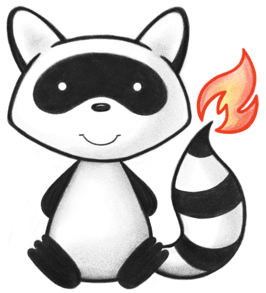
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006import java.math.BigDecimal; 007 008/* 009 Copyright (c) 2011+, HL7, Inc. 010 All rights reserved. 011 012 Redistribution and use in source and binary forms, with or without modification, 013 are permitted provided that the following conditions are met: 014 015 * Redistributions of source code must retain the above copyright notice, this 016 list of conditions and the following disclaimer. 017 * Redistributions in binary form must reproduce the above copyright notice, 018 this list of conditions and the following disclaimer in the documentation 019 and/or other materials provided with the distribution. 020 * Neither the name of HL7 nor the names of its contributors may be used to 021 endorse or promote products derived from this software without specific 022 prior written permission. 023 024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 025 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 027 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 028 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 029 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 030 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 031 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 032 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 033 POSSIBILITY OF SUCH DAMAGE. 034 035*/ 036 037// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 038import java.util.ArrayList; 039import java.util.Date; 040import java.util.List; 041 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.exceptions.FHIRFormatError; 044import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 045import org.hl7.fhir.instance.model.api.ICompositeType; 046 047import ca.uhn.fhir.model.api.annotation.Block; 048import ca.uhn.fhir.model.api.annotation.Child; 049import ca.uhn.fhir.model.api.annotation.DatatypeDef; 050import ca.uhn.fhir.model.api.annotation.Description; 051/** 052 * Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out. 053 */ 054@DatatypeDef(name="Timing") 055public class Timing extends Type implements ICompositeType { 056 057 public enum UnitsOfTime { 058 /** 059 * null 060 */ 061 S, 062 /** 063 * null 064 */ 065 MIN, 066 /** 067 * null 068 */ 069 H, 070 /** 071 * null 072 */ 073 D, 074 /** 075 * null 076 */ 077 WK, 078 /** 079 * null 080 */ 081 MO, 082 /** 083 * null 084 */ 085 A, 086 /** 087 * added to help the parsers with the generic types 088 */ 089 NULL; 090 public static UnitsOfTime fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("s".equals(codeString)) 094 return S; 095 if ("min".equals(codeString)) 096 return MIN; 097 if ("h".equals(codeString)) 098 return H; 099 if ("d".equals(codeString)) 100 return D; 101 if ("wk".equals(codeString)) 102 return WK; 103 if ("mo".equals(codeString)) 104 return MO; 105 if ("a".equals(codeString)) 106 return A; 107 if (Configuration.isAcceptInvalidEnums()) 108 return null; 109 else 110 throw new FHIRException("Unknown UnitsOfTime code '"+codeString+"'"); 111 } 112 public String toCode() { 113 switch (this) { 114 case S: return "s"; 115 case MIN: return "min"; 116 case H: return "h"; 117 case D: return "d"; 118 case WK: return "wk"; 119 case MO: return "mo"; 120 case A: return "a"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 public String getSystem() { 126 switch (this) { 127 case S: return "http://unitsofmeasure.org"; 128 case MIN: return "http://unitsofmeasure.org"; 129 case H: return "http://unitsofmeasure.org"; 130 case D: return "http://unitsofmeasure.org"; 131 case WK: return "http://unitsofmeasure.org"; 132 case MO: return "http://unitsofmeasure.org"; 133 case A: return "http://unitsofmeasure.org"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getDefinition() { 139 switch (this) { 140 case S: return ""; 141 case MIN: return ""; 142 case H: return ""; 143 case D: return ""; 144 case WK: return ""; 145 case MO: return ""; 146 case A: return ""; 147 case NULL: return null; 148 default: return "?"; 149 } 150 } 151 public String getDisplay() { 152 switch (this) { 153 case S: return "second"; 154 case MIN: return "minute"; 155 case H: return "hour"; 156 case D: return "day"; 157 case WK: return "week"; 158 case MO: return "month"; 159 case A: return "year"; 160 case NULL: return null; 161 default: return "?"; 162 } 163 } 164 } 165 166 public static class UnitsOfTimeEnumFactory implements EnumFactory<UnitsOfTime> { 167 public UnitsOfTime fromCode(String codeString) throws IllegalArgumentException { 168 if (codeString == null || "".equals(codeString)) 169 if (codeString == null || "".equals(codeString)) 170 return null; 171 if ("s".equals(codeString)) 172 return UnitsOfTime.S; 173 if ("min".equals(codeString)) 174 return UnitsOfTime.MIN; 175 if ("h".equals(codeString)) 176 return UnitsOfTime.H; 177 if ("d".equals(codeString)) 178 return UnitsOfTime.D; 179 if ("wk".equals(codeString)) 180 return UnitsOfTime.WK; 181 if ("mo".equals(codeString)) 182 return UnitsOfTime.MO; 183 if ("a".equals(codeString)) 184 return UnitsOfTime.A; 185 throw new IllegalArgumentException("Unknown UnitsOfTime code '"+codeString+"'"); 186 } 187 public Enumeration<UnitsOfTime> fromType(PrimitiveType<?> code) throws FHIRException { 188 if (code == null) 189 return null; 190 if (code.isEmpty()) 191 return new Enumeration<UnitsOfTime>(this); 192 String codeString = code.asStringValue(); 193 if (codeString == null || "".equals(codeString)) 194 return null; 195 if ("s".equals(codeString)) 196 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.S); 197 if ("min".equals(codeString)) 198 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.MIN); 199 if ("h".equals(codeString)) 200 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.H); 201 if ("d".equals(codeString)) 202 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.D); 203 if ("wk".equals(codeString)) 204 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.WK); 205 if ("mo".equals(codeString)) 206 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.MO); 207 if ("a".equals(codeString)) 208 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.A); 209 throw new FHIRException("Unknown UnitsOfTime code '"+codeString+"'"); 210 } 211 public String toCode(UnitsOfTime code) { 212 if (code == UnitsOfTime.NULL) 213 return null; 214 if (code == UnitsOfTime.S) 215 return "s"; 216 if (code == UnitsOfTime.MIN) 217 return "min"; 218 if (code == UnitsOfTime.H) 219 return "h"; 220 if (code == UnitsOfTime.D) 221 return "d"; 222 if (code == UnitsOfTime.WK) 223 return "wk"; 224 if (code == UnitsOfTime.MO) 225 return "mo"; 226 if (code == UnitsOfTime.A) 227 return "a"; 228 return "?"; 229 } 230 public String toSystem(UnitsOfTime code) { 231 return code.getSystem(); 232 } 233 } 234 235 public enum DayOfWeek { 236 /** 237 * Monday 238 */ 239 MON, 240 /** 241 * Tuesday 242 */ 243 TUE, 244 /** 245 * Wednesday 246 */ 247 WED, 248 /** 249 * Thursday 250 */ 251 THU, 252 /** 253 * Friday 254 */ 255 FRI, 256 /** 257 * Saturday 258 */ 259 SAT, 260 /** 261 * Sunday 262 */ 263 SUN, 264 /** 265 * added to help the parsers with the generic types 266 */ 267 NULL; 268 public static DayOfWeek fromCode(String codeString) throws FHIRException { 269 if (codeString == null || "".equals(codeString)) 270 return null; 271 if ("mon".equals(codeString)) 272 return MON; 273 if ("tue".equals(codeString)) 274 return TUE; 275 if ("wed".equals(codeString)) 276 return WED; 277 if ("thu".equals(codeString)) 278 return THU; 279 if ("fri".equals(codeString)) 280 return FRI; 281 if ("sat".equals(codeString)) 282 return SAT; 283 if ("sun".equals(codeString)) 284 return SUN; 285 if (Configuration.isAcceptInvalidEnums()) 286 return null; 287 else 288 throw new FHIRException("Unknown DayOfWeek code '"+codeString+"'"); 289 } 290 public String toCode() { 291 switch (this) { 292 case MON: return "mon"; 293 case TUE: return "tue"; 294 case WED: return "wed"; 295 case THU: return "thu"; 296 case FRI: return "fri"; 297 case SAT: return "sat"; 298 case SUN: return "sun"; 299 case NULL: return null; 300 default: return "?"; 301 } 302 } 303 public String getSystem() { 304 switch (this) { 305 case MON: return "http://hl7.org/fhir/days-of-week"; 306 case TUE: return "http://hl7.org/fhir/days-of-week"; 307 case WED: return "http://hl7.org/fhir/days-of-week"; 308 case THU: return "http://hl7.org/fhir/days-of-week"; 309 case FRI: return "http://hl7.org/fhir/days-of-week"; 310 case SAT: return "http://hl7.org/fhir/days-of-week"; 311 case SUN: return "http://hl7.org/fhir/days-of-week"; 312 case NULL: return null; 313 default: return "?"; 314 } 315 } 316 public String getDefinition() { 317 switch (this) { 318 case MON: return "Monday"; 319 case TUE: return "Tuesday"; 320 case WED: return "Wednesday"; 321 case THU: return "Thursday"; 322 case FRI: return "Friday"; 323 case SAT: return "Saturday"; 324 case SUN: return "Sunday"; 325 case NULL: return null; 326 default: return "?"; 327 } 328 } 329 public String getDisplay() { 330 switch (this) { 331 case MON: return "Monday"; 332 case TUE: return "Tuesday"; 333 case WED: return "Wednesday"; 334 case THU: return "Thursday"; 335 case FRI: return "Friday"; 336 case SAT: return "Saturday"; 337 case SUN: return "Sunday"; 338 case NULL: return null; 339 default: return "?"; 340 } 341 } 342 } 343 344 public static class DayOfWeekEnumFactory implements EnumFactory<DayOfWeek> { 345 public DayOfWeek fromCode(String codeString) throws IllegalArgumentException { 346 if (codeString == null || "".equals(codeString)) 347 if (codeString == null || "".equals(codeString)) 348 return null; 349 if ("mon".equals(codeString)) 350 return DayOfWeek.MON; 351 if ("tue".equals(codeString)) 352 return DayOfWeek.TUE; 353 if ("wed".equals(codeString)) 354 return DayOfWeek.WED; 355 if ("thu".equals(codeString)) 356 return DayOfWeek.THU; 357 if ("fri".equals(codeString)) 358 return DayOfWeek.FRI; 359 if ("sat".equals(codeString)) 360 return DayOfWeek.SAT; 361 if ("sun".equals(codeString)) 362 return DayOfWeek.SUN; 363 throw new IllegalArgumentException("Unknown DayOfWeek code '"+codeString+"'"); 364 } 365 public Enumeration<DayOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 366 if (code == null) 367 return null; 368 if (code.isEmpty()) 369 return new Enumeration<DayOfWeek>(this); 370 String codeString = code.asStringValue(); 371 if (codeString == null || "".equals(codeString)) 372 return null; 373 if ("mon".equals(codeString)) 374 return new Enumeration<DayOfWeek>(this, DayOfWeek.MON); 375 if ("tue".equals(codeString)) 376 return new Enumeration<DayOfWeek>(this, DayOfWeek.TUE); 377 if ("wed".equals(codeString)) 378 return new Enumeration<DayOfWeek>(this, DayOfWeek.WED); 379 if ("thu".equals(codeString)) 380 return new Enumeration<DayOfWeek>(this, DayOfWeek.THU); 381 if ("fri".equals(codeString)) 382 return new Enumeration<DayOfWeek>(this, DayOfWeek.FRI); 383 if ("sat".equals(codeString)) 384 return new