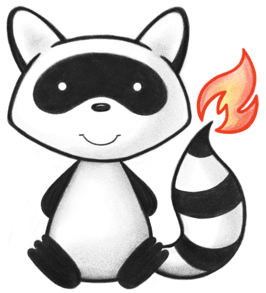
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * An authorization for the supply of glasses and/or contact lenses to a patient. 051 */ 052@ResourceDef(name="VisionPrescription", profile="http://hl7.org/fhir/Profile/VisionPrescription") 053public class VisionPrescription extends DomainResource { 054 055 public enum VisionStatus { 056 /** 057 * The instance is currently in-force. 058 */ 059 ACTIVE, 060 /** 061 * The instance is withdrawn, rescinded or reversed. 062 */ 063 CANCELLED, 064 /** 065 * A new instance the contents of which is not complete. 066 */ 067 DRAFT, 068 /** 069 * The instance was entered in error. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static VisionStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("active".equals(codeString)) 080 return ACTIVE; 081 if ("cancelled".equals(codeString)) 082 return CANCELLED; 083 if ("draft".equals(codeString)) 084 return DRAFT; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown VisionStatus code '"+codeString+"'"); 091 } 092 public String toCode() { 093 switch (this) { 094 case ACTIVE: return "active"; 095 case CANCELLED: return "cancelled"; 096 case DRAFT: return "draft"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 105 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 106 case DRAFT: return "http://hl7.org/fhir/fm-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDefinition() { 113 switch (this) { 114 case ACTIVE: return "The instance is currently in-force."; 115 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 116 case DRAFT: return "A new instance the contents of which is not complete."; 117 case ENTEREDINERROR: return "The instance was entered in error."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case ACTIVE: return "Active"; 125 case CANCELLED: return "Cancelled"; 126 case DRAFT: return "Draft"; 127 case ENTEREDINERROR: return "Entered in Error"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 } 133 134 public static class VisionStatusEnumFactory implements EnumFactory<VisionStatus> { 135 public VisionStatus fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("active".equals(codeString)) 140 return VisionStatus.ACTIVE; 141 if ("cancelled".equals(codeString)) 142 return VisionStatus.CANCELLED; 143 if ("draft".equals(codeString)) 144 return VisionStatus.DRAFT; 145 if ("entered-in-error".equals(codeString)) 146 return VisionStatus.ENTEREDINERROR; 147 throw new IllegalArgumentException("Unknown VisionStatus code '"+codeString+"'"); 148 } 149 public Enumeration<VisionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<VisionStatus>(this); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("active".equals(codeString)) 158 return new Enumeration<VisionStatus>(this, VisionStatus.ACTIVE); 159 if ("cancelled".equals(codeString)) 160 return new Enumeration<VisionStatus>(this, VisionStatus.CANCELLED); 161 if ("draft".equals(codeString)) 162 return new Enumeration<VisionStatus>(this, VisionStatus.DRAFT); 163 if ("entered-in-error".equals(codeString)) 164 return new Enumeration<VisionStatus>(this, VisionStatus.ENTEREDINERROR); 165 throw new FHIRException("Unknown VisionStatus code '"+codeString+"'"); 166 } 167 public String toCode(VisionStatus code) { 168 if (code == VisionStatus.NULL) 169 return null; 170 if (code == VisionStatus.ACTIVE) 171 return "active"; 172 if (code == VisionStatus.CANCELLED) 173 return "cancelled"; 174 if (code == VisionStatus.DRAFT) 175 return "draft"; 176 if (code == VisionStatus.ENTEREDINERROR) 177 return "entered-in-error"; 178 return "?"; 179 } 180 public String toSystem(VisionStatus code) { 181 return code.getSystem(); 182 } 183 } 184 185 public enum VisionEyes { 186 /** 187 * Right Eye 188 */ 189 RIGHT, 190 /** 191 * Left Eye 192 */ 193 LEFT, 194 /** 195 * added to help the parsers with the generic types 196 */ 197 NULL; 198 public static VisionEyes fromCode(String codeString) throws FHIRException { 199 if (codeString == null || "".equals(codeString)) 200 return null; 201 if ("right".equals(codeString)) 202 return RIGHT; 203 if ("left".equals(codeString)) 204 return LEFT; 205 if (Configuration.isAcceptInvalidEnums()) 206 return null; 207 else 208 throw new FHIRException("Unknown VisionEyes code '"+codeString+"'"); 209 } 210 public String toCode() { 211 switch (this) { 212 case RIGHT: return "right"; 213 case LEFT: return "left"; 214 case NULL: return null; 215 default: return "?"; 216 } 217 } 218 public String getSystem() { 219 switch (this) { 220 case RIGHT: return "http://hl7.org/fhir/vision-eye-codes"; 221 case LEFT: return "http://hl7.org/fhir/vision-eye-codes"; 222 case NULL: return null; 223 default: return "?"; 224 } 225 } 226 public String getDefinition() { 227 switch (this) { 228 case RIGHT: return "Right Eye"; 229 case LEFT: return "Left Eye"; 230 case NULL: return null; 231 default: return "?"; 232 } 233 } 234 public String getDisplay() { 235 switch (this) { 236 case RIGHT: return "Right Eye"; 237 case LEFT: return "Left Eye"; 238 case NULL: return null; 239 default: return "?"; 240 } 241 } 242 } 243 244 public static class VisionEyesEnumFactory implements EnumFactory<VisionEyes> { 245 public VisionEyes fromCode(String codeString) throws IllegalArgumentException { 246 if (codeString == null || "".equals(codeString)) 247 if (codeString == null || "".equals(codeString)) 248 return null; 249 if ("right".equals(codeString)) 250 return VisionEyes.RIGHT; 251 if ("left".equals(codeString)) 252 return VisionEyes.LEFT; 253 throw new IllegalArgumentException("Unknown VisionEyes code '"+codeString+"'"); 254 } 255 public Enumeration<VisionEyes> fromType(PrimitiveType<?> code) throws FHIRException { 256 if (code == null) 257 return null; 258 if (code.isEmpty()) 259 return new Enumeration<VisionEyes>(this); 260 String codeString = code.asStringValue(); 261 if (codeString == null || "".equals(codeString)) 262 return null; 263 if ("right".equals(codeString)) 264 return new Enumeration<VisionEyes>(this, VisionEyes.RIGHT); 265 if ("left".equals(codeString)) 266 return new Enumeration<VisionEyes>(this, VisionEyes.LEFT); 267 throw new FHIRException("Unknown VisionEyes code '"+codeString+"'"); 268 } 269 public String toCode(VisionEyes code) { 270 if (code == VisionEyes.NULL) 271 return null; 272 if (code == VisionEyes.RIGHT) 273 return "right"; 274 if (code == VisionEyes.LEFT) 275 return "left"; 276 return "?"; 277 } 278 public String toSystem(VisionEyes code) { 279 return code.getSystem(); 280 } 281 } 282 283 public enum VisionBase { 284 /** 285 * top 286 */ 287 UP, 288 /** 289 * bottom 290 */ 291 DOWN, 292 /** 293 * inner edge 294 */ 295 IN, 296 /** 297 * outer edge 298 */ 299 OUT, 300 /** 301 * added to help the parsers with the generic types 302 */ 303 NULL; 304 public static VisionBase fromCode(String codeString) throws FHIRException { 305 if (codeString == null || "".equals(codeString)) 306 return null; 307 if ("up".equals(codeString)) 308 return UP; 309 if ("down".equals(codeString)) 310 return DOWN; 311 if ("in".equals(codeString)) 312 return IN; 313 if ("out".equals(codeString)) 314 return OUT; 315 if (Configuration.isAcceptInvalidEnums()) 316 return null; 317 else 318 throw new FHIRException("Unknown VisionBase code '"+codeString+"'"); 319 } 320 public String toCode() { 321 switch (this) { 322 case UP: return "up"; 323 case DOWN: return "down"; 324 case IN: return "in"; 325 case OUT: return "out"; 326 case NULL: return null; 327 default: return "?"; 328 } 329 } 330 public String getSystem() { 331 switch (this) { 332 case UP: return "http://hl7.org/fhir/vision-base-codes"; 333 case DOWN: return "http://hl7.org/fhir/vision-base-codes"; 334 case IN: return "http://hl7.org/fhir/vision-base-codes"; 335 case OUT: return "http://hl7.org/fhir/vision-base-codes"; 336 case NULL: return null; 337 default: return "?"; 338 } 339 } 340 public String getDefinition() { 341 switch (this) { 342 case UP: return "top"; 343 case DOWN: return "bottom"; 344 case IN: return "inner edge"; 345 case OUT: return "outer edge"; 346 case NULL: return null; 347 default: return "?"; 348 } 349 } 350 public String getDisplay() { 351 switch (this) { 352 case UP: return "Up"; 353 case DOWN: return "Down"; 354 case IN: return "In"; 355 case OUT: return "Out"; 356 case NULL: return null; 357 default: return "?"; 358 } 359 } 360 } 361 362 public static class VisionBaseEnumFactory implements EnumFactory<VisionBase> { 363 public VisionBase fromCode(String codeString) throws IllegalArgumentException { 364 if (codeString == null || "".equals(codeString)) 365 if (codeString == null || "".equals(codeString)) 366 return null; 367 if ("up".equals(codeString)) 368 return VisionBase.UP; 369 if ("down".equals(codeString)) 370 return VisionBase.DOWN; 371 if ("in".equals(codeString)) 372 return VisionBase.IN; 373 if ("out".equals(codeString)) 374 return VisionBase.OUT; 375 throw new IllegalArgumentException("Unknown VisionBase code '"+codeString+"'"); 376 } 377 public Enumeration<VisionBase> fromType(PrimitiveType<?> code) throws FHIRException { 378 if (code == null) 379 return null; 380 if (code.isEmpty()) 381 return new Enumeration<VisionBase>(this); 382 String codeString = code.asStringValue(); 383 if (codeString == null || "".equals(codeString)) 384 return null; 385 if ("up".equals(codeString)) 386 return new Enumeration<VisionBase>(this, VisionBase.UP); 387 if ("down".equals(codeString)) 388 return new Enumeration<VisionBase>(this, VisionBase.DOWN); 389 if ("in".equals(codeString)) 390 return new Enumeration<VisionBase>(this, VisionBase.IN); 391 if ("out".equals(codeString)) 392 return new Enumeration<VisionBase>(this, VisionBase.OUT); 393 throw new FHIRException("Unknown VisionBase code '"+codeString+"'"); 394 } 395 public String toCode(VisionBase code) { 396 if (code == VisionBase.NULL) 397 return null; 398 if (code == VisionBase.UP) 399 return "up"; 400 if (code == VisionBase.DOWN) 401 return "down"; 402 if (code == VisionBase.IN) 403 return "in"; 404 if (code == VisionBase.OUT) 405 return "out"; 406 return "?"; 407 } 408 public String toSystem(VisionBase code) { 409 return code.getSystem(); 410 } 411 } 412 413 @Block() 414 public static class VisionPrescriptionDispenseComponent extends BackboneElement implements IBaseBackboneElement { 415 /** 416 * Identifies the type of vision correction product which is required for the patient. 417 */ 418 @Child(name = "product", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 419 @Description(shortDefinition="Product to be supplied", formalDefinition="Identifies the type of vision correction product which is required for the patient." ) 420 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vision-product") 421 protected CodeableConcept product; 422 423 /** 424 * The eye for which the lens applies. 425 */ 426 @Child(name = "eye", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 427 @Description(shortDefinition="right | left", formalDefinition="The eye for which the lens applies." ) 428 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vision-eye-codes") 429 protected Enumeration<VisionEyes> eye; 430 431 /** 432 * Lens power measured in diopters (0.25 units). 433 */ 434 @Child(name = "sphere", type = {DecimalType.class}, order=3, min=0, max=1, modifier=false, summary=false) 435 @Description(shortDefinition="Lens sphere", formalDefinition="Lens power measured in diopters (0.25 units)." ) 436 protected DecimalType sphere; 437 438 /** 439 * Power adjustment for astigmatism measured in diopters (0.25 units). 