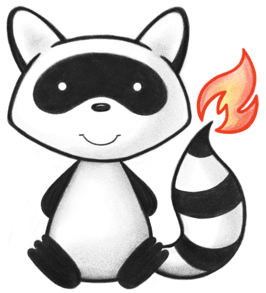
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Thu, Feb 9, 2017 08:03-0500 for FHIR v1.9.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum DeviceSafety { 041 042 /** 043 * Indicates that the device or packaging contains natural rubber that contacts humans 044 */ 045 CONTAINSLATEX, 046 /** 047 * Indicates that natural rubber latex was not used as materials in the manufacture of the medical product and container and the device labeling contains this information. 048 */ 049 LATEXFREE, 050 /** 051 * Indicates that whether the device or packaging contains natural rubber that contacts humans is not indicated on the label Not all medical products that are NOT made with natural rubber latex will be marked 052 */ 053 LATEXUNKNOWN, 054 /** 055 * The device, when used in the MRI environment, has been demonstrated to present no additional risk to the patient or other individual, but may affect the quality of the diagnostic information. The MRI conditions in which the device was tested should be specified in conjunction with the term MR safe since a device which is safe under one set of conditions may not be found to be so under more extreme MRI conditions. 056 */ 057 MRSAFE, 058 /** 059 * An item that is known to pose hazards in all MRI environments. MR Unsafe items include magnetic items such as a pair of ferromagnetic scissors. 060 */ 061 MRUNSAFE, 062 /** 063 * An item that has been demonstrated to pose no known hazards in a specified MRI environment with specified conditions of use. Field conditions that define the MRI environment include, for instance, static magnetic field or specific absorption rate (SAR). 064 */ 065 MRCONDITIONAL, 066 /** 067 * Labeling does not contain MRI Safety information 068 */ 069 MRUNKNOWN, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 public static DeviceSafety fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("contains-latex".equals(codeString)) 078 return CONTAINSLATEX; 079 if ("latex-free".equals(codeString)) 080 return LATEXFREE; 081 if ("latex-unknown".equals(codeString)) 082 return LATEXUNKNOWN; 083 if ("mr-safe".equals(codeString)) 084 return MRSAFE; 085 if ("mr-unsafe".equals(codeString)) 086 return MRUNSAFE; 087 if ("mr-conditional".equals(codeString)) 088 return MRCONDITIONAL; 089 if ("mr-unknown".equals(codeString)) 090 return MRUNKNOWN; 091 throw new FHIRException("Unknown DeviceSafety code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case CONTAINSLATEX: return "contains-latex"; 096 case LATEXFREE: return "latex-free"; 097 case LATEXUNKNOWN: return "latex-unknown"; 098 case MRSAFE: return "mr-safe"; 099 case MRUNSAFE: return "mr-unsafe"; 100 case MRCONDITIONAL: return "mr-conditional"; 101 case MRUNKNOWN: return "mr-unknown"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 return "http://hl7.org/fhir/device-safety"; 108 } 109 public String getDefinition() { 110 switch (this) { 111 case CONTAINSLATEX: return "Indicates that the device or packaging contains natural rubber that contacts humans"; 112 case LATEXFREE: return "Indicates that natural rubber latex was not used as materials in the manufacture of the medical product and container and the device labeling contains this information."; 113 case LATEXUNKNOWN: return "Indicates that whether the device or packaging contains natural rubber that contacts humans is not indicated on the label Not all medical products that are NOT made with natural rubber latex will be marked"; 114 case MRSAFE: return "The device, when used in the MRI environment, has been demonstrated to present no additional risk to the patient or other individual, but may affect the quality of the diagnostic information. The MRI conditions in which the device was tested should be specified in conjunction with the term MR safe since a device which is safe under one set of conditions may not be found to be so under more extreme MRI conditions."; 115 case MRUNSAFE: return "An item that is known to pose hazards in all MRI environments. MR Unsafe items include magnetic items such as a pair of ferromagnetic scissors."; 116 case MRCONDITIONAL: return "An item that has been demonstrated to pose no known hazards in a specified MRI environment with specified conditions of use. Field conditions that define the MRI environment include, for instance, static magnetic field or specific absorption rate (SAR)."; 117 case MRUNKNOWN: return "Labeling does not contain MRI Safety information"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case CONTAINSLATEX: return "Contains Latex"; 125 case LATEXFREE: return "Latex Free"; 126 case LATEXUNKNOWN: return "Latex Unknown"; 127 case MRSAFE: return "MR Safe"; 128 case MRUNSAFE: return "MR Unsafe"; 129 case MRCONDITIONAL: return "MR Conditional"; 130 case MRUNKNOWN: return "MR Unknown"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 136 137}