Enumeration<DayOfWeek>(this, DayOfWeek.SAT); 385 if ("sun".equals(codeString)) 386 return new Enumeration<DayOfWeek>(this, DayOfWeek.SUN); 387 throw new FHIRException("Unknown DayOfWeek code '"+codeString+"'"); 388 } 389 public String toCode(DayOfWeek code) { 390 if (code == DayOfWeek.NULL) 391 return null; 392 if (code == DayOfWeek.MON) 393 return "mon"; 394 if (code == DayOfWeek.TUE) 395 return "tue"; 396 if (code == DayOfWeek.WED) 397 return "wed"; 398 if (code == DayOfWeek.THU) 399 return "thu"; 400 if (code == DayOfWeek.FRI) 401 return "fri"; 402 if (code == DayOfWeek.SAT) 403 return "sat"; 404 if (code == DayOfWeek.SUN) 405 return "sun"; 406 return "?"; 407 } 408 public String toSystem(DayOfWeek code) { 409 return code.getSystem(); 410 } 411 } 412 413 public enum EventTiming { 414 /** 415 * event occurs during the morning 416 */ 417 MORN, 418 /** 419 * event occurs during the afternoon 420 */ 421 AFT, 422 /** 423 * event occurs during the evening 424 */ 425 EVE, 426 /** 427 * event occurs during the night 428 */ 429 NIGHT, 430 /** 431 * event occurs [offset] after subject goes to sleep 432 */ 433 PHS, 434 /** 435 * null 436 */ 437 HS, 438 /** 439 * null 440 */ 441 WAKE, 442 /** 443 * null 444 */ 445 C, 446 /** 447 * null 448 */ 449 CM, 450 /** 451 * null 452 */ 453 CD, 454 /** 455 * null 456 */ 457 CV, 458 /** 459 * null 460 */ 461 AC, 462 /** 463 * null 464 */ 465 ACM, 466 /** 467 * null 468 */ 469 ACD, 470 /** 471 * null 472 */ 473 ACV, 474 /** 475 * null 476 */ 477 PC, 478 /** 479 * null 480 */ 481 PCM, 482 /** 483 * null 484 */ 485 PCD, 486 /** 487 * null 488 */ 489 PCV, 490 /** 491 * added to help the parsers with the generic types 492 */ 493 NULL; 494 public static EventTiming fromCode(String codeString) throws FHIRException { 495 if (codeString == null || "".equals(codeString)) 496 return null; 497 if ("MORN".equals(codeString)) 498 return MORN; 499 if ("AFT".equals(codeString)) 500 return AFT; 501 if ("EVE".equals(codeString)) 502 return EVE; 503 if ("NIGHT".equals(codeString)) 504 return NIGHT; 505 if ("PHS".equals(codeString)) 506 return PHS; 507 if ("HS".equals(codeString)) 508 return HS; 509 if ("WAKE".equals(codeString)) 510 return WAKE; 511 if ("C".equals(codeString)) 512 return C; 513 if ("CM".equals(codeString)) 514 return CM; 515 if ("CD".equals(codeString)) 516 return CD; 517 if ("CV".equals(codeString)) 518 return CV; 519 if ("AC".equals(codeString)) 520 return AC; 521 if ("ACM".equals(codeString)) 522 return ACM; 523 if ("ACD".equals(codeString)) 524 return ACD; 525 if ("ACV".equals(codeString)) 526 return ACV; 527 if ("PC".equals(codeString)) 528 return PC; 529 if ("PCM".equals(codeString)) 530 return PCM; 531 if ("PCD".equals(codeString)) 532 return PCD; 533 if ("PCV".equals(codeString)) 534 return PCV; 535 if (Configuration.isAcceptInvalidEnums()) 536 return null; 537 else 538 throw new FHIRException("Unknown EventTiming code '"+codeString+"'"); 539 } 540 public String toCode() { 541 switch (this) { 542 case MORN: return "MORN"; 543 case AFT: return "AFT"; 544 case EVE: return "EVE"; 545 case NIGHT: return "NIGHT"; 546 case PHS: return "PHS"; 547 case HS: return "HS"; 548 case WAKE: return "WAKE"; 549 case C: return "C"; 550 case CM: return "CM"; 551 case CD: return "CD"; 552 case CV: return "CV"; 553 case AC: return "AC"; 554 case ACM: return "ACM"; 555 case ACD: return "ACD"; 556 case ACV: return "ACV"; 557 case PC: return "PC"; 558 case PCM: return "PCM"; 559 case PCD: return "PCD"; 560 case PCV: return "PCV"; 561 case NULL: return null; 562 default: return "?"; 563 } 564 } 565 public String getSystem() { 566 switch (this) { 567 case MORN: return "http://hl7.org/fhir/event-timing"; 568 case AFT: return "http://hl7.org/fhir/event-timing"; 569 case EVE: return "http://hl7.org/fhir/event-timing"; 570 case NIGHT: return "http://hl7.org/fhir/event-timing"; 571 case PHS: return "http://hl7.org/fhir/event-timing"; 572 case HS: return "http://hl7.org/fhir/v3/TimingEvent"; 573 case WAKE: return "http://hl7.org/fhir/v3/TimingEvent"; 574 case C: return "http://hl7.org/fhir/v3/TimingEvent"; 575 case CM: return "http://hl7.org/fhir/v3/TimingEvent"; 576 case CD: return "http://hl7.org/fhir/v3/TimingEvent"; 577 case CV: return "http://hl7.org/fhir/v3/TimingEvent"; 578 case AC: return "http://hl7.org/fhir/v3/TimingEvent"; 579 case ACM: return "http://hl7.org/fhir/v3/TimingEvent"; 580 case ACD: return "http://hl7.org/fhir/v3/TimingEvent"; 581 case ACV: return "http://hl7.org/fhir/v3/TimingEvent"; 582 case PC: return "http://hl7.org/fhir/v3/TimingEvent"; 583 case PCM: return "http://hl7.org/fhir/v3/TimingEvent"; 584 case PCD: return "http://hl7.org/fhir/v3/TimingEvent"; 585 case PCV: return "http://hl7.org/fhir/v3/TimingEvent"; 586 case NULL: return null; 587 default: return "?"; 588 } 589 } 590 public String getDefinition() { 591 switch (this) { 592 case MORN: return "event occurs during the morning"; 593 case AFT: return "event occurs during the afternoon"; 594 case EVE: return "event occurs during the evening"; 595 case NIGHT: return "event occurs during the night"; 596 case PHS: return "event occurs [offset] after subject goes to sleep"; 597 case HS: return ""; 598 case WAKE: return ""; 599 case C: return ""; 600 case CM: return ""; 601 case CD: return ""; 602 case CV: return ""; 603 case AC: return ""; 604 case ACM: return ""; 605 case ACD: return ""; 606 case ACV: return ""; 607 case PC: return ""; 608 case PCM: return ""; 609 case PCD: return ""; 610 case PCV: return ""; 611 case NULL: return null; 612 default: return "?"; 613 } 614 } 615 public String getDisplay() { 616 switch (this) { 617 case MORN: return "Morning"; 618 case AFT: return "Afternoon"; 619 case EVE: return "Evening"; 620 case NIGHT: return "Night"; 621 case PHS: return "After Sleep"; 622 case HS: return "HS"; 623 case WAKE: return "WAKE"; 624 case C: return "C"; 625 case CM: return "CM"; 626 case CD: return "CD"; 627 case CV: return "CV"; 628 case AC: return "AC"; 629 case ACM: return "ACM"; 630 case ACD: return "ACD"; 631 case ACV: return "ACV"; 632 case PC: return "PC"; 633 case PCM: return "PCM"; 634 case PCD: return "PCD"; 635 case PCV: return "PCV"; 636 case NULL: return null; 637 default: return "?"; 638 } 639 } 640 } 641 642 public static class EventTimingEnumFactory implements EnumFactory<EventTiming> { 643 public EventTiming fromCode(String codeString) throws IllegalArgumentException { 644 if (codeString == null || "".equals(codeString)) 645 if (codeString == null || "".equals(codeString)) 646 return null; 647 if ("MORN".equals(codeString)) 648 return EventTiming.MORN; 649 if ("AFT".equals(codeString)) 650 return EventTiming.AFT; 651 if ("EVE".equals(codeString)) 652 return EventTiming.EVE; 653 if ("NIGHT".equals(codeString)) 654 return EventTiming.NIGHT; 655 if ("PHS".equals(codeString)) 656 return EventTiming.PHS; 657 if ("HS".equals(codeString)) 658 return EventTiming.HS; 659 if ("WAKE".equals(codeString)) 660 return EventTiming.WAKE; 661 if ("C".equals(codeString)) 662 return EventTiming.C; 663 if ("CM".equals(codeString)) 664 return EventTiming.CM; 665 if ("CD".equals(codeString)) 666 return EventTiming.CD; 667 if ("CV".equals(codeString)) 668 return EventTiming.CV; 669 if ("AC".equals(codeString)) 670 return EventTiming.AC; 671 if ("ACM".equals(codeString)) 672 return EventTiming.ACM; 673 if ("ACD".equals(codeString)) 674 return EventTiming.ACD; 675 if ("ACV".equals(codeString)) 676 return EventTiming.ACV; 677 if ("PC".equals(codeString)) 678 return EventTiming.PC; 679 if ("PCM".equals(codeString)) 680 return EventTiming.PCM; 681 if ("PCD".equals(codeString)) 682 return EventTiming.PCD; 683 if ("PCV".equals(codeString)) 684 return EventTiming.PCV; 685 throw new IllegalArgumentException("Unknown EventTiming code '"+codeString+"'"); 686 } 687 public Enumeration<EventTiming> fromType(PrimitiveType<?> code) throws FHIRException { 688 if (code == null) 689 return null; 690 if (code.isEmpty()) 691 return new Enumeration<EventTiming>(this); 692 String codeString = code.asStringValue(); 693 if (codeString == null || "".equals(codeString)) 694 return null; 695 if ("MORN".equals(codeString)) 696 return new Enumeration<EventTiming>(this, EventTiming.MORN); 697 if ("AFT".equals(codeString)) 698 return new Enumeration<EventTiming>(this, EventTiming.AFT); 699 if ("EVE".equals(codeString)) 700 return new Enumeration<EventTiming>(this, EventTiming.EVE); 701 if ("NIGHT".equals(codeString)) 702 return new Enumeration<EventTiming>(this, EventTiming.NIGHT); 703 if ("PHS".equals(codeString)) 704 return new Enumeration<EventTiming>(this, EventTiming.PHS); 705 if ("HS".equals(codeString)) 706 return new Enumeration<EventTiming>(this, EventTiming.HS); 707 if ("WAKE".equals(codeString)) 708 return new Enumeration<EventTiming>(this, EventTiming.WAKE); 709 if ("C".equals(codeString)) 710 return new Enumeration<EventTiming>(this, EventTiming.C); 711 if ("CM".equals(codeString)) 712 return new Enumeration<EventTiming>(this, EventTiming.CM); 713 if ("CD".equals(codeString)) 714 return new Enumeration<EventTiming>(this, EventTiming.CD); 715 if ("CV".equals(codeString)) 716 return new Enumeration<EventTiming>(this, EventTiming.CV); 717 if ("AC".equals(codeString)) 718 return new Enumeration<EventTiming>(this, EventTiming.AC); 719 if ("ACM".equals(codeString)) 720 return new Enumeration<EventTiming>(this, EventTiming.ACM); 721 if ("ACD".equals(codeString)) 722 return new Enumeration<EventTiming>(this, EventTiming.ACD); 723 if ("ACV".equals(codeString)) 724 return new Enumeration<EventTiming>(this, EventTiming.ACV); 725 if ("PC".equals(codeString)) 726 return new Enumeration<EventTiming>(this, EventTiming.PC); 727 if ("PCM".equals(codeString)) 728 return new Enumeration<EventTiming>(this, EventTiming.PCM); 729 if ("PCD".equals(codeString)) 730 return new Enumeration<EventTiming>(this, EventTiming.PCD); 731 if ("PCV".equals(codeString)) 732 return new Enumeration<EventTiming>(this, EventTiming.PCV); 733 throw new FHIRException("Unknown EventTiming code '"+codeString+"'"); 734 } 735 public String toCode(EventTiming code) { 736 if (code == EventTiming.NULL) 737 return null; 738 if (code == EventTiming.MORN) 739 return "MORN"; 740 if (code == EventTiming.AFT) 741 return "AFT"; 742 if (code == EventTiming.EVE) 743 return "EVE"; 744 if (code == EventTiming.NIGHT) 745 return "NIGHT"; 746 if (code == EventTiming.PHS) 747 return "PHS"; 748 if (code == EventTiming.HS) 749 return "HS"; 750 if (code == EventTiming.WAKE) 751 return "WAKE"; 752 if (code == EventTiming.C) 753 return "C"; 754 if (code == EventTiming.CM) 755 return "CM"; 756 if (code == EventTiming.CD) 757 return "CD"; 758 if (code == EventTiming.CV) 759 return "CV"; 760 if (code == EventTiming.AC) 761 return "AC"; 762 if (code == EventTiming.ACM) 763 return "ACM"; 764 if (code == EventTiming.ACD) 765 return "ACD"; 766 if (code == EventTiming.ACV) 767 return "ACV"; 768 if (code == EventTiming.PC) 769 return "PC"; 770 if (code == EventTiming.PCM) 771 return "PCM"; 772 if (code == EventTiming.PCD) 773 return "PCD"; 774 if (code == EventTiming.PCV) 775 return "PCV"; 776 return "?"; 777 } 778 public String toSystem(EventTiming code) { 779 return code.getSystem(); 780 } 781 } 782 783 @Block() 784 public static class TimingRepeatComponent extends Element implements IBaseDatatypeElement { 785 /** 786 * Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule. 787 */ 788 @Child(name = "bounds", type = {Duration.class, Range.class, Period.class}, order=1, min=0, max=1, modifier=false, summary=true) 789 @Description(shortDefinition="Length/Range of lengths, or (Start and/or end) limits", formalDefinition="Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule." ) 790 protected Type bounds; 791 792 /** 793 * A total count of the desired number of repetitions. 