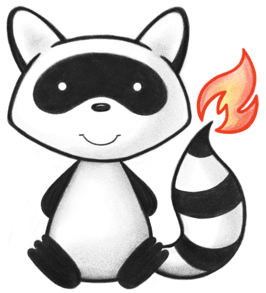
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum FilterOperator { 041 042 /** 043 * The specified property of the code equals the provided value. 044 */ 045 EQUAL, 046 /** 047 * Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, including the provided concept itself (i.e. include child codes) 048 */ 049 ISA, 050 /** 051 * Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, excluding the provided concept itself (i.e. include child codes) 052 */ 053 DESCENDENTOF, 054 /** 055 * The specified property of the code does not have an is-a relationship with the provided value. 056 */ 057 ISNOTA, 058 /** 059 * The specified property of the code matches the regex specified in the provided value. 060 */ 061 REGEX, 062 /** 063 * The specified property of the code is in the set of codes or concepts specified in the provided value (comma separated list). 064 */ 065 IN, 066 /** 067 * The specified property of the code is not in the set of codes or concepts specified in the provided value (comma separated list). 068 */ 069 NOTIN, 070 /** 071 * Includes all concept ids that have a transitive is-a relationship from the concept Id provided as the value, including the provided concept itself (e.g. include parent codes) 072 */ 073 GENERALIZES, 074 /** 075 * The specified property of the code has at least one value (if the specified value is true; if the specified value is false, then matches when the specified property of the code has no values) 076 */ 077 EXISTS, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 public static FilterOperator fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("=".equals(codeString)) 086 return EQUAL; 087 if ("is-a".equals(codeString)) 088 return ISA; 089 if ("descendent-of".equals(codeString)) 090 return DESCENDENTOF; 091 if ("is-not-a".equals(codeString)) 092 return ISNOTA; 093 if ("regex".equals(codeString)) 094 return REGEX; 095 if ("in".equals(codeString)) 096 return IN; 097 if ("not-in".equals(codeString)) 098 return NOTIN; 099 if ("generalizes".equals(codeString)) 100 return GENERALIZES; 101 if ("exists".equals(codeString)) 102 return EXISTS; 103 throw new FHIRException("Unknown FilterOperator code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case EQUAL: return "="; 108 case ISA: return "is-a"; 109 case DESCENDENTOF: return "descendent-of"; 110 case ISNOTA: return "is-not-a"; 111 case REGEX: return "regex"; 112 case IN: return "in"; 113 case NOTIN: return "not-in"; 114 case GENERALIZES: return "generalizes"; 115 case EXISTS: return "exists"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getSystem() { 121 return "http://hl7.org/fhir/filter-operator"; 122 } 123 public String getDefinition() { 124 switch (this) { 125 case EQUAL: return "The specified property of the code equals the provided value."; 126 case ISA: return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, including the provided concept itself (i.e. include child codes)"; 127 case DESCENDENTOF: return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, excluding the provided concept itself (i.e. include child codes)"; 128 case ISNOTA: return "The specified property of the code does not have an is-a relationship with the provided value."; 129 case REGEX: return "The specified property of the code matches the regex specified in the provided value."; 130 case IN: return "The specified property of the code is in the set of codes or concepts specified in the provided value (comma separated list)."; 131 case NOTIN: return "The specified property of the code is not in the set of codes or concepts specified in the provided value (comma separated list)."; 132 case GENERALIZES: return "Includes all concept ids that have a transitive is-a relationship from the concept Id provided as the value, including the provided concept itself (e.g. include parent codes)"; 133 case EXISTS: return "The specified property of the code has at least one value (if the specified value is true; if the specified value is false, then matches when the specified property of the code has no values)"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getDisplay() { 139 switch (this) { 140 case EQUAL: return "Equals"; 141 case ISA: return "Is A (by subsumption)"; 142 case DESCENDENTOF: return "Descendent Of (by subsumption)"; 143 case ISNOTA: return "Not (Is A) (by subsumption)"; 144 case REGEX: return "Regular Expression"; 145 case IN: return "In Set"; 146 case NOTIN: return "Not in Set"; 147 case GENERALIZES: return "Generalizes (by Subsumption)"; 148 case EXISTS: return "Exists"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 154 155}