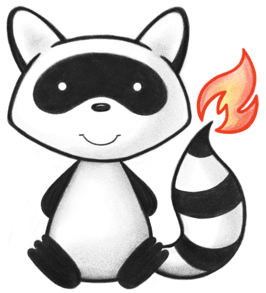
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 035 036 037import org.hl7.fhir.dstu3.model.EnumFactory; 038 039public class DeviceStatusEnumFactory implements EnumFactory<DeviceStatus> { 040 041 public DeviceStatus fromCode(String codeString) throws IllegalArgumentException { 042 if (codeString == null || "".equals(codeString)) 043 return null; 044 if ("active".equals(codeString)) 045 return DeviceStatus.ACTIVE; 046 if ("inactive".equals(codeString)) 047 return DeviceStatus.INACTIVE; 048 if ("entered-in-error".equals(codeString)) 049 return DeviceStatus.ENTEREDINERROR; 050 if ("unknown".equals(codeString)) 051 return DeviceStatus.UNKNOWN; 052 throw new IllegalArgumentException("Unknown DeviceStatus code '" + codeString + "'"); 053 } 054 055 public String toCode(DeviceStatus code) { 056 if (code == DeviceStatus.NULL) 057 return null; 058 if (code == DeviceStatus.ACTIVE) 059 return "active"; 060 if (code == DeviceStatus.INACTIVE) 061 return "inactive"; 062 if (code == DeviceStatus.ENTEREDINERROR) 063 return "entered-in-error"; 064 if (code == DeviceStatus.UNKNOWN) 065 return "unknown"; 066 return "?"; 067 } 068 069 public String toSystem(DeviceStatus code) { 070 return code.getSystem(); 071 } 072 073}