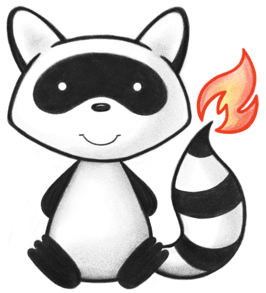
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ExDiagnosistype { 041 042 /** 043 * The diagnosis given as the reason why the patient was admitted to the hospital. 044 */ 045 ADMITTING, 046 /** 047 * A diagnosis made on the basis of medical signs and patient-reported symptoms, rather than diagnostic tests. 048 */ 049 CLINICAL, 050 /** 051 * One of a set of the possible diagnoses that could be connected to the signs, symptoms, and lab findings. 052 */ 053 DIFFERENTIAL, 054 /** 055 * The diagnosis given when the patient is discharged from the hospital. 056 */ 057 DISCHARGE, 058 /** 059 * A diagnosis based significantly on laboratory reports or test results, rather than the physical examination of the patient. 060 */ 061 LABORATORY, 062 /** 063 * A diagnosis which identifies people's responses to situations in their lives, such as a readiness to change or a willingness to accept assistance. 064 */ 065 NURSING, 066 /** 067 * A diagnosis determined prior to birth. 068 */ 069 PRENATAL, 070 /** 071 * The single medical diagnosis that is most relevant to the patient's chief complaint or need for treatment. 072 */ 073 PRINCIPAL, 074 /** 075 * A diagnosis based primarily on the results from medical imaging studies. 076 */ 077 RADIOLOGY, 078 /** 079 * A diagnosis determined using telemedicine techniques. 080 */ 081 REMOTE, 082 /** 083 * The labeling of an illness in a specific historical event using modern knowledge, methods and disease classifications. 084 */ 085 RETROSPECTIVE, 086 /** 087 * A diagnosis determined by the patient. 088 */ 089 SELF, 090 /** 091 * added to help the parsers 092 */ 093 NULL; 094 public static ExDiagnosistype fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("admitting".equals(codeString)) 098 return ADMITTING; 099 if ("clinical".equals(codeString)) 100 return CLINICAL; 101 if ("differential".equals(codeString)) 102 return DIFFERENTIAL; 103 if ("discharge".equals(codeString)) 104 return DISCHARGE; 105 if ("laboratory".equals(codeString)) 106 return LABORATORY; 107 if ("nursing".equals(codeString)) 108 return NURSING; 109 if ("prenatal".equals(codeString)) 110 return PRENATAL; 111 if ("principal".equals(codeString)) 112 return PRINCIPAL; 113 if ("radiology".equals(codeString)) 114 return RADIOLOGY; 115 if ("remote".equals(codeString)) 116 return REMOTE; 117 if ("retrospective".equals(codeString)) 118 return RETROSPECTIVE; 119 if ("self".equals(codeString)) 120 return SELF; 121 throw new FHIRException("Unknown ExDiagnosistype code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case ADMITTING: return "admitting"; 126 case CLINICAL: return "clinical"; 127 case DIFFERENTIAL: return "differential"; 128 case DISCHARGE: return "discharge"; 129 case LABORATORY: return "laboratory"; 130 case NURSING: return "nursing"; 131 case PRENATAL: return "prenatal"; 132 case PRINCIPAL: return "principal"; 133 case RADIOLOGY: return "radiology"; 134 case REMOTE: return "remote"; 135 case RETROSPECTIVE: return "retrospective"; 136 case SELF: return "self"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getSystem() { 142 return "http://hl7.org/fhir/ex-diagnosistype"; 143 } 144 public String getDefinition() { 145 switch (this) { 146 case ADMITTING: return "The diagnosis given as the reason why the patient was admitted to the hospital."; 147 case CLINICAL: return "A diagnosis made on the basis of medical signs and patient-reported symptoms, rather than diagnostic tests."; 148 case DIFFERENTIAL: return "One of a set of the possible diagnoses that could be connected to the signs, symptoms, and lab findings."; 149 case DISCHARGE: return "The diagnosis given when the patient is discharged from the hospital."; 150 case LABORATORY: return "A diagnosis based significantly on laboratory reports or test results, rather than the physical examination of the patient."; 151 case NURSING: return "A diagnosis which identifies people's responses to situations in their lives, such as a readiness to change or a willingness to accept assistance."; 152 case PRENATAL: return "A diagnosis determined prior to birth."; 153 case PRINCIPAL: return "The single medical diagnosis that is most relevant to the patient's chief complaint or need for treatment."; 154 case RADIOLOGY: return "A diagnosis based primarily on the results from medical imaging studies."; 155 case REMOTE: return "A diagnosis determined using telemedicine techniques."; 156 case RETROSPECTIVE: return "The labeling of an illness in a specific historical event using modern knowledge, methods and disease classifications."; 157 case SELF: return "A diagnosis determined by the patient."; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getDisplay() { 163 switch (this) { 164 case ADMITTING: return "Admitting Diagnosis"; 165 case CLINICAL: return "Clinical Diagnosis"; 166 case DIFFERENTIAL: return "Differential Diagnosis"; 167 case DISCHARGE: return "Discharge Diagnosis"; 168 case LABORATORY: return "Laboratory Diagnosis"; 169 case NURSING: return "Nursing Diagnosis"; 170 case PRENATAL: return "Prenatal Diagnosis"; 171 case PRINCIPAL: return "Principal Diagnosis"; 172 case RADIOLOGY: return "Radiology Diagnosis"; 173 case REMOTE: return "Remote Diagnosis"; 174 case RETROSPECTIVE: return "Retrospective Diagnosis"; 175 case SELF: return "Self Diagnosis"; 176 case NULL: return null; 177 default: return "?"; 178 } 179 } 180 181 182}