794 */ 795 @Child(name = "count", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=true) 796 @Description(shortDefinition="Number of times to repeat", formalDefinition="A total count of the desired number of repetitions." ) 797 protected IntegerType count; 798 799 /** 800 * A maximum value for the count of the desired repetitions (e.g. do something 6-8 times). 801 */ 802 @Child(name = "countMax", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=true) 803 @Description(shortDefinition="Maximum number of times to repeat", formalDefinition="A maximum value for the count of the desired repetitions (e.g. do something 6-8 times)." ) 804 protected IntegerType countMax; 805 806 /** 807 * How long this thing happens for when it happens. 808 */ 809 @Child(name = "duration", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=true) 810 @Description(shortDefinition="How long when it happens", formalDefinition="How long this thing happens for when it happens." ) 811 protected DecimalType duration; 812 813 /** 814 * The upper limit of how long this thing happens for when it happens. 815 */ 816 @Child(name = "durationMax", type = {DecimalType.class}, order=5, min=0, max=1, modifier=false, summary=true) 817 @Description(shortDefinition="How long when it happens (Max)", formalDefinition="The upper limit of how long this thing happens for when it happens." ) 818 protected DecimalType durationMax; 819 820 /** 821 * The units of time for the duration, in UCUM units. 822 */ 823 @Child(name = "durationUnit", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 824 @Description(shortDefinition="s | min | h | d | wk | mo | a - unit of time (UCUM)", formalDefinition="The units of time for the duration, in UCUM units." ) 825 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/units-of-time") 826 protected Enumeration<UnitsOfTime> durationUnit; 827 828 /** 829 * The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided). 830 */ 831 @Child(name = "frequency", type = {IntegerType.class}, order=7, min=0, max=1, modifier=false, summary=true) 832 @Description(shortDefinition="Event occurs frequency times per period", formalDefinition="The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided)." ) 833 protected IntegerType frequency; 834 835 /** 836 * If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range. 837 */ 838 @Child(name = "frequencyMax", type = {IntegerType.class}, order=8, min=0, max=1, modifier=false, summary=true) 839 @Description(shortDefinition="Event occurs up to frequencyMax times per period", formalDefinition="If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range." ) 840 protected IntegerType frequencyMax; 841 842 /** 843 * Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. 844 */ 845 @Child(name = "period", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=true) 846 @Description(shortDefinition="Event occurs frequency times per period", formalDefinition="Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period." ) 847 protected DecimalType period; 848 849 /** 850 * If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days. 851 */ 852 @Child(name = "periodMax", type = {DecimalType.class}, order=10, min=0, max=1, modifier=false, summary=true) 853 @Description(shortDefinition="Upper limit of period (3-4 hours)", formalDefinition="If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days." ) 854 protected DecimalType periodMax; 855 856 /** 857 * The units of time for the period in UCUM units. 858 */ 859 @Child(name = "periodUnit", type = {CodeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 860 @Description(shortDefinition="s | min | h | d | wk | mo | a - unit of time (UCUM)", formalDefinition="The units of time for the period in UCUM units." ) 861 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/units-of-time") 862 protected Enumeration<UnitsOfTime> periodUnit; 863 864 /** 865 * If one or more days of week is provided, then the action happens only on the specified day(s). 866 */ 867 @Child(name = "dayOfWeek", type = {CodeType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 868 @Description(shortDefinition="mon | tue | wed | thu | fri | sat | sun", formalDefinition="If one or more days of week is provided, then the action happens only on the specified day(s)." ) 869 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/days-of-week") 870 protected List<Enumeration<DayOfWeek>> dayOfWeek; 871 872 /** 873 * Specified time of day for action to take place. 874 */ 875 @Child(name = "timeOfDay", type = {TimeType.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 876 @Description(shortDefinition="Time of day for action", formalDefinition="Specified time of day for action to take place." ) 877 protected List<TimeType> timeOfDay; 878 879 /** 880 * Real world events that the occurrence of the event should be tied to. 881 */ 882 @Child(name = "when", type = {CodeType.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 883 @Description(shortDefinition="Regular life events the event is tied to", formalDefinition="Real world events that the occurrence of the event should be tied to." ) 884 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/event-timing") 885 protected List<Enumeration<EventTiming>> when; 886 887 /** 888 * The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event. 889 */ 890 @Child(name = "offset", type = {UnsignedIntType.class}, order=15, min=0, max=1, modifier=false, summary=true) 891 @Description(shortDefinition="Minutes from event (before or after)", formalDefinition="The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event." ) 892 protected UnsignedIntType offset; 893 894 private static final long serialVersionUID = -1590643356L; 895 896 /** 897 * Constructor 898 */ 899 public TimingRepeatComponent() { 900 super(); 901 } 902 903 /** 904 * @return {@link #bounds} (Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.) 905 */ 906 public Type getBounds() { 907 return this.bounds; 908 } 909 910 /** 911 * @return {@link #bounds} (Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.) 912 */ 913 public Duration getBoundsDuration() throws FHIRException { 914 if (this.bounds == null) 915 return null; 916 if (!(this.bounds instanceof Duration)) 917 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.bounds.getClass().getName()+" was encountered"); 918 return (Duration) this.bounds; 919 } 920 921 public boolean hasBoundsDuration() { 922 return this != null && this.bounds instanceof Duration; 923 } 924 925 /** 926 * @return {@link #bounds} (Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.) 927 */ 928 public Range getBoundsRange() throws FHIRException { 929 if (this.bounds == null) 930 return null; 931 if (!(this.bounds instanceof Range)) 932 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.bounds.getClass().getName()+" was encountered"); 933 return (Range) this.bounds; 934 } 935 936 public boolean hasBoundsRange() { 937 return this != null && this.bounds instanceof Range; 938 } 939 940 /** 941 * @return {@link #bounds} (Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.) 942 */ 943 public Period getBoundsPeriod() throws FHIRException { 944 if (this.bounds == null) 945 return null; 946 if (!(this.bounds instanceof Period)) 947 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.bounds.getClass().getName()+" was encountered"); 948 return (Period) this.bounds; 949 } 950 951 public boolean hasBoundsPeriod() { 952 return this != null && this.bounds instanceof Period; 953 } 954 955 public boolean hasBounds() { 956 return this.bounds != null && !this.bounds.isEmpty(); 957 } 958 959 /** 960 * @param value {@link #bounds} (Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.) 961 */ 962 public TimingRepeatComponent setBounds(Type value) throws FHIRFormatError { 963 if (value != null && !(value instanceof Duration || value instanceof Range || value instanceof Period)) 964 throw new FHIRFormatError("Not the right type for Timing.repeat.bounds[x]: "+value.fhirType()); 965 this.bounds = value; 966 return this; 967 } 968 969 /** 970 * @return {@link #count} (A total count of the desired number of repetitions.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 971 */ 972 public IntegerType getCountElement() { 973 if (this.count == null) 974 if (Configuration.errorOnAutoCreate()) 975 throw new Error("Attempt to auto-create TimingRepeatComponent.count"); 976 else if (Configuration.doAutoCreate()) 977 this.count = new IntegerType(); // bb 978 return this.count; 979 } 980 981 public boolean hasCountElement() { 982 return this.count != null && !this.count.isEmpty(); 983 } 984 985 public boolean hasCount() { 986 return this.count != null && !this.count.isEmpty(); 987 } 988 989 /** 990 * @param value {@link #count} (A total count of the desired number of repetitions.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 991 */ 992 public TimingRepeatComponent setCountElement(IntegerType value) { 993 this.count = value; 994 return this; 995 } 996 997 /** 998 * @return A total count of the desired number of repetitions. 999 */ 1000 public int getCount() { 1001 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 1002 } 1003 1004 /** 1005 * @param value A total count of the desired number of repetitions. 1006 */ 1007 public TimingRepeatComponent setCount(int value) { 1008 if (this.count == null) 1009 this.count = new IntegerType(); 1010 this.count.setValue(value); 1011 return this; 1012 } 1013 1014 /** 1015 * @return {@link #countMax} (A maximum value for the count of the desired repetitions (e.g. do something 6-8 times).). This is the underlying object with id, value and extensions. The accessor "getCountMax" gives direct access to the value 1016 */ 1017 public IntegerType getCountMaxElement() { 1018 if (this.countMax == null) 1019 if (Configuration.errorOnAutoCreate()) 1020 throw new Error("Attempt to auto-create TimingRepeatComponent.countMax"); 1021 else if (Configuration.doAutoCreate()) 1022 this.countMax = new IntegerType(); // bb 1023 return this.countMax; 1024 } 1025 1026 public boolean hasCountMaxElement() { 1027 return this.countMax != null && !this.countMax.isEmpty(); 1028 } 1029 1030 public boolean hasCountMax() { 1031 return this.countMax != null && !this.countMax.isEmpty(); 1032 } 1033 1034 /** 1035 * @param value {@link #countMax} (A maximum value for the count of the desired repetitions (e.g. do something 6-8 times).). This is the underlying object with id, value and extensions. The accessor "getCountMax" gives direct access to the value 1036 */ 1037 public TimingRepeatComponent setCountMaxElement(IntegerType value) { 1038 this.countMax = value; 1039 return this; 1040 } 1041 1042 /** 1043 * @return A maximum value for the count of the desired repetitions (e.g. do something 6-8 times). 