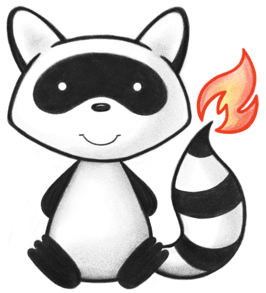
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.exceptions.FHIRFormatError; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.ChildOrder; 049import ca.uhn.fhir.model.api.annotation.Description; 050import ca.uhn.fhir.model.api.annotation.ResourceDef; 051import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 052/** 053 * A value set specifies a set of codes drawn from one or more code systems. 054 */ 055@ResourceDef(name="ValueSet", profile="http://hl7.org/fhir/Profile/ValueSet") 056@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "immutable", "purpose", "copyright", "extensible", "compose", "expansion"}) 057public class ValueSet extends MetadataResource { 058 059 public enum FilterOperator { 060 /** 061 * The specified property of the code equals the provided value. 062 */ 063 EQUAL, 064 /** 065 * Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, including the provided concept itself (i.e. include child codes) 066 */ 067 ISA, 068 /** 069 * Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, excluding the provided concept itself (i.e. include child codes) 070 */ 071 DESCENDENTOF, 072 /** 073 * The specified property of the code does not have an is-a relationship with the provided value. 074 */ 075 ISNOTA, 076 /** 077 * The specified property of the code matches the regex specified in the provided value. 078 */ 079 REGEX, 080 /** 081 * The specified property of the code is in the set of codes or concepts specified in the provided value (comma separated list). 082 */ 083 IN, 084 /** 085 * The specified property of the code is not in the set of codes or concepts specified in the provided value (comma separated list). 086 */ 087 NOTIN, 088 /** 089 * Includes all concept ids that have a transitive is-a relationship from the concept Id provided as the value, including the provided concept itself (e.g. include parent codes) 090 */ 091 GENERALIZES, 092 /** 093 * The specified property of the code has at least one value (if the specified value is true; if the specified value is false, then matches when the specified property of the code has no values) 094 */ 095 EXISTS, 096 /** 097 * added to help the parsers with the generic types 098 */ 099 NULL; 100 public static FilterOperator fromCode(String codeString) throws FHIRException { 101 if (codeString == null || "".equals(codeString)) 102 return null; 103 if ("=".equals(codeString)) 104 return EQUAL; 105 if ("is-a".equals(codeString)) 106 return ISA; 107 if ("descendent-of".equals(codeString)) 108 return DESCENDENTOF; 109 if ("is-not-a".equals(codeString)) 110 return ISNOTA; 111 if ("regex".equals(codeString)) 112 return REGEX; 113 if ("in".equals(codeString)) 114 return IN; 115 if ("not-in".equals(codeString)) 116 return NOTIN; 117 if ("generalizes".equals(codeString)) 118 return GENERALIZES; 119 if ("exists".equals(codeString)) 120 return EXISTS; 121 if (Configuration.isAcceptInvalidEnums()) 122 return null; 123 else 124 throw new FHIRException("Unknown FilterOperator code '"+codeString+"'"); 125 } 126 public String toCode() { 127 switch (this) { 128 case EQUAL: return "="; 129 case ISA: return "is-a"; 130 case DESCENDENTOF: return "descendent-of"; 131 case ISNOTA: return "is-not-a"; 132 case REGEX: return "regex"; 133 case IN: return "in"; 134 case NOTIN: return "not-in"; 135 case GENERALIZES: return "generalizes"; 136 case EXISTS: return "exists"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getSystem() { 142 switch (this) { 143 case EQUAL: return "http://hl7.org/fhir/filter-operator"; 144 case ISA: return "http://hl7.org/fhir/filter-operator"; 145 case DESCENDENTOF: return "http://hl7.org/fhir/filter-operator"; 146 case ISNOTA: return "http://hl7.org/fhir/filter-operator"; 147 case REGEX: return "http://hl7.org/fhir/filter-operator"; 148 case IN: return "http://hl7.org/fhir/filter-operator"; 149 case NOTIN: return "http://hl7.org/fhir/filter-operator"; 150 case GENERALIZES: return "http://hl7.org/fhir/filter-operator"; 151 case EXISTS: return "http://hl7.org/fhir/filter-operator"; 152 case NULL: return null; 153 default: return "?"; 154 } 155 } 156 public String getDefinition() { 157 switch (this) { 158 case EQUAL: return "The specified property of the code equals the provided value."; 159 case ISA: return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, including the provided concept itself (i.e. include child codes)"; 160 case DESCENDENTOF: return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, excluding the provided concept itself (i.e. include child codes)"; 161 case ISNOTA: return "The specified property of the code does not have an is-a relationship with the provided value."; 162 case REGEX: return "The specified property of the code matches the regex specified in the provided value."; 163 case IN: return "The specified property of the code is in the set of codes or concepts specified in the provided value (comma separated list)."; 164 case NOTIN: return "The specified property of the code is not in the set of codes or concepts specified in the provided value (comma separated list)."; 165 case GENERALIZES: return "Includes all concept ids that have a transitive is-a relationship from the concept Id provided as the value, including the provided concept itself (e.g. include parent codes)"; 166 case EXISTS: return "The specified property of the code has at least one value (if the specified value is true; if the specified value is false, then matches when the specified property of the code has no values)"; 167 case NULL: return null; 168 default: return "?"; 169 } 170 } 171 public String getDisplay() { 172 switch (this) { 173 case EQUAL: return "Equals"; 174 case ISA: return "Is A (by subsumption)"; 175 case DESCENDENTOF: return "Descendent Of (by subsumption)"; 176 case ISNOTA: return "Not (Is A) (by subsumption)"; 177 case REGEX: return "Regular Expression"; 178 case IN: return "In Set"; 179 case NOTIN: return "Not in Set"; 180 case GENERALIZES: return "Generalizes (by Subsumption)"; 181 case EXISTS: return "Exists"; 182 case NULL: return null; 183 default: return "?"; 184 } 185 } 186 } 187 188 public static class FilterOperatorEnumFactory implements EnumFactory<FilterOperator> { 189 public FilterOperator fromCode(String codeString) throws IllegalArgumentException { 190 if (codeString == null || "".equals(codeString)) 191 if (codeString == null || "".equals(codeString)) 192 return null; 193 if ("=".equals(codeString)) 194 return FilterOperator.EQUAL; 195 if ("is-a".equals(codeString)) 196 return FilterOperator.ISA; 197 if ("descendent-of".equals(codeString)) 198 return FilterOperator.DESCENDENTOF; 199 if ("is-not-a".equals(codeString)) 200 return FilterOperator.ISNOTA; 201 if ("regex".equals(codeString)) 202 return FilterOperator.REGEX; 203 if ("in".equals(codeString)) 204 return FilterOperator.IN; 205 if ("not-in".equals(codeString)) 206 return FilterOperator.NOTIN; 207 if ("generalizes".equals(codeString)) 208 return FilterOperator.GENERALIZES; 209 if ("exists".equals(codeString)) 210 return FilterOperator.EXISTS; 211 throw new IllegalArgumentException("Unknown FilterOperator code '"+codeString+"'"); 212 } 213 public Enumeration<FilterOperator> fromType(PrimitiveType<?> code) throws FHIRException { 214 if (code == null) 215 return null; 216 if (code.isEmpty()) 217 return new Enumeration<FilterOperator>(this); 218 String codeString = code.asStringValue(); 219 if (codeString == null || "".equals(codeString)) 220 return null; 221 if ("=".equals(codeString)) 222 return new Enumeration<FilterOperator>(this, FilterOperator.EQUAL); 223 if ("is-a".equals(codeString)) 224 return new Enumeration<FilterOperator>(this, FilterOperator.ISA); 225 if ("descendent-of".equals(codeString)) 226 return new Enumeration<FilterOperator>(this, FilterOperator.DESCENDENTOF); 227 if ("is-not-a".equals(codeString)) 228 return new Enumeration<FilterOperator>(this, FilterOperator.ISNOTA); 229 if ("regex".equals(codeString)) 230 return new Enumeration<FilterOperator>(this, FilterOperator.REGEX); 231 if ("in".equals(codeString)) 232 return new Enumeration<FilterOperator>(this, FilterOperator.IN); 233 if ("not-in".equals(codeString)) 234 return new Enumeration<FilterOperator>(this, FilterOperator.NOTIN); 235 if ("generalizes".equals(codeString)) 236 return new Enumeration<FilterOperator>(this, FilterOperator.GENERALIZES); 237 if ("exists".equals(codeString)) 238 return new Enumeration<FilterOperator>(this, FilterOperator.EXISTS); 239 throw new FHIRException("Unknown FilterOperator code '"+codeString+"'"); 240 } 241 public String toCode(FilterOperator code) { 242 if (code == FilterOperator.NULL) 243 return null; 244 if (code == FilterOperator.EQUAL) 245 return "="; 246 if (code == FilterOperator.ISA) 247 return "is-a"; 248 if (code == FilterOperator.DESCENDENTOF) 249 return "descendent-of"; 250 if (code == FilterOperator.ISNOTA) 251 return "is-not-a"; 252 if (code == FilterOperator.REGEX) 253 return "regex"; 254 if (code == FilterOperator.IN) 255 return "in"; 256 if (code == FilterOperator.NOTIN) 257 return "not-in"; 258 if (code == FilterOperator.GENERALIZES) 259 return "generalizes"; 260 if (code == FilterOperator.EXISTS) 261 return "exists"; 262 return "?"; 263 } 264 public String toSystem(FilterOperator code) { 265 return code.getSystem(); 266 } 267 } 268 269 @Block() 270 public static class ValueSetComposeComponent extends BackboneElement implements IBaseBackboneElement { 271 /** 272 * If a locked date is defined, then the Content Logical Definition must be evaluated using the current version as of the locked date for referenced code system(s) and value set instances where ValueSet.compose.include.version is not defined. 273 */ 274 @Child(name = "lockedDate", type = {DateType.class}, order=1, min=0, max=1, modifier=false, summary=true) 275 @Description(shortDefinition="Fixed date for version-less references (transitive)", formalDefinition="If a locked date is defined, then the Content Logical Definition must be evaluated using the current version as of the locked date for referenced code system(s) and value set instances where ValueSet.compose.include.version is not defined." ) 276 protected DateType lockedDate; 277 278 /** 279 * Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable ExpansionProfile (but generally, inactive codes would be expected to be included). 280 */ 281 @Child(name = "inactive", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=true) 282 @Description(shortDefinition="Whether inactive codes are in the value set", formalDefinition="Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable ExpansionProfile (but generally, inactive codes would be expected to be included)." ) 283 protected BooleanType inactive; 284 285 /** 286 * Include one or more codes from a code system or other value set(s). 287 */ 288 @Child(name = "include", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 289 @Description(shortDefinition="Include one or more codes from a code system or other value set(s)", formalDefinition="Include one or more codes from a code system or other value set(s)." ) 290 protected List<ConceptSetComponent> include; 291 292 /** 293 * Exclude one or more codes from the value set based on code system filters and/or other value sets. 294 */ 295 @Child(name = "exclude", type = {ConceptSetComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 296 @Description(shortDefinition="Explicitly exclude codes from a code system or other value sets", formalDefinition="Exclude one or more codes from the value set based on code system filters and/or other value sets." ) 297 protected List<ConceptSetComponent> exclude; 298 299 private static final long serialVersionUID = -765941757L; 300 301 /** 302 * Constructor 303 */ 304 public ValueSetComposeComponent() { 305 super(); 306 } 307 308 /** 309 * @return {@link #lockedDate} (If a locked date is defined, then the Content Logical Definition must be evaluated using the current version as of the locked date for referenced code system(s) and value set instances where ValueSet.compose.include.version is not defined.). This is the underlying object with id, value and extensions. The accessor "getLockedDate" gives direct access to the value 310 */ 311 public DateType getLockedDateElement() { 312 if (this.lockedDate == null) 313 if (Configuration.errorOnAutoCreate()) 314 throw new Error("Attempt to auto-create ValueSetComposeComponent.lockedDate"); 315 else if (Configuration.doAutoCreate()) 316 this.lockedDate = new DateType(); // bb 317 return this.lockedDate; 318 } 319 320 public boolean hasLockedDateElement() { 321 return this.lockedDate != null && !this.lockedDate.isEmpty(); 322 } 323 324 public boolean hasLockedDate() { 325 return this.lockedDate != null && !this.lockedDate.isEmpty(); 326 } 327 328 /** 329 * @param value {@link #lockedDate} (If a locked date is defined, then the Content Logical Definition must be evaluated using the current version as of the locked date for referenced code system(s) and value set instances where ValueSet.compose.include.version is not defined.). This is the underlying object with id, value and extensions. The accessor "getLockedDate" gives direct access to the value 330 */ 331 public ValueSetComposeComponent setLockedDateElement(DateType value) { 332 this.lockedDate = value; 333 return this; 334 } 335 336 /** 337 * @return If a locked date is defined, then the Content Logical Definition must be evaluated using the current version as of the locked date for referenced code system(s) and value set instances where ValueSet.compose.include.version is not defined. 338 */ 339 public Date getLockedDate() { 340 return this.lockedDate == null ? null : this.lockedDate.getValue(); 341 } 342 343 /** 344 * @param value If a locked date is defined, then the Content Logical Definition must be evaluated using the current version as of the locked date for referenced code system(s) and value set instances where ValueSet.compose.include.version is not defined. 345 */ 346 public ValueSetComposeComponent setLockedDate(Date value) { 347 if (value == null) 348 this.lockedDate = null; 349 else { 350 if (this.lockedDate == null) 351 this.lockedDate = new DateType(); 352 this.lockedDate.setValue(value); 353 } 354 return this; 355 } 356 357 /** 358 * @return {@link #inactive} (Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable ExpansionProfile (but generally, inactive codes would be expected to be included).). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 359 */ 360 public BooleanType getInactiveElement() { 361 if (this.inactive == null) 362 if (Configuration.errorOnAutoCreate()) 363 throw new Error("Attempt to auto-create ValueSetComposeComponent.inactive"); 364 else if (Configuration.doAutoCreate()) 365 this.inactive = new BooleanType(); // bb 366 return this.inactive; 367 } 368 369 public boolean hasInactiveElement() { 370 return this.inactive != null && !this.inactive.isEmpty(); 371 } 372 373 public boolean hasInactive() { 374 return this.inactive != null && !this.inactive.isEmpty(); 375 } 376 377 /** 378 * @param value {@link #inactive} (Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable ExpansionProfile (but generally, inactive codes would be expected to be included).). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 379 */ 380 public ValueSetComposeComponent setInactiveElement(BooleanType value) { 381 this.inactive = value; 382 return this; 383 } 384 385 /** 386 * @return Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable ExpansionProfile (but generally, inactive codes would be expected to be included). 387 */ 388 public boolean getInactive() { 389 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 390 } 391 392 /** 393 * @param value Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable ExpansionProfile (but generally, inactive codes would be expected to be included). 394 */ 395 public ValueSetComposeComponent setInactive(boolean value) { 396 if (this.inactive == null) 397 this.inactive = new BooleanType(); 398 this.inactive.setValue(value); 399 return this; 400 } 401 402 /** 403 * @return {@link #include} (Include one or more codes from a code system or other value set(s).) 404 */ 405 public List<ConceptSetComponent> getInclude() { 406 if (this.include == null) 407 this.include = new ArrayList<ConceptSetComponent>(); 408 return this.include; 409 } 410 411 /** 412 * @return Returns a reference to <code>this</code> for easy method chaining 413 */ 414 public ValueSetComposeComponent setInclude(List<ConceptSetComponent> theInclude) { 415 this.include = theInclude; 416 return this; 417 } 418 419 public boolean hasInclude() { 420 if (this.include == null) 421 return false; 422 for (ConceptSetComponent item : this.include) 423 if (!item.isEmpty()) 424 return true; 425 return false; 426 } 427 428 public ConceptSetComponent addInclude() { //3 429 ConceptSetComponent t = new ConceptSetComponent(); 430 if (this.include == null) 431 this.include = new ArrayList<ConceptSetComponent>(); 432 this.include.add(t); 433 return t; 434 } 435 436 public ValueSetComposeComponent addInclude(ConceptSetComponent t) { //3 437 if (t == null) 438 return this; 439 if (this.include == null) 440 this.include = new ArrayList<ConceptSetComponent>(); 441 this.include.add(t); 442 return this; 443 } 444 445 /** 446 * @return The first repetition of repeating field {@link #include}, creating it if it does not already exist 447 */ 448 public ConceptSetComponent getIncludeFirstRep() { 449 if (getInclude().isEmpty()) { 450 addInclude(); 451 } 452 return getInclude().get(0); 453 } 454 455 /** 456 * @return {@link #exclude} (Exclude one or more codes from the value set based on code system filters and/or other value sets.) 457 */ 458 public List<ConceptSetComponent> getExclude() { 459 if (this.exclude == null) 460 this.exclude = new ArrayList<ConceptSetComponent>(); 461 return this.exclude; 462 } 463 464 /** 465 * @return Returns a reference to <code>this</code> for easy method chaining 466 */ 467 public ValueSetComposeComponent setExclude(List<ConceptSetComponent> theExclude) { 468 this.exclude = theExclude; 469 return this; 470 } 471 472 public boolean hasExclude() { 473 if (this.exclude == null) 474 return false; 475 for (ConceptSetComponent item : this.exclude) 476 if (!item.isEmpty()) 477 return true; 478 return false; 479 } 480 481 public ConceptSetComponent addExclude() { //3 482 ConceptSetComponent t = new ConceptSetComponent(); 483 if (this.exclude == null) 484 this.exclude = new ArrayList<ConceptSetComponent>(); 485 this.exclude.add(t); 486 return t; 487 } 488 489 public ValueSetComposeComponent addExclude(ConceptSetComponent t) { //3 490 if (t == null) 491 return this; 492 if (this.exclude == null) 493 this.exclude = new ArrayList<ConceptSetComponent>(); 494 this.exclude.add(t); 495 return this; 496 } 497 498 /** 499 * @return The first repetition of repeating field {@link #exclude}, creating it if it does not already exist 500 */ 501 public ConceptSetComponent getExcludeFirstRep() { 502 if (getExclude().isEmpty()) { 503 addExclude(); 504 } 505 return getExclude().get(0); 506 } 507 508 protected void listChildren(List<Property> children) { 509 super.listChildren(children); 510 children.add(new Property("lockedDate", "date", "If a locked date is defined, then the Content Logical Definition must be evaluated using the current version as of the locked date for referenced code system(s) and value set instances where ValueSet.compose.include.version is not defined.", 0, 1, lockedDate)); 511 children.add(new Property("inactive", "boolean", "Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable ExpansionProfile (but generally, inactive codes would be expected to be included).", 0, 1, inactive)); 512 children.add(new Property("include", "", "Include one or more codes from a code system or other value set(s).", 0, java.lang.Integer.MAX_VALUE, include)); 513 children.add(new Property("exclude", "@ValueSet.compose.include", "Exclude one or more codes from the value set based on code system filters and/or other value sets.", 0, java.lang.Integer.MAX_VALUE, exclude)); 514 } 515 516 @Override 517 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 518 switch (_hash) { 519 case 1391591896: /*lockedDate*/ return new Property("lockedDate", "date", "If a locked date is defined, then the Content Logical Definition must be evaluated using the current version as of the locked date for referenced code system(s) and value set instances where ValueSet.compose.include.version is not defined.", 0, 1, lockedDate); 520 case 24665195: /*inactive*/ return new Property("inactive", "boolean", "Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable ExpansionProfile (but generally, inactive codes would be expected to be included).", 0, 1, inactive); 521 case 1942574248: /*include*/ return new Property("include", "", "Include one or more codes from a code system or other value set(s).", 0, java.lang.Integer.MAX_VALUE, include); 522 case -1321148966: /*exclude*/ return new Property("exclude", "@ValueSet.compose.include", "Exclude one or more codes from the value set based on code system filters and/or other value sets.", 0, java.lang.Integer.MAX_VALUE, exclude); 523 default: return super.getNamedProperty(_hash, _name, _checkValid); 524 } 525 526 } 527 528 @Override 529 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 530 switch (hash) { 531 case 1391591896: /*lockedDate*/ return this.lockedDate == null ? new Base[0] : new Base[] {this.lockedDate}; // DateType 532 case 24665195: /*inactive*/ return this.inactive == null ? new Base[0] : new Base[] {this.inactive}; // BooleanType 533 case 1942574248: /*include*/ return this.include == null ? new Base[0] : this.include.toArray(new Base[this.include.size()]); // ConceptSetComponent 534 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : this.exclude.toArray(new Base[this.exclude.size()]); // ConceptSetComponent 535 default: return super.getProperty(hash, name, checkValid); 536 } 537 538 } 539 540 @Override 541 public Base setProperty(int hash, String name, Base value) throws FHIRException { 542 switch (hash) { 543 case 1391591896: // lockedDate 544 this.lockedDate = castToDate(value); // DateType 545 return value; 546 case 24665195: // inactive 547 this.inactive = castToBoolean(value); // BooleanType 548 return value; 549 case 1942574248: // include 550 this.getInclude().add((ConceptSetComponent) value); // ConceptSetComponent 551 return value; 552 case -1321148966: // exclude 553 this.getExclude().add((ConceptSetComponent) value); // ConceptSetComponent 554 return value; 555 default: return super.setProperty(hash, name, value); 556 } 557 558 } 559 560 @Override 561 public Base setProperty(String name, Base value) throws FHIRException { 562 if (name.equals("lockedDate")) { 563 this.lockedDate = castToDate(value); // DateType 564 } else if (name.equals("inactive")) { 565 this.inactive = castToBoolean(value); // BooleanType 566 } else if (name.equals("include")) { 567 this.getInclude().add((ConceptSetComponent) value); 568 } else if (name.equals("exclude")) { 569 this.getExclude().add((ConceptSetComponent) value); 570 } else 571 return super.setProperty(name, value); 572 return value; 573 } 574 575 @Override 576 public Base makeProperty(int hash, String name) throws FHIRException { 577 switch (hash) { 578 case 1391591896: return getLockedDateElement(); 579 case 24665195: return getInactiveElement(); 580 case 1942574248: return addInclude(); 581 case -1321148966: return addExclude(); 582 default: return super.makeProperty(hash, name); 583 } 584 585 } 586 587 @Override 588 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 589 switch (hash) { 590 case 1391591896: /*lockedDate*/ return new String[] {"date"}; 591 case 24665195: /*inactive*/ return new String[] {"boolean"}; 592 case 1942574248: /*include*/ return new String[] {}; 593 case -1321148966: /*exclude*/ return new String[] {"@ValueSet.compose.include"}; 594 default: return super.getTypesForProperty(hash, name); 595 } 596 597 } 598 599 @Override 600 public Base addChild(String name) throws FHIRException { 601 if (name.equals("lockedDate")) { 602 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.lockedDate"); 603 } 604 else if (name.equals("inactive")) { 605 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.inactive"); 606 } 607 else if (name.equals("include")) { 608 return addInclude(); 609 } 610 else if (name.equals("exclude")) { 611 return addExclude(); 612 } 613 else 614 return super.addChild(name); 615 } 616 617 public ValueSetComposeComponent copy() { 618 ValueSetComposeComponent dst = new ValueSetComposeComponent(); 619 copyValues(dst); 620 dst.lockedDate = lockedDate == null ? null : lockedDate.copy(); 621 dst.inactive = inactive == null ? null : inactive.copy(); 622 if (include != null) { 623 dst.include = new ArrayList<ConceptSetComponent>(); 624 for (ConceptSetComponent i : include) 625 dst.include.add(i.copy()); 626 }; 627 if (exclude != null) { 628 dst.exclude = new ArrayList<ConceptSetComponent>(); 629 for (ConceptSetComponent i : exclude) 630 dst.exclude.add(i.copy()); 631 }; 632 return dst; 633 } 634 635 @Override 636 public boolean equalsDeep(Base other_) { 637 if (!super.equalsDeep(other_)) 638 return false; 639 if (!