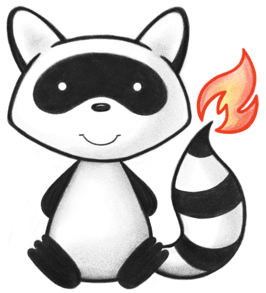
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum EndpointConnectionType { 041 042 /** 043 * IHE Cross Community Patient Discovery Profile (XCPD) - http://wiki.ihe.net/index.php/Cross-Community_Patient_Discovery 044 */ 045 IHEXCPD, 046 /** 047 * IHE Cross Community Access Profile (XCA) - http://wiki.ihe.net/index.php/Cross-Community_Access 048 */ 049 IHEXCA, 050 /** 051 * IHE Cross-Enterprise Document Reliable Exchange (XDR) - http://wiki.ihe.net/index.php/Cross-enterprise_Document_Reliable_Interchange 052 */ 053 IHEXDR, 054 /** 055 * IHE Cross-Enterprise Document Sharing (XDS) - http://wiki.ihe.net/index.php/Cross-Enterprise_Document_Sharing 056 */ 057 IHEXDS, 058 /** 059 * IHE Invoke Image Display (IID) - http://wiki.ihe.net/index.php/Invoke_Image_Display 060 */ 061 IHEIID, 062 /** 063 * DICOMweb RESTful Image Retrieve - http://dicom.nema.org/medical/dicom/current/output/chtml/part18/sect_6.5.html 064 */ 065 DICOMWADORS, 066 /** 067 * DICOMweb RESTful Image query - http://dicom.nema.org/medical/dicom/current/output/chtml/part18/sect_6.7.html 068 */ 069 DICOMQIDORS, 070 /** 071 * DICOMweb RESTful image sending and storage - http://dicom.nema.org/medical/dicom/current/output/chtml/part18/sect_6.6.html 072 */ 073 DICOMSTOWRS, 074 /** 075 * DICOMweb Image Retrieve - http://dicom.nema.org/dicom/2013/output/chtml/part18/sect_6.3.html 076 */ 077 DICOMWADOURI, 078 /** 079 * Interact with the server interface using FHIR's RESTful interface. For details on its version/capabilities you should connect the the value in Endpoint.address and retrieve the FHIR CapabilityStatement. 080 */ 081 HL7FHIRREST, 082 /** 083 * Use the servers FHIR Messaging interface. Details can be found on the messaging.html page in the FHIR Specification. The FHIR server's base address is specified in the Endpoint.address property. 084 */ 085 HL7FHIRMSG, 086 /** 087 * HL7v2 messages over an LLP TCP connection 088 */ 089 HL7V2MLLP, 090 /** 091 * Email delivery using a digital certificate to encrypt the content using the public key, receiver must have the private key to decrypt the content 092 */ 093 SECUREEMAIL, 094 /** 095 * Direct Project information - http://wiki.directproject.org/ 096 */ 097 DIRECTPROJECT, 098 /** 099 * added to help the parsers 100 */ 101 NULL; 102 public static EndpointConnectionType fromCode(String codeString) throws FHIRException { 103 if (codeString == null || "".equals(codeString)) 104 return null; 105 if ("ihe-xcpd".equals(codeString)) 106 return IHEXCPD; 107 if ("ihe-xca".equals(codeString)) 108 return IHEXCA; 109 if ("ihe-xdr".equals(codeString)) 110 return IHEXDR; 111 if ("ihe-xds".equals(codeString)) 112 return IHEXDS; 113 if ("ihe-iid".equals(codeString)) 114 return IHEIID; 115 if ("dicom-wado-rs".equals(codeString)) 116 return DICOMWADORS; 117 if ("dicom-qido-rs".equals(codeString)) 118 return DICOMQIDORS; 119 if ("dicom-stow-rs".equals(codeString)) 120 return DICOMSTOWRS; 121 if ("dicom-wado-uri".equals(codeString)) 122 return DICOMWADOURI; 123 if ("hl7-fhir-rest".equals(codeString)) 124 return HL7FHIRREST; 125 if ("hl7-fhir-msg".equals(codeString)) 126 return HL7FHIRMSG; 127 if ("hl7v2-mllp".equals(codeString)) 128 return HL7V2MLLP; 129 if ("secure-email".equals(codeString)) 130 return SECUREEMAIL; 131 if ("direct-project".equals(codeString)) 132 return DIRECTPROJECT; 133 throw new FHIRException("Unknown EndpointConnectionType code '"+codeString+"'"); 134 } 135 public String toCode() { 136 switch (this) { 137 case IHEXCPD: return "ihe-xcpd"; 138 case IHEXCA: return "ihe-xca"; 139 case IHEXDR: return "ihe-xdr"; 140 case IHEXDS: return "ihe-xds"; 141 case IHEIID: return "ihe-iid"; 142 case DICOMWADORS: return "dicom-wado-rs"; 143 case DICOMQIDORS: return "dicom-qido-rs"; 144 case DICOMSTOWRS: return "dicom-stow-rs"; 145 case DICOMWADOURI: return "dicom-wado-uri"; 146 case HL7FHIRREST: return "hl7-fhir-rest"; 147 case HL7FHIRMSG: return "hl7-fhir-msg"; 148 case HL7V2MLLP: return "hl7v2-mllp"; 149 case SECUREEMAIL: return "secure-email"; 150 case DIRECTPROJECT: return "direct-project"; 151 case NULL: return null; 152 default: return "?"; 153 } 154 } 155 public String getSystem() { 156 return "http://hl7.org/fhir/endpoint-connection-type"; 157 } 158 public String getDefinition() { 159 switch (this) { 160 case IHEXCPD: return "IHE Cross Community Patient Discovery Profile (XCPD) - http://wiki.ihe.net/index.php/Cross-Community_Patient_Discovery"; 161 case IHEXCA: return "IHE Cross Community Access Profile (XCA) - http://wiki.ihe.net/index.php/Cross-Community_Access"; 162 case IHEXDR: return "IHE Cross-Enterprise Document Reliable Exchange (XDR) - http://wiki.ihe.net/index.php/Cross-enterprise_Document_Reliable_Interchange"; 163 case IHEXDS: return "IHE Cross-Enterprise Document Sharing (XDS) - http://wiki.ihe.net/index.php/Cross-Enterprise_Document_Sharing"; 164 case IHEIID: return "IHE Invoke Image Display (IID) - http://wiki.ihe.net/index.php/Invoke_Image_Display"; 165 case DICOMWADORS: return "DICOMweb RESTful Image Retrieve - http://dicom.nema.org/medical/dicom/current/output/chtml/part18/sect_6.5.html"; 166 case DICOMQIDORS: return "DICOMweb RESTful Image query - http://dicom.nema.org/medical/dicom/current/output/chtml/part18/sect_6.7.html"; 167 case DICOMSTOWRS: return "DICOMweb RESTful image sending and storage - http://dicom.nema.org/medical/dicom/current/output/chtml/part18/sect_6.6.html"; 168 case DICOMWADOURI: return "DICOMweb Image Retrieve - http://dicom.nema.org/dicom/2013/output/chtml/part18/sect_6.3.html"; 169 case HL7FHIRREST: return "Interact with the server interface using FHIR's RESTful interface. For details on its version/capabilities you should connect the the value in Endpoint.address and retrieve the FHIR CapabilityStatement."; 170 case HL7FHIRMSG: return "Use the servers FHIR Messaging interface. Details can be found on the messaging.html page in the FHIR Specification. The FHIR server's base address is specified in the Endpoint.address property."; 171 case HL7V2MLLP: return "HL7v2 messages over an LLP TCP connection"; 172 case SECUREEMAIL: return "Email delivery using a digital certificate to encrypt the content using the public key, receiver must have the private key to decrypt the content"; 173 case DIRECTPROJECT: return "Direct Project information - http://wiki.directproject.org/"; 174 case NULL: return null; 175 default: return "?"; 176 } 177 } 178 public String getDisplay() { 179 switch (this) { 180 case IHEXCPD: return "IHE XCPD"; 181 case IHEXCA: return "IHE XCA"; 182 case IHEXDR: return "IHE XDR"; 183 case IHEXDS: return "IHE XDS"; 184 case IHEIID: return "IHE IID"; 185 case DICOMWADORS: return "DICOM WADO-RS"; 186 case DICOMQIDORS: return "DICOM QIDO-RS"; 187 case DICOMSTOWRS: return "DICOM STOW-RS"; 188 case DICOMWADOURI: return "DICOM WADO-URI"; 189 case HL7FHIRREST: return "HL7 FHIR"; 190 case HL7FHIRMSG: return "HL7 FHIR Messaging"; 191 case HL7V2MLLP: return "HL7 v2 MLLP"; 192 case SECUREEMAIL: return "Secure email"; 193 case DIRECTPROJECT: return "Direct Project"; 194 case NULL: return null; 195 default: return "?"; 196 } 197 } 198 199 200}