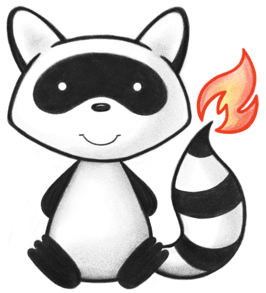
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum CarePlanStatus { 041 042 /** 043 * The plan is in development or awaiting use but is not yet intended to be acted upon. 044 */ 045 DRAFT, 046 /** 047 * The plan is intended to be followed and used as part of patient care. 048 */ 049 ACTIVE, 050 /** 051 * The plan has been temporarily stopped but is expected to resume in the future. 052 */ 053 SUSPENDED, 054 /** 055 * The plan is no longer in use and is not expected to be followed or used in patient care. 056 */ 057 COMPLETED, 058 /** 059 * The plan was entered in error and voided. 060 */ 061 ENTEREDINERROR, 062 /** 063 * The plan has been terminated prior to reaching completion (though it may have been replaced by a new plan). 064 */ 065 CANCELLED, 066 /** 067 * The authoring system doesn't know the current state of the care plan. 068 */ 069 UNKNOWN, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 public static CarePlanStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("draft".equals(codeString)) 078 return DRAFT; 079 if ("active".equals(codeString)) 080 return ACTIVE; 081 if ("suspended".equals(codeString)) 082 return SUSPENDED; 083 if ("completed".equals(codeString)) 084 return COMPLETED; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if ("cancelled".equals(codeString)) 088 return CANCELLED; 089 if ("unknown".equals(codeString)) 090 return UNKNOWN; 091 throw new FHIRException("Unknown CarePlanStatus code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case DRAFT: return "draft"; 096 case ACTIVE: return "active"; 097 case SUSPENDED: return "suspended"; 098 case COMPLETED: return "completed"; 099 case ENTEREDINERROR: return "entered-in-error"; 100 case CANCELLED: return "cancelled"; 101 case UNKNOWN: return "unknown"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 return "http://hl7.org/fhir/care-plan-status"; 108 } 109 public String getDefinition() { 110 switch (this) { 111 case DRAFT: return "The plan is in development or awaiting use but is not yet intended to be acted upon."; 112 case ACTIVE: return "The plan is intended to be followed and used as part of patient care."; 113 case SUSPENDED: return "The plan has been temporarily stopped but is expected to resume in the future."; 114 case COMPLETED: return "The plan is no longer in use and is not expected to be followed or used in patient care."; 115 case ENTEREDINERROR: return "The plan was entered in error and voided."; 116 case CANCELLED: return "The plan has been terminated prior to reaching completion (though it may have been replaced by a new plan)."; 117 case UNKNOWN: return "The authoring system doesn't know the current state of the care plan."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case DRAFT: return "Pending"; 125 case ACTIVE: return "Active"; 126 case SUSPENDED: return "Suspended"; 127 case COMPLETED: return "Completed"; 128 case ENTEREDINERROR: return "Entered In Error"; 129 case CANCELLED: return "Cancelled"; 130 case UNKNOWN: return "Unknown"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 136 137}