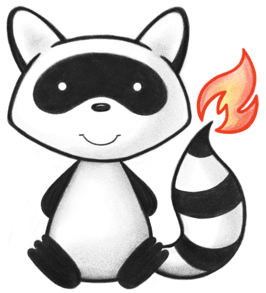
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ExRevenueCenter { 041 042 /** 043 * Anaesthesia. 044 */ 045 _0370, 046 /** 047 * Physical Therapy. 048 */ 049 _0420, 050 /** 051 * Physical Therapy - visit charge. 052 */ 053 _0421, 054 /** 055 * Speech-Language Pathology. 056 */ 057 _0440, 058 /** 059 * Speech-Language Pathology- visit charge 060 */ 061 _0441, 062 /** 063 * Emergency Room 064 */ 065 _0450, 066 /** 067 * Emergency Room - EM/EMTALA 068 */ 069 _0451, 070 /** 071 * Emergency Room - beyond EMTALA 072 */ 073 _0452, 074 /** 075 * Vision Clinic 076 */ 077 _0010, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 public static ExRevenueCenter fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("0370".equals(codeString)) 086 return _0370; 087 if ("0420".equals(codeString)) 088 return _0420; 089 if ("0421".equals(codeString)) 090 return _0421; 091 if ("0440".equals(codeString)) 092 return _0440; 093 if ("0441".equals(codeString)) 094 return _0441; 095 if ("0450".equals(codeString)) 096 return _0450; 097 if ("0451".equals(codeString)) 098 return _0451; 099 if ("0452".equals(codeString)) 100 return _0452; 101 if ("0010".equals(codeString)) 102 return _0010; 103 throw new FHIRException("Unknown ExRevenueCenter code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case _0370: return "0370"; 108 case _0420: return "0420"; 109 case _0421: return "0421"; 110 case _0440: return "0440"; 111 case _0441: return "0441"; 112 case _0450: return "0450"; 113 case _0451: return "0451"; 114 case _0452: return "0452"; 115 case _0010: return "0010"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getSystem() { 121 return "http://hl7.org/fhir/ex-revenue-center"; 122 } 123 public String getDefinition() { 124 switch (this) { 125 case _0370: return "Anaesthesia."; 126 case _0420: return "Physical Therapy."; 127 case _0421: return "Physical Therapy - visit charge."; 128 case _0440: return "Speech-Language Pathology."; 129 case _0441: return "Speech-Language Pathology- visit charge"; 130 case _0450: return "Emergency Room"; 131 case _0451: return "Emergency Room - EM/EMTALA"; 132 case _0452: return "Emergency Room - beyond EMTALA"; 133 case _0010: return "Vision Clinic"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getDisplay() { 139 switch (this) { 140 case _0370: return "Anaesthesia"; 141 case _0420: return "Physical Therapy"; 142 case _0421: return "Physical Therapy - "; 143 case _0440: return "Speech-Language Pathology"; 144 case _0441: return "Speech-Language Pathology - Visit"; 145 case _0450: return "Emergency Room"; 146 case _0451: return "Emergency Room - EM/EMTALA"; 147 case _0452: return "Emergency Room - beyond EMTALA"; 148 case _0010: return "Vision Clinic"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 154 155}