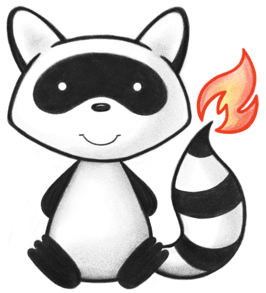
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.exceptions.FHIRFormatError; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044/** 045 * Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care). 046 */ 047@DatatypeDef(name="UsageContext") 048public class UsageContext extends Type implements ICompositeType { 049 050 /** 051 * A code that identifies the type of context being specified by this usage context. 052 */ 053 @Child(name = "code", type = {Coding.class}, order=0, min=1, max=1, modifier=false, summary=true) 054 @Description(shortDefinition="Type of context being specified", formalDefinition="A code that identifies the type of context being specified by this usage context." ) 055 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/usage-context-type") 056 protected Coding code; 057 058 /** 059 * A value that defines the context specified in this context of use. The interpretation of the value is defined by the code. 060 */ 061 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, Range.class}, order=1, min=1, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="Value that defines the context", formalDefinition="A value that defines the context specified in this context of use. The interpretation of the value is defined by the code." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/use-context") 064 protected Type value; 065 066 private static final long serialVersionUID = -1092486508L; 067 068 /** 069 * Constructor 070 */ 071 public UsageContext() { 072 super(); 073 } 074 075 /** 076 * Constructor 077 */ 078 public UsageContext(Coding code, Type value) { 079 super(); 080 this.code = code; 081 this.value = value; 082 } 083 084 /** 085 * @return {@link #code} (A code that identifies the type of context being specified by this usage context.) 086 */ 087 public Coding getCode() { 088 if (this.code == null) 089 if (Configuration.errorOnAutoCreate()) 090 throw new Error("Attempt to auto-create UsageContext.code"); 091 else if (Configuration.doAutoCreate()) 092 this.code = new Coding(); // cc 093 return this.code; 094 } 095 096 public boolean hasCode() { 097 return this.code != null && !this.code.isEmpty(); 098 } 099 100 /** 101 * @param value {@link #code} (A code that identifies the type of context being specified by this usage context.) 102 */ 103 public UsageContext setCode(Coding value) { 104 this.code = value; 105 return this; 106 } 107 108 /** 109 * @return {@link #value} (A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.) 110 */ 111 public Type getValue() { 112 return this.value; 113 } 114 115 /** 116 * @return {@link #value} (A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.) 117 */ 118 public CodeableConcept getValueCodeableConcept() throws FHIRException { 119 if (this.value == null) 120 return null; 121 if (!(this.value instanceof CodeableConcept)) 122 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 123 return (CodeableConcept) this.value; 124 } 125 126 public boolean hasValueCodeableConcept() { 127 return this != null && this.value instanceof CodeableConcept; 128 } 129 130 /** 131 * @return {@link #value} (A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.) 132 */ 133 public Quantity getValueQuantity() throws FHIRException { 134 if (this.value == null) 135 return null; 136 if (!(this.value instanceof Quantity)) 137 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 138 return (Quantity) this.value; 139 } 140 141 public boolean hasValueQuantity() { 142 return this != null && this.value instanceof Quantity; 143 } 144 145 /** 146 * @return {@link #value} (A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.) 147 */ 148 public Range getValueRange() throws FHIRException { 149 if (this.value == null) 150 return null; 151 if (!(this.value instanceof Range)) 152 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 153 return (Range) this.value; 154 } 155 156 public boolean hasValueRange() { 157 return this != null && this.value instanceof Range; 158 } 159 160 public boolean hasValue() { 161 return this.value != null && !this.value.isEmpty(); 162 } 163 164 /** 165 * @param value {@link #value} (A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.) 