440 */ 441 @Child(name = "cylinder", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=false) 442 @Description(shortDefinition="Lens cylinder", formalDefinition="Power adjustment for astigmatism measured in diopters (0.25 units)." ) 443 protected DecimalType cylinder; 444 445 /** 446 * Adjustment for astigmatism measured in integer degrees. 447 */ 448 @Child(name = "axis", type = {IntegerType.class}, order=5, min=0, max=1, modifier=false, summary=false) 449 @Description(shortDefinition="Lens axis", formalDefinition="Adjustment for astigmatism measured in integer degrees." ) 450 protected IntegerType axis; 451 452 /** 453 * Amount of prism to compensate for eye alignment in fractional units. 454 */ 455 @Child(name = "prism", type = {DecimalType.class}, order=6, min=0, max=1, modifier=false, summary=false) 456 @Description(shortDefinition="Lens prism", formalDefinition="Amount of prism to compensate for eye alignment in fractional units." ) 457 protected DecimalType prism; 458 459 /** 460 * The relative base, or reference lens edge, for the prism. 461 */ 462 @Child(name = "base", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=false) 463 @Description(shortDefinition="up | down | in | out", formalDefinition="The relative base, or reference lens edge, for the prism." ) 464 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vision-base-codes") 465 protected Enumeration<VisionBase> base; 466 467 /** 468 * Power adjustment for multifocal lenses measured in diopters (0.25 units). 469 */ 470 @Child(name = "add", type = {DecimalType.class}, order=8, min=0, max=1, modifier=false, summary=false) 471 @Description(shortDefinition="Lens add", formalDefinition="Power adjustment for multifocal lenses measured in diopters (0.25 units)." ) 472 protected DecimalType add; 473 474 /** 475 * Contact lens power measured in diopters (0.25 units). 476 */ 477 @Child(name = "power", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 478 @Description(shortDefinition="Contact lens power", formalDefinition="Contact lens power measured in diopters (0.25 units)." ) 479 protected DecimalType power; 480 481 /** 482 * Back curvature measured in millimeters. 483 */ 484 @Child(name = "backCurve", type = {DecimalType.class}, order=10, min=0, max=1, modifier=false, summary=false) 485 @Description(shortDefinition="Contact lens back curvature", formalDefinition="Back curvature measured in millimeters." ) 486 protected DecimalType backCurve; 487 488 /** 489 * Contact lens diameter measured in millimeters. 490 */ 491 @Child(name = "diameter", type = {DecimalType.class}, order=11, min=0, max=1, modifier=false, summary=false) 492 @Description(shortDefinition="Contact lens diameter", formalDefinition="Contact lens diameter measured in millimeters." ) 493 protected DecimalType diameter; 494 495 /** 496 * The recommended maximum wear period for the lens. 497 */ 498 @Child(name = "duration", type = {SimpleQuantity.class}, order=12, min=0, max=1, modifier=false, summary=false) 499 @Description(shortDefinition="Lens wear duration", formalDefinition="The recommended maximum wear period for the lens." ) 500 protected SimpleQuantity duration; 501 502 /** 503 * Special color or pattern. 504 */ 505 @Child(name = "color", type = {StringType.class}, order=13, min=0, max=1, modifier=false, summary=false) 506 @Description(shortDefinition="Color required", formalDefinition="Special color or pattern." ) 507 protected StringType color; 508 509 /** 510 * Brand recommendations or restrictions. 511 */ 512 @Child(name = "brand", type = {StringType.class}, order=14, min=0, max=1, modifier=false, summary=false) 513 @Description(shortDefinition="Brand required", formalDefinition="Brand recommendations or restrictions." ) 514 protected StringType brand; 515 516 /** 517 * Notes for special requirements such as coatings and lens materials. 518 */ 519 @Child(name = "note", type = {Annotation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 520 @Description(shortDefinition="Notes for coatings", formalDefinition="Notes for special requirements such as coatings and lens materials." ) 521 protected List<Annotation> note; 522 523 private static final long serialVersionUID = 400998008L; 524 525 /** 526 * Constructor 527 */ 528 public VisionPrescriptionDispenseComponent() { 529 super(); 530 } 531 532 /** 533 * @return {@link #product} (Identifies the type of vision correction product which is required for the patient.) 534 */ 535 public CodeableConcept getProduct() { 536 if (this.product == null) 537 if (Configuration.errorOnAutoCreate()) 538 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.product"); 539 else if (Configuration.doAutoCreate()) 540 this.product = new CodeableConcept(); // cc 541 return this.product; 542 } 543 544 public boolean hasProduct() { 545 return this.product != null && !this.product.isEmpty(); 546 } 547 548 /** 549 * @param value {@link #product} (Identifies the type of vision correction product which is required for the patient.) 550 */ 551 public VisionPrescriptionDispenseComponent setProduct(CodeableConcept value) { 552 this.product = value; 553 return this; 554 } 555 556 /** 557 * @return {@link #eye} (The eye for which the lens applies.). This is the underlying object with id, value and extensions. The accessor "getEye" gives direct access to the value 558 */ 559 public Enumeration<VisionEyes> getEyeElement() { 560 if (this.eye == null) 561 if (Configuration.errorOnAutoCreate()) 562 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.eye"); 563 else if (Configuration.doAutoCreate()) 564 this.eye = new Enumeration<VisionEyes>(new VisionEyesEnumFactory()); // bb 565 return this.eye; 566 } 567 568 public boolean hasEyeElement() { 569 return this.eye != null && !this.eye.isEmpty(); 570 } 571 572 public boolean hasEye() { 573 return this.eye != null && !this.eye.isEmpty(); 574 } 575 576 /** 577 * @param value {@link #eye} (The eye for which the lens applies.). This is the underlying object with id, value and extensions. The accessor "getEye" gives direct access to the value 578 */ 579 public VisionPrescriptionDispenseComponent setEyeElement(Enumeration<VisionEyes> value) { 580 this.eye = value; 581 return this; 582 } 583 584 /** 585 * @return The eye for which the lens applies. 586 */ 587 public VisionEyes getEye() { 588 return this.eye == null ? null : this.eye.getValue(); 589 } 590 591 /** 592 * @param value The eye for which the lens applies. 593 */ 594 public VisionPrescriptionDispenseComponent setEye(VisionEyes value) { 595 if (value == null) 596 this.eye = null; 597 else { 598 if (this.eye == null) 599 this.eye = new Enumeration<VisionEyes>(new VisionEyesEnumFactory()); 600 this.eye.setValue(value); 601 } 602 return this; 603 } 604 605 /** 606 * @return {@link #sphere} (Lens power measured in diopters (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getSphere" gives direct access to the value 607 */ 608 public DecimalType getSphereElement() { 609 if (this.sphere == null) 610 if (Configuration.errorOnAutoCreate()) 611 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.sphere"); 612 else if (Configuration.doAutoCreate()) 613 this.sphere = new DecimalType(); // bb 614 return this.sphere; 615 } 616 617 public boolean hasSphereElement() { 618 return this.sphere != null && !this.sphere.isEmpty(); 619 } 620 621 public boolean hasSphere() { 622 return this.sphere != null && !this.sphere.isEmpty(); 623 } 624 625 /** 626 * @param value {@link #sphere} (Lens power measured in diopters (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getSphere" gives direct access to the value 627 */ 628 public VisionPrescriptionDispenseComponent setSphereElement(DecimalType value) { 629 this.sphere = value; 630 return this; 631 } 632 633 /** 634 * @return Lens power measured in diopters (0.25 units). 635 */ 636 public BigDecimal getSphere() { 637 return this.sphere == null ? null : this.sphere.getValue(); 638 } 639 640 /** 641 * @param value Lens power measured in diopters (0.25 units). 642 */ 643 public VisionPrescriptionDispenseComponent setSphere(BigDecimal value) { 644 if (value == null) 645 this.sphere = null; 646 else { 647 if (this.sphere == null) 648 this.sphere = new DecimalType(); 649 this.sphere.setValue(value); 650 } 651 return this; 652 } 653 654 /** 655 * @param value Lens power measured in diopters (0.25 units). 656 */ 657 public VisionPrescriptionDispenseComponent setSphere(long value) { 658 this.sphere = new DecimalType(); 659 this.sphere.setValue(value); 660 return this; 661 } 662 663 /** 664 * @param value Lens power measured in diopters (0.25 units). 665 */ 666 public VisionPrescriptionDispenseComponent setSphere(double value) { 667 this.sphere = new DecimalType(); 668 this.sphere.setValue(value); 669 return this; 670 } 671 672 /** 673 * @return {@link #cylinder} (Power adjustment for astigmatism measured in diopters (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getCylinder" gives direct access to the value 674 */ 675 public DecimalType getCylinderElement() { 676 if (this.cylinder == null) 677 if (Configuration.errorOnAutoCreate()) 678 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.cylinder"); 679 else if (Configuration.doAutoCreate()) 680 this.cylinder = new DecimalType(); // bb 681 return this.cylinder; 682 } 683 684 public boolean hasCylinderElement() { 685 return this.cylinder != null && !this.cylinder.isEmpty(); 686 } 687 688 public boolean hasCylinder() { 689 return this.cylinder != null && !this.cylinder.isEmpty(); 690 } 691 692 /** 693 * @param value {@link #cylinder} (Power adjustment for astigmatism measured in diopters (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getCylinder" gives direct access to the value 694 */ 695 public VisionPrescriptionDispenseComponent setCylinderElement(DecimalType value) { 696 this.cylinder = value; 697 return this; 698 } 699 700 /** 701 * @return Power adjustment for astigmatism measured in diopters (0.25 units). 702 */ 703 public BigDecimal getCylinder() { 704 return this.cylinder == null ? null : this.cylinder.getValue(); 705 } 706 707 /** 708 * @param value Power adjustment for astigmatism measured in diopters (0.25 units). 709 */ 710 public VisionPrescriptionDispenseComponent setCylinder(BigDecimal value) { 711 if (value == null) 712 this.cylinder = null; 713 else { 714 if (this.cylinder == null) 715 this.cylinder = new DecimalType(); 716 this.cylinder.setValue(value); 717 } 718 return this; 719 } 720 721 /** 722 * @param value Power adjustment for astigmatism measured in diopters (0.25 units). 723 */ 724 public VisionPrescriptionDispenseComponent setCylinder(long value) { 725 this.cylinder = new DecimalType(); 726 this.cylinder.setValue(value); 727 return this; 728 } 729 730 /** 731 * @param value Power adjustment for astigmatism measured in diopters (0.25 units). 732 */ 733 public VisionPrescriptionDispenseComponent setCylinder(double value) { 734 this.cylinder = new DecimalType(); 735 this.cylinder.setValue(value); 736 return this; 737 } 738 739 /** 740 * @return {@link #axis} (Adjustment for astigmatism measured in integer degrees.). This is the underlying object with id, value and extensions. The accessor "getAxis" gives direct access to the value 741 */ 742 public IntegerType getAxisElement() { 743 if (this.axis == null) 744 if (Configuration.errorOnAutoCreate()) 745 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.axis"); 746 else if (Configuration.doAutoCreate()) 747 this.axis = new IntegerType(); // bb 748 return this.axis; 749 } 750 751 public boolean hasAxisElement() { 752 return this.axis != null && !this.axis.isEmpty(); 753 } 754 755 public boolean hasAxis() { 756 return this.axis != null && !this.axis.isEmpty(); 757 } 758 759 /** 760 * @param value {@link #axis} (Adjustment for astigmatism measured in integer degrees.). This is the underlying object with id, value and extensions. The accessor "getAxis" gives direct access to the value 761 */ 762 public VisionPrescriptionDispenseComponent setAxisElement(IntegerType value) { 763 this.axis = value; 764 return this; 765 } 766 767 /** 768 * @return Adjustment for astigmatism measured in integer degrees. 