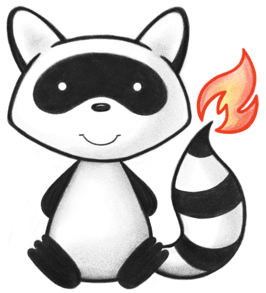
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum BenefitSubcategory { 041 042 /** 043 * Medical Care. 044 */ 045 _1, 046 /** 047 * Surgical. 048 */ 049 _2, 050 /** 051 * Consultation. 052 */ 053 _3, 054 /** 055 * Diagnostic XRay. 056 */ 057 _4, 058 /** 059 * Diagnostic Lab. 060 */ 061 _5, 062 /** 063 * Renal Supplies excluding Dialysis. 064 */ 065 _14, 066 /** 067 * Diagnostic Dental. 068 */ 069 _23, 070 /** 071 * Periodontics. 072 */ 073 _24, 074 /** 075 * Restorative. 076 */ 077 _25, 078 /** 079 * Endodontics. 080 */ 081 _26, 082 /** 083 * Maxillofacilial Prosthetics. 084 */ 085 _27, 086 /** 087 * Adjunctive Dental Services. 088 */ 089 _28, 090 /** 091 * Health Benefit Plan Coverage. 092 */ 093 _30, 094 /** 095 * Dental Care. 096 */ 097 _35, 098 /** 099 * Dental Crowns. 100 */ 101 _36, 102 /** 103 * Dental Accident. 104 */ 105 _37, 106 /** 107 * Hospital Room and Board. 108 */ 109 _49, 110 /** 111 * Major Medical. 112 */ 113 _55, 114 /** 115 * Medically Related Transportation. 116 */ 117 _56, 118 /** 119 * In-vitro Fertilization. 120 */ 121 _61, 122 /** 123 * MRI Scan. 124 */ 125 _62, 126 /** 127 * Donor Procedures such as organ harvest. 128 */ 129 _63, 130 /** 131 * Maternity. 132 */ 133 _69, 134 /** 135 * Renal dialysis. 136 */ 137 _76, 138 /** 139 * Medical Coverage. 140 */ 141 F1, 142 /** 143 * Dental Coverage. 144 */ 145 F3, 146 /** 147 * Hearing Coverage. 148 */ 149 F4, 150 /** 151 * Vision Coverage. 152 */ 153 F6, 154 /** 155 * added to help the parsers 156 */ 157 NULL; 158 public static BenefitSubcategory fromCode(String codeString) throws FHIRException { 159 if (codeString == null || "".equals(codeString)) 160 return null; 161 if ("1".equals(codeString)) 162 return _1; 163 if ("2".equals(codeString)) 164 return _2; 165 if ("3".equals(codeString)) 166 return _3; 167 if ("4".equals(codeString)) 168 return _4; 169 if ("5".equals(codeString)) 170 return _5; 171 if ("14".equals(codeString)) 172 return _14; 173 if ("23".equals(codeString)) 174 return _23; 175 if ("24".equals(codeString)) 176 return _24; 177 if ("25".equals(codeString)) 178 return _25; 179 if ("26".equals(codeString)) 180 return _26; 181 if ("27".equals(codeString)) 182 return _27; 183 if ("28".equals(codeString)) 184 return _28; 185 if ("30".equals(codeString)) 186 return _30; 187 if ("35".equals(codeString)) 188 return _35; 189 if ("36".equals(codeString)) 190 return _36; 191 if ("37".equals(codeString)) 192 return _37; 193 if ("49".equals(codeString)) 194 return _49; 195 if ("55".equals(codeString)) 196 return _55; 197 if ("56".equals(codeString)) 198 return _56; 199 if ("61".equals(codeString)) 200 return _61; 201 if ("62".equals(codeString)) 202 return _62; 203 if ("63".equals(codeString)) 204 return _63; 205 if ("69".equals(codeString)) 206 return _69; 207 if ("76".equals(codeString)) 208 return _76; 209 if ("F1".equals(codeString)) 210 return F1; 211 if ("F3".equals(codeString)) 212 return F3; 213 if ("F4".equals(codeString)) 214 return F4; 215 if ("F6".equals(codeString)) 216 return F6; 217 throw new FHIRException("Unknown BenefitSubcategory code '"+codeString+"'"); 218 } 219 public String toCode() { 220 switch (this) { 221 case _1: return "1"; 222 case _2: return "2"; 223 case _3: return "3"; 224 case _4: return "4"; 225 case _5: return "5"; 226 case _14: return "14"; 227 case _23: return "23"; 228 case _24: return "24"; 229 case _25: return "25"; 230 case _26: return "26"; 231 case _27: return "27"; 232 case _28: return "28"; 233 case _30: return "30"; 234 case _35: return "35"; 235 case _36: return "36"; 236 case _37: return "37"; 237 case _49: return "49"; 238 case _55: return "55"; 239 case _56: return "56"; 240 case _61: return "61"; 241 case _62: return "62"; 242 case _63: return "63"; 243 case _69: return "69"; 244 case _76: return "76"; 245 case F1: return "F1"; 246 case F3: return "F3"; 247 case F4: return "F4"; 248 case F6: return "F6"; 249 case NULL: return null; 250 default: return "?"; 251 } 252 } 253 public String getSystem() { 254 return "http://hl7.org/fhir/benefit-subcategory"; 255 } 256 public String getDefinition() { 257 switch (this) { 258 case _1: return "Medical Care."; 259 case _2: return "Surgical."; 260 case _3: return "Consultation."; 261 case _4: return "Diagnostic XRay."; 262 case _5: return "Diagnostic Lab."; 263 case _14: return "Renal Supplies excluding Dialysis."; 264 case _23: return "Diagnostic Dental."; 265 case _24: return "Periodontics."; 266 case _25: return "Restorative."; 267 case _26: return "Endodontics."; 268 case _27: return "Maxillofacilial Prosthetics."; 269 case _28: return "Adjunctive Dental Services."; 270 case _30: return "Health Benefit Plan Coverage."; 271 case _35: return "Dental Care."; 272 case _36: return "Dental Crowns."; 273 case _37: return "Dental Accident."; 274 case _49: return "Hospital Room and Board."; 275 case _55: return "Major Medical."; 276 case _56: return "Medically Related Transportation."; 277 case _61: return "In-vitro Fertilization."; 278 case _62: return "MRI Scan."; 279 case _63: return "Donor Procedures such as organ harvest."; 280 case _69: return "Maternity."; 281 case _76: return "Renal dialysis."; 282 case F1: return "Medical Coverage."; 283 case F3: return "Dental Coverage."; 284 case F4: return "Hearing Coverage."; 285 case F6: return "Vision Coverage."; 286 case NULL: return null; 287 default: return "?"; 288 } 289 } 290 public String getDisplay() { 291 switch (this) { 292 case _1: return "Medical Care"; 293 case _2: return "Surgical"; 294 case _3: return "Consultation"; 295 case _4: return "Diagnostic XRay"; 296 case _5: return "Diagnostic Lab"; 297 case _14: return "Renal Supplies"; 298 case _23: return "Diagnostic Dental"; 299 case _24: return "Periodontics"; 300 case _25: return "Restorative"; 301 case _26: return "Endodontics"; 302 case _27: return "Maxillofacilial Prosthetics"; 303 case _28: return "Adjunctive Dental Services"; 304 case _30: return "Health Benefit Plan Coverage"; 305 case _35: return "Dental Care"; 306 case _36: return "Dental Crowns"; 307 case _37: return "Dental Accident"; 308 case _49: return "Hospital Room and Board"; 309 case _55: return "Major Medical"; 310 case _56: return "Medically Related Transportation"; 311 case _61: return "In-vitro Fertilization"; 312 case _62: return "MRI Scan"; 313 case _63: return "Donor Procedures"; 314 case _69: return "Maternity"; 315 case _76: return "Renal Dialysis"; 316 case F1: return "Medical Coverage"; 317 case F3: return "Dental Coverage"; 318 case F4: return "Hearing Coverage"; 319 case F6: return "Vision Coverage"; 320 case NULL: return null; 321 default: return "?"; 322 } 323 } 324 325 326}