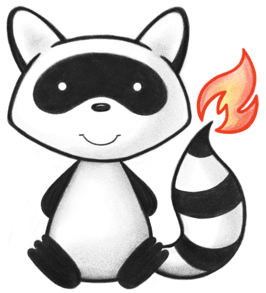
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ContractStatus { 041 042 /** 043 * Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced. 044 */ 045 AMENDED, 046 /** 047 * Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced. 048 */ 049 APPENDED, 050 /** 051 * Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted. 052 */ 053 CANCELLED, 054 /** 055 * Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7.Comparable FHIR and v.3 status codes: on hold; pended; suspended. 056 */ 057 DISPUTED, 058 /** 059 * Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status. 060 */ 061 ENTEREDINERROR, 062 /** 063 * Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active. 064 */ 065 EXECUTABLE, 066 /** 067 * Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed. 068 */ 069 EXECUTED, 070 /** 071 * Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held. 072 */ 073 NEGOTIABLE, 074 /** 075 * Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template','form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new. 076 */ 077 OFFERED, 078 /** 079 * Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended. 080 */ 081 POLICY, 082 /** 083 * Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled. 084 */ 085 REJECTED, 086 /** 087 * Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded. 088 */ 089 RENEWED, 090 /** 091 * A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified. 092 */ 093 REVOKED, 094 /** 095 * Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated. 096 */ 097 RESOLVED, 098 /** 099 * Contract reaches its expiry date. It may or may not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted. 100 */ 101 TERMINATED, 102 /** 103 * added to help the parsers 104 */ 105 NULL; 106 public static ContractStatus fromCode(String codeString) throws FHIRException { 107 if (codeString == null || "".equals(codeString)) 108 return null; 109 if ("amended".equals(codeString)) 110 return AMENDED; 111 if ("appended".equals(codeString)) 112 return APPENDED; 113 if ("cancelled".equals(codeString)) 114 return CANCELLED; 115 if ("disputed".equals(codeString)) 116 return DISPUTED; 117 if ("entered-in-error".equals(codeString)) 118 return ENTEREDINERROR; 119 if ("executable".equals(codeString)) 120 return EXECUTABLE; 121 if ("executed".equals(codeString)) 122 return EXECUTED; 123 if ("negotiable".equals(codeString)) 124 return NEGOTIABLE; 125 if ("offered".equals(codeString)) 126 return OFFERED; 127 if ("policy".equals(codeString)) 128 return POLICY; 129 if ("rejected".equals(codeString)) 130 return REJECTED; 131 if ("renewed".equals(codeString)) 132 return RENEWED; 133 if ("revoked".equals(codeString)) 134 return REVOKED; 135 if ("resolved".equals(codeString)) 136 return RESOLVED; 137 if ("terminated".equals(codeString)) 138 return TERMINATED; 139 throw new FHIRException("Unknown ContractStatus code '"+codeString+"'"); 140 } 141 public String toCode() { 142 switch (this) { 143 case AMENDED: return "amended"; 144 case APPENDED: return "appended"; 145 case CANCELLED: return "cancelled"; 146 case DISPUTED: return "disputed"; 147 case ENTEREDINERROR: return "entered-in-error"; 148 case EXECUTABLE: return "executable"; 149 case EXECUTED: return "executed"; 150 case NEGOTIABLE: return "negotiable"; 151 case OFFERED: return "offered"; 152 case POLICY: return "policy"; 153 case REJECTED: return "rejected"; 154 case RENEWED: return "renewed"; 155 case REVOKED: return "revoked"; 156 case RESOLVED: return "resolved"; 157 case TERMINATED: return "terminated"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getSystem() { 163 return "http://hl7.org/fhir/contract-status"; 164 } 165 public String getDefinition() { 166 switch (this) { 167 case AMENDED: return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced."; 168 case APPENDED: return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced."; 169 case CANCELLED: return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted."; 170 case DISPUTED: return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7.Comparable FHIR and v.3 status codes: on hold; pended; suspended."; 171 case ENTEREDINERROR: return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status."; 172 case EXECUTABLE: return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active."; 173 case EXECUTED: return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed."; 174 case NEGOTIABLE: return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held."; 175 case OFFERED: return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template','form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new."; 176 case POLICY: return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended."; 177 case REJECTED: return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled."; 178 case RENEWED: return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded."; 179 case REVOKED: return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified."; 180 case RESOLVED: return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated."; 181 case TERMINATED: return "Contract reaches its expiry date. It may or may not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted."; 182 case NULL: return null; 183 default: return "?"; 184 } 185 } 186 public String getDisplay() { 187 switch (this) { 188 case AMENDED: return "Amended"; 189 case APPENDED: return "Appended"; 190 case CANCELLED: return "Cancelled"; 191 case DISPUTED: return "Disputed"; 192 case ENTEREDINERROR: return "Entered in Error"; 193 case EXECUTABLE: return "Executable"; 194 case EXECUTED: return "Executed"; 195 case NEGOTIABLE: return "Negotiable"; 196 case OFFERED: return "Offered"; 197 case POLICY: return "Policy"; 198 case REJECTED: return "Rejected"; 199 case RENEWED: return "Renewed"; 200 case REVOKED: return "Revoked"; 201 case RESOLVED: return "Resolved"; 202 case TERMINATED: return "Terminated"; 203 case NULL: return null; 204 default: return "?"; 205 } 206 } 207 208 209}