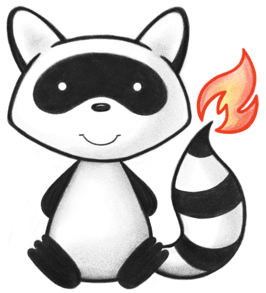
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.exceptions.FHIRFormatError; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046/** 047 * A description of a triggering event. 048 */ 049@DatatypeDef(name="TriggerDefinition") 050public class TriggerDefinition extends Type implements ICompositeType { 051 052 public enum TriggerType { 053 /** 054 * The trigger occurs in response to a specific named event 055 */ 056 NAMEDEVENT, 057 /** 058 * The trigger occurs at a specific time or periodically as described by a timing or schedule 059 */ 060 PERIODIC, 061 /** 062 * The trigger occurs whenever data of a particular type is added 063 */ 064 DATAADDED, 065 /** 066 * The trigger occurs whenever data of a particular type is modified 067 */ 068 DATAMODIFIED, 069 /** 070 * The trigger occurs whenever data of a particular type is removed 071 */ 072 DATAREMOVED, 073 /** 074 * The trigger occurs whenever data of a particular type is accessed 075 */ 076 DATAACCESSED, 077 /** 078 * The trigger occurs whenever access to data of a particular type is completed 079 */ 080 DATAACCESSENDED, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 public static TriggerType fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("named-event".equals(codeString)) 089 return NAMEDEVENT; 090 if ("periodic".equals(codeString)) 091 return PERIODIC; 092 if ("data-added".equals(codeString)) 093 return DATAADDED; 094 if ("data-modified".equals(codeString)) 095 return DATAMODIFIED; 096 if ("data-removed".equals(codeString)) 097 return DATAREMOVED; 098 if ("data-accessed".equals(codeString)) 099 return DATAACCESSED; 100 if ("data-access-ended".equals(codeString)) 101 return DATAACCESSENDED; 102 if (Configuration.isAcceptInvalidEnums()) 103 return null; 104 else 105 throw new FHIRException("Unknown TriggerType code '"+codeString+"'"); 106 } 107 public String toCode() { 108 switch (this) { 109 case NAMEDEVENT: return "named-event"; 110 case PERIODIC: return "periodic"; 111 case DATAADDED: return "data-added"; 112 case DATAMODIFIED: return "data-modified"; 113 case DATAREMOVED: return "data-removed"; 114 case DATAACCESSED: return "data-accessed"; 115 case DATAACCESSENDED: return "data-access-ended"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getSystem() { 121 switch (this) { 122 case NAMEDEVENT: return "http://hl7.org/fhir/trigger-type"; 123 case PERIODIC: return "http://hl7.org/fhir/trigger-type"; 124 case DATAADDED: return "http://hl7.org/fhir/trigger-type"; 125 case DATAMODIFIED: return "http://hl7.org/fhir/trigger-type"; 126 case DATAREMOVED: return "http://hl7.org/fhir/trigger-type"; 127 case DATAACCESSED: return "http://hl7.org/fhir/trigger-type"; 128 case DATAACCESSENDED: return "http://hl7.org/fhir/trigger-type"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 public String getDefinition() { 134 switch (this) { 135 case NAMEDEVENT: return "The trigger occurs in response to a specific named event"; 136 case PERIODIC: return "The trigger occurs at a specific time or periodically as described by a timing or schedule"; 137 case DATAADDED: return "The trigger occurs whenever data of a particular type is added"; 138 case DATAMODIFIED: return "The trigger occurs whenever data of a particular type is modified"; 139 case DATAREMOVED: return "The trigger occurs whenever data of a particular type is removed"; 140 case DATAACCESSED: return "The trigger occurs whenever data of a particular type is accessed"; 141 case DATAACCESSENDED: return "The trigger occurs whenever access to data of a particular type is completed"; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 public String getDisplay() { 147 switch (this) { 148 case NAMEDEVENT: return "Named Event"; 149 case PERIODIC: return "Periodic"; 150 case DATAADDED: return "Data Added"; 151 case DATAMODIFIED: return "Data Modified"; 