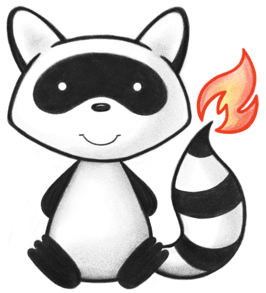
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum GoalStatus { 041 042 /** 043 * A goal is proposed for this patient 044 */ 045 PROPOSED, 046 /** 047 * A proposed goal was accepted or acknowledged 048 */ 049 ACCEPTED, 050 /** 051 * A goal is planned for this patient 052 */ 053 PLANNED, 054 /** 055 * The goal is being sought but has not yet been reached. (Also applies if goal was reached in the past but there has been regression and goal is being sought again) 056 */ 057 INPROGRESS, 058 /** 059 * The goal is on schedule for the planned timelines 060 */ 061 ONTARGET, 062 /** 063 * The goal is ahead of the planned timelines 064 */ 065 AHEADOFTARGET, 066 /** 067 * The goal is behind the planned timelines 068 */ 069 BEHINDTARGET, 070 /** 071 * The goal has been met, but ongoing activity is needed to sustain the goal objective 072 */ 073 SUSTAINING, 074 /** 075 * The goal has been met and no further action is needed 076 */ 077 ACHIEVED, 078 /** 079 * The goal remains a long term objective but is no longer being actively pursued for a temporary period of time. 080 */ 081 ONHOLD, 082 /** 083 * The previously accepted goal is no longer being sought 084 */ 085 CANCELLED, 086 /** 087 * The goal was entered in error and voided. 088 */ 089 ENTEREDINERROR, 090 /** 091 * A proposed goal was rejected 092 */ 093 REJECTED, 094 /** 095 * added to help the parsers 096 */ 097 NULL; 098 public static GoalStatus fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("proposed".equals(codeString)) 102 return PROPOSED; 103 if ("accepted".equals(codeString)) 104 return ACCEPTED; 105 if ("planned".equals(codeString)) 106 return PLANNED; 107 if ("in-progress".equals(codeString)) 108 return INPROGRESS; 109 if ("on-target".equals(codeString)) 110 return ONTARGET; 111 if ("ahead-of-target".equals(codeString)) 112 return AHEADOFTARGET; 113 if ("behind-target".equals(codeString)) 114 return BEHINDTARGET; 115 if ("sustaining".equals(codeString)) 116 return SUSTAINING; 117 if ("achieved".equals(codeString)) 118 return ACHIEVED; 119 if ("on-hold".equals(codeString)) 120 return ONHOLD; 121 if ("cancelled".equals(codeString)) 122 return CANCELLED; 123 if ("entered-in-error".equals(codeString)) 124 return ENTEREDINERROR; 125 if ("rejected".equals(codeString)) 126 return REJECTED; 127 throw new FHIRException("Unknown GoalStatus code '"+codeString+"'"); 128 } 129 public String toCode() { 130 switch (this) { 131 case PROPOSED: return "proposed"; 132 case ACCEPTED: return "accepted"; 133 case PLANNED: return "planned"; 134 case INPROGRESS: return "in-progress"; 135 case ONTARGET: return "on-target"; 136 case AHEADOFTARGET: return "ahead-of-target"; 137 case BEHINDTARGET: return "behind-target"; 138 case SUSTAINING: return "sustaining"; 139 case ACHIEVED: return "achieved"; 140 case ONHOLD: return "on-hold"; 141 case CANCELLED: return "cancelled"; 142 case ENTEREDINERROR: return "entered-in-error"; 143 case REJECTED: return "rejected"; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getSystem() { 149 return "http://hl7.org/fhir/goal-status"; 150 } 151 public String getDefinition() { 152 switch (this) { 153 case PROPOSED: return "A goal is proposed for this patient"; 154 case ACCEPTED: return "A proposed goal was accepted or acknowledged"; 155 case PLANNED: return "A goal is planned for this patient"; 156 case INPROGRESS: return "The goal is being sought but has not yet been reached. (Also applies if goal was reached in the past but there has been regression and goal is being sought again)"; 157 case ONTARGET: return "The goal is on schedule for the planned timelines"; 158 case AHEADOFTARGET: return "The goal is ahead of the planned timelines"; 159 case BEHINDTARGET: return "The goal is behind the planned timelines"; 160 case SUSTAINING: return "The goal has been met, but ongoing activity is needed to sustain the goal objective"; 161 case ACHIEVED: return "The goal has been met and no further action is needed"; 162 case ONHOLD: return "The goal remains a long term objective but is no longer being actively pursued for a temporary period of time."; 163 case CANCELLED: return "The previously accepted goal is no longer being sought"; 164 case ENTEREDINERROR: return "The goal was entered in error and voided."; 165 case REJECTED: return "A proposed goal was rejected"; 166 case NULL: return null; 167 default: return "?"; 168 } 169 } 170 public String getDisplay() { 171 switch (this) { 172 case PROPOSED: return "Proposed"; 173 case ACCEPTED: return "Accepted"; 174 case PLANNED: return "Planned"; 175 case INPROGRESS: return "In Progress"; 176 case ONTARGET: return "On Target"; 177 case AHEADOFTARGET: return "Ahead of Target"; 178 case BEHINDTARGET: return "Behind Target"; 179 case SUSTAINING: return "Sustaining"; 180 case ACHIEVED: return "Achieved"; 181 case ONHOLD: return "On Hold"; 182 case CANCELLED: return "Cancelled"; 183 case ENTEREDINERROR: return "Entered In Error"; 184 case REJECTED: return "Rejected"; 185 case NULL: return null; 186 default: return "?"; 187 } 188 } 189 190 191}