1044 */ 1045 public int getCountMax() { 1046 return this.countMax == null || this.countMax.isEmpty() ? 0 : this.countMax.getValue(); 1047 } 1048 1049 /** 1050 * @param value A maximum value for the count of the desired repetitions (e.g. do something 6-8 times). 1051 */ 1052 public TimingRepeatComponent setCountMax(int value) { 1053 if (this.countMax == null) 1054 this.countMax = new IntegerType(); 1055 this.countMax.setValue(value); 1056 return this; 1057 } 1058 1059 /** 1060 * @return {@link #duration} (How long this thing happens for when it happens.). This is the underlying object with id, value and extensions. The accessor "getDuration" gives direct access to the value 1061 */ 1062 public DecimalType getDurationElement() { 1063 if (this.duration == null) 1064 if (Configuration.errorOnAutoCreate()) 1065 throw new Error("Attempt to auto-create TimingRepeatComponent.duration"); 1066 else if (Configuration.doAutoCreate()) 1067 this.duration = new DecimalType(); // bb 1068 return this.duration; 1069 } 1070 1071 public boolean hasDurationElement() { 1072 return this.duration != null && !this.duration.isEmpty(); 1073 } 1074 1075 public boolean hasDuration() { 1076 return this.duration != null && !this.duration.isEmpty(); 1077 } 1078 1079 /** 1080 * @param value {@link #duration} (How long this thing happens for when it happens.). This is the underlying object with id, value and extensions. The accessor "getDuration" gives direct access to the value 1081 */ 1082 public TimingRepeatComponent setDurationElement(DecimalType value) { 1083 this.duration = value; 1084 return this; 1085 } 1086 1087 /** 1088 * @return How long this thing happens for when it happens. 1089 */ 1090 public BigDecimal getDuration() { 1091 return this.duration == null ? null : this.duration.getValue(); 1092 } 1093 1094 /** 1095 * @param value How long this thing happens for when it happens. 1096 */ 1097 public TimingRepeatComponent setDuration(BigDecimal value) { 1098 if (value == null) 1099 this.duration = null; 1100 else { 1101 if (this.duration == null) 1102 this.duration = new DecimalType(); 1103 this.duration.setValue(value); 1104 } 1105 return this; 1106 } 1107 1108 /** 1109 * @param value How long this thing happens for when it happens. 1110 */ 1111 public TimingRepeatComponent setDuration(long value) { 1112 this.duration = new DecimalType(); 1113 this.duration.setValue(value); 1114 return this; 1115 } 1116 1117 /** 1118 * @param value How long this thing happens for when it happens. 1119 */ 1120 public TimingRepeatComponent setDuration(double value) { 1121 this.duration = new DecimalType(); 1122 this.duration.setValue(value); 1123 return this; 1124 } 1125 1126 /** 1127 * @return {@link #durationMax} (The upper limit of how long this thing happens for when it happens.). This is the underlying object with id, value and extensions. The accessor "getDurationMax" gives direct access to the value 1128 */ 1129 public DecimalType getDurationMaxElement() { 1130 if (this.durationMax == null) 1131 if (Configuration.errorOnAutoCreate()) 1132 throw new Error("Attempt to auto-create TimingRepeatComponent.durationMax"); 1133 else if (Configuration.doAutoCreate()) 1134 this.durationMax = new DecimalType(); // bb 1135 return this.durationMax; 1136 } 1137 1138 public boolean hasDurationMaxElement() { 1139 return this.durationMax != null && !this.durationMax.isEmpty(); 1140 } 1141 1142 public boolean hasDurationMax() { 1143 return this.durationMax != null && !this.durationMax.isEmpty(); 1144 } 1145 1146 /** 1147 * @param value {@link #durationMax} (The upper limit of how long this thing happens for when it happens.). This is the underlying object with id, value and extensions. The accessor "getDurationMax" gives direct access to the value 1148 */ 1149 public TimingRepeatComponent setDurationMaxElement(DecimalType value) { 1150 this.durationMax = value; 1151 return this; 1152 } 1153 1154 /** 1155 * @return The upper limit of how long this thing happens for when it happens. 1156 */ 1157 public BigDecimal getDurationMax() { 1158 return this.durationMax == null ? null : this.durationMax.getValue(); 1159 } 1160 1161 /** 1162 * @param value The upper limit of how long this thing happens for when it happens. 1163 */ 1164 public TimingRepeatComponent setDurationMax(BigDecimal value) { 1165 if (value == null) 1166 this.durationMax = null; 1167 else { 1168 if (this.durationMax == null) 1169 this.durationMax = new DecimalType(); 1170 this.durationMax.setValue(value); 1171 } 1172 return this; 1173 } 1174 1175 /** 1176 * @param value The upper limit of how long this thing happens for when it happens. 1177 */ 1178 public TimingRepeatComponent setDurationMax(long value) { 1179 this.durationMax = new DecimalType(); 1180 this.durationMax.setValue(value); 1181 return this; 1182 } 1183 1184 /** 1185 * @param value The upper limit of how long this thing happens for when it happens. 1186 */ 1187 public TimingRepeatComponent setDurationMax(double value) { 1188 this.durationMax = new DecimalType(); 1189 this.durationMax.setValue(value); 1190 return this; 1191 } 1192 1193 /** 1194 * @return {@link #durationUnit} (The units of time for the duration, in UCUM units.). This is the underlying object with id, value and extensions. The accessor "getDurationUnit" gives direct access to the value 1195 */ 1196 public Enumeration<UnitsOfTime> getDurationUnitElement() { 1197 if (this.durationUnit == null) 1198 if (Configuration.errorOnAutoCreate()) 1199 throw new Error("Attempt to auto-create TimingRepeatComponent.durationUnit"); 1200 else if (Configuration.doAutoCreate()) 1201 this.durationUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); // bb 1202 return this.durationUnit; 1203 } 1204 1205 public boolean hasDurationUnitElement() { 1206 return this.durationUnit != null && !this.durationUnit.isEmpty(); 1207 } 1208 1209 public boolean hasDurationUnit() { 1210 return this.durationUnit != null && !this.durationUnit.isEmpty(); 1211 } 1212 1213 /** 1214 * @param value {@link #durationUnit} (The units of time for the duration, in UCUM units.). This is the underlying object with id, value and extensions. The accessor "getDurationUnit" gives direct access to the value 1215 */ 1216 public TimingRepeatComponent setDurationUnitElement(Enumeration<UnitsOfTime> value) { 1217 this.durationUnit = value; 1218 return this; 1219 } 1220 1221 /** 1222 * @return The units of time for the duration, in UCUM units. 1223 */ 1224 public UnitsOfTime getDurationUnit() { 1225 return this.durationUnit == null ? null : this.durationUnit.getValue(); 1226 } 1227 1228 /** 1229 * @param value The units of time for the duration, in UCUM units. 1230 */ 1231 public TimingRepeatComponent setDurationUnit(UnitsOfTime value) { 1232 if (value == null) 1233 this.durationUnit = null; 1234 else { 1235 if (this.durationUnit == null) 1236 this.durationUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); 1237 this.durationUnit.setValue(value); 1238 } 1239 return this; 1240 } 1241 1242 /** 1243 * @return {@link #frequency} (The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided).). This is the underlying object with id, value and extensions. The accessor "getFrequency" gives direct access to the value 1244 */ 1245 public IntegerType getFrequencyElement() { 1246 if (this.frequency == null) 1247 if (Configuration.errorOnAutoCreate()) 1248 throw new Error("Attempt to auto-create TimingRepeatComponent.frequency"); 1249 else if (Configuration.doAutoCreate()) 1250 this.frequency = new IntegerType(); // bb 1251 return this.frequency; 1252 } 1253 1254 public boolean hasFrequencyElement() { 1255 return this.frequency != null && !this.frequency.isEmpty(); 1256 } 1257 1258 public boolean hasFrequency() { 1259 return this.frequency != null && !this.frequency.isEmpty(); 1260 } 1261 1262 /** 1263 * @param value {@link #frequency} (The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided).). This is the underlying object with id, value and extensions. The accessor "getFrequency" gives direct access to the value 1264 */ 1265 public TimingRepeatComponent setFrequencyElement(IntegerType value) { 1266 this.frequency = value; 1267 return this; 1268 } 1269 1270 /** 1271 * @return The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided). 1272 */ 1273 public int getFrequency() { 1274 return this.frequency == null || this.frequency.isEmpty() ? 0 : this.frequency.getValue(); 1275 } 1276 1277 /** 1278 * @param value The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided). 1279 */ 1280 public TimingRepeatComponent setFrequency(int value) { 1281 if (this.frequency == null) 1282 this.frequency = new IntegerType(); 1283 this.frequency.setValue(value); 1284 return this; 1285 } 1286 1287 /** 1288 * @return {@link #frequencyMax} (If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.). This is the underlying object with id, value and extensions. The accessor "getFrequencyMax" gives direct access to the value 1289 */ 1290 public IntegerType getFrequencyMaxElement() { 1291 if (this.frequencyMax == null) 1292 if (Configuration.errorOnAutoCreate()) 1293 throw new Error("Attempt to auto-create TimingRepeatComponent.frequencyMax"); 1294 else if (Configuration.doAutoCreate()) 1295 this.frequencyMax = new IntegerType(); // bb 1296 return this.frequencyMax; 1297 } 1298 1299 public boolean hasFrequencyMaxElement() { 1300 return this.frequencyMax != null && !this.frequencyMax.isEmpty(); 1301 } 1302 1303 public boolean hasFrequencyMax() { 1304 return this.frequencyMax != null && !this.frequencyMax.isEmpty(); 1305 } 1306 1307 /** 1308 * @param value {@link #frequencyMax} (If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.). This is the underlying object with id, value and extensions. The accessor "getFrequencyMax" gives direct access to the value 1309 */ 1310 public TimingRepeatComponent setFrequencyMaxElement(IntegerType value) { 1311 this.frequencyMax = value; 1312 return this; 1313 } 1314 1315 /** 1316 * @return If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range. 1317 */ 1318 public int getFrequencyMax() { 1319 return this.frequencyMax == null || this.frequencyMax.isEmpty() ? 0 : this.frequencyMax.getValue(); 1320 } 1321 1322 /** 1323 * @param value If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range. 