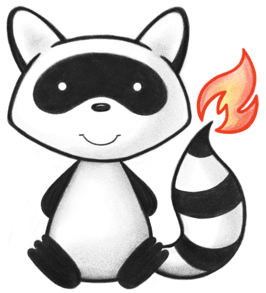
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum CarePlanIntent { 041 042 /** 043 * The care plan is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 044 */ 045 PROPOSAL, 046 /** 047 * The care plan represents an intention to ensure something occurs without providing an authorization for others to act 048 */ 049 PLAN, 050 /** 051 * The care plan represents a request/demand and authorization for action 052 */ 053 ORDER, 054 /** 055 * The care plan represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. 056 057Refer to [[[RequestGroup]]] for additional information on how this status is used 058 */ 059 OPTION, 060 /** 061 * added to help the parsers 062 */ 063 NULL; 064 public static CarePlanIntent fromCode(String codeString) throws FHIRException { 065 if (codeString == null || "".equals(codeString)) 066 return null; 067 if ("proposal".equals(codeString)) 068 return PROPOSAL; 069 if ("plan".equals(codeString)) 070 return PLAN; 071 if ("order".equals(codeString)) 072 return ORDER; 073 if ("option".equals(codeString)) 074 return OPTION; 075 throw new FHIRException("Unknown CarePlanIntent code '"+codeString+"'"); 076 } 077 public String toCode() { 078 switch (this) { 079 case PROPOSAL: return "proposal"; 080 case PLAN: return "plan"; 081 case ORDER: return "order"; 082 case OPTION: return "option"; 083 case NULL: return null; 084 default: return "?"; 085 } 086 } 087 public String getSystem() { 088 return "http://hl7.org/fhir/care-plan-intent"; 089 } 090 public String getDefinition() { 091 switch (this) { 092 case PROPOSAL: return "The care plan is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 093 case PLAN: return "The care plan represents an intention to ensure something occurs without providing an authorization for others to act"; 094 case ORDER: return "The care plan represents a request/demand and authorization for action"; 095 case OPTION: return "The care plan represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests.\n\nRefer to [[[RequestGroup]]] for additional information on how this status is used"; 096 case NULL: return null; 097 default: return "?"; 098 } 099 } 100 public String getDisplay() { 101 switch (this) { 102 case PROPOSAL: return "Proposal"; 103 case PLAN: return "Plan"; 104 case ORDER: return "Order"; 105 case OPTION: return "Option"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 111 112}