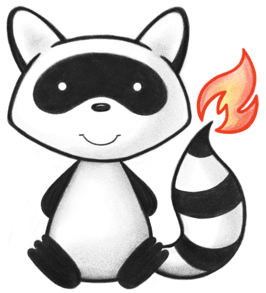
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum EpisodeOfCareStatus { 041 042 /** 043 * This episode of care is planned to start at the date specified in the period.start. During this status, an organization may perform assessments to determine if the patient is eligible to receive services, or be organizing to make resources available to provide care services. 044 */ 045 PLANNED, 046 /** 047 * This episode has been placed on a waitlist, pending the episode being made active (or cancelled). 048 */ 049 WAITLIST, 050 /** 051 * This episode of care is current. 052 */ 053 ACTIVE, 054 /** 055 * This episode of care is on hold, the organization has limited responsibility for the patient (such as while on respite). 056 */ 057 ONHOLD, 058 /** 059 * This episode of care is finished and the organization is not expecting to be providing further care to the patient. Can also be known as "closed", "completed" or other similar terms. 060 */ 061 FINISHED, 062 /** 063 * The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow. 064 */ 065 CANCELLED, 066 /** 067 * This instance should not have been part of this patient's medical record. 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 public static EpisodeOfCareStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("planned".equals(codeString)) 078 return PLANNED; 079 if ("waitlist".equals(codeString)) 080 return WAITLIST; 081 if ("active".equals(codeString)) 082 return ACTIVE; 083 if ("onhold".equals(codeString)) 084 return ONHOLD; 085 if ("finished".equals(codeString)) 086 return FINISHED; 087 if ("cancelled".equals(codeString)) 088 return CANCELLED; 089 if ("entered-in-error".equals(codeString)) 090 return ENTEREDINERROR; 091 throw new FHIRException("Unknown EpisodeOfCareStatus code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case PLANNED: return "planned"; 096 case WAITLIST: return "waitlist"; 097 case ACTIVE: return "active"; 098 case ONHOLD: return "onhold"; 099 case FINISHED: return "finished"; 100 case CANCELLED: return "cancelled"; 101 case ENTEREDINERROR: return "entered-in-error"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 return "http://hl7.org/fhir/episode-of-care-status"; 108 } 109 public String getDefinition() { 110 switch (this) { 111 case PLANNED: return "This episode of care is planned to start at the date specified in the period.start. During this status, an organization may perform assessments to determine if the patient is eligible to receive services, or be organizing to make resources available to provide care services."; 112 case WAITLIST: return "This episode has been placed on a waitlist, pending the episode being made active (or cancelled)."; 113 case ACTIVE: return "This episode of care is current."; 114 case ONHOLD: return "This episode of care is on hold, the organization has limited responsibility for the patient (such as while on respite)."; 115 case FINISHED: return "This episode of care is finished and the organization is not expecting to be providing further care to the patient. Can also be known as \"closed\", \"completed\" or other similar terms."; 116 case CANCELLED: return "The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow."; 117 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case PLANNED: return "Planned"; 125 case WAITLIST: return "Waitlist"; 126 case ACTIVE: return "Active"; 127 case ONHOLD: return "On Hold"; 128 case FINISHED: return "Finished"; 129 case CANCELLED: return "Cancelled"; 130 case ENTEREDINERROR: return "Entered in Error"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 136 137}