506 10507 @Override 10508 public boolean equalsShallow(Base other_) { 10509 if (!super.equalsShallow(other_)) 10510 return false; 10511 if (!(other_ instanceof TeardownActionComponent)) 10512 return false; 10513 TeardownActionComponent o = (TeardownActionComponent) other_; 10514 return true; 10515 } 10516 10517 public boolean isEmpty() { 10518 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation); 10519 } 10520 10521 public String fhirType() { 10522 return "TestScript.teardown.action"; 10523 10524 } 10525 10526 } 10527 10528 /** 10529 * A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance. 10530 */ 10531 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 10532 @Description(shortDefinition="Additional identifier for the test script", formalDefinition="A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 10533 protected Identifier identifier; 10534 10535 /** 10536 * Explaination of why this test script is needed and why it has been designed as it has. 10537 */ 10538 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 10539 @Description(shortDefinition="Why this test script is defined", formalDefinition="Explaination of why this test script is needed and why it has been designed as it has." ) 10540 protected MarkdownType purpose; 10541 10542 /** 10543 * A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script. 10544 */ 10545 @Child(name = "copyright", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 10546 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script." ) 10547 protected MarkdownType copyright; 10548 10549 /** 10550 * An abstract server used in operations within this test script in the origin element. 10551 */ 10552 @Child(name = "origin", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10553 @Description(shortDefinition="An abstract server representing a client or sender in a message exchange", formalDefinition="An abstract server used in operations within this test script in the origin element." ) 10554 protected List<TestScriptOriginComponent> origin; 10555 10556 /** 10557 * An abstract server used in operations within this test script in the destination element. 10558 */ 10559 @Child(name = "destination", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10560 @Description(shortDefinition="An abstract server representing a destination or receiver in a message exchange", formalDefinition="An abstract server used in operations within this test script in the destination element." ) 10561 protected List<TestScriptDestinationComponent> destination; 10562 10563 /** 10564 * The required capability must exist and are assumed to function correctly on the FHIR server being tested. 10565 */ 10566 @Child(name = "metadata", type = {}, order=5, min=0, max=1, modifier=false, summary=false) 10567 @Description(shortDefinition="Required capability that is assumed to function correctly on the FHIR server being tested", formalDefinition="The required capability must exist and are assumed to function correctly on the FHIR server being tested." ) 10568 protected TestScriptMetadataComponent metadata; 10569 10570 /** 10571 * Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute. 10572 */ 10573 @Child(name = "fixture", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10574 @Description(shortDefinition="Fixture in the test script - by reference (uri)", formalDefinition="Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute." ) 10575 protected List<TestScriptFixtureComponent> fixture; 10576 10577 /** 10578 * Reference to the profile to be used for validation. 10579 */ 10580 @Child(name = "profile", type = {Reference.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10581 @Description(shortDefinition="Reference of the validation profile", formalDefinition="Reference to the profile to be used for validation." ) 10582 protected List<Reference> profile; 10583 /** 10584 * The actual objects that are the target of the reference (Reference to the profile to be used for validation.) 10585 */ 10586 protected List<Resource> profileTarget; 10587 10588 10589 /** 10590 * Variable is set based either on element value in response body or on header field value in the response headers. 10591 */ 10592 @Child(name = "variable", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10593 @Description(shortDefinition="Placeholder for evaluated elements", formalDefinition="Variable is set based either on element value in response body or on header field value in the response headers." ) 10594 protected List<TestScriptVariableComponent> variable; 10595 10596 /** 10597 * Assert rule to be used in one or more asserts within the test script. 10598 */ 10599 @Child(name = "rule", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10600 @Description(shortDefinition="Assert rule used within the test script", formalDefinition="Assert rule to be used in one or more asserts within the test script." ) 10601 protected List<TestScriptRuleComponent> rule; 10602 10603 /** 10604 * Contains one or more rules. Offers a way to group rules so assertions could reference the group of rules and have them all applied. 10605 */ 10606 @Child(name = "ruleset", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10607 @Description(shortDefinition="Assert ruleset used within the test script", formalDefinition="Contains one or more rules. Offers a way to group rules so assertions could reference the group of rules and have them all applied." ) 10608 protected List<TestScriptRulesetComponent> ruleset; 10609 10610 /** 10611 * A series of required setup operations before tests are executed. 10612 */ 10613 @Child(name = "setup", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 10614 @Description(shortDefinition="A series of required setup operations before tests are executed", formalDefinition="A series of required setup operations before tests are executed." ) 10615 protected TestScriptSetupComponent setup; 10616 10617 /** 10618 * A test in this script. 10619 */ 10620 @Child(name = "test", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10621 @Description(shortDefinition="A test in this script", formalDefinition="A test in this script." ) 10622 protected List<TestScriptTestComponent> test; 10623 10624 /** 10625 * A series of operations required to clean up after the all the tests are executed (successfully or otherwise). 10626 */ 10627 @Child(name = "teardown", type = {}, order=13, min=0, max=1, modifier=false, summary=false) 10628 @Description(shortDefinition="A series of required clean up steps", formalDefinition="A series of operations required to clean up after the all the tests are executed (successfully or otherwise)." ) 10629 protected TestScriptTeardownComponent teardown; 10630 10631 private static final long serialVersionUID = 1164952373L; 10632 10633 /** 10634 * Constructor 10635 */ 10636 public TestScript() { 10637 super(); 10638 } 10639 10640 /** 10641 * Constructor 10642 */ 10643 public TestScript(UriType url, StringType name, Enumeration<PublicationStatus> status) { 10644 super(); 10645 this.url = url; 10646 this.name = name; 10647 this.status = status; 10648 } 10649 10650 /** 10651 * @return {@link #url} (An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this test script is (or will be) published. The URL SHOULD include the major version of the test script. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 10652 */ 10653 public UriType getUrlElement() { 10654 if (this.url == null) 10655 if (Configuration.errorOnAutoCreate()) 10656 throw new Error("Attempt to auto-create TestScript.url"); 10657 else if (Configuration.doAutoCreate()) 10658 this.url = new UriType(); // bb 10659 return this.url; 10660 } 10661 10662 public boolean hasUrlElement() { 10663 return this.url != null && !this.url.isEmpty(); 10664 } 10665 10666 public boolean hasUrl() { 10667 return this.url != null && !this.url.isEmpty(); 10668 } 10669 10670 /** 10671 * @param value {@link #url} (An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this test script is (or will be) published. The URL SHOULD include the major version of the test script. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 10672 */ 10673 public TestScript setUrlElement(UriType value) { 10674 this.url = value; 10675 return this; 10676 } 10677 10678 /** 10679 * @return An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this test script is (or will be) published. The URL SHOULD include the major version of the test script. For more information see [Technical and Business Versions](resource.html#versions). 10680 */ 10681 public String getUrl() { 10682 return this.url == null ? null : this.url.getValue(); 10683 } 10684 10685 /** 10686 * @param value An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this test script is (or will be) published. The URL SHOULD include the major version of the test script. For more information see [Technical and Business Versions](resource.html#versions). 