1324 */ 1325 public TimingRepeatComponent setFrequencyMax(int value) { 1326 if (this.frequencyMax == null) 1327 this.frequencyMax = new IntegerType(); 1328 this.frequencyMax.setValue(value); 1329 return this; 1330 } 1331 1332 /** 1333 * @return {@link #period} (Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period.). This is the underlying object with id, value and extensions. The accessor "getPeriod" gives direct access to the value 1334 */ 1335 public DecimalType getPeriodElement() { 1336 if (this.period == null) 1337 if (Configuration.errorOnAutoCreate()) 1338 throw new Error("Attempt to auto-create TimingRepeatComponent.period"); 1339 else if (Configuration.doAutoCreate()) 1340 this.period = new DecimalType(); // bb 1341 return this.period; 1342 } 1343 1344 public boolean hasPeriodElement() { 1345 return this.period != null && !this.period.isEmpty(); 1346 } 1347 1348 public boolean hasPeriod() { 1349 return this.period != null && !this.period.isEmpty(); 1350 } 1351 1352 /** 1353 * @param value {@link #period} (Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period.). This is the underlying object with id, value and extensions. The accessor "getPeriod" gives direct access to the value 1354 */ 1355 public TimingRepeatComponent setPeriodElement(DecimalType value) { 1356 this.period = value; 1357 return this; 1358 } 1359 1360 /** 1361 * @return Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. 1362 */ 1363 public BigDecimal getPeriod() { 1364 return this.period == null ? null : this.period.getValue(); 1365 } 1366 1367 /** 1368 * @param value Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. 1369 */ 1370 public TimingRepeatComponent setPeriod(BigDecimal value) { 1371 if (value == null) 1372 this.period = null; 1373 else { 1374 if (this.period == null) 1375 this.period = new DecimalType(); 1376 this.period.setValue(value); 1377 } 1378 return this; 1379 } 1380 1381 /** 1382 * @param value Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. 1383 */ 1384 public TimingRepeatComponent setPeriod(long value) { 1385 this.period = new DecimalType(); 1386 this.period.setValue(value); 1387 return this; 1388 } 1389 1390 /** 1391 * @param value Indicates the duration of time over which repetitions are to occur; e.g. to express "3 times per day", 3 would be the frequency and "1 day" would be the period. 1392 */ 1393 public TimingRepeatComponent setPeriod(double value) { 1394 this.period = new DecimalType(); 1395 this.period.setValue(value); 1396 return this; 1397 } 1398 1399 /** 1400 * @return {@link #periodMax} (If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days.). This is the underlying object with id, value and extensions. The accessor "getPeriodMax" gives direct access to the value 1401 */ 1402 public DecimalType getPeriodMaxElement() { 1403 if (this.periodMax == null) 1404 if (Configuration.errorOnAutoCreate()) 1405 throw new Error("Attempt to auto-create TimingRepeatComponent.periodMax"); 1406 else if (Configuration.doAutoCreate()) 1407 this.periodMax = new DecimalType(); // bb 1408 return this.periodMax; 1409 } 1410 1411 public boolean hasPeriodMaxElement() { 1412 return this.periodMax != null && !this.periodMax.isEmpty(); 1413 } 1414 1415 public boolean hasPeriodMax() { 1416 return this.periodMax != null && !this.periodMax.isEmpty(); 1417 } 1418 1419 /** 1420 * @param value {@link #periodMax} (If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days.). This is the underlying object with id, value and extensions. The accessor "getPeriodMax" gives direct access to the value 1421 */ 1422 public TimingRepeatComponent setPeriodMaxElement(DecimalType value) { 1423 this.periodMax = value; 1424 return this; 1425 } 1426 1427 /** 1428 * @return If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days. 1429 */ 1430 public BigDecimal getPeriodMax() { 1431 return this.periodMax == null ? null : this.periodMax.getValue(); 1432 } 1433 1434 /** 1435 * @param value If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days. 1436 */ 1437 public TimingRepeatComponent setPeriodMax(BigDecimal value) { 1438 if (value == null) 1439 this.periodMax = null; 1440 else { 1441 if (this.periodMax == null) 1442 this.periodMax = new DecimalType(); 1443 this.periodMax.setValue(value); 1444 } 1445 return this; 1446 } 1447 1448 /** 1449 * @param value If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days. 1450 */ 1451 public TimingRepeatComponent setPeriodMax(long value) { 1452 this.periodMax = new DecimalType(); 1453 this.periodMax.setValue(value); 1454 return this; 1455 } 1456 1457 /** 1458 * @param value If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as "do this once every 3-5 days. 1459 */ 1460 public TimingRepeatComponent setPeriodMax(double value) { 1461 this.periodMax = new DecimalType(); 1462 this.periodMax.setValue(value); 1463 return this; 1464 } 1465 1466 /** 1467 * @return {@link #periodUnit} (The units of time for the period in UCUM units.). This is the underlying object with id, value and extensions. The accessor "getPeriodUnit" gives direct access to the value 1468 */ 1469 public Enumeration<UnitsOfTime> getPeriodUnitElement() { 1470 if (this.periodUnit == null) 1471 if (Configuration.errorOnAutoCreate()) 1472 throw new Error("Attempt to auto-create TimingRepeatComponent.periodUnit"); 1473 else if (Configuration.doAutoCreate()) 1474 this.periodUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); // bb 1475 return this.periodUnit; 1476 } 1477 1478 public boolean hasPeriodUnitElement() { 1479 return this.periodUnit != null && !this.periodUnit.isEmpty(); 1480 } 1481 1482 public boolean hasPeriodUnit() { 1483 return this.periodUnit != null && !this.periodUnit.isEmpty(); 1484 } 1485 1486 /** 1487 * @param value {@link #periodUnit} (The units of time for the period in UCUM units.). This is the underlying object with id, value and extensions. The accessor "getPeriodUnit" gives direct access to the value 1488 */ 1489 public TimingRepeatComponent setPeriodUnitElement(Enumeration<UnitsOfTime> value) { 1490 this.periodUnit = value; 1491 return this; 1492 } 1493 1494 /** 1495 * @return The units of time for the period in UCUM units. 1496 */ 1497 public UnitsOfTime getPeriodUnit() { 1498 return this.periodUnit == null ? null : this.periodUnit.getValue(); 1499 } 1500 1501 /** 1502 * @param value The units of time for the period in UCUM units. 1503 */ 1504 public TimingRepeatComponent setPeriodUnit(UnitsOfTime value) { 1505 if (value == null) 1506 this.periodUnit = null; 1507 else { 1508 if (this.periodUnit == null) 1509 this.periodUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); 1510 this.periodUnit.setValue(value); 1511 } 1512 return this; 1513 } 1514 1515 /** 1516 * @return {@link #dayOfWeek} (If one or more days of week is provided, then the action happens only on the specified day(s).) 1517 */ 1518 public List<Enumeration<DayOfWeek>> getDayOfWeek() { 1519 if (this.dayOfWeek == null) 1520 this.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 1521 return this.dayOfWeek; 1522 } 1523 1524 /** 1525 * @return Returns a reference to <code>this</code> for easy method chaining 1526 */ 1527 public TimingRepeatComponent setDayOfWeek(List<Enumeration<DayOfWeek>> theDayOfWeek) { 1528 this.dayOfWeek = theDayOfWeek; 1529 return this; 1530 } 1531 1532 public boolean hasDayOfWeek() { 1533 if (this.dayOfWeek == null) 1534 return false; 1535 for (Enumeration<DayOfWeek> item : this.dayOfWeek) 1536 if (!item.isEmpty()) 1537 return true; 1538 return false; 1539 } 1540 1541 /** 1542 * @return {@link #dayOfWeek} (If one or more days of week is provided, then the action happens only on the specified day(s).) 1543 */ 1544 public Enumeration<DayOfWeek> addDayOfWeekElement() {//2 1545 Enumeration<DayOfWeek> t = new Enumeration<DayOfWeek>(new DayOfWeekEnumFactory()); 1546 if (this.dayOfWeek == null) 1547 this.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 1548 this.dayOfWeek.add(t); 1549 return t; 1550 } 1551 1552 /** 1553 * @param value {@link #dayOfWeek} (If one or more days of week is provided, then the action happens only on the specified day(s).) 1554 */ 1555 public TimingRepeatComponent addDayOfWeek(DayOfWeek value) { //1 1556 Enumeration<DayOfWeek> t = new Enumeration<DayOfWeek>(new DayOfWeekEnumFactory()); 1557 t.setValue(value); 1558 if (this.dayOfWeek == null) 1559 this.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 1560 this.dayOfWeek.add(t); 1561 return this; 1562 } 1563 1564 /** 1565 * @param value {@link #dayOfWeek} (If one or more days of week is provided, then the action happens only on the specified day(s).) 1566 */ 1567 public boolean hasDayOfWeek(DayOfWeek value) { 1568 if (this.dayOfWeek == null) 1569 return false; 1570 for (Enumeration<DayOfWeek> v : this.dayOfWeek) 1571 if (v.getValue().equals(value)) // code 1572 return true; 1573 return false; 1574 } 1575 1576 /** 1577 * @return {@link #timeOfDay} (Specified time of day for action to take place.) 1578 */ 1579 public List<TimeType> getTimeOfDay() { 1580 if (this.timeOfDay == null) 1581 this.timeOfDay = new ArrayList<TimeType>(); 1582 return this.timeOfDay; 1583 } 1584 1585 /** 1586 * @return Returns a reference to <code>this</code> for easy method chaining 1587 */ 1588 public TimingRepeatComponent setTimeOfDay(List<TimeType> theTimeOfDay) { 1589 this.timeOfDay = theTimeOfDay; 1590 return this; 1591 } 1592 1593 public boolean hasTimeOfDay() { 1594 if (this.timeOfDay == null) 1595 return false; 1596 for (TimeType item : this.timeOfDay) 1597 if (!item.isEmpty()) 1598 return true; 1599 return false; 1600 } 1601 1602 /** 1603 * @return {@link #timeOfDay} (Specified time of day for action to take place.) 1604 */ 1605 public TimeType addTimeOfDayElement() {//2 1606 TimeType t = new TimeType(); 1607 if (this.timeOfDay == null) 1608 this.timeOfDay = new ArrayList<TimeType>(); 1609 this.timeOfDay.add(t); 1610 return t; 1611 } 1612 1613 /** 1614 * @param value {@link #timeOfDay} (Specified time of day for action to take place.) 1615 */ 1616 public TimingRepeatComponent addTimeOfDay(String value) { //1 1617 TimeType t = new TimeType(); 1618 t.setValue(value); 1619 if (this.timeOfDay == null) 1620 this.timeOfDay = new ArrayList<TimeType>(); 1621 this.timeOfDay.add(t); 1622 return this; 1623 } 1624 1625 /** 1626 * @param value {@link #timeOfDay} (Specified time of day for action to take place.) 