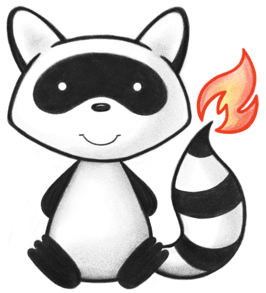
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum EncounterLocationStatus { 041 042 /** 043 * The patient is planned to be moved to this location at some point in the future. 044 */ 045 PLANNED, 046 /** 047 * The patient is currently at this location, or was between the period specified. 048 049A system may update these records when the patient leaves the location to either reserved, or completed 050 */ 051 ACTIVE, 052 /** 053 * This location is held empty for this patient. 054 */ 055 RESERVED, 056 /** 057 * The patient was at this location during the period specified. 058 059Not to be used when the patient is currently at the location 060 */ 061 COMPLETED, 062 /** 063 * added to help the parsers 064 */ 065 NULL; 066 public static EncounterLocationStatus fromCode(String codeString) throws FHIRException { 067 if (codeString == null || "".equals(codeString)) 068 return null; 069 if ("planned".equals(codeString)) 070 return PLANNED; 071 if ("active".equals(codeString)) 072 return ACTIVE; 073 if ("reserved".equals(codeString)) 074 return RESERVED; 075 if ("completed".equals(codeString)) 076 return COMPLETED; 077 throw new FHIRException("Unknown EncounterLocationStatus code '"+codeString+"'"); 078 } 079 public String toCode() { 080 switch (this) { 081 case PLANNED: return "planned"; 082 case ACTIVE: return "active"; 083 case RESERVED: return "reserved"; 084 case COMPLETED: return "completed"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 return "http://hl7.org/fhir/encounter-location-status"; 091 } 092 public String getDefinition() { 093 switch (this) { 094 case PLANNED: return "The patient is planned to be moved to this location at some point in the future."; 095 case ACTIVE: return "The patient is currently at this location, or was between the period specified.\r\rA system may update these records when the patient leaves the location to either reserved, or completed"; 096 case RESERVED: return "This location is held empty for this patient."; 097 case COMPLETED: return "The patient was at this location during the period specified.\r\rNot to be used when the patient is currently at the location"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getDisplay() { 103 switch (this) { 104 case PLANNED: return "Planned"; 105 case ACTIVE: return "Active"; 106 case RESERVED: return "Reserved"; 107 case COMPLETED: return "Completed"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 113 114}