10687 */ 10688 public TestScript setUrl(String value) { 10689 if (this.url == null) 10690 this.url = new UriType(); 10691 this.url.setValue(value); 10692 return this; 10693 } 10694 10695 /** 10696 * @return {@link #identifier} (A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance.) 10697 */ 10698 public Identifier getIdentifier() { 10699 if (this.identifier == null) 10700 if (Configuration.errorOnAutoCreate()) 10701 throw new Error("Attempt to auto-create TestScript.identifier"); 10702 else if (Configuration.doAutoCreate()) 10703 this.identifier = new Identifier(); // cc 10704 return this.identifier; 10705 } 10706 10707 public boolean hasIdentifier() { 10708 return this.identifier != null && !this.identifier.isEmpty(); 10709 } 10710 10711 /** 10712 * @param value {@link #identifier} (A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance.) 10713 */ 10714 public TestScript setIdentifier(Identifier value) { 10715 this.identifier = value; 10716 return this; 10717 } 10718 10719 /** 10720 * @return {@link #version} (The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 10721 */ 10722 public StringType getVersionElement() { 10723 if (this.version == null) 10724 if (Configuration.errorOnAutoCreate()) 10725 throw new Error("Attempt to auto-create TestScript.version"); 10726 else if (Configuration.doAutoCreate()) 10727 this.version = new StringType(); // bb 10728 return this.version; 10729 } 10730 10731 public boolean hasVersionElement() { 10732 return this.version != null && !this.version.isEmpty(); 10733 } 10734 10735 public boolean hasVersion() { 10736 return this.version != null && !this.version.isEmpty(); 10737 } 10738 10739 /** 10740 * @param value {@link #version} (The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 10741 */ 10742 public TestScript setVersionElement(StringType value) { 10743 this.version = value; 10744 return this; 10745 } 10746 10747 /** 10748 * @return The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 10749 */ 10750 public String getVersion() { 10751 return this.version == null ? null : this.version.getValue(); 10752 } 10753 10754 /** 10755 * @param value The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 10756 */ 10757 public TestScript setVersion(String value) { 10758 if (Utilities.noString(value)) 10759 this.version = null; 10760 else { 10761 if (this.version == null) 10762 this.version = new StringType(); 10763 this.version.setValue(value); 10764 } 10765 return this; 10766 } 10767 10768 /** 10769 * @return {@link #name} (A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 10770 */ 10771 public StringType getNameElement() { 10772 if (this.name == null) 10773 if (Configuration.errorOnAutoCreate()) 10774 throw new Error("Attempt to auto-create TestScript.name"); 10775 else if (Configuration.doAutoCreate()) 10776 this.name = new StringType(); // bb 10777 return this.name; 10778 } 10779 10780 public boolean hasNameElement() { 10781 return this.name != null && !this.name.isEmpty(); 10782 } 10783 10784 public boolean hasName() { 10785 return this.name != null && !this.name.isEmpty(); 10786 } 10787 10788 /** 10789 * @param value {@link #name} (A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 10790 */ 10791 public TestScript setNameElement(StringType value) { 10792 this.name = value; 10793 return this; 10794 } 10795 10796 /** 10797 * @return A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation. 10798 */ 10799 public String getName() { 10800 return this.name == null ? null : this.name.getValue(); 10801 } 10802 10803 /** 10804 * @param value A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation. 10805 */ 10806 public TestScript setName(String value) { 10807 if (this.name == null) 10808 this.name = new StringType(); 10809 this.name.setValue(value); 10810 return this; 10811 } 10812 10813 /** 10814 * @return {@link #title} (A short, descriptive, user-friendly title for the test script.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 10815 */ 10816 public StringType getTitleElement() { 10817 if (this.title == null) 10818 if (Configuration.errorOnAutoCreate()) 10819 throw new Error("Attempt to auto-create TestScript.title"); 10820 else if (Configuration.doAutoCreate()) 10821 this.title = new StringType(); // bb 10822 return this.title; 10823 } 10824 10825 public boolean hasTitleElement() { 10826 return this.title != null && !this.title.isEmpty(); 10827 } 10828 10829 public boolean hasTitle() { 10830 return this.title != null && !this.title.isEmpty(); 10831 } 10832 10833 /** 10834 * @param value {@link #title} (A short, descriptive, user-friendly title for the test script.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 10835 */ 10836 public TestScript setTitleElement(StringType value) { 10837 this.title = value; 10838 return this; 10839 } 10840 10841 /** 10842 * @return A short, descriptive, user-friendly title for the test script. 10843 */ 10844 public String getTitle() { 10845 return this.title == null ? null : this.title.getValue(); 10846 } 10847 10848 /** 10849 * @param value A short, descriptive, user-friendly title for the test script. 10850 */ 10851 public TestScript setTitle(String value) { 10852 if (Utilities.noString(value)) 10853 this.title = null; 10854 else { 10855 if (this.title == null) 10856 this.title = new StringType(); 10857 this.title.setValue(value); 10858 } 10859 return this; 10860 } 10861 10862 /** 10863 * @return {@link #status} (The status of this test script. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 10864 */ 10865 public Enumeration<PublicationStatus> getStatusElement() { 10866 if (this.status == null) 10867 if (Configuration.errorOnAutoCreate()) 10868 throw new Error("Attempt to auto-create TestScript.status"); 10869 else if (Configuration.doAutoCreate()) 10870 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 10871 return this.status; 10872 } 10873 10874 public boolean hasStatusElement() { 10875 return this.status != null && !this.status.isEmpty(); 10876 } 10877 10878 public boolean hasStatus() { 10879 return this.status != null && !this.status.isEmpty(); 10880 } 10881 10882 /** 10883 * @param value {@link #status} (The status of this test script. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 10884 */ 10885 public TestScript setStatusElement(Enumeration<PublicationStatus> value) { 10886 this.status = value; 10887 return this; 10888 } 10889 10890 /** 10891 * @return The status of this test script. Enables tracking the life-cycle of the content. 10892 */ 10893 public PublicationStatus getStatus() { 10894 return this.status == null ? null : this.status.getValue(); 10895 } 10896 10897 /** 10898 * @param value The status of this test script. Enables tracking the life-cycle of the content. 10899 */ 10900 public TestScript setStatus(PublicationStatus value) { 10901 if (this.status == null) 10902 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 10903 this.status.setValue(value); 10904 return this; 10905 } 10906 10907 /** 10908 * @return {@link #experimental} (A boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 10909 */ 10910 public BooleanType getExperimentalElement() { 10911 if (this.experimental == null) 10912 if (Configuration.errorOnAutoCreate()) 10913 throw new Error("Attempt to auto-create TestScript.experimental"); 10914 else if (Configuration.doAutoCreate()) 10915 this.experimental = new BooleanType(); // bb 10916 return this.experimental; 10917 } 10918 10919 public boolean hasExperimentalElement() { 10920 return this.experimental != null && !this.experimental.isEmpty(); 10921 } 10922 10923 public boolean hasExperimental() { 10924 return this.experimental != null && !this.experimental.isEmpty(); 10925 } 10926 10927 /** 10928 * @param value {@link #experimental} (A boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 10929 */ 10930 public TestScript setExperimentalElement(BooleanType value) { 10931 this.experimental = value; 10932 return this; 10933 } 10934 10935 /** 10936 * @return A boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 10937 */ 10938 public boolean getExperimental() { 10939 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 10940 } 10941 10942 /** 10943 * @param value A boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 10944 */ 10945 public TestScript setExperimental(boolean value) { 10946 if (this.experimental == null) 10947 this.experimental = new BooleanType(); 10948 this.experimental.setValue(value); 10949 return this; 10950 } 10951 10952 /** 10953 * @return {@link #date} (The date (and optionally time) when the test script was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 10954 */ 10955 public DateTimeType getDateElement() { 10956 if (this.date == null) 10957 if (Configuration.errorOnAutoCreate()) 10958 throw new Error("Attempt to auto-create TestScript.date"); 10959 else if (Configuration.doAutoCreate()) 10960 this.date = new DateTimeType(); // bb 10961 return this.date; 10962 } 10963 10964 public boolean hasDateElement() { 10965 return this.date != null && !this.date.isEmpty(); 10966 } 10967 10968 public boolean hasDate() { 10969 return this.date != null && !this.date.isEmpty(); 10970 } 10971 10972 /** 10973 * @param value {@link #date} (The date (and optionally time) when the test script was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 10974 */ 10975 public TestScript setDateElement(DateTimeType value) { 10976 this.date = value; 10977 return this; 10978 } 10979 10980 /** 10981 * @return The date (and optionally time) when the test script was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes. 10982 */ 10983 public Date getDate() { 10984 return this.date == null ? null : this.date.getValue(); 10985 } 10986 10987 /** 10988 * @param value The date (and optionally time) when the test script was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes. 10989 */ 10990 public TestScript setDate(Date value) { 10991 if (value == null) 10992 this.date = null; 10993 else { 10994 if (this.date == null) 10995 this.date = new DateTimeType(); 10996 this.date.setValue(value); 10997 } 10998 return this; 10999 } 11000 11001 /** 11002 * @return {@link #publisher} (The name of the individual or organization that published the test script.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 11003 */ 11004 public StringType getPublisherElement() { 11005 if (this.publisher == null) 11006 if (Configuration.errorOnAutoCreate()) 11007 throw new Error("Attempt to auto-create TestScript.publisher"); 11008 else if (Configuration.doAutoCreate()) 11009 this.publisher = new StringType(); // bb 11010 return this.publisher; 11011 } 11012 11013 public boolean hasPublisherElement() { 11014 return this.publisher != null && !this.publisher.isEmpty(); 11015 } 11016 11017 public boolean hasPublisher() { 11018 return this.publisher != null && !this.publisher.isEmpty(); 11019 } 11020 11021 /** 11022 * @param value {@link #publisher} (The name of the individual or organization that published the test script.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 11023 */ 11024 public TestScript setPublisherElement(StringType value) { 11025 this.publisher = value; 11026 return this; 11027 } 11028 11029 /** 11030 * @return The name of the individual or organization that published the test script. 11031 */ 11032 public String getPublisher() { 11033 return this.publisher == null ? null : this.publisher.getValue(); 11034 } 11035 11036 /** 11037 * @param value The name of the individual or organization that published the test script. 