769 */ 770 public int getAxis() { 771 return this.axis == null || this.axis.isEmpty() ? 0 : this.axis.getValue(); 772 } 773 774 /** 775 * @param value Adjustment for astigmatism measured in integer degrees. 776 */ 777 public VisionPrescriptionDispenseComponent setAxis(int value) { 778 if (this.axis == null) 779 this.axis = new IntegerType(); 780 this.axis.setValue(value); 781 return this; 782 } 783 784 /** 785 * @return {@link #prism} (Amount of prism to compensate for eye alignment in fractional units.). This is the underlying object with id, value and extensions. The accessor "getPrism" gives direct access to the value 786 */ 787 public DecimalType getPrismElement() { 788 if (this.prism == null) 789 if (Configuration.errorOnAutoCreate()) 790 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.prism"); 791 else if (Configuration.doAutoCreate()) 792 this.prism = new DecimalType(); // bb 793 return this.prism; 794 } 795 796 public boolean hasPrismElement() { 797 return this.prism != null && !this.prism.isEmpty(); 798 } 799 800 public boolean hasPrism() { 801 return this.prism != null && !this.prism.isEmpty(); 802 } 803 804 /** 805 * @param value {@link #prism} (Amount of prism to compensate for eye alignment in fractional units.). This is the underlying object with id, value and extensions. The accessor "getPrism" gives direct access to the value 806 */ 807 public VisionPrescriptionDispenseComponent setPrismElement(DecimalType value) { 808 this.prism = value; 809 return this; 810 } 811 812 /** 813 * @return Amount of prism to compensate for eye alignment in fractional units. 814 */ 815 public BigDecimal getPrism() { 816 return this.prism == null ? null : this.prism.getValue(); 817 } 818 819 /** 820 * @param value Amount of prism to compensate for eye alignment in fractional units. 821 */ 822 public VisionPrescriptionDispenseComponent setPrism(BigDecimal value) { 823 if (value == null) 824 this.prism = null; 825 else { 826 if (this.prism == null) 827 this.prism = new DecimalType(); 828 this.prism.setValue(value); 829 } 830 return this; 831 } 832 833 /** 834 * @param value Amount of prism to compensate for eye alignment in fractional units. 835 */ 836 public VisionPrescriptionDispenseComponent setPrism(long value) { 837 this.prism = new DecimalType(); 838 this.prism.setValue(value); 839 return this; 840 } 841 842 /** 843 * @param value Amount of prism to compensate for eye alignment in fractional units. 844 */ 845 public VisionPrescriptionDispenseComponent setPrism(double value) { 846 this.prism = new DecimalType(); 847 this.prism.setValue(value); 848 return this; 849 } 850 851 /** 852 * @return {@link #base} (The relative base, or reference lens edge, for the prism.). This is the underlying object with id, value and extensions. The accessor "getBase" gives direct access to the value 853 */ 854 public Enumeration<VisionBase> getBaseElement() { 855 if (this.base == null) 856 if (Configuration.errorOnAutoCreate()) 857 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.base"); 858 else if (Configuration.doAutoCreate()) 859 this.base = new Enumeration<VisionBase>(new VisionBaseEnumFactory()); // bb 860 return this.base; 861 } 862 863 public boolean hasBaseElement() { 864 return this.base != null && !this.base.isEmpty(); 865 } 866 867 public boolean hasBase() { 868 return this.base != null && !this.base.isEmpty(); 869 } 870 871 /** 872 * @param value {@link #base} (The relative base, or reference lens edge, for the prism.). This is the underlying object with id, value and extensions. The accessor "getBase" gives direct access to the value 873 */ 874 public VisionPrescriptionDispenseComponent setBaseElement(Enumeration<VisionBase> value) { 875 this.base = value; 876 return this; 877 } 878 879 /** 880 * @return The relative base, or reference lens edge, for the prism. 881 */ 882 public VisionBase getBase() { 883 return this.base == null ? null : this.base.getValue(); 884 } 885 886 /** 887 * @param value The relative base, or reference lens edge, for the prism. 888 */ 889 public VisionPrescriptionDispenseComponent setBase(VisionBase value) { 890 if (value == null) 891 this.base = null; 892 else { 893 if (this.base == null) 894 this.base = new Enumeration<VisionBase>(new VisionBaseEnumFactory()); 895 this.base.setValue(value); 896 } 897 return this; 898 } 899 900 /** 901 * @return {@link #add} (Power adjustment for multifocal lenses measured in diopters (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getAdd" gives direct access to the value 902 */ 903 public DecimalType getAddElement() { 904 if (this.add == null) 905 if (Configuration.errorOnAutoCreate()) 906 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.add"); 907 else if (Configuration.doAutoCreate()) 908 this.add = new DecimalType(); // bb 909 return this.add; 910 } 911 912 public boolean hasAddElement() { 913 return this.add != null && !this.add.isEmpty(); 914 } 915 916 public boolean hasAdd() { 917 return this.add != null && !this.add.isEmpty(); 918 } 919 920 /** 921 * @param value {@link #add} (Power adjustment for multifocal lenses measured in diopters (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getAdd" gives direct access to the value 922 */ 923 public VisionPrescriptionDispenseComponent setAddElement(DecimalType value) { 924 this.add = value; 925 return this; 926 } 927 928 /** 929 * @return Power adjustment for multifocal lenses measured in diopters (0.25 units). 930 */ 931 public BigDecimal getAdd() { 932 return this.add == null ? null : this.add.getValue(); 933 } 934 935 /** 936 * @param value Power adjustment for multifocal lenses measured in diopters (0.25 units). 937 */ 938 public VisionPrescriptionDispenseComponent setAdd(BigDecimal value) { 939 if (value == null) 940 this.add = null; 941 else { 942 if (this.add == null) 943 this.add = new DecimalType(); 944 this.add.setValue(value); 945 } 946 return this; 947 } 948 949 /** 950 * @param value Power adjustment for multifocal lenses measured in diopters (0.25 units). 951 */ 952 public VisionPrescriptionDispenseComponent setAdd(long value) { 953 this.add = new DecimalType(); 954 this.add.setValue(value); 955 return this; 956 } 957 958 /** 959 * @param value Power adjustment for multifocal lenses measured in diopters (0.25 units). 960 */ 961 public VisionPrescriptionDispenseComponent setAdd(double value) { 962 this.add = new DecimalType(); 963 this.add.setValue(value); 964 return this; 965 } 966 967 /** 968 * @return {@link #power} (Contact lens power measured in diopters (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getPower" gives direct access to the value 969 */ 970 public DecimalType getPowerElement() { 971 if (this.power == null) 972 if (Configuration.errorOnAutoCreate()) 973 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.power"); 974 else if (Configuration.doAutoCreate()) 975 this.power = new DecimalType(); // bb 976 return this.power; 977 } 978 979 public boolean hasPowerElement() { 980 return this.power != null && !this.power.isEmpty(); 981 } 982 983 public boolean hasPower() { 984 return this.power != null && !this.power.isEmpty(); 985 } 986 987 /** 988 * @param value {@link #power} (Contact lens power measured in diopters (0.25 units).). This is the underlying object with id, value and extensions. The accessor "getPower" gives direct access to the value 989 */ 990 public VisionPrescriptionDispenseComponent setPowerElement(DecimalType value) { 991 this.power = value; 992 return this; 993 } 994 995 /** 996 * @return Contact lens power measured in diopters (0.25 units). 997 */ 998 public BigDecimal getPower() { 999 return this.power == null ? null : this.power.getValue(); 1000 } 1001 1002 /** 1003 * @param value Contact lens power measured in diopters (0.25 units). 1004 */ 1005 public VisionPrescriptionDispenseComponent setPower(BigDecimal value) { 1006 if (value == null) 1007 this.power = null; 1008 else { 1009 if (this.power == null) 1010 this.power = new DecimalType(); 1011 this.power.setValue(value); 1012 } 1013 return this; 1014 } 1015 1016 /** 1017 * @param value Contact lens power measured in diopters (0.25 units). 1018 */ 1019 public VisionPrescriptionDispenseComponent setPower(long value) { 1020 this.power = new DecimalType(); 1021 this.power.setValue(value); 1022 return this; 1023 } 1024 1025 /** 1026 * @param value Contact lens power measured in diopters (0.25 units). 1027 */ 1028 public VisionPrescriptionDispenseComponent setPower(double value) { 1029 this.power = new DecimalType(); 1030 this.power.setValue(value); 1031 return this; 1032 } 1033 1034 /** 1035 * @return {@link #backCurve} (Back curvature measured in millimeters.). This is the underlying object with id, value and extensions. The accessor "getBackCurve" gives direct access to the value 1036 */ 1037 public DecimalType getBackCurveElement() { 1038 if (this.backCurve == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.backCurve"); 1041 else if (Configuration.doAutoCreate()) 1042 this.backCurve = new DecimalType(); // bb 1043 return this.backCurve; 1044 } 1045 1046 public boolean hasBackCurveElement() { 1047 return this.backCurve != null && !this.backCurve.isEmpty(); 1048 } 1049 1050 public boolean hasBackCurve() { 1051 return this.backCurve != null && !this.backCurve.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #backCurve} (Back curvature measured in millimeters.). This is the underlying object with id, value and extensions. The accessor "getBackCurve" gives direct access to the value 1056 */ 1057 public VisionPrescriptionDispenseComponent setBackCurveElement(DecimalType value) { 1058 this.backCurve = value; 1059 return this; 1060 } 1061 1062 /** 1063 * @return Back curvature measured in millimeters. 1064 */ 1065 public BigDecimal getBackCurve() { 1066 return this.backCurve == null ? null : this.backCurve.getValue(); 1067 } 1068 1069 /** 1070 * @param value Back curvature measured in millimeters. 1071 */ 1072 public VisionPrescriptionDispenseComponent setBackCurve(BigDecimal value) { 1073 if (value == null) 1074 this.backCurve = null; 1075 else { 1076 if (this.backCurve == null) 1077 this.backCurve = new DecimalType(); 1078 this.backCurve.setValue(value); 1079 } 1080 return this; 1081 } 1082 1083 /** 1084 * @param value Back curvature measured in millimeters. 1085 */ 1086 public VisionPrescriptionDispenseComponent setBackCurve(long value) { 1087 this.backCurve = new DecimalType(); 1088 this.backCurve.setValue(value); 1089 return this; 1090 } 1091 1092 /** 1093 * @param value Back curvature measured in millimeters. 