166 */ 167 public UsageContext setValue(Type value) throws FHIRFormatError { 168 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range)) 169 throw new FHIRFormatError("Not the right type for UsageContext.value[x]: "+value.fhirType()); 170 this.value = value; 171 return this; 172 } 173 174 protected void listChildren(List<Property> children) { 175 super.listChildren(children); 176 children.add(new Property("code", "Coding", "A code that identifies the type of context being specified by this usage context.", 0, 1, code)); 177 children.add(new Property("value[x]", "CodeableConcept|Quantity|Range", "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 0, 1, value)); 178 } 179 180 @Override 181 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 182 switch (_hash) { 183 case 3059181: /*code*/ return new Property("code", "Coding", "A code that identifies the type of context being specified by this usage context.", 0, 1, code); 184 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 0, 1, value); 185 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 0, 1, value); 186 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 0, 1, value); 187 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 0, 1, value); 188 case 2030761548: /*valueRange*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 0, 1, value); 189 default: return super.getNamedProperty(_hash, _name, _checkValid); 190 } 191 192 } 193 194 @Override 195 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 196 switch (hash) { 197 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Coding 198 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 199 default: return super.getProperty(hash, name, checkValid); 200 } 201 202 } 203 204 @Override 205 public Base setProperty(int hash, String name, Base value) throws FHIRException { 206 switch (hash) { 207 case 3059181: // code 208 this.code = castToCoding(value); // Coding 209 return value; 210 case 111972721: // value 211 this.value = castToType(value); // Type 212 return value; 213 default: return super.setProperty(hash, name, value); 214 } 215 216 } 217 218 @Override 219 public Base setProperty(String name, Base value) throws FHIRException { 220 if (name.equals("code")) { 221 this.code = castToCoding(value); // Coding 222 } else if (name.equals("value[x]")) { 223 this.value = castToType(value); // Type 224 } else 225 return super.setProperty(name, value); 226 return value; 227 } 228 229 @Override 230 public Base makeProperty(int hash, String name) throws FHIRException { 231 switch (hash) { 232 case 3059181: return getCode(); 233 case -1410166417: return getValue(); 234 case 111972721: return getValue(); 235 default: return super.makeProperty(hash, name); 236 } 237 238 } 239 240 @Override 241 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 242 switch (hash) { 243 case 3059181: /*code*/ return new String[] {"Coding"}; 244 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "Range"}; 245 default: return super.getTypesForProperty(hash, name); 246 } 247 248 } 249 250 @Override 251 public Base addChild(String name) throws FHIRException { 252 if (name.equals("code")) { 253 this.code = new Coding(); 254 return this.code; 255 } 256 else if (name.equals("valueCodeableConcept")) { 257 this.value = new CodeableConcept(); 258 return this.value; 259 } 260 else if (name.equals("valueQuantity")) { 261 this.value = new Quantity(); 262 return this.value; 263 } 264 else if (name.equals("valueRange")) { 265 this.value = new Range(); 266 return this.value; 267 } 268 else 269 return super.addChild(name); 270 } 271 272 public String fhirType() { 273 return "UsageContext"; 274 275 } 276 277 public UsageContext copy() { 278 UsageContext dst = new UsageContext(); 279 copyValues(dst); 280 dst.code = code == null ? null : code.copy(); 281 dst.value = value == null ? null : value.copy(); 282 return dst; 283 } 284 285 protected UsageContext typedCopy() { 286 return copy(); 287 } 288 289 @Override 290 public boolean equalsDeep(Base other_) { 291 if (!super.equalsDeep(other_)) 292 return false; 293 if (!(other_ instanceof UsageContext)) 294 return false; 295 UsageContext o = (UsageContext) other_; 296 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 297 } 298 299 @Override 300 public boolean equalsShallow(Base other_) { 301 if (!super.equalsShallow(other_)) 302 return false; 303 if (!(other_ instanceof UsageContext)) 304 return false; 305 UsageContext o = (UsageContext) other_; 306 return true; 307 } 308 309 public boolean isEmpty() { 310 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 311 } 312 313 314}