(other_ instanceof ValueSetComposeComponent)) 640 return false; 641 ValueSetComposeComponent o = (ValueSetComposeComponent) other_; 642 return compareDeep(lockedDate, o.lockedDate, true) && compareDeep(inactive, o.inactive, true) && compareDeep(include, o.include, true) 643 && compareDeep(exclude, o.exclude, true); 644 } 645 646 @Override 647 public boolean equalsShallow(Base other_) { 648 if (!super.equalsShallow(other_)) 649 return false; 650 if (!(other_ instanceof ValueSetComposeComponent)) 651 return false; 652 ValueSetComposeComponent o = (ValueSetComposeComponent) other_; 653 return compareValues(lockedDate, o.lockedDate, true) && compareValues(inactive, o.inactive, true); 654 } 655 656 public boolean isEmpty() { 657 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(lockedDate, inactive, include 658 , exclude); 659 } 660 661 public String fhirType() { 662 return "ValueSet.compose"; 663 664 } 665 666 } 667 668 @Block() 669 public static class ConceptSetComponent extends BackboneElement implements IBaseBackboneElement { 670 /** 671 * An absolute URI which is the code system from which the selected codes come from. 672 */ 673 @Child(name = "system", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=true) 674 @Description(shortDefinition="The system the codes come from", formalDefinition="An absolute URI which is the code system from which the selected codes come from." ) 675 protected UriType system; 676 677 /** 678 * The version of the code system that the codes are selected from. 679 */ 680 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 681 @Description(shortDefinition="Specific version of the code system referred to", formalDefinition="The version of the code system that the codes are selected from." ) 682 protected StringType version; 683 684 /** 685 * Specifies a concept to be included or excluded. 686 */ 687 @Child(name = "concept", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 688 @Description(shortDefinition="A concept defined in the system", formalDefinition="Specifies a concept to be included or excluded." ) 689 protected List<ConceptReferenceComponent> concept; 690 691 /** 692 * Select concepts by specify a matching criteria based on the properties (including relationships) defined by the system. If multiple filters are specified, they SHALL all be true. 693 */ 694 @Child(name = "filter", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=true) 695 @Description(shortDefinition="Select codes/concepts by their properties (including relationships)", formalDefinition="Select concepts by specify a matching criteria based on the properties (including relationships) defined by the system. If multiple filters are specified, they SHALL all be true." ) 696 protected List<ConceptSetFilterComponent> filter; 697 698 /** 699 * Selects concepts found in this value set. This is an absolute URI that is a reference to ValueSet.url. 700 */ 701 @Child(name = "valueSet", type = {UriType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 702 @Description(shortDefinition="Select only contents included in this value set", formalDefinition="Selects concepts found in this value set. This is an absolute URI that is a reference to ValueSet.url." ) 703 protected List<UriType> valueSet; 704 705 private static final long serialVersionUID = -1322183438L; 706 707 /** 708 * Constructor 709 */ 710 public ConceptSetComponent() { 711 super(); 712 } 713 714 /** 715 * @return {@link #system} (An absolute URI which is the code system from which the selected codes come from.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 716 */ 717 public UriType getSystemElement() { 718 if (this.system == null) 719 if (Configuration.errorOnAutoCreate()) 720 throw new Error("Attempt to auto-create ConceptSetComponent.system"); 721 else if (Configuration.doAutoCreate()) 722 this.system = new UriType(); // bb 723 return this.system; 724 } 725 726 public boolean hasSystemElement() { 727 return this.system != null && !this.system.isEmpty(); 728 } 729 730 public boolean hasSystem() { 731 return this.system != null && !this.system.isEmpty(); 732 } 733 734 /** 735 * @param value {@link #system} (An absolute URI which is the code system from which the selected codes come from.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 736 */ 737 public ConceptSetComponent setSystemElement(UriType value) { 738 this.system = value; 739 return this; 740 } 741 742 /** 743 * @return An absolute URI which is the code system from which the selected codes come from. 744 */ 745 public String getSystem() { 746 return this.system == null ? null : this.system.getValue(); 747 } 748 749 /** 750 * @param value An absolute URI which is the code system from which the selected codes come from. 751 */ 752 public ConceptSetComponent setSystem(String value) { 753 if (Utilities.noString(value)) 754 this.system = null; 755 else { 756 if (this.system == null) 757 this.system = new UriType(); 758 this.system.setValue(value); 759 } 760 return this; 761 } 762 763 /** 764 * @return {@link #version} (The version of the code system that the codes are selected from.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 765 */ 766 public StringType getVersionElement() { 767 if (this.version == null) 768 if (Configuration.errorOnAutoCreate()) 769 throw new Error("Attempt to auto-create ConceptSetComponent.version"); 770 else if (Configuration.doAutoCreate()) 771 this.version = new StringType(); // bb 772 return this.version; 773 } 774 775 public boolean hasVersionElement() { 776 return this.version != null && !this.version.isEmpty(); 777 } 778 779 public boolean hasVersion() { 780 return this.version != null && !this.version.isEmpty(); 781 } 782 783 /** 784 * @param value {@link #version} (The version of the code system that the codes are selected from.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 785 */ 786 public ConceptSetComponent setVersionElement(StringType value) { 787 this.version = value; 788 return this; 789 } 790 791 /** 792 * @return The version of the code system that the codes are selected from. 793 */ 794 public String getVersion() { 795 return this.version == null ? null : this.version.getValue(); 796 } 797 798 /** 799 * @param value The version of the code system that the codes are selected from. 800 */ 801 public ConceptSetComponent setVersion(String value) { 802 if (Utilities.noString(value)) 803 this.version = null; 804 else { 805 if (this.version == null) 806 this.version = new StringType(); 807 this.version.setValue(value); 808 } 809 return this; 810 } 811 812 /** 813 * @return {@link #concept} (Specifies a concept to be included or excluded.) 814 */ 815 public List<ConceptReferenceComponent> getConcept() { 816 if (this.concept == null) 817 this.concept = new ArrayList<ConceptReferenceComponent>(); 818 return this.concept; 819 } 820 821 /** 822 * @return Returns a reference to <code>this</code> for easy method chaining 823 */ 824 public ConceptSetComponent setConcept(List<ConceptReferenceComponent> theConcept) { 825 this.concept = theConcept; 826 return this; 827 } 828 829 public boolean hasConcept() { 830 if (this.concept == null) 831 return false; 832 for (ConceptReferenceComponent item : this.concept) 833 if (!item.isEmpty()) 834 return true; 835 return false; 836 } 837 838 public ConceptReferenceComponent addConcept() { //3 839 ConceptReferenceComponent t = new ConceptReferenceComponent(); 840 if (this.concept == null) 841 this.concept = new ArrayList<ConceptReferenceComponent>(); 842 this.concept.add(t); 843 return t; 844 } 845 846 public ConceptSetComponent addConcept(ConceptReferenceComponent t) { //3 847 if (t == null) 848 return this; 849 if (this.concept == null) 850 this.concept = new ArrayList<ConceptReferenceComponent>(); 851 this.concept.add(t); 852 return this; 853 } 854 855 /** 856 * @return The first repetition of repeating field {@link #concept}, creating it if it does not already exist 857 */ 858 public ConceptReferenceComponent getConceptFirstRep() { 859 if (getConcept().isEmpty()) { 860 addConcept(); 861 } 862 return getConcept().get(0); 863 } 864 865 /** 866 * @return {@link #filter} (Select concepts by specify a matching criteria based on the properties (including relationships) defined by the system. If multiple filters are specified, they SHALL all be true.) 867 */ 868 public List<ConceptSetFilterComponent> getFilter() { 869 if (this.filter == null) 870 this.filter = new ArrayList<ConceptSetFilterComponent>(); 871 return this.filter; 872 } 873 874 /** 875 * @return Returns a reference to <code>this</code> for easy method chaining 876 */ 877 public ConceptSetComponent setFilter(List<ConceptSetFilterComponent> theFilter) { 878 this.filter = theFilter; 879 return this; 880 } 881 882 public boolean hasFilter() { 883 if (this.filter == null) 884 return false; 885 for (ConceptSetFilterComponent item : this.filter) 886 if (!item.isEmpty()) 887 return true; 888 return false; 889 } 890 891 public ConceptSetFilterComponent addFilter() { //3 892 ConceptSetFilterComponent t = new ConceptSetFilterComponent(); 893 if (this.filter == null) 894 this.filter = new ArrayList<ConceptSetFilterComponent>(); 895 this.filter.add(t); 896 return t; 897 } 898 899 public ConceptSetComponent addFilter(ConceptSetFilterComponent t) { //3 900 if (t == null) 901 return this; 902 if (this.filter == null) 903 this.filter = new ArrayList<ConceptSetFilterComponent>(); 904 this.filter.add(t); 905 return this; 906 } 907 908 /** 909 * @return The first repetition of repeating field {@link #filter}, creating it if it does not already exist 910 */ 911 public ConceptSetFilterComponent getFilterFirstRep() { 912 if (getFilter().isEmpty()) { 913 addFilter(); 914 } 915 return getFilter().get(0); 916 } 917 918 /** 919 * @return {@link #valueSet} (Selects concepts found in this value set. This is an absolute URI that is a reference to ValueSet.url.) 920 */ 921 public List<UriType> getValueSet() { 922 if (this.valueSet == null) 923 this.valueSet = new ArrayList<UriType>(); 924 return this.valueSet; 925 } 926 927 /** 928 * @return Returns a reference to <code>this</code> for easy method chaining 929 */ 930 public ConceptSetComponent setValueSet(List<UriType> theValueSet) { 931 this.valueSet = theValueSet; 932 return this; 933 } 934 935 public boolean hasValueSet() { 936 if (this.valueSet == null) 937 return false; 938 for (UriType item : this.valueSet) 939 if (!item.isEmpty()) 940 return true; 941 return false; 942 } 943 944 /** 945 * @return {@link #valueSet} (Selects concepts found in this value set. This is an absolute URI that is a reference to ValueSet.url.) 946 */ 947 public UriType addValueSetElement() {//2 948 UriType t = new UriType(); 949 if (this.valueSet == null) 950 this.valueSet = new ArrayList<UriType>(); 951 this.valueSet.add(t); 952 return t; 953 } 954 955 /** 956 * @param value {@link #valueSet} (Selects concepts found in this value set. This is an absolute URI that is a reference to ValueSet.url.) 957 */ 958 public ConceptSetComponent addValueSet(String value) { //1 959 UriType t = new UriType(); 960 t.setValue(value); 961 if (this.valueSet == null) 962 this.valueSet = new ArrayList<UriType>(); 963 this.valueSet.add(t); 964 return this; 965 } 966 967 /** 968 * @param value {@link #valueSet} (Selects concepts found in this value set. This is an absolute URI that is a reference to ValueSet.url.) 969 */ 970 public boolean hasValueSet(String value) { 971 if (this.valueSet == null) 972 return false; 973 for (UriType v : this.valueSet) 974 if (v.getValue().equals(value)) // uri 975 return true; 976 return false; 977 } 978 979 protected void listChildren(List<Property> children) { 980 super.listChildren(children); 981 children.add(new Property("system", "uri", "An absolute URI which is the code system from which the selected codes come from.", 0, 1, system)); 982 children.add(new Property("version", "string", "The version of the code system that the codes are selected from.", 0, 1, version)); 983 children.add(new Property("concept", "", "Specifies a concept to be included or excluded.", 0, java.lang.Integer.MAX_VALUE, concept)); 984 children.add(new Property("filter", "", "Select concepts by specify a matching criteria based on the properties (including relationships) defined by the system. If multiple filters are specified, they SHALL all be true.", 0, java.lang.Integer.MAX_VALUE, filter)); 985 children.add(new Property("valueSet", "uri", "Selects concepts found in this value set. This is an absolute URI that is a reference to ValueSet.url.", 0, java.lang.Integer.MAX_VALUE, valueSet)); 986 } 987 988 @Override 989 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 990 switch (_hash) { 991 case -887328209: /*system*/ return new Property("system", "uri", "An absolute URI which is the code system from which the selected codes come from.", 0, 1, system); 992 case 351608024: /*version*/ return new Property("version", "string", "The version of the code system that the codes are selected from.", 0, 1, version); 993 case 951024232: /*concept*/ return new Property("concept", "", "Specifies a concept to be included or excluded.", 0, java.lang.Integer.MAX_VALUE, concept); 994 case -1274492040: /*filter*/ return new Property("filter", "", "Select concepts by specify a matching criteria based on the properties (including relationships) defined by the system. If multiple filters are specified, they SHALL all be true.", 0, java.lang.Integer.MAX_VALUE, filter); 995 case -1410174671: /*valueSet*/ return new Property("valueSet", "uri", "Selects concepts found in this value set. This is an absolute URI that is a reference to ValueSet.url.", 0, java.lang.Integer.MAX_VALUE, valueSet); 996 default: return super.getNamedProperty(_hash, _name, _checkValid); 997 } 998 999 } 1000 1001 @Override 1002 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1003 switch (hash) { 1004 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 1005 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1006 case 951024232: /*concept*/ return this.concept == null ? new Base[0] : this.concept.toArray(new Base[this.concept.size()]); // ConceptReferenceComponent 1007 case -1274492040: /*filter*/ return this.filter == null ? new Base[0] : this.filter.toArray(new Base[this.filter.size()]); // ConceptSetFilterComponent 1008 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : this.valueSet.toArray(new Base[this.valueSet.size()]); // UriType 1009 default: return super.getProperty(hash, name, checkValid); 1010 } 1011 1012 } 1013 1014 @Override 1015 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1016 switch (hash) { 1017 case -887328209: // system 1018 this.system = castToUri(value); // UriType 1019 return value; 1020 case 351608024: // version 1021 this.version = castToString(value); // StringType 1022 return value; 1023 case 951024232: // concept 1024 this.getConcept().add((ConceptReferenceComponent) value); // ConceptReferenceComponent 1025 return value; 1026 case -1274492040: // filter 1027 this.getFilter().add((ConceptSetFilterComponent) value); // ConceptSetFilterComponent 1028 return value; 1029 case -1410174671: // valueSet 1030 this.getValueSet().add(castToUri(value)); // UriType 1031 return value; 1032 default: return super.setProperty(hash, name, value); 1033 } 1034 1035 } 1036 1037 @Override 1038 public Base setProperty(String name, Base value) throws FHIRException { 1039 if (name.equals("system")) { 1040 this.system = castToUri(value); // UriType 1041 } else if (name.equals("version")) { 1042 this.version = castToString(value); // StringType 1043 } else if (name.equals("concept")) { 1044 this.getConcept().add((ConceptReferenceComponent) value); 1045 } else if (name.equals("filter")) { 1046 this.getFilter().add((ConceptSetFilterComponent) value); 1047 } else if (name.equals("valueSet")) { 1048 this.getValueSet().add(castToUri(value)); 1049 } else 1050 return super.setProperty(name, value); 1051 return value; 1052 } 1053 1054 @Override 1055 public Base makeProperty(int hash, String name) throws FHIRException { 1056 switch (hash) { 1057 case -887328209: return getSystemElement(); 1058 case 351608024: return getVersionElement(); 1059 case 951024232: return addConcept(); 1060 case -1274492040: return addFilter(); 1061 case -1410174671: return addValueSetElement(); 1062 default: return super.makeProperty(hash, name); 1063 } 1064 1065 } 1066 1067 @Override 1068 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1069 switch (hash) { 1070 case -887328209: /*system*/ return new String[] {"uri"}; 1071 case 351608024: /*version*/ return new String[] {"string"}; 1072 case 951024232: /*concept*/ return new String[] {}; 1073 case -1274492040: /*filter*/ return new String[] {}; 1074 case -1410174671: /*valueSet*/ return new String[] {"uri"}; 1075 default: return super.getTypesForProperty(hash, name); 1076 } 1077 1078 } 1079 1080 @Override 1081 public Base addChild(String name) throws FHIRException { 1082 if (name.equals("system")) { 1083 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.system"); 1084 } 1085 else if (name.equals("version")) { 1086 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 1087 } 1088 else if (name.equals("concept")) { 1089 return addConcept(); 1090 } 1091 else if (name.equals("filter")) { 1092 return addFilter(); 1093 } 1094 else if (name.equals("valueSet")) { 1095 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.valueSet"); 1096 } 1097 else 1098 return super.addChild(name); 1099 } 1100 1101 public ConceptSetComponent copy() { 1102 ConceptSetComponent dst = new ConceptSetComponent(); 1103 copyValues(dst); 1104 dst.system = system == null ? null : system.copy(); 1105 dst.version = version == null ? null : version.copy(); 1106 if (concept != null) { 1107 dst.concept = new ArrayList<ConceptReferenceComponent>(); 1108 for (ConceptReferenceComponent i : concept) 1109 dst.concept.add(i.copy()); 1110 }; 1111 if (filter != null) { 1112 dst.filter = new ArrayList<ConceptSetFilterComponent>(); 1113 for (ConceptSetFilterComponent i : filter) 1114 dst.filter.add(i.copy()); 1115 }; 1116 if (valueSet != null) { 1117 dst.valueSet = new ArrayList<UriType>(); 1118 for (UriType i : valueSet) 1119 dst.valueSet.add(i.copy()); 1120 }; 1121 return dst; 1122 } 1123 1124 @Override 1125 public boolean equalsDeep(Base other_) { 1126 if (!super.equalsDeep(other_)) 1127 return false; 1128 if (!(other_ instanceof ConceptSetComponent)) 1129 return false; 1130 ConceptSetComponent o = (ConceptSetComponent) other_; 1131 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) && compareDeep(concept, o.concept, true) 1132 && compareDeep(filter, o.filter, true) && compareDeep(valueSet, o.valueSet, true); 1133 } 1134 1135 @Override 1136 public boolean equalsShallow(Base other_) { 1137 if (!super.equalsShallow(other_)) 1138 return false; 1139 if (!(other_ instanceof ConceptSetComponent)) 1140 return false; 1141 ConceptSetComponent o = (ConceptSetComponent) other_; 1142 return compareValues(system, o.system, true) && compareValues(version, o.version, true) && compareValues(valueSet, o.valueSet, true) 1143 ; 1144 } 1145 1146 public boolean isEmpty() { 1147 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, version, concept 1148 , filter, valueSet); 1149 } 1150 1151 public String fhirType() { 1152 return "ValueSet.compose.include"; 1153 1154 } 1155 1156 } 1157 1158 @Block() 1159 public static class ConceptReferenceComponent extends BackboneElement implements IBaseBackboneElement { 1160 /** 1161 * Specifies a code for the concept to be included or excluded. 1162 */ 1163 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1164 @Description(shortDefinition="Code or expression from system", formalDefinition="Specifies a code for the concept to be included or excluded." ) 1165 protected CodeType code; 1166 1167 /** 1168 * The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system. 1169 */ 1170 @Child(name = "display", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1171 @Description(shortDefinition="Text to display for this code for this value set in this valueset", formalDefinition="The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system." ) 1172 protected StringType display; 1173 1174 /** 1175 * Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc. 1176 */ 1177 @Child(name = "designation", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1178 @Description(shortDefinition="Additional representations for this concept", formalDefinition="Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc." ) 1179 protected List<ConceptReferenceDesignationComponent> designation; 1180 1181 private static final long serialVersionUID = 260579971L; 1182 1183 /** 1184 * Constructor 1185 */ 1186 public ConceptReferenceComponent() { 1187 super(); 1188 } 1189 1190 /** 1191 * Constructor 1192 */ 1193 public ConceptReferenceComponent(CodeType code) { 1194 super(); 1195 this.code = code; 1196 } 1197 1198 /** 1199 * @return {@link #code} (Specifies a code for the concept to be included or excluded.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1200 */ 1201 public CodeType getCodeElement() { 1202 if (this.code == null) 1203 if (Configuration.errorOnAutoCreate()) 1204 throw new Error("Attempt to auto-create ConceptReferenceComponent.code"); 1205 else if (Configuration.doAutoCreate()) 1206 this.code = new CodeType(); // bb 1207 return this.code; 1208 } 1209 1210 public boolean hasCodeElement() { 1211 return this.code != null && !this.code.isEmpty(); 1212 } 1213 1214 public boolean hasCode() { 1215 return this.code != null && !this.code.isEmpty(); 1216 } 1217 1218 /** 1219 * @param value {@link #code} (Specifies a code for the concept to be included or excluded.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1220 */ 1221 public ConceptReferenceComponent setCodeElement(CodeType value) { 1222 this.code = value; 1223 return this; 1224 } 1225 1226 /** 1227 * @return Specifies a code for the concept to be included or excluded. 1228 */ 1229 public String getCode() { 1230 return this.code == null ? null : this.code.getValue(); 1231 } 1232 1233 /** 1234 * @param value Specifies a code for the concept to be included or excluded. 1235 */ 1236 public ConceptReferenceComponent setCode(String value) { 1237 if (this.code == null) 1238 this.code = new CodeType(); 1239 this.code.setValue(value); 1240 return this; 1241 } 1242 1243 /** 1244 * @return {@link #display} (The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1245 */ 1246 public StringType getDisplayElement() { 1247 if (this.display == null) 1248 if (Configuration.errorOnAutoCreate()) 1249 throw new Error("Attempt to auto-create ConceptReferenceComponent.display"); 1250 else if (Configuration.doAutoCreate()) 1251 this.display = new StringType(); // bb 1252 return this.display; 1253 } 1254 1255 public boolean hasDisplayElement() { 1256 return this.display != null && !this.display.isEmpty(); 1257 } 1258 1259 public boolean hasDisplay() { 1260 return this.display != null && !this.display.isEmpty(); 1261 } 1262 1263 /** 1264 * @param value {@link #display} (The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 1265 */ 1266 public ConceptReferenceComponent setDisplayElement(StringType value) { 1267 this.display = value; 1268 return this; 1269 } 1270 1271 /** 1272 * @return The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system. 1273 */ 1274 public String getDisplay() { 1275 return this.display == null ? null : this.display.getValue(); 1276 } 1277 1278 /** 1279 * @param value The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system. 1280 */ 1281 public ConceptReferenceComponent setDisplay(String value) { 1282 if (Utilities.noString(value)) 1283 this.display = null; 1284 else { 1285 if (this.display == null) 1286 this.display = new StringType(); 1287 this.display.setValue(value); 1288 } 1289 return this; 1290 } 1291 1292 /** 1293 * @return {@link #designation} (Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.) 