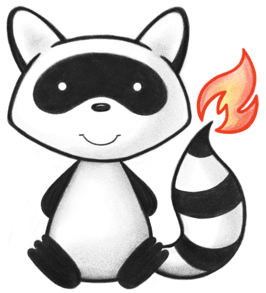
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ItemType { 041 042 /** 043 * An item with no direct answer but should have at least one child item. 044 */ 045 GROUP, 046 /** 047 * Text for display that will not capture an answer or have child items. 048 */ 049 DISPLAY, 050 /** 051 * An item that defines a specific answer to be captured, and may have child items. 052(the answer provided in the QuestionnaireResponse should be of the defined datatype) 053 */ 054 QUESTION, 055 /** 056 * Question with a yes/no answer (valueBoolean) 057 */ 058 BOOLEAN, 059 /** 060 * Question with is a real number answer (valueDecimal) 061 */ 062 DECIMAL, 063 /** 064 * Question with an integer answer (valueInteger) 065 */ 066 INTEGER, 067 /** 068 * Question with a date answer (valueDate) 069 */ 070 DATE, 071 /** 072 * Question with a date and time answer (valueDateTime) 073 */ 074 DATETIME, 075 /** 076 * Question with a time (hour:minute:second) answer independent of date. (valueTime) 077 */ 078 TIME, 079 /** 080 * Question with a short (few words to short sentence) free-text entry answer (valueString) 081 */ 082 STRING, 083 /** 084 * Question with a long (potentially multi-paragraph) free-text entry answer (valueString) 085 */ 086 TEXT, 087 /** 088 * Question with a URL (website, FTP site, etc.) answer (valueUri) 089 */ 090 URL, 091 /** 092 * Question with a Coding drawn from a list of options (specified in either the option property, or via the valueset referenced in the options property) as an answer (valueCoding) 093 */ 094 CHOICE, 095 /** 096 * Answer is a Coding drawn from a list of options (as with the choice type) or a free-text entry in a string (valueCoding or valueString) 097 */ 098 OPENCHOICE, 099 /** 100 * Question with binary content such as a image, PDF, etc. as an answer (valueAttachment) 101 */ 102 ATTACHMENT, 103 /** 104 * Question with a reference to another resource (practitioner, organization, etc.) as an answer (valueReference) 105 */ 106 REFERENCE, 107 /** 108 * Question with a combination of a numeric value and unit, potentially with a comparator (<, >, etc.) as an answer. (valueQuantity) 109There is an extension 'http://hl7.org/fhir/StructureDefinition/questionnaire-unit' that can be used to define what unit whould be captured (or the a unit that has a ucum conversion from the provided unit) 110 */ 111 QUANTITY, 112 /** 113 * added to help the parsers 114 */ 115 NULL; 116 public static ItemType fromCode(String codeString) throws FHIRException { 117 if (codeString == null || "".equals(codeString)) 118 return null; 119 if ("group".equals(codeString)) 120 return GROUP; 121 if ("display".equals(codeString)) 122 return DISPLAY; 123 if ("question".equals(codeString)) 124 return QUESTION; 125 if ("boolean".equals(codeString)) 126 return BOOLEAN; 127 if ("decimal".equals(codeString)) 128 return DECIMAL; 129 if ("integer".equals(codeString)) 130 return INTEGER; 131 if ("date".equals(codeString)) 132 return DATE; 133 if ("dateTime".equals(codeString)) 134 return DATETIME; 135 if ("time".equals(codeString)) 136 return TIME; 137 if ("string".equals(codeString)) 138 return STRING; 139 if ("text".equals(codeString)) 140 return TEXT; 141 if ("url".equals(codeString)) 142 return URL; 143 if ("choice".equals(codeString)) 144 return CHOICE; 145 if ("open-choice".equals(codeString)) 146 return OPENCHOICE; 147 if ("attachment".equals(codeString)) 148 return ATTACHMENT; 149 if ("reference".equals(codeString)) 150 return REFERENCE; 151 if ("quantity".equals(codeString)) 152 return QUANTITY; 153 throw new FHIRException("Unknown ItemType code '"+codeString+"'"); 154 } 155 public String toCode() { 156 switch (this) { 157 case GROUP: return "group"; 158 case