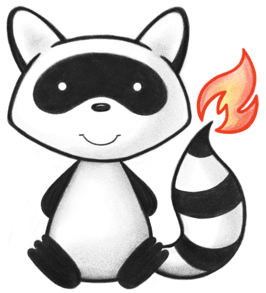
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 035 036 037import org.hl7.fhir.exceptions.FHIRException; 038 039public enum DeviceStatus { 040 041 /** 042 * The Device is available for use. Note: This means for *implanted devices* the device is implanted in the patient. 043 */ 044 ACTIVE, 045 /** 046 * The Device is no longer available for use (e.g. lost, expired, damaged). Note: This means for *implanted devices* the device has been removed from the patient. 047 */ 048 INACTIVE, 049 /** 050 * The Device was entered in error and voided. 051 */ 052 ENTEREDINERROR, 053 /** 054 * The status of the device has not been determined. 055 */ 056 UNKNOWN, 057 /** 058 * added to help the parsers 059 */ 060 NULL; 061 062 public String getDefinition() { 063 switch (this) { 064 case ACTIVE: 065 return "The Device is available for use. Note: This means for *implanted devices* the device is implanted in the patient."; 066 case INACTIVE: 067 return "The Device is no longer available for use (e.g. lost, expired, damaged). Note: This means for *implanted devices* the device has been removed from the patient."; 068 case ENTEREDINERROR: 069 return "The Device was entered in error and voided."; 070 case UNKNOWN: 071 return "The status of the device has not been determined."; 072 default: 073 return "?"; 074 } 075 } 076 077 public String getDisplay() { 078 switch (this) { 079 case ACTIVE: 080 return "Active"; 081 case INACTIVE: 082 return "Inactive"; 083 case ENTEREDINERROR: 084 return "Entered in Error"; 085 case UNKNOWN: 086 return "Unknown"; 087 default: 088 return "?"; 089 } 090 } 091 092 public String getSystem() { 093 return "http://hl7.org/fhir/device-status"; 094 } 095 096 public String toCode() { 097 switch (this) { 098 case ACTIVE: 099 return "active"; 100 case INACTIVE: 101 return "inactive"; 102 case ENTEREDINERROR: 103 return "entered-in-error"; 104 case UNKNOWN: 105 return "unknown"; 106 default: 107 return "?"; 108 } 109 } 110 111 public static DeviceStatus fromCode(String codeString) throws FHIRException { 112 if (codeString == null || "".equals(codeString)) 113 return null; 114 if ("active".equals(codeString)) 115 return ACTIVE; 116 if ("inactive".equals(codeString)) 117 return INACTIVE; 118 if ("entered-in-error".equals(codeString)) 119 return ENTEREDINERROR; 120 if ("unknown".equals(codeString)) 121 return UNKNOWN; 122 throw new FHIRException("Unknown DeviceStatus code '" + codeString + "'"); 123 } 124 125 126}