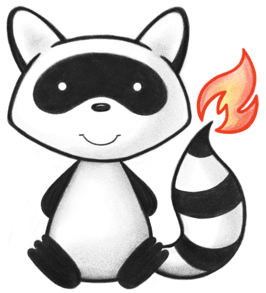
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum EncounterStatus { 041 042 /** 043 * The Encounter has not yet started. 044 */ 045 PLANNED, 046 /** 047 * The Patient is present for the encounter, however is not currently meeting with a practitioner. 048 */ 049 ARRIVED, 050 /** 051 * The patient has been assessed for the priority of their treatment based on the severity of their condition. 052 */ 053 TRIAGED, 054 /** 055 * The Encounter has begun and the patient is present / the practitioner and the patient are meeting. 056 */ 057 INPROGRESS, 058 /** 059 * The Encounter has begun, but the patient is temporarily on leave. 060 */ 061 ONLEAVE, 062 /** 063 * The Encounter has ended. 064 */ 065 FINISHED, 066 /** 067 * The Encounter has ended before it has begun. 068 */ 069 CANCELLED, 070 /** 071 * This instance should not have been part of this patient's medical record. 072 */ 073 ENTEREDINERROR, 074 /** 075 * The encounter status is unknown. Note that "unknown" is a value of last resort and every attempt should be made to provide a meaningful value other than "unknown". 076 */ 077 UNKNOWN, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 public static EncounterStatus fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("planned".equals(codeString)) 086 return PLANNED; 087 if ("arrived".equals(codeString)) 088 return ARRIVED; 089 if ("triaged".equals(codeString)) 090 return TRIAGED; 091 if ("in-progress".equals(codeString)) 092 return INPROGRESS; 093 if ("onleave".equals(codeString)) 094 return ONLEAVE; 095 if ("finished".equals(codeString)) 096 return FINISHED; 097 if ("cancelled".equals(codeString)) 098 return CANCELLED; 099 if ("entered-in-error".equals(codeString)) 100 return ENTEREDINERROR; 101 if ("unknown".equals(codeString)) 102 return UNKNOWN; 103 throw new FHIRException("Unknown EncounterStatus code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case PLANNED: return "planned"; 108 case ARRIVED: return "arrived"; 109 case TRIAGED: return "triaged"; 110 case INPROGRESS: return "in-progress"; 111 case ONLEAVE: return "onleave"; 112 case FINISHED: return "finished"; 113 case CANCELLED: return "cancelled"; 114 case ENTEREDINERROR: return "entered-in-error"; 115 case UNKNOWN: return "unknown"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getSystem() { 121 return "http://hl7.org/fhir/encounter-status"; 122 } 123 public String getDefinition() { 124 switch (this) { 125 case PLANNED: return "The Encounter has not yet started."; 126 case ARRIVED: return "The Patient is present for the encounter, however is not currently meeting with a practitioner."; 127 case TRIAGED: return "The patient has been assessed for the priority of their treatment based on the severity of their condition."; 128 case INPROGRESS: return "The Encounter has begun and the patient is present / the practitioner and the patient are meeting."; 129 case ONLEAVE: return "The Encounter has begun, but the patient is temporarily on leave."; 130 case FINISHED: return "The Encounter has ended."; 131 case CANCELLED: return "The Encounter has ended before it has begun."; 132 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 133 case UNKNOWN: return "The encounter status is unknown. Note that \"unknown\" is a value of last resort and every attempt should be made to provide a meaningful value other than \"unknown\"."; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getDisplay() { 139 switch (this) { 140 case PLANNED: return "Planned"; 141 case ARRIVED: return "Arrived"; 142 case TRIAGED: return "Triaged"; 143 case INPROGRESS: return "In Progress"; 144 case ONLEAVE: return "On Leave"; 145 case FINISHED: return "Finished"; 146 case CANCELLED: return "Cancelled"; 147 case ENTEREDINERROR: return "Entered in Error"; 148 case UNKNOWN: return "Unknown"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 154 155}