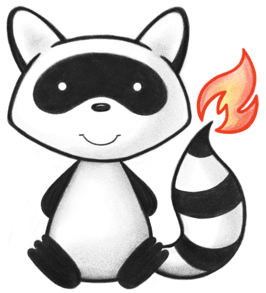
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum CarePlanActivityStatus { 041 042 /** 043 * Activity is planned but no action has yet been taken. 044 */ 045 NOTSTARTED, 046 /** 047 * Appointment or other booking has occurred but activity has not yet begun. 048 */ 049 SCHEDULED, 050 /** 051 * Activity has been started but is not yet complete. 052 */ 053 INPROGRESS, 054 /** 055 * Activity was started but has temporarily ceased with an expectation of resumption at a future time. 056 */ 057 ONHOLD, 058 /** 059 * The activities have been completed (more or less) as planned. 060 */ 061 COMPLETED, 062 /** 063 * The activities have been ended prior to completion (perhaps even before they were started). 064 */ 065 CANCELLED, 066 /** 067 * The authoring system doesn't know the current state of the activity. 068 */ 069 UNKNOWN, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 public static CarePlanActivityStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("not-started".equals(codeString)) 078 return NOTSTARTED; 079 if ("scheduled".equals(codeString)) 080 return SCHEDULED; 081 if ("in-progress".equals(codeString)) 082 return INPROGRESS; 083 if ("on-hold".equals(codeString)) 084 return ONHOLD; 085 if ("completed".equals(codeString)) 086 return COMPLETED; 087 if ("cancelled".equals(codeString)) 088 return CANCELLED; 089 if ("unknown".equals(codeString)) 090 return UNKNOWN; 091 throw new FHIRException("Unknown CarePlanActivityStatus code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case NOTSTARTED: return "not-started"; 096 case SCHEDULED: return "scheduled"; 097 case INPROGRESS: return "in-progress"; 098 case ONHOLD: return "on-hold"; 099 case COMPLETED: return "completed"; 100 case CANCELLED: return "cancelled"; 101 case UNKNOWN: return "unknown"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 return "http://hl7.org/fhir/care-plan-activity-status"; 108 } 109 public String getDefinition() { 110 switch (this) { 111 case NOTSTARTED: return "Activity is planned but no action has yet been taken."; 112 case SCHEDULED: return "Appointment or other booking has occurred but activity has not yet begun."; 113 case INPROGRESS: return "Activity has been started but is not yet complete."; 114 case ONHOLD: return "Activity was started but has temporarily ceased with an expectation of resumption at a future time."; 115 case COMPLETED: return "The activities have been completed (more or less) as planned."; 116 case CANCELLED: return "The activities have been ended prior to completion (perhaps even before they were started)."; 117 case UNKNOWN: return "The authoring system doesn't know the current state of the activity."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case NOTSTARTED: return "Not Started"; 125 case SCHEDULED: return "Scheduled"; 126 case INPROGRESS: return "In Progress"; 127 case ONHOLD: return "On Hold"; 128 case COMPLETED: return "Completed"; 129 case CANCELLED: return "Cancelled"; 130 case UNKNOWN: return "Unknown"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 136 137}