1094 */ 1095 public VisionPrescriptionDispenseComponent setBackCurve(double value) { 1096 this.backCurve = new DecimalType(); 1097 this.backCurve.setValue(value); 1098 return this; 1099 } 1100 1101 /** 1102 * @return {@link #diameter} (Contact lens diameter measured in millimeters.). This is the underlying object with id, value and extensions. The accessor "getDiameter" gives direct access to the value 1103 */ 1104 public DecimalType getDiameterElement() { 1105 if (this.diameter == null) 1106 if (Configuration.errorOnAutoCreate()) 1107 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.diameter"); 1108 else if (Configuration.doAutoCreate()) 1109 this.diameter = new DecimalType(); // bb 1110 return this.diameter; 1111 } 1112 1113 public boolean hasDiameterElement() { 1114 return this.diameter != null && !this.diameter.isEmpty(); 1115 } 1116 1117 public boolean hasDiameter() { 1118 return this.diameter != null && !this.diameter.isEmpty(); 1119 } 1120 1121 /** 1122 * @param value {@link #diameter} (Contact lens diameter measured in millimeters.). This is the underlying object with id, value and extensions. The accessor "getDiameter" gives direct access to the value 1123 */ 1124 public VisionPrescriptionDispenseComponent setDiameterElement(DecimalType value) { 1125 this.diameter = value; 1126 return this; 1127 } 1128 1129 /** 1130 * @return Contact lens diameter measured in millimeters. 1131 */ 1132 public BigDecimal getDiameter() { 1133 return this.diameter == null ? null : this.diameter.getValue(); 1134 } 1135 1136 /** 1137 * @param value Contact lens diameter measured in millimeters. 1138 */ 1139 public VisionPrescriptionDispenseComponent setDiameter(BigDecimal value) { 1140 if (value == null) 1141 this.diameter = null; 1142 else { 1143 if (this.diameter == null) 1144 this.diameter = new DecimalType(); 1145 this.diameter.setValue(value); 1146 } 1147 return this; 1148 } 1149 1150 /** 1151 * @param value Contact lens diameter measured in millimeters. 1152 */ 1153 public VisionPrescriptionDispenseComponent setDiameter(long value) { 1154 this.diameter = new DecimalType(); 1155 this.diameter.setValue(value); 1156 return this; 1157 } 1158 1159 /** 1160 * @param value Contact lens diameter measured in millimeters. 1161 */ 1162 public VisionPrescriptionDispenseComponent setDiameter(double value) { 1163 this.diameter = new DecimalType(); 1164 this.diameter.setValue(value); 1165 return this; 1166 } 1167 1168 /** 1169 * @return {@link #duration} (The recommended maximum wear period for the lens.) 1170 */ 1171 public SimpleQuantity getDuration() { 1172 if (this.duration == null) 1173 if (Configuration.errorOnAutoCreate()) 1174 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.duration"); 1175 else if (Configuration.doAutoCreate()) 1176 this.duration = new SimpleQuantity(); // cc 1177 return this.duration; 1178 } 1179 1180 public boolean hasDuration() { 1181 return this.duration != null && !this.duration.isEmpty(); 1182 } 1183 1184 /** 1185 * @param value {@link #duration} (The recommended maximum wear period for the lens.) 1186 */ 1187 public VisionPrescriptionDispenseComponent setDuration(SimpleQuantity value) { 1188 this.duration = value; 1189 return this; 1190 } 1191 1192 /** 1193 * @return {@link #color} (Special color or pattern.). This is the underlying object with id, value and extensions. The accessor "getColor" gives direct access to the value 1194 */ 1195 public StringType getColorElement() { 1196 if (this.color == null) 1197 if (Configuration.errorOnAutoCreate()) 1198 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.color"); 1199 else if (Configuration.doAutoCreate()) 1200 this.color = new StringType(); // bb 1201 return this.color; 1202 } 1203 1204 public boolean hasColorElement() { 1205 return this.color != null && !this.color.isEmpty(); 1206 } 1207 1208 public boolean hasColor() { 1209 return this.color != null && !this.color.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #color} (Special color or pattern.). This is the underlying object with id, value and extensions. The accessor "getColor" gives direct access to the value 1214 */ 1215 public VisionPrescriptionDispenseComponent setColorElement(StringType value) { 1216 this.color = value; 1217 return this; 1218 } 1219 1220 /** 1221 * @return Special color or pattern. 1222 */ 1223 public String getColor() { 1224 return this.color == null ? null : this.color.getValue(); 1225 } 1226 1227 /** 1228 * @param value Special color or pattern. 1229 */ 1230 public VisionPrescriptionDispenseComponent setColor(String value) { 1231 if (Utilities.noString(value)) 1232 this.color = null; 1233 else { 1234 if (this.color == null) 1235 this.color = new StringType(); 1236 this.color.setValue(value); 1237 } 1238 return this; 1239 } 1240 1241 /** 1242 * @return {@link #brand} (Brand recommendations or restrictions.). This is the underlying object with id, value and extensions. The accessor "getBrand" gives direct access to the value 1243 */ 1244 public StringType getBrandElement() { 1245 if (this.brand == null) 1246 if (Configuration.errorOnAutoCreate()) 1247 throw new Error("Attempt to auto-create VisionPrescriptionDispenseComponent.brand"); 1248 else if (Configuration.doAutoCreate()) 1249 this.brand = new StringType(); // bb 1250 return this.brand; 1251 } 1252 1253 public boolean hasBrandElement() { 1254 return this.brand != null && !this.brand.isEmpty(); 1255 } 1256 1257 public boolean hasBrand() { 1258 return this.brand != null && !this.brand.isEmpty(); 1259 } 1260 1261 /** 1262 * @param value {@link #brand} (Brand recommendations or restrictions.). This is the underlying object with id, value and extensions. The accessor "getBrand" gives direct access to the value 1263 */ 1264 public VisionPrescriptionDispenseComponent setBrandElement(StringType value) { 1265 this.brand = value; 1266 return this; 1267 } 1268 1269 /** 1270 * @return Brand recommendations or restrictions. 1271 */ 1272 public String getBrand() { 1273 return this.brand == null ? null : this.brand.getValue(); 1274 } 1275 1276 /** 1277 * @param value Brand recommendations or restrictions. 1278 */ 1279 public VisionPrescriptionDispenseComponent setBrand(String value) { 1280 if (Utilities.noString(value)) 1281 this.brand = null; 1282 else { 1283 if (this.brand == null) 1284 this.brand = new StringType(); 1285 this.brand.setValue(value); 1286 } 1287 return this; 1288 } 1289 1290 /** 1291 * @return {@link #note} (Notes for special requirements such as coatings and lens materials.) 1292 */ 1293 public List<Annotation> getNote() { 1294 if (this.note == null) 1295 this.note = new ArrayList<Annotation>(); 1296 return this.note; 1297 } 1298 1299 /** 1300 * @return Returns a reference to <code>this</code> for easy method chaining 1301 */ 1302 public VisionPrescriptionDispenseComponent setNote(List<Annotation> theNote) { 1303 this.note = theNote; 1304 return this; 1305 } 1306 1307 public boolean hasNote() { 1308 if (this.note == null) 1309 return false; 1310 for (Annotation item : this.note) 1311 if (!item.isEmpty()) 1312 return true; 1313 return false; 1314 } 1315 1316 public Annotation addNote() { //3 1317 Annotation t = new Annotation(); 1318 if (this.note == null) 1319 this.note = new ArrayList<Annotation>(); 1320 this.note.add(t); 1321 return t; 1322 } 1323 1324 public VisionPrescriptionDispenseComponent addNote(Annotation t) { //3 1325 if (t == null) 1326 return this; 1327 if (this.note == null) 1328 this.note = new ArrayList<Annotation>(); 1329 this.note.add(t); 1330 return this; 1331 } 1332 1333 /** 1334 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1335 */ 1336 public Annotation getNoteFirstRep() { 1337 if (getNote().isEmpty()) { 1338 addNote(); 1339 } 1340 return getNote().get(0); 1341 } 1342 1343 protected void listChildren(List<Property> children) { 1344 super.listChildren(children); 1345 children.add(new Property("product", "CodeableConcept", "Identifies the type of vision correction product which is required for the patient.", 0, 1, product)); 1346 children.add(new Property("eye", "code", "The eye for which the lens applies.", 0, 1, eye)); 1347 children.add(new Property("sphere", "decimal", "Lens power measured in diopters (0.25 units).", 0, 1, sphere)); 1348 children.add(new Property("cylinder", "decimal", "Power adjustment for astigmatism measured in diopters (0.25 units).", 0, 1, cylinder)); 1349 children.add(new Property("axis", "integer", "Adjustment for astigmatism measured in integer degrees.", 0, 1, axis)); 1350 children.add(new Property("prism", "decimal", "Amount of prism to compensate for eye alignment in fractional units.", 0, 1, prism)); 1351 children.add(new Property("base", "code", "The relative base, or reference lens edge, for the prism.", 0, 1, base)); 1352 children.add(new Property("add", "decimal", "Power adjustment for multifocal lenses measured in diopters (0.25 units).", 0, 1, add)); 1353 children.add(new Property("power", "decimal", "Contact lens power measured in diopters (0.25 units).", 0, 1, power)); 1354 children.add(new Property("backCurve", "decimal", "Back curvature measured in millimeters.", 0, 1, backCurve)); 1355 children.add(new Property("diameter", "decimal", "Contact lens diameter measured in millimeters.", 0, 1, diameter)); 1356 children.add(new Property("duration", "SimpleQuantity", "The recommended maximum wear period for the lens.", 0, 1, duration)); 1357 children.add(new Property("color", "string", "Special color or pattern.", 0, 1, color)); 1358 children.add(new Property("brand", "string", "Brand recommendations or restrictions.", 0, 1, brand)); 1359 children.add(new Property("note", "Annotation", "Notes for special requirements such as coatings and lens materials.", 0, java.lang.Integer.MAX_VALUE, note)); 1360 } 1361 1362 @Override 1363 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1364 switch (_hash) { 1365 case -309474065: /*product*/ return new Property("product", "CodeableConcept", "Identifies the type of vision correction product which is required for the patient.", 0, 1, product); 1366 case 100913: /*eye*/ return new Property("eye", "code", "The eye for which the lens applies.", 0, 1, eye); 1367 case -895981619: /*sphere*/ return new Property("sphere", "decimal", "Lens power measured in diopters (0.25 units).", 0, 1, sphere); 1368 case -349378602: /*cylinder*/ return new Property("cylinder", "decimal", "Power adjustment for astigmatism measured in diopters (0.25 units).", 0, 1, cylinder); 1369 case 3008417: /*axis*/ return new Property("axis", "integer", "Adjustment for astigmatism measured in integer degrees.", 0, 1, axis); 1370 case 106935105: /*prism*/ return new Property("prism", "decimal", "Amount of prism to compensate for eye alignment in fractional units.", 0, 1, prism); 1371 case 3016401: /*base*/ return new Property("base", "code", "The relative base, or reference lens edge, for the prism.", 0, 1, base); 1372 case 96417: /*add*/ return new Property("add", "decimal", "Power adjustment for multifocal lenses measured in diopters (0.25 units).", 0, 1, add); 1373 case 106858757: /*power*/ return new Property("power", "decimal", "Contact lens power measured in diopters (0.25 units).", 0, 1, power); 1374 case 1309344840: /*backCurve*/ return new Property("backCurve", "decimal", "Back curvature measured in millimeters.", 0, 1, backCurve); 1375 case -233204595: /*diameter*/ return new Property("diameter", "decimal", "Contact lens diameter measured in millimeters.", 0, 1, diameter); 1376 case -1992012396: /*duration*/ return new Property("duration", "SimpleQuantity", "The recommended maximum wear period for the lens.", 0, 1, duration); 1377 case 94842723: /*color*/ return new Property("color", "string", "Special color or pattern.", 0, 1, color); 1378 case 93997959: /*brand*/ return new Property("brand", "string", "Brand recommendations or restrictions.", 0, 1, brand); 1379 case 3387378: /*note*/ return new Property("note", "Annotation", "Notes for special requirements such as coatings and lens materials.", 0, java.lang.Integer.MAX_VALUE, note); 1380 default: return super.getNamedProperty(_hash, _name, _checkValid); 1381 } 1382 1383 } 1384 1385 @Override 1386 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1387 switch (hash) { 1388 case -309474065: /*product*/ return this.product == null ? new