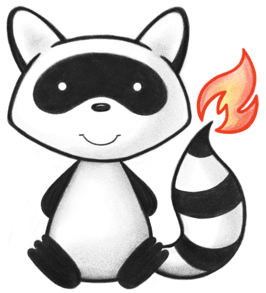
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum MapTransform { 041 042 /** 043 * create(type : string) - type is passed through to the application on the standard API, and must be known by it 044 */ 045 CREATE, 046 /** 047 * copy(source) 048 */ 049 COPY, 050 /** 051 * truncate(source, length) - source must be stringy type 052 */ 053 TRUNCATE, 054 /** 055 * escape(source, fmt1, fmt2) - change source from one kind of escaping to another (plain, java, xml, json). note that this is for when the string itself is escaped 056 */ 057 ESCAPE, 058 /** 059 * cast(source, type?) - case source from one type to another. target type can be left as implicit if there is one and only one target type known 060 */ 061 CAST, 062 /** 063 * append(source...) - source is element or string 064 */ 065 APPEND, 066 /** 067 * translate(source, uri_of_map) - use the translate operation 068 */ 069 TRANSLATE, 070 /** 071 * reference(source : object) - return a string that references the provided tree properly 072 */ 073 REFERENCE, 074 /** 075 * Perform a date operation. *Parameters to be documented* 076 */ 077 DATEOP, 078 /** 079 * Generate a random UUID (in lowercase). No Parameters 080 */ 081 UUID, 082 /** 083 * Return the appropriate string to put in a reference that refers to the resource provided as a parameter 084 */ 085 POINTER, 086 /** 087 * Execute the supplied fluentpath expression and use the value returned by that 088 */ 089 EVALUATE, 090 /** 091 * Create a CodeableConcept. Parameters = (text) or (system. Code[, display]) 092 */ 093 CC, 094 /** 095 * Create a Coding. Parameters = (system. Code[, display]) 096 */ 097 C, 098 /** 099 * Create a quantity. Parameters = (text) or (value, unit, [system, code]) where text is the natural representation e.g. [comparator]value[space]unit 100 */ 101 QTY, 102 /** 103 * Create an identifier. Parameters = (system, value[, type]) where type is a code from the identifier type value set 104 */ 105 ID, 106 /** 107 * Create a contact details. Parameters = (value) or (system, value). If no system is provided, the system should be inferred from the content of the value 108 */ 109 CP, 110 /** 111 * added to help the parsers 112 */ 113 NULL; 114 public static MapTransform fromCode(String codeString) throws FHIRException { 115 if (codeString == null || "".equals(codeString)) 116 return null; 117 if ("create".equals(codeString)) 118 return CREATE; 119 if ("copy".equals(codeString)) 120 return COPY; 121 if ("truncate".equals(codeString)) 122 return TRUNCATE; 123 if ("escape".equals(codeString)) 124 return ESCAPE; 125 if ("cast".equals(codeString)) 126 return CAST; 127 if ("append".equals(codeString)) 128 return APPEND; 129 if ("translate".equals(codeString)) 130 return TRANSLATE; 131 if ("reference".equals(codeString)) 132 return REFERENCE; 133 if ("dateOp".equals(codeString)) 134 return DATEOP; 135 if ("uuid".equals(codeString)) 136 return UUID; 137 if ("pointer".equals(codeString)) 138 return POINTER; 139 if ("evaluate".equals(codeString)) 140 return EVALUATE; 141 if ("cc".equals(codeString)) 142 return CC; 143 if ("c".equals(codeString)) 144 return C; 145 if ("qty".equals(codeString)) 146 return QTY; 147 if ("id".equals(codeString)) 148 return ID; 149 if ("cp".equals(codeString)) 150 return CP; 151 throw new FHIRException("Unknown MapTransform code '"+codeString+"'"); 152 } 153 public String toCode() { 154 switch (this) { 155 case CREATE: return "create"; 156 case COPY: return "copy"; 157 case TRUNCATE: return "truncate"; 158 case ESCAPE: return "escape"; 159 case CAST: return "cast"; 160 case APPEND: return "append"; 161 case TRANSLATE: return "translate"; 162 case REFERENCE: return "reference"; 163 case DATEOP: return "dateOp"; 164 case UUID: return "uuid"; 165 case POINTER: return "pointer"; 166 case EVALUATE: return "evaluate"; 167 case CC: return "cc"; 168 case C: return "c"; 169 case QTY: return "qty"; 170 case ID: return "id"; 171 case CP: return "cp"; 172 case NULL: return null; 173 default: return "?"; 174 } 175 } 176 public String getSystem() { 177 return "http://hl7.org/fhir/map-transform"; 178 } 179 public String getDefinition() { 180 switch (this) { 181 case CREATE: return "create(type : string) - type is passed through to the application on the standard API, and must be known by it"; 182 case COPY: return "copy(source)"; 183 case TRUNCATE: return "truncate(source, length) - source must be stringy type"; 184 case ESCAPE: return "escape(source, fmt1, fmt2) - change source from one kind of escaping to another (plain, java, xml, json). note that this is for when the string itself is escaped"; 185 case CAST: return "cast(source, type?) - case source from one type to another. target type can be left as implicit if there is one and only one target type known"; 186 case APPEND: return "append(source...) - source is element or string"; 187 case TRANSLATE: return "translate(source, uri_of_map) - use the translate operation"; 188 case REFERENCE: return "reference(source : object) - return a string that references the provided tree properly"; 189 case DATEOP: return "Perform a date operation. *Parameters to be documented*"; 190 case UUID: return "Generate a random UUID (in lowercase). No Parameters"; 191 case POINTER: return "Return the appropriate string to put in a reference that refers to the resource provided as a parameter"; 192 case EVALUATE: return "Execute the supplied fluentpath expression and use the value returned by that"; 193 case CC: return "Create a CodeableConcept. Parameters = (text) or (system. Code[, display])"; 194 case C: return "Create a Coding. Parameters = (system. Code[, display])"; 195 case QTY: return "Create a quantity. Parameters = (text) or (value, unit, [system, code]) where text is the natural representation e.g. [comparator]value[space]unit"; 196 case ID: return "Create an identifier. Parameters = (system, value[, type]) where type is a code from the identifier type value set"; 197 case CP: return "Create a contact details. Parameters = (value) or (system, value). If no system is provided, the system should be inferred from the content of the value"; 198 case NULL: return null; 199 default: return "?"; 200 } 201 } 202 public String getDisplay() { 203 switch (this) { 204 case CREATE: return "create"; 205 case COPY: return "copy"; 206 case TRUNCATE: return "truncate"; 207 case ESCAPE: return "escape"; 208 case CAST: return "cast"; 209 case APPEND: return "append"; 210 case TRANSLATE: return "translate"; 211 case REFERENCE: return "reference"; 212 case DATEOP: return "dateOp"; 213 case UUID: return "uuid"; 214 case POINTER: return "pointer"; 215 case EVALUATE: return "evaluate"; 216 case CC: return "cc"; 217 case C: return "c"; 218 case QTY: return "qty"; 219 case ID: return "id"; 220 case CP: return "cp"; 221 case NULL: return null; 222 default: return "?"; 223 } 224 } 225 226 227}