1627 */ 1628 public boolean hasTimeOfDay(String value) { 1629 if (this.timeOfDay == null) 1630 return false; 1631 for (TimeType v : this.timeOfDay) 1632 if (v.getValue().equals(value)) // time 1633 return true; 1634 return false; 1635 } 1636 1637 /** 1638 * @return {@link #when} (Real world events that the occurrence of the event should be tied to.) 1639 */ 1640 public List<Enumeration<EventTiming>> getWhen() { 1641 if (this.when == null) 1642 this.when = new ArrayList<Enumeration<EventTiming>>(); 1643 return this.when; 1644 } 1645 1646 /** 1647 * @return Returns a reference to <code>this</code> for easy method chaining 1648 */ 1649 public TimingRepeatComponent setWhen(List<Enumeration<EventTiming>> theWhen) { 1650 this.when = theWhen; 1651 return this; 1652 } 1653 1654 public boolean hasWhen() { 1655 if (this.when == null) 1656 return false; 1657 for (Enumeration<EventTiming> item : this.when) 1658 if (!item.isEmpty()) 1659 return true; 1660 return false; 1661 } 1662 1663 /** 1664 * @return {@link #when} (Real world events that the occurrence of the event should be tied to.) 1665 */ 1666 public Enumeration<EventTiming> addWhenElement() {//2 1667 Enumeration<EventTiming> t = new Enumeration<EventTiming>(new EventTimingEnumFactory()); 1668 if (this.when == null) 1669 this.when = new ArrayList<Enumeration<EventTiming>>(); 1670 this.when.add(t); 1671 return t; 1672 } 1673 1674 /** 1675 * @param value {@link #when} (Real world events that the occurrence of the event should be tied to.) 1676 */ 1677 public TimingRepeatComponent addWhen(EventTiming value) { //1 1678 Enumeration<EventTiming> t = new Enumeration<EventTiming>(new EventTimingEnumFactory()); 1679 t.setValue(value); 1680 if (this.when == null) 1681 this.when = new ArrayList<Enumeration<EventTiming>>(); 1682 this.when.add(t); 1683 return this; 1684 } 1685 1686 /** 1687 * @param value {@link #when} (Real world events that the occurrence of the event should be tied to.) 1688 */ 1689 public boolean hasWhen(EventTiming value) { 1690 if (this.when == null) 1691 return false; 1692 for (Enumeration<EventTiming> v : this.when) 1693 if (v.getValue().equals(value)) // code 1694 return true; 1695 return false; 1696 } 1697 1698 /** 1699 * @return {@link #offset} (The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.). This is the underlying object with id, value and extensions. The accessor "getOffset" gives direct access to the value 1700 */ 1701 public UnsignedIntType getOffsetElement() { 1702 if (this.offset == null) 1703 if (Configuration.errorOnAutoCreate()) 1704 throw new Error("Attempt to auto-create TimingRepeatComponent.offset"); 1705 else if (Configuration.doAutoCreate()) 1706 this.offset = new UnsignedIntType(); // bb 1707 return this.offset; 1708 } 1709 1710 public boolean hasOffsetElement() { 1711 return this.offset != null && !this.offset.isEmpty(); 1712 } 1713 1714 public boolean hasOffset() { 1715 return this.offset != null && !this.offset.isEmpty(); 1716 } 1717 1718 /** 1719 * @param value {@link #offset} (The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.). This is the underlying object with id, value and extensions. The accessor "getOffset" gives direct access to the value 1720 */ 1721 public TimingRepeatComponent setOffsetElement(UnsignedIntType value) { 1722 this.offset = value; 1723 return this; 1724 } 1725 1726 /** 1727 * @return The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event. 1728 */ 1729 public int getOffset() { 1730 return this.offset == null || this.offset.isEmpty() ? 0 : this.offset.getValue(); 1731 } 1732 1733 /** 1734 * @param value The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event. 1735 */ 1736 public TimingRepeatComponent setOffset(int value) { 1737 if (this.offset == null) 1738 this.offset = new UnsignedIntType(); 1739 this.offset.setValue(value); 1740 return this; 1741 } 1742 1743 protected void listChildren(List<Property> children) { 1744 super.listChildren(children); 1745 children.add(new Property("bounds[x]", "Duration|Range|Period", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds)); 1746 children.add(new Property("count", "integer", "A total count of the desired number of repetitions.", 0, 1, count)); 1747 children.add(new Property("countMax", "integer", "A maximum value for the count of the desired repetitions (e.g. do something 6-8 times).", 0, 1, countMax)); 1748 children.add(new Property("duration", "decimal", "How long this thing happens for when it happens.", 0, 1, duration)); 1749 children.add(new Property("durationMax", "decimal", "The upper limit of how long this thing happens for when it happens.", 0, 1, durationMax)); 1750 children.add(new Property("durationUnit", "code", "The units of time for the duration, in UCUM units.", 0, 1, durationUnit)); 1751 children.add(new Property("frequency", "integer", "The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided).", 0, 1, frequency)); 1752 children.add(new Property("frequencyMax", "integer", "If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.", 0, 1, frequencyMax)); 1753 children.add(new Property("period", "decimal", "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period.", 0, 1, period)); 1754 children.add(new Property("periodMax", "decimal", "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.", 0, 1, periodMax)); 1755 children.add(new Property("periodUnit", "code", "The units of time for the period in UCUM units.", 0, 1, periodUnit)); 1756 children.add(new Property("dayOfWeek", "code", "If one or more days of week is provided, then the action happens only on the specified day(s).", 0, java.lang.Integer.MAX_VALUE, dayOfWeek)); 1757 children.add(new Property("timeOfDay", "time", "Specified time of day for action to take place.", 0, java.lang.Integer.MAX_VALUE, timeOfDay)); 1758 children.add(new Property("when", "code", "Real world events that the occurrence of the event should be tied to.", 0, java.lang.Integer.MAX_VALUE, when)); 1759 children.add(new Property("offset", "unsignedInt", "The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.", 0, 1, offset)); 1760 } 1761 1762 @Override 1763 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1764 switch (_hash) { 1765 case -1149635157: /*bounds[x]*/ return new Property("bounds[x]", "Duration|Range|Period", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds); 1766 case -1383205195: /*bounds*/ return new Property("bounds[x]", "Duration|Range|Period", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds); 1767 case -189193367: /*boundsDuration*/ return new Property("bounds[x]", "Duration|Range|Period", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds); 1768 case -1001768056: /*boundsRange*/ return new Property("bounds[x]", "Duration|Range|Period", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds); 1769 case -1043481386: /*boundsPeriod*/ return new Property("bounds[x]", "Duration|Range|Period", "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 0, 1, bounds); 1770 case 94851343: /*count*/ return new Property("count", "integer", "A total count of the desired number of repetitions.", 0, 1, count); 1771 case -372044331: /*countMax*/ return new Property("countMax", "integer", "A maximum value for the count of the desired repetitions (e.g. do something 6-8 times).", 0, 1, countMax); 1772 case -1992012396: /*duration*/ return new Property("duration", "decimal", "How long this thing happens for when it happens.", 0, 1, duration); 1773 case -478083280: /*durationMax*/ return new Property("durationMax", "decimal", "The upper limit of how long this thing happens for when it happens.", 0, 1, durationMax); 1774 case -1935429320: /*durationUnit*/ return new Property("durationUnit", "code", "The units of time for the duration, in UCUM units.", 0, 1, durationUnit); 1775 case -70023844: /*frequency*/ return new Property("frequency", "integer", "The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided).", 0, 1, frequency); 1776 case 1273846376: /*frequencyMax*/ return new Property("frequencyMax", "integer", "If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.", 0, 1, frequencyMax); 1777 case -991726143: /*period*/ return new Property("period", "decimal", "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period.", 0, 1, period); 1778 case 566580195: /*periodMax*/ return new Property("periodMax", "decimal", "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.", 0, 1, periodMax); 1779 case 384367333: /*periodUnit*/ return new Property("periodUnit", "code", "The units of time for the period in UCUM units.", 0, 1, periodUnit); 1780 case -730552025: /*dayOfWeek*/ return new Property("dayOfWeek", "code", "If one or more days of week is provided, then the action happens only on the specified day(s).", 0, java.lang.Integer.MAX_VALUE, dayOfWeek); 1781 case 21434232: /*timeOfDay*/ return new Property("timeOfDay", "time", "Specified time of day for action to take place.", 0, java.lang.Integer.MAX_VALUE, timeOfDay); 1782 case 3648314: /*when*/ return new Property("when", "code", "Real world events that the occurrence of the event should be tied to.", 0, java.lang.Integer.MAX_VALUE, when); 1783 case -1019779949: /*offset*/ return new Property("offset", "unsignedInt", "The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.", 0, 1, offset); 1784 default: return super.getNamedProperty(_hash, _name, _checkValid); 1785 } 1786 1787 } 1788 1789 @Override 1790 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1791 switch (hash) { 1792 case -1383205195: /*bounds*/ return this.bounds == null ? new Base[0] : new Base[] {this.bounds}; // Type 1793 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // IntegerType 1794 case -372044331: /*countMax*/ return this.countMax == null ? new Base[0] : new Base[] {this.countMax}; // IntegerType 1795 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // DecimalType 1796 case -478083280: /*durationMax*/ return this.durationMax == null ? new Base[0] : new Base[] {this.durationMax}; // DecimalType 1797 case -1935429320: /*durationUnit*/ return this.durationUnit == null ? new Base[0] : new Base[] {this.durationUnit}; // Enumeration<UnitsOfTime> 1798 case -70023844: /*frequency*/ return this.frequency == null ? new Base[0] : new Base[] {this.frequency}; // IntegerType 1799 case 1273846376: /*frequencyMax*/ return this.frequencyMax == null ? new Base[0] : new Base[] {this.frequencyMax}; // IntegerType 1800 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // DecimalType 1801 case 566580195: /*periodMax*/ return this.periodMax == null ? new Base[0] : new Base[] {this.periodMax}; // DecimalType 1802 case 384367333: /*periodUnit*/ return this.periodUnit == null ? new Base[0] : new Base[] {this.periodUnit}; // Enumeration<UnitsOfTime> 1803 case -730552025: /*dayOfWeek*/ return this.dayOfWeek == null ? new Base[0] : this.dayOfWeek.toArray(new Base[this.dayOfWeek.size()]); // Enumeration<DayOfWeek> 1804 case 21434232: /*timeOfDay*/ return this.timeOfDay == null ? new Base[0] : this.timeOfDay.toArray(new Base[this.timeOfDay.size()]); // TimeType 1805 case 3648314: /*when*/ return this.when == null ? new Base[0] : this.when.toArray(new Base[this.when.size()]); // Enumeration<EventTiming> 1806 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // UnsignedIntType 1807 default: return super.getProperty(hash, name, checkValid); 1808 } 1809 1810 } 1811 1812 @Override 1813 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1814 switch (hash) { 1815 case -1383205195: // bounds 1816 this.bounds = castToType(value); // Type 