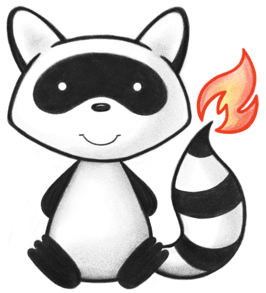
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum EncounterDiet { 041 042 /** 043 * Food without meat, poultry or seafood. 044 */ 045 VEGETARIAN, 046 /** 047 * Excludes dairy products. 048 */ 049 DAIRYFREE, 050 /** 051 * Excludes ingredients containing nuts. 052 */ 053 NUTFREE, 054 /** 055 * Excludes ingredients containing gluten. 056 */ 057 GLUTENFREE, 058 /** 059 * Food without meat, poultry, seafood, eggs, dairy products and other animal-derived substances. 060 */ 061 VEGAN, 062 /** 063 * Foods that conform to Islamic law. 064 */ 065 HALAL, 066 /** 067 * Foods that conform to Jewish dietary law. 068 */ 069 KOSHER, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 public static EncounterDiet fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("vegetarian".equals(codeString)) 078 return VEGETARIAN; 079 if ("dairy-free".equals(codeString)) 080 return DAIRYFREE; 081 if ("nut-free".equals(codeString)) 082 return NUTFREE; 083 if ("gluten-free".equals(codeString)) 084 return GLUTENFREE; 085 if ("vegan".equals(codeString)) 086 return VEGAN; 087 if ("halal".equals(codeString)) 088 return HALAL; 089 if ("kosher".equals(codeString)) 090 return KOSHER; 091 throw new FHIRException("Unknown EncounterDiet code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case VEGETARIAN: return "vegetarian"; 096 case DAIRYFREE: return "dairy-free"; 097 case NUTFREE: return "nut-free"; 098 case GLUTENFREE: return "gluten-free"; 099 case VEGAN: return "vegan"; 100 case HALAL: return "halal"; 101 case KOSHER: return "kosher"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 return "http://hl7.org/fhir/diet"; 108 } 109 public String getDefinition() { 110 switch (this) { 111 case VEGETARIAN: return "Food without meat, poultry or seafood."; 112 case DAIRYFREE: return "Excludes dairy products."; 113 case NUTFREE: return "Excludes ingredients containing nuts."; 114 case GLUTENFREE: return "Excludes ingredients containing gluten."; 115 case VEGAN: return "Food without meat, poultry, seafood, eggs, dairy products and other animal-derived substances."; 116 case HALAL: return "Foods that conform to Islamic law."; 117 case KOSHER: return "Foods that conform to Jewish dietary law."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case VEGETARIAN: return "Vegetarian"; 125 case DAIRYFREE: return "Dairy Free"; 126 case NUTFREE: return "Nut Free"; 127 case GLUTENFREE: return "Gluten Free"; 128 case VEGAN: return "Vegan"; 129 case HALAL: return "Halal"; 130 case KOSHER: return "Kosher"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 136 137}