11038 */ 11039 public TestScript setPublisher(String value) { 11040 if (Utilities.noString(value)) 11041 this.publisher = null; 11042 else { 11043 if (this.publisher == null) 11044 this.publisher = new StringType(); 11045 this.publisher.setValue(value); 11046 } 11047 return this; 11048 } 11049 11050 /** 11051 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 11052 */ 11053 public List<ContactDetail> getContact() { 11054 if (this.contact == null) 11055 this.contact = new ArrayList<ContactDetail>(); 11056 return this.contact; 11057 } 11058 11059 /** 11060 * @return Returns a reference to <code>this</code> for easy method chaining 11061 */ 11062 public TestScript setContact(List<ContactDetail> theContact) { 11063 this.contact = theContact; 11064 return this; 11065 } 11066 11067 public boolean hasContact() { 11068 if (this.contact == null) 11069 return false; 11070 for (ContactDetail item : this.contact) 11071 if (!item.isEmpty()) 11072 return true; 11073 return false; 11074 } 11075 11076 public ContactDetail addContact() { //3 11077 ContactDetail t = new ContactDetail(); 11078 if (this.contact == null) 11079 this.contact = new ArrayList<ContactDetail>(); 11080 this.contact.add(t); 11081 return t; 11082 } 11083 11084 public TestScript addContact(ContactDetail t) { //3 11085 if (t == null) 11086 return this; 11087 if (this.contact == null) 11088 this.contact = new ArrayList<ContactDetail>(); 11089 this.contact.add(t); 11090 return this; 11091 } 11092 11093 /** 11094 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 11095 */ 11096 public ContactDetail getContactFirstRep() { 11097 if (getContact().isEmpty()) { 11098 addContact(); 11099 } 11100 return getContact().get(0); 11101 } 11102 11103 /** 11104 * @return {@link #description} (A free text natural language description of the test script from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 11105 */ 11106 public MarkdownType getDescriptionElement() { 11107 if (this.description == null) 11108 if (Configuration.errorOnAutoCreate()) 11109 throw new Error("Attempt to auto-create TestScript.description"); 11110 else if (Configuration.doAutoCreate()) 11111 this.description = new MarkdownType(); // bb 11112 return this.description; 11113 } 11114 11115 public boolean hasDescriptionElement() { 11116 return this.description != null && !this.description.isEmpty(); 11117 } 11118 11119 public boolean hasDescription() { 11120 return this.description != null && !this.description.isEmpty(); 11121 } 11122 11123 /** 11124 * @param value {@link #description} (A free text natural language description of the test script from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 11125 */ 11126 public TestScript setDescriptionElement(MarkdownType value) { 11127 this.description = value; 11128 return this; 11129 } 11130 11131 /** 11132 * @return A free text natural language description of the test script from a consumer's perspective. 11133 */ 11134 public String getDescription() { 11135 return this.description == null ? null : this.description.getValue(); 11136 } 11137 11138 /** 11139 * @param value A free text natural language description of the test script from a consumer's perspective. 11140 */ 11141 public TestScript setDescription(String value) { 11142 if (value == null) 11143 this.description = null; 11144 else { 11145 if (this.description == null) 11146 this.description = new MarkdownType(); 11147 this.description.setValue(value); 11148 } 11149 return this; 11150 } 11151 11152 /** 11153 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate test script instances.) 11154 */ 11155 public List<UsageContext> getUseContext() { 11156 if (this.useContext == null) 11157 this.useContext = new ArrayList<UsageContext>(); 11158 return this.useContext; 11159 } 11160 11161 /** 11162 * @return Returns a reference to <code>this</code> for easy method chaining 11163 */ 11164 public TestScript setUseContext(List<UsageContext> theUseContext) { 11165 this.useContext = theUseContext; 11166 return this; 11167 } 11168 11169 public boolean hasUseContext() { 11170 if (this.useContext == null) 11171 return false; 11172 for (UsageContext item : this.useContext) 11173 if (!item.isEmpty()) 11174 return true; 11175 return false; 11176 } 11177 11178 public UsageContext addUseContext() { //3 11179 UsageContext t = new UsageContext(); 11180 if (this.useContext == null) 11181 this.useContext = new ArrayList<UsageContext>(); 11182 this.useContext.add(t); 11183 return t; 11184 } 11185 11186 public TestScript addUseContext(UsageContext t) { //3 11187 if (t == null) 11188 return this; 11189 if (this.useContext == null) 11190 this.useContext = new ArrayList<UsageContext>(); 11191 this.useContext.add(t); 11192 return this; 11193 } 11194 11195 /** 11196 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 11197 */ 11198 public UsageContext getUseContextFirstRep() { 11199 if (getUseContext().isEmpty()) { 11200 addUseContext(); 11201 } 11202 return getUseContext().get(0); 11203 } 11204 11205 /** 11206 * @return {@link #jurisdiction} (A legal or geographic region in which the test script is intended to be used.) 11207 */ 11208 public List<CodeableConcept> getJurisdiction() { 11209 if (this.jurisdiction == null) 11210 this.jurisdiction = new ArrayList<CodeableConcept>(); 11211 return this.jurisdiction; 11212 } 11213 11214 /** 11215 * @return Returns a reference to <code>this</code> for easy method chaining 11216 */ 11217 public TestScript setJurisdiction(List<CodeableConcept> theJurisdiction) { 11218 this.jurisdiction = theJurisdiction; 11219 return this; 11220 } 11221 11222 public boolean hasJurisdiction() { 11223 if (this.jurisdiction == null) 11224 return false; 11225 for (CodeableConcept item : this.jurisdiction) 11226 if (!item.isEmpty()) 11227 return true; 11228 return false; 11229 } 11230 11231 public CodeableConcept addJurisdiction() { //3 11232 CodeableConcept t = new CodeableConcept(); 11233 if (this.jurisdiction == null) 11234 this.jurisdiction = new ArrayList<CodeableConcept>(); 11235 this.jurisdiction.add(t); 11236 return t; 11237 } 11238 11239 public TestScript addJurisdiction(CodeableConcept t) { //3 11240 if (t == null) 11241 return this; 11242 if (this.jurisdiction == null) 11243 this.jurisdiction = new ArrayList<CodeableConcept>(); 11244 this.jurisdiction.add(t); 11245 return this; 11246 } 11247 11248 /** 11249 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 11250 */ 11251 public CodeableConcept getJurisdictionFirstRep() { 11252 if (getJurisdiction().isEmpty()) { 11253 addJurisdiction(); 11254 } 11255 return getJurisdiction().get(0); 11256 } 11257 11258 /** 11259 * @return {@link #purpose} (Explaination of why this test script is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 11260 */ 11261 public MarkdownType getPurposeElement() { 11262 if (this.purpose == null) 11263 if (Configuration.errorOnAutoCreate()) 11264 throw new Error("Attempt to auto-create TestScript.purpose"); 11265 else if (Configuration.doAutoCreate()) 11266 this.purpose = new MarkdownType(); // bb 11267 return this.purpose; 11268 } 11269 11270 public boolean hasPurposeElement() { 11271 return this.purpose != null && !this.purpose.isEmpty(); 11272 } 11273 11274 public boolean hasPurpose() { 11275 return this.purpose != null && !this.purpose.isEmpty(); 11276 } 11277 11278 /** 11279 * @param value {@link #purpose} (Explaination of why this test script is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 11280 */ 11281 public TestScript setPurposeElement(MarkdownType value) { 11282 this.purpose = value; 11283 return this; 11284 } 11285 11286 /** 11287 * @return Explaination of why this test script is needed and why it has been designed as it has. 11288 */ 11289 public String getPurpose() { 11290 return this.purpose == null ? null : this.purpose.getValue(); 11291 } 11292 11293 /** 11294 * @param value Explaination of why this test script is needed and why it has been designed as it has. 11295 */ 11296 public TestScript setPurpose(String value) { 11297 if (value == null) 11298 this.purpose = null; 11299 else { 11300 if (this.purpose == null) 11301 this.purpose = new MarkdownType(); 11302 this.purpose.setValue(value); 11303 } 11304 return this; 11305 } 11306 11307 /** 11308 * @return {@link #copyright} (A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 11309 */ 11310 public MarkdownType getCopyrightElement() { 11311 if (this.copyright == null) 11312 if (Configuration.errorOnAutoCreate()) 11313 throw new Error("Attempt to auto-create TestScript.copyright"); 11314 else if (Configuration.doAutoCreate()) 11315 this.copyright = new MarkdownType(); // bb 11316 return this.copyright; 11317 } 11318 11319 public boolean hasCopyrightElement() { 11320 return this.copyright != null && !this.copyright.isEmpty(); 11321 } 11322 11323 public boolean hasCopyright() { 11324 return this.copyright != null && !this.copyright.isEmpty(); 11325 } 11326 11327 /** 11328 * @param value {@link #copyright} (A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 11329 */ 11330 public TestScript setCopyrightElement(MarkdownType value) { 11331 this.copyright = value; 11332 return this; 11333 } 11334 11335 /** 11336 * @return A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script. 11337 */ 11338 public String getCopyright() { 11339 return this.copyright == null ? null : this.copyright.getValue(); 11340 } 11341 11342 /** 11343 * @param value A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script. 11344 */ 11345 public TestScript setCopyright(String value) { 11346 if (value == null) 11347 this.copyright = null; 11348 else { 11349 if (this.copyright == null) 11350 this.copyright = new MarkdownType(); 11351 this.copyright.setValue(value); 11352 } 11353 return this; 11354 } 11355 11356 /** 11357 * @return {@link #origin} (An abstract server used in operations within this test script in the origin element.) 