152 case DATAREMOVED: return "Data Removed"; 153 case DATAACCESSED: return "Data Accessed"; 154 case DATAACCESSENDED: return "Data Access Ended"; 155 case NULL: return null; 156 default: return "?"; 157 } 158 } 159 } 160 161 public static class TriggerTypeEnumFactory implements EnumFactory<TriggerType> { 162 public TriggerType fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("named-event".equals(codeString)) 167 return TriggerType.NAMEDEVENT; 168 if ("periodic".equals(codeString)) 169 return TriggerType.PERIODIC; 170 if ("data-added".equals(codeString)) 171 return TriggerType.DATAADDED; 172 if ("data-modified".equals(codeString)) 173 return TriggerType.DATAMODIFIED; 174 if ("data-removed".equals(codeString)) 175 return TriggerType.DATAREMOVED; 176 if ("data-accessed".equals(codeString)) 177 return TriggerType.DATAACCESSED; 178 if ("data-access-ended".equals(codeString)) 179 return TriggerType.DATAACCESSENDED; 180 throw new IllegalArgumentException("Unknown TriggerType code '"+codeString+"'"); 181 } 182 public Enumeration<TriggerType> fromType(PrimitiveType<?> code) throws FHIRException { 183 if (code == null) 184 return null; 185 if (code.isEmpty()) 186 return new Enumeration<TriggerType>(this); 187 String codeString = code.asStringValue(); 188 if (codeString == null || "".equals(codeString)) 189 return null; 190 if ("named-event".equals(codeString)) 191 return new Enumeration<TriggerType>(this, TriggerType.NAMEDEVENT); 192 if ("periodic".equals(codeString)) 193 return new Enumeration<TriggerType>(this, TriggerType.PERIODIC); 194 if ("data-added".equals(codeString)) 195 return new Enumeration<TriggerType>(this, TriggerType.DATAADDED); 196 if ("data-modified".equals(codeString)) 197 return new Enumeration<TriggerType>(this, TriggerType.DATAMODIFIED); 198 if ("data-removed".equals(codeString)) 199 return new Enumeration<TriggerType>(this, TriggerType.DATAREMOVED); 200 if ("data-accessed".equals(codeString)) 201 return new Enumeration<TriggerType>(this, TriggerType.DATAACCESSED); 202 if ("data-access-ended".equals(codeString)) 203 return new Enumeration<TriggerType>(this, TriggerType.DATAACCESSENDED); 204 throw new FHIRException("Unknown TriggerType code '"+codeString+"'"); 205 } 206 public String toCode(TriggerType code) { 207 if (code == TriggerType.NULL) 208 return null; 209 if (code == TriggerType.NAMEDEVENT) 210 return "named-event"; 211 if (code == TriggerType.PERIODIC) 212 return "periodic"; 213 if (code == TriggerType.DATAADDED) 214 return "data-added"; 215 if (code == TriggerType.DATAMODIFIED) 216 return "data-modified"; 217 if (code == TriggerType.DATAREMOVED) 218 return "data-removed"; 219 if (code == TriggerType.DATAACCESSED) 220 return "data-accessed"; 221 if (code == TriggerType.DATAACCESSENDED) 222 return "data-access-ended"; 223 return "?"; 224 } 225 public String toSystem(TriggerType code) { 226 return code.getSystem(); 227 } 228 } 229 230 /** 231 * The type of triggering event. 232 */ 233 @Child(name = "type", type = {CodeType.class}, order=0, min=1, max=1, modifier=false, summary=true) 234 @Description(shortDefinition="named-event | periodic | data-added | data-modified | data-removed | data-accessed | data-access-ended", formalDefinition="The type of triggering event." ) 235 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/trigger-type") 236 protected Enumeration<TriggerType> type; 237 238 /** 239 * The name of the event (if this is a named-event trigger). 240 */ 241 @Child(name = "eventName", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 242 @Description(shortDefinition="Triggering event name", formalDefinition="The name of the event (if this is a named-event trigger)." ) 243 protected StringType eventName; 244 245 /** 246 * The timing of the event (if this is a period trigger). 