1294 */ 1295 public List<ConceptReferenceDesignationComponent> getDesignation() { 1296 if (this.designation == null) 1297 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1298 return this.designation; 1299 } 1300 1301 /** 1302 * @return Returns a reference to <code>this</code> for easy method chaining 1303 */ 1304 public ConceptReferenceComponent setDesignation(List<ConceptReferenceDesignationComponent> theDesignation) { 1305 this.designation = theDesignation; 1306 return this; 1307 } 1308 1309 public boolean hasDesignation() { 1310 if (this.designation == null) 1311 return false; 1312 for (ConceptReferenceDesignationComponent item : this.designation) 1313 if (!item.isEmpty()) 1314 return true; 1315 return false; 1316 } 1317 1318 public ConceptReferenceDesignationComponent addDesignation() { //3 1319 ConceptReferenceDesignationComponent t = new ConceptReferenceDesignationComponent(); 1320 if (this.designation == null) 1321 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1322 this.designation.add(t); 1323 return t; 1324 } 1325 1326 public ConceptReferenceComponent addDesignation(ConceptReferenceDesignationComponent t) { //3 1327 if (t == null) 1328 return this; 1329 if (this.designation == null) 1330 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1331 this.designation.add(t); 1332 return this; 1333 } 1334 1335 /** 1336 * @return The first repetition of repeating field {@link #designation}, creating it if it does not already exist 1337 */ 1338 public ConceptReferenceDesignationComponent getDesignationFirstRep() { 1339 if (getDesignation().isEmpty()) { 1340 addDesignation(); 1341 } 1342 return getDesignation().get(0); 1343 } 1344 1345 protected void listChildren(List<Property> children) { 1346 super.listChildren(children); 1347 children.add(new Property("code", "code", "Specifies a code for the concept to be included or excluded.", 0, 1, code)); 1348 children.add(new Property("display", "string", "The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.", 0, 1, display)); 1349 children.add(new Property("designation", "", "Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.", 0, java.lang.Integer.MAX_VALUE, designation)); 1350 } 1351 1352 @Override 1353 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1354 switch (_hash) { 1355 case 3059181: /*code*/ return new Property("code", "code", "Specifies a code for the concept to be included or excluded.", 0, 1, code); 1356 case 1671764162: /*display*/ return new Property("display", "string", "The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.", 0, 1, display); 1357 case -900931593: /*designation*/ return new Property("designation", "", "Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.", 0, java.lang.Integer.MAX_VALUE, designation); 1358 default: return super.getNamedProperty(_hash, _name, _checkValid); 1359 } 1360 1361 } 1362 1363 @Override 1364 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1365 switch (hash) { 1366 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 1367 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 1368 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : this.designation.toArray(new Base[this.designation.size()]); // ConceptReferenceDesignationComponent 1369 default: return super.getProperty(hash, name, checkValid); 1370 } 1371 1372 } 1373 1374 @Override 1375 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1376 switch (hash) { 1377 case 3059181: // code 1378 this.code = castToCode(value); // CodeType 1379 return value; 1380 case 1671764162: // display 1381 this.display = castToString(value); // StringType 1382 return value; 1383 case -900931593: // designation 1384 this.getDesignation().add((ConceptReferenceDesignationComponent) value); // ConceptReferenceDesignationComponent 1385 return value; 1386 default: return super.setProperty(hash, name, value); 1387 } 1388 1389 } 1390 1391 @Override 1392 public Base setProperty(String name, Base value) throws FHIRException { 1393 if (name.equals("code")) { 1394 this.code = castToCode(value); // CodeType 1395 } else if (name.equals("display")) { 1396 this.display = castToString(value); // StringType 1397 } else if (name.equals("designation")) { 1398 this.getDesignation().add((ConceptReferenceDesignationComponent) value); 1399 } else 1400 return super.setProperty(name, value); 1401 return value; 1402 } 1403 1404 @Override 1405 public Base makeProperty(int hash, String name) throws FHIRException { 1406 switch (hash) { 1407 case 3059181: return getCodeElement(); 1408 case 1671764162: return getDisplayElement(); 1409 case -900931593: return addDesignation(); 1410 default: return super.makeProperty(hash, name); 1411 } 1412 1413 } 1414 1415 @Override 1416 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1417 switch (hash) { 1418 case 3059181: /*code*/ return new String[] {"code"}; 1419 case 1671764162: /*display*/ return new String[] {"string"}; 1420 case -900931593: /*designation*/ return new String[] {}; 1421 default: return super.getTypesForProperty(hash, name); 1422 } 1423 1424 } 1425 1426 @Override 1427 public Base addChild(String name) throws FHIRException { 1428 if (name.equals("code")) { 1429 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.code"); 1430 } 1431 else if (name.equals("display")) { 1432 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.display"); 1433 } 1434 else if (name.equals("designation")) { 1435 return addDesignation(); 1436 } 1437 else 1438 return super.addChild(name); 1439 } 1440 1441 public ConceptReferenceComponent copy() { 1442 ConceptReferenceComponent dst = new ConceptReferenceComponent(); 1443 copyValues(dst); 1444 dst.code = code == null ? null : code.copy(); 1445 dst.display = display == null ? null : display.copy(); 1446 if (designation != null) { 1447 dst.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1448 for (ConceptReferenceDesignationComponent i : designation) 1449 dst.designation.add(i.copy()); 1450 }; 1451 return dst; 1452 } 1453 1454 @Override 1455 public boolean equalsDeep(Base other_) { 1456 if (!super.equalsDeep(other_)) 1457 return false; 1458 if (!(other_ instanceof ConceptReferenceComponent)) 1459 return false; 1460 ConceptReferenceComponent o = (ConceptReferenceComponent) other_; 1461 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) && compareDeep(designation, o.designation, true) 1462 ; 1463 } 1464 1465 @Override 1466 public boolean equalsShallow(Base other_) { 1467 if (!super.equalsShallow(other_)) 1468 return false; 1469 if (!(other_ instanceof ConceptReferenceComponent)) 1470 return false; 1471 ConceptReferenceComponent o = (ConceptReferenceComponent) other_; 1472 return compareValues(code, o.code, true) && compareValues(display, o.display, true); 1473 } 1474 1475 public boolean isEmpty() { 1476 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, designation 1477 ); 1478 } 1479 1480 public String fhirType() { 1481 return "ValueSet.compose.include.concept"; 1482 1483 } 1484 1485 } 1486 1487 @Block() 1488 public static class ConceptReferenceDesignationComponent extends BackboneElement implements IBaseBackboneElement { 1489 /** 1490 * The language this designation is defined for. 1491 */ 1492 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1493 @Description(shortDefinition="Human language of the designation", formalDefinition="The language this designation is defined for." ) 1494 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 1495 protected CodeType language; 1496 1497 /** 1498 * A code that details how this designation would be used. 1499 */ 1500 @Child(name = "use", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=false) 1501 @Description(shortDefinition="Details how this designation would be used", formalDefinition="A code that details how this designation would be used." ) 1502 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/designation-use") 1503 protected Coding use; 1504 1505 /** 1506 * The text value for this designation. 1507 */ 1508 @Child(name = "value", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=false) 1509 @Description(shortDefinition="The text value for this designation", formalDefinition="The text value for this designation." ) 1510 protected StringType value; 1511 1512 private static final long serialVersionUID = 1515662414L; 1513 1514 /** 1515 * Constructor 1516 */ 1517 public ConceptReferenceDesignationComponent() { 1518 super(); 1519 } 1520 1521 /** 1522 * Constructor 1523 */ 1524 public ConceptReferenceDesignationComponent(StringType value) { 1525 super(); 1526 this.value = value; 1527 } 1528 1529 /** 1530 * @return {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1531 */ 1532 public CodeType getLanguageElement() { 1533 if (this.language == null) 1534 if (Configuration.errorOnAutoCreate()) 1535 throw new Error("Attempt to auto-create ConceptReferenceDesignationComponent.language"); 1536 else if (Configuration.doAutoCreate()) 1537 this.language = new CodeType(); // bb 1538 return this.language; 1539 } 1540 1541 public boolean hasLanguageElement() { 1542 return this.language != null && !this.language.isEmpty(); 1543 } 1544 1545 public boolean hasLanguage() { 1546 return this.language != null && !this.language.isEmpty(); 1547 } 1548 1549 /** 1550 * @param value {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1551 */ 1552 public ConceptReferenceDesignationComponent setLanguageElement(CodeType value) { 1553 this.language = value; 1554 return this; 1555 } 1556 1557 /** 1558 * @return The language this designation is defined for. 1559 */ 1560 public String getLanguage() { 1561 return this.language == null ? null : this.language.getValue(); 1562 } 1563 1564 /** 1565 * @param value The language this designation is defined for. 1566 */ 1567 public ConceptReferenceDesignationComponent setLanguage(String value) { 1568 if (Utilities.noString(value)) 1569 this.language = null; 1570 else { 1571 if (this.language == null) 1572 this.language = new CodeType(); 1573 this.language.setValue(value); 1574 } 1575 return this; 1576 } 1577 1578 /** 1579 * @return {@link #use} (A code that details how this designation would be used.) 1580 */ 1581 public Coding getUse() { 1582 if (this.use == null) 1583 if (Configuration.errorOnAutoCreate()) 1584 throw new Error("Attempt to auto-create ConceptReferenceDesignationComponent.use"); 1585 else if (Configuration.doAutoCreate()) 1586 this.use = new Coding(); // cc 1587 return this.use; 1588 } 1589 1590 public boolean hasUse() { 1591 return this.use != null && !this.use.isEmpty(); 1592 } 1593 1594 /** 1595 * @param value {@link #use} (A code that details how this designation would be used.) 1596 */ 1597 public ConceptReferenceDesignationComponent setUse(Coding value) { 1598 this.use = value; 1599 return this; 1600 } 1601 1602 /** 1603 * @return {@link #value} (The text value for this designation.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1604 */ 1605 public StringType getValueElement() { 1606 if (this.value == null) 1607 if (Configuration.errorOnAutoCreate()) 1608 throw new Error("Attempt to auto-create ConceptReferenceDesignationComponent.value"); 1609 else if (Configuration.doAutoCreate()) 1610 this.value = new StringType(); // bb 1611 return this.value; 1612 } 1613 1614 public boolean hasValueElement() { 1615 return this.value != null && !this.value.isEmpty(); 1616 } 1617 1618 public boolean hasValue() { 1619 return this.value != null && !this.value.isEmpty(); 1620 } 1621 1622 /** 1623 * @param value {@link #value} (The text value for this designation.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1624 */ 1625 public ConceptReferenceDesignationComponent setValueElement(StringType value) { 1626 this.value = value; 1627 return this; 1628 } 1629 1630 /** 1631 * @return The text value for this designation. 1632 */ 1633 public String getValue() { 1634 return this.value == null ? null : this.value.getValue(); 1635 } 1636 1637 /** 1638 * @param value The text value for this designation. 1639 */ 1640 public ConceptReferenceDesignationComponent setValue(String value) { 1641 if (this.value == null) 1642 this.value = new StringType(); 1643 this.value.setValue(value); 1644 return this; 1645 } 1646 1647 protected void listChildren(List<Property> children) { 1648 super.listChildren(children); 1649 children.add(new Property("language", "code", "The language this designation is defined for.", 0, 1, language)); 1650 children.add(new Property("use", "Coding", "A code that details how this designation would be used.", 0, 1, use)); 1651 children.add(new Property("value", "string", "The text value for this designation.", 0, 1, value)); 1652 } 1653 1654 @Override 1655 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1656 switch (_hash) { 1657 case -1613589672: /*language*/ return new Property("language", "code", "The language this designation is defined for.", 0, 1, language); 1658 case 116103: /*use*/ return new Property("use", "Coding", "A code that details how this designation would be used.", 0, 1, use); 1659 case 111972721: /*value*/ return new Property("value", "string", "The text value for this designation.", 0, 1, value); 1660 default: return super.getNamedProperty(_hash, _name, _checkValid); 1661 } 1662 1663 } 1664 1665 @Override 1666 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1667 switch (hash) { 1668 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 1669 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Coding 1670 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 1671 default: return super.getProperty(hash, name, checkValid); 1672 } 1673 1674 } 1675 1676 @Override 1677 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1678 switch (hash) { 1679 case -1613589672: // language 1680 this.language = castToCode(value); // CodeType 1681 return value; 1682 case 116103: // use 1683 this.use = castToCoding(value); // Coding 1684 return value; 1685 case 111972721: // value 1686 this.value = castToString(value); // StringType 1687 return value; 1688 default: return super.setProperty(hash, name, value); 1689 } 1690 1691 } 1692 1693 @Override 1694 public Base setProperty(String name, Base value) throws FHIRException { 1695 if (name.equals("language")) { 1696 this.language = castToCode(value); // CodeType 1697 } else if (name.equals("use")) { 1698 this.use = castToCoding(value); // Coding 1699 } else if (name.equals("value")) { 1700 this.value = castToString(value); // StringType 1701 } else 1702 return super.setProperty(name, value); 1703 return value; 1704 } 1705 1706 @Override 1707 public Base makeProperty(int hash, String name) throws FHIRException { 1708 switch (hash) { 1709 case -1613589672: return getLanguageElement(); 1710 case 116103: return getUse(); 1711 case 111972721: return getValueElement(); 1712 default: return super.makeProperty(hash, name); 1713 } 1714 1715 } 1716 1717 @Override 1718 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1719 switch (hash) { 1720 case -1613589672: /*language*/ return new String[] {"code"}; 1721 case 116103: /*use*/ return new String[] {"Coding"}; 1722 case 111972721: /*value*/ return new String[] {"string"}; 1723 default: return super.getTypesForProperty(hash, name); 1724 } 1725 1726 } 1727 1728 @Override 1729 public Base addChild(String name) throws FHIRException { 1730 if (name.equals("language")) { 1731 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.language"); 1732 } 1733 else if (name.equals("use")) { 1734 this.use = new Coding(); 1735 return this.use; 1736 } 1737 else if (name.equals("value")) { 1738 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.value"); 1739 } 1740 else 1741 return super.addChild(name); 1742 } 1743 1744 public ConceptReferenceDesignationComponent copy() { 1745 ConceptReferenceDesignationComponent dst = new ConceptReferenceDesignationComponent(); 1746 copyValues(dst); 1747 dst.language = language == null ? null : language.copy(); 1748 dst.use = use == null ? null : use.copy(); 1749 dst.value = value == null ? null : value.copy(); 1750 return dst; 1751 } 1752 1753 @Override 1754 public boolean equalsDeep(Base other_) { 1755 if (!super.equalsDeep(other_)) 1756 return false; 1757 if (!(other_ instanceof ConceptReferenceDesignationComponent)) 1758 return false; 1759 ConceptReferenceDesignationComponent o = (ConceptReferenceDesignationComponent) other_; 1760 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true) && compareDeep(value, o.value, true) 1761 ; 1762 } 1763 1764 @Override 1765 public boolean equalsShallow(Base other_) { 1766 if (!super.equalsShallow(other_)) 1767 return false; 1768 if (!(other_ instanceof ConceptReferenceDesignationComponent)) 1769 return false; 1770 ConceptReferenceDesignationComponent o = (ConceptReferenceDesignationComponent) other_; 1771 return compareValues(language, o.language, true) && compareValues(value, o.value, true); 1772 } 1773 1774 public boolean isEmpty() { 1775 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, use, value); 1776 } 1777 1778 public String fhirType() { 1779 return "ValueSet.compose.include.concept.designation"; 1780 1781 } 1782 1783 } 1784 1785 @Block() 1786 public static class ConceptSetFilterComponent extends BackboneElement implements IBaseBackboneElement { 1787 /** 1788 * A code that identifies a property defined in the code system. 1789 */ 1790 @Child(name = "property", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1791 @Description(shortDefinition="A property defined by the code system", formalDefinition="A code that identifies a property defined in the code system." ) 1792 protected CodeType property; 1793 1794 /** 1795 * The kind of operation to perform as a part of the filter criteria. 1796 */ 1797 @Child(name = "op", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1798 @Description(shortDefinition="= | is-a | descendent-of | is-not-a | regex | in | not-in | generalizes | exists", formalDefinition="The kind of operation to perform as a part of the filter criteria." ) 1799 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/filter-operator") 1800 protected Enumeration<FilterOperator> op; 1801 1802 /** 1803 * The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'. 1804 */ 1805 @Child(name = "value", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1806 @Description(shortDefinition="Code from the system, or regex criteria, or boolean value for exists", formalDefinition="The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'." ) 1807 protected CodeType value; 1808 1809 private static final long serialVersionUID = 1985515000L; 1810 1811 /** 1812 * Constructor 1813 */ 1814 public ConceptSetFilterComponent() { 1815 super(); 1816 } 1817 1818 /** 1819 * Constructor 1820 */ 1821 public ConceptSetFilterComponent(CodeType property, Enumeration<FilterOperator> op, CodeType value) { 1822 super(); 1823 this.property = property; 1824 this.op = op; 1825 this.value = value; 1826 } 1827 1828 /** 1829 * @return {@link #property} (A code that identifies a property defined in the code system.). This is the underlying object with id, value and extensions. The accessor "getProperty" gives direct access to the value 1830 */ 1831 public CodeType getPropertyElement() { 1832 if (this.property == null) 1833 if (Configuration.errorOnAutoCreate()) 1834 throw new Error("Attempt to auto-create ConceptSetFilterComponent.property"); 1835 else if (Configuration.doAutoCreate()) 1836 this.property = new CodeType(); // bb 1837 return this.property; 1838 } 1839 1840 public boolean hasPropertyElement() { 1841 return this.property != null && !this.property.isEmpty(); 1842 } 1843 1844 public boolean hasProperty() { 1845 return this.property != null && !this.property.isEmpty(); 1846 } 1847 1848 /** 1849 * @param value {@link #property} (A code that identifies a property defined in the code system.). This is the underlying object with id, value and extensions. The accessor "getProperty" gives direct access to the value 1850 */ 1851 public ConceptSetFilterComponent setPropertyElement(CodeType value) { 1852 this.property = value; 1853 return this; 1854 } 1855 1856 /** 1857 * @return A code that identifies a property defined in the code system. 1858 */ 1859 public String getProperty() { 1860 return this.property == null ? null : this.property.getValue(); 1861 } 1862 1863 /** 1864 * @param value A code that identifies a property defined in the code system. 1865 */ 1866 public ConceptSetFilterComponent setProperty(String value) { 1867 if (this.property == null) 1868 this.property = new CodeType(); 1869 this.property.setValue(value); 1870 return this; 1871 } 1872 1873 /** 1874 * @return {@link #op} (The kind of operation to perform as a part of the filter criteria.). This is the underlying object with id, value and extensions. The accessor "getOp" gives direct access to the value 1875 */ 1876 public Enumeration<FilterOperator> getOpElement() { 1877 if (this.op == null) 1878 if (Configuration.errorOnAutoCreate()) 1879 throw new Error("Attempt to auto-create ConceptSetFilterComponent.op"); 1880 else if (Configuration.doAutoCreate()) 1881 this.op = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); // bb 1882 return this.op; 1883 } 1884 1885 public boolean hasOpElement() { 1886 return this.op != null && !this.op.isEmpty(); 1887 } 1888 1889 public boolean hasOp() { 1890 return this.op != null && !this.op.isEmpty(); 1891 } 1892 1893 /** 1894 * @param value {@link #op} (The kind of operation to perform as a part of the filter criteria.). This is the underlying object with id, value and extensions. The accessor "getOp" gives direct access to the value 1895 */ 1896 public ConceptSetFilterComponent setOpElement(Enumeration<FilterOperator> value) { 1897 this.op = value; 1898 return this; 1899 } 1900 1901 /** 1902 * @return The kind of operation to perform as a part of the filter criteria. 1903 */ 1904 public FilterOperator getOp() { 1905 return this.op == null ? null : this.op.getValue(); 1906 } 1907 1908 /** 1909 * @param value The kind of operation to perform as a part of the filter criteria. 1910 */ 1911 public ConceptSetFilterComponent setOp(FilterOperator value) { 1912 if (this.op == null) 1913 this.op = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); 1914 this.op.setValue(value); 1915 return this; 1916 } 1917 1918 /** 1919 * @return {@link #value} (The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1920 */ 1921 public CodeType getValueElement() { 1922 if (this.value == null) 1923 if (Configuration.errorOnAutoCreate()) 1924 throw new Error("Attempt to auto-create ConceptSetFilterComponent.value"); 1925 else if (Configuration.doAutoCreate()) 1926 this.value = new CodeType(); // bb 1927 return this.value; 1928 } 1929 1930 public boolean hasValueElement() { 1931 return this.value != null && !this.value.isEmpty(); 1932 } 1933 1934 public boolean hasValue() { 1935 return this.value != null && !this.value.isEmpty(); 1936 } 1937 1938 /** 1939 * @param value {@link #value} (The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1940 */ 1941 public ConceptSetFilterComponent setValueElement(CodeType value) { 1942 this.value = value; 1943 return this; 1944 } 1945 1946 /** 1947 * @return The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'. 1948 */ 1949 public String getValue() { 1950 return this.value == null ? null : this.value.getValue(); 1951 } 1952 1953 /** 1954 * @param value The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'. 1955 */ 1956 public ConceptSetFilterComponent setValue(String value) { 1957 if (this.value == null) 1958 this.value = new CodeType(); 1959 this.value.setValue(value); 1960 return this; 1961 } 1962 1963 protected void listChildren(List<Property> children) { 1964 super.listChildren(children); 1965 children.add(new Property("property", "code", "A code that identifies a property defined in the code system.", 0, 1, property)); 1966 children.add(new Property("op", "code", "The kind of operation to perform as a part of the filter criteria.", 0, 1, op)); 1967 children.add(new Property("value", "code", "The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.", 0, 1, value)); 1968 } 1969 1970 @Override 1971 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1972 switch (_hash) { 1973 case -993141291: /*property*/ return new Property("property", "code", "A code that identifies a property defined in the code system.", 0, 1, property); 1974 case 3553: /*op*/ return new Property("op", "code", "The kind of operation to perform as a part of the filter criteria.", 0, 1, op); 1975 case 111972721: /*value*/ return new Property("value", "code", "The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.", 0, 1, value); 1976 default: return super.getNamedProperty(_hash, _name, _checkValid); 1977 } 1978 1979 } 1980 1981 @Override 1982 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1983 switch (hash) { 1984 case -993141291: /*property*/ return this.property == null ? new Base[0] : new Base[] {this.property}; // CodeType 1985 case 3553: /*op*/ return this.op == null ? new Base[0] : new Base[] {this.op}; // Enumeration<FilterOperator> 1986 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // CodeType 1987 default: return super.getProperty(hash, name, checkValid); 1988 } 1989 1990 } 1991 1992 @Override 1993 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1994 switch (hash) { 1995 case -993141291: // property 1996 this.property = castToCode(value); // CodeType 1997 return value; 1998 case 3553: // op 1999 value = new FilterOperatorEnumFactory().fromType(castToCode(value)); 2000 this.op = (Enumeration) value; // Enumeration<FilterOperator> 2001 return value; 2002 case 111972721: // value 2003 this.value = castToCode(value); // CodeType 2004 return value; 2005 default: return super.setProperty(hash, name, value); 2006 } 2007 2008 } 2009 2010 @Override 2011 public Base setProperty(String name, Base value) throws FHIRException { 2012 if (name.equals("property")) { 2013 this.property = castToCode(value); // CodeType 2014 } else if (name.equals("op")) { 2015 value = new FilterOperatorEnumFactory().fromType(castToCode(value)); 2016 this.op = (Enumeration) value; // Enumeration<FilterOperator> 2017 } else if (name.equals("value")) { 2018 this.value = castToCode(value); // CodeType 2019 } else 2020 return super.setProperty(name, value); 2021 return value; 2022 } 2023 2024 @Override 2025 public Base makeProperty(int hash, String name) throws FHIRException { 2026 switch (hash) { 2027 case -993141291: return getPropertyElement(); 2028 case 3553: return getOpElement(); 2029 case 111972721: return getValueElement(); 2030 default: return super.makeProperty(hash, name); 2031 } 2032 2033 } 2034 2035 @Override 2036 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2037 switch (hash) { 2038 case -993141291: /*property*/ return new String[] {"code"}; 2039 case 3553: /*op*/ return new String[] {"code"}; 2040 case 111972721: /*value*/ return new String[] {"code"}; 2041 default: return super.getTypesForProperty(hash, name); 2042 } 2043 2044 } 2045 2046 @Override 2047 public Base addChild(String name) throws FHIRException { 2048 if (name.equals("property")) { 2049 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.property"); 2050 } 2051 else if (name.equals("op")) { 2052 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.op"); 2053 } 2054 else if (name.equals("value")) { 2055 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.value"); 2056 } 2057 else 2058 return super.addChild(name); 2059 } 2060 2061 public ConceptSetFilterComponent copy() { 2062 ConceptSetFilterComponent dst = new ConceptSetFilterComponent(); 2063 copyValues(dst); 2064 dst.property = property == null ? null : property.copy(); 2065 dst.op = op == null ? null : op.copy(); 2066 dst.value = value == null ? null : value.copy(); 2067 return dst; 2068 } 2069 2070 @Override 2071 public boolean equalsDeep(Base other_) { 2072 if (!super.equalsDeep(other_)) 2073 return false; 2074 if (!