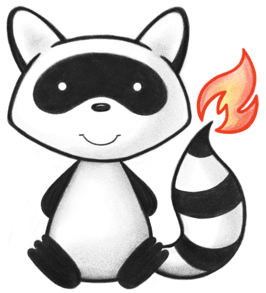
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum EncounterDischargeDisposition { 041 042 /** 043 * The patient was dicharged and has indicated that they are going to return home afterwards. 044 */ 045 HOME, 046 /** 047 * The patient was transferred to another healthcare facility. 048 */ 049 OTHERHCF, 050 /** 051 * The patient has been discharged into palliative care. 052 */ 053 HOSP, 054 /** 055 * The patient has been discharged into long-term care where is likely to be monitored through an ongoing episode-of-care. 056 */ 057 LONG, 058 /** 059 * The patient self discharged against medical advice. 060 */ 061 AADVICE, 062 /** 063 * The patient has deceased during this encounter. 064 */ 065 EXP, 066 /** 067 * The patient has been transferred to a psychiatric facility. 068 */ 069 PSY, 070 /** 071 * The patient was discharged and is to receive post acute care rehabilitation services. 072 */ 073 REHAB, 074 /** 075 * The patient has been discharged to a skilled nursing facility for the patient to receive additional care. 076 */ 077 SNF, 078 /** 079 * The discharge disposition has not otherwise defined. 080 */ 081 OTH, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 public static EncounterDischargeDisposition fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("home".equals(codeString)) 090 return HOME; 091 if ("other-hcf".equals(codeString)) 092 return OTHERHCF; 093 if ("hosp".equals(codeString)) 094 return HOSP; 095 if ("long".equals(codeString)) 096 return LONG; 097 if ("aadvice".equals(codeString)) 098 return AADVICE; 099 if ("exp".equals(codeString)) 100 return EXP; 101 if ("psy".equals(codeString)) 102 return PSY; 103 if ("rehab".equals(codeString)) 104 return REHAB; 105 if ("snf".equals(codeString)) 106 return SNF; 107 if ("oth".equals(codeString)) 108 return OTH; 109 throw new FHIRException("Unknown EncounterDischargeDisposition code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case HOME: return "home"; 114 case OTHERHCF: return "other-hcf"; 115 case HOSP: return "hosp"; 116 case LONG: return "long"; 117 case AADVICE: return "aadvice"; 118 case EXP: return "exp"; 119 case PSY: return "psy"; 120 case REHAB: return "rehab"; 121 case SNF: return "snf"; 122 case OTH: return "oth"; 123 case NULL: return null; 124 default: return "?"; 125 } 126 } 127 public String getSystem() { 128 return "http://hl7.org/fhir/discharge-disposition"; 129 } 130 public String getDefinition() { 131 switch (this) { 132 case HOME: return "The patient was dicharged and has indicated that they are going to return home afterwards."; 133 case OTHERHCF: return "The patient was transferred to another healthcare facility."; 134 case HOSP: return "The patient has been discharged into palliative care."; 135 case LONG: return "The patient has been discharged into long-term care where is likely to be monitored through an ongoing episode-of-care."; 136 case AADVICE: return "The patient self discharged against medical advice."; 137 case EXP: return "The patient has deceased during this encounter."; 138 case PSY: return "The patient has been transferred to a psychiatric facility."; 139 case REHAB: return "The patient was discharged and is to receive post acute care rehabilitation services."; 140 case SNF: return "The patient has been discharged to a skilled nursing facility for the patient to receive additional care."; 141 case OTH: return "The discharge disposition has not otherwise defined."; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 public String getDisplay() { 147 switch (this) { 148 case HOME: return "Home"; 149 case OTHERHCF: return "Other healthcare facility"; 150 case HOSP: return "Hospice"; 151 case LONG: return "Long-term care"; 152 case AADVICE: return "Left against advice"; 153 case EXP: return "Expired"; 154 case PSY: return "Psychiatric hospital"; 155 case REHAB: return "Rehabilitation"; 156 case SNF: return "Skilled nursing facility"; 157 case OTH: return "Other"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 163 164}