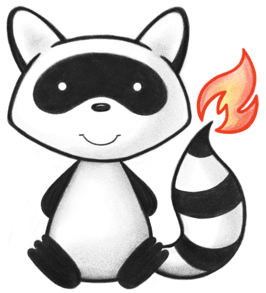
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ChargeitemStatus { 041 042 /** 043 * The charge item has been entered, but the charged service is not yet complete, so it shall not be billed yet but might be used in the context of pre-authorization 044 */ 045 PLANNED, 046 /** 047 * The charge item is ready for billing 048 */ 049 BILLABLE, 050 /** 051 * The charge item has been determined to be not billable (e.g. due to rules associated with the billing code) 052 */ 053 NOTBILLABLE, 054 /** 055 * The processing of the charge was aborted 056 */ 057 ABORTED, 058 /** 059 * The charge item has been billed (e.g. a billing engine has generated financial transactions by applying the associated ruled for the charge item to the context of the Encounter, and placed them into Claims/Invoices 060 */ 061 BILLED, 062 /** 063 * The charge item has been entered in error and should not be processed for billing 064 */ 065 ENTEREDINERROR, 066 /** 067 * The authoring system does not know which of the status values currently applies for this charge item Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 068 */ 069 UNKNOWN, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 public static ChargeitemStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("planned".equals(codeString)) 078 return PLANNED; 079 if ("billable".equals(codeString)) 080 return BILLABLE; 081 if ("not-billable".equals(codeString)) 082 return NOTBILLABLE; 083 if ("aborted".equals(codeString)) 084 return ABORTED; 085 if ("billed".equals(codeString)) 086 return BILLED; 087 if ("entered-in-error".equals(codeString)) 088 return ENTEREDINERROR; 089 if ("unknown".equals(codeString)) 090 return UNKNOWN; 091 throw new FHIRException("Unknown ChargeitemStatus code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case PLANNED: return "planned"; 096 case BILLABLE: return "billable"; 097 case NOTBILLABLE: return "not-billable"; 098 case ABORTED: return "aborted"; 099 case BILLED: return "billed"; 100 case ENTEREDINERROR: return "entered-in-error"; 101 case UNKNOWN: return "unknown"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 return "http://hl7.org/fhir/chargeitem-status"; 108 } 109 public String getDefinition() { 110 switch (this) { 111 case PLANNED: return "The charge item has been entered, but the charged service is not yet complete, so it shall not be billed yet but might be used in the context of pre-authorization"; 112 case BILLABLE: return "The charge item is ready for billing"; 113 case NOTBILLABLE: return "The charge item has been determined to be not billable (e.g. due to rules associated with the billing code)"; 114 case ABORTED: return "The processing of the charge was aborted"; 115 case BILLED: return "The charge item has been billed (e.g. a billing engine has generated financial transactions by applying the associated ruled for the charge item to the context of the Encounter, and placed them into Claims/Invoices"; 116 case ENTEREDINERROR: return "The charge item has been entered in error and should not be processed for billing"; 117 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this charge item Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case PLANNED: return "Planned"; 125 case BILLABLE: return "Billable"; 126 case NOTBILLABLE: return "Not billable"; 127 case ABORTED: return "Aborted"; 128 case BILLED: return "Billed"; 129 case ENTEREDINERROR: return "Entered in Error"; 130 case UNKNOWN: return "Unknown"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 136 137}