247 */ 248 @Child(name = "eventTiming", type = {Timing.class, Schedule.class, DateType.class, DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 249 @Description(shortDefinition="Timing of the event", formalDefinition="The timing of the event (if this is a period trigger)." ) 250 protected Type eventTiming; 251 252 /** 253 * The triggering data of the event (if this is a data trigger). 254 */ 255 @Child(name = "eventData", type = {DataRequirement.class}, order=3, min=0, max=1, modifier=false, summary=true) 256 @Description(shortDefinition="Triggering data of the event", formalDefinition="The triggering data of the event (if this is a data trigger)." ) 257 protected DataRequirement eventData; 258 259 private static final long serialVersionUID = -1695534864L; 260 261 /** 262 * Constructor 263 */ 264 public TriggerDefinition() { 265 super(); 266 } 267 268 /** 269 * Constructor 270 */ 271 public TriggerDefinition(Enumeration<TriggerType> type) { 272 super(); 273 this.type = type; 274 } 275 276 /** 277 * @return {@link #type} (The type of triggering event.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 278 */ 279 public Enumeration<TriggerType> getTypeElement() { 280 if (this.type == null) 281 if (Configuration.errorOnAutoCreate()) 282 throw new Error("Attempt to auto-create TriggerDefinition.type"); 283 else if (Configuration.doAutoCreate()) 284 this.type = new Enumeration<TriggerType>(new TriggerTypeEnumFactory()); // bb 285 return this.type; 286 } 287 288 public boolean hasTypeElement() { 289 return this.type != null && !this.type.isEmpty(); 290 } 291 292 public boolean hasType() { 293 return this.type != null && !this.type.isEmpty(); 294 } 295 296 /** 297 * @param value {@link #type} (The type of triggering event.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 298 */ 299 public TriggerDefinition setTypeElement(Enumeration<TriggerType> value) { 300 this.type = value; 301 return this; 302 } 303 304 /** 305 * @return The type of triggering event. 306 */ 307 public TriggerType getType() { 308 return this.type == null ? null : this.type.getValue(); 309 } 310 311 /** 312 * @param value The type of triggering event. 313 */ 314 public TriggerDefinition setType(TriggerType value) { 315 if (this.type == null) 316 this.type = new Enumeration<TriggerType>(new TriggerTypeEnumFactory()); 317 this.type.setValue(value); 318 return this; 319 } 320 321 /** 322 * @return {@link #eventName} (The name of the event (if this is a named-event trigger).). This is the underlying object with id, value and extensions. The accessor "getEventName" gives direct access to the value 323 */ 324 public StringType getEventNameElement() { 325 if (this.eventName == null) 326 if (Configuration.errorOnAutoCreate()) 327 throw new Error("Attempt to auto-create TriggerDefinition.eventName"); 328 else if (Configuration.doAutoCreate()) 329 this.eventName = new StringType(); // bb 330 return this.eventName; 331 } 332 333 public boolean hasEventNameElement() { 334 return this.eventName != null && !this.eventName.isEmpty(); 335 } 336 337 public boolean hasEventName() { 338 return this.eventName != null && !this.eventName.isEmpty(); 339 } 340 341 /** 342 * @param value {@link #eventName} (The name of the event (if this is a named-event trigger).). This is the underlying object with id, value and extensions. The accessor "getEventName" gives direct access to the value 343 */ 344 public TriggerDefinition setEventNameElement(StringType value) { 345 this.eventName = value; 346 return this; 347 } 348 349 /** 350 * @return The name of the event (if this is a named-event trigger). 351 */ 352 public String getEventName() { 353 return this.eventName == null ? null : this.eventName.getValue(); 354 } 355 356 /** 357 * @param value The name of the event (if this is a named-event trigger). 