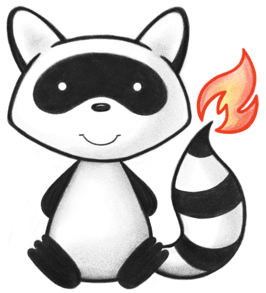
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum LocationPhysicalType { 041 042 /** 043 * A collection of buildings or other locations such as a site or a campus. 044 */ 045 SI, 046 /** 047 * Any Building or structure. This may contain rooms, corridors, wings, etc. It may not have walls, or a roof, but is considered a defined/allocated space. 048 */ 049 BU, 050 /** 051 * A Wing within a Building, this often contains levels, rooms and corridors. 052 */ 053 WI, 054 /** 055 * A Ward is a section of a medical facility that may contain rooms and other types of location. 056 */ 057 WA, 058 /** 059 * A Level in a multi-level Building/Structure. 060 */ 061 LVL, 062 /** 063 * Any corridor within a Building, that may connect rooms. 064 */ 065 CO, 066 /** 067 * A space that is allocated as a room, it may have walls/roof etc., but does not require these. 068 */ 069 RO, 070 /** 071 * A space that is allocated for sleeping/laying on. This is not the physical bed/trolley that may be moved about, but the space it may occupy. 072 */ 073 BD, 074 /** 075 * A means of transportation. 076 */ 077 VE, 078 /** 079 * A residential dwelling. Usually used to reference a location that a person/patient may reside. 080 */ 081 HO, 082 /** 083 * A container that can store goods, equipment, medications or other items. 084 */ 085 CA, 086 /** 087 * A defined path to travel between 2 points that has a known name. 088 */ 089 RD, 090 /** 091 * A defined physical boundary of something, such as a flood risk zone, region, postcode 092 */ 093 AREA, 094 /** 095 * A wide scope that covers a conceptual domain, such as a Nation (Country wide community or Federal Government - e.g. Ministry of Health), Province or State (community or Government), Business (throughout the enterprise), Nation with a business scope of an agency (e.g. CDC, FDA etc.) or a Business segment (UK Pharmacy), not just an physical boundry 096 */ 097 JDN, 098 /** 099 * added to help the parsers 100 */ 101 NULL; 102 public static LocationPhysicalType fromCode(String codeString) throws FHIRException { 103 if (codeString == null || "".equals(codeString)) 104 return null; 105 if ("si".equals(codeString)) 106 return SI; 107 if ("bu".equals(codeString)) 108 return BU; 109 if ("wi".equals(codeString)) 110 return WI; 111 if ("wa".equals(codeString)) 112 return WA; 113 if ("lvl".equals(codeString)) 114 return LVL; 115 if ("co".equals(codeString)) 116 return CO; 117 if ("ro".equals(codeString)) 118 return RO; 119 if ("bd".equals(codeString)) 120 return BD; 121 if ("ve".equals(codeString)) 122 return VE; 123 if ("ho".equals(codeString)) 124 return HO; 125 if ("ca".equals(codeString)) 126 return CA; 127 if ("rd".equals(codeString)) 128 return RD; 129 if ("area".equals(codeString)) 130 return AREA; 131 if ("jdn".equals(codeString)) 132 return JDN; 133 throw new FHIRException("Unknown LocationPhysicalType code '"+codeString+"'"); 134 } 135 public String toCode() { 136 switch (this) { 137 case SI: return "si"; 138 case BU: return "bu"; 139 case WI: return "wi"; 140 case WA: return "wa"; 141 case LVL: return "lvl"; 142 case CO: return "co"; 143 case RO: return "ro"; 144 case BD: return "bd"; 145 case VE: return "ve"; 146 case HO: return "ho"; 147 case CA: return "ca"; 148 case RD: return "rd"; 149 case AREA: return "area"; 150 case JDN: return "jdn"; 151 case NULL: return null; 152 default: return "?"; 153 } 154 } 155 public String getSystem() { 156 return "http://hl7.org/fhir/location-physical-type"; 157 } 158 public String getDefinition() { 159 switch (this) { 160 case SI: return "A collection of buildings or other locations such as a site or a campus."; 161 case BU: return "Any Building or structure. This may contain rooms, corridors, wings, etc. It may not have walls, or a roof, but is considered a defined/allocated space."; 162 case WI: return "A Wing within a Building, this often contains levels, rooms and corridors."; 163 case WA: return "A Ward is a section of a medical facility that may contain rooms and other types of location."; 164 case LVL: return "A Level in a multi-level Building/Structure."; 165 case CO: return "Any corridor within a Building, that may connect rooms."; 166 case RO: return "A space that is allocated as a room, it may have walls/roof etc., but does not require these."; 167 case BD: return "A space that is allocated for sleeping/laying on. This is not the physical bed/trolley that may be moved about, but the space it may occupy."; 168 case VE: return "A means of transportation."; 169 case HO: return "A residential dwelling. Usually used to reference a location that a person/patient may reside."; 170 case CA: return "A container that can store goods, equipment, medications or other items."; 171 case RD: return "A defined path to travel between 2 points that has a known name."; 172 case AREA: return "A defined physical boundary of something, such as a flood risk zone, region, postcode"; 173 case JDN: return "A wide scope that covers a conceptual domain, such as a Nation (Country wide community or Federal Government - e.g. Ministry of Health), Province or State (community or Government), Business (throughout the enterprise), Nation with a business scope of an agency (e.g. CDC, FDA etc.) or a Business segment (UK Pharmacy), not just an physical boundry"; 174 case NULL: return null; 175 default: return "?"; 176 } 177 } 178 public String getDisplay() { 179 switch (this) { 180 case SI: return "Site"; 181 case BU: return "Building"; 182 case WI: return "Wing"; 183 case WA: return "Ward"; 184 case LVL: return "Level"; 185 case CO: return "Corridor"; 186 case RO: return "Room"; 187 case BD: return "Bed"; 188 case VE: return "Vehicle"; 189 case HO: return "House"; 190 case CA: return "Cabinet"; 191 case RD: return "Road"; 192 case AREA: return "Area"; 193 case JDN: return "Jurisdiction"; 194 case NULL: return null; 195 default: return "?"; 196 } 197 } 198 199 200}