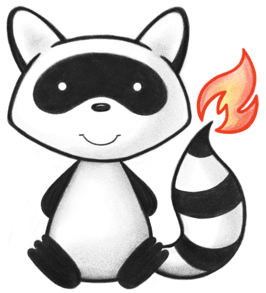
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ActionRelationshipType { 041 042 /** 043 * The action must be performed before the start of the related action 044 */ 045 BEFORESTART, 046 /** 047 * The action must be performed before the related action 048 */ 049 BEFORE, 050 /** 051 * The action must be performed before the end of the related action 052 */ 053 BEFOREEND, 054 /** 055 * The action must be performed concurrent with the start of the related action 056 */ 057 CONCURRENTWITHSTART, 058 /** 059 * The action must be performed concurrent with the related action 060 */ 061 CONCURRENT, 062 /** 063 * The action must be performed concurrent with the end of the related action 064 */ 065 CONCURRENTWITHEND, 066 /** 067 * The action must be performed after the start of the related action 068 */ 069 AFTERSTART, 070 /** 071 * The action must be performed after the related action 072 */ 073 AFTER, 074 /** 075 * The action must be performed after the end of the related action 076 */ 077 AFTEREND, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 public static ActionRelationshipType fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("before-start".equals(codeString)) 086 return BEFORESTART; 087 if ("before".equals(codeString)) 088 return BEFORE; 089 if ("before-end".equals(codeString)) 090 return BEFOREEND; 091 if ("concurrent-with-start".equals(codeString)) 092 return CONCURRENTWITHSTART; 093 if ("concurrent".equals(codeString)) 094 return CONCURRENT; 095 if ("concurrent-with-end".equals(codeString)) 096 return CONCURRENTWITHEND; 097 if ("after-start".equals(codeString)) 098 return AFTERSTART; 099 if ("after".equals(codeString)) 100 return AFTER; 101 if ("after-end".equals(codeString)) 102 return AFTEREND; 103 throw new FHIRException("Unknown ActionRelationshipType code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case BEFORESTART: return "before-start"; 108 case BEFORE: return "before"; 109 case BEFOREEND: return "before-end"; 110 case CONCURRENTWITHSTART: return "concurrent-with-start"; 111 case CONCURRENT: return "concurrent"; 112 case CONCURRENTWITHEND: return "concurrent-with-end"; 113 case AFTERSTART: return "after-start"; 114 case AFTER: return "after"; 115 case AFTEREND: return "after-end"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getSystem() { 121 return "http://hl7.org/fhir/action-relationship-type"; 122 } 123 public String getDefinition() { 124 switch (this) { 125 case BEFORESTART: return "The action must be performed before the start of the related action"; 126 case BEFORE: return "The action must be performed before the related action"; 127 case BEFOREEND: return "The action must be performed before the end of the related action"; 128 case CONCURRENTWITHSTART: return "The action must be performed concurrent with the start of the related action"; 129 case CONCURRENT: return "The action must be performed concurrent with the related action"; 130 case CONCURRENTWITHEND: return "The action must be performed concurrent with the end of the related action"; 131 case AFTERSTART: return "The action must be performed after the start of the related action"; 132 case AFTER: return "The action must be performed after the related action"; 133 case AFTEREND: return "The action must be performed after the end of the related action"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getDisplay() { 139 switch (this) { 140 case BEFORESTART: return "Before Start"; 141 case BEFORE: return "Before"; 142 case BEFOREEND: return "Before End"; 143 case CONCURRENTWITHSTART: return "Concurrent With Start"; 144 case CONCURRENT: return "Concurrent"; 145 case CONCURRENTWITHEND: return "Concurrent With End"; 146 case AFTERSTART: return "After Start"; 147 case AFTER: return "After"; 148 case AFTEREND: return "After End"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 154 155}