Base[0] : new Base[] {this.product}; // CodeableConcept 1389 case 100913: /*eye*/ return this.eye == null ? new Base[0] : new Base[] {this.eye}; // Enumeration<VisionEyes> 1390 case -895981619: /*sphere*/ return this.sphere == null ? new Base[0] : new Base[] {this.sphere}; // DecimalType 1391 case -349378602: /*cylinder*/ return this.cylinder == null ? new Base[0] : new Base[] {this.cylinder}; // DecimalType 1392 case 3008417: /*axis*/ return this.axis == null ? new Base[0] : new Base[] {this.axis}; // IntegerType 1393 case 106935105: /*prism*/ return this.prism == null ? new Base[0] : new Base[] {this.prism}; // DecimalType 1394 case 3016401: /*base*/ return this.base == null ? new Base[0] : new Base[] {this.base}; // Enumeration<VisionBase> 1395 case 96417: /*add*/ return this.add == null ? new Base[0] : new Base[] {this.add}; // DecimalType 1396 case 106858757: /*power*/ return this.power == null ? new Base[0] : new Base[] {this.power}; // DecimalType 1397 case 1309344840: /*backCurve*/ return this.backCurve == null ? new Base[0] : new Base[] {this.backCurve}; // DecimalType 1398 case -233204595: /*diameter*/ return this.diameter == null ? new Base[0] : new Base[] {this.diameter}; // DecimalType 1399 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // SimpleQuantity 1400 case 94842723: /*color*/ return this.color == null ? new Base[0] : new Base[] {this.color}; // StringType 1401 case 93997959: /*brand*/ return this.brand == null ? new Base[0] : new Base[] {this.brand}; // StringType 1402 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1403 default: return super.getProperty(hash, name, checkValid); 1404 } 1405 1406 } 1407 1408 @Override 1409 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1410 switch (hash) { 1411 case -309474065: // product 1412 this.product = castToCodeableConcept(value); // CodeableConcept 1413 return value; 1414 case 100913: // eye 1415 value = new VisionEyesEnumFactory().fromType(castToCode(value)); 1416 this.eye = (Enumeration) value; // Enumeration<VisionEyes> 1417 return value; 1418 case -895981619: // sphere 1419 this.sphere = castToDecimal(value); // DecimalType 1420 return value; 1421 case -349378602: // cylinder 1422 this.cylinder = castToDecimal(value); // DecimalType 1423 return value; 1424 case 3008417: // axis 1425 this.axis = castToInteger(value); // IntegerType 1426 return value; 1427 case 106935105: // prism 1428 this.prism = castToDecimal(value); // DecimalType 1429 return value; 1430 case 3016401: // base 1431 value = new VisionBaseEnumFactory().fromType(castToCode(value)); 1432 this.base = (Enumeration) value; // Enumeration<VisionBase> 1433 return value; 1434 case 96417: // add 1435 this.add = castToDecimal(value); // DecimalType 1436 return value; 1437 case 106858757: // power 1438 this.power = castToDecimal(value); // DecimalType 1439 return value; 1440 case 1309344840: // backCurve 1441 this.backCurve = castToDecimal(value); // DecimalType 1442 return value; 1443 case -233204595: // diameter 1444 this.diameter = castToDecimal(value); // DecimalType 1445 return value; 1446 case -1992012396: // duration 1447 this.duration = castToSimpleQuantity(value); // SimpleQuantity 1448 return value; 1449 case 94842723: // color 1450 this.color = castToString(value); // StringType 1451 return value; 1452 case 93997959: // brand 1453 this.brand = castToString(value); // StringType 1454 return value; 1455 case 3387378: // note 1456 this.getNote().add(castToAnnotation(value)); // Annotation 1457 return value; 1458 default: return super.setProperty(hash, name, value); 1459 } 1460 1461 } 1462 1463 @Override 1464 public Base setProperty(String name, Base value) throws FHIRException { 1465 if (name.equals("product")) { 1466 this.product = castToCodeableConcept(value); // CodeableConcept 1467 } else if (name.equals("eye")) { 1468 value = new VisionEyesEnumFactory().fromType(castToCode(value)); 1469 this.eye = (Enumeration) value; // Enumeration<VisionEyes> 1470 } else if (name.equals("sphere")) { 1471 this.sphere = castToDecimal(value); // DecimalType 1472 } else if (name.equals("cylinder")) { 1473 this.cylinder = castToDecimal(value); // DecimalType 1474 } else if (name.equals("axis")) { 1475 this.axis = castToInteger(value); // IntegerType 1476 } else if (name.equals("prism")) { 1477 this.prism = castToDecimal(value); // DecimalType 1478 } else if (name.equals("base")) { 1479 value = new VisionBaseEnumFactory().fromType(castToCode(value)); 1480 this.base = (Enumeration) value; // Enumeration<VisionBase> 1481 } else if (name.equals("add")) { 1482 this.add = castToDecimal(value); // DecimalType 1483 } else if (name.equals("power")) { 1484 this.power = castToDecimal(value); // DecimalType 1485 } else if (name.equals("backCurve")) { 1486 this.backCurve = castToDecimal(value); // DecimalType 1487 } else if (name.equals("diameter")) { 1488 this.diameter = castToDecimal(value); // DecimalType 1489 } else if (name.equals("duration")) { 1490 this.duration = castToSimpleQuantity(value); // SimpleQuantity 1491 } else if (name.equals("color")) { 1492 this.color = castToString(value); // StringType 1493 } else if (name.equals("brand")) { 1494 this.brand = castToString(value); // StringType 1495 } else if (name.equals("note")) { 1496 this.getNote().add(castToAnnotation(value)); 1497 } else 1498 return super.setProperty(name, value); 1499 return value; 1500 } 1501 1502 @Override 1503 public Base makeProperty(int hash, String name) throws FHIRException { 1504 switch (hash) { 1505 case -309474065: return getProduct(); 1506 case 100913: return getEyeElement(); 1507 case -895981619: return getSphereElement(); 1508 case -349378602: return getCylinderElement(); 1509 case 3008417: return getAxisElement(); 1510 case 106935105: return getPrismElement(); 1511 case 3016401: return getBaseElement(); 1512 case 96417: return getAddElement(); 1513 case 106858757: return getPowerElement(); 1514 case 1309344840: return getBackCurveElement(); 1515 case -233204595: return getDiameterElement(); 1516 case -1992012396: return getDuration(); 1517 case 94842723: return getColorElement(); 1518 case 93997959: return getBrandElement(); 1519 case 3387378: return addNote(); 1520 default: return super.makeProperty(hash, name); 1521 } 1522 1523 } 1524 1525 @Override 1526 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1527 switch (hash) { 1528 case -309474065: /*product*/ return new String[] {"CodeableConcept"}; 1529 case 100913: /*eye*/ return new String[] {"code"}; 1530 case -895981619: /*sphere*/ return new String[] {"decimal"}; 1531 case -349378602: /*cylinder*/ return new String[] {"decimal"}; 1532 case 3008417: /*axis*/ return new String[] {"integer"}; 1533 case 106935105: /*prism*/ return new String[] {"decimal"}; 1534 case 3016401: /*base*/ return new String[] {"code"}; 1535 case 96417: /*add*/ return new String[] {"decimal"}; 1536 case 106858757: /*power*/ return new String[] {"decimal"}; 1537 case 1309344840: /*backCurve*/ return new String[] {"decimal"}; 1538 case -233204595: /*diameter*/ return new String[] {"decimal"}; 1539 case -1992012396: /*duration*/ return new String[] {"SimpleQuantity"}; 1540 case 94842723: /*color*/ return new String[] {"string"}; 1541 case 93997959: /*brand*/ return new String[] {"string"}; 1542 case 3387378: /*note*/ return new String[] {"Annotation"}; 1543 default: return super.getTypesForProperty(hash, name); 1544 } 1545 1546 } 1547 1548 @Override 1549 public Base addChild(String name) throws FHIRException { 1550 if (name.equals("product")) { 1551 this.product = new CodeableConcept(); 1552 return this.product; 1553 } 1554 else if (name.equals("eye")) { 1555 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.eye"); 1556 } 1557 else if (name.equals("sphere")) { 1558 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.sphere"); 1559 } 1560 else if (name.equals("cylinder")) { 1561 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.cylinder"); 1562 } 1563 else if (name.equals("axis")) { 1564 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.axis"); 1565 } 1566 else if (name.equals("prism")) { 1567 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.prism"); 1568 } 1569 else if (name.equals("base")) { 1570 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.base"); 1571 } 1572 else if (name.equals("add")) { 1573 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.add"); 1574 } 1575 else if (name.equals("power")) { 1576 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.power"); 1577 } 1578 else if (name.equals("backCurve")) { 1579 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.backCurve"); 1580 } 1581 else if (name.equals("diameter")) { 1582 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.diameter"); 1583 } 1584 else if (name.equals("duration")) { 1585 this.duration = new SimpleQuantity(); 1586 return this.duration; 1587 } 1588 else if (name.equals("color")) { 1589 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.color"); 1590 } 1591 else if (name.equals("brand")) { 1592 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.brand"); 1593 } 1594 else if (name.equals("note")) { 1595 return addNote(); 1596 } 1597 else 1598 return super.addChild(name); 1599 } 1600 1601 public VisionPrescriptionDispenseComponent copy() { 1602 VisionPrescriptionDispenseComponent dst = new VisionPrescriptionDispenseComponent(); 1603 copyValues(dst); 1604 dst.product = product == null ? null : product.copy(); 1605 dst.eye = eye == null ? null : eye.copy(); 1606 dst.sphere = sphere == null ? null : sphere.copy(); 1607 dst.cylinder = cylinder == null ? null : cylinder.copy(); 1608 dst.axis = axis == null ? null : axis.copy(); 1609 dst.prism = prism == null ? null : prism.copy(); 1610 dst.base = base == null ? null : base.copy(); 1611 dst.add = add == null ? null : add.copy(); 1612 dst.power = power == null ? null : power.copy(); 1613 dst.backCurve = backCurve == null ? null : backCurve.copy(); 1614 dst.diameter = diameter == null ? null : diameter.copy(); 1615 dst.duration = duration == null ? null : duration.copy(); 1616 dst.color = color == null ? null : color.copy(); 1617 dst.brand = brand == null ? null : brand.copy(); 1618 if (note != null) { 1619 dst.note = new ArrayList<Annotation>(); 1620 for (Annotation i : note) 1621 dst.note.add(i.copy()); 1622 }; 1623 return dst; 1624 } 1625 1626 @Override 1627 public boolean equalsDeep(Base other_) { 1628 if (!super.equalsDeep(other_)) 1629 return false; 1630 if (!(other_ instanceof VisionPrescriptionDispenseComponent)) 1631 return false; 1632 VisionPrescriptionDispenseComponent o = (VisionPrescriptionDispenseComponent) other_; 1633 return compareDeep(product, o.product, true) && compareDeep(eye, o.eye, true) && compareDeep(sphere, o.sphere, true) 1634 && compareDeep(cylinder, o.cylinder, true) && compareDeep(axis, o.axis, true) && compareDeep(prism, o.prism, true) 1635 && compareDeep(base, o.base, true) && compareDeep(add, o.add, true) && compareDeep(power, o.power, true) 1636 && compareDeep(backCurve, o.backCurve, true) && compareDeep(diameter, o.diameter, true) && compareDeep(duration, o.duration, true) 1637 && compareDeep(color, o.color, true) && compareDeep(brand, o.brand, true) && compareDeep(note, o.note, true) 1638 ; 1639 } 1640 1641 @Override 1642 public boolean equalsShallow(Base other_) { 1643 if (!super.equalsShallow(other_)) 1644 return false; 1645 if (!