358 */ 359 public TriggerDefinition setEventName(String value) { 360 if (Utilities.noString(value)) 361 this.eventName = null; 362 else { 363 if (this.eventName == null) 364 this.eventName = new StringType(); 365 this.eventName.setValue(value); 366 } 367 return this; 368 } 369 370 /** 371 * @return {@link #eventTiming} (The timing of the event (if this is a period trigger).) 372 */ 373 public Type getEventTiming() { 374 return this.eventTiming; 375 } 376 377 /** 378 * @return {@link #eventTiming} (The timing of the event (if this is a period trigger).) 379 */ 380 public Timing getEventTimingTiming() throws FHIRException { 381 if (this.eventTiming == null) 382 return null; 383 if (!(this.eventTiming instanceof Timing)) 384 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.eventTiming.getClass().getName()+" was encountered"); 385 return (Timing) this.eventTiming; 386 } 387 388 public boolean hasEventTimingTiming() { 389 return this != null && this.eventTiming instanceof Timing; 390 } 391 392 /** 393 * @return {@link #eventTiming} (The timing of the event (if this is a period trigger).) 394 */ 395 public Reference getEventTimingReference() throws FHIRException { 396 if (this.eventTiming == null) 397 return null; 398 if (!(this.eventTiming instanceof Reference)) 399 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.eventTiming.getClass().getName()+" was encountered"); 400 return (Reference) this.eventTiming; 401 } 402 403 public boolean hasEventTimingReference() { 404 return this != null && this.eventTiming instanceof Reference; 405 } 406 407 /** 408 * @return {@link #eventTiming} (The timing of the event (if this is a period trigger).) 409 */ 410 public DateType getEventTimingDateType() throws FHIRException { 411 if (this.eventTiming == null) 412 return null; 413 if (!(this.eventTiming instanceof DateType)) 414 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.eventTiming.getClass().getName()+" was encountered"); 415 return (DateType) this.eventTiming; 416 } 417 418 public boolean hasEventTimingDateType() { 419 return this != null && this.eventTiming instanceof DateType; 420 } 421 422 /** 423 * @return {@link #eventTiming} (The timing of the event (if this is a period trigger).) 424 */ 425 public DateTimeType getEventTimingDateTimeType() throws FHIRException { 426 if (this.eventTiming == null) 427 return null; 428 if (!(this.eventTiming instanceof DateTimeType)) 429 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.eventTiming.getClass().getName()+" was encountered"); 430 return (DateTimeType) this.eventTiming; 431 } 432 433 public boolean hasEventTimingDateTimeType() { 434 return this != null && this.eventTiming instanceof DateTimeType; 435 } 436 437 public boolean hasEventTiming() { 438 return this.eventTiming != null && !this.eventTiming.isEmpty(); 439 } 440 441 /** 442 * @param value {@link #eventTiming} (The timing of the event (if this is a period trigger).) 443 */ 444 public TriggerDefinition setEventTiming(Type value) throws FHIRFormatError { 445 if (value != null && !(value instanceof Timing || value instanceof Reference || value instanceof DateType || value instanceof DateTimeType)) 446 throw new FHIRFormatError("Not the right type for TriggerDefinition.eventTiming[x]: "+value.fhirType()); 447 this.eventTiming = value; 448 return this; 449 } 450 451 /** 452 * @return {@link #eventData} (The triggering data of the event (if this is a data trigger).) 453 */ 454 public DataRequirement getEventData() { 455 if (this.eventData == null) 456 if (Configuration.errorOnAutoCreate()) 457 throw new Error("Attempt to auto-create TriggerDefinition.eventData"); 458 else if (Configuration.doAutoCreate()) 459 this.eventData = new DataRequirement(); // cc 460 return this.eventData; 461 } 462 463 public boolean hasEventData() { 464 return this.eventData != null && !this.eventData.isEmpty(); 465 } 466 467 /** 468 * @param value {@link #eventData} (The triggering data of the event (if this is a data trigger).) 