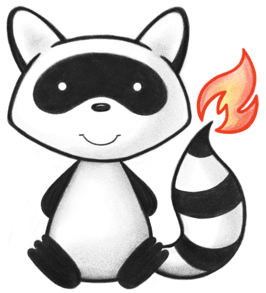
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum Appointmentstatus { 041 042 /** 043 * None of the participant(s) have finalized their acceptance of the appointment request, and the start/end time may not be set yet. 044 */ 045 PROPOSED, 046 /** 047 * Some or all of the participant(s) have not finalized their acceptance of the appointment request. 048 */ 049 PENDING, 050 /** 051 * All participant(s) have been considered and the appointment is confirmed to go ahead at the date/times specified. 052 */ 053 BOOKED, 054 /** 055 * Some of the patients have arrived. 056 */ 057 ARRIVED, 058 /** 059 * This appointment has completed and may have resulted in an encounter. 060 */ 061 FULFILLED, 062 /** 063 * The appointment has been cancelled. 064 */ 065 CANCELLED, 066 /** 067 * Some or all of the participant(s) have not/did not appear for the appointment (usually the patient). 068 */ 069 NOSHOW, 070 /** 071 * This instance should not have been part of this patient's medical record. 072 */ 073 ENTEREDINERROR, 074 /** 075 * added to help the parsers 076 */ 077 NULL; 078 public static Appointmentstatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("proposed".equals(codeString)) 082 return PROPOSED; 083 if ("pending".equals(codeString)) 084 return PENDING; 085 if ("booked".equals(codeString)) 086 return BOOKED; 087 if ("arrived".equals(codeString)) 088 return ARRIVED; 089 if ("fulfilled".equals(codeString)) 090 return FULFILLED; 091 if ("cancelled".equals(codeString)) 092 return CANCELLED; 093 if ("noshow".equals(codeString)) 094 return NOSHOW; 095 if ("entered-in-error".equals(codeString)) 096 return ENTEREDINERROR; 097 throw new FHIRException("Unknown Appointmentstatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case PROPOSED: return "proposed"; 102 case PENDING: return "pending"; 103 case BOOKED: return "booked"; 104 case ARRIVED: return "arrived"; 105 case FULFILLED: return "fulfilled"; 106 case CANCELLED: return "cancelled"; 107 case NOSHOW: return "noshow"; 108 case ENTEREDINERROR: return "entered-in-error"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getSystem() { 114 return "http://hl7.org/fhir/appointmentstatus"; 115 } 116 public String getDefinition() { 117 switch (this) { 118 case PROPOSED: return "None of the participant(s) have finalized their acceptance of the appointment request, and the start/end time may not be set yet."; 119 case PENDING: return "Some or all of the participant(s) have not finalized their acceptance of the appointment request."; 120 case BOOKED: return "All participant(s) have been considered and the appointment is confirmed to go ahead at the date/times specified."; 121 case ARRIVED: return "Some of the patients have arrived."; 122 case FULFILLED: return "This appointment has completed and may have resulted in an encounter."; 123 case CANCELLED: return "The appointment has been cancelled."; 124 case NOSHOW: return "Some or all of the participant(s) have not/did not appear for the appointment (usually the patient)."; 125 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case PROPOSED: return "Proposed"; 133 case PENDING: return "Pending"; 134 case BOOKED: return "Booked"; 135 case ARRIVED: return "Arrived"; 136 case FULFILLED: return "Fulfilled"; 137 case CANCELLED: return "Cancelled"; 138 case NOSHOW: return "No Show"; 139 case ENTEREDINERROR: return "Entered in error"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 145 146}