1817 return value; 1818 case 94851343: // count 1819 this.count = castToInteger(value); // IntegerType 1820 return value; 1821 case -372044331: // countMax 1822 this.countMax = castToInteger(value); // IntegerType 1823 return value; 1824 case -1992012396: // duration 1825 this.duration = castToDecimal(value); // DecimalType 1826 return value; 1827 case -478083280: // durationMax 1828 this.durationMax = castToDecimal(value); // DecimalType 1829 return value; 1830 case -1935429320: // durationUnit 1831 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 1832 this.durationUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 1833 return value; 1834 case -70023844: // frequency 1835 this.frequency = castToInteger(value); // IntegerType 1836 return value; 1837 case 1273846376: // frequencyMax 1838 this.frequencyMax = castToInteger(value); // IntegerType 1839 return value; 1840 case -991726143: // period 1841 this.period = castToDecimal(value); // DecimalType 1842 return value; 1843 case 566580195: // periodMax 1844 this.periodMax = castToDecimal(value); // DecimalType 1845 return value; 1846 case 384367333: // periodUnit 1847 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 1848 this.periodUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 1849 return value; 1850 case -730552025: // dayOfWeek 1851 value = new DayOfWeekEnumFactory().fromType(castToCode(value)); 1852 this.getDayOfWeek().add((Enumeration) value); // Enumeration<DayOfWeek> 1853 return value; 1854 case 21434232: // timeOfDay 1855 this.getTimeOfDay().add(castToTime(value)); // TimeType 1856 return value; 1857 case 3648314: // when 1858 value = new EventTimingEnumFactory().fromType(castToCode(value)); 1859 this.getWhen().add((Enumeration) value); // Enumeration<EventTiming> 1860 return value; 1861 case -1019779949: // offset 1862 this.offset = castToUnsignedInt(value); // UnsignedIntType 1863 return value; 1864 default: return super.setProperty(hash, name, value); 1865 } 1866 1867 } 1868 1869 @Override 1870 public Base setProperty(String name, Base value) throws FHIRException { 1871 if (name.equals("bounds[x]")) { 1872 this.bounds = castToType(value); // Type 1873 } else if (name.equals("count")) { 1874 this.count = castToInteger(value); // IntegerType 1875 } else if (name.equals("countMax")) { 1876 this.countMax = castToInteger(value); // IntegerType 1877 } else if (name.equals("duration")) { 1878 this.duration = castToDecimal(value); // DecimalType 1879 } else if (name.equals("durationMax")) { 1880 this.durationMax = castToDecimal(value); // DecimalType 1881 } else if (name.equals("durationUnit")) { 1882 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 1883 this.durationUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 1884 } else if (name.equals("frequency")) { 1885 this.frequency = castToInteger(value); // IntegerType 1886 } else if (name.equals("frequencyMax")) { 1887 this.frequencyMax = castToInteger(value); // IntegerType 1888 } else if (name.equals("period")) { 1889 this.period = castToDecimal(value); // DecimalType 1890 } else if (name.equals("periodMax")) { 1891 this.periodMax = castToDecimal(value); // DecimalType 1892 } else if (name.equals("periodUnit")) { 1893 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 1894 this.periodUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 1895 } else if (name.equals("dayOfWeek")) { 1896 value = new DayOfWeekEnumFactory().fromType(castToCode(value)); 1897 this.getDayOfWeek().add((Enumeration) value); 1898 } else if (name.equals("timeOfDay")) { 1899 this.getTimeOfDay().add(castToTime(value)); 1900 } else if (name.equals("when")) { 1901 value = new EventTimingEnumFactory().fromType(castToCode(value)); 1902 this.getWhen().add((Enumeration) value); 1903 } else if (name.equals("offset")) { 1904 this.offset = castToUnsignedInt(value); // UnsignedIntType 1905 } else 1906 return super.setProperty(name, value); 1907 return value; 1908 } 1909 1910 @Override 1911 public Base makeProperty(int hash, String name) throws FHIRException { 1912 switch (hash) { 1913 case -1149635157: return getBounds(); 1914 case -1383205195: return getBounds(); 1915 case 94851343: return getCountElement(); 1916 case -372044331: return getCountMaxElement(); 1917 case -1992012396: return getDurationElement(); 1918 case -478083280: return getDurationMaxElement(); 1919 case -1935429320: return getDurationUnitElement(); 1920 case -70023844: return getFrequencyElement(); 1921 case 1273846376: return getFrequencyMaxElement(); 1922 case -991726143: return getPeriodElement(); 1923 case 566580195: return getPeriodMaxElement(); 1924 case 384367333: return getPeriodUnitElement(); 1925 case -730552025: return addDayOfWeekElement(); 1926 case 21434232: return addTimeOfDayElement(); 1927 case 3648314: return addWhenElement(); 1928 case -1019779949: return getOffsetElement(); 1929 default: return super.makeProperty(hash, name); 1930 } 1931 1932 } 1933 1934 @Override 1935 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1936 switch (hash) { 1937 case -1383205195: /*bounds*/ return new String[] {"Duration", "Range", "Period"}; 1938 case 94851343: /*count*/ return new String[] {"integer"}; 1939 case -372044331: /*countMax*/ return new String[] {"integer"}; 1940 case -1992012396: /*duration*/ return new String[] {"decimal"}; 1941 case -478083280: /*durationMax*/ return new String[] {"decimal"}; 1942 case -1935429320: /*durationUnit*/ return new String[] {"code"}; 1943 case -70023844: /*frequency*/ return new String[] {"integer"}; 1944 case 1273846376: /*frequencyMax*/ return new String[] {"integer"}; 1945 case -991726143: /*period*/ return new String[] {"decimal"}; 1946 case 566580195: /*periodMax*/ return new String[] {"decimal"}; 1947 case 384367333: /*periodUnit*/ return new String[] {"code"}; 1948 case -730552025: /*dayOfWeek*/ return new String[] {"code"}; 1949 case 21434232: /*timeOfDay*/ return new String[] {"time"}; 1950 case 3648314: /*when*/ return new String[] {"code"}; 1951 case -1019779949: /*offset*/ return new String[] {"unsignedInt"}; 1952 default: return super.getTypesForProperty(hash, name); 1953 } 1954 1955 } 1956 1957 @Override 1958 public Base addChild(String name) throws FHIRException { 1959 if (name.equals("boundsDuration")) { 1960 this.bounds = new Duration(); 1961 return this.bounds; 1962 } 1963 else if (name.equals("boundsRange")) { 1964 this.bounds = new Range(); 1965 return this.bounds; 1966 } 1967 else if (name.equals("boundsPeriod")) { 1968 this.bounds = new Period(); 1969 return this.bounds; 1970 } 1971 else if (name.equals("count")) { 1972 throw new FHIRException("Cannot call addChild on a singleton property Timing.count"); 1973 } 1974 else if (name.equals("countMax")) { 1975 throw new FHIRException("Cannot call addChild on a singleton property Timing.countMax"); 1976 } 1977 else if (name.equals("duration")) { 1978 throw new FHIRException("Cannot call addChild on a singleton property Timing.duration"); 1979 } 1980 else if (name.equals("durationMax")) { 1981 throw new FHIRException("Cannot call addChild on a singleton property Timing.durationMax"); 1982 } 1983 else if (name.equals("durationUnit")) { 1984 throw new FHIRException("Cannot call addChild on a singleton property Timing.durationUnit"); 1985 } 1986 else if (name.equals("frequency")) { 1987 throw new FHIRException("Cannot call addChild on a singleton property Timing.frequency"); 1988 } 1989 else if (name.equals("frequencyMax")) { 1990 throw new FHIRException("Cannot call addChild on a singleton property Timing.frequencyMax"); 1991 } 1992 else if (name.equals("period")) { 1993 throw new FHIRException("Cannot call addChild on a singleton property Timing.period"); 1994 } 1995 else if (name.equals("periodMax")) { 1996 throw new FHIRException("Cannot call addChild on a singleton property Timing.periodMax"); 1997 } 1998 else if (name.equals("periodUnit")) { 1999 throw new FHIRException("Cannot call addChild on a singleton property Timing.periodUnit"); 2000 } 2001 else if (name.equals("dayOfWeek")) { 2002 throw new FHIRException("Cannot call addChild on a singleton property Timing.dayOfWeek"); 2003 } 2004 else if (name.equals("timeOfDay")) { 2005 throw new FHIRException("Cannot call addChild on a singleton property Timing.timeOfDay"); 2006 } 2007 else if (name.equals("when")) { 2008 throw new FHIRException("Cannot call addChild on a singleton property Timing.when"); 2009 } 2010 else if (name.equals("offset")) { 2011 throw new FHIRException("Cannot call addChild on a singleton property Timing.offset"); 2012 } 2013 else 2014 return super.addChild(name); 2015 } 2016 2017 public TimingRepeatComponent copy() { 2018 TimingRepeatComponent dst = new TimingRepeatComponent(); 2019 copyValues(dst); 2020 dst.bounds = bounds == null ? null : bounds.copy(); 2021 dst.count = count == null ? null : count.copy(); 2022 dst.countMax = countMax == null ? null : countMax.copy(); 2023 dst.duration = duration == null ? null : duration.copy(); 2024 dst.durationMax = durationMax == null ? null : durationMax.copy(); 2025 dst.durationUnit = durationUnit == null ? null : durationUnit.copy(); 2026 dst.frequency = frequency == null ? null : frequency.copy(); 2027 dst.frequencyMax = frequencyMax == null ? null : frequencyMax.copy(); 2028 dst.period = period == null ? null : period.copy(); 2029 dst.periodMax = periodMax == null ? null : periodMax.copy(); 2030 dst.periodUnit = periodUnit == null ? null : periodUnit.copy(); 2031 if (dayOfWeek != null) { 2032 dst.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 2033 for (Enumeration<DayOfWeek> i : dayOfWeek) 2034 dst.dayOfWeek.add(i.copy()); 2035 }; 2036 if (timeOfDay != null) { 2037 dst.timeOfDay = new ArrayList<TimeType>(); 2038 for (TimeType i : timeOfDay) 2039 dst.timeOfDay.add(i.copy()); 2040 }; 2041 if (when != null) { 2042 dst.when = new ArrayList<Enumeration<EventTiming>>(); 2043 for (Enumeration<EventTiming> i : when) 2044 dst.when.add(i.copy()); 2045 }; 2046 dst.offset = offset == null ? null : offset.copy(); 2047 return dst; 2048 } 2049 2050 @Override 2051 public boolean equalsDeep(Base other_) { 2052 if (!super.equalsDeep(other_)) 2053 return false; 2054 if (!(other_ instanceof TimingRepeatComponent)) 2055 return false; 2056 TimingRepeatComponent o = (TimingRepeatComponent) other_; 2057 return compareDeep(bounds, o.bounds, true) && compareDeep(count, o.count, true) && compareDeep(countMax, o.countMax, true) 2058 && compareDeep(duration, o.duration, true) && compareDeep(durationMax, o.durationMax, true) && compareDeep(durationUnit, o.durationUnit, true) 2059 && compareDeep(frequency, o.frequency, true) && compareDeep(frequencyMax, o.frequencyMax, true) 2060 && compareDeep(period, o.period, true) && compareDeep(periodMax, o.periodMax, true) && compareDeep(periodUnit, o.periodUnit, true) 2061 && compareDeep(dayOfWeek, o.dayOfWeek, true) && compareDeep(timeOfDay, o.timeOfDay, true) && compareDeep(when, o.when, true) 2062 && compareDeep(offset, o.offset, true); 2063 } 2064 2065 @Override 2066 public boolean equalsShallow(Base other_) { 2067 if (!super.equalsShallow(other_)) 2068 return false; 2069 if (!