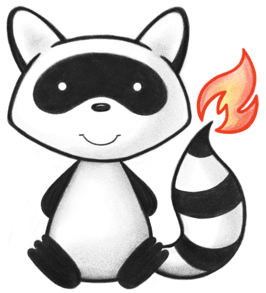
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum DicomAuditLifecycle { 041 042 /** 043 * null 044 */ 045 _1, 046 /** 047 * null 048 */ 049 _2, 050 /** 051 * null 052 */ 053 _3, 054 /** 055 * null 056 */ 057 _4, 058 /** 059 * null 060 */ 061 _5, 062 /** 063 * null 064 */ 065 _6, 066 /** 067 * null 068 */ 069 _7, 070 /** 071 * null 072 */ 073 _8, 074 /** 075 * null 076 */ 077 _9, 078 /** 079 * null 080 */ 081 _10, 082 /** 083 * null 084 */ 085 _11, 086 /** 087 * null 088 */ 089 _12, 090 /** 091 * null 092 */ 093 _13, 094 /** 095 * null 096 */ 097 _14, 098 /** 099 * null 100 */ 101 _15, 102 /** 103 * added to help the parsers 104 */ 105 NULL; 106 public static DicomAuditLifecycle fromCode(String codeString) throws FHIRException { 107 if (codeString == null || "".equals(codeString)) 108 return null; 109 if ("1".equals(codeString)) 110 return _1; 111 if ("2".equals(codeString)) 112 return _2; 113 if ("3".equals(codeString)) 114 return _3; 115 if ("4".equals(codeString)) 116 return _4; 117 if ("5".equals(codeString)) 118 return _5; 119 if ("6".equals(codeString)) 120 return _6; 121 if ("7".equals(codeString)) 122 return _7; 123 if ("8".equals(codeString)) 124 return _8; 125 if ("9".equals(codeString)) 126 return _9; 127 if ("10".equals(codeString)) 128 return _10; 129 if ("11".equals(codeString)) 130 return _11; 131 if ("12".equals(codeString)) 132 return _12; 133 if ("13".equals(codeString)) 134 return _13; 135 if ("14".equals(codeString)) 136 return _14; 137 if ("15".equals(codeString)) 138 return _15; 139 throw new FHIRException("Unknown DicomAuditLifecycle code '"+codeString+"'"); 140 } 141 public String toCode() { 142 switch (this) { 143 case _1: return "1"; 144 case _2: return "2"; 145 case _3: return "3"; 146 case _4: return "4"; 147 case _5: return "5"; 148 case _6: return "6"; 149 case _7: return "7"; 150 case _8: return "8"; 151 case _9: return "9"; 152 case _10: return "10"; 153 case _11: return "11"; 154 case _12: return "12"; 155 case _13: return "13"; 156 case _14: return "14"; 157 case _15: return "15"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getSystem() { 163 return "http://hl7.org/fhir/dicom-audit-lifecycle"; 164 } 165 public String getDefinition() { 166 switch (this) { 167 case _1: return ""; 168 case _2: return ""; 169 case _3: return ""; 170 case _4: return ""; 171 case _5: return ""; 172 case _6: return ""; 173 case _7: return ""; 174 case _8: return ""; 175 case _9: return ""; 176 case _10: return ""; 177 case _11: return ""; 178 case _12: return ""; 179 case _13: return ""; 180 case _14: return ""; 181 case _15: return ""; 182 case NULL: return null; 183 default: return "?"; 184 } 185 } 186 public String getDisplay() { 187 switch (this) { 188 case _1: return "Origination / Creation"; 189 case _2: return "Import / Copy"; 190 case _3: return "Amendment"; 191 case _4: return "Verification"; 192 case _5: return "Translation"; 193 case _6: return "Access / Use"; 194 case _7: return "De-identification"; 195 case _8: return "Aggregation / summarization / derivation"; 196 case _9: return "Report"; 197 case _10: return "Export"; 198 case _11: return "Disclosure"; 199 case _12: return "Receipt of disclosure"; 200 case _13: return "Archiving"; 201 case _14: return "Logical deletion"; 202 case _15: return "Permanent erasure / Physical destruction"; 203 case NULL: return null; 204 default: return "?"; 205 } 206 } 207 208 209}