11358 */ 11359 public List<TestScriptOriginComponent> getOrigin() { 11360 if (this.origin == null) 11361 this.origin = new ArrayList<TestScriptOriginComponent>(); 11362 return this.origin; 11363 } 11364 11365 /** 11366 * @return Returns a reference to <code>this</code> for easy method chaining 11367 */ 11368 public TestScript setOrigin(List<TestScriptOriginComponent> theOrigin) { 11369 this.origin = theOrigin; 11370 return this; 11371 } 11372 11373 public boolean hasOrigin() { 11374 if (this.origin == null) 11375 return false; 11376 for (TestScriptOriginComponent item : this.origin) 11377 if (!item.isEmpty()) 11378 return true; 11379 return false; 11380 } 11381 11382 public TestScriptOriginComponent addOrigin() { //3 11383 TestScriptOriginComponent t = new TestScriptOriginComponent(); 11384 if (this.origin == null) 11385 this.origin = new ArrayList<TestScriptOriginComponent>(); 11386 this.origin.add(t); 11387 return t; 11388 } 11389 11390 public TestScript addOrigin(TestScriptOriginComponent t) { //3 11391 if (t == null) 11392 return this; 11393 if (this.origin == null) 11394 this.origin = new ArrayList<TestScriptOriginComponent>(); 11395 this.origin.add(t); 11396 return this; 11397 } 11398 11399 /** 11400 * @return The first repetition of repeating field {@link #origin}, creating it if it does not already exist 11401 */ 11402 public TestScriptOriginComponent getOriginFirstRep() { 11403 if (getOrigin().isEmpty()) { 11404 addOrigin(); 11405 } 11406 return getOrigin().get(0); 11407 } 11408 11409 /** 11410 * @return {@link #destination} (An abstract server used in operations within this test script in the destination element.) 11411 */ 11412 public List<TestScriptDestinationComponent> getDestination() { 11413 if (this.destination == null) 11414 this.destination = new ArrayList<TestScriptDestinationComponent>(); 11415 return this.destination; 11416 } 11417 11418 /** 11419 * @return Returns a reference to <code>this</code> for easy method chaining 11420 */ 11421 public TestScript setDestination(List<TestScriptDestinationComponent> theDestination) { 11422 this.destination = theDestination; 11423 return this; 11424 } 11425 11426 public boolean hasDestination() { 11427 if (this.destination == null) 11428 return false; 11429 for (TestScriptDestinationComponent item : this.destination) 11430 if (!item.isEmpty()) 11431 return true; 11432 return false; 11433 } 11434 11435 public TestScriptDestinationComponent addDestination() { //3 11436 TestScriptDestinationComponent t = new TestScriptDestinationComponent(); 11437 if (this.destination == null) 11438 this.destination = new ArrayList<TestScriptDestinationComponent>(); 11439 this.destination.add(t); 11440 return t; 11441 } 11442 11443 public TestScript addDestination(TestScriptDestinationComponent t) { //3 11444 if (t == null) 11445 return this; 11446 if (this.destination == null) 11447 this.destination = new ArrayList<TestScriptDestinationComponent>(); 11448 this.destination.add(t); 11449 return this; 11450 } 11451 11452 /** 11453 * @return The first repetition of repeating field {@link #destination}, creating it if it does not already exist 11454 */ 11455 public TestScriptDestinationComponent getDestinationFirstRep() { 11456 if (getDestination().isEmpty()) { 11457 addDestination(); 11458 } 11459 return getDestination().get(0); 11460 } 11461 11462 /** 11463 * @return {@link #metadata} (The required capability must exist and are assumed to function correctly on the FHIR server being tested.) 11464 */ 11465 public TestScriptMetadataComponent getMetadata() { 11466 if (this.metadata == null) 11467 if (Configuration.errorOnAutoCreate()) 11468 throw new Error("Attempt to auto-create TestScript.metadata"); 11469 else if (Configuration.doAutoCreate()) 11470 this.metadata = new TestScriptMetadataComponent(); // cc 11471 return this.metadata; 11472 } 11473 11474 public boolean hasMetadata() { 11475 return this.metadata != null && !this.metadata.isEmpty(); 11476 } 11477 11478 /** 11479 * @param value {@link #metadata} (The required capability must exist and are assumed to function correctly on the FHIR server being tested.) 11480 */ 11481 public TestScript setMetadata(TestScriptMetadataComponent value) { 11482 this.metadata = value; 11483 return this; 11484 } 11485 11486 /** 11487 * @return {@link #fixture} (Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.) 11488 */ 11489 public List<TestScriptFixtureComponent> getFixture() { 11490 if (this.fixture == null) 11491 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 11492 return this.fixture; 11493 } 11494 11495 /** 11496 * @return Returns a reference to <code>this</code> for easy method chaining 11497 */ 11498 public TestScript setFixture(List<TestScriptFixtureComponent> theFixture) { 11499 this.fixture = theFixture; 11500 return this; 11501 } 11502 11503 public boolean hasFixture() { 11504 if (this.fixture == null) 11505 return false; 11506 for (TestScriptFixtureComponent item : this.fixture) 11507 if (!item.isEmpty()) 11508 return true; 11509 return false; 11510 } 11511 11512 public TestScriptFixtureComponent addFixture() { //3 11513 TestScriptFixtureComponent t = new TestScriptFixtureComponent(); 11514 if (this.fixture == null) 11515 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 11516 this.fixture.add(t); 11517 return t; 11518 } 11519 11520 public TestScript addFixture(TestScriptFixtureComponent t) { //3 11521 if (t == null) 11522 return this; 11523 if (this.fixture == null) 11524 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 11525 this.fixture.add(t); 11526 return this; 11527 } 11528 11529 /** 11530 * @return The first repetition of repeating field {@link #fixture}, creating it if it does not already exist 11531 */ 11532 public TestScriptFixtureComponent getFixtureFirstRep() { 11533 if (getFixture().isEmpty()) { 11534 addFixture(); 11535 } 11536 return getFixture().get(0); 11537 } 11538 11539 /** 11540 * @return {@link #profile} (Reference to the profile to be used for validation.) 11541 */ 11542 public List<Reference> getProfile() { 11543 if (this.profile == null) 11544 this.profile = new ArrayList<Reference>(); 11545 return this.profile; 11546 } 11547 11548 /** 11549 * @return Returns a reference to <code>this</code> for easy method chaining 11550 */ 11551 public TestScript setProfile(List<Reference> theProfile) { 11552 this.profile = theProfile; 11553 return this; 11554 } 11555 11556 public boolean hasProfile() { 11557 if (this.profile == null) 11558 return false; 11559 for (Reference item : this.profile) 11560 if (!item.isEmpty()) 11561 return true; 11562 return false; 11563 } 11564 11565 public Reference addProfile() { //3 11566 Reference t = new Reference(); 11567 if (this.profile == null) 11568 this.profile = new ArrayList<Reference>(); 11569 this.profile.add(t); 11570 return t; 11571 } 11572 11573 public TestScript addProfile(Reference t) { //3 11574 if (t == null) 11575 return this; 11576 if (this.profile == null) 11577 this.profile = new ArrayList<Reference>(); 11578 this.profile.add(t); 11579 return this; 11580 } 11581 11582 /** 11583 * @return The first repetition of repeating field {@link #profile}, creating it if it does not already exist 11584 */ 11585 public Reference getProfileFirstRep() { 11586 if (getProfile().isEmpty()) { 11587 addProfile(); 11588 } 11589 return getProfile().get(0); 11590 } 11591 11592 /** 11593 * @deprecated Use Reference#setResource(IBaseResource) instead 11594 */ 11595 @Deprecated 11596 public List<Resource> getProfileTarget() { 11597 if (this.profileTarget == null) 11598 this.profileTarget = new ArrayList<Resource>(); 11599 return this.profileTarget; 11600 } 11601 11602 /** 11603 * @return {@link #variable} (Variable is set based either on element value in response body or on header field value in the response headers.) 11604 */ 11605 public List<TestScriptVariableComponent> getVariable() { 11606 if (this.variable == null) 11607 this.variable = new ArrayList<TestScriptVariableComponent>(); 11608 return this.variable; 11609 } 11610 11611 /** 11612 * @return Returns a reference to <code>this</code> for easy method chaining 11613 */ 11614 public TestScript setVariable(List<TestScriptVariableComponent> theVariable) { 11615 this.variable = theVariable; 11616 return this; 11617 } 11618 11619 public boolean hasVariable() { 11620 if (this.variable == null) 11621 return false; 11622 for (TestScriptVariableComponent item : this.variable) 11623 if (!item.isEmpty()) 11624 return true; 11625 return false; 11626 } 11627 11628 public TestScriptVariableComponent addVariable() { //3 11629 TestScriptVariableComponent t = new TestScriptVariableComponent(); 11630 if (this.variable == null) 11631 this.variable = new ArrayList<TestScriptVariableComponent>(); 11632 this.variable.add(t); 11633 return t; 11634 } 11635 11636 public TestScript addVariable(TestScriptVariableComponent t) { //3 11637 if (t == null) 11638 return this; 11639 if (this.variable == null) 11640 this.variable = new ArrayList<TestScriptVariableComponent>(); 11641 this.variable.add(t); 11642 return this; 11643 } 11644 11645 /** 11646 * @return The first repetition of repeating field {@link #variable}, creating it if it does not already exist 11647 */ 11648 public TestScriptVariableComponent getVariableFirstRep() { 11649 if (getVariable().isEmpty()) { 11650 addVariable(); 11651 } 11652 return getVariable().get(0); 11653 } 11654 11655 /** 11656 * @return {@link #rule} (Assert rule to be used in one or more asserts within the test script.) 11657 */ 11658 public List<TestScriptRuleComponent> getRule() { 11659 if (this.rule == null) 11660 this.rule = new ArrayList<TestScriptRuleComponent>(); 11661 return this.rule; 11662 } 11663 11664 /** 11665 * @return Returns a reference to <code>this</code> for easy method chaining 11666 */ 11667 public TestScript setRule(List<TestScriptRuleComponent> theRule) { 11668 this.rule = theRule; 11669 return this; 11670 } 11671 11672 public boolean hasRule() { 11673 if (this.rule == null) 11674 return false; 11675 for (TestScriptRuleComponent item : this.rule) 11676 if (!item.isEmpty()) 11677 return true; 11678 return false; 11679 } 11680 11681 public TestScriptRuleComponent addRule() { //3 11682 TestScriptRuleComponent t = new TestScriptRuleComponent(); 11683 if (this.rule == null) 11684 this.rule = new ArrayList<TestScriptRuleComponent>(); 11685 this.rule.add(t); 11686 return t; 11687 } 11688 11689 public TestScript addRule(TestScriptRuleComponent t) { //3 11690 if (t == null) 11691 return this; 11692 if (this.rule == null) 11693 this.rule = new ArrayList<TestScriptRuleComponent>(); 11694 this.rule.add(t); 11695 return this; 11696 } 11697 11698 /** 11699 * @return The first repetition of repeating field {@link #rule}, creating it if it does not already exist 11700 */ 11701 public TestScriptRuleComponent getRuleFirstRep() { 11702 if (getRule().isEmpty()) { 11703 addRule(); 11704 } 11705 return getRule().get(0); 11706 } 11707 11708 /** 11709 * @return {@link #ruleset} (Contains one or more rules. Offers a way to group rules so assertions could reference the group of rules and have them all applied.) 