469 */ 470 public TriggerDefinition setEventData(DataRequirement value) { 471 this.eventData = value; 472 return this; 473 } 474 475 protected void listChildren(List<Property> children) { 476 super.listChildren(children); 477 children.add(new Property("type", "code", "The type of triggering event.", 0, 1, type)); 478 children.add(new Property("eventName", "string", "The name of the event (if this is a named-event trigger).", 0, 1, eventName)); 479 children.add(new Property("eventTiming[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a period trigger).", 0, 1, eventTiming)); 480 children.add(new Property("eventData", "DataRequirement", "The triggering data of the event (if this is a data trigger).", 0, 1, eventData)); 481 } 482 483 @Override 484 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 485 switch (_hash) { 486 case 3575610: /*type*/ return new Property("type", "code", "The type of triggering event.", 0, 1, type); 487 case 31228997: /*eventName*/ return new Property("eventName", "string", "The name of the event (if this is a named-event trigger).", 0, 1, eventName); 488 case 1120539260: /*eventTiming[x]*/ return new Property("eventTiming[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a period trigger).", 0, 1, eventTiming); 489 case 125465476: /*eventTiming*/ return new Property("eventTiming[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a period trigger).", 0, 1, eventTiming); 490 case 1285594350: /*eventTimingTiming*/ return new Property("eventTiming[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a period trigger).", 0, 1, eventTiming); 491 case -171794393: /*eventTimingReference*/ return new Property("eventTiming[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a period trigger).", 0, 1, eventTiming); 492 case 376272210: /*eventTimingDate*/ return new Property("eventTiming[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a period trigger).", 0, 1, eventTiming); 493 case -1923726529: /*eventTimingDateTime*/ return new Property("eventTiming[x]", "Timing|Reference(Schedule)|date|dateTime", "The timing of the event (if this is a period trigger).", 0, 1, eventTiming); 494 case 30931300: /*eventData*/ return new Property("eventData", "DataRequirement", "The triggering data of the event (if this is a data trigger).", 0, 1, eventData); 495 default: return super.getNamedProperty(_hash, _name, _checkValid); 496 } 497 498 } 499 500 @Override 501 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 502 switch (hash) { 503 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<TriggerType> 504 case 31228997: /*eventName*/ return this.eventName == null ? new Base[0] : new Base[] {this.eventName}; // StringType 505 case 125465476: /*eventTiming*/ return this.eventTiming == null ? new Base[0] : new Base[] {this.eventTiming}; // Type 506 case 30931300: /*eventData*/ return this.eventData == null ? new Base[0] : new Base[] {this.eventData}; // DataRequirement 507 default: return super.getProperty(hash, name, checkValid); 508 } 509 510 } 511 512 @Override 513 public Base setProperty(int hash, String name, Base value) throws FHIRException { 514 switch (hash) { 515 case 3575610: // type 516 value = new TriggerTypeEnumFactory().fromType(castToCode(value)); 517 this.type = (Enumeration) value; // Enumeration<TriggerType> 518 return value; 519 case 31228997: // eventName 520 this.eventName = castToString(value); // StringType 521 return value; 522 case 125465476: // eventTiming 523 this.eventTiming = castToType(value); // Type 524 return value; 525 case 30931300: // eventData 526 this.eventData = castToDataRequirement(value); // DataRequirement 527 return value; 528 default: return super.setProperty(hash, name, value); 529 } 530 531 } 532 533 @Override 534 public Base setProperty(String name, Base value) throws FHIRException { 535 if (name.equals("type")) { 536 value = new