(other_ instanceof ConceptSetFilterComponent)) 2075 return false; 2076 ConceptSetFilterComponent o = (ConceptSetFilterComponent) other_; 2077 return compareDeep(property, o.property, true) && compareDeep(op, o.op, true) && compareDeep(value, o.value, true) 2078 ; 2079 } 2080 2081 @Override 2082 public boolean equalsShallow(Base other_) { 2083 if (!super.equalsShallow(other_)) 2084 return false; 2085 if (!(other_ instanceof ConceptSetFilterComponent)) 2086 return false; 2087 ConceptSetFilterComponent o = (ConceptSetFilterComponent) other_; 2088 return compareValues(property, o.property, true) && compareValues(op, o.op, true) && compareValues(value, o.value, true) 2089 ; 2090 } 2091 2092 public boolean isEmpty() { 2093 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(property, op, value); 2094 } 2095 2096 public String fhirType() { 2097 return "ValueSet.compose.include.filter"; 2098 2099 } 2100 2101 } 2102 2103 @Block() 2104 public static class ValueSetExpansionComponent extends BackboneElement implements IBaseBackboneElement { 2105 /** 2106 * An identifier that uniquely identifies this expansion of the valueset. Systems may re-use the same identifier as long as the expansion and the definition remain the same, but are not required to do so. 2107 */ 2108 @Child(name = "identifier", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2109 @Description(shortDefinition="Uniquely identifies this expansion", formalDefinition="An identifier that uniquely identifies this expansion of the valueset. Systems may re-use the same identifier as long as the expansion and the definition remain the same, but are not required to do so." ) 2110 protected UriType identifier; 2111 2112 /** 2113 * The time at which the expansion was produced by the expanding system. 2114 */ 2115 @Child(name = "timestamp", type = {DateTimeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2116 @Description(shortDefinition="Time ValueSet expansion happened", formalDefinition="The time at which the expansion was produced by the expanding system." ) 2117 protected DateTimeType timestamp; 2118 2119 /** 2120 * The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter. 2121 */ 2122 @Child(name = "total", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2123 @Description(shortDefinition="Total number of codes in the expansion", formalDefinition="The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter." ) 2124 protected IntegerType total; 2125 2126 /** 2127 * If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL not be present. 2128 */ 2129 @Child(name = "offset", type = {IntegerType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2130 @Description(shortDefinition="Offset at which this resource starts", formalDefinition="If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL not be present." ) 2131 protected IntegerType offset; 2132 2133 /** 2134 * A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion. 2135 */ 2136 @Child(name = "parameter", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2137 @Description(shortDefinition="Parameter that controlled the expansion process", formalDefinition="A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion." ) 2138 protected List<ValueSetExpansionParameterComponent> parameter; 2139 2140 /** 2141 * The codes that are contained in the value set expansion. 2142 */ 2143 @Child(name = "contains", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2144 @Description(shortDefinition="Codes in the value set", formalDefinition="The codes that are contained in the value set expansion." ) 2145 protected List<ValueSetExpansionContainsComponent> contains; 2146 2147 private static final long serialVersionUID = -43471993L; 2148 2149 /** 2150 * Constructor 2151 */ 2152 public ValueSetExpansionComponent() { 2153 super(); 2154 } 2155 2156 /** 2157 * Constructor 2158 */ 2159 public ValueSetExpansionComponent(UriType identifier, DateTimeType timestamp) { 2160 super(); 2161 this.identifier = identifier; 2162 this.timestamp = timestamp; 2163 } 2164 2165 /** 2166 * @return {@link #identifier} (An identifier that uniquely identifies this expansion of the valueset. Systems may re-use the same identifier as long as the expansion and the definition remain the same, but are not required to do so.). This is the underlying object with id, value and extensions. The accessor "getIdentifier" gives direct access to the value 2167 */ 2168 public UriType getIdentifierElement() { 2169 if (this.identifier == null) 2170 if (Configuration.errorOnAutoCreate()) 2171 throw new Error("Attempt to auto-create ValueSetExpansionComponent.identifier"); 2172 else if (Configuration.doAutoCreate()) 2173 this.identifier = new UriType(); // bb 2174 return this.identifier; 2175 } 2176 2177 public boolean hasIdentifierElement() { 2178 return this.identifier != null && !this.identifier.isEmpty(); 2179 } 2180 2181 public boolean hasIdentifier() { 2182 return this.identifier != null && !this.identifier.isEmpty(); 2183 } 2184 2185 /** 2186 * @param value {@link #identifier} (An identifier that uniquely identifies this expansion of the valueset. Systems may re-use the same identifier as long as the expansion and the definition remain the same, but are not required to do so.). This is the underlying object with id, value and extensions. The accessor "getIdentifier" gives direct access to the value 2187 */ 2188 public ValueSetExpansionComponent setIdentifierElement(UriType value) { 2189 this.identifier = value; 2190 return this; 2191 } 2192 2193 /** 2194 * @return An identifier that uniquely identifies this expansion of the valueset. Systems may re-use the same identifier as long as the expansion and the definition remain the same, but are not required to do so. 2195 */ 2196 public String getIdentifier() { 2197 return this.identifier == null ? null : this.identifier.getValue(); 2198 } 2199 2200 /** 2201 * @param value An identifier that uniquely identifies this expansion of the valueset. Systems may re-use the same identifier as long as the expansion and the definition remain the same, but are not required to do so. 2202 */ 2203 public ValueSetExpansionComponent setIdentifier(String value) { 2204 if (this.identifier == null) 2205 this.identifier = new UriType(); 2206 this.identifier.setValue(value); 2207 return this; 2208 } 2209 2210 /** 2211 * @return {@link #timestamp} (The time at which the expansion was produced by the expanding system.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value 2212 */ 2213 public DateTimeType getTimestampElement() { 2214 if (this.timestamp == null) 2215 if (Configuration.errorOnAutoCreate()) 2216 throw new Error("Attempt to auto-create ValueSetExpansionComponent.timestamp"); 2217 else if (Configuration.doAutoCreate()) 2218 this.timestamp = new DateTimeType(); // bb 2219 return this.timestamp; 2220 } 2221 2222 public boolean hasTimestampElement() { 2223 return this.timestamp != null && !this.timestamp.isEmpty(); 2224 } 2225 2226 public boolean hasTimestamp() { 2227 return this.timestamp != null && !this.timestamp.isEmpty(); 2228 } 2229 2230 /** 2231 * @param value {@link #timestamp} (The time at which the expansion was produced by the expanding system.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value 2232 */ 2233 public ValueSetExpansionComponent setTimestampElement(DateTimeType value) { 2234 this.timestamp = value; 2235 return this; 2236 } 2237 2238 /** 2239 * @return The time at which the expansion was produced by the expanding system. 2240 */ 2241 public Date getTimestamp() { 2242 return this.timestamp == null ? null : this.timestamp.getValue(); 2243 } 2244 2245 /** 2246 * @param value The time at which the expansion was produced by the expanding system. 2247 */ 2248 public ValueSetExpansionComponent setTimestamp(Date value) { 2249 if (this.timestamp == null) 2250 this.timestamp = new DateTimeType(); 2251 this.timestamp.setValue(value); 2252 return this; 2253 } 2254 2255 /** 2256 * @return {@link #total} (The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.). This is the underlying object with id, value and extensions. The accessor "getTotal" gives direct access to the value 2257 */ 2258 public IntegerType getTotalElement() { 2259 if (this.total == null) 2260 if (Configuration.errorOnAutoCreate()) 2261 throw new Error("Attempt to auto-create ValueSetExpansionComponent.total"); 2262 else if (Configuration.doAutoCreate()) 2263 this.total = new IntegerType(); // bb 2264 return this.total; 2265 } 2266 2267 public boolean hasTotalElement() { 2268 return this.total != null && !this.total.isEmpty(); 2269 } 2270 2271 public boolean hasTotal() { 2272 return this.total != null && !this.total.isEmpty(); 2273 } 2274 2275 /** 2276 * @param value {@link #total} (The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.). This is the underlying object with id, value and extensions. The accessor "getTotal" gives direct access to the value 2277 */ 2278 public ValueSetExpansionComponent setTotalElement(IntegerType value) { 2279 this.total = value; 2280 return this; 2281 } 2282 2283 /** 2284 * @return The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter. 2285 */ 2286 public int getTotal() { 2287 return this.total == null || this.total.isEmpty() ? 0 : this.total.getValue(); 2288 } 2289 2290 /** 2291 * @param value The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter. 2292 */ 2293 public ValueSetExpansionComponent setTotal(int value) { 2294 if (this.total == null) 2295 this.total = new IntegerType(); 2296 this.total.setValue(value); 2297 return this; 2298 } 2299 2300 /** 2301 * @return {@link #offset} (If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL not be present.). This is the underlying object with id, value and extensions. The accessor "getOffset" gives direct access to the value 2302 */ 2303 public IntegerType getOffsetElement() { 2304 if (this.offset == null) 2305 if (Configuration.errorOnAutoCreate()) 2306 throw new Error("Attempt to auto-create ValueSetExpansionComponent.offset"); 2307 else if (Configuration.doAutoCreate()) 2308 this.offset = new IntegerType(); // bb 2309 return this.offset; 2310 } 2311 2312 public boolean hasOffsetElement() { 2313 return this.offset != null && !this.offset.isEmpty(); 2314 } 2315 2316 public boolean hasOffset() { 2317 return this.offset != null && !this.offset.isEmpty(); 2318 } 2319 2320 /** 2321 * @param value {@link #offset} (If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL not be present.). This is the underlying object with id, value and extensions. The accessor "getOffset" gives direct access to the value 2322 */ 2323 public ValueSetExpansionComponent setOffsetElement(IntegerType value) { 2324 this.offset = value; 2325 return this; 2326 } 2327 2328 /** 2329 * @return If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL not be present. 2330 */ 2331 public int getOffset() { 2332 return this.offset == null || this.offset.isEmpty() ? 0 : this.offset.getValue(); 2333 } 2334 2335 /** 2336 * @param value If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL not be present. 2337 */ 2338 public ValueSetExpansionComponent setOffset(int value) { 2339 if (this.offset == null) 2340 this.offset = new IntegerType(); 2341 this.offset.setValue(value); 2342 return this; 2343 } 2344 2345 /** 2346 * @return {@link #parameter} (A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.) 2347 */ 2348 public List<ValueSetExpansionParameterComponent> getParameter() { 2349 if (this.parameter == null) 2350 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2351 return this.parameter; 2352 } 2353 2354 /** 2355 * @return Returns a reference to <code>this</code> for easy method chaining 2356 */ 2357 public ValueSetExpansionComponent setParameter(List<ValueSetExpansionParameterComponent> theParameter) { 2358 this.parameter = theParameter; 2359 return this; 2360 } 2361 2362 public boolean hasParameter() { 2363 if (this.parameter == null) 2364 return false; 2365 for (ValueSetExpansionParameterComponent item : this.parameter) 2366 if (!item.isEmpty()) 2367 return true; 2368 return false; 2369 } 2370 2371 public ValueSetExpansionParameterComponent addParameter() { //3 2372 ValueSetExpansionParameterComponent t = new ValueSetExpansionParameterComponent(); 2373 if (this.parameter == null) 2374 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2375 this.parameter.add(t); 2376 return t; 2377 } 2378 2379 public ValueSetExpansionComponent addParameter(ValueSetExpansionParameterComponent t) { //3 2380 if (t == null) 2381 return this; 2382 if (this.parameter == null) 2383 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2384 this.parameter.add(t); 2385 return this; 2386 } 2387 2388 /** 2389 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist 2390 */ 2391 public ValueSetExpansionParameterComponent getParameterFirstRep() { 2392 if (getParameter().isEmpty()) { 2393 addParameter(); 2394 } 2395 return getParameter().get(0); 2396 } 2397 2398 /** 2399 * @return {@link #contains} (The codes that are contained in the value set expansion.) 2400 */ 2401 public List<ValueSetExpansionContainsComponent> getContains() { 2402 if (this.contains == null) 2403 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 2404 return this.contains; 2405 } 2406 2407 /** 2408 * @return Returns a reference to <code>this</code> for easy method chaining 2409 */ 2410 public ValueSetExpansionComponent setContains(List<ValueSetExpansionContainsComponent> theContains) { 2411 this.contains = theContains; 2412 return this; 2413 } 2414 2415 public boolean hasContains() { 2416 if (this.contains == null) 2417 return false; 2418 for (ValueSetExpansionContainsComponent item : this.contains) 2419 if (!item.isEmpty()) 2420 return true; 2421 return false; 2422 } 2423 2424 public ValueSetExpansionContainsComponent addContains() { //3 2425 ValueSetExpansionContainsComponent t = new ValueSetExpansionContainsComponent(); 2426 if (this.contains == null) 2427 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 2428 this.contains.add(t); 2429 return t; 2430 } 2431 2432 public ValueSetExpansionComponent addContains(ValueSetExpansionContainsComponent t) { //3 2433 if (t == null) 2434 return this; 2435 if (this.contains == null) 2436 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 2437 this.contains.add(t); 2438 return this; 2439 } 2440 2441 /** 2442 * @return The first repetition of repeating field {@link #contains}, creating it if it does not already exist 2443 */ 2444 public ValueSetExpansionContainsComponent getContainsFirstRep() { 2445 if (getContains().isEmpty()) { 2446 addContains(); 2447 } 2448 return getContains().get(0); 2449 } 2450 2451 protected void listChildren(List<Property> children) { 2452 super.listChildren(children); 2453 children.add(new Property("identifier", "uri", "An identifier that uniquely identifies this expansion of the valueset. Systems may re-use the same identifier as long as the expansion and the definition remain the same, but are not required to do so.", 0, 1, identifier)); 2454 children.add(new Property("timestamp", "dateTime", "The time at which the expansion was produced by the expanding system.", 0, 1, timestamp)); 2455 children.add(new Property("total", "integer", "The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.", 0, 1, total)); 2456 children.add(new Property("offset", "integer", "If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL not be present.", 0, 1, offset)); 2457 children.add(new Property("parameter", "", "A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.", 0, java.lang.Integer.MAX_VALUE, parameter)); 2458 children.add(new Property("contains", "", "The codes that are contained in the value set expansion.", 0, java.lang.Integer.MAX_VALUE, contains)); 2459 } 2460 2461 @Override 2462 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2463 switch (_hash) { 2464 case -1618432855: /*identifier*/ return new Property("identifier", "uri", "An identifier that uniquely identifies this expansion of the valueset. Systems may re-use the same identifier as long as the expansion and the definition remain the same, but are not required to do so.", 0, 1, identifier); 2465 case 55126294: /*timestamp*/ return new Property("timestamp", "dateTime", "The time at which the expansion was produced by the expanding system.", 0, 1, timestamp); 2466 case 110549828: /*total*/ return new Property("total", "integer", "The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.", 0, 1, total); 2467 case -1019779949: /*offset*/ return new Property("offset", "integer", "If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL not be present.", 0, 1, offset); 2468 case 1954460585: /*parameter*/ return new Property("parameter", "", "A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.", 0, java.lang.Integer.MAX_VALUE, parameter); 2469 case -567445985: /*contains*/ return new Property("contains", "", "The codes that are contained in the value set expansion.", 0, java.lang.Integer.MAX_VALUE, contains); 2470 default: return super.getNamedProperty(_hash, _name, _checkValid); 2471 } 2472 2473 } 2474 2475 @Override 2476 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2477 switch (hash) { 2478 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // UriType 2479 case 55126294: /*timestamp*/ return this.timestamp == null ? new Base[0] : new Base[] {this.timestamp}; // DateTimeType 2480 case 110549828: /*total*/ return this.total == null ? new Base[0] : new Base[] {this.total}; // IntegerType 2481 case -1019779949: /*offset*/ return this.offset == null ? new Base[0] : new Base[] {this.offset}; // IntegerType 2482 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // ValueSetExpansionParameterComponent 2483 case -567445985: /*contains*/ return this.contains == null ? new Base[0] : this.contains.toArray(new Base[this.contains.size()]); // ValueSetExpansionContainsComponent 2484 default: return super.getProperty(hash, name, checkValid); 2485 } 2486 2487 } 2488 2489 @Override 2490 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2491 switch (hash) { 2492 case -1618432855: // identifier 2493 this.identifier = castToUri(value); // UriType 2494 return value; 2495 case 55126294: // timestamp 2496 this.timestamp = castToDateTime(value); // DateTimeType 2497 return value; 2498 case 110549828: // total 2499 this.total = castToInteger(value); // IntegerType 2500 return value; 2501 case -1019779949: // offset 2502 this.offset = castToInteger(value); // IntegerType 2503 return value; 2504 case 1954460585: // parameter 2505 this.getParameter().add((ValueSetExpansionParameterComponent) value); // ValueSetExpansionParameterComponent 2506 return value; 2507 case -567445985: // contains 2508 this.getContains().add((ValueSetExpansionContainsComponent) value); // ValueSetExpansionContainsComponent 2509 return value; 2510 default: return super.setProperty(hash, name, value); 2511 } 2512 2513 } 2514 2515 @Override 2516 public Base setProperty(String name, Base value) throws FHIRException { 2517 if (name.equals("identifier")) { 2518 this.identifier = castToUri(value); // UriType 2519 } else if (name.equals("timestamp")) { 2520 this.timestamp = castToDateTime(value); // DateTimeType 2521 } else if (name.equals("total")) { 2522 this.total = castToInteger(value); // IntegerType 2523 } else if (name.equals("offset")) { 2524 this.offset = castToInteger(value); // IntegerType 2525 } else if (name.equals("parameter")) { 2526 this.getParameter().add((ValueSetExpansionParameterComponent) value); 2527 } else if (name.equals("contains")) { 2528 this.getContains().add((ValueSetExpansionContainsComponent) value); 2529 } else 2530 return super.setProperty(name, value); 2531 return value; 2532 } 2533 2534 @Override 2535 public Base makeProperty(int hash, String name) throws FHIRException { 2536 switch (hash) { 2537 case -1618432855: return getIdentifierElement(); 2538 case 55126294: return getTimestampElement(); 2539 case 110549828: return getTotalElement(); 2540 case -1019779949: return getOffsetElement(); 2541 case 1954460585: return addParameter(); 2542 case -567445985: return addContains(); 2543 default: return super.makeProperty(hash, name); 2544 } 2545 2546 } 2547 2548 @Override 2549 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2550 switch (hash) { 2551 case -1618432855: /*identifier*/ return new String[] {"uri"}; 2552 case 55126294: /*timestamp*/ return new String[] {"dateTime"}; 2553 case 110549828: /*total*/ return new String[] {"integer"}; 2554 case -1019779949: /*offset*/ return new String[] {"integer"}; 2555 case 1954460585: /*parameter*/ return new String[] {}; 2556 case -567445985: /*contains*/ return new String[] {}; 2557 default: return super.getTypesForProperty(hash, name); 2558 } 2559 2560 } 2561 2562 @Override 2563 public Base addChild(String name) throws FHIRException { 2564 if (name.equals("identifier")) { 2565 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.identifier"); 2566 } 2567 else if (name.equals("timestamp")) { 2568 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.timestamp"); 2569 } 2570 else if (name.equals("total")) { 2571 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.total"); 2572 } 2573 else if (name.equals("offset")) { 2574 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.offset"); 2575 } 2576 else if (name.equals("parameter")) { 2577 return addParameter(); 2578 } 2579 else if (name.equals("contains")) { 2580 return addContains(); 2581 } 2582 else 2583 return super.addChild(name); 2584 } 2585 2586 public ValueSetExpansionComponent copy() { 2587 ValueSetExpansionComponent dst = new ValueSetExpansionComponent(); 2588 copyValues(dst); 2589 dst.identifier = identifier == null ? null : identifier.copy(); 2590 dst.timestamp = timestamp == null ? null : timestamp.copy(); 2591 dst.total = total == null ? null : total.copy(); 2592 dst.offset = offset == null ? null : offset.copy(); 2593 if (parameter != null) { 2594 dst.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2595 for (ValueSetExpansionParameterComponent i : parameter) 2596 dst.parameter.add(i.copy()); 2597 }; 2598 if (contains != null) { 2599 dst.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 2600 for (ValueSetExpansionContainsComponent i : contains) 2601 dst.contains.add(i.copy()); 2602 }; 2603 return dst; 2604 } 2605 2606 @Override 2607 public boolean equalsDeep(Base other_) { 2608 if (!super.equalsDeep(other_)) 2609 return false; 2610 if (!(other_ instanceof ValueSetExpansionComponent)) 2611 return false; 2612 ValueSetExpansionComponent o = (ValueSetExpansionComponent) other_; 2613 return compareDeep(identifier, o.identifier, true) && compareDeep(timestamp, o.timestamp, true) 2614 && compareDeep(total, o.total, true) && compareDeep(offset, o.offset, true) && compareDeep(parameter, o.parameter, true) 2615 && compareDeep(contains, o.contains, true); 2616 } 2617 2618 @Override 2619 public boolean equalsShallow(Base other_) { 2620 if (!super.equalsShallow(other_)) 2621 return false; 2622 if (!