11710 */ 11711 public List<TestScriptRulesetComponent> getRuleset() { 11712 if (this.ruleset == null) 11713 this.ruleset = new ArrayList<TestScriptRulesetComponent>(); 11714 return this.ruleset; 11715 } 11716 11717 /** 11718 * @return Returns a reference to <code>this</code> for easy method chaining 11719 */ 11720 public TestScript setRuleset(List<TestScriptRulesetComponent> theRuleset) { 11721 this.ruleset = theRuleset; 11722 return this; 11723 } 11724 11725 public boolean hasRuleset() { 11726 if (this.ruleset == null) 11727 return false; 11728 for (TestScriptRulesetComponent item : this.ruleset) 11729 if (!item.isEmpty()) 11730 return true; 11731 return false; 11732 } 11733 11734 public TestScriptRulesetComponent addRuleset() { //3 11735 TestScriptRulesetComponent t = new TestScriptRulesetComponent(); 11736 if (this.ruleset == null) 11737 this.ruleset = new ArrayList<TestScriptRulesetComponent>(); 11738 this.ruleset.add(t); 11739 return t; 11740 } 11741 11742 public TestScript addRuleset(TestScriptRulesetComponent t) { //3 11743 if (t == null) 11744 return this; 11745 if (this.ruleset == null) 11746 this.ruleset = new ArrayList<TestScriptRulesetComponent>(); 11747 this.ruleset.add(t); 11748 return this; 11749 } 11750 11751 /** 11752 * @return The first repetition of repeating field {@link #ruleset}, creating it if it does not already exist 11753 */ 11754 public TestScriptRulesetComponent getRulesetFirstRep() { 11755 if (getRuleset().isEmpty()) { 11756 addRuleset(); 11757 } 11758 return getRuleset().get(0); 11759 } 11760 11761 /** 11762 * @return {@link #setup} (A series of required setup operations before tests are executed.) 11763 */ 11764 public TestScriptSetupComponent getSetup() { 11765 if (this.setup == null) 11766 if (Configuration.errorOnAutoCreate()) 11767 throw new Error("Attempt to auto-create TestScript.setup"); 11768 else if (Configuration.doAutoCreate()) 11769 this.setup = new TestScriptSetupComponent(); // cc 11770 return this.setup; 11771 } 11772 11773 public boolean hasSetup() { 11774 return this.setup != null && !this.setup.isEmpty(); 11775 } 11776 11777 /** 11778 * @param value {@link #setup} (A series of required setup operations before tests are executed.) 11779 */ 11780 public TestScript setSetup(TestScriptSetupComponent value) { 11781 this.setup = value; 11782 return this; 11783 } 11784 11785 /** 11786 * @return {@link #test} (A test in this script.) 11787 */ 11788 public List<TestScriptTestComponent> getTest() { 11789 if (this.test == null) 11790 this.test = new ArrayList<TestScriptTestComponent>(); 11791 return this.test; 11792 } 11793 11794 /** 11795 * @return Returns a reference to <code>this</code> for easy method chaining 11796 */ 11797 public TestScript setTest(List<TestScriptTestComponent> theTest) { 11798 this.test = theTest; 11799 return this; 11800 } 11801 11802 public boolean hasTest() { 11803 if (this.test == null) 11804 return false; 11805 for (TestScriptTestComponent item : this.test) 11806 if (!item.isEmpty()) 11807 return true; 11808 return false; 11809 } 11810 11811 public TestScriptTestComponent addTest() { //3 11812 TestScriptTestComponent t = new TestScriptTestComponent(); 11813 if (this.test == null) 11814 this.test = new ArrayList<TestScriptTestComponent>(); 11815 this.test.add(t); 11816 return t; 11817 } 11818 11819 public TestScript addTest(TestScriptTestComponent t) { //3 11820 if (t == null) 11821 return this; 11822 if (this.test == null) 11823 this.test = new ArrayList<TestScriptTestComponent>(); 11824 this.test.add(t); 11825 return this; 11826 } 11827 11828 /** 11829 * @return The first repetition of repeating field {@link #test}, creating it if it does not already exist 11830 */ 11831 public TestScriptTestComponent getTestFirstRep() { 11832 if (getTest().isEmpty()) { 11833 addTest(); 11834 } 11835 return getTest().get(0); 11836 } 11837 11838 /** 11839 * @return {@link #teardown} (A series of operations required to clean up after the all the tests are executed (successfully or otherwise).) 11840 */ 11841 public TestScriptTeardownComponent getTeardown() { 11842 if (this.teardown == null) 11843 if (Configuration.errorOnAutoCreate()) 11844 throw new Error("Attempt to auto-create TestScript.teardown"); 11845 else if (Configuration.doAutoCreate()) 11846 this.teardown = new TestScriptTeardownComponent(); // cc 11847 return this.teardown; 11848 } 11849 11850 public boolean hasTeardown() { 11851 return this.teardown != null && !this.teardown.isEmpty(); 11852 } 11853 11854 /** 11855 * @param value {@link #teardown} (A series of operations required to clean up after the all the tests are executed (successfully or otherwise).) 11856 */ 11857 public TestScript setTeardown(TestScriptTeardownComponent value) { 11858 this.teardown = value; 11859 return this; 11860 } 11861 11862 protected void listChildren(List<Property> children) { 11863 super.listChildren(children); 11864 children.add(new Property("url", "uri", "An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this test script is (or will be) published. The URL SHOULD include the major version of the test script. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 11865 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier)); 11866 children.add(new Property("version", "string", "The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 11867 children.add(new Property("name", "string", "A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 11868 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the test script.", 0, 1, title)); 11869 children.add(new Property("status", "code", "The status of this test script. Enables tracking the life-cycle of the content.", 0, 1, status)); 11870 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 11871 children.add(new Property("date", "dateTime", "The date (and optionally time) when the test script was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes.", 0, 1, date)); 11872 children.add(new Property("publisher", "string", "The name of the individual or organization that published the test script.", 0, 1, publisher)); 11873 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 11874 children.add(new Property("description", "markdown", "A free text natural language description of the test script from a consumer's perspective.", 0, 1, description)); 11875 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate test script instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 11876 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the test script is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 11877 children.add(new Property("purpose", "markdown", "Explaination of why this test script is needed and why it has been designed as it has.", 0, 1, purpose)); 11878 children.add(new Property("copyright", "markdown", "A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.", 0, 1, copyright)); 11879 children.add(new Property("origin", "", "An abstract server used in operations within this test script in the origin element.", 0, java.lang.Integer.MAX_VALUE, origin)); 11880 children.add(new Property("destination", "", "An abstract server used in operations within this test script in the destination element.", 0, java.lang.Integer.MAX_VALUE, destination)); 11881 children.add(new Property("metadata", "", "The required capability must exist and are assumed to function correctly on the FHIR server being tested.", 0, 1, metadata)); 11882 children.add(new Property("fixture", "", "Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.", 0, java.lang.Integer.MAX_VALUE, fixture)); 11883 children.add(new Property("profile", "Reference(Any)", "Reference to the profile to be used for validation.", 0, java.lang.Integer.MAX_VALUE, profile)); 11884 children.add(new Property("variable", "", "Variable is set based either on element value in response body or on header field value in the response headers.", 0, java.lang.Integer.MAX_VALUE, variable)); 11885 children.add(new Property("rule", "", "Assert rule to be used in one or more asserts within the test script.", 0, java.lang.Integer.MAX_VALUE, rule)); 11886 children.add(new Property("ruleset", "", "Contains one or more rules. Offers a way to group rules so assertions could reference the group of rules and have them all applied.", 0, java.lang.Integer.MAX_VALUE, ruleset)); 11887 children.add(new Property("setup", "", "A series of required setup operations before tests are executed.", 0, 1, setup)); 11888 children.add(new Property("test", "", "A test in this script.", 0, java.lang.Integer.MAX_VALUE, test)); 11889 children.add(new Property("teardown", "", "A series of operations required to clean up after the all the tests are executed (successfully or otherwise).", 0, 1, teardown)); 11890 } 11891 11892 @Override 11893 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11894 switch (_hash) { 11895 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this test script is (or will be) published. The URL SHOULD include the major version of the test script. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 11896 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier); 11897 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 11898 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 11899 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the test script.", 0, 1, title); 11900 case -892481550: /*status*/ return new Property("status", "code", "The status of this test script. Enables tracking the life-cycle of the content.", 0, 1, status); 11901 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 11902 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the test script was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes.", 0, 1, date); 11903 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the test script.", 0, 1, publisher); 11904 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 11905 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the test script from a consumer's perspective.", 0, 1, description); 11906 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate test script instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 11907 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the test script is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 11908 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this test script is needed and why it has been designed as it has.", 0, 1, purpose); 11909 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.", 0, 1, copyright); 11910 case -1008619738: /*origin*/ return new Property("origin", "", "An abstract server used in operations within this test script in the origin element.", 0, java.lang.Integer.MAX_VALUE, origin); 11911 case -1429847026: /*destination*/ return new Property("destination", "", "An abstract server used in operations within this test script in the destination element.", 0, java.lang.Integer.MAX_VALUE, destination); 11912 case -450004177: /*metadata*/ return new Property("metadata", "", "The required capability must exist and are assumed to function correctly on the FHIR server being tested.", 0, 1, metadata); 11913 case -843449847: /*fixture*/ return new Property("fixture", "", "Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.", 0, java.lang.Integer.MAX_VALUE, fixture); 11914 case -309425751: /*profile*/ return new Property("profile", "Reference(Any)", "Reference to the profile to be used for validation.", 0, java.lang.Integer.MAX_VALUE, profile); 11915 case -1249586564: /*variable*/ return new Property("variable", "", "Variable is set based either on element value in response body or on header field value in the response headers.", 0, java.lang.Integer.MAX_VALUE, variable); 11916 case 3512060: /*rule*/ return new Property("rule", "", "Assert rule to be used in one or more asserts within the test script.", 0, java.lang.Integer.MAX_VALUE, rule); 11917 case 1548678118: /*ruleset*/ return new Property("ruleset", "", "Contains one or more rules. Offers a way to group rules so assertions could reference the group of rules and have them all applied.", 0, java.lang.Integer.MAX_VALUE, ruleset); 11918 case 109329021: /*setup*/ return new Property("setup", "", "A series of required setup operations before tests are executed.", 0, 1, setup); 11919 case 3556498: /*test*/ return new Property("test", "", "A test in this script.", 0, java.lang.Integer.MAX_VALUE, test); 11920 case -1663474172: /*teardown*/ return new Property("teardown", "", "A series of operations required to clean up after the all the tests are executed (successfully or otherwise).", 0, 1, teardown); 11921 default: return super.getNamedProperty(_hash, _name, _checkValid); 11922 } 11923 11924 } 11925 11926 @Override 11927 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11928 switch (hash) { 11929 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 11930 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 11931 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 11932 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 11933 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 11934 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 11935 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 11936 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 11937 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 11938 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 11939 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 11940 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 11941 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 11942 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 11943 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 11944 case -1008619738: /*origin*/ return this.origin == null ? new Base[0] : this.origin.toArray(new Base[this.origin.size()]); // TestScriptOriginComponent 11945 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : this.destination.toArray(new Base[this.destination.size()]); // TestScriptDestinationComponent 11946 case -450004177: /*metadata*/ return this.metadata == null ? new Base[0] : new Base[] {this.metadata}; // TestScriptMetadataComponent 11947 case -843449847: /*fixture*/ return this.fixture == null ? new Base[0] : this.fixture.toArray(new Base[this.fixture.size()]); // TestScriptFixtureComponent 11948 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // Reference 11949 case -1249586564: /*variable*/ return this.variable == null ? new Base[0] : this.variable.toArray(new Base[this.variable.size()]); // TestScriptVariableComponent 11950 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // TestScriptRuleComponent 11951 case 1548678118: /*ruleset*/ return this.ruleset == null ? new Base[0] : this.ruleset.toArray(new Base[this.ruleset.size()]); // TestScriptRulesetComponent 11952 case 109329021: /*setup*/ return this.setup == null ? new Base[0] : new Base[] {this.setup}; // TestScriptSetupComponent 11953 case 3556498: /*test*/ return this.test == null ? new Base[0] : this.test.toArray(new Base[this.test.size()]); // TestScriptTestComponent 11954 case -1663474172: /*teardown*/ return this.teardown == null ? new Base[0] : new Base[] {this.teardown}; // TestScriptTeardownComponent 11955 default: return super.getProperty(hash, name, checkValid); 11956 } 11957 11958 } 11959 11960 @Override 11961 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11962 switch (hash) { 11963 case 116079: // url 11964 this.url = castToUri(value); // UriType 11965 return value; 11966 case -1618432855: // identifier 11967 this.identifier = castToIdentifier(value); // Identifier 11968 return value; 11969 case 351608024: // version 11970 this.version = castToString(value); // StringType 11971 return value; 11972 case 3373707: // name 11973 this.name = castToString(value); // StringType 11974 return value; 11975 case 110371416: // title 11976 this.title = castToString(value); // StringType 11977 return value; 11978 case -892481550: // status 11979 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 11980 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 11981 return value; 11982 case -404562712: // experimental 11983 this.experimental = castToBoolean(value); // BooleanType 11984 return value; 11985 case 3076014: // date 11986 this.date = castToDateTime(value); // DateTimeType 11987 return value; 11988 case 1447404028: // publisher 11989 this.publisher = castToString(value); // StringType 11990 return value; 11991 case 951526432: // contact 11992 this.getContact().add(castToContactDetail(value)); // ContactDetail 11993 return value; 11994 case -1724546052: // description 11995 this.description = castToMarkdown(value); // MarkdownType 11996 return value; 11997 case -669707736: // useContext 11998 this.getUseContext().add(castToUsageContext(value)); // UsageContext 11999 return value; 12000 case -507075711: // jurisdiction 12001 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 12002 return value; 12003 case -220463842: // purpose 12004 this.purpose = castToMarkdown(value); // MarkdownType 12005 return value; 12006 case 1522889671: // copyright 12007 this.copyright = castToMarkdown(value); // MarkdownType 12008 return value; 12009 case -1008619738: // origin 12010 this.getOrigin().add((TestScriptOriginComponent) value); // TestScriptOriginComponent 12011 return value; 12012 case -1429847026: // destination 12013 this.getDestination().add((TestScriptDestinationComponent) value); // TestScriptDestinationComponent 12014 return value; 12015 case -450004177: // metadata 12016 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 12017 return value; 12018 case -843449847: // fixture 12019 this.getFixture().add((TestScriptFixtureComponent) value); // TestScriptFixtureComponent 12020 return value; 12021 case -309425751: // profile 12022 this.getProfile().add(castToReference(value)); // Reference 12023 return value; 12024 case -1249586564: // variable 12025 this.getVariable().add((TestScriptVariableComponent) value); // TestScriptVariableComponent 12026 return value; 12027 case 3512060: // rule 12028 this.getRule().add((TestScriptRuleComponent) value); // TestScriptRuleComponent 12029 return value; 12030 case 1548678118: // ruleset 12031 this.getRuleset().add((TestScriptRulesetComponent) value); // TestScriptRulesetComponent 12032 return value; 12033 case 109329021: // setup 12034 this.setup = (TestScriptSetupComponent) value; // TestScriptSetupComponent 12035 return value; 12036 case 3556498: // test 12037 this.getTest().add((TestScriptTestComponent) value); // TestScriptTestComponent 12038 return value; 12039 case -1663474172: // teardown 12040 this.teardown = (TestScriptTeardownComponent) value; // TestScriptTeardownComponent 12041 return value; 12042 default: return super.setProperty(hash, name, value); 12043 } 12044 12045 } 12046 12047 @Override 12048 public Base setProperty(String name, Base value) throws FHIRException { 12049 if (name.equals("url")) { 12050 this.url = castToUri(value); // UriType 12051 } else if (name.equals("identifier")) { 12052 this.identifier = castToIdentifier(value); // Identifier 12053 } else if (name.equals("version")) { 12054 this.version = castToString(value); // StringType 12055 } else if (name.equals("name")) { 12056 this.name = castToString(value); // StringType 12057 } else if (name.equals("title")) { 12058 this.title = castToString(value); // StringType 12059 } else if (name.equals("status")) { 12060 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 12061 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 12062 } else if (name.equals("experimental")) { 12063 this.experimental = castToBoolean(value); // BooleanType 12064 } else if (name.equals("date")) { 12065 this.date = castToDateTime(value); // DateTimeType 12066 } else if (name.equals("publisher")) { 12067 this.publisher = castToString(value); // StringType 12068 } else if (name.equals("contact")) { 12069 this.getContact().add(castToContactDetail(value)); 12070 } else if (name.equals("description")) { 12071 this.description = castToMarkdown(value); // MarkdownType 12072 } else if (name.equals("useContext")) { 12073 this.getUseContext().add(castToUsageContext(value)); 12074 } else if (name.equals("jurisdiction")) { 12075 this.getJurisdiction().add(castToCodeableConcept(value)); 12076 } else if (name.equals("purpose")) { 12077 this.purpose = castToMarkdown(value); // MarkdownType 12078 } else if (name.equals("copyright")) { 12079 this.copyright = castToMarkdown(value); // MarkdownType 12080 } else if (name.equals("origin")) { 12081 this.getOrigin().add((TestScriptOriginComponent) value); 12082 } else if (name.equals("destination")) { 12083 this.getDestination().add((TestScriptDestinationComponent) value); 12084 } else if (name.equals("metadata")) { 12085 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 12086 } else if (name.equals("fixture")) { 12087 this.getFixture().add((TestScriptFixtureComponent) value); 12088 } else if (name.equals("profile")) { 12089 this.getProfile().add(castToReference(value)); 12090 } else if (name.equals("variable")) { 12091 this.getVariable().add((TestScriptVariableComponent) value); 12092 } else if (name.equals("rule")) { 12093 this.getRule().add((TestScriptRuleComponent) value); 12094 } else if (name.equals("ruleset")) { 12095 this.getRuleset().add((TestScriptRulesetComponent) value); 12096 } else if (name.equals("setup")) { 12097 this.setup = (TestScriptSetupComponent) value; // TestScriptSetupComponent 12098 } else if (name.equals("test")) { 12099 this.getTest().add((TestScriptTestComponent) value); 12100 } else if (name.equals("teardown")) { 12101 this.teardown = (TestScriptTeardownComponent) value; // TestScriptTeardownComponent 12102 } else 12103 return super.setProperty(name, value); 12104 return value; 12105 } 12106 12107 @Override 12108 public Base makeProperty(int hash, String name) throws FHIRException { 12109 switch (hash) { 12110 case 116079: return getUrlElement(); 12111 case -1618432855: return getIdentifier(); 12112 case 351608024: return getVersionElement(); 12113 case 3373707: return getNameElement(); 12114 case 110371416: return getTitleElement(); 12115 case -892481550: return getStatusElement(); 12116 case -404562712: return getExperimentalElement(); 12117 case 3076014: return getDateElement(); 12118 case 1447404028: return getPublisherElement(); 12119 case 951526432: return addContact(); 12120 case -1724546052: return getDescriptionElement(); 12121 case -669707736: return addUseContext(); 12122 case -507075711: return addJurisdiction(); 12123 case -220463842: return getPurposeElement(); 12124 case 1522889671: return getCopyrightElement(); 12125 case -1008619738: return addOrigin(); 12126 case -1429847026: return addDestination(); 12127 case -450004177: return getMetadata(); 12128 case -843449847: return addFixture(); 12129 case -309425751: return addProfile(); 12130 case -1249586564: return addVariable(); 12131 case 3512060: return addRule(); 12132 case 1548678118: return addRuleset(); 12133 case 109329021: return getSetup(); 12134 case 3556498: return addTest(); 12135 case -1663474172: return getTeardown(); 12136 default: return super.makeProperty(hash, name); 12137 } 12138 12139 } 12140 12141 @Override 12142 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12143 switch (hash) { 12144 case 116079: /*url*/ return new String[] {"uri"}; 12145 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 12146 case 351608024: /*version*/ return new String[] {"string"}; 12147 case 3373707: /*name*/ return new String[] {"string"}; 12148 case 110371416: /*title*/ return new String[] {"string"}; 12149 case -892481550: /*status*/ return new String[] {"code"}; 12150 case -404562712: /*experimental*/ return new String[] {"boolean"}; 12151 case 3076014: /*date*/ return new String[] {"dateTime"}; 12152 case 1447404028: /*publisher*/ return new String[] {"string"}; 12153 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 12154 case -1724546052: /*description*/ return new String[] {"markdown"}; 12155 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 12156 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 12157 case -220463842: /*purpose*/ return new String[] {"markdown"}; 12158 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 12159 case -1008619738: /*origin*/ return new String[] {}; 12160 case -1429847026: /*destination*/ return new String[] {}; 12161 case -450004177: /*metadata*/ return new String[] {}; 12162 case -843449847: /*fixture*/ return new String[] {}; 12163 case -309425751: /*profile*/ return new String[] {"Reference"}; 12164 case -1249586564: /*variable*/ return new String[] {}; 12165 case 3512060: /*rule*/ return new String[] {}; 12166 case 1548678118: /*ruleset*/ return new String[] {}; 12167 case 109329021: /*setup*/ return new String[] {}; 12168 case 3556498: /*test*/ return new String[] {}; 12169 case -1663474172: /*teardown*/ return new String[] {}; 12170 default: return super.getTypesForProperty(hash, name); 12171 } 12172 12173 } 12174 12175 @Override 12176 public Base addChild(String name) throws FHIRException { 12177 if (name.equals("url")) { 12178 throw new FHIRException("Cannot call addChild on a singleton property TestScript.url"); 12179 } 12180 else if (name.equals("identifier")) { 12181 this.identifier = new Identifier(); 12182 return this.identifier; 12183 } 12184 else if (name.equals("version")) { 12185 throw new FHIRException("Cannot call addChild on a singleton property TestScript.version"); 12186 } 12187 else if (name.equals("name")) { 12188 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 12189 } 12190 else if (name.equals("title")) { 12191 throw new FHIRException("Cannot call addChild on a singleton property TestScript.title"); 12192 } 12193 else if (name.equals("status")) { 12194 throw new FHIRException("Cannot call addChild on a singleton property TestScript.status"); 12195 } 12196 else if (name.equals("experimental")) { 12197 throw new FHIRException("Cannot call addChild on a singleton property TestScript.experimental"); 12198 } 12199 else if (name.equals("date")) { 12200 throw new FHIRException("Cannot call addChild on a singleton property TestScript.date"); 12201 } 12202 else if (name.equals("publisher")) { 12203 throw new FHIRException("Cannot call addChild on a singleton property TestScript.publisher"); 12204 } 12205 else if (name.equals("contact")) { 12206 return addContact(); 12207 } 12208 else if (name.equals("description")) { 12209 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 12210 } 12211 else if (name.equals("useContext")) { 12212 return addUseContext(); 12213 } 12214 else if (name.equals("jurisdiction")) { 12215 return addJurisdiction(); 12216 } 12217 else if (name.equals("purpose")) { 12218 throw new FHIRException("Cannot call addChild on a singleton property TestScript.purpose"); 12219 } 12220 else if (name.equals("copyright")) { 12221 throw new FHIRException("Cannot call addChild on a singleton property TestScript.copyright"); 12222 } 12223 else if (name.equals("origin")) { 12224 return addOrigin(); 12225 } 12226 else if (name.equals("destination")) { 12227 return addDestination(); 12228 } 12229 else if (name.equals("metadata")) { 12230 this.metadata = new TestScriptMetadataComponent(); 12231 return this.metadata; 12232 } 12233 else if (name.equals("fixture")) { 12234 return addFixture(); 12235 } 12236 else if (name.equals("profile")) { 12237 return addProfile(); 12238 } 12239 else if (name.equals("variable")) { 12240 return addVariable(); 12241 } 12242 else if (name.equals("rule")) { 12243 return addRule(); 12244 } 12245 else if (name.equals("ruleset")) { 12246 return addRuleset(); 12247 } 12248 else if (name.equals("setup")) { 12249 this.setup = new TestScriptSetupComponent(); 12250 return this.setup; 12251 } 12252 else if (name.equals("test")) { 12253 return addTest(); 12254 } 12255 else if (name.equals("teardown")) { 12256 this.teardown = new TestScriptTeardownComponent(); 12257 return this.teardown; 12258 } 12259 else 12260 return super.addChild(name); 12261 } 12262 12263 public String fhirType() { 12264 return "TestScript"; 12265 12266 } 12267 12268 public TestScript copy() { 12269 TestScript dst = new TestScript(); 12270 copyValues(dst); 12271 dst.url = url == null ? null : url.copy(); 12272 dst.identifier = identifier == null ? null : identifier.copy(); 12273 dst.version = version == null ? null : version.copy(); 12274 dst.name = name == null ? null : name.copy(); 12275 dst.title = title == null ? null : title.copy(); 12276 dst.status = status == null ? null : status.copy(); 12277 dst.experimental = experimental == null ? null : experimental.copy(); 12278 dst.date = date == null ? null : date.copy(); 12279 dst.publisher = publisher == null ? null : publisher.copy(); 12280 if (contact != null) { 12281 dst.contact = new ArrayList<ContactDetail>(); 12282 for (ContactDetail i : contact) 12283 dst.contact.add(i.copy()); 12284 }; 12285 dst.description = description == null ? null : description.copy(); 12286 if (useContext != null) { 12287 dst.useContext = new ArrayList<UsageContext>(); 12288 for (UsageContext i : useContext) 12289 dst.useContext.add(i.copy()); 12290 }; 12291 if (jurisdiction != null) { 12292 dst.jurisdiction = new ArrayList<CodeableConcept>(); 12293 for (CodeableConcept i : jurisdiction) 12294 dst.jurisdiction.add(i.copy()); 12295 }; 12296 dst.purpose = purpose == null ? null : purpose.copy(); 12297 dst.copyright = copyright == null ? null : copyright.copy(); 12298 if (origin != null) { 12299 dst.origin = new ArrayList<TestScriptOriginComponent>(); 12300 for (TestScriptOriginComponent i : origin) 12301 dst.origin.add(i.copy()); 12302 }; 12303 if (destination != null) { 12304 dst.destination = new ArrayList<TestScriptDestinationComponent>(); 12305 for (TestScriptDestinationComponent i : destination) 12306 dst.destination.add(i.copy()); 12307 }; 12308 dst.metadata = metadata == null ? null : metadata.copy(); 12309 if (fixture != null) { 12310 dst.fixture = new ArrayList<TestScriptFixtureComponent>(); 12311 for (TestScriptFixtureComponent i : fixture) 12312 dst.fixture.add(i.copy()); 12313 }; 12314 if (profile != null) { 12315 dst.profile = new ArrayList<Reference>(); 12316 for (Reference i : profile) 12317 dst.profile.add(i.copy()); 12318 }; 12319 if (variable != null) { 12320 dst.variable = new ArrayList<TestScriptVariableComponent>(); 12321 for (TestScriptVariableComponent i : variable) 12322 dst.variable.add(i.copy()); 12323 }; 12324 if (rule != null) { 12325 dst.rule = new ArrayList<TestScriptRuleComponent>(); 12326 for (TestScriptRuleComponent i : rule) 12327 dst.rule.add(i.copy()); 12328 }; 12329 if (ruleset != null) { 12330 dst.ruleset = new ArrayList<TestScriptRulesetComponent>(); 12331 for (TestScriptRulesetComponent i : ruleset) 12332 dst.ruleset.add(i.copy()); 12333 }; 12334 dst.setup = setup == null ? null : setup.copy(); 12335 if (test != null) { 12336 dst.test = new ArrayList<TestScriptTestComponent>(); 12337 for (TestScriptTestComponent i : test) 12338 dst.test.add(i.copy()); 12339 }; 12340 dst.teardown = teardown == null ? null : teardown.copy(); 12341 return dst; 12342 } 12343 12344 protected TestScript typedCopy() { 12345 return copy(); 12346 } 12347 12348 @Override 12349 public boolean equalsDeep(Base other_) { 12350 if (!super.equalsDeep(other_)) 12351 return false; 12352 if (!