TriggerTypeEnumFactory().fromType(castToCode(value)); 537 this.type = (Enumeration) value; // Enumeration<TriggerType> 538 } else if (name.equals("eventName")) { 539 this.eventName = castToString(value); // StringType 540 } else if (name.equals("eventTiming[x]")) { 541 this.eventTiming = castToType(value); // Type 542 } else if (name.equals("eventData")) { 543 this.eventData = castToDataRequirement(value); // DataRequirement 544 } else 545 return super.setProperty(name, value); 546 return value; 547 } 548 549 @Override 550 public Base makeProperty(int hash, String name) throws FHIRException { 551 switch (hash) { 552 case 3575610: return getTypeElement(); 553 case 31228997: return getEventNameElement(); 554 case 1120539260: return getEventTiming(); 555 case 125465476: return getEventTiming(); 556 case 30931300: return getEventData(); 557 default: return super.makeProperty(hash, name); 558 } 559 560 } 561 562 @Override 563 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 564 switch (hash) { 565 case 3575610: /*type*/ return new String[] {"code"}; 566 case 31228997: /*eventName*/ return new String[] {"string"}; 567 case 125465476: /*eventTiming*/ return new String[] {"Timing", "Reference", "date", "dateTime"}; 568 case 30931300: /*eventData*/ return new String[] {"DataRequirement"}; 569 default: return super.getTypesForProperty(hash, name); 570 } 571 572 } 573 574 @Override 575 public Base addChild(String name) throws FHIRException { 576 if (name.equals("type")) { 577 throw new FHIRException("Cannot call addChild on a singleton property TriggerDefinition.type"); 578 } 579 else if (name.equals("eventName")) { 580 throw new FHIRException("Cannot call addChild on a singleton property TriggerDefinition.eventName"); 581 } 582 else if (name.equals("eventTimingTiming")) { 583 this.eventTiming = new Timing(); 584 return this.eventTiming; 585 } 586 else if (name.equals("eventTimingReference")) { 587 this.eventTiming = new Reference(); 588 return this.eventTiming; 589 } 590 else if (name.equals("eventTimingDate")) { 591 this.eventTiming = new DateType(); 592 return this.eventTiming; 593 } 594 else if (name.equals("eventTimingDateTime")) { 595 this.eventTiming = new DateTimeType(); 596 return this.eventTiming; 597 } 598 else if (name.equals("eventData")) { 599 this.eventData = new DataRequirement(); 600 return this.eventData; 601 } 602 else 603 return super.addChild(name); 604 } 605 606 public String fhirType() { 607 return "TriggerDefinition"; 608 609 } 610 611 public TriggerDefinition copy() { 612 TriggerDefinition dst = new TriggerDefinition(); 613 copyValues(dst); 614 dst.type = type == null ? null : type.copy(); 615 dst.eventName = eventName == null ? null : eventName.copy(); 616 dst.eventTiming = eventTiming == null ? null : eventTiming.copy(); 617 dst.eventData = eventData == null ? null : eventData.copy(); 618 return dst; 619 } 620 621 protected TriggerDefinition typedCopy() { 622 return copy(); 623 } 624 625 @Override 626 public boolean equalsDeep(Base other_) { 627 if (!super.equalsDeep(other_)) 628 return false; 629 if (!(other_ instanceof TriggerDefinition)) 630 return false; 631 TriggerDefinition o = (TriggerDefinition) other_; 632 return compareDeep(type, o.type, true) && compareDeep(eventName, o.eventName, true) && compareDeep(eventTiming, o.eventTiming, true) 633 && compareDeep(eventData, o.eventData, true); 634 } 635 636 @Override 637 public boolean equalsShallow(Base other_) { 638 if (!super.equalsShallow(other_)) 639 return false; 640 if (!(other_ instanceof TriggerDefinition)) 641 return false; 642 TriggerDefinition o = (TriggerDefinition) other_; 643 return compareValues(type, o.type, true) && compareValues(eventName, o.eventName, true); 644 } 645 646 public boolean isEmpty() { 647 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, eventName, eventTiming 648 , eventData); 649 } 650 651 652}