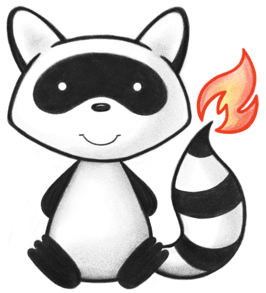
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum Hl7WorkGroup { 041 042 /** 043 * Community Based Collaborative Care (http://www.hl7.org/Special/committees/cbcc/index.cfm) 044 */ 045 CBCC, 046 /** 047 * Clinical Decision Support (http://www.hl7.org/Special/committees/dss/index.cfm) 048 */ 049 CDS, 050 /** 051 * Clinical Quality Information (http://www.hl7.org/Special/committees/cqi/index.cfm) 052 */ 053 CQI, 054 /** 055 * Clinical Genomics (http://www.hl7.org/Special/committees/clingenomics/index.cfm) 056 */ 057 CG, 058 /** 059 * Health Care Devices (http://www.hl7.org/Special/committees/healthcaredevices/index.cfm) 060 */ 061 DEV, 062 /** 063 * Electronic Health Records (http://www.hl7.org/Special/committees/ehr/index.cfm) 064 */ 065 EHR, 066 /** 067 * FHIR Infrastructure (http://www.hl7.org/Special/committees/fiwg/index.cfm) 068 */ 069 FHIR, 070 /** 071 * Financial Management (http://www.hl7.org/Special/committees/fm/index.cfm) 072 */ 073 FM, 074 /** 075 * Health Standards Integration (http://www.hl7.org/Special/committees/hsi/index.cfm) 076 */ 077 HSI, 078 /** 079 * Imaging Integration (http://www.hl7.org/Special/committees/imagemgt/index.cfm) 080 */ 081 II, 082 /** 083 * Infrastructure And Messaging (http://www.hl7.org/Special/committees/inm/index.cfm) 084 */ 085 INM, 086 /** 087 * Implementable Technology Specifications (http://www.hl7.org/Special/committees/xml/index.cfm) 088 */ 089 ITS, 090 /** 091 * Orders and Observations (http://www.hl7.org/Special/committees/orders/index.cfm) 092 */ 093 OO, 094 /** 095 * Patient Administration (http://www.hl7.org/Special/committees/pafm/index.cfm) 096 */ 097 PA, 098 /** 099 * Patient Care (http://www.hl7.org/Special/committees/patientcare/index.cfm) 100 */ 101 PC, 102 /** 103 * Public Health and Emergency Response (http://www.hl7.org/Special/committees/pher/index.cfm) 104 */ 105 PHER, 106 /** 107 * Pharmacy (http://www.hl7.org/Special/committees/medication/index.cfm) 108 */ 109 PHX, 110 /** 111 * Regulated Clinical Research Information Management (http://www.hl7.org/Special/committees/rcrim/index.cfm) 112 */ 113 RCRIM, 114 /** 115 * Structured Documents (http://www.hl7.org/Special/committees/structure/index.cfm) 116 */ 117 SD, 118 /** 119 * Security (http://www.hl7.org/Special/committees/secure/index.cfm) 120 */ 121 SEC, 122 /** 123 * US Realm Taskforce (http://wiki.hl7.org/index.php?title=US_Realm_Task_Force) 124 */ 125 US, 126 /** 127 * Vocabulary (http://www.hl7.org/Special/committees/Vocab/index.cfm) 128 */ 129 VOCAB, 130 /** 131 * Application Implementation and Design (http://www.hl7.org/Special/committees/java/index.cfm) 132 */ 133 AID, 134 /** 135 * added to help the parsers 136 */ 137 NULL; 138 public static Hl7WorkGroup fromCode(String codeString) throws FHIRException { 139 if (codeString == null || "".equals(codeString)) 140 return null; 141 if ("cbcc".equals(codeString)) 142 return CBCC; 143 if ("cds".equals(codeString)) 144 return CDS; 145 if ("cqi".equals(codeString)) 146 return CQI; 147 if ("cg".equals(codeString)) 148 return CG; 149 if ("dev".equals(codeString)) 150 return DEV; 151 if ("ehr".equals(codeString)) 152 return EHR; 153 if ("fhir".equals(codeString)) 154 return FHIR; 155 if ("fm".equals(codeString)) 156 return FM; 157 if ("hsi".equals(codeString)) 158 return HSI; 159 if ("ii".equals(codeString)) 160 return II; 161 if ("inm".equals(codeString)) 162 return INM; 163 if ("its".equals(codeString)) 164 return ITS; 165 if ("oo".equals(codeString)) 166 return OO; 167 if ("pa".equals(codeString)) 168 return PA; 169 if ("pc".equals(codeString)) 170 return PC; 171 if ("pher".equals(codeString)) 172 return PHER; 173 if ("phx".equals(codeString)) 174 return PHX; 175 if ("rcrim".equals(codeString)) 176 return RCRIM; 177 if ("sd".equals(codeString)) 178 return SD; 179 if ("sec".equals(codeString)) 180 return SEC; 181 if ("us".equals(codeString)) 182 return US; 183 if ("vocab".equals(codeString)) 184 return VOCAB; 185 if ("aid".equals(codeString)) 186 return AID; 187 throw new FHIRException("Unknown Hl7WorkGroup code '"+codeString+"'"); 188 } 189 public String toCode() { 190 switch (this) { 191 case CBCC: return "cbcc"; 192 case CDS: return "cds"; 193 case CQI: return "cqi"; 194 case CG: return "cg"; 195 case DEV: return "dev"; 196 case EHR: return "ehr"; 197 case FHIR: return "fhir"; 198 case FM: