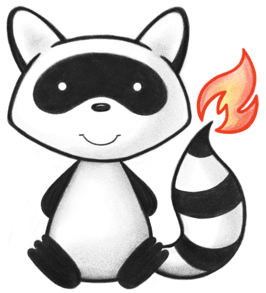
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum IssueType { 041 042 /** 043 * Content invalid against the specification or a profile. 044 */ 045 INVALID, 046 /** 047 * A structural issue in the content such as wrong namespace, or unable to parse the content completely, or invalid json syntax. 048 */ 049 STRUCTURE, 050 /** 051 * A required element is missing. 052 */ 053 REQUIRED, 054 /** 055 * An element value is invalid. 056 */ 057 VALUE, 058 /** 059 * A content validation rule failed - e.g. a schematron rule. 060 */ 061 INVARIANT, 062 /** 063 * An authentication/authorization/permissions issue of some kind. 064 */ 065 SECURITY, 066 /** 067 * The client needs to initiate an authentication process. 068 */ 069 LOGIN, 070 /** 071 * The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable). 072 */ 073 UNKNOWN, 074 /** 075 * User session expired; a login may be required. 076 */ 077 EXPIRED, 078 /** 079 * The user does not have the rights to perform this action. 080 */ 081 FORBIDDEN, 082 /** 083 * Some information was not or may not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes. 084 */ 085 SUPPRESSED, 086 /** 087 * Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged. 088 */ 089 PROCESSING, 090 /** 091 * The resource or profile is not supported. 092 */ 093 NOTSUPPORTED, 094 /** 095 * An attempt was made to create a duplicate record. 096 */ 097 DUPLICATE, 098 /** 099 * The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture. 100 */ 101 NOTFOUND, 102 /** 103 * Provided content is too long (typically, this is a denial of service protection type of error). 104 */ 105 TOOLONG, 106 /** 107 * The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code. 108 */ 109 CODEINVALID, 110 /** 111 * An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized. 112 */ 113 EXTENSION, 114 /** 115 * The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT. 116 */ 117 TOOCOSTLY, 118 /** 119 * The content/operation failed to pass some business rule, and so could not proceed. 120 */ 121 BUSINESSRULE, 122 /** 123 * Content could not be accepted because of an edit conflict (i.e. version aware updates) (In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the conflict is discovered further into the application architecture.) 124 */ 125 CONFLICT, 126 /** 127 * Not all data sources typically accessed could be reached, or responded in time, so the returned information may not be complete. 128 */ 129 INCOMPLETE, 130 /** 131 * Transient processing issues. The system receiving the error may be able to resubmit the same content once an underlying issue is resolved. 132 */ 133 TRANSIENT, 134 /** 135 * A resource/record locking failure (usually in an underlying database). 136 */ 137 LOCKERROR, 138 /** 139 * The persistent store is unavailable; e.g. the database is down for maintenance or similar action. 140 */ 141 NOSTORE, 142 /** 143 * An unexpected internal error has occurred. 144 */ 145 EXCEPTION, 146 /** 147 * An internal timeout has occurred. 148 */ 149 TIMEOUT, 150 /** 151 * The system is not prepared to handle this request due to load management. 152 */ 153 THROTTLED, 154 /** 155 * A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.). 156 */ 157 INFORMATIONAL, 158 /** 159 * added to help the parsers 160 */ 161 NULL; 162 public static IssueType fromCode(String codeString) throws FHIRException { 163 if (codeString == null || "".equals(codeString)) 164 return null; 165 if ("invalid".equals(codeString)) 166 return INVALID; 167 if ("structure".equals(codeString)) 168 return STRUCTURE; 169 if ("required".equals(codeString)) 170 return REQUIRED; 171 if ("value".equals(codeString)) 172 return VALUE; 173 if ("invariant".equals(codeString)) 174 return INVARIANT; 175 if ("security".equals(codeString)) 176 return SECURITY; 177 if ("login".equals(codeString)) 178 return LOGIN; 179 if ("unknown".equals(codeString)) 180 return UNKNOWN; 181 if ("expired".equals(codeString)) 182 return EXPIRED; 183 if ("forbidden".equals(codeString)) 184 return FORBIDDEN; 185 if ("suppressed".equals(codeString)) 186 return SUPPRESSED; 187 if ("processing".equals(codeString)) 188 return PROCESSING; 189 if ("not-supported".equals(codeString)) 190 return NOTSUPPORTED; 191 if ("duplicate".equals(codeString)) 192 return DUPLICATE; 193 if ("not-found".equals(codeString)) 194 return NOTFOUND; 195 if ("too-long".equals(codeString)) 196 return TOOLONG; 197 if ("code-invalid".equals(codeString)) 198 return CODEINVALID; 199 if ("extension".equals(codeString)) 200 return EXTENSION; 201 if ("too-costly".equals(codeString)) 202 return TOOCOSTLY; 203 if ("business-rule".equals(codeString)) 204 return BUSINESSRULE; 205 if ("conflict".equals(codeString)) 206 return CONFLICT; 207 if ("incomplete".equals(codeString)) 208 return INCOMPLETE; 209 if ("transient".equals(codeString)) 210 return TRANSIENT; 211 if ("lock-error".equals(codeString)) 212 return LOCKERROR; 213 if ("no-store".equals(codeString)) 214 return NOSTORE; 215 if ("exception".equals(codeString)) 216 return EXCEPTION; 217 if ("timeout".equals(codeString)) 218 return TIMEOUT; 219 if ("throttled".equals(codeString)) 220 return THROTTLED; 221 if ("informational".equals(codeString)) 222 return INFORMATIONAL; 223 throw new FHIRException("Unknown IssueType code '"+codeString+"'"); 224 } 225 public String toCode() { 226 switch (this) { 227 case INVALID: return "invalid"; 228 case STRUCTURE: return "structure"; 229 case REQUIRED: return "required"; 230 case VALUE: return "value"; 231 case INVARIANT: return "invariant"; 232 case SECURITY: return "security"; 233 case LOGIN: return "login"; 234 case UNKNOWN: return "unknown"; 235 case EXPIRED: return "expired"; 236 case FORBIDDEN: return "forbidden"; 237 case SUPPRESSED: return "suppressed"; 238 case PROCESSING: return "processing"; 239 case NOTSUPPORTED: return "not-supported"; 240 case DUPLICATE: return "duplicate"; 241 case NOTFOUND: return "not-found"; 242 case TOOLONG: return "too-long"; 243 case CODEINVALID: return "code-invalid"; 244 case EXTENSION: return "extension"; 245 case TOOCOSTLY: return "too-costly"; 246 case BUSINESSRULE: return "business-rule"; 247 case CONFLICT: return "conflict"; 248 case INCOMPLETE: return "incomplete"; 249 case TRANSIENT: return "transient"; 250 case LOCKERROR: return "lock-error"; 251 case NOSTORE: return "no-store"; 252 case EXCEPTION: return "exception"; 253 case TIMEOUT: return "timeout"; 254 case THROTTLED: return "throttled"; 255 case INFORMATIONAL: return "informational"; 256 case NULL: return null; 257 default: return "?"; 258 } 259 } 260 public String getSystem() { 261 return "http://hl7.org/fhir/issue-type"; 262 } 263 public String getDefinition() { 264 switch (this) { 265 case INVALID: return "Content invalid against the specification or a profile."; 266 case STRUCTURE: return "A structural issue in the content such as wrong namespace, or unable to parse the content completely, or invalid json syntax."; 267 case REQUIRED: return "A required element is missing."; 268 case VALUE: return "An element value is invalid."; 269 case INVARIANT: return "A content validation rule failed - e.g. a schematron rule."; 270 case SECURITY: return "An authentication/authorization/permissions issue of some kind."; 271 case LOGIN: return "The client needs to initiate an authentication process."; 272 case UNKNOWN: return "The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable)."; 273 case EXPIRED: return "User session expired; a login may be required."; 274 case FORBIDDEN: return "The user does not have the rights to perform this action."; 275 case SUPPRESSED: return "Some information was not or may not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes."; 276 case PROCESSING: return "Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged."; 277 case NOTSUPPORTED: return "The resource or profile is not supported."; 278 case DUPLICATE: return "An attempt was made to create a duplicate record."; 279 case NOTFOUND: return "The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture."; 280 case TOOLONG: return "Provided content is too long (typically, this is a denial of service protection type of error)."; 281 case CODEINVALID: return "The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code."; 282 case EXTENSION: return "An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized."; 283 case TOOCOSTLY: return "The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT."; 284 case BUSINESSRULE: return "The content/operation failed to pass some business rule, and so could not proceed."; 285 case CONFLICT: return "Content could not be accepted because of an edit conflict (i.e. version aware updates) (In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the conflict is discovered further into the application architecture.)"; 286 case INCOMPLETE: return "Not all data sources typically accessed could be reached, or responded in time, so the returned information may not be complete."; 287 case TRANSIENT: return "Transient processing issues. The system receiving the error may be able to resubmit the same content once an underlying issue is resolved."; 288 case LOCKERROR: return "A resource/record locking failure (usually in an underlying database)."; 289 case NOSTORE: return "The persistent store is unavailable; e.g. the database is down for maintenance or similar action."; 290 case EXCEPTION: return "An unexpected internal error has occurred."; 291 case TIMEOUT: return "An internal timeout has occurred."; 292 case THROTTLED: return "The system is not prepared to handle this request due to load management."; 293 case INFORMATIONAL: return "A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.)."; 294 case NULL: return null; 295 default: return "?"; 296 } 297 } 298 public String getDisplay() { 299 switch (this) { 300 case INVALID: return "Invalid Content"; 301 case STRUCTURE: return "Structural Issue"; 302 case REQUIRED: return "Required element missing"; 303 case VALUE: return "Element value invalid"; 304 case INVARIANT: return "Validation rule failed"; 305 case SECURITY: return "Security Problem"; 306 case LOGIN: return "Login Required"; 307 case UNKNOWN: return "Unknown User"; 308 case EXPIRED: return "Session Expired"; 309 case FORBIDDEN: return "Forbidden"; 310 case SUPPRESSED: return "Information Suppressed"; 311 case PROCESSING: return "Processing Failure"; 312 case NOTSUPPORTED: return "Content not supported"; 313 case DUPLICATE: return "Duplicate"; 314 case NOTFOUND: return "Not Found"; 315 case TOOLONG: return "Content Too Long"; 316 case CODEINVALID: return "Invalid Code"; 317 case EXTENSION: return "Unacceptable Extension"; 318 case TOOCOSTLY: return "Operation Too Costly"; 319 case BUSINESSRULE: return "Business Rule Violation"; 320 case CONFLICT: return "Edit Version Conflict"; 321 case INCOMPLETE: return "Incomplete Results"; 322 case TRANSIENT: return "Transient Issue"; 323 case LOCKERROR: return "Lock Error"; 324 case NOSTORE: return "No Store Available"; 325 case EXCEPTION: return "Exception"; 326 case TIMEOUT: return "Timeout"; 327 case THROTTLED: return "Throttled"; 328 case INFORMATIONAL: return "Informational Note"; 329 case NULL: return null; 330 default: return "?"; 331 } 332 } 333 334 335}