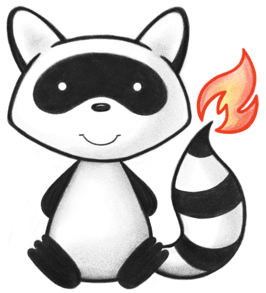
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum BasicResourceType { 041 042 /** 043 * An assertion of permission for an activity or set of activities to occur, possibly subject to particular limitations; e.g. surgical consent, information disclosure consent, etc. 044 */ 045 CONSENT, 046 /** 047 * A request that care of a particular type be provided to a patient. Could involve the transfer of care, a consult, etc. 048 */ 049 REFERRAL, 050 /** 051 * An undesired reaction caused by exposure to some agent (e.g. a medication, immunization, food, or environmental agent). 052 */ 053 ADVEVENT, 054 /** 055 * A request that a time be scheduled for a type of service for a specified patient, potentially subject to other constraints 056 */ 057 APTMTREQ, 058 /** 059 * The transition of a patient or set of material from one location to another 060 */ 061 TRANSFER, 062 /** 063 * The specification of a set of food and/or other nutritional material to be delivered to a patient. 064 */ 065 DIET, 066 /** 067 * An occurrence of a non-care-related event in the healthcare domain, such as approvals, reviews, etc. 068 */ 069 ADMINACT, 070 /** 071 * Record of a situation where a subject was exposed to a substance. Usually of interest to public health. 072 */ 073 EXPOSURE, 074 /** 075 * A formalized inquiry into the circumstances surrounding a particular unplanned event or potential event for the purposes of identifying possible causes and contributing factors for the event 076 */ 077 INVESTIGATION, 078 /** 079 * A financial instrument used to track costs, charges or other amounts. 080 */ 081 ACCOUNT, 082 /** 083 * A request for payment for goods and/or services. Includes the idea of a healthcare insurance claim. 084 */ 085 INVOICE, 086 /** 087 * The determination of what will be paid against a particular invoice based on coverage, plan rules, etc. 088 */ 089 ADJUDICAT, 090 /** 091 * A request for a pre-determination of the cost that would be paid under an insurance plan for a hypothetical claim for goods or services 092 */ 093 PREDETREQ, 094 /** 095 * An adjudication of what would be paid under an insurance plan for a hypothetical claim for goods or services 096 */ 097 PREDETERMINE, 098 /** 099 * An investigation to determine information about a particular therapy or product 100 */ 101 STUDY, 102 /** 103 * A set of (possibly conditional) steps to be taken to achieve some aim. Includes study protocols, treatment protocols, emergency protocols, etc. 104 */ 105 PROTOCOL, 106 /** 107 * added to help the parsers 108 */ 109 NULL; 110 public static BasicResourceType fromCode(String codeString) throws FHIRException { 111 if (codeString == null || "".equals(codeString)) 112 return null; 113 if ("consent".equals(codeString)) 114 return CONSENT; 115 if ("referral".equals(codeString)) 116 return REFERRAL; 117 if ("advevent".equals(codeString)) 118 return ADVEVENT; 119 if ("aptmtreq".equals(codeString)) 120 return APTMTREQ; 121 if ("transfer".equals(codeString)) 122 return TRANSFER; 123 if ("diet".equals(codeString)) 124 return DIET; 125 if ("adminact".equals(codeString)) 126 return ADMINACT; 127 if ("exposure".equals(codeString)) 128 return EXPOSURE; 129 if ("investigation".equals(codeString)) 130 return INVESTIGATION; 131 if ("account".equals(codeString)) 132 return ACCOUNT; 133 if ("invoice".equals(codeString)) 134 return INVOICE; 135 if ("adjudicat".equals(codeString)) 136 return ADJUDICAT; 137 if ("predetreq".equals(codeString)) 138 return PREDETREQ; 139 if ("predetermine".equals(codeString)) 140 return PREDETERMINE; 141 if ("study".equals(codeString)) 142 return STUDY; 143 if ("protocol".equals(codeString)) 144 return PROTOCOL; 145 throw new FHIRException("Unknown BasicResourceType code '"+codeString+"'"); 146 } 147 public String toCode() { 148 switch (this) { 149 case CONSENT: return "consent"; 150 case REFERRAL: return "referral"; 151 case ADVEVENT: return "advevent"; 152 case APTMTREQ: return "aptmtreq"; 153 case TRANSFER: return "transfer"; 154 case DIET: return "diet"; 155 case ADMINACT: return "adminact"; 156 case EXPOSURE: return "exposure"; 157 case INVESTIGATION: return "investigation"; 158 case ACCOUNT: return "account"; 159 case INVOICE: return "invoice"; 160 case ADJUDICAT: return "adjudicat"; 161 case PREDETREQ: return "predetreq"; 162 case PREDETERMINE: return "predetermine"; 163 case STUDY: return "study"; 164 case PROTOCOL: return "protocol"; 165 case NULL: return null; 166 default: return "?"; 167 } 168 } 169 public String getSystem() { 170 return "http://hl7.org/fhir/basic-resource-type"; 171 } 172 public String getDefinition() { 173 switch (this) { 174 case CONSENT: return "An assertion of permission for an activity or set of activities to occur, possibly subject to particular limitations; e.g. surgical consent, information disclosure consent, etc."; 175 case REFERRAL: return "A request that care of a particular type be provided to a patient. Could involve the transfer of care, a consult, etc."; 176 case ADVEVENT: return "An undesired reaction caused by exposure to some agent (e.g. a medication, immunization, food, or environmental agent)."; 177 case APTMTREQ: return "A request that a time be scheduled for a type of service for a specified patient, potentially subject to other constraints"; 178 case TRANSFER: return "The transition of a patient or set of material from one location to another"; 179 case DIET: return "The specification of a set of food and/or other nutritional material to be delivered to a patient."; 180 case ADMINACT: return "An occurrence of a non-care-related event in the healthcare domain, such as approvals, reviews, etc."; 181 case EXPOSURE: return "Record of a situation where a subject was exposed to a substance. Usually of interest to public health."; 182 case INVESTIGATION: return "A formalized inquiry into the circumstances surrounding a particular unplanned event or potential event for the purposes of identifying possible causes and contributing factors for the event"; 183 case ACCOUNT: return "A financial instrument used to track costs, charges or other amounts."; 184 case INVOICE: return "A request for payment for goods and/or services. Includes the idea of a healthcare insurance claim."; 185 case ADJUDICAT: return "The determination of what will be paid against a particular invoice based on coverage, plan rules, etc."; 186 case PREDETREQ: return "A request for a pre-determination of the cost that would be paid under an insurance plan for a hypothetical claim for goods or services"; 187 case PREDETERMINE: return "An adjudication of what would be paid under an insurance plan for a hypothetical claim for goods or services"; 188 case STUDY: return "An investigation to determine information about a particular therapy or product"; 189 case PROTOCOL: return "A set of (possibly conditional) steps to be taken to achieve some aim. Includes study protocols, treatment protocols, emergency protocols, etc."; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getDisplay() { 195 switch (this) { 196 case CONSENT: return "Consent"; 197 case REFERRAL: return "Referral"; 198 case ADVEVENT: return "Adverse Event"; 199 case APTMTREQ: return "Appointment Request"; 200 case TRANSFER: return "Transfer"; 201 case DIET: return "Diet"; 202 case ADMINACT: return "Administrative Activity"; 203 case EXPOSURE: return "Exposure"; 204 case INVESTIGATION: return "Investigation"; 205 case ACCOUNT: return "Account"; 206 case INVOICE: return "Invoice"; 207 case ADJUDICAT: return "Invoice Adjudication"; 208 case PREDETREQ: return "Pre-determination Request"; 209 case PREDETERMINE: return "Predetermination"; 210 case STUDY: return "Study"; 211 case PROTOCOL: return "Protocol"; 212 case NULL: return null; 213 default: return "?"; 214 } 215 } 216 217 218}