(other_ instanceof ValueSetExpansionComponent)) 2623 return false; 2624 ValueSetExpansionComponent o = (ValueSetExpansionComponent) other_; 2625 return compareValues(identifier, o.identifier, true) && compareValues(timestamp, o.timestamp, true) 2626 && compareValues(total, o.total, true) && compareValues(offset, o.offset, true); 2627 } 2628 2629 public boolean isEmpty() { 2630 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, timestamp, total 2631 , offset, parameter, contains); 2632 } 2633 2634 public String fhirType() { 2635 return "ValueSet.expansion"; 2636 2637 } 2638 2639 } 2640 2641 @Block() 2642 public static class ValueSetExpansionParameterComponent extends BackboneElement implements IBaseBackboneElement { 2643 /** 2644 * The name of the parameter. 2645 */ 2646 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2647 @Description(shortDefinition="Name as assigned by the server", formalDefinition="The name of the parameter." ) 2648 protected StringType name; 2649 2650 /** 2651 * The value of the parameter. 2652 */ 2653 @Child(name = "value", type = {StringType.class, BooleanType.class, IntegerType.class, DecimalType.class, UriType.class, CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2654 @Description(shortDefinition="Value of the named parameter", formalDefinition="The value of the parameter." ) 2655 protected Type value; 2656 2657 private static final long serialVersionUID = 1172641169L; 2658 2659 /** 2660 * Constructor 2661 */ 2662 public ValueSetExpansionParameterComponent() { 2663 super(); 2664 } 2665 2666 /** 2667 * Constructor 2668 */ 2669 public ValueSetExpansionParameterComponent(StringType name) { 2670 super(); 2671 this.name = name; 2672 } 2673 2674 /** 2675 * @return {@link #name} (The name of the parameter.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2676 */ 2677 public StringType getNameElement() { 2678 if (this.name == null) 2679 if (Configuration.errorOnAutoCreate()) 2680 throw new Error("Attempt to auto-create ValueSetExpansionParameterComponent.name"); 2681 else if (Configuration.doAutoCreate()) 2682 this.name = new StringType(); // bb 2683 return this.name; 2684 } 2685 2686 public boolean hasNameElement() { 2687 return this.name != null && !this.name.isEmpty(); 2688 } 2689 2690 public boolean hasName() { 2691 return this.name != null && !this.name.isEmpty(); 2692 } 2693 2694 /** 2695 * @param value {@link #name} (The name of the parameter.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2696 */ 2697 public ValueSetExpansionParameterComponent setNameElement(StringType value) { 2698 this.name = value; 2699 return this; 2700 } 2701 2702 /** 2703 * @return The name of the parameter. 2704 */ 2705 public String getName() { 2706 return this.name == null ? null : this.name.getValue(); 2707 } 2708 2709 /** 2710 * @param value The name of the parameter. 2711 */ 2712 public ValueSetExpansionParameterComponent setName(String value) { 2713 if (this.name == null) 2714 this.name = new StringType(); 2715 this.name.setValue(value); 2716 return this; 2717 } 2718 2719 /** 2720 * @return {@link #value} (The value of the parameter.) 2721 */ 2722 public Type getValue() { 2723 return this.value; 2724 } 2725 2726 /** 2727 * @return {@link #value} (The value of the parameter.) 2728 */ 2729 public StringType getValueStringType() throws FHIRException { 2730 if (this.value == null) 2731 return null; 2732 if (!(this.value instanceof StringType)) 2733 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 2734 return (StringType) this.value; 2735 } 2736 2737 public boolean hasValueStringType() { 2738 return this.value instanceof StringType; 2739 } 2740 2741 /** 2742 * @return {@link #value} (The value of the parameter.) 2743 */ 2744 public BooleanType getValueBooleanType() throws FHIRException { 2745 if (this.value == null) 2746 return null; 2747 if (!(this.value instanceof BooleanType)) 2748 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2749 return (BooleanType) this.value; 2750 } 2751 2752 public boolean hasValueBooleanType() { 2753 return this.value instanceof BooleanType; 2754 } 2755 2756 /** 2757 * @return {@link #value} (The value of the parameter.) 2758 */ 2759 public IntegerType getValueIntegerType() throws FHIRException { 2760 if (this.value == null) 2761 return null; 2762 if (!(this.value instanceof IntegerType)) 2763 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 2764 return (IntegerType) this.value; 2765 } 2766 2767 public boolean hasValueIntegerType() { 2768 return this.value instanceof IntegerType; 2769 } 2770 2771 /** 2772 * @return {@link #value} (The value of the parameter.) 2773 */ 2774 public DecimalType getValueDecimalType() throws FHIRException { 2775 if (this.value == null) 2776 return null; 2777 if (!(this.value instanceof DecimalType)) 2778 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 2779 return (DecimalType) this.value; 2780 } 2781 2782 public boolean hasValueDecimalType() { 2783 return this.value instanceof DecimalType; 2784 } 2785 2786 /** 2787 * @return {@link #value} (The value of the parameter.) 2788 */ 2789 public UriType getValueUriType() throws FHIRException { 2790 if (this.value == null) 2791 return null; 2792 if (!(this.value instanceof UriType)) 2793 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 2794 return (UriType) this.value; 2795 } 2796 2797 public boolean hasValueUriType() { 2798 return this.value instanceof UriType; 2799 } 2800 2801 /** 2802 * @return {@link #value} (The value of the parameter.) 2803 */ 2804 public CodeType getValueCodeType() throws FHIRException { 2805 if (this.value == null) 2806 return null; 2807 if (!(this.value instanceof CodeType)) 2808 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 2809 return (CodeType) this.value; 2810 } 2811 2812 public boolean hasValueCodeType() { 2813 return this.value instanceof CodeType; 2814 } 2815 2816 public boolean hasValue() { 2817 return this.value != null && !this.value.isEmpty(); 2818 } 2819 2820 /** 2821 * @param value {@link #value} (The value of the parameter.) 2822 */ 2823 public ValueSetExpansionParameterComponent setValue(Type value) throws FHIRFormatError { 2824 if (value != null && !(value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof DecimalType || value instanceof UriType || value instanceof CodeType)) 2825 throw new FHIRFormatError("Not the right type for ValueSet.expansion.parameter.value[x]: "+value.fhirType()); 2826 this.value = value; 2827 return this; 2828 } 2829 2830 protected void listChildren(List<Property> children) { 2831 super.listChildren(children); 2832 children.add(new Property("name", "string", "The name of the parameter.", 0, 1, name)); 2833 children.add(new Property("value[x]", "string|boolean|integer|decimal|uri|code", "The value of the parameter.", 0, 1, value)); 2834 } 2835 2836 @Override 2837 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2838 switch (_hash) { 2839 case 3373707: /*name*/ return new Property("name", "string", "The name of the parameter.", 0, 1, name); 2840 case -1410166417: /*value[x]*/ return new Property("value[x]", "string|boolean|integer|decimal|uri|code", "The value of the parameter.", 0, 1, value); 2841 case 111972721: /*value*/ return new Property("value[x]", "string|boolean|integer|decimal|uri|code", "The value of the parameter.", 0, 1, value); 2842 case -1424603934: /*valueString*/ return new Property("value[x]", "string|boolean|integer|decimal|uri|code", "The value of the parameter.", 0, 1, value); 2843 case 733421943: /*valueBoolean*/ return new Property("value[x]", "string|boolean|integer|decimal|uri|code", "The value of the parameter.", 0, 1, value); 2844 case -1668204915: /*valueInteger*/ return new Property("value[x]", "string|boolean|integer|decimal|uri|code", "The value of the parameter.", 0, 1, value); 2845 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "string|boolean|integer|decimal|uri|code", "The value of the parameter.", 0, 1, value); 2846 case -1410172357: /*valueUri*/ return new Property("value[x]", "string|boolean|integer|decimal|uri|code", "The value of the parameter.", 0, 1, value); 2847 case -766209282: /*valueCode*/ return new Property("value[x]", "string|boolean|integer|decimal|uri|code", "The value of the parameter.", 0, 1, value); 2848 default: return super.getNamedProperty(_hash, _name, _checkValid); 2849 } 2850 2851 } 2852 2853 @Override 2854 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2855 switch (hash) { 2856 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2857 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 2858 default: return super.getProperty(hash, name, checkValid); 2859 } 2860 2861 } 2862 2863 @Override 2864 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2865 switch (hash) { 2866 case 3373707: // name 2867 this.name = castToString(value); // StringType 2868 return value; 2869 case 111972721: // value 2870 this.value = castToType(value); // Type 2871 return value; 2872 default: return super.setProperty(hash, name, value); 2873 } 2874 2875 } 2876 2877 @Override 2878 public Base setProperty(String name, Base value) throws FHIRException { 2879 if (name.equals("name")) { 2880 this.name = castToString(value); // StringType 2881 } else if (name.equals("value[x]")) { 2882 this.value = castToType(value); // Type 2883 } else 2884 return super.setProperty(name, value); 2885 return value; 2886 } 2887 2888 @Override 2889 public Base makeProperty(int hash, String name) throws FHIRException { 2890 switch (hash) { 2891 case 3373707: return getNameElement(); 2892 case -1410166417: return getValue(); 2893 case 111972721: return getValue(); 2894 default: return super.makeProperty(hash, name); 2895 } 2896 2897 } 2898 2899 @Override 2900 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2901 switch (hash) { 2902 case 3373707: /*name*/ return new String[] {"string"}; 2903 case 111972721: /*value*/ return new String[] {"string", "boolean", "integer", "decimal", "uri", "code"}; 2904 default: return super.getTypesForProperty(hash, name); 2905 } 2906 2907 } 2908 2909 @Override 2910 public Base addChild(String name) throws FHIRException { 2911 if (name.equals("name")) { 2912 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.name"); 2913 } 2914 else if (name.equals("valueString")) { 2915 this.value = new StringType(); 2916 return this.value; 2917 } 2918 else if (name.equals("valueBoolean")) { 2919 this.value = new BooleanType(); 2920 return this.value; 2921 } 2922 else if (name.equals("valueInteger")) { 2923 this.value = new IntegerType(); 2924 return this.value; 2925 } 2926 else if (name.equals("valueDecimal")) { 2927 this.value = new DecimalType(); 2928 return this.value; 2929 } 2930 else if (name.equals("valueUri")) { 2931 this.value = new UriType(); 2932 return this.value; 2933 } 2934 else if (name.equals("valueCode")) { 2935 this.value = new CodeType(); 2936 return this.value; 2937 } 2938 else 2939 return super.addChild(name); 2940 } 2941 2942 public ValueSetExpansionParameterComponent copy() { 2943 ValueSetExpansionParameterComponent dst = new ValueSetExpansionParameterComponent(); 2944 copyValues(dst); 2945 dst.name = name == null ? null : name.copy(); 2946 dst.value = value == null ? null : value.copy(); 2947 return dst; 2948 } 2949 2950 @Override 2951 public boolean equalsDeep(Base other_) { 2952 if (!super.equalsDeep(other_)) 2953 return false; 2954 if (!(other_ instanceof ValueSetExpansionParameterComponent)) 2955 return false; 2956 ValueSetExpansionParameterComponent o = (ValueSetExpansionParameterComponent) other_; 2957 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 2958 } 2959 2960 @Override 2961 public boolean equalsShallow(Base other_) { 2962 if (!super.equalsShallow(other_)) 2963 return false; 2964 if (!(other_ instanceof ValueSetExpansionParameterComponent)) 2965 return false; 2966 ValueSetExpansionParameterComponent o = (ValueSetExpansionParameterComponent) other_; 2967 return compareValues(name, o.name, true); 2968 } 2969 2970 public boolean isEmpty() { 2971 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 2972 } 2973 2974 public String fhirType() { 2975 return "ValueSet.expansion.parameter"; 2976 2977 } 2978 2979 } 2980 2981 @Block() 2982 public static class ValueSetExpansionContainsComponent extends BackboneElement implements IBaseBackboneElement { 2983 /** 2984 * An absolute URI which is the code system in which the code for this item in the expansion is defined. 2985 */ 2986 @Child(name = "system", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2987 @Description(shortDefinition="System value for the code", formalDefinition="An absolute URI which is the code system in which the code for this item in the expansion is defined." ) 2988 protected UriType system; 2989 2990 /** 2991 * If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value. 2992 */ 2993 @Child(name = "abstract", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2994 @Description(shortDefinition="If user cannot select this entry", formalDefinition="If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value." ) 2995 protected BooleanType abstract_; 2996 2997 /** 2998 * If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. 2999 */ 3000 @Child(name = "inactive", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 3001 @Description(shortDefinition="If concept is inactive in the code system", formalDefinition="If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data." ) 3002 protected BooleanType inactive; 3003 3004 /** 3005 * The version of this code system that defined this code and/or display. This should only be used with code systems that do not enforce concept permanence. 3006 */ 3007 @Child(name = "version", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 3008 @Description(shortDefinition="Version in which this code/display is defined", formalDefinition="The version of this code system that defined this code and/or display. This should only be used with code systems that do not enforce concept permanence." ) 3009 protected StringType version; 3010 3011 /** 3012 * The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set. 3013 */ 3014 @Child(name = "code", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 3015 @Description(shortDefinition="Code - if blank, this is not a selectable code", formalDefinition="The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set." ) 3016 protected CodeType code; 3017 3018 /** 3019 * The recommended display for this item in the expansion. 3020 */ 3021 @Child(name = "display", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 3022 @Description(shortDefinition="User display for the concept", formalDefinition="The recommended display for this item in the expansion." ) 3023 protected StringType display; 3024 3025 /** 3026 * Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation. 3027 */ 3028 @Child(name = "designation", type = {ConceptReferenceDesignationComponent.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3029 @Description(shortDefinition="Additional representations for this item", formalDefinition="Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation." ) 3030 protected List<ConceptReferenceDesignationComponent> designation; 3031 3032 /** 3033 * Other codes and entries contained under this entry in the hierarchy. 3034 */ 3035 @Child(name = "contains", type = {ValueSetExpansionContainsComponent.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3036 @Description(shortDefinition="Codes contained under this entry", formalDefinition="Other codes and entries contained under this entry in the hierarchy." ) 3037 protected List<ValueSetExpansionContainsComponent> contains; 3038 3039 private static final long serialVersionUID = 719458860L; 3040 3041 /** 3042 * Constructor 3043 */ 3044 public ValueSetExpansionContainsComponent() { 3045 super(); 3046 } 3047 3048 /** 3049 * @return {@link #system} (An absolute URI which is the code system in which the code for this item in the expansion is defined.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 3050 */ 3051 public UriType getSystemElement() { 3052 if (this.system == null) 3053 if (Configuration.errorOnAutoCreate()) 3054 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.system"); 3055 else if (Configuration.doAutoCreate()) 3056 this.system = new UriType(); // bb 3057 return this.system; 3058 } 3059 3060 public boolean hasSystemElement() { 3061 return this.system != null && !this.system.isEmpty(); 3062 } 3063 3064 public boolean hasSystem() { 3065 return this.system != null && !this.system.isEmpty(); 3066 } 3067 3068 /** 3069 * @param value {@link #system} (An absolute URI which is the code system in which the code for this item in the expansion is defined.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 3070 */ 3071 public ValueSetExpansionContainsComponent setSystemElement(UriType value) { 3072 this.system = value; 3073 return this; 3074 } 3075 3076 /** 3077 * @return An absolute URI which is the code system in which the code for this item in the expansion is defined. 3078 */ 3079 public String getSystem() { 3080 return this.system == null ? null : this.system.getValue(); 3081 } 3082 3083 /** 3084 * @param value An absolute URI which is the code system in which the code for this item in the expansion is defined. 3085 */ 3086 public ValueSetExpansionContainsComponent setSystem(String value) { 3087 if (Utilities.noString(value)) 3088 this.system = null; 3089 else { 3090 if (this.system == null) 3091 this.system = new UriType(); 3092 this.system.setValue(value); 3093 } 3094 return this; 3095 } 3096 3097 /** 3098 * @return {@link #abstract_} (If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.). This is the underlying object with id, value and extensions. The accessor "getAbstract" gives direct access to the value 3099 */ 3100 public BooleanType getAbstractElement() { 3101 if (this.abstract_ == null) 3102 if (Configuration.errorOnAutoCreate()) 3103 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.abstract_"); 3104 else if (Configuration.doAutoCreate()) 3105 this.abstract_ = new BooleanType(); // bb 3106 return this.abstract_; 3107 } 3108 3109 public boolean hasAbstractElement() { 3110 return this.abstract_ != null && !this.abstract_.isEmpty(); 3111 } 3112 3113 public boolean hasAbstract() { 3114 return this.abstract_ != null && !this.abstract_.isEmpty(); 3115 } 3116 3117 /** 3118 * @param value {@link #abstract_} (If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.). This is the underlying object with id, value and extensions. The accessor "getAbstract" gives direct access to the value 3119 */ 3120 public ValueSetExpansionContainsComponent setAbstractElement(BooleanType value) { 3121 this.abstract_ = value; 3122 return this; 3123 } 3124 3125 /** 3126 * @return If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value. 3127 */ 3128 public boolean getAbstract() { 3129 return this.abstract_ == null || this.abstract_.isEmpty() ? false : this.abstract_.getValue(); 3130 } 3131 3132 /** 3133 * @param value If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value. 3134 */ 3135 public ValueSetExpansionContainsComponent setAbstract(boolean value) { 3136 if (this.abstract_ == null) 3137 this.abstract_ = new BooleanType(); 3138 this.abstract_.setValue(value); 3139 return this; 3140 } 3141 3142 /** 3143 * @return {@link #inactive} (If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data.). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 3144 */ 3145 public BooleanType getInactiveElement() { 3146 if (this.inactive == null) 3147 if (Configuration.errorOnAutoCreate()) 3148 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.inactive"); 3149 else if (Configuration.doAutoCreate()) 3150 this.inactive = new BooleanType(); // bb 3151 return this.inactive; 3152 } 3153 3154 public boolean hasInactiveElement() { 3155 return this.inactive != null && !this.inactive.isEmpty(); 3156 } 3157 3158 public boolean hasInactive() { 3159 return this.inactive != null && !this.inactive.isEmpty(); 3160 } 3161 3162 /** 3163 * @param value {@link #inactive} (If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data.). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 3164 */ 3165 public ValueSetExpansionContainsComponent setInactiveElement(BooleanType value) { 3166 this.inactive = value; 3167 return this; 3168 } 3169 3170 /** 3171 * @return If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. 3172 */ 3173 public boolean getInactive() { 3174 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 3175 } 3176 3177 /** 3178 * @param value If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. 3179 */ 3180 public ValueSetExpansionContainsComponent setInactive(boolean value) { 3181 if (this.inactive == null) 3182 this.inactive = new BooleanType(); 3183 this.inactive.setValue(value); 3184 return this; 3185 } 3186 3187 /** 3188 * @return {@link #version} (The version of this code system that defined this code and/or display. This should only be used with code systems that do not enforce concept permanence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3189 */ 3190 public StringType getVersionElement() { 3191 if (this.version == null) 3192 if (Configuration.errorOnAutoCreate()) 3193 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.version"); 3194 else if (Configuration.doAutoCreate()) 3195 this.version = new StringType(); // bb 3196 return this.version; 3197 } 3198 3199 public boolean hasVersionElement() { 3200 return this.version != null && !this.version.isEmpty(); 3201 } 3202 3203 public boolean hasVersion() { 3204 return this.version != null && !this.version.isEmpty(); 3205 } 3206 3207 /** 3208 * @param value {@link #version} (The version of this code system that defined this code and/or display. This should only be used with code systems that do not enforce concept permanence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3209 */ 3210 public ValueSetExpansionContainsComponent setVersionElement(StringType value) { 3211 this.version = value; 3212 return this; 3213 } 3214 3215 /** 3216 * @return The version of this code system that defined this code and/or display. This should only be used with code systems that do not enforce concept permanence. 3217 */ 3218 public String getVersion() { 3219 return this.version == null ? null : this.version.getValue(); 3220 } 3221 3222 /** 3223 * @param value The version of this code system that defined this code and/or display. This should only be used with code systems that do not enforce concept permanence. 3224 */ 3225 public ValueSetExpansionContainsComponent setVersion(String value) { 3226 if (Utilities.noString(value)) 3227 this.version = null; 3228 else { 3229 if (this.version == null) 3230 this.version = new StringType(); 3231 this.version.setValue(value); 3232 } 3233 return this; 3234 } 3235 3236 /** 3237 * @return {@link #code} (The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3238 */ 3239 public CodeType getCodeElement() { 3240 if (this.code == null) 3241 if (Configuration.errorOnAutoCreate()) 3242 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.code"); 3243 else if (Configuration.doAutoCreate()) 3244 this.code = new CodeType(); // bb 3245 return this.code; 3246 } 3247 3248 public boolean hasCodeElement() { 3249 return this.code != null && !this.code.isEmpty(); 3250 } 3251 3252 public boolean hasCode() { 3253 return this.code != null && !this.code.isEmpty(); 3254 } 3255 3256 /** 3257 * @param value {@link #code} (The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 3258 */ 3259 public ValueSetExpansionContainsComponent setCodeElement(CodeType value) { 3260 this.code = value; 3261 return this; 3262 } 3263 3264 /** 3265 * @return The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set. 3266 */ 3267 public String getCode() { 3268 return this.code == null ? null : this.code.getValue(); 3269 } 3270 3271 /** 3272 * @param value The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set. 3273 */ 3274 public ValueSetExpansionContainsComponent setCode(String value) { 3275 if (Utilities.noString(value)) 3276 this.code = null; 3277 else { 3278 if (this.code == null) 3279 this.code = new CodeType(); 3280 this.code.setValue(value); 3281 } 3282 return this; 3283 } 3284 3285 /** 3286 * @return {@link #display} (The recommended display for this item in the expansion.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 3287 */ 3288 public StringType getDisplayElement() { 3289 if (this.display == null) 3290 if (Configuration.errorOnAutoCreate()) 3291 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.display"); 3292 else if (Configuration.doAutoCreate()) 3293 this.display = new StringType(); // bb 3294 return this.display; 3295 } 3296 3297 public boolean hasDisplayElement() { 3298 return this.display != null && !this.display.isEmpty(); 3299 } 3300 3301 public boolean hasDisplay() { 3302 return this.display != null && !this.display.isEmpty(); 3303 } 3304 3305 /** 3306 * @param value {@link #display} (The recommended display for this item in the expansion.). This is the underlying object with id, value and extensions. The accessor "getDisplay" gives direct access to the value 3307 */ 3308 public ValueSetExpansionContainsComponent setDisplayElement(StringType value) { 3309 this.display = value; 3310 return this; 3311 } 3312 3313 /** 3314 * @return The recommended display for this item in the expansion. 