(other_ instanceof VisionPrescriptionDispenseComponent)) 1646 return false; 1647 VisionPrescriptionDispenseComponent o = (VisionPrescriptionDispenseComponent) other_; 1648 return compareValues(eye, o.eye, true) && compareValues(sphere, o.sphere, true) && compareValues(cylinder, o.cylinder, true) 1649 && compareValues(axis, o.axis, true) && compareValues(prism, o.prism, true) && compareValues(base, o.base, true) 1650 && compareValues(add, o.add, true) && compareValues(power, o.power, true) && compareValues(backCurve, o.backCurve, true) 1651 && compareValues(diameter, o.diameter, true) && compareValues(color, o.color, true) && compareValues(brand, o.brand, true) 1652 ; 1653 } 1654 1655 public boolean isEmpty() { 1656 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(product, eye, sphere, cylinder 1657 , axis, prism, base, add, power, backCurve, diameter, duration, color, brand 1658 , note); 1659 } 1660 1661 public String fhirType() { 1662 return "VisionPrescription.dispense"; 1663 1664 } 1665 1666 } 1667 1668 /** 1669 * Business identifier which may be used by other parties to reference or identify the prescription. 1670 */ 1671 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1672 @Description(shortDefinition="Business identifier", formalDefinition="Business identifier which may be used by other parties to reference or identify the prescription." ) 1673 protected List<Identifier> identifier; 1674 1675 /** 1676 * The status of the resource instance. 1677 */ 1678 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 1679 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 1680 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 1681 protected Enumeration<VisionStatus> status; 1682 1683 /** 1684 * A link to a resource representing the person to whom the vision products will be supplied. 1685 */ 1686 @Child(name = "patient", type = {Patient.class}, order=2, min=0, max=1, modifier=false, summary=false) 1687 @Description(shortDefinition="Who prescription is for", formalDefinition="A link to a resource representing the person to whom the vision products will be supplied." ) 1688 protected Reference patient; 1689 1690 /** 1691 * The actual object that is the target of the reference (A link to a resource representing the person to whom the vision products will be supplied.) 1692 */ 1693 protected Patient patientTarget; 1694 1695 /** 1696 * A link to a resource that identifies the particular occurrence of contact between patient and health care provider. 1697 */ 1698 @Child(name = "encounter", type = {Encounter.class}, order=3, min=0, max=1, modifier=false, summary=false) 1699 @Description(shortDefinition="Created during encounter / admission / stay", formalDefinition="A link to a resource that identifies the particular occurrence of contact between patient and health care provider." ) 1700 protected Reference encounter; 1701 1702 /** 1703 * The actual object that is the target of the reference (A link to a resource that identifies the particular occurrence of contact between patient and health care provider.) 1704 */ 1705 protected Encounter encounterTarget; 1706 1707 /** 1708 * The date (and perhaps time) when the prescription was written. 1709 */ 1710 @Child(name = "dateWritten", type = {DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1711 @Description(shortDefinition="When prescription was authorized", formalDefinition="The date (and perhaps time) when the prescription was written." ) 1712 protected DateTimeType dateWritten; 1713 1714 /** 1715 * The healthcare professional responsible for authorizing the prescription. 1716 */ 1717 @Child(name = "prescriber", type = {Practitioner.class}, order=5, min=0, max=1, modifier=false, summary=false) 1718 @Description(shortDefinition="Who authorizes the vision product", formalDefinition="The healthcare professional responsible for authorizing the prescription." ) 1719 protected Reference prescriber; 1720 1721 /** 1722 * The actual object that is the target of the reference (The healthcare professional responsible for authorizing the prescription.) 1723 */ 1724 protected Practitioner prescriberTarget; 1725 1726 /** 1727 * Can be the reason or the indication for writing the prescription. 1728 */ 1729 @Child(name = "reason", type = {CodeableConcept.class, Condition.class}, order=6, min=0, max=1, modifier=false, summary=false) 1730 @Description(shortDefinition="Reason or indication for writing the prescription", formalDefinition="Can be the reason or the indication for writing the prescription." ) 1731 protected Type reason; 1732 1733 /** 1734 * Deals with details of the dispense part of the supply specification. 1735 */ 1736 @Child(name = "dispense", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1737 @Description(shortDefinition="Vision supply authorization", formalDefinition="Deals with details of the dispense part of the supply specification." ) 1738 protected List<VisionPrescriptionDispenseComponent> dispense; 1739 1740 private static final long serialVersionUID = 603347490L; 1741 1742 /** 1743 * Constructor 1744 */ 1745 public VisionPrescription() { 1746 super(); 1747 } 1748 1749 /** 1750 * @return {@link #identifier} (Business identifier which may be used by other parties to reference or identify the prescription.) 1751 */ 1752 public List<Identifier> getIdentifier() { 1753 if (this.identifier == null) 1754 this.identifier = new ArrayList<Identifier>(); 1755 return this.identifier; 1756 } 1757 1758 /** 1759 * @return Returns a reference to <code>this</code> for easy method chaining 1760 */ 1761 public VisionPrescription setIdentifier(List<Identifier> theIdentifier) { 1762 this.identifier = theIdentifier; 1763 return this; 1764 } 1765 1766 public boolean hasIdentifier() { 1767 if (this.identifier == null) 1768 return false; 1769 for (Identifier item : this.identifier) 1770 if (!item.isEmpty()) 1771 return true; 1772 return false; 1773 } 1774 1775 public Identifier addIdentifier() { //3 1776 Identifier t = new Identifier(); 1777 if (this.identifier == null) 1778 this.identifier = new ArrayList<Identifier>(); 1779 this.identifier.add(t); 1780 return t; 1781 } 1782 1783 public VisionPrescription addIdentifier(Identifier t) { //3 1784 if (t == null) 1785 return this; 1786 if (this.identifier == null) 1787 this.identifier = new ArrayList<Identifier>(); 1788 this.identifier.add(t); 1789 return this; 1790 } 1791 1792 /** 1793 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1794 */ 1795 public Identifier getIdentifierFirstRep() { 1796 if (getIdentifier().isEmpty()) { 1797 addIdentifier(); 1798 } 1799 return getIdentifier().get(0); 1800 } 1801 1802 /** 1803 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1804 */ 1805 public Enumeration<VisionStatus> getStatusElement() { 1806 if (this.status == null) 1807 if (Configuration.errorOnAutoCreate()) 1808 throw new Error("Attempt to auto-create VisionPrescription.status"); 1809 else if (Configuration.doAutoCreate()) 1810 this.status = new Enumeration<VisionStatus>(new VisionStatusEnumFactory()); // bb 1811 return this.status; 1812 } 1813 1814 public boolean hasStatusElement() { 1815 return this.status != null && !this.status.isEmpty(); 1816 } 1817 1818 public boolean hasStatus() { 1819 return this.status != null && !this.status.isEmpty(); 1820 } 1821 1822 /** 1823 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1824 */ 1825 public VisionPrescription setStatusElement(Enumeration<VisionStatus> value) { 1826 this.status = value; 1827 return this; 1828 } 1829 1830 /** 1831 * @return The status of the resource instance. 1832 */ 1833 public VisionStatus getStatus() { 1834 return this.status == null ? null : this.status.getValue(); 1835 } 1836 1837 /** 1838 * @param value The status of the resource instance. 1839 */ 1840 public VisionPrescription setStatus(VisionStatus value) { 1841 if (value == null) 1842 this.status = null; 1843 else { 1844 if (this.status == null) 1845 this.status = new Enumeration<VisionStatus>(new VisionStatusEnumFactory()); 1846 this.status.setValue(value); 1847 } 1848 return this; 1849 } 1850 1851 /** 1852 * @return {@link #patient} (A link to a resource representing the person to whom the vision products will be supplied.) 1853 */ 1854 public Reference getPatient() { 1855 if (this.patient == null) 1856 if (Configuration.errorOnAutoCreate()) 1857 throw new Error("Attempt to auto-create VisionPrescription.patient"); 1858 else if (Configuration.doAutoCreate()) 1859 this.patient = new Reference(); // cc 1860 return this.patient; 1861 } 1862 1863 public boolean hasPatient() { 1864 return this.patient != null && !this.patient.isEmpty(); 1865 } 1866 1867 /** 1868 * @param value {@link #patient} (A link to a resource representing the person to whom the vision products will be supplied.) 1869 */ 1870 public VisionPrescription setPatient(Reference value) { 1871 this.patient = value; 1872 return this; 1873 } 1874 1875 /** 1876 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A link to a resource representing the person to whom the vision products will be supplied.) 1877 */ 1878 public Patient getPatientTarget() { 1879 if (this.patientTarget == null) 1880 if (Configuration.errorOnAutoCreate()) 1881 throw new Error("Attempt to auto-create VisionPrescription.patient"); 1882 else if (Configuration.doAutoCreate()) 1883 this.patientTarget = new Patient(); // aa 1884 return this.patientTarget; 1885 } 1886 1887 /** 1888 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A link to a resource representing the person to whom the vision products will be supplied.) 1889 */ 1890 public VisionPrescription setPatientTarget(Patient value) { 1891 this.patientTarget = value; 1892 return this; 1893 } 1894 1895 /** 1896 * @return {@link #encounter} (A link to a resource that identifies the particular occurrence of contact between patient and health care provider.) 1897 */ 1898 public Reference getEncounter() { 1899 if (this.encounter == null) 1900 if (Configuration.errorOnAutoCreate()) 1901 throw new Error("Attempt to auto-create VisionPrescription.encounter"); 1902 else if (Configuration.doAutoCreate()) 1903 this.encounter = new Reference(); // cc 1904 return this.encounter; 1905 } 1906 1907 public boolean hasEncounter() { 1908 return this.encounter != null && !this.encounter.isEmpty(); 1909 } 1910 1911 /** 1912 * @param value {@link #encounter} (A link to a resource that identifies the particular occurrence of contact between patient and health care provider.) 1913 */ 1914 public VisionPrescription setEncounter(Reference value) { 1915 this.encounter = value; 1916 return this; 1917 } 1918 1919 /** 1920 * @return {@link #encounter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A link to a resource that identifies the particular occurrence of contact between patient and health care provider.) 1921 */ 1922 public Encounter getEncounterTarget() { 1923 if (this.encounterTarget == null) 1924 if (Configuration.errorOnAutoCreate()) 1925 throw new Error("Attempt to auto-create VisionPrescription.encounter"); 1926 else if (Configuration.doAutoCreate()) 1927 this.encounterTarget = new Encounter(); // aa 1928 return this.encounterTarget; 1929 } 1930 1931 /** 1932 * @param value {@link #encounter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A link to a resource that identifies the particular occurrence of contact between patient and health care provider.) 