DISPLAY: return "display"; 159 case QUESTION: return "question"; 160 case BOOLEAN: return "boolean"; 161 case DECIMAL: return "decimal"; 162 case INTEGER: return "integer"; 163 case DATE: return "date"; 164 case DATETIME: return "dateTime"; 165 case TIME: return "time"; 166 case STRING: return "string"; 167 case TEXT: return "text"; 168 case URL: return "url"; 169 case CHOICE: return "choice"; 170 case OPENCHOICE: return "open-choice"; 171 case ATTACHMENT: return "attachment"; 172 case REFERENCE: return "reference"; 173 case QUANTITY: return "quantity"; 174 case NULL: return null; 175 default: return "?"; 176 } 177 } 178 public String getSystem() { 179 return "http://hl7.org/fhir/item-type"; 180 } 181 public String getDefinition() { 182 switch (this) { 183 case GROUP: return "An item with no direct answer but should have at least one child item."; 184 case DISPLAY: return "Text for display that will not capture an answer or have child items."; 185 case QUESTION: return "An item that defines a specific answer to be captured, and may have child items.\n(the answer provided in the QuestionnaireResponse should be of the defined datatype)"; 186 case BOOLEAN: return "Question with a yes/no answer (valueBoolean)"; 187 case DECIMAL: return "Question with is a real number answer (valueDecimal)"; 188 case INTEGER: return "Question with an integer answer (valueInteger)"; 189 case DATE: return "Question with a date answer (valueDate)"; 190 case DATETIME: return "Question with a date and time answer (valueDateTime)"; 191 case TIME: return "Question with a time (hour:minute:second) answer independent of date. (valueTime)"; 192 case STRING: return "Question with a short (few words to short sentence) free-text entry answer (valueString)"; 193 case TEXT: return "Question with a long (potentially multi-paragraph) free-text entry answer (valueString)"; 194 case URL: return "Question with a URL (website, FTP site, etc.) answer (valueUri)"; 195 case CHOICE: return "Question with a Coding drawn from a list of options (specified in either the option property, or via the valueset referenced in the options property) as an answer (valueCoding)"; 196 case OPENCHOICE: return "Answer is a Coding drawn from a list of options (as with the choice type) or a free-text entry in a string (valueCoding or valueString)"; 197 case ATTACHMENT: return "Question with binary content such as a image, PDF, etc. as an answer (valueAttachment)"; 198 case REFERENCE: return "Question with a reference to another resource (practitioner, organization, etc.) as an answer (valueReference)"; 199 case QUANTITY: return "Question with a combination of a numeric value and unit, potentially with a comparator (<, >, etc.) as an answer. (valueQuantity)\nThere is an extension 'http://hl7.org/fhir/StructureDefinition/questionnaire-unit' that can be used to define what unit whould be captured (or the a unit that has a ucum conversion from the provided unit)"; 200 case NULL: return null; 201 default: return "?"; 202 } 203 } 204 public String getDisplay() { 205 switch (this) { 206 case GROUP: return "Group"; 207 case DISPLAY: return "Display"; 208 case QUESTION: return "Question"; 209 case BOOLEAN: return "Boolean"; 210 case DECIMAL: return "Decimal"; 211 case INTEGER: return "Integer"; 212 case DATE: return "Date"; 213 case DATETIME: return "Date Time"; 214 case TIME: return "Time"; 215 case STRING: return "String"; 216 case TEXT: return "Text"; 217 case URL: return "Url"; 218 case CHOICE: return "Choice"; 219 case OPENCHOICE: return "Open Choice"; 220 case ATTACHMENT: return "Attachment"; 221 case REFERENCE: return "Reference"; 222 case QUANTITY: return "Quantity"; 223 case NULL: return null; 224 default: return "?"; 225 } 226 } 227 228 229}