3315 */ 3316 public String getDisplay() { 3317 return this.display == null ? null : this.display.getValue(); 3318 } 3319 3320 /** 3321 * @param value The recommended display for this item in the expansion. 3322 */ 3323 public ValueSetExpansionContainsComponent setDisplay(String value) { 3324 if (Utilities.noString(value)) 3325 this.display = null; 3326 else { 3327 if (this.display == null) 3328 this.display = new StringType(); 3329 this.display.setValue(value); 3330 } 3331 return this; 3332 } 3333 3334 /** 3335 * @return {@link #designation} (Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation.) 3336 */ 3337 public List<ConceptReferenceDesignationComponent> getDesignation() { 3338 if (this.designation == null) 3339 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 3340 return this.designation; 3341 } 3342 3343 /** 3344 * @return Returns a reference to <code>this</code> for easy method chaining 3345 */ 3346 public ValueSetExpansionContainsComponent setDesignation(List<ConceptReferenceDesignationComponent> theDesignation) { 3347 this.designation = theDesignation; 3348 return this; 3349 } 3350 3351 public boolean hasDesignation() { 3352 if (this.designation == null) 3353 return false; 3354 for (ConceptReferenceDesignationComponent item : this.designation) 3355 if (!item.isEmpty()) 3356 return true; 3357 return false; 3358 } 3359 3360 public ConceptReferenceDesignationComponent addDesignation() { //3 3361 ConceptReferenceDesignationComponent t = new ConceptReferenceDesignationComponent(); 3362 if (this.designation == null) 3363 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 3364 this.designation.add(t); 3365 return t; 3366 } 3367 3368 public ValueSetExpansionContainsComponent addDesignation(ConceptReferenceDesignationComponent t) { //3 3369 if (t == null) 3370 return this; 3371 if (this.designation == null) 3372 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 3373 this.designation.add(t); 3374 return this; 3375 } 3376 3377 /** 3378 * @return The first repetition of repeating field {@link #designation}, creating it if it does not already exist 3379 */ 3380 public ConceptReferenceDesignationComponent getDesignationFirstRep() { 3381 if (getDesignation().isEmpty()) { 3382 addDesignation(); 3383 } 3384 return getDesignation().get(0); 3385 } 3386 3387 /** 3388 * @return {@link #contains} (Other codes and entries contained under this entry in the hierarchy.) 3389 */ 3390 public List<ValueSetExpansionContainsComponent> getContains() { 3391 if (this.contains == null) 3392 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3393 return this.contains; 3394 } 3395 3396 /** 3397 * @return Returns a reference to <code>this</code> for easy method chaining 3398 */ 3399 public ValueSetExpansionContainsComponent setContains(List<ValueSetExpansionContainsComponent> theContains) { 3400 this.contains = theContains; 3401 return this; 3402 } 3403 3404 public boolean hasContains() { 3405 if (this.contains == null) 3406 return false; 3407 for (ValueSetExpansionContainsComponent item : this.contains) 3408 if (!item.isEmpty()) 3409 return true; 3410 return false; 3411 } 3412 3413 public ValueSetExpansionContainsComponent addContains() { //3 3414 ValueSetExpansionContainsComponent t = new ValueSetExpansionContainsComponent(); 3415 if (this.contains == null) 3416 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3417 this.contains.add(t); 3418 return t; 3419 } 3420 3421 public ValueSetExpansionContainsComponent addContains(ValueSetExpansionContainsComponent t) { //3 3422 if (t == null) 3423 return this; 3424 if (this.contains == null) 3425 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3426 this.contains.add(t); 3427 return this; 3428 } 3429 3430 /** 3431 * @return The first repetition of repeating field {@link #contains}, creating it if it does not already exist 3432 */ 3433 public ValueSetExpansionContainsComponent getContainsFirstRep() { 3434 if (getContains().isEmpty()) { 3435 addContains(); 3436 } 3437 return getContains().get(0); 3438 } 3439 3440 protected void listChildren(List<Property> children) { 3441 super.listChildren(children); 3442 children.add(new Property("system", "uri", "An absolute URI which is the code system in which the code for this item in the expansion is defined.", 0, 1, system)); 3443 children.add(new Property("abstract", "boolean", "If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.", 0, 1, abstract_)); 3444 children.add(new Property("inactive", "boolean", "If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data.", 0, 1, inactive)); 3445 children.add(new Property("version", "string", "The version of this code system that defined this code and/or display. This should only be used with code systems that do not enforce concept permanence.", 0, 1, version)); 3446 children.add(new Property("code", "code", "The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.", 0, 1, code)); 3447 children.add(new Property("display", "string", "The recommended display for this item in the expansion.", 0, 1, display)); 3448 children.add(new Property("designation", "@ValueSet.compose.include.concept.designation", "Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation.", 0, java.lang.Integer.MAX_VALUE, designation)); 3449 children.add(new Property("contains", "@ValueSet.expansion.contains", "Other codes and entries contained under this entry in the hierarchy.", 0, java.lang.Integer.MAX_VALUE, contains)); 3450 } 3451 3452 @Override 3453 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3454 switch (_hash) { 3455 case -887328209: /*system*/ return new Property("system", "uri", "An absolute URI which is the code system in which the code for this item in the expansion is defined.", 0, 1, system); 3456 case 1732898850: /*abstract*/ return new Property("abstract", "boolean", "If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.", 0, 1, abstract_); 3457 case 24665195: /*inactive*/ return new Property("inactive", "boolean", "If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data.", 0, 1, inactive); 3458 case 351608024: /*version*/ return new Property("version", "string", "The version of this code system that defined this code and/or display. This should only be used with code systems that do not enforce concept permanence.", 0, 1, version); 3459 case 3059181: /*code*/ return new Property("code", "code", "The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.", 0, 1, code); 3460 case 1671764162: /*display*/ return new Property("display", "string", "The recommended display for this item in the expansion.", 0, 1, display); 3461 case -900931593: /*designation*/ return new Property("designation", "@ValueSet.compose.include.concept.designation", "Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation.", 0, java.lang.Integer.MAX_VALUE, designation); 3462 case -567445985: /*contains*/ return new Property("contains", "@ValueSet.expansion.contains", "Other codes and entries contained under this entry in the hierarchy.", 0, java.lang.Integer.MAX_VALUE, contains); 3463 default: return super.getNamedProperty(_hash, _name, _checkValid); 3464 } 3465 3466 } 3467 3468 @Override 3469 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3470 switch (hash) { 3471 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 3472 case 1732898850: /*abstract*/ return this.abstract_ == null ? new Base[0] : new Base[] {this.abstract_}; // BooleanType 3473 case 24665195: /*inactive*/ return this.inactive == null ? new Base[0] : new Base[] {this.inactive}; // BooleanType 3474 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3475 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 3476 case 1671764162: /*display*/ return this.display == null ? new Base[0] : new Base[] {this.display}; // StringType 3477 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : this.designation.toArray(new Base[this.designation.size()]); // ConceptReferenceDesignationComponent 3478 case -567445985: /*contains*/ return this.contains == null ? new Base[0] : this.contains.toArray(new Base[this.contains.size()]); // ValueSetExpansionContainsComponent 3479 default: return super.getProperty(hash, name, checkValid); 3480 } 3481 3482 } 3483 3484 @Override 3485 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3486 switch (hash) { 3487 case -887328209: // system 3488 this.system = castToUri(value); // UriType 3489 return value; 3490 case 1732898850: // abstract 3491 this.abstract_ = castToBoolean(value); // BooleanType 3492 return value; 3493 case 24665195: // inactive 3494 this.inactive = castToBoolean(value); // BooleanType 3495 return value; 3496 case 351608024: // version 3497 this.version = castToString(value); // StringType 3498 return value; 3499 case 3059181: // code 3500 this.code = castToCode(value); // CodeType 3501 return value; 3502 case 1671764162: // display 3503 this.display = castToString(value); // StringType 3504 return value; 3505 case -900931593: // designation 3506 this.getDesignation().add((ConceptReferenceDesignationComponent) value); // ConceptReferenceDesignationComponent 3507 return value; 3508 case -567445985: // contains 3509 this.getContains().add((ValueSetExpansionContainsComponent) value); // ValueSetExpansionContainsComponent 3510 return value; 3511 default: return super.setProperty(hash, name, value); 3512 } 3513 3514 } 3515 3516 @Override 3517 public Base setProperty(String name, Base value) throws FHIRException { 3518 if (name.equals("system")) { 3519 this.system = castToUri(value); // UriType 3520 } else if (name.equals("abstract")) { 3521 this.abstract_ = castToBoolean(value); // BooleanType 3522 } else if (name.equals("inactive")) { 3523 this.inactive = castToBoolean(value); // BooleanType 3524 } else if (name.equals("version")) { 3525 this.version = castToString(value); // StringType 3526 } else if (name.equals("code")) { 3527 this.code = castToCode(value); // CodeType 3528 } else if (name.equals("display")) { 3529 this.display = castToString(value); // StringType 3530 } else if (name.equals("designation")) { 3531 this.getDesignation().add((ConceptReferenceDesignationComponent) value); 3532 } else if (name.equals("contains")) { 3533 this.getContains().add((ValueSetExpansionContainsComponent) value); 3534 } else 3535 return super.setProperty(name, value); 3536 return value; 3537 } 3538 3539 @Override 3540 public Base makeProperty(int hash, String name) throws FHIRException { 3541 switch (hash) { 3542 case -887328209: return getSystemElement(); 3543 case 1732898850: return getAbstractElement(); 3544 case 24665195: return getInactiveElement(); 3545 case 351608024: return getVersionElement(); 3546 case 3059181: return getCodeElement(); 3547 case 1671764162: return getDisplayElement(); 3548 case -900931593: return addDesignation(); 3549 case -567445985: return addContains(); 3550 default: return super.makeProperty(hash, name); 3551 } 3552 3553 } 3554 3555 @Override 3556 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3557 switch (hash) { 3558 case -887328209: /*system*/ return new String[] {"uri"}; 3559 case 1732898850: /*abstract*/ return new String[] {"boolean"}; 3560 case 24665195: /*inactive*/ return new String[] {"boolean"}; 3561 case 351608024: /*version*/ return new String[] {"string"}; 3562 case 3059181: /*code*/ return new String[] {"code"}; 3563 case 1671764162: /*display*/ return new String[] {"string"}; 3564 case -900931593: /*designation*/ return new String[] {"@ValueSet.compose.include.concept.designation"}; 3565 case -567445985: /*contains*/ return new String[] {"@ValueSet.expansion.contains"}; 3566 default: return super.getTypesForProperty(hash, name); 3567 } 3568 3569 } 3570 3571 @Override 3572 public Base addChild(String name) throws FHIRException { 3573 if (name.equals("system")) { 3574 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.system"); 3575 } 3576 else if (name.equals("abstract")) { 3577 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.abstract"); 3578 } 3579 else if (name.equals("inactive")) { 3580 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.inactive"); 3581 } 3582 else if (name.equals("version")) { 3583 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 3584 } 3585 else if (name.equals("code")) { 3586 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.code"); 3587 } 3588 else if (name.equals("display")) { 3589 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.display"); 3590 } 3591 else if (name.equals("designation")) { 3592 return addDesignation(); 3593 } 3594 else if (name.equals("contains")) { 3595 return addContains(); 3596 } 3597 else 3598 return super.addChild(name); 3599 } 3600 3601 public ValueSetExpansionContainsComponent copy() { 3602 ValueSetExpansionContainsComponent dst = new ValueSetExpansionContainsComponent(); 3603 copyValues(dst); 3604 dst.system = system == null ? null : system.copy(); 3605 dst.abstract_ = abstract_ == null ? null : abstract_.copy(); 3606 dst.inactive = inactive == null ? null : inactive.copy(); 3607 dst.version = version == null ? null : version.copy(); 3608 dst.code = code == null ? null : code.copy(); 3609 dst.display = display == null ? null : display.copy(); 3610 if (designation != null) { 3611 dst.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 3612 for (ConceptReferenceDesignationComponent i : designation) 3613 dst.designation.add(i.copy()); 3614 }; 3615 if (contains != null) { 3616 dst.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3617 for (ValueSetExpansionContainsComponent i : contains) 3618 dst.contains.add(i.copy()); 3619 }; 3620 return dst; 3621 } 3622 3623 @Override 3624 public boolean equalsDeep(Base other_) { 3625 if (!super.equalsDeep(other_)) 3626 return false; 3627 if (!(other_ instanceof ValueSetExpansionContainsComponent)) 3628 return false; 3629 ValueSetExpansionContainsComponent o = (ValueSetExpansionContainsComponent) other_; 3630 return compareDeep(system, o.system, true) && compareDeep(abstract_, o.abstract_, true) && compareDeep(inactive, o.inactive, true) 3631 && compareDeep(version, o.version, true) && compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 3632 && compareDeep(designation, o.designation, true) && compareDeep(contains, o.contains, true); 3633 } 3634 3635 @Override 3636 public boolean equalsShallow(Base other_) { 3637 if (!super.equalsShallow(other_)) 3638 return false; 3639 if (!(other_ instanceof ValueSetExpansionContainsComponent)) 3640 return false; 3641 ValueSetExpansionContainsComponent o = (ValueSetExpansionContainsComponent) other_; 3642 return compareValues(system, o.system, true) && compareValues(abstract_, o.abstract_, true) && compareValues(inactive, o.inactive, true) 3643 && compareValues(version, o.version, true) && compareValues(code, o.code, true) && compareValues(display, o.display, true) 3644 ; 3645 } 3646 3647 public boolean isEmpty() { 3648 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, abstract_, inactive 3649 , version, code, display, designation, contains); 3650 } 3651 3652 public String fhirType() { 3653 return "ValueSet.expansion.contains"; 3654 3655 } 3656 3657 } 3658 3659 /** 3660 * A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance. 3661 */ 3662 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3663 @Description(shortDefinition="Additional identifier for the value set", formalDefinition="A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 3664 protected List<Identifier> identifier; 3665 3666 /** 3667 * If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change. 3668 */ 3669 @Child(name = "immutable", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=true) 3670 @Description(shortDefinition="Indicates whether or not any change to the content logical definition may occur", formalDefinition="If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change." ) 3671 protected BooleanType immutable; 3672 3673 /** 3674 * Explaination of why this value set is needed and why it has been designed as it has. 3675 */ 3676 @Child(name = "purpose", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3677 @Description(shortDefinition="Why this value set is defined", formalDefinition="Explaination of why this value set is needed and why it has been designed as it has." ) 3678 protected MarkdownType purpose; 3679 3680 /** 3681 * A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set. 3682 */ 3683 @Child(name = "copyright", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 3684 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set." ) 3685 protected MarkdownType copyright; 3686 3687 /** 3688 * Whether this is intended to be used with an extensible binding or not. 3689 */ 3690 @Child(name = "extensible", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3691 @Description(shortDefinition="Whether this is intended to be used with an extensible binding", formalDefinition="Whether this is intended to be used with an extensible binding or not." ) 3692 protected BooleanType extensible; 3693 3694 /** 3695 * A set of criteria that define the content logical definition of the value set by including or excluding codes from outside this value set. This I also known as the "Content Logical Definition" (CLD). 3696 */ 3697 @Child(name = "compose", type = {}, order=5, min=0, max=1, modifier=false, summary=false) 3698 @Description(shortDefinition="Definition of the content of the value set (CLD)", formalDefinition="A set of criteria that define the content logical definition of the value set by including or excluding codes from outside this value set. This I also known as the \"Content Logical Definition\" (CLD)." ) 3699 protected ValueSetComposeComponent compose; 3700 3701 /** 3702 * A value set can also be "expanded", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed. 3703 */ 3704 @Child(name = "expansion", type = {}, order=6, min=0, max=1, modifier=false, summary=false) 3705 @Description(shortDefinition="Used when the value set is \"expanded\"", formalDefinition="A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed." ) 3706 protected ValueSetExpansionComponent expansion; 3707 3708 private static final long serialVersionUID = -173192200L; 3709 3710 /** 3711 * Constructor 3712 */ 3713 public ValueSet() { 3714 super(); 3715 } 3716 3717 /** 3718 * Constructor 3719 */ 3720 public ValueSet(Enumeration<PublicationStatus> status) { 3721 super(); 3722 this.status = status; 3723 } 3724 3725 /** 3726 * @return {@link #url} (An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this value set is (or will be) published. The URL SHOULD include the major version of the value set. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3727 */ 3728 public UriType getUrlElement() { 3729 if (this.url == null) 3730 if (Configuration.errorOnAutoCreate()) 3731 throw new Error("Attempt to auto-create ValueSet.url"); 3732 else if (Configuration.doAutoCreate()) 3733 this.url = new UriType(); // bb 3734 return this.url; 3735 } 3736 3737 public boolean hasUrlElement() { 3738 return this.url != null && !this.url.isEmpty(); 3739 } 3740 3741 public boolean hasUrl() { 3742 return this.url != null && !this.url.isEmpty(); 3743 } 3744 3745 /** 3746 * @param value {@link #url} (An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this value set is (or will be) published. The URL SHOULD include the major version of the value set. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3747 */ 3748 public ValueSet setUrlElement(UriType value) { 3749 this.url = value; 3750 return this; 3751 } 3752 3753 /** 3754 * @return An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this value set is (or will be) published. The URL SHOULD include the major version of the value set. For more information see [Technical and Business Versions](resource.html#versions). 3755 */ 3756 public String getUrl() { 3757 return this.url == null ? null : this.url.getValue(); 3758 } 3759 3760 /** 3761 * @param value An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this value set is (or will be) published. The URL SHOULD include the major version of the value set. For more information see [Technical and Business Versions](resource.html#versions). 3762 */ 3763 public ValueSet setUrl(String value) { 3764 if (Utilities.noString(value)) 3765 this.url = null; 3766 else { 3767 if (this.url == null) 3768 this.url = new UriType(); 3769 this.url.setValue(value); 3770 } 3771 return this; 3772 } 3773 3774 /** 3775 * @return {@link #identifier} (A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.) 3776 */ 3777 public List<Identifier> getIdentifier() { 3778 if (this.identifier == null) 3779 this.identifier = new ArrayList<Identifier>(); 3780 return this.identifier; 3781 } 3782 3783 /** 3784 * @return Returns a reference to <code>this</code> for easy method chaining 3785 */ 3786 public ValueSet setIdentifier(List<Identifier> theIdentifier) { 3787 this.identifier = theIdentifier; 3788 return this; 3789 } 3790 3791 public boolean hasIdentifier() { 3792 if (this.identifier == null) 3793 return false; 3794 for (Identifier item : this.identifier) 3795 if (!item.isEmpty()) 3796 return true; 3797 return false; 3798 } 3799 3800 public Identifier addIdentifier() { //3 3801 Identifier t = new Identifier(); 3802 if (this.identifier == null) 3803 this.identifier = new ArrayList<Identifier>(); 3804 this.identifier.add(t); 3805 return t; 3806 } 3807 3808 public ValueSet addIdentifier(Identifier t) { //3 3809 if (t == null) 3810 return this; 3811 if (this.identifier == null) 3812 this.identifier = new ArrayList<Identifier>(); 3813 this.identifier.add(t); 3814 return this; 3815 } 3816 3817 /** 3818 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 3819 */ 3820 public Identifier getIdentifierFirstRep() { 3821 if (getIdentifier().isEmpty()) { 3822 addIdentifier(); 3823 } 3824 return getIdentifier().get(0); 3825 } 3826 3827 /** 3828 * @return {@link #version} (The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3829 */ 3830 public StringType getVersionElement() { 3831 if (this.version == null) 3832 if (Configuration.errorOnAutoCreate()) 3833 throw new Error("Attempt to auto-create ValueSet.version"); 3834 else if (Configuration.doAutoCreate()) 3835 this.version = new StringType(); // bb 3836 return this.version; 3837 } 3838 3839 public boolean hasVersionElement() { 3840 return this.version != null && !this.version.isEmpty(); 3841 } 3842 3843 public boolean hasVersion() { 3844 return this.version != null && !this.version.isEmpty(); 3845 } 3846 3847 /** 3848 * @param value {@link #version} (The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3849 */ 3850 public ValueSet setVersionElement(StringType value) { 3851 this.version = value; 3852 return this; 3853 } 3854 3855 /** 3856 * @return The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 3857 */ 3858 public String getVersion() { 3859 return this.version == null ? null : this.version.getValue(); 3860 } 3861 3862 /** 3863 * @param value The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 3864 */ 3865 public ValueSet setVersion(String value) { 3866 if (Utilities.noString(value)) 3867 this.version = null; 3868 else { 3869 if (this.version == null) 3870 this.version = new StringType(); 3871 this.version.setValue(value); 3872 } 3873 return this; 3874 } 3875 3876 /** 3877 * @return {@link #name} (A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3878 */ 3879 public StringType getNameElement() { 3880 if (this.name == null) 3881 if (Configuration.errorOnAutoCreate()) 3882 throw new Error("Attempt to auto-create ValueSet.name"); 3883 else if (Configuration.doAutoCreate()) 3884 this.name = new StringType(); // bb 3885 return this.name; 3886 } 3887 3888 public boolean hasNameElement() { 3889 return this.name != null && !this.name.isEmpty(); 3890 } 3891 3892 public boolean hasName() { 3893 return this.name != null && !this.name.isEmpty(); 3894 } 3895 3896 /** 3897 * @param value {@link #name} (A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3898 */ 3899 public ValueSet setNameElement(StringType value) { 3900 this.name = value; 3901 return this; 3902 } 3903 3904 /** 3905 * @return A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3906 */ 3907 public String getName() { 3908 return this.name == null ? null : this.name.getValue(); 3909 } 3910 3911 /** 3912 * @param value A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3913 */ 3914 public ValueSet setName(String value) { 3915 if (Utilities.noString(value)) 3916 this.name = null; 3917 else { 3918 if (this.name == null) 3919 this.name = new StringType(); 3920 this.name.setValue(value); 3921 } 3922 return this; 3923 } 3924 3925 /** 3926 * @return {@link #title} (A short, descriptive, user-friendly title for the value set.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3927 */ 3928 public StringType getTitleElement() { 3929 if (this.title == null) 3930 if (Configuration.errorOnAutoCreate()) 3931 throw new Error("Attempt to auto-create ValueSet.title"); 3932 else if (Configuration.doAutoCreate()) 3933 this.title = new StringType(); // bb 3934 return this.title; 3935 } 3936 3937 public boolean hasTitleElement() { 3938 return this.title != null && !this.title.isEmpty(); 3939 } 3940 3941 public boolean hasTitle() { 3942 return this.title != null && !this.title.isEmpty(); 3943 } 3944 3945 /** 3946 * @param value {@link #title} (A short, descriptive, user-friendly title for the value set.