(other_ instanceof TestScript)) 12353 return false; 12354 TestScript o = (TestScript) other_; 12355 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 12356 && compareDeep(origin, o.origin, true) && compareDeep(destination, o.destination, true) && compareDeep(metadata, o.metadata, true) 12357 && compareDeep(fixture, o.fixture, true) && compareDeep(profile, o.profile, true) && compareDeep(variable, o.variable, true) 12358 && compareDeep(rule, o.rule, true) && compareDeep(ruleset, o.ruleset, true) && compareDeep(setup, o.setup, true) 12359 && compareDeep(test, o.test, true) && compareDeep(teardown, o.teardown, true); 12360 } 12361 12362 @Override 12363 public boolean equalsShallow(Base other_) { 12364 if (!super.equalsShallow(other_)) 12365 return false; 12366 if (!(other_ instanceof TestScript)) 12367 return false; 12368 TestScript o = (TestScript) other_; 12369 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true); 12370 } 12371 12372 public boolean isEmpty() { 12373 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright 12374 , origin, destination, metadata, fixture, profile, variable, rule, ruleset, setup 12375 , test, teardown); 12376 } 12377 12378 @Override 12379 public ResourceType getResourceType() { 12380 return ResourceType.TestScript; 12381 } 12382 12383 /** 12384 * Search parameter: <b>date</b> 12385 * <p> 12386 * Description: <b>The test script publication date</b><br> 12387 * Type: <b>date</b><br> 12388 * Path: <b>TestScript.date</b><br> 12389 * </p> 12390 */ 12391 @SearchParamDefinition(name="date", path="TestScript.date", description="The test script publication date", type="date" ) 12392 public static final String SP_DATE = "date"; 12393 /** 12394 * <b>Fluent Client</b> search parameter constant for <b>date</b> 12395 * <p> 12396 * Description: <b>The test script publication date</b><br> 12397 * Type: <b>date</b><br> 12398 * Path: <b>TestScript.date</b><br> 12399 * </p> 12400 */ 12401 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 12402 12403 /** 12404 * Search parameter: <b>identifier</b> 12405 * <p> 12406 * Description: <b>External identifier for the test script</b><br> 12407 * Type: <b>token</b><br> 12408 * Path: <b>TestScript.identifier</b><br> 12409 * </p> 12410 */ 12411 @SearchParamDefinition(name="identifier", path="TestScript.identifier", description="External identifier for the test script", type="token" ) 12412 public static final String SP_IDENTIFIER = "identifier"; 12413 /** 12414 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 12415 * <p> 12416 * Description: <b>External identifier for the test script</b><br> 12417 * Type: <b>token</b><br> 12418 * Path: <b>TestScript.identifier</b><br> 12419 * </p> 12420 */ 12421 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 12422 12423 /** 12424 * Search parameter: <b>jurisdiction</b> 12425 * <p> 12426 * Description: <b>Intended jurisdiction for the test script</b><br> 12427 * Type: <b>token</b><br> 12428 * Path: <b>TestScript.jurisdiction</b><br> 12429 * </p> 12430 */ 12431 @SearchParamDefinition(name="jurisdiction", path="TestScript.jurisdiction", description="Intended jurisdiction for the test script", type="token" ) 12432 public static final String SP_JURISDICTION = "jurisdiction"; 12433 /** 12434 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 12435 * <p> 12436 * Description: <b>Intended jurisdiction for the test script</b><br> 12437 * Type: <b>token</b><br> 12438 * Path: <b>TestScript.jurisdiction</b><br> 12439 * </p> 12440 */ 12441 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 12442 12443 /** 12444 * Search parameter: <b>name</b> 12445 * <p> 12446 * Description: <b>Computationally friendly name of the test script</b><br> 12447 * Type: <b>string</b><br> 12448 * Path: <b>TestScript.name</b><br> 12449 * </p> 12450 */ 12451 @SearchParamDefinition(name="name", path="TestScript.name", description="Computationally friendly name of the test script", type="string" ) 12452 public static final String SP_NAME = "name"; 12453 /** 12454 * <b>Fluent Client</b> search parameter constant for <b>name</b> 12455 * <p> 12456 * Description: <b>Computationally friendly name of the test script</b><br> 12457 * Type: <b>string</b><br> 12458 * Path: <b>TestScript.name</b><br> 12459 * </p> 12460 */ 12461 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 12462 12463 /** 12464 * Search parameter: <b>description</b> 12465 * <p> 12466 * Description: <b>The description of the test script</b><br> 12467 * Type: <b>string</b><br> 12468 * Path: <b>TestScript.description</b><br> 12469 * </p> 12470 */ 12471 @SearchParamDefinition(name="description", path="TestScript.description", description="The description of the test script", type="string" ) 12472 public static final String SP_DESCRIPTION = "description"; 12473 /** 12474 * <b>Fluent Client</b> search parameter constant for <b>description</b> 12475 * <p> 12476 * Description: <b>The description of the test script</b><br> 12477 * Type: <b>string</b><br> 12478 * Path: <b>TestScript.description</b><br> 12479 * </p> 12480 */ 12481 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 12482 12483 /** 12484 * Search parameter: <b>publisher</b> 12485 * <p> 12486 * Description: <b>Name of the publisher of the test script</b><br> 12487 * Type: <b>string</b><br> 12488 * Path: <b>TestScript.publisher</b><br> 12489 * </p> 12490 */ 12491 @SearchParamDefinition(name="publisher", path="TestScript.publisher", description="Name of the publisher of the test script", type="string" ) 12492 public static final String SP_PUBLISHER = "publisher"; 12493 /** 12494 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 12495 * <p> 12496 * Description: <b>Name of the publisher of the test script</b><br> 12497 * Type: <b>string</b><br> 12498 * Path: <b>TestScript.publisher</b><br> 12499 * </p> 12500 */ 12501 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 12502 12503 /** 12504 * Search parameter: <b>testscript-capability</b> 12505 * <p> 12506 * Description: <b>TestScript required and validated capability</b><br> 12507 * Type: <b>string</b><br> 12508 * Path: <b>TestScript.metadata.capability.description</b><br> 12509 * </p> 12510 */ 12511 @SearchParamDefinition(name="testscript-capability", path="TestScript.metadata.capability.description", description="TestScript required and validated capability", type="string" ) 12512 public static final String SP_TESTSCRIPT_CAPABILITY = "testscript-capability"; 12513 /** 12514 * <b>Fluent Client</b> search parameter constant for <b>testscript-capability</b> 12515 * <p> 12516 * Description: <b>TestScript required and validated capability</b><br> 12517 * Type: <b>string</b><br> 12518 * Path: <b>TestScript.metadata.capability.description</b><br> 12519 * </p> 12520 */ 12521 public static final ca.uhn.fhir.rest.gclient.StringClientParam TESTSCRIPT_CAPABILITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TESTSCRIPT_CAPABILITY); 12522 12523 /** 12524 * Search parameter: <b>title</b> 12525 * <p> 12526 * Description: <b>The human-friendly name of the test script</b><br> 12527 * Type: <b>string</b><br> 12528 * Path: <b>TestScript.title</b><br> 12529 * </p> 12530 */ 12531 @SearchParamDefinition(name="title", path="TestScript.title", description="The human-friendly name of the test script", type="string" ) 12532 public static final String SP_TITLE = "title"; 12533 /** 12534 * <b>Fluent Client</b> search parameter constant for <b>title</b> 12535 * <p> 12536 * Description: <b>The human-friendly name of the test script</b><br> 12537 * Type: <b>string</b><br> 12538 * Path: <b>TestScript.title</b><br> 12539 * </p> 12540 */ 12541 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 12542 12543 /** 12544 * Search parameter: <b>version</b> 12545 * <p> 12546 * Description: <b>The business version of the test script</b><br> 12547 * Type: <b>token</b><br> 12548 * Path: <b>TestScript.version</b><br> 12549 * </p> 12550 */ 12551 @SearchParamDefinition(name="version", path="TestScript.version", description="The business version of the test script", type="token" ) 12552 public static final String SP_VERSION = "version"; 12553 /** 12554 * <b>Fluent Client</b> search parameter constant for <b>version</b> 12555 * <p> 12556 * Description: <b>The business version of the test script</b><br> 12557 * Type: <b>token</b><br> 12558 * Path: <b>TestScript.version</b><br> 12559 * </p> 12560 */ 12561 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 12562 12563 /** 12564 * Search parameter: <b>url</b> 12565 * <p> 12566 * Description: <b>The uri that identifies the test script</b><br> 12567 * Type: <b>uri</b><br> 12568 * Path: <b>TestScript.url</b><br> 12569 * </p> 12570 */ 12571 @SearchParamDefinition(name="url", path="TestScript.url", description="The uri that identifies the test script", type="uri" ) 12572 public static final String SP_URL = "url"; 12573 /** 12574 * <b>Fluent Client</b> search parameter constant for <b>url</b> 12575 * <p> 12576 * Description: <b>The uri that identifies the test script</b><br> 12577 * Type: <b>uri</b><br> 12578 * Path: <b>TestScript.url</b><br> 12579 * </p> 12580 */ 12581 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 12582 12583 /** 12584 * Search parameter: <b>status</b> 12585 * <p> 12586 * Description: <b>The current status of the test script</b><br> 12587 * Type: <b>token</b><br> 12588 * Path: <b>TestScript.status</b><br> 12589 * </p> 12590 */ 12591 @SearchParamDefinition(name="status", path="TestScript.status", description="The current status of the test script", type="token" ) 12592 public static final String SP_STATUS = "status"; 12593 /** 12594 * <b>Fluent Client</b> search parameter constant for <b>status</b> 12595 * <p> 12596 * Description: <b>The current status of the test script</b><br> 12597 * Type: <b>token</b><br> 12598 * Path: <b>TestScript.status</b><br> 12599 * </p> 12600 */ 12601 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 12602 12603 12604}