return "fm"; 199 case HSI: return "hsi"; 200 case II: return "ii"; 201 case INM: return "inm"; 202 case ITS: return "its"; 203 case OO: return "oo"; 204 case PA: return "pa"; 205 case PC: return "pc"; 206 case PHER: return "pher"; 207 case PHX: return "phx"; 208 case RCRIM: return "rcrim"; 209 case SD: return "sd"; 210 case SEC: return "sec"; 211 case US: return "us"; 212 case VOCAB: return "vocab"; 213 case AID: return "aid"; 214 case NULL: return null; 215 default: return "?"; 216 } 217 } 218 public String getSystem() { 219 return "http://hl7.org/fhir/hl7-work-group"; 220 } 221 public String getDefinition() { 222 switch (this) { 223 case CBCC: return "Community Based Collaborative Care (http://www.hl7.org/Special/committees/cbcc/index.cfm)"; 224 case CDS: return "Clinical Decision Support (http://www.hl7.org/Special/committees/dss/index.cfm)"; 225 case CQI: return "Clinical Quality Information (http://www.hl7.org/Special/committees/cqi/index.cfm)"; 226 case CG: return "Clinical Genomics (http://www.hl7.org/Special/committees/clingenomics/index.cfm)"; 227 case DEV: return "Health Care Devices (http://www.hl7.org/Special/committees/healthcaredevices/index.cfm)"; 228 case EHR: return "Electronic Health Records (http://www.hl7.org/Special/committees/ehr/index.cfm)"; 229 case FHIR: return "FHIR Infrastructure (http://www.hl7.org/Special/committees/fiwg/index.cfm)"; 230 case FM: return "Financial Management (http://www.hl7.org/Special/committees/fm/index.cfm)"; 231 case HSI: return "Health Standards Integration (http://www.hl7.org/Special/committees/hsi/index.cfm)"; 232 case II: return "Imaging Integration (http://www.hl7.org/Special/committees/imagemgt/index.cfm)"; 233 case INM: return "Infrastructure And Messaging (http://www.hl7.org/Special/committees/inm/index.cfm)"; 234 case ITS: return "Implementable Technology Specifications (http://www.hl7.org/Special/committees/xml/index.cfm)"; 235 case OO: return "Orders and Observations (http://www.hl7.org/Special/committees/orders/index.cfm)"; 236 case PA: return "Patient Administration (http://www.hl7.org/Special/committees/pafm/index.cfm)"; 237 case PC: return "Patient Care (http://www.hl7.org/Special/committees/patientcare/index.cfm)"; 238 case PHER: return "Public Health and Emergency Response (http://www.hl7.org/Special/committees/pher/index.cfm)"; 239 case PHX: return "Pharmacy (http://www.hl7.org/Special/committees/medication/index.cfm)"; 240 case RCRIM: return "Regulated Clinical Research Information Management (http://www.hl7.org/Special/committees/rcrim/index.cfm)"; 241 case SD: return "Structured Documents (http://www.hl7.org/Special/committees/structure/index.cfm)"; 242 case SEC: return "Security (http://www.hl7.org/Special/committees/secure/index.cfm)"; 243 case US: return "US Realm Taskforce (http://wiki.hl7.org/index.php?title=US_Realm_Task_Force)"; 244 case VOCAB: return "Vocabulary (http://www.hl7.org/Special/committees/Vocab/index.cfm)"; 245 case AID: return "Application Implementation and Design (http://www.hl7.org/Special/committees/java/index.cfm)"; 246 case NULL: return null; 247 default: return "?"; 248 } 249 } 250 public String getDisplay() { 251 switch (this) { 252 case CBCC: return "Community Based Collaborative Care"; 253 case CDS: return "Clinical Decision Support"; 254 case CQI: return "Clinical Quality Information"; 255 case CG: return "Clinical Genomics"; 256 case DEV: return "Health Care Devices"; 257 case EHR: return "Electronic Health Records"; 258 case FHIR: return "FHIR Infrastructure"; 259 case FM: return "Financial Management"; 260 case HSI: return "Health Standards Integration"; 261 case II: return "Imaging Integration"; 262 case INM: return "Infrastructure And Messaging"; 263 case ITS: return "Implementable Technology Specifications"; 264 case OO: return "Orders and Observations"; 265 case PA: return "Patient Administration"; 266 case PC: return "Patient Care"; 267 case PHER: return "Public Health and Emergency Response"; 268 case PHX: return "Pharmacy"; 269 case RCRIM: return "Regulated Clinical Research Information Management"; 270 case SD: return "Structured Documents"; 271 case SEC: return "Security"; 272 case US: return "US Realm Taskforce"; 273 case VOCAB: return "Vocabulary"; 274 case AID: return "Application Implementation and Design"; 275 case NULL: return null; 276 default: return "?"; 277 } 278 } 279 280 281}