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3947 */ 3948 public ValueSet setTitleElement(StringType value) { 3949 this.title = value; 3950 return this; 3951 } 3952 3953 /** 3954 * @return A short, descriptive, user-friendly title for the value set. 3955 */ 3956 public String getTitle() { 3957 return this.title == null ? null : this.title.getValue(); 3958 } 3959 3960 /** 3961 * @param value A short, descriptive, user-friendly title for the value set. 3962 */ 3963 public ValueSet setTitle(String value) { 3964 if (Utilities.noString(value)) 3965 this.title = null; 3966 else { 3967 if (this.title == null) 3968 this.title = new StringType(); 3969 this.title.setValue(value); 3970 } 3971 return this; 3972 } 3973 3974 /** 3975 * @return {@link #status} (The status of this value set. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3976 */ 3977 public Enumeration<PublicationStatus> getStatusElement() { 3978 if (this.status == null) 3979 if (Configuration.errorOnAutoCreate()) 3980 throw new Error("Attempt to auto-create ValueSet.status"); 3981 else if (Configuration.doAutoCreate()) 3982 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3983 return this.status; 3984 } 3985 3986 public boolean hasStatusElement() { 3987 return this.status != null && !this.status.isEmpty(); 3988 } 3989 3990 public boolean hasStatus() { 3991 return this.status != null && !this.status.isEmpty(); 3992 } 3993 3994 /** 3995 * @param value {@link #status} (The status of this value set. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3996 */ 3997 public ValueSet setStatusElement(Enumeration<PublicationStatus> value) { 3998 this.status = value; 3999 return this; 4000 } 4001 4002 /** 4003 * @return The status of this value set. Enables tracking the life-cycle of the content. 4004 */ 4005 public PublicationStatus getStatus() { 4006 return this.status == null ? null : this.status.getValue(); 4007 } 4008 4009 /** 4010 * @param value The status of this value set. Enables tracking the life-cycle of the content. 4011 */ 4012 public ValueSet setStatus(PublicationStatus value) { 4013 if (this.status == null) 4014 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 4015 this.status.setValue(value); 4016 return this; 4017 } 4018 4019 /** 4020 * @return {@link #experimental} (A boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 4021 */ 4022 public BooleanType getExperimentalElement() { 4023 if (this.experimental == null) 4024 if (Configuration.errorOnAutoCreate()) 4025 throw new Error("Attempt to auto-create ValueSet.experimental"); 4026 else if (Configuration.doAutoCreate()) 4027 this.experimental = new BooleanType(); // bb 4028 return this.experimental; 4029 } 4030 4031 public boolean hasExperimentalElement() { 4032 return this.experimental != null && !this.experimental.isEmpty(); 4033 } 4034 4035 public boolean hasExperimental() { 4036 return this.experimental != null && !this.experimental.isEmpty(); 4037 } 4038 4039 /** 4040 * @param value {@link #experimental} (A boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 4041 */ 4042 public ValueSet setExperimentalElement(BooleanType value) { 4043 this.experimental = value; 4044 return this; 4045 } 4046 4047 /** 4048 * @return A boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 4049 */ 4050 public boolean getExperimental() { 4051 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 4052 } 4053 4054 /** 4055 * @param value A boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 4056 */ 4057 public ValueSet setExperimental(boolean value) { 4058 if (this.experimental == null) 4059 this.experimental = new BooleanType(); 4060 this.experimental.setValue(value); 4061 return this; 4062 } 4063 4064 /** 4065 * @return {@link #date} (The date (and optionally time) when the value set was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the value set changes. (e.g. the 'content logical definition').). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4066 */ 4067 public DateTimeType getDateElement() { 4068 if (this.date == null) 4069 if (Configuration.errorOnAutoCreate()) 4070 throw new Error("Attempt to auto-create ValueSet.date"); 4071 else if (Configuration.doAutoCreate()) 4072 this.date = new DateTimeType(); // bb 4073 return this.date; 4074 } 4075 4076 public boolean hasDateElement() { 4077 return this.date != null && !this.date.isEmpty(); 4078 } 4079 4080 public boolean hasDate() { 4081 return this.date != null && !this.date.isEmpty(); 4082 } 4083 4084 /** 4085 * @param value {@link #date} (The date (and optionally time) when the value set was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the value set changes. (e.g. the 'content logical definition').). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4086 */ 4087 public ValueSet setDateElement(DateTimeType value) { 4088 this.date = value; 4089 return this; 4090 } 4091 4092 /** 4093 * @return The date (and optionally time) when the value set was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the value set changes. (e.g. the 'content logical definition'). 4094 */ 4095 public Date getDate() { 4096 return this.date == null ? null : this.date.getValue(); 4097 } 4098 4099 /** 4100 * @param value The date (and optionally time) when the value set was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the value set changes. (e.g. the 'content logical definition'). 4101 */ 4102 public ValueSet setDate(Date value) { 4103 if (value == null) 4104 this.date = null; 4105 else { 4106 if (this.date == null) 4107 this.date = new DateTimeType(); 4108 this.date.setValue(value); 4109 } 4110 return this; 4111 } 4112 4113 /** 4114 * @return {@link #publisher} (The name of the individual or organization that published the value set.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 4115 */ 4116 public StringType getPublisherElement() { 4117 if (this.publisher == null) 4118 if (Configuration.errorOnAutoCreate()) 4119 throw new Error("Attempt to auto-create ValueSet.publisher"); 4120 else if (Configuration.doAutoCreate()) 4121 this.publisher = new StringType(); // bb 4122 return this.publisher; 4123 } 4124 4125 public boolean hasPublisherElement() { 4126 return this.publisher != null && !this.publisher.isEmpty(); 4127 } 4128 4129 public boolean hasPublisher() { 4130 return this.publisher != null && !this.publisher.isEmpty(); 4131 } 4132 4133 /** 4134 * @param value {@link #publisher} (The name of the individual or organization that published the value set.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 4135 */ 4136 public ValueSet setPublisherElement(StringType value) { 4137 this.publisher = value; 4138 return this; 4139 } 4140 4141 /** 4142 * @return The name of the individual or organization that published the value set. 4143 */ 4144 public String getPublisher() { 4145 return this.publisher == null ? null : this.publisher.getValue(); 4146 } 4147 4148 /** 4149 * @param value The name of the individual or organization that published the value set. 4150 */ 4151 public ValueSet setPublisher(String value) { 4152 if (Utilities.noString(value)) 4153 this.publisher = null; 4154 else { 4155 if (this.publisher == null) 4156 this.publisher = new StringType(); 4157 this.publisher.setValue(value); 4158 } 4159 return this; 4160 } 4161 4162 /** 4163 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 4164 */ 4165 public List<ContactDetail> getContact() { 4166 if (this.contact == null) 4167 this.contact = new ArrayList<ContactDetail>(); 4168 return this.contact; 4169 } 4170 4171 /** 4172 * @return Returns a reference to <code>this</code> for easy method chaining 4173 */ 4174 public ValueSet setContact(List<ContactDetail> theContact) { 4175 this.contact = theContact; 4176 return this; 4177 } 4178 4179 public boolean hasContact() { 4180 if (this.contact == null) 4181 return false; 4182 for (ContactDetail item : this.contact) 4183 if (!item.isEmpty()) 4184 return true; 4185 return false; 4186 } 4187 4188 public ContactDetail addContact() { //3 4189 ContactDetail t = new ContactDetail(); 4190 if (this.contact == null) 4191 this.contact = new ArrayList<ContactDetail>(); 4192 this.contact.add(t); 4193 return t; 4194 } 4195 4196 public ValueSet addContact(ContactDetail t) { //3 4197 if (t == null) 4198 return this; 4199 if (this.contact == null) 4200 this.contact = new ArrayList<ContactDetail>(); 4201 this.contact.add(t); 4202 return this; 4203 } 4204 4205 /** 4206 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 4207 */ 4208 public ContactDetail getContactFirstRep() { 4209 if (getContact().isEmpty()) { 4210 addContact(); 4211 } 4212 return getContact().get(0); 4213 } 4214 4215 /** 4216 * @return {@link #description} (A free text natural language description of the value set from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4217 */ 4218 public MarkdownType getDescriptionElement() { 4219 if (this.description == null) 4220 if (Configuration.errorOnAutoCreate()) 4221 throw new Error("Attempt to auto-create ValueSet.description"); 4222 else if (Configuration.doAutoCreate()) 4223 this.description = new MarkdownType(); // bb 4224 return this.description; 4225 } 4226 4227 public boolean hasDescriptionElement() { 4228 return this.description != null && !this.description.isEmpty(); 4229 } 4230 4231 public boolean hasDescription() { 4232 return this.description != null && !this.description.isEmpty(); 4233 } 4234 4235 /** 4236 * @param value {@link #description} (A free text natural language description of the value set from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4237 */ 4238 public ValueSet setDescriptionElement(MarkdownType value) { 4239 this.description = value; 4240 return this; 4241 } 4242 4243 /** 4244 * @return A free text natural language description of the value set from a consumer's perspective. 4245 */ 4246 public String getDescription() { 4247 return this.description == null ? null : this.description.getValue(); 4248 } 4249 4250 /** 4251 * @param value A free text natural language description of the value set from a consumer's perspective. 4252 */ 4253 public ValueSet setDescription(String value) { 4254 if (value == null) 4255 this.description = null; 4256 else { 4257 if (this.description == null) 4258 this.description = new MarkdownType(); 4259 this.description.setValue(value); 4260 } 4261 return this; 4262 } 4263 4264 /** 4265 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate value set instances.) 4266 */ 4267 public List<UsageContext> getUseContext() { 4268 if (this.useContext == null) 4269 this.useContext = new ArrayList<UsageContext>(); 4270 return this.useContext; 4271 } 4272 4273 /** 4274 * @return Returns a reference to <code>this</code> for easy method chaining 4275 */ 4276 public ValueSet setUseContext(List<UsageContext> theUseContext) { 4277 this.useContext = theUseContext; 4278 return this; 4279 } 4280 4281 public boolean hasUseContext() { 4282 if (this.useContext == null) 4283 return false; 4284 for (UsageContext item : this.useContext) 4285 if (!item.isEmpty()) 4286 return true; 4287 return false; 4288 } 4289 4290 public UsageContext addUseContext() { //3 4291 UsageContext t = new UsageContext(); 4292 if (this.useContext == null) 4293 this.useContext = new ArrayList<UsageContext>(); 4294 this.useContext.add(t); 4295 return t; 4296 } 4297 4298 public ValueSet addUseContext(UsageContext t) { //3 4299 if (t == null) 4300 return this; 4301 if (this.useContext == null) 4302 this.useContext = new ArrayList<UsageContext>(); 4303 this.useContext.add(t); 4304 return this; 4305 } 4306 4307 /** 4308 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 4309 */ 4310 public UsageContext getUseContextFirstRep() { 4311 if (getUseContext().isEmpty()) { 4312 addUseContext(); 4313 } 4314 return getUseContext().get(0); 4315 } 4316 4317 /** 4318 * @return {@link #jurisdiction} (A legal or geographic region in which the value set is intended to be used.) 4319 */ 4320 public List<CodeableConcept> getJurisdiction() { 4321 if (this.jurisdiction == null) 4322 this.jurisdiction = new ArrayList<CodeableConcept>(); 4323 return this.jurisdiction; 4324 } 4325 4326 /** 4327 * @return Returns a reference to <code>this</code> for easy method chaining 4328 */ 4329 public ValueSet setJurisdiction(List<CodeableConcept> theJurisdiction) { 4330 this.jurisdiction = theJurisdiction; 4331 return this; 4332 } 4333 4334 public boolean hasJurisdiction() { 4335 if (this.jurisdiction == null) 4336 return false; 4337 for (CodeableConcept item : this.jurisdiction) 4338 if (!item.isEmpty()) 4339 return true; 4340 return false; 4341 } 4342 4343 public CodeableConcept addJurisdiction() { //3 4344 CodeableConcept t = new CodeableConcept(); 4345 if (this.jurisdiction == null) 4346 this.jurisdiction = new ArrayList<CodeableConcept>(); 4347 this.jurisdiction.add(t); 4348 return t; 4349 } 4350 4351 public ValueSet addJurisdiction(CodeableConcept t) { //3 4352 if (t == null) 4353 return this; 4354 if (this.jurisdiction == null) 4355 this.jurisdiction = new ArrayList<CodeableConcept>(); 4356 this.jurisdiction.add(t); 4357 return this; 4358 } 4359 4360 /** 4361 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 4362 */ 4363 public CodeableConcept getJurisdictionFirstRep() { 4364 if (getJurisdiction().isEmpty()) { 4365 addJurisdiction(); 4366 } 4367 return getJurisdiction().get(0); 4368 } 4369 4370 /** 4371 * @return {@link #immutable} (If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.). This is the underlying object with id, value and extensions. The accessor "getImmutable" gives direct access to the value 4372 */ 4373 public BooleanType getImmutableElement() { 4374 if (this.immutable == null) 4375 if (Configuration.errorOnAutoCreate()) 4376 throw new Error("Attempt to auto-create ValueSet.immutable"); 4377 else if (Configuration.doAutoCreate()) 4378 this.immutable = new BooleanType(); // bb 4379 return this.immutable; 4380 } 4381 4382 public boolean hasImmutableElement() { 4383 return this.immutable != null && !this.immutable.isEmpty(); 4384 } 4385 4386 public boolean hasImmutable() { 4387 return this.immutable != null && !this.immutable.isEmpty(); 4388 } 4389 4390 /** 4391 * @param value {@link #immutable} (If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.). This is the underlying object with id, value and extensions. The accessor "getImmutable" gives direct access to the value 4392 */ 4393 public ValueSet setImmutableElement(BooleanType value) { 4394 this.immutable = value; 4395 return this; 4396 } 4397 4398 /** 4399 * @return If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change. 4400 */ 4401 public boolean getImmutable() { 4402 return this.immutable == null || this.immutable.isEmpty() ? false : this.immutable.getValue(); 4403 } 4404 4405 /** 4406 * @param value If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change. 4407 */ 4408 public ValueSet setImmutable(boolean value) { 4409 if (this.immutable == null) 4410 this.immutable = new BooleanType(); 4411 this.immutable.setValue(value); 4412 return this; 4413 } 4414 4415 /** 4416 * @return {@link #purpose} (Explaination of why this value set is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4417 */ 4418 public MarkdownType getPurposeElement() { 4419 if (this.purpose == null) 4420 if (Configuration.errorOnAutoCreate()) 4421 throw new Error("Attempt to auto-create ValueSet.purpose"); 4422 else if (Configuration.doAutoCreate()) 4423 this.purpose = new MarkdownType(); // bb 4424 return this.purpose; 4425 } 4426 4427 public boolean hasPurposeElement() { 4428 return this.purpose != null && !this.purpose.isEmpty(); 4429 } 4430 4431 public boolean hasPurpose() { 4432 return this.purpose != null && !this.purpose.isEmpty(); 4433 } 4434 4435 /** 4436 * @param value {@link #purpose} (Explaination of why this value set is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4437 */ 4438 public ValueSet setPurposeElement(MarkdownType value) { 4439 this.purpose = value; 4440 return this; 4441 } 4442 4443 /** 4444 * @return Explaination of why this value set is needed and why it has been designed as it has. 4445 */ 4446 public String getPurpose() { 4447 return this.purpose == null ? null : this.purpose.getValue(); 4448 } 4449 4450 /** 4451 * @param value Explaination of why this value set is needed and why it has been designed as it has. 4452 */ 4453 public ValueSet setPurpose(String value) { 4454 if (value == null) 4455 this.purpose = null; 4456 else { 4457 if (this.purpose == null) 4458 this.purpose = new MarkdownType(); 4459 this.purpose.setValue(value); 4460 } 4461 return this; 4462 } 4463 4464 /** 4465 * @return {@link #copyright} (A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4466 */ 4467 public MarkdownType getCopyrightElement() { 4468 if (this.copyright == null) 4469 if (Configuration.errorOnAutoCreate()) 4470 throw new Error("Attempt to auto-create ValueSet.copyright"); 4471 else if (Configuration.doAutoCreate()) 4472 this.copyright = new MarkdownType(); // bb 4473 return this.copyright; 4474 } 4475 4476 public boolean hasCopyrightElement() { 4477 return this.copyright != null && !this.copyright.isEmpty(); 4478 } 4479 4480 public boolean hasCopyright() { 4481 return this.copyright != null && !this.copyright.isEmpty(); 4482 } 4483 4484 /** 4485 * @param value {@link #copyright} (A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4486 */ 4487 public ValueSet setCopyrightElement(MarkdownType value) { 4488 this.copyright = value; 4489 return this; 4490 } 4491 4492 /** 4493 * @return A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set. 4494 */ 4495 public String getCopyright() { 4496 return this.copyright == null ? null : this.copyright.getValue(); 4497 } 4498 4499 /** 4500 * @param value A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set. 4501 */ 4502 public ValueSet setCopyright(String value) { 4503 if (value == null) 4504 this.copyright = null; 4505 else { 4506 if (this.copyright == null) 4507 this.copyright = new MarkdownType(); 4508 this.copyright.setValue(value); 4509 } 4510 return this; 4511 } 4512 4513 /** 4514 * @return {@link #extensible} (Whether this is intended to be used with an extensible binding or not.). This is the underlying object with id, value and extensions. The accessor "getExtensible" gives direct access to the value 4515 */ 4516 public BooleanType getExtensibleElement() { 4517 if (this.extensible == null) 4518 if (Configuration.errorOnAutoCreate()) 4519 throw new Error("Attempt to auto-create ValueSet.extensible"); 4520 else if (Configuration.doAutoCreate()) 4521 this.extensible = new BooleanType(); // bb 4522 return this.extensible; 4523 } 4524 4525 public boolean hasExtensibleElement() { 4526 return this.extensible != null && !this.extensible.isEmpty(); 4527 } 4528 4529 public boolean hasExtensible() { 4530 return this.extensible != null && !this.extensible.isEmpty(); 4531 } 4532 4533 /** 4534 * @param value {@link #extensible} (Whether this is intended to be used with an extensible binding or not.). This is the underlying object with id, value and extensions. The accessor "getExtensible" gives direct access to the value 4535 */ 4536 public ValueSet setExtensibleElement(BooleanType value) { 4537 this.extensible = value; 4538 return this; 4539 } 4540 4541 /** 4542 * @return Whether this is intended to be used with an extensible binding or not. 4543 */ 4544 public boolean getExtensible() { 4545 return this.extensible == null || this.extensible.isEmpty() ? false : this.extensible.getValue(); 4546 } 4547 4548 /** 4549 * @param value Whether this is intended to be used with an extensible binding or not. 4550 */ 4551 public ValueSet setExtensible(boolean value) { 4552 if (this.extensible == null) 4553 this.extensible = new BooleanType(); 4554 this.extensible.setValue(value); 4555 return this; 4556 } 4557 4558 /** 4559 * @return {@link #compose} (A set of criteria that define the content logical definition of the value set by including or excluding codes from outside this value set. This I also known as the "Content Logical Definition" (CLD).) 4560 */ 4561 public ValueSetComposeComponent getCompose() { 4562 if (this.compose == null) 4563 if (Configuration.errorOnAutoCreate()) 4564 throw new Error("Attempt to auto-create ValueSet.compose"); 4565 else if (Configuration.doAutoCreate()) 4566 this.compose = new ValueSetComposeComponent(); // cc 4567 return this.compose; 4568 } 4569 4570 public boolean hasCompose() { 4571 return this.compose != null && !this.compose.isEmpty(); 4572 } 4573 4574 /** 4575 * @param value {@link #compose} (A set of criteria that define the content logical definition of the value set by including or excluding codes from outside this value set. This I also known as the "Content Logical Definition" (CLD).) 4576 */ 4577 public ValueSet setCompose(ValueSetComposeComponent value) { 4578 this.compose = value; 4579 return this; 4580 } 4581 4582 /** 4583 * @return {@link #expansion} (A value set can also be "expanded", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.) 4584 */ 4585 public ValueSetExpansionComponent getExpansion() { 4586 if (this.expansion == null) 4587 if (Configuration.errorOnAutoCreate()) 4588 throw new Error("Attempt to auto-create ValueSet.expansion"); 4589 else if (Configuration.doAutoCreate()) 4590 this.expansion = new ValueSetExpansionComponent(); // cc 4591 return this.expansion; 4592 } 4593 4594 public boolean hasExpansion() { 4595 return this.expansion != null && !this.expansion.isEmpty(); 4596 } 4597 4598 /** 4599 * @param value {@link #expansion} (A value set can also be "expanded", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.) 4600 */ 4601 public ValueSet setExpansion(ValueSetExpansionComponent value) { 4602 this.expansion = value; 4603 return this; 4604 } 4605 4606 protected void listChildren(List<Property> children) { 4607 super.listChildren(children); 4608 children.add(new Property("url", "uri", "An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this value set is (or will be) published. The URL SHOULD include the major version of the value set. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 4609 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4610 children.add(new Property("version", "string", "The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 4611 children.add(new Property("name", "string", "A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 4612 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the value set.", 0, 1, title)); 4613 children.add(new Property("status", "code", "The status of this value set. Enables tracking the life-cycle of the content.", 0, 1, status)); 4614 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 4615 children.add(new Property("date", "dateTime", "The date (and optionally time) when the value set was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the value set changes. (e.g. the 'content logical definition').", 0, 1, date)); 4616 children.add(new Property("publisher", "string", "The name of the individual or organization that published the value set.", 0, 1, publisher)); 4617 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 4618 children.add(new Property("description", "markdown", "A free text natural language description of the value set from a consumer's perspective.", 0, 1, description)); 4619 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate value set instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 4620 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the value set is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 4621 children.add(new Property("immutable", "boolean", "If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.", 0, 1, immutable)); 4622 children.add(new Property("purpose", "markdown", "Explaination of why this value set is needed and why it has been designed as it has.", 0, 1, purpose)); 4623 children.add(new Property("copyright", "markdown", "A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.", 0, 1, copyright)); 4624 children.add(new Property("extensible", "boolean", "Whether this is intended to be used with an extensible binding or not.", 0, 1, extensible)); 4625 children.add(new Property("compose", "", "A set of criteria that define the content logical definition of the value set by including or excluding codes from outside this value set. This I also known as the \"Content Logical Definition\" (CLD).", 0, 1, compose)); 4626 children.add(new Property("expansion", "", "A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.", 0, 1, expansion)); 4627 } 4628 4629 @Override 4630 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4631 switch (_hash) { 4632 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this value set is (or will be) published. The URL SHOULD include the major version of the value set. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 4633 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 4634 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 4635 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 4636 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the value set.", 0, 1, title); 4637 case -892481550: /*status*/ return new Property("status", "code", "The status of this value set. Enables tracking the life-cycle of the content.", 0, 1, status); 4638 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 4639 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the value set was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the value set changes. (e.g. the 'content logical definition').", 0, 1, date); 4640 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the value set.", 0, 1, publisher); 4641 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 4642 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the value set from a consumer's perspective.", 0, 1, description); 4643 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate value set instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 4644 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the value set is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 4645 case 1596987778: /*immutable*/ return new Property("immutable", "boolean", "If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.", 0, 1, immutable); 4646 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this value set is needed and why it has been designed as it has.", 0, 1, purpose); 4647 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.", 0, 1, copyright); 4648 case -1809433861: /*extensible*/ return new Property("extensible", "boolean", "Whether this is intended to be used with an extensible binding or not.", 0, 1, extensible); 4649 case 950497682: /*compose*/ return new Property("compose", "", "A set of criteria that define the content logical definition of the value set by including or excluding codes from outside this value set. This I also known as the \"Content Logical Definition\" (CLD).", 0, 1, compose); 4650 case 17878207: /*expansion*/ return new Property("expansion", "", "A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.", 0, 1, expansion); 4651 default: return super.getNamedProperty(_hash, _name, _checkValid); 4652 } 4653 4654 } 4655 4656 @Override 4657 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4658 switch (hash) { 4659 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 4660 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4661 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 4662 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 4663 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 4664 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 4665 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 4666 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4667 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 4668 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4669 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 4670 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4671 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4672 case 1596987778: /*immutable*/ return this.immutable == null ? new Base[0] : new Base[] {this.immutable}; // BooleanType 4673 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 4674 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 4675 case -1809433861: /*extensible*/ return this.extensible == null ? new Base[0] : new Base[] {this.extensible}; // BooleanType 4676 case 950497682: /*compose*/ return this.compose == null ? new Base[0] : new Base[] {this.compose}; // ValueSetComposeComponent 4677 case 17878207: /*expansion*/ return this.expansion == null ? new Base[0] : new Base[] {this.expansion}; // ValueSetExpansionComponent 4678 default: return super.getProperty(hash, name, checkValid); 4679 } 4680 4681 } 4682 4683 @Override 4684 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4685 switch (hash) { 4686 case 116079: // url 4687 this.url = castToUri(value); // UriType 4688 return value; 4689 case -1618432855: // identifier 4690 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4691 return value; 4692 case 351608024: // version 4693 this.version = castToString(value); // StringType 4694 return value; 4695 case 3373707: // name 4696 this.name = castToString(value); // StringType 4697 return value; 4698 case 110371416: // title 4699 this.title = castToString(value); // StringType 4700 return value; 4701 case -892481550: // status 4702 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4703 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4704 return value; 4705 case -404562712: // experimental 4706 this.experimental = castToBoolean(value); // BooleanType 4707 return value; 4708 case 3076014: // date 4709 this.date = castToDateTime(value); // DateTimeType 4710 return value; 4711 case 1447404028: // publisher 4712 this.publisher = castToString(value); // StringType 4713 return value; 4714 case 951526432: // contact 4715 this.getContact().add(castToContactDetail(value)); // ContactDetail 4716 return value; 4717 case -1724546052: // description 4718 this.description = castToMarkdown(value); // MarkdownType 4719 return value; 4720 case -669707736: // useContext 4721 this.getUseContext().add(castToUsageContext(value)); // UsageContext 4722 return value; 4723 case -507075711: // jurisdiction 4724 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4725 return value; 4726 case 1596987778: // immutable 4727 this.immutable = castToBoolean(value); // BooleanType 4728 return value; 4729 case -220463842: // purpose 4730 this.purpose = castToMarkdown(value); // MarkdownType 4731 return value; 4732 case 1522889671: // copyright 4733 this.copyright = castToMarkdown(value); // MarkdownType 4734 return value; 4735 case -1809433861: // extensible 4736 this.extensible = castToBoolean(value); // BooleanType 4737 return value; 4738 case 950497682: // compose 4739 this.compose = (ValueSetComposeComponent) value; // ValueSetComposeComponent 4740 return value; 4741 case 17878207: // expansion 4742 this.expansion = (ValueSetExpansionComponent) value; // ValueSetExpansionComponent 4743 return value; 4744 default: return super.setProperty(hash, name, value); 4745 } 4746 4747 } 4748 4749 @Override 4750 public Base setProperty(String name, Base value) throws FHIRException { 4751 if (name.equals("url")) { 4752 this.url = castToUri(value); // UriType 4753 } else if (name.equals("identifier")) { 4754 this.getIdentifier().add(castToIdentifier(value)); 4755 } else if (name.equals("version")) { 4756 this.version = castToString(value); // StringType 4757 } else if (name.equals("name")) { 4758 this.name = castToString(value); // StringType 4759 } else if (name.equals("title")) { 4760 this.title = castToString(value); // StringType 4761 } else if (name.equals("status")) { 4762 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4763 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4764 } else if (name.equals("experimental")) { 4765 this.experimental = castToBoolean(value); // BooleanType 4766 } else if (name.equals("date")) { 4767 this.date = castToDateTime(value); // DateTimeType 4768 } else if (name.equals("publisher")) { 4769 this.publisher = castToString(value); // StringType 4770 } else if (name.equals("contact")) { 4771 this.getContact().add(castToContactDetail(value)); 4772 } else if (name.equals("description")) { 4773 this.description = castToMarkdown(value); // MarkdownType 4774 } else if (name.equals("useContext")) { 4775 this.getUseContext().add(castToUsageContext(value)); 4776 } else if (name.equals("jurisdiction")) { 4777 this.getJurisdiction().add(castToCodeableConcept(value)); 4778 } else if (name.equals("immutable")) { 4779 this.immutable = castToBoolean(value); // BooleanType 4780 } else if (name.equals("purpose")) { 4781 this.purpose = castToMarkdown(value); // MarkdownType 4782 } else if (name.equals("copyright")) { 4783 this.copyright = castToMarkdown(value); // MarkdownType 4784 } else if (name.equals("extensible")) { 4785 this.extensible = castToBoolean(value); // BooleanType 4786 } else if (name.equals("compose")) { 4787 this.compose = (ValueSetComposeComponent) value; // ValueSetComposeComponent 4788 } else if (name.equals("expansion")) { 4789 this.expansion = (ValueSetExpansionComponent) value; // ValueSetExpansionComponent 4790 } else 4791 return super.setProperty(name, value); 4792 return value; 4793 } 4794 4795 @Override 4796 public Base makeProperty(int hash, String name) throws FHIRException { 4797 switch (hash) { 4798 case 116079: return getUrlElement(); 4799 case -1618432855: return addIdentifier(); 4800 case 351608024: return getVersionElement(); 4801 case 3373707: return getNameElement(); 4802 case 110371416: return getTitleElement(); 4803 case -892481550: return getStatusElement(); 4804 case -404562712: return getExperimentalElement(); 4805 case 3076014: return getDateElement(); 4806 case 1447404028: return getPublisherElement(); 4807 case 951526432: return addContact(); 4808 case -1724546052: return getDescriptionElement(); 4809 case -669707736: return addUseContext(); 4810 case -507075711: return addJurisdiction(); 4811 case 1596987778: return getImmutableElement(); 4812 case -220463842: return getPurposeElement(); 4813 case 1522889671: return getCopyrightElement(); 4814 case -1809433861: return getExtensibleElement(); 4815 case 950497682: return getCompose(); 4816 case 17878207: return getExpansion(); 4817 default: return super.makeProperty(hash, name); 4818 } 4819 4820 } 4821 4822 @Override 4823 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4824 switch (hash) { 4825 case 116079: /*url*/ return new String[] {"uri"}; 4826 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4827 case 351608024: /*version*/ return new String[] {"string"}; 4828 case 3373707: /*name*/ return new String[] {"string"}; 4829 case 110371416: /*title*/ return new String[] {"string"}; 4830 case -892481550: /*status*/ return new String[] {"code"}; 4831 case -404562712: /*experimental*/ return new String[] {"boolean"}; 4832 case 3076014: /*date*/ return new String[] {"dateTime"}; 4833 case 1447404028: /*publisher*/ return new String[] {"string"}; 4834 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4835 case -1724546052: /*description*/ return new String[] {"markdown"}; 4836 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4837 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 4838 case 1596987778: /*immutable*/ return new String[] {"boolean"}; 4839 case -220463842: /*purpose*/ return new String[] {"markdown"}; 4840 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 4841 case -1809433861: /*extensible*/ return new String[] {"boolean"}; 4842 case 950497682: /*compose*/ return new String[] {}; 4843 case 17878207: /*expansion*/ return new String[] {}; 4844 default: return super.getTypesForProperty(hash, name); 4845 } 4846 4847 } 4848 4849 @Override 4850 public Base addChild(String name) throws FHIRException { 4851 if (name.equals("url")) { 4852 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.url"); 4853 } 4854 else if (name.equals("identifier")) { 4855 return addIdentifier(); 4856 } 4857 else if (name.equals("version")) { 4858 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 4859 } 4860 else if (name.equals("name")) { 4861 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.name"); 4862 } 4863 else if (name.equals("title")) { 4864 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.title"); 4865 } 4866 else if (name.equals("status")) { 4867 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.status"); 4868 } 4869 else if (name.equals("experimental")) { 4870 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.experimental"); 4871 } 4872 else if (name.equals("date")) { 4873 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.date"); 4874 } 4875 else if (name.equals("publisher")) { 4876 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.publisher"); 4877 } 4878 else if (name.equals("contact")) { 4879 return addContact(); 4880 } 4881 else if (name.equals("description")) { 4882 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.description"); 4883 } 4884 else if (name.equals("useContext")) { 4885 return addUseContext(); 4886 } 4887 else if (name.equals("jurisdiction")) { 4888 return addJurisdiction(); 4889 } 4890 else if (name.equals("immutable")) { 4891 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.immutable"); 4892 } 4893 else if (name.equals("purpose")) { 4894 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.purpose"); 4895 } 4896 else if (name.equals("copyright")) { 4897 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.copyright"); 4898 } 4899 else if (name.equals("extensible")) { 4900 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.extensible"); 4901 } 4902 else if (name.equals("compose")) { 4903 this.compose = new ValueSetComposeComponent(); 4904 return this.compose; 4905 } 4906 else if (name.equals("expansion")) { 4907 this.expansion = new ValueSetExpansionComponent(); 4908 return this.expansion; 4909 } 4910 else 4911 return super.addChild(name); 4912 } 4913 4914 public String fhirType() { 4915 return "ValueSet"; 4916 4917 } 4918 4919 public ValueSet copy() { 4920 ValueSet dst = new ValueSet(); 4921 copyValues(dst); 4922 dst.url = url == null ? null : url.copy(); 4923 if (identifier != null) { 4924 dst.identifier = new ArrayList<Identifier>(); 4925 for (Identifier i : identifier) 4926 dst.identifier.add(i.copy()); 4927 }; 4928 dst.version = version == null ? null : version.copy(); 4929 dst.name = name == null ? null : name.copy(); 4930 dst.title = title == null ? null : title.copy(); 4931 dst.status = status == null ? null : status.copy(); 4932 dst.experimental = experimental == null ? null : experimental.copy(); 4933 dst.date = date == null ? null : date.copy(); 4934 dst.publisher = publisher == null ? null : publisher.copy(); 4935 if (contact != null) { 4936 dst.contact = new ArrayList<ContactDetail>(); 4937 for (ContactDetail i : contact) 4938 dst.contact.add(i.copy()); 4939 }; 4940 dst.description = description == null ? null : description.copy(); 4941 if (useContext != null) { 4942 dst.useContext = new ArrayList<UsageContext>(); 4943 for (UsageContext i : useContext) 4944 dst.useContext.add(i.copy()); 4945 }; 4946 if (jurisdiction != null) { 4947 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4948 for (CodeableConcept i : jurisdiction) 4949 dst.jurisdiction.add(i.copy()); 4950 }; 4951 dst.immutable = immutable == null ? null : immutable.copy(); 4952 dst.purpose = purpose == null ? null : purpose.copy(); 4953 dst.copyright = copyright == null ? null : copyright.copy(); 4954 dst.extensible = extensible == null ? null : extensible.copy(); 4955 dst.compose = compose == null ? null : compose.copy(); 4956 dst.expansion = expansion == null ? null : expansion.copy(); 4957 return dst; 4958 } 4959 4960 protected ValueSet typedCopy() { 4961 return copy(); 4962 } 4963 4964 @Override 4965 public boolean equalsDeep(Base other_) { 4966 if (!super.equalsDeep(other_)) 4967 return false; 4968 if (!(other_ instanceof ValueSet)) 4969 return false; 4970 ValueSet o = (ValueSet) other_; 4971 return compareDeep(identifier, o.identifier, true) && compareDeep(immutable, o.immutable, true) 4972 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(extensible, o.extensible, true) 4973 && compareDeep(compose, o.compose, true) && compareDeep(expansion, o.expansion, true); 4974 } 4975 4976 @Override 4977 public boolean equalsShallow(Base other_) { 4978 if (!super.equalsShallow(other_)) 4979 return false; 4980 if (!(other_ instanceof ValueSet)) 4981 return false; 4982 ValueSet o = (ValueSet) other_; 4983 return compareValues(immutable, o.immutable, true) && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 4984 && compareValues(extensible, o.extensible, true); 4985 } 4986 4987 public boolean isEmpty() { 4988 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, immutable, purpose 4989 , copyright, extensible, compose, expansion); 4990 } 4991 4992 @Override 4993 public ResourceType getResourceType() { 4994 return ResourceType.ValueSet; 4995 } 4996 4997 /** 4998 * Search parameter: <b>date</b> 4999 * <p> 5000 * Description: <b>The value set publication date</b><br> 5001 * Type: <b>date</b><br> 5002 * Path: <b>ValueSet.date</b><br> 5003 * </p> 5004 */ 5005 @SearchParamDefinition(name="date", path="ValueSet.date", description="The value set publication date", type="date" ) 5006 public static final String SP_DATE = "date"; 5007 /** 5008 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5009 * <p> 5010 * Description: <b>The value set publication date</b><br> 5011 * Type: <b>date</b><br> 5012 * Path: <b>ValueSet.date</b><br> 5013 * </p> 5014 */ 5015 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 5016 5017 /** 5018 * Search parameter: <b>reference</b> 5019 * <p> 5020 * Description: <b>A code system included or excluded in the value set or an imported value set</b><br> 5021 * Type: <b>uri</b><br> 5022 * Path: <b>ValueSet.compose.include.system</b><br> 5023 * </p> 5024 */ 5025 @SearchParamDefinition(name="reference", path="ValueSet.compose.include.system", description="A code system included or excluded in the value set or an imported value set", type="uri" ) 5026 public static final String SP_REFERENCE = "reference"; 5027 /** 5028 * <b>Fluent Client</b> search parameter constant for <b>reference</b> 5029 * <p> 5030 * Description: <b>A code system included or excluded in the value set or an imported value set</b><br> 5031 * Type: <b>uri</b><br> 5032 * Path: <b>ValueSet.compose.include.system</b><br> 5033 * </p> 5034 */ 5035 public static final ca.uhn.fhir.rest.gclient.UriClientParam REFERENCE = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_REFERENCE); 5036 5037 /** 5038 * Search parameter: <b>identifier</b> 5039 * <p> 5040 * Description: <b>External identifier for the value set</b><br> 5041 * Type: <b>token</b><br> 5042 * Path: <b>ValueSet.identifier</b><br> 5043 * </p> 5044 */ 5045 @SearchParamDefinition(name="identifier", path="ValueSet.identifier", description="External identifier for the value set", type="token" ) 5046 public static final String SP_IDENTIFIER = "identifier"; 5047 /** 5048 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5049 * <p> 5050 * Description: <b>External identifier for the value set</b><br> 5051 * Type: <b>token</b><br> 5052 * Path: <b>ValueSet.identifier</b><br> 5053 * </p> 5054 */ 5055 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 5056 5057 /** 5058 * Search parameter: <b>jurisdiction</b> 5059 * <p> 5060 * Description: <b>Intended jurisdiction for the value set</b><br> 5061 * Type: <b>token</b><br> 5062 * Path: <b>ValueSet.jurisdiction</b><br> 5063 * </p> 5064 */ 5065 @SearchParamDefinition(name="jurisdiction", path="ValueSet.jurisdiction", description="Intended jurisdiction for the value set", type="token" ) 5066 public static final String SP_JURISDICTION = "jurisdiction"; 5067 /** 5068 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5069 * <p> 5070 * Description: <b>Intended jurisdiction for the value set</b><br> 5071 * Type: <b>token</b><br> 5072 * Path: <b>ValueSet.jurisdiction</b><br> 5073 * </p> 5074 */ 5075 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 5076 5077 /** 5078 * Search parameter: <b>name</b> 5079 * <p> 5080 * Description: <b>Computationally friendly name of the value set</b><br> 5081 * Type: <b>string</b><br> 5082 * Path: <b>ValueSet.name</b><br> 5083 * </p> 5084 */ 5085 @SearchParamDefinition(name="name", path="ValueSet.name", description="Computationally friendly name of the value set", type="string" ) 5086 public static final String SP_NAME = "name"; 5087 /** 5088 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5089 * <p> 5090 * Description: <b>Computationally friendly name of the value set</b><br> 5091 * Type: <b>string</b><br> 5092 * Path: <b>ValueSet.name</b><br> 5093 * </p> 5094 */ 5095 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 5096 5097 /** 5098 * Search parameter: <b>description</b> 5099 * <p> 5100 * Description: <b>The description of the value set</b><br> 5101 * Type: <b>string</b><br> 5102 * Path: <b>ValueSet.description</b><br> 5103 * </p> 5104 */ 5105 @SearchParamDefinition(name="description", path="ValueSet.description", description="The description of the value set", type="string" ) 5106 public static final String SP_DESCRIPTION = "description"; 5107 /** 5108 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5109 * <p> 5110 * Description: <b>The description of the value set</b><br> 5111 * Type: <b>string</b><br> 5112 * Path: <b>ValueSet.description</b><br> 5113 * </p> 5114 */ 5115 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 5116 5117 /** 5118 * Search parameter: <b>publisher</b> 5119 * <p> 5120 * Description: <b>Name of the publisher of the value set</b><br> 5121 * Type: <b>string</b><br> 5122 * Path: <b>ValueSet.publisher</b><br> 5123 * </p> 5124 */ 5125 @SearchParamDefinition(name="publisher", path="ValueSet.publisher", description="Name of the publisher of the value set", type="string" ) 5126 public static final String SP_PUBLISHER = "publisher"; 5127 /** 5128 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5129 * <p> 5130 * Description: <b>Name of the publisher of the value set</b><br> 5131 * Type: <b>string</b><br> 5132 * Path: <b>ValueSet.publisher</b><br> 5133 * </p> 5134 */ 5135 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 5136 5137 /** 5138 * Search parameter: <b>title</b> 5139 * <p> 5140 * Description: <b>The human-friendly name of the value set</b><br> 5141 * Type: <b>string</b><br> 5142 * Path: <b>ValueSet.title</b><br> 5143 * </p> 5144 */ 5145 @SearchParamDefinition(name="title", path="ValueSet.title", description="The human-friendly name of the value set", type="string" ) 5146 public static final String SP_TITLE = "title"; 5147 /** 5148 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5149 * <p> 5150 * Description: <b>The human-friendly name of the value set</b><br> 5151 * Type: <b>string</b><br> 5152 * Path: <b>ValueSet.title</b><br> 5153 * </p> 5154 */ 5155 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 5156 5157 /** 5158 * Search parameter: <b>version</b> 5159 * <p> 5160 * Description: <b>The business version of the value set</b><br> 5161 * Type: <b>token</b><br> 5162 * Path: <b>ValueSet.version</b><br> 5163 * </p> 5164 */ 5165 @SearchParamDefinition(name="version", path="ValueSet.version", description="The business version of the value set", type="token" ) 5166 public static final String SP_VERSION = "version"; 5167 /** 5168 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5169 * <p> 5170 * Description: <b>The business version of the value set</b><br> 5171 * Type: <b>token</b><br> 5172 * Path: <b>ValueSet.version</b><br> 5173 * </p> 5174 */ 5175 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 5176 5177 /** 5178 * Search parameter: <b>url</b> 5179 * <p> 5180 * Description: <b>The uri that identifies the value set</b><br> 5181 * Type: <b>uri</b><br> 5182 * Path: <b>ValueSet.url</b><br> 5183 * </p> 5184 */ 5185 @SearchParamDefinition(name="url", path="ValueSet.url", description="The uri that identifies the value set", type="uri" ) 5186 public static final String SP_URL = "url"; 5187 /** 5188 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5189 * <p> 5190 * Description: <b>The uri that identifies the value set</b><br> 5191 * Type: <b>uri</b><br> 5192 * Path: <b>ValueSet.url</b><br> 5193 * </p> 5194 */ 5195 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5196 5197 /** 5198 * Search parameter: <b>status</b> 5199 * <p> 5200 * Description: <b>The current status of the value set</b><br> 5201 * Type: <b>token</b><br> 5202 * Path: <b>ValueSet.status</b><br> 5203 * </p> 5204 */ 5205 @SearchParamDefinition(name="status", path="ValueSet.status", description="The current status of the value set", type="token" ) 5206 public static final String SP_STATUS = "status"; 5207 /** 5208 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5209 * <p> 5210 * Description: <b>The current status of the value set</b><br> 5211 * Type: <b>token</b><br> 5212 * Path: <b>ValueSet.status</b><br> 5213 * </p> 5214 */ 5215 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 5216 5217 /** 5218 * Search parameter: <b>expansion</b> 5219 * <p> 5220 * Description: <b>Uniquely identifies this expansion</b><br> 5221 * Type: <b>uri</b><br> 5222 * Path: <b>ValueSet.expansion.identifier</b><br> 5223 * </p> 5224 */ 5225 @SearchParamDefinition(name="expansion", path="ValueSet.expansion.identifier", description="Uniquely identifies this expansion", type="uri" ) 5226 public static final String SP_EXPANSION = "expansion"; 5227 /** 5228 * <b>Fluent Client</b> search parameter constant for <b>expansion</b> 5229 * <p> 5230 * Description: <b>Uniquely identifies this expansion</b><br> 5231 * Type: <b>uri</b><br> 5232 * Path: <b>ValueSet.expansion.identifier</b><br> 5233 * </p> 5234 */ 5235 public static final ca.uhn.fhir.rest.gclient.UriClientParam EXPANSION = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_EXPANSION); 5236 5237 5238}