(other_ instanceof TimingRepeatComponent)) 2070 return false; 2071 TimingRepeatComponent o = (TimingRepeatComponent) other_; 2072 return compareValues(count, o.count, true) && compareValues(countMax, o.countMax, true) && compareValues(duration, o.duration, true) 2073 && compareValues(durationMax, o.durationMax, true) && compareValues(durationUnit, o.durationUnit, true) 2074 && compareValues(frequency, o.frequency, true) && compareValues(frequencyMax, o.frequencyMax, true) 2075 && compareValues(period, o.period, true) && compareValues(periodMax, o.periodMax, true) && compareValues(periodUnit, o.periodUnit, true) 2076 && compareValues(dayOfWeek, o.dayOfWeek, true) && compareValues(timeOfDay, o.timeOfDay, true) && compareValues(when, o.when, true) 2077 && compareValues(offset, o.offset, true); 2078 } 2079 2080 public boolean isEmpty() { 2081 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(bounds, count, countMax 2082 , duration, durationMax, durationUnit, frequency, frequencyMax, period, periodMax 2083 , periodUnit, dayOfWeek, timeOfDay, when, offset); 2084 } 2085 2086 public String fhirType() { 2087 return "Timing.repeat"; 2088 2089 } 2090 2091 } 2092 2093 /** 2094 * Identifies specific times when the event occurs. 2095 */ 2096 @Child(name = "event", type = {DateTimeType.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2097 @Description(shortDefinition="When the event occurs", formalDefinition="Identifies specific times when the event occurs." ) 2098 protected List<DateTimeType> event; 2099 2100 /** 2101 * A set of rules that describe when the event is scheduled. 2102 */ 2103 @Child(name = "repeat", type = {}, order=1, min=0, max=1, modifier=false, summary=true) 2104 @Description(shortDefinition="When the event is to occur", formalDefinition="A set of rules that describe when the event is scheduled." ) 2105 protected TimingRepeatComponent repeat; 2106 2107 /** 2108 * A code for the timing schedule. Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code). 2109 */ 2110 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 2111 @Description(shortDefinition="BID | TID | QID | AM | PM | QD | QOD | Q4H | Q6H +", formalDefinition="A code for the timing schedule. Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code)." ) 2112 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/timing-abbreviation") 2113 protected CodeableConcept code; 2114 2115 private static final long serialVersionUID = 791565112L; 2116 2117 /** 2118 * Constructor 2119 */ 2120 public Timing() { 2121 super(); 2122 } 2123 2124 /** 2125 * @return {@link #event} (Identifies specific times when the event occurs.) 2126 */ 2127 public List<DateTimeType> getEvent() { 2128 if (this.event == null) 2129 this.event = new ArrayList<DateTimeType>(); 2130 return this.event; 2131 } 2132 2133 /** 2134 * @return Returns a reference to <code>this</code> for easy method chaining 2135 */ 2136 public Timing setEvent(List<DateTimeType> theEvent) { 2137 this.event = theEvent; 2138 return this; 2139 } 2140 2141 public boolean hasEvent() { 2142 if (this.event == null) 2143 return false; 2144 for (DateTimeType item : this.event) 2145 if (!item.isEmpty()) 2146 return true; 2147 return false; 2148 } 2149 2150 /** 2151 * @return {@link #event} (Identifies specific times when the event occurs.) 2152 */ 2153 public DateTimeType addEventElement() {//2 2154 DateTimeType t = new DateTimeType(); 2155 if (this.event == null) 2156 this.event = new ArrayList<DateTimeType>(); 2157 this.event.add(t); 2158 return t; 2159 } 2160 2161 /** 2162 * @param value {@link #event} (Identifies specific times when the event occurs.) 2163 */ 2164 public Timing addEvent(Date value) { //1 2165 DateTimeType t = new DateTimeType(); 2166 t.setValue(value); 2167 if (this.event == null) 2168 this.event = new ArrayList<DateTimeType>(); 2169 this.event.add(t); 2170 return this; 2171 } 2172 2173 /** 2174 * @param value {@link #event} (Identifies specific times when the event occurs.) 2175 */ 2176 public boolean hasEvent(Date value) { 2177 if (this.event == null) 2178 return false; 2179 for (DateTimeType v : this.event) 2180 if (v.getValue().equals(value)) // dateTime 2181 return true; 2182 return false; 2183 } 2184 2185 /** 2186 * @return {@link #repeat} (A set of rules that describe when the event is scheduled.) 2187 */ 2188 public TimingRepeatComponent getRepeat() { 2189 if (this.repeat == null) 2190 if (Configuration.errorOnAutoCreate()) 2191 throw new Error("Attempt to auto-create Timing.repeat"); 2192 else if (Configuration.doAutoCreate()) 2193 this.repeat = new TimingRepeatComponent(); // cc 2194 return this.repeat; 2195 } 2196 2197 public boolean hasRepeat() { 2198 return this.repeat != null && !this.repeat.isEmpty(); 2199 } 2200 2201 /** 2202 * @param value {@link #repeat} (A set of rules that describe when the event is scheduled.) 2203 */ 2204 public Timing setRepeat(TimingRepeatComponent value) { 2205 this.repeat = value; 2206 return this; 2207 } 2208 2209 /** 2210 * @return {@link #code} (A code for the timing schedule. Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).) 2211 */ 2212 public CodeableConcept getCode() { 2213 if (this.code == null) 2214 if (Configuration.errorOnAutoCreate()) 2215 throw new Error("Attempt to auto-create Timing.code"); 2216 else if (Configuration.doAutoCreate()) 2217 this.code = new CodeableConcept(); // cc 2218 return this.code; 2219 } 2220 2221 public boolean hasCode() { 2222 return this.code != null && !this.code.isEmpty(); 2223 } 2224 2225 /** 2226 * @param value {@link #code} (A code for the timing schedule. Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).) 2227 */ 2228 public Timing setCode(CodeableConcept value) { 2229 this.code = value; 2230 return this; 2231 } 2232 2233 protected void listChildren(List<Property> children) { 2234 super.listChildren(children); 2235 children.add(new Property("event", "dateTime", "Identifies specific times when the event occurs.", 0, java.lang.Integer.MAX_VALUE, event)); 2236 children.add(new Property("repeat", "", "A set of rules that describe when the event is scheduled.", 0, 1, repeat)); 2237 children.add(new Property("code", "CodeableConcept", "A code for the timing schedule. Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).", 0, 1, code)); 2238 } 2239 2240 @Override 2241 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2242 switch (_hash) { 2243 case 96891546: /*event*/ return new Property("event", "dateTime", "Identifies specific times when the event occurs.", 0, java.lang.Integer.MAX_VALUE, event); 2244 case -934531685: /*repeat*/ return new Property("repeat", "", "A set of rules that describe when the event is scheduled.", 0, 1, repeat); 2245 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code for the timing schedule. Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).", 0, 1, code); 2246 default: return super.getNamedProperty(_hash, _name, _checkValid); 2247 } 2248 2249 } 2250 2251 @Override 2252 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2253 switch (hash) { 2254 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // DateTimeType 2255 case -934531685: /*repeat*/ return this.repeat == null ? new Base[0] : new Base[] {this.repeat}; // TimingRepeatComponent 2256 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2257 default: return super.getProperty(hash, name, checkValid); 2258 } 2259 2260 } 2261 2262 @Override 2263 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2264 switch (hash) { 2265 case 96891546: // event 2266 this.getEvent().add(castToDateTime(value)); // DateTimeType 2267 return value; 2268 case -934531685: // repeat 2269 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 2270 return value; 2271 case 3059181: // code 2272 this.code = castToCodeableConcept(value); // CodeableConcept 2273 return value; 2274 default: return super.setProperty(hash, name, value); 2275 } 2276 2277 } 2278 2279 @Override 2280 public Base setProperty(String name, Base value) throws FHIRException { 2281 if (name.equals("event")) { 2282 this.getEvent().add(castToDateTime(value)); 2283 } else if (name.equals("repeat")) { 2284 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 2285 } else if (name.equals("code")) { 2286 this.code = castToCodeableConcept(value); // CodeableConcept 2287 } else 2288 return super.setProperty(name, value); 2289 return value; 2290 } 2291 2292 @Override 2293 public Base makeProperty(int hash, String name) throws FHIRException { 2294 switch (hash) { 2295 case 96891546: return addEventElement(); 2296 case -934531685: return getRepeat(); 2297 case 3059181: return getCode(); 2298 default: return super.makeProperty(hash, name); 2299 } 2300 2301 } 2302 2303 @Override 2304 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2305 switch (hash) { 2306 case 96891546: /*event*/ return new String[] {"dateTime"}; 2307 case -934531685: /*repeat*/ return new String[] {}; 2308 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2309 default: return super.getTypesForProperty(hash, name); 2310 } 2311 2312 } 2313 2314 @Override 2315 public Base addChild(String name) throws FHIRException { 2316 if (name.equals("event")) { 2317 throw new FHIRException("Cannot call addChild on a singleton property Timing.event"); 2318 } 2319 else if (name.equals("repeat")) { 2320 this.repeat = new TimingRepeatComponent(); 2321 return this.repeat; 2322 } 2323 else if (name.equals("code")) { 2324 this.code = new CodeableConcept(); 2325 return this.code; 2326 } 2327 else 2328 return super.addChild(name); 2329 } 2330 2331 public String fhirType() { 2332 return "Timing"; 2333 2334 } 2335 2336 public Timing copy() { 2337 Timing dst = new Timing(); 2338 copyValues(dst); 2339 if (event != null) { 2340 dst.event = new ArrayList<DateTimeType>(); 2341 for (DateTimeType i : event) 2342 dst.event.add(i.copy()); 2343 }; 2344 dst.repeat = repeat == null ? null : repeat.copy(); 2345 dst.code = code == null ? null : code.copy(); 2346 return dst; 2347 } 2348 2349 protected Timing typedCopy() { 2350 return copy(); 2351 } 2352 2353 @Override 2354 public boolean equalsDeep(Base other_) { 2355 if (!super.equalsDeep(other_)) 2356 return false; 2357 if (!(other_ instanceof Timing)) 2358 return false; 2359 Timing o = (Timing) other_; 2360 return compareDeep(event, o.event, true) && compareDeep(repeat, o.repeat, true) && compareDeep(code, o.code, true) 2361 ; 2362 } 2363 2364 @Override 2365 public boolean equalsShallow(Base other_) { 2366 if (!super.equalsShallow(other_)) 2367 return false; 2368 if (!(other_ instanceof Timing)) 2369 return false; 2370 Timing o = (Timing) other_; 2371 return compareValues(event, o.event, true); 2372 } 2373 2374 public boolean isEmpty() { 2375 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(event, repeat, code); 2376 } 2377 2378 2379}