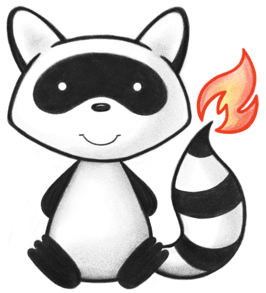
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum DataelementStringency { 041 042 /** 043 * The data element is sufficiently well-constrained that multiple pieces of data captured according to the constraints of the data element will be comparable (though in some cases, a degree of automated conversion/normalization may be required). 044 */ 045 COMPARABLE, 046 /** 047 * The data element is fully specified down to a single value set, single unit of measure, single data type, etc. Multiple pieces of data associated with this data element are fully comparable. 048 */ 049 FULLYSPECIFIED, 050 /** 051 * The data element allows multiple units of measure having equivalent meaning; e.g. "cc" (cubic centimeter) and "mL" (milliliter). 052 */ 053 EQUIVALENT, 054 /** 055 * The data element allows multiple units of measure that are convertable between each other (e.g. inches and centimeters) and/or allows data to be captured in multiple value sets for which a known mapping exists allowing conversion of meaning. 056 */ 057 CONVERTABLE, 058 /** 059 * A convertable data element where unit conversions are different only by a power of 10; e.g. g, mg, kg. 060 */ 061 SCALEABLE, 062 /** 063 * The data element is unconstrained in units, choice of data types and/or choice of vocabulary such that automated comparison of data captured using the data element is not possible. 064 */ 065 FLEXIBLE, 066 /** 067 * added to help the parsers 068 */ 069 NULL; 070 public static DataelementStringency fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("comparable".equals(codeString)) 074 return COMPARABLE; 075 if ("fully-specified".equals(codeString)) 076 return FULLYSPECIFIED; 077 if ("equivalent".equals(codeString)) 078 return EQUIVALENT; 079 if ("convertable".equals(codeString)) 080 return CONVERTABLE; 081 if ("scaleable".equals(codeString)) 082 return SCALEABLE; 083 if ("flexible".equals(codeString)) 084 return FLEXIBLE; 085 throw new FHIRException("Unknown DataelementStringency code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case COMPARABLE: return "comparable"; 090 case FULLYSPECIFIED: return "fully-specified"; 091 case EQUIVALENT: return "equivalent"; 092 case CONVERTABLE: return "convertable"; 093 case SCALEABLE: return "scaleable"; 094 case FLEXIBLE: return "flexible"; 095 case NULL: return null; 096 default: return "?"; 097 } 098 } 099 public String getSystem() { 100 return "http://hl7.org/fhir/dataelement-stringency"; 101 } 102 public String getDefinition() { 103 switch (this) { 104 case COMPARABLE: return "The data element is sufficiently well-constrained that multiple pieces of data captured according to the constraints of the data element will be comparable (though in some cases, a degree of automated conversion/normalization may be required)."; 105 case FULLYSPECIFIED: return "The data element is fully specified down to a single value set, single unit of measure, single data type, etc. Multiple pieces of data associated with this data element are fully comparable."; 106 case EQUIVALENT: return "The data element allows multiple units of measure having equivalent meaning; e.g. \"cc\" (cubic centimeter) and \"mL\" (milliliter)."; 107 case CONVERTABLE: return "The data element allows multiple units of measure that are convertable between each other (e.g. inches and centimeters) and/or allows data to be captured in multiple value sets for which a known mapping exists allowing conversion of meaning."; 108 case SCALEABLE: return "A convertable data element where unit conversions are different only by a power of 10; e.g. g, mg, kg."; 109 case FLEXIBLE: return "The data element is unconstrained in units, choice of data types and/or choice of vocabulary such that automated comparison of data captured using the data element is not possible."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case COMPARABLE: return "Comparable"; 117 case FULLYSPECIFIED: return "Fully Specified"; 118 case EQUIVALENT: return "Equivalent"; 119 case CONVERTABLE: return "Convertable"; 120 case SCALEABLE: return "Scaleable"; 121 case FLEXIBLE: return "Flexible"; 122 case NULL: return null; 123 default: return "?"; 124 } 125 } 126 127 128}