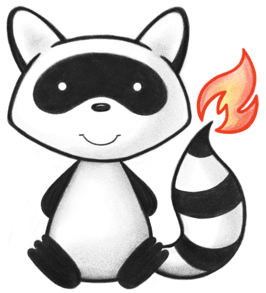
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum Iso21089Lifecycle { 041 042 /** 043 * occurs when an agent makes any change to record entry content currently residing in storage considered permanent (persistent) 044 */ 045 _2, 046 /** 047 * occurs when an agent causes the system to create and move archive artifacts containing record entry content, typically to long-term offline storage 048 */ 049 _14, 050 /** 051 * occurs when an agent causes the system to capture the agent??s digital signature (or equivalent indication) during formal validation of record entry content 052 */ 053 _4, 054 /** 055 * occurs when an agent causes the system to decode record entry content from a cipher 056 */ 057 _27, 058 /** 059 * occurs when an agent causes the system to scrub record entry content to reduce the association between a set of identifying data and the data subject in a way that may or may not be reversible 060 */ 061 _10, 062 /** 063 * occurs when an agent causes the system to tag record entry(ies) as obsolete, erroneous or untrustworthy, to warn against its future use 064 */ 065 _17, 066 /** 067 * occurs when an agent causes the system to permanently erase record entry content from the system 068 */ 069 _16, 070 /** 071 * occurs when an agent causes the system to release, transfer, provision access to, or otherwise divulge record entry content 072 */ 073 _7, 074 /** 075 * occurs when an agent causes the system to encode record entry content in a cipher 076 */ 077 _26, 078 /** 079 * occurs when an agent causes the system to selectively pull out a subset of record entry content, based on explicit criteria 080 */ 081 _13, 082 /** 083 * occurs when an agent causes the system to connect related record entries 084 */ 085 _21, 086 /** 087 * occurs when an agent causes the system to combine or join content from two or more record entries, resulting in a single logical record entry 088 */ 089 _19, 090 /** 091 * occurs when an agent causes the system to: a) initiate capture of potential record content, and b) incorporate that content into the storage considered a permanent part of the health record 092 */ 093 _1, 094 /** 095 * occurs when an agent causes the system to remove record entry content to reduce the association between a set of identifying data and the data subject in a way that may be reversible 096 */ 097 _11, 098 /** 099 * occurs when an agent causes the system to recreate or restore full status to record entries previously deleted or deprecated 100 */ 101 _18, 102 /** 103 * occurs when an agent causes the system to: a) initiate capture of data content from elseware, and b) incorporate that content into the storage considered a permanent part of the health record 104 */ 105 _9, 106 /** 107 * occurs when an agent causes the system to produce and deliver record entry content in a particular form and manner 108 */ 109 _6, 110 /** 111 * occurs when an agent causes the system to restore information to data that allows identification of information source and/or information subject 112 */ 113 _12, 114 /** 115 * occurs when an agent causes the system to remove a tag or other cues for special access management had required to fulfill organizational policy under the legal doctrine of ??duty to preserve? 116 */ 117 _24, 118 /** 119 * occurs when an agent causes the system to recreate record entries and their content from a previous created archive artifact 120 */ 121 _15, 122 /** 123 * occurs when an agent causes the system to change the form, language or code system used to represent record entry content 124 */ 125 _3, 126 /** 127 * occurs when an agent causes the system to send record entry content from one (EHR/PHR/other) system to another 128 */ 129 _8, 130 /** 131 * occurs when an agent causes the system to disconnect two or more record entries previously connected, rendering them separate (disconnected) again 132 */ 133 _22, 134 /** 135 * occurs when an agent causes the system to reverse a previous record entry merge operation, rendering them separate again 136 */ 137 _20, 138 /** 139 * occurs when an agent causes the system to confirm compliance of data or data objects with regulations, requirements, specifications, or other imposed conditions based on organizational policy 140 */ 141 _25, 142 /** 143 * added to help the parsers 144 */ 145 NULL; 146 public static Iso21089Lifecycle fromCode(String codeString) throws FHIRException { 147 if (codeString == null || "".equals(codeString)) 148 return null; 149 if ("2".equals(codeString)) 150 return _2; 151 if ("14".equals(codeString)) 152 return _14; 153 if ("4".equals(codeString)) 154 return _4; 155 if ("27".equals(codeString)) 156 return _27; 157 if ("10".equals(codeString)) 158 return _10; 159 if ("17".equals(codeString)) 160 return _17; 161 if ("16".equals(codeString)) 162 return _16; 163 if ("7".equals(codeString)) 164 return _7; 165 if ("26".equals(codeString)) 166 return _26; 167 if ("13".equals(codeString)) 168 return _13; 169 if ("21".equals(codeString)) 170 return _21; 171 if ("19".equals(codeString)) 172 return _19; 173 if ("1".equals(codeString)) 174 return _1; 175 if ("11".equals(codeString)) 176 return _11; 177 if ("18".equals(codeString)) 178 return _18; 179 if ("9".equals(codeString)) 180 return _9; 181 if ("6".equals(codeString)) 182 return _6; 183 if ("12".equals(codeString)) 184 return _12; 185 if ("24".equals(codeString)) 186 return _24; 187 if ("15".equals(codeString)) 188 return _15; 189 if ("3".equals(codeString)) 190 return _3; 191 if ("8".equals(codeString)) 192 return _8; 193 if ("22".equals(codeString)) 194 return _22; 195 if ("20".equals(codeString)) 196 return _20; 197 if ("25".equals(codeString)) 198 return _25; 199 throw new FHIRException("Unknown Iso21089Lifecycle code '"+codeString+"'"); 200 } 201 public String toCode() { 202 switch (this) { 203 case _2: return "2"; 204 case _14: return "14"; 205 case _4: return "4"; 206 case _27: return "27"; 207 case _10: return "10"; 208 case _17: return "17"; 209 case _16: return "16"; 210 case _7: return "7"; 211 case _26: return "26"; 212 case _13: return "13"; 213 case _21: return "21"; 214 case _19: return "19"; 215 case _1: return "1"; 216 case _11: return "11"; 217 case _18: return "18"; 218 case _9: return "9"; 219 case _6: return "6"; 220 case _12: return "12"; 221 case _24: return "24"; 222 case _15: return "15"; 223 case _3: return "3"; 224 case _8: return "8"; 225 case _22: return "22"; 226 case _20: return "20"; 227 case _25: return "25"; 228 case NULL: return null; 229 default: return "?"; 230 } 231 } 232 public String getSystem() { 233 return "http://hl7.org/fhir/iso-21089-lifecycle"; 234 } 235 public String getDefinition() { 236 switch (this) { 237 case _2: return "occurs when an agent makes any change to record entry content currently residing in storage considered permanent (persistent)"; 238 case _14: return "occurs when an agent causes the system to create and move archive artifacts containing record entry content, typically to long-term offline storage"; 239 case _4: return "occurs when an agent causes the system to capture the agent??s digital signature (or equivalent indication) during formal validation of record entry content"; 240 case _27: return "occurs when an agent causes the system to decode record entry content from a cipher"; 241 case _10: return "occurs when an agent causes the system to scrub record entry content to reduce the association between a set of identifying data and the data subject in a way that may or may not be reversible"; 242 case _17: return "occurs when an agent causes the system to tag record entry(ies) as obsolete, erroneous or untrustworthy, to warn against its future use"; 243 case _16: return "occurs when an agent causes the system to permanently erase record entry content from the system"; 244 case _7: return "occurs when an agent causes the system to release, transfer, provision access to, or otherwise divulge record entry content"; 245 case _26: return "occurs when an agent causes the system to encode record entry content in a cipher"; 246 case _13: return "occurs when an agent causes the system to selectively pull out a subset of record entry content, based on explicit criteria"; 247 case _21: return "occurs when an agent causes the system to connect related record entries"; 248 case _19: return "occurs when an agent causes the system to combine or join content from two or more record entries, resulting in a single logical record entry"; 249 case _1: return "occurs when an agent causes the system to: a) initiate capture of potential record content, and b) incorporate that content into the storage considered a permanent part of the health record"; 250 case _11: return "occurs when an agent causes the system to remove record entry content to reduce the association between a set of identifying data and the data subject in a way that may be reversible"; 251 case _18: return "occurs when an agent causes the system to recreate or restore full status to record entries previously deleted or deprecated"; 252 case _9: return "occurs when an agent causes the system to: a) initiate capture of data content from elseware, and b) incorporate that content into the storage considered a permanent part of the health record"; 253 case _6: return "occurs when an agent causes the system to produce and deliver record entry content in a particular form and manner"; 254 case _12: return "occurs when an agent causes the system to restore information to data that allows identification of information source and/or information subject"; 255 case _24: return "occurs when an agent causes the system to remove a tag or other cues for special access management had required to fulfill organizational policy under the legal doctrine of ??duty to preserve?"; 256 case _15: return "occurs when an agent causes the system to recreate record entries and their content from a previous created archive artifact"; 257 case _3: return "occurs when an agent causes the system to change the form, language or code system used to represent record entry content"; 258 case _8: return "occurs when an agent causes the system to send record entry content from one (EHR/PHR/other) system to another"; 259 case _22: return "occurs when an agent causes the system to disconnect two or more record entries previously connected, rendering them separate (disconnected) again"; 260 case _20: return "occurs when an agent causes the system to reverse a previous record entry merge operation, rendering them separate again"; 261 case _25: return "occurs when an agent causes the system to confirm compliance of data or data objects with regulations, requirements, specifications, or other imposed conditions based on organizational policy"; 262 case NULL: return null; 263 default: return "?"; 264 } 265 } 266 public String getDisplay() { 267 switch (this) { 268 case _2: return "Amend (Update) - Lifeycle Event"; 269 case _14: return "Archive - Lifeycle Event"; 270 case _4: return "Attest - Lifecycle Event"; 271 case _27: return "Decrypt - Lifecycle Event"; 272 case _10: return "De-Identify (Anononymize) - Lifecycle Event"; 273 case _17: return "Deprecate - Lifecycle Event"; 274 case _16: return "Destroy/Delete - Lifecycle Event"; 275 case _7: return "Disclose - Lifecycle Event"; 276 case _26: return "Encrypt - Lifecycle Event"; 277 case _13: return "Extract - Lifecycle Event"; 278 case _21: return "Link - Lifecycle Event"; 279 case _19: return "Merge - Lifecycle Event"; 280 case _1: return "Originate/Retain - Record Lifecyle Event"; 281 case _11: return "Pseudonymize - Lifecycle Event"; 282 case _18: return "Re-activate - Lifecycle Event"; 283 case _9: return "Receive/Retain - Lifecycle Event"; 284 case _6: return "Report (Output) - Lifecycle Event"; 285 case _12: return "Re-identify - Lifecycle Event"; 286 case _24: return "Remove Legal Hold - Lifecycle Event"; 287 case _15: return "Restore - Lifecycle Event"; 288 case _3: return "Transform/Translate - Lifecycle Event"; 289 case _8: return "Transmit - Lifecycle Event"; 290 case _22: return "Unlink - Lifecycle Event"; 291 case _20: return "Unmerge - Lifecycle Event"; 292 case _25: return "Verify - Lifecycle Event"; 293 case NULL: return null; 294 default: return "?"; 295 } 296 } 297 298 299}