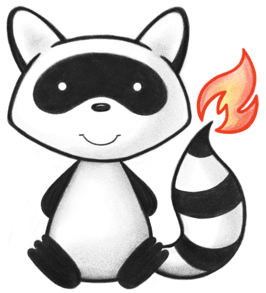
001package org.hl7.fhir.dstu3.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.net.URI; 035import java.net.URISyntaxException; 036 037import org.apache.commons.lang3.StringUtils; 038 039import ca.uhn.fhir.model.api.annotation.DatatypeDef; 040 041/** 042 * Primitive type "uri" in FHIR: any valid URI. Sometimes constrained to be only an absolute URI, and sometimes constrained to be a literal reference 043 */ 044@DatatypeDef(name = "uri") 045public class UriType extends PrimitiveType<String> { 046 047 private static final long serialVersionUID = 3L; 048 049 /** 050 * Constructor 051 */ 052 public UriType() { 053 // nothing 054 } 055 056 /** 057 * Constructor 058 */ 059 public UriType(String theValue) { 060 setValueAsString(theValue); 061 } 062 063 /** 064 * Constructor 065 */ 066 public UriType(URI theValue) { 067 setValue(theValue.toString()); 068 } 069 070 @Override 071 public UriType copy() { 072 return new UriType(getValue()); 073 } 074 075 @Override 076 protected String encode(String theValue) { 077 return theValue; 078 } 079 080 /** 081 * Compares the given string to the string representation of this URI. In many cases it is preferable to use this 082 * instead of the standard {@link #equals(Object)} method, since that method returns <code>false</code> unless it is 083 * passed an instance of {@link UriType} 084 */ 085 public boolean equals(String theString) { 086 return StringUtils.equals(getValueAsString(), theString); 087 } 088 089 @Override 090 public int hashCode() { 091 final int prime = 31; 092 int result = 1; 093 094 String normalize = normalize(getValue()); 095 result = prime * result + ((normalize == null) ? 0 : normalize.hashCode()); 096 097 return result; 098 } 099 100 private String normalize(String theValue) { 101 if (theValue == null) { 102 return null; 103 } 104 try { 105 URI retVal = new URI(getValue()).normalize(); 106 String urlString = retVal.toString(); 107 if (urlString.endsWith("/") && urlString.length() > 1) { 108 retVal = new URI(urlString.substring(0, urlString.length() - 1)); 109 } 110 return retVal.toASCIIString(); 111 } catch (URISyntaxException e) { 112 // ourLog.debug("Failed to normalize URL '{}', message was: {}", urlString, e.toString()); 113 return theValue; 114 } 115 } 116 117 @Override 118 protected String parse(String theValue) { 119 return theValue; 120 } 121 122 /** 123 * Creates a new OidType instance which uses the given OID as the content (and prepends "urn:oid:" to the OID string 124 * in the value of the newly created OidType, per the FHIR specification). 125 * 126 * @param theOid 127 * The OID to use (<code>null</code> is acceptable and will result in a UriDt instance with a 128 * <code>null</code> value) 129 * @return A new UriDt instance 130 */ 131 public static OidType fromOid(String theOid) { 132 if (theOid == null) { 133 return new OidType(); 134 } 135 return new OidType("urn:oid:" + theOid); 136 } 137 138 @Override 139 public boolean equalsDeep(Base obj) { 140 if (!super.equalsDeep(obj)) 141 return false; 142 if (this == obj) 143 return true; 144 if (obj == null) 145 return false; 146 if (getClass() != obj.getClass()) 147 return false; 148 149 UriType other = (UriType) obj; 150 if (getValue() == null && other.getValue() == null) { 151 return true; 152 } 153 if (getValue() == null || other.getValue() == null) { 154 return false; 155 } 156 if (getValue().equals(other.getValue())) { 157 return true; 158 } 159 160 String normalize = normalize(getValue()); 161 String normalize2 = normalize(other.getValue()); 162 return normalize.equals(normalize2); 163 } 164 165 public String fhirType() { 166 return "uri"; 167 } 168 169}