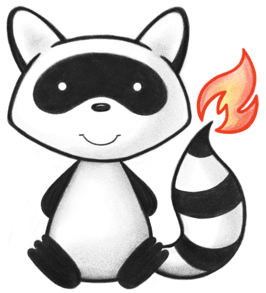
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum Adjudication { 041 042 /** 043 * Total submitted 044 */ 045 TOTAL, 046 /** 047 * Patient Co-Payment 048 */ 049 COPAY, 050 /** 051 * Amount of the change which is considered for adjudication 052 */ 053 ELIGIBLE, 054 /** 055 * Amount deducted from the eligible amount prior to adjudication 056 */ 057 DEDUCTIBLE, 058 /** 059 * Eligible Percentage 060 */ 061 ELIGPERCENT, 062 /** 063 * Emergency Department 064 */ 065 TAX, 066 /** 067 * Amount payable under the coverage 068 */ 069 BENEFIT, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 public static Adjudication fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("total".equals(codeString)) 078 return TOTAL; 079 if ("copay".equals(codeString)) 080 return COPAY; 081 if ("eligible".equals(codeString)) 082 return ELIGIBLE; 083 if ("deductible".equals(codeString)) 084 return DEDUCTIBLE; 085 if ("eligpercent".equals(codeString)) 086 return ELIGPERCENT; 087 if ("tax".equals(codeString)) 088 return TAX; 089 if ("benefit".equals(codeString)) 090 return BENEFIT; 091 throw new FHIRException("Unknown Adjudication code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case TOTAL: return "total"; 096 case COPAY: return "copay"; 097 case ELIGIBLE: return "eligible"; 098 case DEDUCTIBLE: return "deductible"; 099 case ELIGPERCENT: return "eligpercent"; 100 case TAX: return "tax"; 101 case BENEFIT: return "benefit"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 return "http://hl7.org/fhir/adjudication"; 108 } 109 public String getDefinition() { 110 switch (this) { 111 case TOTAL: return "Total submitted"; 112 case COPAY: return "Patient Co-Payment"; 113 case ELIGIBLE: return "Amount of the change which is considered for adjudication"; 114 case DEDUCTIBLE: return "Amount deducted from the eligible amount prior to adjudication"; 115 case ELIGPERCENT: return "Eligible Percentage"; 116 case TAX: return "Emergency Department"; 117 case BENEFIT: return "Amount payable under the coverage"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case TOTAL: return "Total"; 125 case COPAY: return "CoPay"; 126 case ELIGIBLE: return "Eligible Amount"; 127 case DEDUCTIBLE: return "Deductable"; 128 case ELIGPERCENT: return "Eligible %"; 129 case TAX: return "Emergency Department"; 130 case BENEFIT: return "Benefit Amount"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 136 137}