1933 */ 1934 public VisionPrescription setEncounterTarget(Encounter value) { 1935 this.encounterTarget = value; 1936 return this; 1937 } 1938 1939 /** 1940 * @return {@link #dateWritten} (The date (and perhaps time) when the prescription was written.). This is the underlying object with id, value and extensions. The accessor "getDateWritten" gives direct access to the value 1941 */ 1942 public DateTimeType getDateWrittenElement() { 1943 if (this.dateWritten == null) 1944 if (Configuration.errorOnAutoCreate()) 1945 throw new Error("Attempt to auto-create VisionPrescription.dateWritten"); 1946 else if (Configuration.doAutoCreate()) 1947 this.dateWritten = new DateTimeType(); // bb 1948 return this.dateWritten; 1949 } 1950 1951 public boolean hasDateWrittenElement() { 1952 return this.dateWritten != null && !this.dateWritten.isEmpty(); 1953 } 1954 1955 public boolean hasDateWritten() { 1956 return this.dateWritten != null && !this.dateWritten.isEmpty(); 1957 } 1958 1959 /** 1960 * @param value {@link #dateWritten} (The date (and perhaps time) when the prescription was written.). This is the underlying object with id, value and extensions. The accessor "getDateWritten" gives direct access to the value 1961 */ 1962 public VisionPrescription setDateWrittenElement(DateTimeType value) { 1963 this.dateWritten = value; 1964 return this; 1965 } 1966 1967 /** 1968 * @return The date (and perhaps time) when the prescription was written. 1969 */ 1970 public Date getDateWritten() { 1971 return this.dateWritten == null ? null : this.dateWritten.getValue(); 1972 } 1973 1974 /** 1975 * @param value The date (and perhaps time) when the prescription was written. 1976 */ 1977 public VisionPrescription setDateWritten(Date value) { 1978 if (value == null) 1979 this.dateWritten = null; 1980 else { 1981 if (this.dateWritten == null) 1982 this.dateWritten = new DateTimeType(); 1983 this.dateWritten.setValue(value); 1984 } 1985 return this; 1986 } 1987 1988 /** 1989 * @return {@link #prescriber} (The healthcare professional responsible for authorizing the prescription.) 1990 */ 1991 public Reference getPrescriber() { 1992 if (this.prescriber == null) 1993 if (Configuration.errorOnAutoCreate()) 1994 throw new Error("Attempt to auto-create VisionPrescription.prescriber"); 1995 else if (Configuration.doAutoCreate()) 1996 this.prescriber = new Reference(); // cc 1997 return this.prescriber; 1998 } 1999 2000 public boolean hasPrescriber() { 2001 return this.prescriber != null && !this.prescriber.isEmpty(); 2002 } 2003 2004 /** 2005 * @param value {@link #prescriber} (The healthcare professional responsible for authorizing the prescription.) 2006 */ 2007 public VisionPrescription setPrescriber(Reference value) { 2008 this.prescriber = value; 2009 return this; 2010 } 2011 2012 /** 2013 * @return {@link #prescriber} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The healthcare professional responsible for authorizing the prescription.) 2014 */ 2015 public Practitioner getPrescriberTarget() { 2016 if (this.prescriberTarget == null) 2017 if (Configuration.errorOnAutoCreate()) 2018 throw new Error("Attempt to auto-create VisionPrescription.prescriber"); 2019 else if (Configuration.doAutoCreate()) 2020 this.prescriberTarget = new Practitioner(); // aa 2021 return this.prescriberTarget; 2022 } 2023 2024 /** 2025 * @param value {@link #prescriber} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The healthcare professional responsible for authorizing the prescription.) 2026 */ 2027 public VisionPrescription setPrescriberTarget(Practitioner value) { 2028 this.prescriberTarget = value; 2029 return this; 2030 } 2031 2032 /** 2033 * @return {@link #reason} (Can be the reason or the indication for writing the prescription.) 2034 */ 2035 public Type getReason() { 2036 return this.reason; 2037 } 2038 2039 /** 2040 * @return {@link #reason} (Can be the reason or the indication for writing the prescription.) 2041 */ 2042 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 2043 if (this.reason == null) 2044 return null; 2045 if (!(this.reason instanceof CodeableConcept)) 2046 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.reason.getClass().getName()+" was encountered"); 2047 return (CodeableConcept) this.reason; 2048 } 2049 2050 public boolean hasReasonCodeableConcept() { 2051 return this != null && this.reason instanceof CodeableConcept; 2052 } 2053 2054 /** 2055 * @return {@link #reason} (Can be the reason or the indication for writing the prescription.) 2056 */ 2057 public Reference getReasonReference() throws FHIRException { 2058 if (this.reason == null) 2059 return null; 2060 if (!(this.reason instanceof Reference)) 2061 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.reason.getClass().getName()+" was encountered"); 2062 return (Reference) this.reason; 2063 } 2064 2065 public boolean hasReasonReference() { 2066 return this != null && this.reason instanceof Reference; 2067 } 2068 2069 public boolean hasReason() { 2070 return this.reason != null && !this.reason.isEmpty(); 2071 } 2072 2073 /** 2074 * @param value {@link #reason} (Can be the reason or the indication for writing the prescription.) 2075 */ 2076 public VisionPrescription setReason(Type value) throws FHIRFormatError { 2077 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2078 throw new FHIRFormatError("Not the right type for VisionPrescription.reason[x]: "+value.fhirType()); 2079 this.reason = value; 2080 return this; 2081 } 2082 2083 /** 2084 * @return {@link #dispense} (Deals with details of the dispense part of the supply specification.) 2085 */ 2086 public List<VisionPrescriptionDispenseComponent> getDispense() { 2087 if (this.dispense == null) 2088 this.dispense = new ArrayList<VisionPrescriptionDispenseComponent>(); 2089 return this.dispense; 2090 } 2091 2092 /** 2093 * @return Returns a reference to <code>this</code> for easy method chaining 2094 */ 2095 public VisionPrescription setDispense(List<VisionPrescriptionDispenseComponent> theDispense) { 2096 this.dispense = theDispense; 2097 return this; 2098 } 2099 2100 public boolean hasDispense() { 2101 if (this.dispense == null) 2102 return false; 2103 for (VisionPrescriptionDispenseComponent item : this.dispense) 2104 if (!item.isEmpty()) 2105 return true; 2106 return false; 2107 } 2108 2109 public VisionPrescriptionDispenseComponent addDispense() { //3 2110 VisionPrescriptionDispenseComponent t = new VisionPrescriptionDispenseComponent(); 2111 if (this.dispense == null) 2112 this.dispense = new ArrayList<VisionPrescriptionDispenseComponent>(); 2113 this.dispense.add(t); 2114 return t; 2115 } 2116 2117 public VisionPrescription addDispense(VisionPrescriptionDispenseComponent t) { //3 2118 if (t == null) 2119 return this; 2120 if (this.dispense == null) 2121 this.dispense = new ArrayList<VisionPrescriptionDispenseComponent>(); 2122 this.dispense.add(t); 2123 return this; 2124 } 2125 2126 /** 2127 * @return The first repetition of repeating field {@link #dispense}, creating it if it does not already exist 2128 */ 2129 public VisionPrescriptionDispenseComponent getDispenseFirstRep() { 2130 if (getDispense().isEmpty()) { 2131 addDispense(); 2132 } 2133 return getDispense().get(0); 2134 } 2135 2136 protected void listChildren(List<Property> children) { 2137 super.listChildren(children); 2138 children.add(new Property("identifier", "Identifier", "Business identifier which may be used by other parties to reference or identify the prescription.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2139 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2140 children.add(new Property("patient", "Reference(Patient)", "A link to a resource representing the person to whom the vision products will be supplied.", 0, 1, patient)); 2141 children.add(new Property("encounter", "Reference(Encounter)", "A link to a resource that identifies the particular occurrence of contact between patient and health care provider.", 0, 1, encounter)); 2142 children.add(new Property("dateWritten", "dateTime", "The date (and perhaps time) when the prescription was written.", 0, 1, dateWritten)); 2143 children.add(new Property("prescriber", "Reference(Practitioner)", "The healthcare professional responsible for authorizing the prescription.", 0, 1, prescriber)); 2144 children.add(new Property("reason[x]", "CodeableConcept|Reference(Condition)", "Can be the reason or the indication for writing the prescription.", 0, 1, reason)); 2145 children.add(new Property("dispense", "", "Deals with details of the dispense part of the supply specification.", 0, java.lang.Integer.MAX_VALUE, dispense)); 2146 } 2147 2148 @Override 2149 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2150 switch (_hash) { 2151 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier which may be used by other parties to reference or identify the prescription.", 0, java.lang.Integer.MAX_VALUE, identifier); 2152 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2153 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "A link to a resource representing the person to whom the vision products will be supplied.", 0, 1, patient); 2154 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "A link to a resource that identifies the particular occurrence of contact between patient and health care provider.", 0, 1, encounter); 2155 case -1496880759: /*dateWritten*/ return new Property("dateWritten", "dateTime", "The date (and perhaps time) when the prescription was written.", 0, 1, dateWritten); 2156 case 1430631077: /*prescriber*/ return new Property("prescriber", "Reference(Practitioner)", "The healthcare professional responsible for authorizing the prescription.", 0, 1, prescriber); 2157 case -669418564: /*reason[x]*/ return new Property("reason[x]", "CodeableConcept|Reference(Condition)", "Can be the reason or the indication for writing the prescription.", 0, 1, reason); 2158 case -934964668: /*reason*/ return new Property("reason[x]", "CodeableConcept|Reference(Condition)", "Can be the reason or the indication for writing the prescription.", 0, 1, reason); 2159 case -610155331: /*reasonCodeableConcept*/ return new Property("reason[x]", "CodeableConcept|Reference(Condition)", "Can be the reason or the indication for writing the prescription.", 0, 1, reason); 2160 case -1146218137: /*reasonReference*/ return new Property("reason[x]", "CodeableConcept|Reference(Condition)", "Can be the reason or the indication for writing the prescription.", 0, 1, reason); 2161 case 284885341: /*dispense*/ return new Property("dispense", "", "Deals with details of the dispense part of the supply specification.", 0, java.lang.Integer.MAX_VALUE, dispense); 2162 default: return super.getNamedProperty(_hash, _name, _checkValid); 2163 } 2164 2165 } 2166 2167 @Override 2168 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2169 switch (hash) { 2170 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2171 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<VisionStatus> 2172 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2173 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2174 case -1496880759: /*dateWritten*/ return this.dateWritten == null ? new Base[0] : new Base[] {this.dateWritten}; // DateTimeType 2175 case 1430631077: /*prescriber*/ return this.prescriber == null ? new Base[0] : new Base[] {this.prescriber}; // Reference 2176 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // Type 2177 case 284885341: /*dispense*/ return this.dispense == null ? new Base[0] : this.dispense.toArray(new Base[this.dispense.size()]); // VisionPrescriptionDispenseComponent 2178 default: return super.getProperty(hash, name, checkValid); 2179 } 2180 2181 } 2182 2183 @Override 2184 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2185 switch (hash) { 2186 case -1618432855: // identifier 2187 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2188 return value; 2189 case -892481550: // status 2190 value = new VisionStatusEnumFactory().fromType(castToCode(value)); 2191 this.status = (Enumeration) value; // Enumeration<VisionStatus> 2192 return value; 2193 case -791418107: // patient 2194 this.patient = castToReference(value); // Reference 2195 return value; 2196 case 1524132147: // encounter 2197 this.encounter = castToReference(value); // Reference 2198 return value; 2199 case -1496880759: // dateWritten 2200 this.dateWritten = castToDateTime(value); // DateTimeType 2201 return value; 2202 case 1430631077: // prescriber 2203 this.prescriber = castToReference(value); // Reference 2204 return value; 2205 case -934964668: // reason 2206 this.reason = castToType(value); // Type 2207 return value; 2208 case 284885341: // dispense 2209 this.getDispense().add((VisionPrescriptionDispenseComponent) value); // VisionPrescriptionDispenseComponent 2210 return value; 2211 default: return super.setProperty(hash, name, value); 2212 } 2213 2214 } 2215 2216 @Override 2217 public Base setProperty(String name, Base value) throws FHIRException { 2218 if (name.equals("identifier")) { 2219 this.getIdentifier().add(castToIdentifier(value)); 2220 } else if (name.equals("status")) { 2221 value = new VisionStatusEnumFactory().fromType(castToCode(value)); 2222 this.status = (Enumeration) value; // Enumeration<VisionStatus> 2223 } else if (name.equals("patient")) { 2224 this.patient = castToReference(value); // Reference 2225 } else if (name.equals("encounter")) { 2226 this.encounter = castToReference(value); // Reference 2227 } else if (name.equals("dateWritten")) { 2228 this.dateWritten = castToDateTime(value); // DateTimeType 2229 } else if (name.equals("prescriber")) { 2230 this.prescriber = castToReference(value); // Reference 2231 } else if (name.equals("reason[x]")) { 2232 this.reason = castToType(value); // Type 2233 } else if (name.equals("dispense")) { 2234 this.getDispense().add((VisionPrescriptionDispenseComponent) value); 2235 } else 2236 return super.setProperty(name, value); 2237 return value; 2238 } 2239 2240 @Override 2241 public Base makeProperty(int hash, String name) throws FHIRException { 2242 switch (hash) { 2243 case -1618432855: return addIdentifier(); 2244 case -892481550: return getStatusElement(); 2245 case -791418107: return getPatient(); 2246 case 1524132147: return getEncounter(); 2247 case -1496880759: return getDateWrittenElement(); 2248 case 1430631077: return getPrescriber(); 2249 case -669418564: return getReason(); 2250 case -934964668: return getReason(); 2251 case 284885341: return addDispense(); 2252 default: return super.makeProperty(hash, name); 2253 } 2254 2255 } 2256 2257 @Override 2258 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2259 switch (hash) { 2260 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2261 case -892481550: /*status*/ return new String[] {"code"}; 2262 case -791418107: /*patient*/ return new String[] {"Reference"}; 2263 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2264 case -1496880759: /*dateWritten*/ return new String[] {"dateTime"}; 2265 case 1430631077: /*prescriber*/ return new String[] {"Reference"}; 2266 case -934964668: /*reason*/ return new String[] {"CodeableConcept", "Reference"}; 2267 case 284885341: /*dispense*/ return new String[] {}; 2268 default: return super.getTypesForProperty(hash, name); 2269 } 2270 2271 } 2272 2273 @Override 2274 public Base addChild(String name) throws FHIRException { 2275 if (name.equals("identifier")) { 2276 return addIdentifier(); 2277 } 2278 else if (name.equals("status")) { 2279 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.status"); 2280 } 2281 else if (name.equals("patient")) { 2282 this.patient = new Reference(); 2283 return this.patient; 2284 } 2285 else if (name.equals("encounter")) { 2286 this.encounter = new Reference(); 2287 return this.encounter; 2288 } 2289 else if (name.equals("dateWritten")) { 2290 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.dateWritten"); 2291 } 2292 else if (name.equals("prescriber")) { 2293 this.prescriber = new Reference(); 2294 return this.prescriber; 2295 } 2296 else if (name.equals("reasonCodeableConcept")) { 2297 this.reason = new CodeableConcept(); 2298 return this.reason; 2299 } 2300 else if (name.equals("reasonReference")) { 2301 this.reason = new Reference(); 2302 return this.reason; 2303 } 2304 else if (name.equals("dispense")) { 2305 return addDispense(); 2306 } 2307 else 2308 return super.addChild(name); 2309 } 2310 2311 public String fhirType() { 2312 return "VisionPrescription"; 2313 2314 } 2315 2316 public VisionPrescription copy() { 2317 VisionPrescription dst = new VisionPrescription(); 2318 copyValues(dst); 2319 if (identifier != null) { 2320 dst.identifier = new ArrayList<Identifier>(); 2321 for (Identifier i : identifier) 2322 dst.identifier.add(i.copy()); 2323 }; 2324 dst.status = status == null ? null : status.copy(); 2325 dst.patient = patient == null ? null : patient.copy(); 2326 dst.encounter = encounter == null ? null : encounter.copy(); 2327 dst.dateWritten = dateWritten == null ? null : dateWritten.copy(); 2328 dst.prescriber = prescriber == null ? null : prescriber.copy(); 2329 dst.reason = reason == null ? null : reason.copy(); 2330 if (dispense != null) { 2331 dst.dispense = new ArrayList<VisionPrescriptionDispenseComponent>(); 2332 for (VisionPrescriptionDispenseComponent i : dispense) 2333 dst.dispense.add(i.copy()); 2334 }; 2335 return dst; 2336 } 2337 2338 protected VisionPrescription typedCopy() { 2339 return copy(); 2340 } 2341 2342 @Override 2343 public boolean equalsDeep(Base other_) { 2344 if (!super.equalsDeep(other_)) 2345 return false; 2346 if (!(other_ instanceof VisionPrescription)) 2347 return false; 2348 VisionPrescription o = (VisionPrescription) other_; 2349 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(patient, o.patient, true) 2350 && compareDeep(encounter, o.encounter, true) && compareDeep(dateWritten, o.dateWritten, true) && compareDeep(prescriber, o.prescriber, true) 2351 && compareDeep(reason, o.reason, true) && compareDeep(dispense, o.dispense, true); 2352 } 2353 2354 @Override 2355 public boolean equalsShallow(Base other_) { 2356 if (!super.equalsShallow(other_)) 2357 return false; 2358 if (!(other_ instanceof VisionPrescription)) 2359 return false; 2360 VisionPrescription o = (VisionPrescription) other_; 2361 return compareValues(status, o.status, true) && compareValues(dateWritten, o.dateWritten, true); 2362 } 2363 2364 public boolean isEmpty() { 2365 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, patient 2366 , encounter, dateWritten, prescriber, reason, dispense); 2367 } 2368 2369 @Override 2370 public ResourceType getResourceType() { 2371 return ResourceType.VisionPrescription; 2372 } 2373 2374 /** 2375 * Search parameter: <b>prescriber</b> 2376 * <p> 2377 * Description: <b>Who authorizes the vision product</b><br> 2378 * Type: <b>reference</b><br> 2379 * Path: <b>VisionPrescription.prescriber</b><br> 2380 * </p> 2381 */ 2382 @SearchParamDefinition(name="prescriber", path="VisionPrescription.prescriber", description="Who authorizes the vision product", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2383 public static final String SP_PRESCRIBER = "prescriber"; 2384 /** 2385 * <b>Fluent Client</b> search parameter constant for <b>prescriber</b> 2386 * <p> 2387 * Description: <b>Who authorizes the vision product</b><br> 2388 * Type: <b>reference</b><br> 2389 * Path: <b>VisionPrescription.prescriber</b><br> 2390 * </p> 2391 */ 2392 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRESCRIBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRESCRIBER); 2393 2394/** 2395 * Constant for fluent queries to be used to add include statements. Specifies 2396 * the path value of "<b>VisionPrescription:prescriber</b>". 2397 */ 2398 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRESCRIBER = new ca.uhn.fhir.model.api.Include("VisionPrescription:prescriber").toLocked(); 2399 2400 /** 2401 * Search parameter: <b>identifier</b> 2402 * <p> 2403 * Description: <b>Return prescriptions with this external identifier</b><br> 2404 * Type: <b>token</b><br> 2405 * Path: <b>VisionPrescription.identifier</b><br> 2406 * </p> 2407 */ 2408 @SearchParamDefinition(name="identifier", path="VisionPrescription.identifier", description="Return prescriptions with this external identifier", type="token" ) 2409 public static final String SP_IDENTIFIER = "identifier"; 2410 /** 2411 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2412 * <p> 2413 * Description: <b>Return prescriptions with this external identifier</b><br> 2414 * Type: <b>token</b><br> 2415 * Path: <b>VisionPrescription.identifier</b><br> 2416 * </p> 2417 */ 2418 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2419 2420 /** 2421 * Search parameter: <b>patient</b> 2422 * <p> 2423 * Description: <b>The identity of a patient to list dispenses for</b><br> 2424 * Type: <b>reference</b><br> 2425 * Path: <b>VisionPrescription.patient</b><br> 2426 * </p> 2427 */ 2428 @SearchParamDefinition(name="patient", path="VisionPrescription.patient", description="The identity of a patient to list dispenses for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 2429 public static final String SP_PATIENT = "patient"; 2430 /** 2431 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2432 * <p> 2433 * Description: <b>The identity of a patient to list dispenses for</b><br> 2434 * Type: <b>reference</b><br> 2435 * Path: <b>VisionPrescription.patient</b><br> 2436 * </p> 2437 */ 2438 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2439 2440/** 2441 * Constant for fluent queries to be used to add include statements. Specifies 2442 * the path value of "<b>VisionPrescription:patient</b>". 2443 */ 2444 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("VisionPrescription:patient").toLocked(); 2445 2446 /** 2447 * Search parameter: <b>datewritten</b> 2448 * <p> 2449 * Description: <b>Return prescriptions written on this date</b><br> 2450 * Type: <b>date</b><br> 2451 * Path: <b>VisionPrescription.dateWritten</b><br> 2452 * </p> 2453 */ 2454 @SearchParamDefinition(name="datewritten", path="VisionPrescription.dateWritten", description="Return prescriptions written on this date", type="date" ) 2455 public static final String SP_DATEWRITTEN = "datewritten"; 2456 /** 2457 * <b>Fluent Client</b> search parameter constant for <b>datewritten</b> 2458 * <p> 2459 * Description: <b>Return prescriptions written on this date</b><br> 2460 * Type: <b>date</b><br> 2461 * Path: <b>VisionPrescription.dateWritten</b><br> 2462 * </p> 2463 */ 2464 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATEWRITTEN = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATEWRITTEN); 2465 2466 /** 2467 * Search parameter: <b>encounter</b> 2468 * <p> 2469 * Description: <b>Return prescriptions with this encounter identifier</b><br> 2470 * Type: <b>reference</b><br> 2471 * Path: <b>VisionPrescription.encounter</b><br> 2472 * </p> 2473 */ 2474 @SearchParamDefinition(name="encounter", path="VisionPrescription.encounter", description="Return prescriptions with this encounter identifier", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 2475 public static final String SP_ENCOUNTER = "encounter"; 2476 /** 2477 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2478 * <p> 2479 * Description: <b>Return prescriptions with this encounter identifier</b><br> 2480 * Type: <b>reference</b><br> 2481 * Path: <b>VisionPrescription.encounter</b><br> 2482 * </p> 2483 */ 2484 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 2485 2486/** 2487 * Constant for fluent queries to be used to add include statements. Specifies 2488 * the path value of "<b>VisionPrescription:encounter</b>". 2489 */ 2490 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("VisionPrescription:encounter").toLocked(); 2491 2492 2493}